STM32L476G_EVAL BSP User Manual
|
stm32l476g_eval_audio.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_eval_audio.h 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32l476g_eval_audio.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32L476G_EVAL_AUDIO_H 00041 #define __STM32L476G_EVAL_AUDIO_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 /* Include audio component Driver */ 00049 #include "../Components/wm8994/wm8994.h" 00050 #include "stm32l476g_eval.h" 00051 00052 /** @addtogroup BSP 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM32L476G_EVAL 00057 * @{ 00058 */ 00059 00060 /** @addtogroup STM32L476G_EVAL_AUDIO 00061 * @{ 00062 */ 00063 00064 /** @defgroup STM32L476G_EVAL_AUDIO_Exported_Types Exported Types 00065 * @{ 00066 */ 00067 00068 /** 00069 * @} 00070 */ 00071 00072 /** @defgroup STM32L476G_EVAL_AUDIO_Exported_Constants Exported Constants 00073 * @{ 00074 */ 00075 00076 /** @defgroup BSP_Audio_Out_Option BSP Audio Out Option 00077 * @{ 00078 */ 00079 #define BSP_AUDIO_OUT_CIRCULARMODE ((uint32_t)0x00000001) /* BUFFER CIRCULAR MODE */ 00080 #define BSP_AUDIO_OUT_NORMALMODE ((uint32_t)0x00000002) /* BUFFER NORMAL MODE */ 00081 #define BSP_AUDIO_OUT_STEREOMODE ((uint32_t)0x00000004) /* STEREO MODE */ 00082 #define BSP_AUDIO_OUT_MONOMODE ((uint32_t)0x00000008) /* MONO MODE */ 00083 /** 00084 * @} 00085 */ 00086 00087 /** @defgroup BSP_Audio_Sample_Rate BSP Audio Sample Rate 00088 * @{ 00089 */ 00090 #define BSP_AUDIO_FREQUENCY_96K SAI_AUDIO_FREQUENCY_96K 00091 #define BSP_AUDIO_FREQUENCY_48K SAI_AUDIO_FREQUENCY_48K 00092 #define BSP_AUDIO_FREQUENCY_44K SAI_AUDIO_FREQUENCY_44K 00093 #define BSP_AUDIO_FREQUENCY_32K SAI_AUDIO_FREQUENCY_32K 00094 #define BSP_AUDIO_FREQUENCY_22K SAI_AUDIO_FREQUENCY_22K 00095 #define BSP_AUDIO_FREQUENCY_16K SAI_AUDIO_FREQUENCY_16K 00096 #define BSP_AUDIO_FREQUENCY_11K SAI_AUDIO_FREQUENCY_11K 00097 #define BSP_AUDIO_FREQUENCY_8K SAI_AUDIO_FREQUENCY_8K 00098 /** 00099 * @} 00100 */ 00101 /*------------------------------------------------------------------------------ 00102 USER SAI defines parameters 00103 -----------------------------------------------------------------------------*/ 00104 /** @defgroup CODEC_AudioFrame_SLOT_TDMMode Codex Audio frame slot in TDM mode 00105 * @brief In W8994 codec the Audio frame contains 4 slots : TDM Mode 00106 * TDM format : 00107 * +------------------|------------------|--------------------|-------------------+ 00108 * | CODEC_SLOT0 Left | CODEC_SLOT1 Left | CODEC_SLOT0 Right | CODEC_SLOT1 Right | 00109 * +------------------------------------------------------------------------------+ 00110 * @{ 00111 */ 00112 /* To have 2 separate audio stream in Both headphone and speaker the 4 slot must be activated */ 00113 #define CODEC_AUDIOFRAME_SLOT_0123 SAI_SLOTACTIVE_0 | SAI_SLOTACTIVE_1 | SAI_SLOTACTIVE_2 | SAI_SLOTACTIVE_3 00114 /* To have an audio stream in headphone only SAI Slot 0 and Slot 2 must be activated */ 00115 #define CODEC_AUDIOFRAME_SLOT_02 SAI_SLOTACTIVE_0 | SAI_SLOTACTIVE_2 00116 /* To have an audio stream in speaker only SAI Slot 1 and Slot 3 must be activated */ 00117 #define CODEC_AUDIOFRAME_SLOT_13 SAI_SLOTACTIVE_1 | SAI_SLOTACTIVE_3 00118 /** 00119 * @} 00120 */ 00121 00122 /* SAI peripheral configuration defines */ 00123 #define AUDIO_SAIx SAI1_Block_B 00124 #define AUDIO_SAIx_CLK_ENABLE() __HAL_RCC_SAI1_CLK_ENABLE() 00125 #define AUDIO_SAIx_MCKB_SCKB_SDB_FSB_AF GPIO_AF13_SAI1 00126 00127 #define AUDIO_SAIx_MCKB_SCKB_SDB_FSB_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00128 #define AUDIO_SAIx_FSB_PIN GPIO_PIN_9 00129 #define AUDIO_SAIx_SCKB_PIN GPIO_PIN_8 00130 #define AUDIO_SAIx_SDB_PIN GPIO_PIN_6 00131 #define AUDIO_SAIx_MCKB_PIN GPIO_PIN_7 00132 #define AUDIO_SAIx_MCKB_SCKB_SDB_FSB_GPIO_PORT GPIOF 00133 00134 #define AUDIO_SAIx_SDA_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00135 #define AUDIO_SAIx_SDA_PIN GPIO_PIN_6 00136 #define AUDIO_SAIx_SDA_GPIO_PORT GPIOD 00137 00138 /* SAI DMA Stream definitions */ 00139 #define AUDIO_SAIx_DMAx_CLK_ENABLE() __HAL_RCC_DMA2_CLK_ENABLE() 00140 #define AUDIO_SAIx_DMAx_CHANNEL DMA2_Channel2 00141 #define AUDIO_SAIx_DMAx_IRQ DMA2_Channel2_IRQn 00142 #define AUDIO_SAIx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00143 #define AUDIO_SAIx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00144 #define DMA_MAX_SZE 0xFFFF 00145 00146 #define AUDIO_SAIx_DMAx_IRQHandler DMA2_Channel2_IRQHandler 00147 00148 /* Select the interrupt preemption priority for the DMA interrupt */ 00149 #define AUDIO_OUT_IRQ_PREPRIO 5 /* Select the preemption priority level(0 is the highest) */ 00150 00151 /*------------------------------------------------------------------------------ 00152 AUDIO IN CONFIGURATION 00153 ------------------------------------------------------------------------------*/ 00154 /* DFSDM Configuration defines */ 00155 #define AUDIO_DFSDMx_LEFT_CHANNEL DFSDM_Channel4 00156 #define AUDIO_DFSDMx_RIGHT_CHANNEL DFSDM_Channel3 00157 #define AUDIO_DFSDMx_LEFT_FILTER DFSDM_Filter0 00158 #define AUDIO_DFSDMx_RIGHT_FILTER DFSDM_Filter1 00159 #define AUDIO_DFSDMx_CLK_ENABLE() __HAL_RCC_DFSDM_CLK_ENABLE() 00160 #define AUDIO_DFSDMx_CKOUT_PIN GPIO_PIN_2 00161 #define AUDIO_DFSDMx_DMIC_DATIN_PIN GPIO_PIN_0 00162 #define AUDIO_DFSDMx_CKOUT_DMIC_DATIN_GPIO_PORT GPIOC 00163 #define AUDIO_DFSDMx_CKOUT_DMIC_DATIN_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00164 #define AUDIO_DFSDMx_CKOUT_DMIC_DATIN_AF GPIO_AF6_DFSDM 00165 00166 /* DFSDM DMA Right and Left channels definitions */ 00167 #define AUDIO_DFSDMx_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00168 #define AUDIO_DFSDMx_DMAx_LEFT_CHANNEL DMA1_Channel4 00169 #define AUDIO_DFSDMx_DMAx_RIGHT_CHANNEL DMA1_Channel5 00170 #define AUDIO_DFSDMx_DMAx_LEFT_IRQ DMA1_Channel4_IRQn 00171 #define AUDIO_DFSDMx_DMAx_RIGHT_IRQ DMA1_Channel5_IRQn 00172 #define AUDIO_DFSDMx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_WORD 00173 #define AUDIO_DFSDMx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_WORD 00174 00175 #define AUDIO_DFSDM_DMAx_LEFT_IRQHandler DMA1_Channel4_IRQHandler 00176 #define AUDIO_DFSDM_DMAx_RIGHT_IRQHandler DMA1_Channel5_IRQHandler 00177 00178 /* Select the interrupt preemption priority and subpriority for the IT/DMA interrupt */ 00179 #define AUDIO_IN_IRQ_PREPRIO 6 /* Select the preemption priority level(0 is the highest) */ 00180 00181 /*------------------------------------------------------------------------------ 00182 CONFIGURATION: Audio Driver Configuration parameters 00183 ------------------------------------------------------------------------------*/ 00184 00185 #define AUDIODATA_SIZE 2 /* 16-bits audio data size */ 00186 00187 /* Audio status definition */ 00188 #define AUDIO_OK 0 00189 #define AUDIO_ERROR 1 00190 #define AUDIO_TIMEOUT 2 00191 00192 /* AudioFreq * DataSize (2 bytes) * NumChannels (Stereo: 2) */ 00193 #define DEFAULT_AUDIO_IN_FREQ SAI_AUDIO_FREQUENCY_16K 00194 #define DEFAULT_AUDIO_IN_BIT_RESOLUTION 16 00195 #define DEFAULT_AUDIO_IN_CHANNEL_NBR 2 /* Mono = 1, Stereo = 2 */ 00196 #define DEFAULT_AUDIO_IN_VOLUME 64 00197 00198 /* PCM buffer input size in word */ 00199 #define INTERNAL_BUFF_SIZE 256 00200 00201 /*------------------------------------------------------------------------------ 00202 OPTIONAL Configuration defines parameters 00203 ------------------------------------------------------------------------------*/ 00204 00205 /* Delay for the Codec to be correctly reset */ 00206 #define CODEC_RESET_DELAY 5 00207 00208 /** 00209 * @} 00210 */ 00211 00212 /** @defgroup STM32L476G_EVAL_AUDIO_Exported_Variables Exported Variables 00213 * @{ 00214 */ 00215 extern __IO uint16_t AudioInVolume; 00216 /** 00217 * @} 00218 */ 00219 00220 /** @defgroup STM32L476G_EVAL_AUDIO_Exported_Macros Exported Macros 00221 * @{ 00222 */ 00223 #define DMA_MAX(_X_) (((_X_) <= DMA_MAX_SZE)? (_X_):DMA_MAX_SZE) 00224 00225 /** 00226 * @} 00227 */ 00228 00229 /* Exported functions --------------------------------------------------------*/ 00230 /** @defgroup STM32L476G_EVAL_AUDIO_Exported_Functions Exported Functions 00231 * @{ 00232 */ 00233 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00234 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size); 00235 uint8_t BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00236 uint8_t BSP_AUDIO_OUT_Pause(void); 00237 uint8_t BSP_AUDIO_OUT_Resume(void); 00238 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option); 00239 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume); 00240 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq); 00241 void BSP_AUDIO_OUT_SetAudioFrameSlot(uint32_t AudioFrameSlot); 00242 void BSP_AUDIO_OUT_ChangeAudioConfig(uint32_t AudioOutOption); 00243 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd); 00244 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output); 00245 00246 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00247 /* This function is called when the requested data has been completely transferred.*/ 00248 void BSP_AUDIO_OUT_TransferComplete_CallBack(void); 00249 00250 /* This function is called when half of the requested buffer has been transferred. */ 00251 void BSP_AUDIO_OUT_HalfTransfer_CallBack(void); 00252 00253 /* This function is called when an Interrupt due to transfer error on or peripheral 00254 error occurs. */ 00255 void BSP_AUDIO_OUT_Error_CallBack(void); 00256 00257 uint8_t BSP_AUDIO_IN_Init(uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr); 00258 uint8_t BSP_AUDIO_IN_Record(uint16_t *pData, uint32_t Size); 00259 uint8_t BSP_AUDIO_IN_SetFrequency(uint32_t AudioFreq); 00260 uint8_t BSP_AUDIO_IN_Stop(void); 00261 uint8_t BSP_AUDIO_IN_Pause(void); 00262 uint8_t BSP_AUDIO_IN_Resume(void); 00263 uint8_t BSP_AUDIO_IN_SetVolume(uint8_t Volume); 00264 void BSP_AUDIO_IN_DeInit(void); 00265 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00266 /* This function should be implemented by the user application. 00267 It is called into this driver when the current buffer is filled to prepare the next 00268 buffer pointer and its size. */ 00269 void BSP_AUDIO_IN_TransferComplete_CallBack(void); 00270 void BSP_AUDIO_IN_HalfTransfer_CallBack(void); 00271 00272 /* This function is called when an Interrupt due to transfer error on or peripheral 00273 error occurs. */ 00274 void BSP_AUDIO_IN_Error_Callback(void); 00275 00276 /** 00277 * @} 00278 */ 00279 00280 /** 00281 * @} 00282 */ 00283 00284 /** 00285 * @} 00286 */ 00287 00288 /** 00289 * @} 00290 */ 00291 00292 #ifdef __cplusplus 00293 } 00294 #endif 00295 00296 #endif /* __STM32L476G_EVAL_AUDIO_H */ 00297 00298 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Sun Jun 21 2015 23:46:41 for STM32L476G_EVAL BSP User Manual by
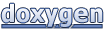