STM32L152D_EVAL BSP User Manual
|
stm32l152d_eval_tsensor.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152d_eval_tsensor.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the I2C STLM75 00006 * temperature sensor mounted on STM32L152D-EVAL board . 00007 * It implements a high level communication layer for read and write 00008 * from/to this sensor. The needed STM32L152xD hardware resources (I2C and 00009 * GPIO) are defined in stm32l152d_eval.h file, and the initialization is 00010 * performed in TSENSOR_IO_Init() function declared in stm32l152d_eval.c 00011 * file. 00012 * You can easily tailor this driver to any other development board, 00013 * by just adapting the defines for hardware resources and 00014 * TSENSOR_IO_Init() function. 00015 * 00016 * +--------------------------------------------------------------------+ 00017 * | Pin assignment | 00018 * +----------------------------------------+--------------+------------+ 00019 * | STM32L152xD I2C Pins | STLM75 | Pin | 00020 * +----------------------------------------+--------------+------------+ 00021 * | EVAL_I2C2_SDA_PIN | SDA | 1 | 00022 * | EVAL_I2C2_SCL_PIN | SCL | 2 | 00023 * | EVAL_I2C2_SMBUS_PIN | OS/INT | 3 | 00024 * | . | GND | 4 (0V) | 00025 * | . | A2 | 5 | 00026 * | . | A1 | 6 | 00027 * | . | A0 | 7 | 00028 * | . | VDD | 8 (3.3V)| 00029 * +----------------------------------------+--------------+------------+ 00030 ****************************************************************************** 00031 * @attention 00032 * 00033 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00034 * 00035 * Redistribution and use in source and binary forms, with or without modification, 00036 * are permitted provided that the following conditions are met: 00037 * 1. Redistributions of source code must retain the above copyright notice, 00038 * this list of conditions and the following disclaimer. 00039 * 2. Redistributions in binary form must reproduce the above copyright notice, 00040 * this list of conditions and the following disclaimer in the documentation 00041 * and/or other materials provided with the distribution. 00042 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00043 * may be used to endorse or promote products derived from this software 00044 * without specific prior written permission. 00045 * 00046 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00047 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00048 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00049 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00050 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00051 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00052 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00053 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00054 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00055 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00056 * 00057 ****************************************************************************** 00058 */ 00059 00060 /* Includes ------------------------------------------------------------------*/ 00061 #include "stm32l152d_eval_tsensor.h" 00062 00063 /** @addtogroup BSP 00064 * @{ 00065 */ 00066 00067 /** @addtogroup STM32L152D_EVAL 00068 * @{ 00069 */ 00070 00071 /** @addtogroup STM32L152D_EVAL_TSENSOR STM32L152D-EVAL TSENSOR 00072 * @brief This file includes the STLM75 Temperature Sensor driver of 00073 * STM32L152D-EVAL board. 00074 * @{ 00075 */ 00076 00077 00078 00079 /** @defgroup STM32L152D_EVAL_TSENSOR_Private_Variables Private Variables 00080 * @{ 00081 */ 00082 static TSENSOR_DrvTypeDef *tsensor_drv; 00083 __IO uint16_t TSENSORAddress = 0; 00084 /** 00085 * @} 00086 */ 00087 00088 00089 /** @defgroup STM32L152D_EVAL_I2C_TSENSOR_Exported_Functions Exported Functions 00090 * @{ 00091 */ 00092 00093 /** 00094 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 00095 * @retval TSENSOR status 00096 */ 00097 uint32_t BSP_TSENSOR_Init(void) 00098 { 00099 uint8_t ret = TSENSOR_ERROR; 00100 TSENSOR_InitTypeDef tsensor_initstruct = {0}; 00101 00102 /* Temperature Sensor Initialization */ 00103 if(Stlm75Drv.IsReady(TSENSOR_I2C_ADDRESS_A01, TSENSOR_MAX_TRIALS) == HAL_OK) 00104 { 00105 /* Initialize the temperature sensor driver structure */ 00106 TSENSORAddress = TSENSOR_I2C_ADDRESS_A01; 00107 tsensor_drv = &Stlm75Drv; 00108 00109 ret = TSENSOR_OK; 00110 } 00111 else 00112 { 00113 if(Stlm75Drv.IsReady(TSENSOR_I2C_ADDRESS_A02, TSENSOR_MAX_TRIALS) == HAL_OK) 00114 { 00115 /* Initialize the temperature sensor driver structure */ 00116 TSENSORAddress = TSENSOR_I2C_ADDRESS_A02; 00117 tsensor_drv = &Stlm75Drv; 00118 00119 ret = TSENSOR_OK; 00120 } 00121 else 00122 { 00123 ret = TSENSOR_ERROR; 00124 } 00125 } 00126 00127 if (ret == TSENSOR_OK) 00128 { 00129 /* Configure Temperature Sensor : Conversion 9 bits in continuous mode */ 00130 /* Alert outside range Limit Temperature 12� <-> 24�c */ 00131 tsensor_initstruct.AlertMode = STLM75_INTERRUPT_MODE; 00132 tsensor_initstruct.ConversionMode = STLM75_CONTINUOUS_MODE; 00133 tsensor_initstruct.TemperatureLimitHigh = 24; 00134 tsensor_initstruct.TemperatureLimitLow = 12; 00135 00136 /* TSENSOR Init */ 00137 tsensor_drv->Init(TSENSORAddress, &tsensor_initstruct); 00138 00139 ret = TSENSOR_OK; 00140 } 00141 00142 return ret; 00143 } 00144 00145 /** 00146 * @brief Returns the Temperature Sensor status. 00147 * @retval The Temperature Sensor status. 00148 */ 00149 uint8_t BSP_TSENSOR_ReadStatus(void) 00150 { 00151 return (tsensor_drv->ReadStatus(TSENSORAddress)); 00152 } 00153 00154 /** 00155 * @brief Read Temperature register of STLM75. 00156 * @retval STLM75 measured temperature value. 00157 */ 00158 uint16_t BSP_TSENSOR_ReadTemp(void) 00159 { 00160 return tsensor_drv->ReadTemp(TSENSORAddress); 00161 00162 } 00163 00164 /** 00165 * @} 00166 */ 00167 00168 00169 /** 00170 * @} 00171 */ 00172 00173 00174 /** 00175 * @} 00176 */ 00177 00178 /** 00179 * @} 00180 */ 00181 00182 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Aug 24 2017 17:57:47 for STM32L152D_EVAL BSP User Manual by
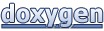