STM32L152D_EVAL BSP User Manual
|
stm32l152d_eval_sram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152d_eval_sram.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to drive the 00006 * IS61WV51216BLL SRAM memory mounted on STM32L152D-EVAL board. 00007 @verbatim 00008 ============================================================================== 00009 ##### How to use this driver ##### 00010 ============================================================================== 00011 [..] 00012 (#) This driver is used to drive the IS61WV102416BLL-10M SRAM external memory mounted 00013 on STM32L152D-EVAL evaluation board. 00014 00015 (#) This driver does not need a specific component driver for the SRAM device 00016 to be included with. 00017 00018 (#) Initialization steps: 00019 (++) Initialize the SRAM external memory using the BSP_SRAM_Init() function. This 00020 function includes the MSP layer hardware resources initialization and the 00021 FSMC controller configuration to interface with the external SRAM memory. 00022 00023 (#) SRAM read/write operations 00024 (++) SRAM external memory can be accessed with read/write operations once it is 00025 initialized. 00026 Read/write operation can be performed with AHB access using the functions 00027 BSP_SRAM_ReadData()/BSP_SRAM_WriteData(), or by DMA transfer using the functions 00028 BSP_SRAM_ReadData_DMA()/BSP_SRAM_WriteData_DMA(). 00029 (++) The AHB access is performed with 16-bit width transaction, the DMA transfer 00030 configuration is fixed at single (no burst) halfword transfer 00031 (see the SRAM_MspInit() static function). 00032 (++) User can implement his own functions for read/write access with his desired 00033 configurations. 00034 (++) If interrupt mode is used for DMA transfer, the function BSP_SRAM_DMA_IRQHandler() 00035 is called in IRQ handler file, to serve the generated interrupt once the DMA 00036 transfer is complete. 00037 @endverbatim 00038 ****************************************************************************** 00039 * @attention 00040 * 00041 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00042 * 00043 * Redistribution and use in source and binary forms, with or without modification, 00044 * are permitted provided that the following conditions are met: 00045 * 1. Redistributions of source code must retain the above copyright notice, 00046 * this list of conditions and the following disclaimer. 00047 * 2. Redistributions in binary form must reproduce the above copyright notice, 00048 * this list of conditions and the following disclaimer in the documentation 00049 * and/or other materials provided with the distribution. 00050 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00051 * may be used to endorse or promote products derived from this software 00052 * without specific prior written permission. 00053 * 00054 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00055 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00056 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00057 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00058 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00059 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00060 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00061 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00062 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00063 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00064 * 00065 ****************************************************************************** 00066 */ 00067 00068 /* Includes ------------------------------------------------------------------*/ 00069 #include "stm32l152d_eval_sram.h" 00070 00071 /** @addtogroup BSP 00072 * @{ 00073 */ 00074 00075 /** @addtogroup STM32L152D_EVAL 00076 * @{ 00077 */ 00078 00079 /** @defgroup STM32L152D_EVAL_SRAM STM32L152D-EVAL SRAM 00080 * @{ 00081 */ 00082 00083 00084 /* Private variables ---------------------------------------------------------*/ 00085 00086 /** @defgroup STM32L152D_EVAL_SRAM_Private_Variables Private Variables 00087 * @{ 00088 */ 00089 static SRAM_HandleTypeDef sramHandle; 00090 static FSMC_NORSRAM_TimingTypeDef Timing; 00091 00092 /** 00093 * @} 00094 */ 00095 00096 /* Private function prototypes -----------------------------------------------*/ 00097 00098 /** @defgroup STM32L152D_EVAL_SRAM_Private_Functions Private Functions 00099 * @{ 00100 */ 00101 static void SRAM_MspInit(void); 00102 00103 /** 00104 * @} 00105 */ 00106 00107 /* Private functions ---------------------------------------------------------*/ 00108 00109 /** @defgroup STM32L152D_EVAL_SRAM_Exported_Functions Exported Functions 00110 * @{ 00111 */ 00112 00113 /** 00114 * @brief Initializes the SRAM device. 00115 * @retval SRAM status 00116 */ 00117 uint8_t BSP_SRAM_Init(void) 00118 { 00119 sramHandle.Instance = FSMC_NORSRAM_DEVICE; 00120 sramHandle.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00121 00122 /* SRAM device configuration */ 00123 Timing.AddressSetupTime = 2; 00124 Timing.AddressHoldTime = 1; 00125 Timing.DataSetupTime = 2; 00126 Timing.BusTurnAroundDuration = 1; 00127 Timing.CLKDivision = 2; 00128 Timing.DataLatency = 2; 00129 Timing.AccessMode = FSMC_ACCESS_MODE_A; 00130 00131 sramHandle.Init.NSBank = FSMC_NORSRAM_BANK3; 00132 sramHandle.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00133 sramHandle.Init.MemoryType = FSMC_MEMORY_TYPE_SRAM; 00134 sramHandle.Init.MemoryDataWidth = SRAM_MEMORY_WIDTH; 00135 sramHandle.Init.BurstAccessMode = SRAM_BURSTACCESS; 00136 sramHandle.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00137 sramHandle.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00138 sramHandle.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00139 sramHandle.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00140 sramHandle.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00141 sramHandle.Init.ExtendedMode = FSMC_EXTENDED_MODE_DISABLE; 00142 sramHandle.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00143 sramHandle.Init.WriteBurst = SRAM_WRITEBURST; 00144 00145 /* SRAM controller initialization */ 00146 SRAM_MspInit(); 00147 if(HAL_SRAM_Init(&sramHandle, &Timing, &Timing) != HAL_OK) 00148 { 00149 return SRAM_ERROR; 00150 } 00151 else 00152 { 00153 return SRAM_OK; 00154 } 00155 } 00156 00157 /** 00158 * @brief Reads an amount of data from the SRAM device in polling mode. 00159 * @param uwStartAddress: Read start address 00160 * @param pData: Pointer to data to be read 00161 * @param uwDataSize: Size of read data from the memory 00162 * @retval SRAM status 00163 */ 00164 uint8_t BSP_SRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00165 { 00166 if(HAL_SRAM_Read_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00167 { 00168 return SRAM_ERROR; 00169 } 00170 else 00171 { 00172 return SRAM_OK; 00173 } 00174 } 00175 00176 /** 00177 * @brief Reads an amount of data from the SRAM device in DMA mode. 00178 * @param uwStartAddress: Read start address 00179 * @param pData: Pointer to data to be read 00180 * @param uwDataSize: Size of read data from the memory 00181 * @retval SRAM status 00182 */ 00183 uint8_t BSP_SRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00184 { 00185 if(HAL_SRAM_Read_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00186 { 00187 return SRAM_ERROR; 00188 } 00189 else 00190 { 00191 return SRAM_OK; 00192 } 00193 } 00194 00195 /** 00196 * @brief Writes an amount of data from the SRAM device in polling mode. 00197 * @param uwStartAddress: Write start address 00198 * @param pData: Pointer to data to be written 00199 * @param uwDataSize: Size of written data from the memory 00200 * @retval SRAM status 00201 */ 00202 uint8_t BSP_SRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00203 { 00204 if(HAL_SRAM_Write_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00205 { 00206 return SRAM_ERROR; 00207 } 00208 else 00209 { 00210 return SRAM_OK; 00211 } 00212 } 00213 00214 /** 00215 * @brief Writes an amount of data from the SRAM device in DMA mode. 00216 * @param uwStartAddress: Write start address 00217 * @param pData: Pointer to data to be written 00218 * @param uwDataSize: Size of written data from the memory 00219 * @retval SRAM status 00220 */ 00221 uint8_t BSP_SRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00222 { 00223 if(HAL_SRAM_Write_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00224 { 00225 return SRAM_ERROR; 00226 } 00227 else 00228 { 00229 return SRAM_OK; 00230 } 00231 } 00232 00233 /** 00234 * @brief Handles SRAM DMA transfer interrupt request. 00235 * @retval None 00236 */ 00237 void BSP_SRAM_DMA_IRQHandler(void) 00238 { 00239 HAL_DMA_IRQHandler(sramHandle.hdma); 00240 } 00241 00242 /** @addtogroup STM32L152D_EVAL_SRAM_Private_Functions 00243 * @{ 00244 */ 00245 00246 /** 00247 * @brief Initializes SRAM MSP. 00248 * @retval None 00249 */ 00250 static void SRAM_MspInit(void) 00251 { 00252 static DMA_HandleTypeDef dmaHandle; 00253 GPIO_InitTypeDef gpioinitstruct; 00254 SRAM_HandleTypeDef *hsram = &sramHandle; 00255 00256 /* Enable FSMC clock */ 00257 __HAL_RCC_FSMC_CLK_ENABLE(); 00258 00259 /* Enable chosen DMAx clock */ 00260 SRAM_DMAx_CLK_ENABLE(); 00261 00262 /* Enable GPIOs clock */ 00263 __HAL_RCC_GPIOD_CLK_ENABLE(); 00264 __HAL_RCC_GPIOE_CLK_ENABLE(); 00265 __HAL_RCC_GPIOF_CLK_ENABLE(); 00266 __HAL_RCC_GPIOG_CLK_ENABLE(); 00267 00268 /* Common GPIO configuration */ 00269 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00270 gpioinitstruct.Pull = GPIO_PULLUP; 00271 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00272 gpioinitstruct.Alternate = GPIO_AF12_FSMC; 00273 00274 /* Common GPIO configuration */ 00275 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00276 gpioinitstruct.Pull = GPIO_PULLUP; 00277 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00278 gpioinitstruct.Alternate = GPIO_AF12_FSMC; 00279 00280 /* GPIOD configuration */ 00281 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 |GPIO_PIN_5 | GPIO_PIN_8 | GPIO_PIN_9 | 00282 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00283 00284 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00285 00286 /* GPIOE configuration */ 00287 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00288 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | 00289 GPIO_PIN_15; 00290 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00291 00292 /* GPIOF configuration */ 00293 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00294 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | 00295 GPIO_PIN_14 | GPIO_PIN_15; 00296 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00297 00298 /* GPIOG configuration */ 00299 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00300 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_10; 00301 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00302 00303 /* Configure common DMA parameters */ 00304 dmaHandle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00305 dmaHandle.Init.PeriphInc = DMA_PINC_ENABLE; 00306 dmaHandle.Init.MemInc = DMA_MINC_ENABLE; 00307 dmaHandle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00308 dmaHandle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00309 dmaHandle.Init.Mode = DMA_NORMAL; 00310 dmaHandle.Init.Priority = DMA_PRIORITY_HIGH; 00311 00312 dmaHandle.Instance = SRAM_DMAx_CHANNEL; 00313 00314 /* Associate the DMA handle */ 00315 __HAL_LINKDMA(hsram, hdma, dmaHandle); 00316 00317 /* Deinitialize the Stream for new transfer */ 00318 HAL_DMA_DeInit(&dmaHandle); 00319 00320 /* Configure the DMA Stream */ 00321 HAL_DMA_Init(&dmaHandle); 00322 00323 /* NVIC configuration for DMA transfer complete interrupt */ 00324 HAL_NVIC_SetPriority(SRAM_DMAx_IRQn, 5, 0); 00325 HAL_NVIC_EnableIRQ(SRAM_DMAx_IRQn); 00326 } 00327 00328 /** 00329 * @} 00330 */ 00331 00332 /** 00333 * @} 00334 */ 00335 00336 /** 00337 * @} 00338 */ 00339 00340 /** 00341 * @} 00342 */ 00343 00344 /** 00345 * @} 00346 */ 00347 00348 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Aug 24 2017 17:57:47 for STM32L152D_EVAL BSP User Manual by
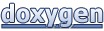