STM32L152D_EVAL BSP User Manual
|
stm32l152d_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152d_eval_lcd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00006 * mounted on STM32L152D-EVAL evaluation board. 00007 @verbatim 00008 ============================================================================== 00009 ##### How to use this driver ##### 00010 ============================================================================== 00011 [..] 00012 (#) This driver is used to drive indirectly an LCD TFT. 00013 00014 (#) This driver supports the AM-240320L8TNQW00H (ILI9320), AM-240320LDTNQW-05H (ILI9325) 00015 AM-240320LDTNQW-02H (SPFD5408B) and AM240320LGTNQW-01H (HX8347D) LCD 00016 mounted on MB895 daughter board 00017 00018 (#) The ILI9320, ILI9325, SPFD5408B and HX8347D components driver MUST be included with this driver. 00019 00020 (#) Initialization steps: 00021 (++) Initialize the LCD using the BSP_LCD_Init() function. 00022 00023 (#) Display on LCD 00024 (++) Clear the hole LCD using yhe BSP_LCD_Clear() function or only one specified 00025 string line using the BSP_LCD_ClearStringLine() function. 00026 (++) Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00027 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00028 (++) Display a string line on the specified position (x,y in pixel) and align mode 00029 using the BSP_LCD_DisplayStringAtLine() function. 00030 (++) Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap, raw picture) 00031 on LCD using a set of functions. 00032 @endverbatim 00033 ****************************************************************************** 00034 * @attention 00035 * 00036 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00037 * 00038 * Redistribution and use in source and binary forms, with or without modification, 00039 * are permitted provided that the following conditions are met: 00040 * 1. Redistributions of source code must retain the above copyright notice, 00041 * this list of conditions and the following disclaimer. 00042 * 2. Redistributions in binary form must reproduce the above copyright notice, 00043 * this list of conditions and the following disclaimer in the documentation 00044 * and/or other materials provided with the distribution. 00045 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00046 * may be used to endorse or promote products derived from this software 00047 * without specific prior written permission. 00048 * 00049 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00050 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00051 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00052 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00053 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00054 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00055 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00056 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00057 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00058 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00059 * 00060 ****************************************************************************** 00061 */ 00062 00063 /* Includes ------------------------------------------------------------------*/ 00064 #include "stm32l152d_eval_lcd.h" 00065 #include "../../../Utilities/Fonts/fonts.h" 00066 #include "../../../Utilities/Fonts/font24.c" 00067 #include "../../../Utilities/Fonts/font20.c" 00068 #include "../../../Utilities/Fonts/font16.c" 00069 #include "../../../Utilities/Fonts/font12.c" 00070 #include "../../../Utilities/Fonts/font8.c" 00071 00072 /** @addtogroup BSP 00073 * @{ 00074 */ 00075 00076 /** @addtogroup STM32L152D_EVAL 00077 * @{ 00078 */ 00079 00080 /** @defgroup STM32L152D_EVAL_LCD STM32L152D-EVAL LCD 00081 * @{ 00082 */ 00083 00084 00085 /** @defgroup STM32L152D_EVAL_LCD_Private_Defines Private Defines 00086 * @{ 00087 */ 00088 #define POLY_X(Z) ((int32_t)((pPoints + (Z))->X)) 00089 #define POLY_Y(Z) ((int32_t)((pPoints + (Z))->Y)) 00090 00091 #define MAX_HEIGHT_FONT 17 00092 #define MAX_WIDTH_FONT 24 00093 #define OFFSET_BITMAP 54 00094 /** 00095 * @} 00096 */ 00097 00098 /** @defgroup STM32L152D_EVAL_LCD_Private_Macros Private Macros 00099 * @{ 00100 */ 00101 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00102 00103 /** 00104 * @} 00105 */ 00106 00107 /** @defgroup STM32L152D_EVAL_LCD_Private_Variables Private Variables 00108 * @{ 00109 */ 00110 LCD_DrawPropTypeDef DrawProp; 00111 00112 static LCD_DrvTypeDef *lcd_drv; 00113 00114 /* Max size of bitmap will based on a font24 (17x24) */ 00115 static uint8_t bitmap[MAX_HEIGHT_FONT*MAX_WIDTH_FONT*2+OFFSET_BITMAP] = {0}; 00116 00117 static uint32_t LCD_SwapXY = 0; 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM32L152D_EVAL_LCD_Private_Functions Private Functions 00123 * @{ 00124 */ 00125 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode); 00126 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar); 00127 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00128 /** 00129 * @} 00130 */ 00131 00132 00133 /** @defgroup STM32L152D_EVAL_LCD_Exported_Functions Exported Functions 00134 * @{ 00135 */ 00136 00137 /** 00138 * @brief Initializes the LCD. 00139 * @retval LCD state 00140 */ 00141 uint8_t BSP_LCD_Init(void) 00142 { 00143 uint8_t ret = LCD_ERROR; 00144 00145 /* Default value for draw propriety */ 00146 DrawProp.BackColor = 0xFFFF; 00147 DrawProp.pFont = &Font24; 00148 DrawProp.TextColor = 0x0000; 00149 00150 if(hx8347d_drv.ReadID() == HX8347D_ID) 00151 { 00152 lcd_drv = &hx8347d_drv; 00153 ret = LCD_OK; 00154 } 00155 else if(spfd5408_drv.ReadID() == SPFD5408_ID) 00156 { 00157 lcd_drv = &spfd5408_drv; 00158 ret = LCD_OK; 00159 } 00160 else if(ili9320_drv.ReadID() == ILI9320_ID) 00161 { 00162 lcd_drv = &ili9320_drv; 00163 LCD_SwapXY = 1; 00164 ret = LCD_OK; 00165 } 00166 else if(ili9325_drv.ReadID() == ILI9325_ID) 00167 { 00168 lcd_drv = &ili9325_drv; 00169 LCD_SwapXY = 1; 00170 ret = LCD_OK; 00171 } 00172 00173 if(ret != LCD_ERROR) 00174 { 00175 /* LCD Init */ 00176 lcd_drv->Init(); 00177 00178 /* Initialize the font */ 00179 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00180 } 00181 00182 return ret; 00183 } 00184 00185 /** 00186 * @brief Gets the LCD X size. 00187 * @retval Used LCD X size 00188 */ 00189 uint32_t BSP_LCD_GetXSize(void) 00190 { 00191 return(lcd_drv->GetLcdPixelWidth()); 00192 } 00193 00194 /** 00195 * @brief Gets the LCD Y size. 00196 * @retval Used LCD Y size 00197 */ 00198 uint32_t BSP_LCD_GetYSize(void) 00199 { 00200 return(lcd_drv->GetLcdPixelHeight()); 00201 } 00202 00203 /** 00204 * @brief Gets the LCD text color. 00205 * @retval Used text color. 00206 */ 00207 uint16_t BSP_LCD_GetTextColor(void) 00208 { 00209 return DrawProp.TextColor; 00210 } 00211 00212 /** 00213 * @brief Gets the LCD background color. 00214 * @retval Used background color 00215 */ 00216 uint16_t BSP_LCD_GetBackColor(void) 00217 { 00218 return DrawProp.BackColor; 00219 } 00220 00221 /** 00222 * @brief Sets the LCD text color. 00223 * @param Color: Text color code RGB(5-6-5) 00224 * @retval None 00225 */ 00226 void BSP_LCD_SetTextColor(uint16_t Color) 00227 { 00228 DrawProp.TextColor = Color; 00229 } 00230 00231 /** 00232 * @brief Sets the LCD background color. 00233 * @param Color: Background color code RGB(5-6-5) 00234 * @retval None 00235 */ 00236 void BSP_LCD_SetBackColor(uint16_t Color) 00237 { 00238 DrawProp.BackColor = Color; 00239 } 00240 00241 /** 00242 * @brief Sets the LCD text font. 00243 * @param pFonts: Font to be used 00244 * @retval None 00245 */ 00246 void BSP_LCD_SetFont(sFONT *pFonts) 00247 { 00248 DrawProp.pFont = pFonts; 00249 } 00250 00251 /** 00252 * @brief Gets the LCD text font. 00253 * @retval Used font 00254 */ 00255 sFONT *BSP_LCD_GetFont(void) 00256 { 00257 return DrawProp.pFont; 00258 } 00259 00260 /** 00261 * @brief Clears the hole LCD. 00262 * @param Color: Color of the background 00263 * @retval None 00264 */ 00265 void BSP_LCD_Clear(uint16_t Color) 00266 { 00267 uint32_t counter = 0; 00268 00269 uint32_t color_backup = DrawProp.TextColor; 00270 DrawProp.TextColor = Color; 00271 00272 for(counter = 0; counter < BSP_LCD_GetYSize(); counter++) 00273 { 00274 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00275 } 00276 00277 DrawProp.TextColor = color_backup; 00278 BSP_LCD_SetTextColor(DrawProp.TextColor); 00279 } 00280 00281 /** 00282 * @brief Clears the selected line. 00283 * @param Line: Line to be cleared 00284 * This parameter can be one of the following values: 00285 * @arg 0..9: if the Current fonts is Font16x24 00286 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00287 * @arg 0..29: if the Current fonts is Font8x8 00288 * @retval None 00289 */ 00290 void BSP_LCD_ClearStringLine(uint16_t Line) 00291 { 00292 uint32_t colorbackup = DrawProp.TextColor; 00293 DrawProp.TextColor = DrawProp.BackColor;; 00294 00295 /* Draw a rectangle with background color */ 00296 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00297 00298 DrawProp.TextColor = colorbackup; 00299 BSP_LCD_SetTextColor(DrawProp.TextColor); 00300 } 00301 00302 /** 00303 * @brief Displays one character. 00304 * @param Xpos: Start column address 00305 * @param Ypos: Line where to display the character shape. 00306 * @param Ascii: Character ascii code 00307 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00308 * @retval None 00309 */ 00310 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00311 { 00312 LCD_DrawChar(Ypos, Xpos, &DrawProp.pFont->table[(Ascii-' ') *\ 00313 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00314 } 00315 00316 /** 00317 * @brief Displays characters on the LCD. 00318 * @param Xpos: X position (in pixel) 00319 * @param Ypos: Y position (in pixel) 00320 * @param pText: Pointer to string to display on LCD 00321 * @param Mode: Display mode 00322 * This parameter can be one of the following values: 00323 * @arg CENTER_MODE 00324 * @arg RIGHT_MODE 00325 * @arg LEFT_MODE 00326 * @retval None 00327 */ 00328 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *pText, Line_ModeTypdef Mode) 00329 { 00330 uint16_t refcolumn = 1, counter = 0; 00331 uint32_t size = 0, ysize = 0; 00332 uint8_t *ptr = pText; 00333 00334 /* Get the text size */ 00335 while (*ptr++) size ++ ; 00336 00337 /* Characters number per line */ 00338 ysize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00339 00340 switch (Mode) 00341 { 00342 case CENTER_MODE: 00343 { 00344 refcolumn = Xpos + ((ysize - size)* DrawProp.pFont->Width) / 2; 00345 break; 00346 } 00347 case LEFT_MODE: 00348 { 00349 refcolumn = Xpos; 00350 break; 00351 } 00352 case RIGHT_MODE: 00353 { 00354 refcolumn = Xpos + ((ysize - size)*DrawProp.pFont->Width); 00355 break; 00356 } 00357 default: 00358 { 00359 refcolumn = Xpos; 00360 break; 00361 } 00362 } 00363 00364 /* Send the string character by character on lCD */ 00365 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (counter*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00366 { 00367 /* Display one character on LCD */ 00368 BSP_LCD_DisplayChar(refcolumn, Ypos, *pText); 00369 /* Decrement the column position by 16 */ 00370 refcolumn += DrawProp.pFont->Width; 00371 /* Point on the next character */ 00372 pText++; 00373 counter++; 00374 } 00375 } 00376 00377 /** 00378 * @brief Displays a character on the LCD. 00379 * @param Line: Line where to display the character shape 00380 * This parameter can be one of the following values: 00381 * @arg 0..9: if the Current fonts is Font16x24 00382 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00383 * @arg 0..29: if the Current fonts is Font8x8 00384 * @param pText: Pointer to string to display on LCD 00385 * @retval None 00386 */ 00387 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *pText) 00388 { 00389 BSP_LCD_DisplayStringAt(0, LINE(Line),pText, LEFT_MODE); 00390 } 00391 00392 /** 00393 * @brief Reads an LCD pixel. 00394 * @param Xpos: X position 00395 * @param Ypos: Y position 00396 * @retval RGB pixel color 00397 */ 00398 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00399 { 00400 uint16_t ret = 0; 00401 00402 if(lcd_drv->ReadPixel != NULL) 00403 { 00404 ret = lcd_drv->ReadPixel(Xpos, Ypos); 00405 } 00406 00407 return ret; 00408 } 00409 00410 /** 00411 * @brief Draws an horizontal line. 00412 * @param Xpos: X position 00413 * @param Ypos: Y position 00414 * @param Length: Line length 00415 * @retval None 00416 */ 00417 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00418 { 00419 uint32_t index = 0; 00420 00421 if(lcd_drv->DrawHLine != NULL) 00422 { 00423 if (LCD_SwapXY) 00424 { 00425 uint16_t tmp = Ypos; 00426 Ypos = Xpos; 00427 Xpos = tmp; 00428 } 00429 00430 lcd_drv->DrawHLine(DrawProp.TextColor, Ypos, Xpos, Length); 00431 } 00432 else 00433 { 00434 for(index = 0; index < Length; index++) 00435 { 00436 LCD_DrawPixel((Ypos + index), Xpos, DrawProp.TextColor); 00437 } 00438 } 00439 } 00440 00441 /** 00442 * @brief Draws a vertical line. 00443 * @param Xpos: X position 00444 * @param Ypos: Y position 00445 * @param Length: Line length 00446 * @retval None 00447 */ 00448 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00449 { 00450 uint32_t index = 0; 00451 00452 if(lcd_drv->DrawVLine != NULL) 00453 { 00454 if (LCD_SwapXY) 00455 { 00456 uint16_t tmp = Ypos; 00457 Ypos = Xpos; 00458 Xpos = tmp; 00459 } 00460 00461 LCD_SetDisplayWindow(Ypos, Xpos, 1, Length); 00462 lcd_drv->DrawVLine(DrawProp.TextColor, Ypos, Xpos, Length); 00463 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00464 } 00465 else 00466 { 00467 for(index = 0; index < Length; index++) 00468 { 00469 LCD_DrawPixel(Ypos, Xpos + index, DrawProp.TextColor); 00470 } 00471 } 00472 } 00473 00474 /** 00475 * @brief Draws an uni-line (between two points). 00476 * @param X1: Point 1 X position 00477 * @param Y1: Point 1 Y position 00478 * @param X2: Point 2 X position 00479 * @param Y2: Point 2 Y position 00480 * @retval None 00481 */ 00482 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2) 00483 { 00484 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00485 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00486 curpixel = 0; 00487 00488 deltax = ABS(Y2 - Y1); /* The difference between the x's */ 00489 deltay = ABS(X2 - X1); /* The difference between the y's */ 00490 x = Y1; /* Start x off at the first pixel */ 00491 y = X1; /* Start y off at the first pixel */ 00492 00493 if (Y2 >= Y1) /* The x-values are increasing */ 00494 { 00495 xinc1 = 1; 00496 xinc2 = 1; 00497 } 00498 else /* The x-values are decreasing */ 00499 { 00500 xinc1 = -1; 00501 xinc2 = -1; 00502 } 00503 00504 if (X2 >= X1) /* The y-values are increasing */ 00505 { 00506 yinc1 = 1; 00507 yinc2 = 1; 00508 } 00509 else /* The y-values are decreasing */ 00510 { 00511 yinc1 = -1; 00512 yinc2 = -1; 00513 } 00514 00515 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00516 { 00517 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00518 yinc2 = 0; /* Don't change the y for every iteration */ 00519 den = deltax; 00520 num = deltax / 2; 00521 numadd = deltay; 00522 numpixels = deltax; /* There are more x-values than y-values */ 00523 } 00524 else /* There is at least one y-value for every x-value */ 00525 { 00526 xinc2 = 0; /* Don't change the x for every iteration */ 00527 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00528 den = deltay; 00529 num = deltay / 2; 00530 numadd = deltax; 00531 numpixels = deltay; /* There are more y-values than x-values */ 00532 } 00533 00534 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00535 { 00536 LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00537 num += numadd; /* Increase the numerator by the top of the fraction */ 00538 if (num >= den) /* Check if numerator >= denominator */ 00539 { 00540 num -= den; /* Calculate the new numerator value */ 00541 x += xinc1; /* Change the x as appropriate */ 00542 y += yinc1; /* Change the y as appropriate */ 00543 } 00544 x += xinc2; /* Change the x as appropriate */ 00545 y += yinc2; /* Change the y as appropriate */ 00546 } 00547 } 00548 00549 /** 00550 * @brief Draws a rectangle. 00551 * @param Xpos: X position 00552 * @param Ypos: Y position 00553 * @param Width: Rectangle width 00554 * @param Height: Rectangle height 00555 * @retval None 00556 */ 00557 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00558 { 00559 /* Draw horizontal lines */ 00560 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00561 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00562 00563 /* Draw vertical lines */ 00564 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00565 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00566 } 00567 00568 /** 00569 * @brief Draws a circle. 00570 * @param Xpos: X position 00571 * @param Ypos: Y position 00572 * @param Radius: Circle radius 00573 * @retval None 00574 */ 00575 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00576 { 00577 int32_t decision; /* Decision Variable */ 00578 uint32_t curx; /* Current X Value */ 00579 uint32_t cury; /* Current Y Value */ 00580 00581 decision = 3 - (Radius << 1); 00582 curx = 0; 00583 cury = Radius; 00584 00585 while (curx <= cury) 00586 { 00587 LCD_DrawPixel((Ypos + curx), (Xpos - cury), DrawProp.TextColor); 00588 00589 LCD_DrawPixel((Ypos - curx), (Xpos - cury), DrawProp.TextColor); 00590 00591 LCD_DrawPixel((Ypos + cury), (Xpos - curx), DrawProp.TextColor); 00592 00593 LCD_DrawPixel((Ypos - cury), (Xpos - curx), DrawProp.TextColor); 00594 00595 LCD_DrawPixel((Ypos + curx), (Xpos + cury), DrawProp.TextColor); 00596 00597 LCD_DrawPixel((Ypos - curx), (Xpos + cury), DrawProp.TextColor); 00598 00599 LCD_DrawPixel((Ypos + cury), (Xpos + curx), DrawProp.TextColor); 00600 00601 LCD_DrawPixel((Ypos - cury), (Xpos + curx), DrawProp.TextColor); 00602 00603 /* Initialize the font */ 00604 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00605 00606 if (decision < 0) 00607 { 00608 decision += (curx << 2) + 6; 00609 } 00610 else 00611 { 00612 decision += ((curx - cury) << 2) + 10; 00613 cury--; 00614 } 00615 curx++; 00616 } 00617 } 00618 00619 /** 00620 * @brief Draws an poly-line (between many points). 00621 * @param pPoints: Pointer to the points array 00622 * @param PointCount: Number of points 00623 * @retval None 00624 */ 00625 void BSP_LCD_DrawPolygon(pPoint pPoints, uint16_t PointCount) 00626 { 00627 int16_t x = 0, y = 0; 00628 00629 if(PointCount < 2) 00630 { 00631 return; 00632 } 00633 00634 BSP_LCD_DrawLine(pPoints->X, pPoints->Y, (pPoints+PointCount-1)->X, (pPoints+PointCount-1)->Y); 00635 00636 while(--PointCount) 00637 { 00638 x = pPoints->X; 00639 y = pPoints->Y; 00640 pPoints++; 00641 BSP_LCD_DrawLine(x, y, pPoints->X, pPoints->Y); 00642 } 00643 00644 } 00645 00646 /** 00647 * @brief Draws an ellipse on LCD. 00648 * @param Xpos: X position 00649 * @param Ypos: Y position 00650 * @param XRadius: Ellipse X radius 00651 * @param YRadius: Ellipse Y radius 00652 * @retval None 00653 */ 00654 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00655 { 00656 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00657 float k = 0, rad1 = 0, rad2 = 0; 00658 00659 rad1 = YRadius; 00660 rad2 = XRadius; 00661 00662 k = (float)(rad2/rad1); 00663 00664 do { 00665 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00666 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00667 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00668 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00669 00670 e2 = err; 00671 if (e2 <= x) { 00672 err += ++x*2+1; 00673 if (-y == x && e2 <= y) e2 = 0; 00674 } 00675 if (e2 > y) err += ++y*2+1; 00676 } 00677 while (y <= 0); 00678 } 00679 00680 /** 00681 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 00682 * @param Xpos: Bmp X position in the LCD 00683 * @param Ypos: Bmp Y position in the LCD 00684 * @param pBmp: Pointer to Bmp picture address in the internal Flash 00685 * @retval None 00686 */ 00687 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pBmp) 00688 { 00689 uint32_t height = 0, width = 0; 00690 00691 if (LCD_SwapXY) 00692 { 00693 uint16_t tmp = Ypos; 00694 Ypos = Xpos; 00695 Xpos = tmp; 00696 } 00697 00698 /* Read bitmap width */ 00699 width = *(uint16_t *) (pBmp + 18); 00700 width |= (*(uint16_t *) (pBmp + 20)) << 16; 00701 00702 /* Read bitmap height */ 00703 height = *(uint16_t *) (pBmp + 22); 00704 height |= (*(uint16_t *) (pBmp + 24)) << 16; 00705 00706 /* Remap Ypos, hx8347d works with inverted X in case of bitmap */ 00707 /* X = 0, cursor is on Bottom corner */ 00708 if(lcd_drv == &hx8347d_drv) 00709 { 00710 Ypos = BSP_LCD_GetYSize() - Ypos - height; 00711 } 00712 00713 LCD_SetDisplayWindow(Ypos, Xpos, width, height); 00714 00715 if(lcd_drv->DrawBitmap != NULL) 00716 { 00717 lcd_drv->DrawBitmap(Ypos, Xpos, pBmp); 00718 } 00719 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00720 } 00721 00722 /** 00723 * @brief Draws a full rectangle. 00724 * @param Xpos: X position 00725 * @param Ypos: Y position 00726 * @param Width: Rectangle width 00727 * @param Height: Rectangle height 00728 * @retval None 00729 */ 00730 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00731 { 00732 BSP_LCD_SetTextColor(DrawProp.TextColor); 00733 do 00734 { 00735 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00736 } 00737 while(Height--); 00738 } 00739 00740 /** 00741 * @brief Draws a full circle. 00742 * @param Xpos: X position 00743 * @param Ypos: Y position 00744 * @param Radius: Circle radius 00745 * @retval None 00746 */ 00747 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00748 { 00749 int32_t decision; /* Decision Variable */ 00750 uint32_t curx; /* Current X Value */ 00751 uint32_t cury; /* Current Y Value */ 00752 00753 decision = 3 - (Radius << 1); 00754 00755 curx = 0; 00756 cury = Radius; 00757 00758 BSP_LCD_SetTextColor(DrawProp.TextColor); 00759 00760 while (curx <= cury) 00761 { 00762 if(cury > 0) 00763 { 00764 BSP_LCD_DrawVLine(Xpos + curx, Ypos - cury, 2*cury); 00765 BSP_LCD_DrawVLine(Xpos - curx, Ypos - cury, 2*cury); 00766 } 00767 00768 if(curx > 0) 00769 { 00770 BSP_LCD_DrawVLine(Xpos - cury, Ypos - curx, 2*curx); 00771 BSP_LCD_DrawVLine(Xpos + cury, Ypos - curx, 2*curx); 00772 } 00773 if (decision < 0) 00774 { 00775 decision += (curx << 2) + 6; 00776 } 00777 else 00778 { 00779 decision += ((curx - cury) << 2) + 10; 00780 cury--; 00781 } 00782 curx++; 00783 } 00784 00785 BSP_LCD_SetTextColor(DrawProp.TextColor); 00786 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00787 } 00788 00789 /** 00790 * @brief Fill triangle. 00791 * @param X1: specifies the point 1 x position. 00792 * @param Y1: specifies the point 1 y position. 00793 * @param X2: specifies the point 2 x position. 00794 * @param Y2: specifies the point 2 y position. 00795 * @param X3: specifies the point 3 x position. 00796 * @param Y3: specifies the point 3 y position. 00797 * @retval None 00798 */ 00799 void BSP_LCD_FillTriangle(uint16_t X1, uint16_t X2, uint16_t X3, uint16_t Y1, uint16_t Y2, uint16_t Y3) 00800 { 00801 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00802 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00803 curpixel = 0; 00804 00805 deltax = ABS(X2 - X1); /* The difference between the x's */ 00806 deltay = ABS(Y2 - Y1); /* The difference between the y's */ 00807 x = X1; /* Start x off at the first pixel */ 00808 y = Y1; /* Start y off at the first pixel */ 00809 00810 if (X2 >= X1) /* The x-values are increasing */ 00811 { 00812 xinc1 = 1; 00813 xinc2 = 1; 00814 } 00815 else /* The x-values are decreasing */ 00816 { 00817 xinc1 = -1; 00818 xinc2 = -1; 00819 } 00820 00821 if (Y2 >= Y1) /* The y-values are increasing */ 00822 { 00823 yinc1 = 1; 00824 yinc2 = 1; 00825 } 00826 else /* The y-values are decreasing */ 00827 { 00828 yinc1 = -1; 00829 yinc2 = -1; 00830 } 00831 00832 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00833 { 00834 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00835 yinc2 = 0; /* Don't change the y for every iteration */ 00836 den = deltax; 00837 num = deltax / 2; 00838 numadd = deltay; 00839 numpixels = deltax; /* There are more x-values than y-values */ 00840 } 00841 else /* There is at least one y-value for every x-value */ 00842 { 00843 xinc2 = 0; /* Don't change the x for every iteration */ 00844 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00845 den = deltay; 00846 num = deltay / 2; 00847 numadd = deltax; 00848 numpixels = deltay; /* There are more y-values than x-values */ 00849 } 00850 00851 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00852 { 00853 BSP_LCD_DrawLine(x, y, X3, Y3); 00854 00855 num += numadd; /* Increase the numerator by the top of the fraction */ 00856 if (num >= den) /* Check if numerator >= denominator */ 00857 { 00858 num -= den; /* Calculate the new numerator value */ 00859 x += xinc1; /* Change the x as appropriate */ 00860 y += yinc1; /* Change the y as appropriate */ 00861 } 00862 x += xinc2; /* Change the x as appropriate */ 00863 y += yinc2; /* Change the y as appropriate */ 00864 } 00865 } 00866 00867 /** 00868 * @brief Displays a full poly-line (between many points). 00869 * @param pPoints: pointer to the points array. 00870 * @param PointCount: Number of points. 00871 * @retval None 00872 */ 00873 void BSP_LCD_FillPolygon(pPoint pPoints, uint16_t PointCount) 00874 { 00875 00876 int16_t x = 0, y = 0, x2 = 0, y2 = 0, xcenter = 0, ycenter = 0, xfirst = 0, yfirst = 0, pixelx = 0, pixely = 0, counter = 0; 00877 uint16_t imageleft = 0, imageright = 0, imagetop = 0, imagebottom = 0; 00878 00879 imageleft = imageright = pPoints->X; 00880 imagetop= imagebottom = pPoints->Y; 00881 00882 for(counter = 1; counter < PointCount; counter++) 00883 { 00884 pixelx = POLY_X(counter); 00885 if(pixelx < imageleft) 00886 { 00887 imageleft = pixelx; 00888 } 00889 if(pixelx > imageright) 00890 { 00891 imageright = pixelx; 00892 } 00893 00894 pixely = POLY_Y(counter); 00895 if(pixely < imagetop) 00896 { 00897 imagetop = pixely; 00898 } 00899 if(pixely > imagebottom) 00900 { 00901 imagebottom = pixely; 00902 } 00903 } 00904 00905 if(PointCount < 2) 00906 { 00907 return; 00908 } 00909 00910 xcenter = (imageleft + imageright)/2; 00911 ycenter = (imagebottom + imagetop)/2; 00912 00913 xfirst = pPoints->X; 00914 yfirst = pPoints->Y; 00915 00916 while(--PointCount) 00917 { 00918 x = pPoints->X; 00919 y = pPoints->Y; 00920 pPoints++; 00921 x2 = pPoints->X; 00922 y2 = pPoints->Y; 00923 00924 BSP_LCD_FillTriangle(x, x2, xcenter, y, y2, ycenter); 00925 BSP_LCD_FillTriangle(x, xcenter, x2, y, ycenter, y2); 00926 BSP_LCD_FillTriangle(xcenter, x2, x, ycenter, y2, y); 00927 } 00928 00929 BSP_LCD_FillTriangle(xfirst, x2, xcenter, yfirst, y2, ycenter); 00930 BSP_LCD_FillTriangle(xfirst, xcenter, x2, yfirst, ycenter, y2); 00931 BSP_LCD_FillTriangle(xcenter, x2, xfirst, ycenter, y2, yfirst); 00932 } 00933 00934 /** 00935 * @brief Draws a full ellipse. 00936 * @param Xpos: X position 00937 * @param Ypos: Y position 00938 * @param XRadius: Ellipse X radius 00939 * @param YRadius: Ellipse Y radius 00940 * @retval None 00941 */ 00942 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00943 { 00944 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00945 float k = 0, rad1 = 0, rad2 = 0; 00946 00947 rad1 = YRadius; 00948 rad2 = XRadius; 00949 00950 k = (float)(rad2/rad1); 00951 00952 do 00953 { 00954 BSP_LCD_DrawVLine((Xpos+y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00955 BSP_LCD_DrawVLine((Xpos-y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00956 00957 e2 = err; 00958 if (e2 <= x) 00959 { 00960 err += ++x*2+1; 00961 if (-y == x && e2 <= y) e2 = 0; 00962 } 00963 if (e2 > y) err += ++y*2+1; 00964 } 00965 while (y <= 0); 00966 } 00967 00968 /** 00969 * @brief Enables the display. 00970 * @retval None 00971 */ 00972 void BSP_LCD_DisplayOn(void) 00973 { 00974 lcd_drv->DisplayOn(); 00975 } 00976 00977 /** 00978 * @brief Disables the display. 00979 * @retval None 00980 */ 00981 void BSP_LCD_DisplayOff(void) 00982 { 00983 lcd_drv->DisplayOff(); 00984 } 00985 00986 /** 00987 * @} 00988 */ 00989 00990 /** @addtogroup STM32L152D_EVAL_LCD_Private_Functions 00991 * @{ 00992 */ 00993 00994 /****************************************************************************** 00995 Static Function 00996 *******************************************************************************/ 00997 /** 00998 * @brief Draws a pixel on LCD. 00999 * @param Xpos: X position 01000 * @param Ypos: Y position 01001 * @param RGBCode: Pixel color in RGB mode (5-6-5) 01002 * @retval None 01003 */ 01004 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode) 01005 { 01006 if (LCD_SwapXY) 01007 { 01008 uint16_t tmp = Ypos; 01009 Ypos = Xpos; 01010 Xpos = tmp; 01011 } 01012 01013 if(lcd_drv->WritePixel != NULL) 01014 { 01015 lcd_drv->WritePixel(Xpos, Ypos, RGBCode); 01016 } 01017 } 01018 01019 /** 01020 * @brief Draws a character on LCD. 01021 * @param Xpos: Line where to display the character shape 01022 * @param Ypos: Start column address 01023 * @param pChar: Pointer to the character data 01024 * @retval None 01025 */ 01026 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar) 01027 { 01028 uint32_t counterh = 0, counterw = 0, index = 0; 01029 uint16_t height = 0, width = 0; 01030 uint8_t offset = 0; 01031 uint8_t *pchar = NULL; 01032 uint32_t line = 0; 01033 01034 01035 height = DrawProp.pFont->Height; 01036 width = DrawProp.pFont->Width; 01037 01038 /* Fill bitmap header*/ 01039 *(uint16_t *) (bitmap + 2) = (uint16_t)(height*width*2+OFFSET_BITMAP); 01040 *(uint16_t *) (bitmap + 4) = (uint16_t)((height*width*2+OFFSET_BITMAP)>>16); 01041 *(uint16_t *) (bitmap + 10) = OFFSET_BITMAP; 01042 *(uint16_t *) (bitmap + 18) = (uint16_t)(width); 01043 *(uint16_t *) (bitmap + 20) = (uint16_t)((width)>>16); 01044 *(uint16_t *) (bitmap + 22) = (uint16_t)(height); 01045 *(uint16_t *) (bitmap + 24) = (uint16_t)((height)>>16); 01046 01047 offset = 8 *((width + 7)/8) - width ; 01048 01049 for(counterh = 0; counterh < height; counterh++) 01050 { 01051 pchar = ((uint8_t *)pChar + (width + 7)/8 * counterh); 01052 01053 if(((width + 7)/8) == 3) 01054 { 01055 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01056 } 01057 01058 if(((width + 7)/8) == 2) 01059 { 01060 line = (pchar[0]<< 8) | pchar[1]; 01061 } 01062 01063 if(((width + 7)/8) == 1) 01064 { 01065 line = pchar[0]; 01066 } 01067 01068 for (counterw = 0; counterw < width; counterw++) 01069 { 01070 /* Image in the bitmap is written from the bottom to the top */ 01071 /* Need to invert image in the bitmap */ 01072 index = (((height-counterh-1)*width)+(counterw))*2+OFFSET_BITMAP; 01073 if(line & (1 << (width- counterw + offset- 1))) 01074 { 01075 bitmap[index] = (uint8_t)DrawProp.TextColor; 01076 bitmap[index+1] = (uint8_t)(DrawProp.TextColor >> 8); 01077 } 01078 else 01079 { 01080 bitmap[index] = (uint8_t)DrawProp.BackColor; 01081 bitmap[index+1] = (uint8_t)(DrawProp.BackColor >> 8); 01082 } 01083 } 01084 } 01085 01086 BSP_LCD_DrawBitmap(Ypos, Xpos, bitmap); 01087 } 01088 01089 /** 01090 * @brief Sets display window. 01091 * @param Xpos: LCD X position 01092 * @param Ypos: LCD Y position 01093 * @param Width: LCD window width 01094 * @param Height: LCD window height 01095 * @retval None 01096 */ 01097 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01098 { 01099 if(lcd_drv->SetDisplayWindow != NULL) 01100 { 01101 lcd_drv->SetDisplayWindow(Xpos, Ypos, Width, Height); 01102 } 01103 } 01104 01105 /** 01106 * @} 01107 */ 01108 01109 /** 01110 * @} 01111 */ 01112 01113 /** 01114 * @} 01115 */ 01116 01117 /** 01118 * @} 01119 */ 01120 01121 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Aug 24 2017 17:57:47 for STM32L152D_EVAL BSP User Manual by
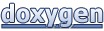