STM32L152D_EVAL BSP User Manual
|
stm32l152d_eval_audio.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152d_eval_audio.h 00004 * @author MCD Application Team 00005 * @brief This file contains the common defines and functions prototypes for 00006 * stm32l152d_eval_audio.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32L152D_EVAL_AUDIO_H 00039 #define __STM32L152D_EVAL_AUDIO_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 /* Include audio component Driver */ 00047 #include "../Components/cs43l22/cs43l22.h" 00048 #include "stm32l152d_eval.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32L152D_EVAL 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM32L152D_EVAL_AUDIO 00059 * @{ 00060 */ 00061 00062 00063 /** @defgroup STM32L152D_EVAL_AUDIO_OUT_Exported_Constants Exported Constants 00064 * @{ 00065 */ 00066 00067 00068 /*------------------------------------------------------------------------------ 00069 AUDIO OUT CONFIGURATION 00070 ------------------------------------------------------------------------------*/ 00071 00072 /* I2S peripheral configuration defines */ 00073 #define I2SOUT SPI2 00074 #define I2SOUT_CLK_ENABLE() __HAL_RCC_SPI2_CLK_ENABLE() 00075 #define I2SOUT_SCK_SD_WS_AF GPIO_AF5_SPI2 00076 #define I2SOUT_SCK_SD_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00077 #define I2SOUT_MCK_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00078 #define I2SOUT_WS_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00079 #define I2SOUT_WS_PIN GPIO_PIN_12 /* PB.12*/ 00080 #define I2SOUT_SCK_PIN GPIO_PIN_13 /* PB.13*/ 00081 #define I2SOUT_SD_PIN GPIO_PIN_15 /* PB.15*/ 00082 #define I2SOUT_MCK_PIN GPIO_PIN_6 /* PC.06*/ 00083 #define I2SOUT_SCK_SD_GPIO_PORT GPIOB 00084 #define I2SOUT_WS_GPIO_PORT GPIOB 00085 #define I2SOUT_MCK_GPIO_PORT GPIOC 00086 00087 /* I2S DMA Channel definitions */ 00088 #define I2SOUT_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00089 #define I2SOUT_DMAx_CHANNEL DMA1_Channel5 00090 #define I2SOUT_DMAx_IRQ DMA1_Channel5_IRQn 00091 #define I2SOUT_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00092 #define I2SOUT_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00093 #define DMA_MAX_SZE 0xFFFF 00094 00095 #define I2SOUT_IRQHandler DMA1_Channel5_IRQHandler 00096 00097 /* Select the interrupt preemption priority and subpriority for the DMA interrupt */ 00098 #define AUDIO_OUT_IRQ_PREPRIO 5 /* Select the preemption priority level(0 is the highest) */ 00099 00100 /*------------------------------------------------------------------------------ 00101 AUDIO IN CONFIGURATION 00102 ------------------------------------------------------------------------------*/ 00103 00104 /* ADC peripheral configuration defines */ 00105 #define AUDIO_IN_ADC_PIN GPIO_PIN_3 /* PA.03*/ 00106 #define AUDIO_IN_ADC_PORT GPIOA 00107 #define AUDIO_IN_ADC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00108 #define AUDIO_IN_ADC_CHANNEL ADC_CHANNEL_3 00109 00110 /* ADC DMA Channel definitions */ 00111 #define AUDIO_IN_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00112 #define AUDIO_IN_DMAx_CHANNEL DMA1_Channel1 00113 #define AUDIO_IN_DMAx_IRQ DMA1_Channel1_IRQn 00114 #define AUDIO_IN_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00115 #define AUDIO_IN_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00116 00117 #define AUDIO_IN_IRQHandler DMA1_Channel1_IRQHandler 00118 00119 /* Select the interrupt preemption priority and subpriority for the IT/DMA interrupt */ 00120 #define AUDIO_IN_IRQ_PREPRIO 6 /* Select the preemption priority level(0 is the highest) */ 00121 00122 /* OPAMP peripheral configuration defines */ 00123 #define AUDIO_IN_OPAMP_PIN (GPIO_PIN_1 | GPIO_PIN_2) /* PA.01 & PA.02*/ 00124 #define AUDIO_IN_OPAMP_PORT GPIOA 00125 #define AUDIO_IN_OPAMP_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00126 00127 00128 /*------------------------------------------------------------------------------ 00129 CONFIGURATION: Audio Driver Configuration parameters 00130 ------------------------------------------------------------------------------*/ 00131 00132 /* Audio status definition */ 00133 #define AUDIO_OK 0 00134 #define AUDIO_ERROR 1 00135 #define AUDIO_TIMEOUT 2 00136 00137 /* AudioFreq * DataSize (2 bytes) * NumChannels (Stereo: 2) */ 00138 #define DEFAULT_AUDIO_IN_FREQ 32000 00139 #define DEFAULT_AUDIO_IN_BIT_RESOLUTION 16 00140 #define DEFAULT_AUDIO_IN_CHANNEL_NBR 1 /* Mono = 1, Stereo = 2 */ 00141 #define DEFAULT_AUDIO_IN_VOLUME 64 00142 00143 /* PDM buffer input size */ 00144 #define INTERNAL_BUFF_SIZE 128*DEFAULT_AUDIO_IN_FREQ/16000*DEFAULT_AUDIO_IN_CHANNEL_NBR 00145 00146 /*------------------------------------------------------------------------------ 00147 OPTIONAL Configuration defines parameters 00148 ------------------------------------------------------------------------------*/ 00149 00150 00151 /** 00152 * @} 00153 */ 00154 00155 /** @defgroup STM32L152D_EVAL_AUDIO_Exported_Variables Exported Variables 00156 * @{ 00157 */ 00158 extern __IO uint16_t AudioInVolume; 00159 /** 00160 * @} 00161 */ 00162 00163 /** @defgroup STM32L152D_EVAL_AUDIO_Exported_Macros Exported Macros 00164 * @{ 00165 */ 00166 #define DMA_MAX(_X_) (((_X_) <= DMA_MAX_SZE)? (_X_):DMA_MAX_SZE) 00167 00168 /** 00169 * @} 00170 */ 00171 00172 /** @addtogroup STM32L152D_EVAL_AUDIO_Exported_Functions 00173 * @{ 00174 */ 00175 00176 /** @addtogroup STM32L152D_EVAL_AUDIO_OUT_Exported_Functions 00177 * @{ 00178 */ 00179 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00180 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size); 00181 uint8_t BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00182 uint8_t BSP_AUDIO_OUT_Pause(void); 00183 uint8_t BSP_AUDIO_OUT_Resume(void); 00184 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option); 00185 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume); 00186 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq); 00187 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Command); 00188 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output); 00189 00190 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00191 /* This function is called when the requested data has been completely transferred.*/ 00192 void BSP_AUDIO_OUT_TransferComplete_CallBack(void); 00193 00194 /* This function is called when half of the requested buffer has been transferred. */ 00195 void BSP_AUDIO_OUT_HalfTransfer_CallBack(void); 00196 00197 /* This function is called when an Interrupt due to transfer error on or peripheral 00198 error occurs. */ 00199 void BSP_AUDIO_OUT_Error_CallBack(void); 00200 00201 /** 00202 * @} 00203 */ 00204 00205 /** @addtogroup STM32L152D_EVAL_AUDIO_IN_Exported_Functions 00206 * @{ 00207 */ 00208 uint8_t BSP_AUDIO_IN_Init(uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr); 00209 uint8_t BSP_AUDIO_IN_Record(uint16_t *pData, uint32_t Size); 00210 uint8_t BSP_AUDIO_IN_Stop(void); 00211 uint8_t BSP_AUDIO_IN_Pause(void); 00212 uint8_t BSP_AUDIO_IN_Resume(void); 00213 uint8_t BSP_AUDIO_IN_SetVolume(uint8_t Volume); 00214 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00215 /* This function should be implemented by the user application. 00216 It is called into this driver when the current buffer is filled to prepare the next 00217 buffer pointer and its size. */ 00218 void BSP_AUDIO_IN_TransferComplete_CallBack(void); 00219 void BSP_AUDIO_IN_HalfTransfer_CallBack(void); 00220 00221 /* This function is called when an Interrupt due to transfer error on or peripheral 00222 error occurs. */ 00223 void BSP_AUDIO_IN_Error_Callback(void); 00224 00225 /** 00226 * @} 00227 */ 00228 00229 /** 00230 * @} 00231 */ 00232 00233 /** 00234 * @} 00235 */ 00236 00237 /** 00238 * @} 00239 */ 00240 00241 /** 00242 * @} 00243 */ 00244 00245 #ifdef __cplusplus 00246 } 00247 #endif 00248 00249 #endif /* __STM32L152D_EVAL_AUDIO_H */ 00250 00251 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Aug 24 2017 17:57:47 for STM32L152D_EVAL BSP User Manual by
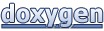