STM32L152D_EVAL BSP User Manual
|
stm32l152d_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152d_eval.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage Leds, 00006 * push-button and COM ports 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l152d_eval.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @defgroup STM32L152D_EVAL STM32L152D-EVAL 00045 * @{ 00046 */ 00047 00048 /** @defgroup STM32L152D_EVAL_Common STM32L152D-EVAL Common 00049 * @{ 00050 */ 00051 00052 /** @defgroup STM32L152D_EVAL_Private_TypesDefinitions Private Types Definitions 00053 * @{ 00054 */ 00055 00056 typedef struct 00057 { 00058 __IO uint16_t LCD_REG_R; /* Read Register */ 00059 __IO uint16_t LCD_RAM_R; /* Read RAM */ 00060 __IO uint16_t LCD_REG_W; /* Write Register */ 00061 __IO uint16_t LCD_RAM_W; /* Write RAM */ 00062 } TFT_LCD_TypeDef; 00063 00064 /** 00065 * @} 00066 */ 00067 00068 /** @defgroup STM32L152D_EVAL_Private_Defines Private Defines 00069 * @{ 00070 */ 00071 00072 /* LINK EEPROM SPI */ 00073 #define EEPROM_CMD_WREN 0x06 /*!< Write enable instruction */ 00074 #define EEPROM_CMD_WRDI 0x04 /*!< Write disable instruction */ 00075 #define EEPROM_CMD_RDSR 0x05 /*!< Read Status Register instruction */ 00076 #define EEPROM_CMD_WRSR 0x01 /*!< Write Status Register instruction */ 00077 #define EEPROM_CMD_WRITE 0x02 /*!< Write to Memory instruction */ 00078 #define EEPROM_CMD_READ 0x03 /*!< Read from Memory instruction */ 00079 00080 #define EEPROM_WIP_FLAG 0x01 /*!< Write In Progress (WIP) flag */ 00081 00082 /** 00083 * @brief STM32L152D EVAL BSP Driver version number 00084 */ 00085 #define __STM32L152D_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00086 #define __STM32L152D_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00087 #define __STM32L152D_EVAL_BSP_VERSION_SUB2 (0x07) /*!< [15:8] sub2 version */ 00088 #define __STM32L152D_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00089 #define __STM32L152D_EVAL_BSP_VERSION ((__STM32L152D_EVAL_BSP_VERSION_MAIN << 24)\ 00090 |(__STM32L152D_EVAL_BSP_VERSION_SUB1 << 16)\ 00091 |(__STM32L152D_EVAL_BSP_VERSION_SUB2 << 8 )\ 00092 |(__STM32L152D_EVAL_BSP_VERSION_RC)) 00093 00094 00095 /* Note: LCD /CS is CE4 - Bank 4 of NOR/SRAM Bank 1~4 */ 00096 #define TFT_LCD_BASE ((uint32_t)(0x60000000 | 0x0C000000)) 00097 #define TFT_LCD ((TFT_LCD_TypeDef *) TFT_LCD_BASE) 00098 00099 /** 00100 * @} 00101 */ 00102 00103 00104 /** @defgroup STM32L152D_EVAL_Private_Variables Private Variables 00105 * @{ 00106 */ 00107 /** 00108 * @brief LED variables 00109 */ 00110 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00111 LED2_GPIO_PORT, 00112 LED3_GPIO_PORT, 00113 LED4_GPIO_PORT}; 00114 00115 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00116 LED2_PIN, 00117 LED3_PIN, 00118 LED4_PIN}; 00119 00120 /** 00121 * @brief BUTTON variables 00122 */ 00123 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {TAMPER_BUTTON_GPIO_PORT, 00124 SEL_JOY_GPIO_PORT, 00125 LEFT_JOY_GPIO_PORT, 00126 RIGHT_JOY_GPIO_PORT, 00127 DOWN_JOY_GPIO_PORT, 00128 UP_JOY_GPIO_PORT}; 00129 00130 const uint16_t BUTTON_PIN[BUTTONn] = {TAMPER_BUTTON_PIN, 00131 SEL_JOY_PIN, 00132 LEFT_JOY_PIN, 00133 RIGHT_JOY_PIN, 00134 DOWN_JOY_PIN, 00135 UP_JOY_PIN}; 00136 00137 const uint8_t BUTTON_IRQn[BUTTONn] = {TAMPER_BUTTON_EXTI_IRQn, 00138 SEL_JOY_EXTI_IRQn, 00139 LEFT_JOY_EXTI_IRQn, 00140 RIGHT_JOY_EXTI_IRQn, 00141 DOWN_JOY_EXTI_IRQn, 00142 UP_JOY_EXTI_IRQn}; 00143 00144 /** 00145 * @brief JOYSTICK variables 00146 */ 00147 GPIO_TypeDef* JOY_PORT[JOYn] = {SEL_JOY_GPIO_PORT, 00148 LEFT_JOY_GPIO_PORT, 00149 RIGHT_JOY_GPIO_PORT, 00150 DOWN_JOY_GPIO_PORT, 00151 UP_JOY_GPIO_PORT}; 00152 00153 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00154 LEFT_JOY_PIN, 00155 RIGHT_JOY_PIN, 00156 DOWN_JOY_PIN, 00157 UP_JOY_PIN}; 00158 00159 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00160 LEFT_JOY_EXTI_IRQn, 00161 RIGHT_JOY_EXTI_IRQn, 00162 DOWN_JOY_EXTI_IRQn, 00163 UP_JOY_EXTI_IRQn}; 00164 00165 /** 00166 * @brief COM variables 00167 */ 00168 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00169 00170 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00171 00172 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00173 00174 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00175 00176 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00177 00178 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00179 00180 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00181 00182 /** 00183 * @brief BUS variables 00184 */ 00185 #ifdef HAL_SPI_MODULE_ENABLED 00186 uint32_t SpixTimeout = EVAL_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00187 static SPI_HandleTypeDef heval_Spi; 00188 #endif /* HAL_SPI_MODULE_ENABLED */ 00189 00190 #ifdef HAL_I2C_MODULE_ENABLED 00191 uint32_t I2cxTimeout = EVAL_I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00192 I2C_HandleTypeDef heval_I2c; 00193 #endif /* HAL_I2C_MODULE_ENABLED */ 00194 00195 /** 00196 * @} 00197 */ 00198 00199 #if defined(HAL_SRAM_MODULE_ENABLED) 00200 00201 static void FSMC_BANK4_WriteData(uint16_t Data); 00202 static void FSMC_BANK4_WriteReg(uint8_t Reg); 00203 static uint16_t FSMC_BANK4_ReadData(void); 00204 static void FSMC_BANK4_Init(void); 00205 static void FSMC_BANK4_MspInit(void); 00206 00207 /* LCD IO functions */ 00208 void LCD_IO_Init(void); 00209 void LCD_IO_WriteData(uint16_t RegValue); 00210 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00211 void LCD_IO_WriteReg(uint8_t Reg); 00212 uint16_t LCD_IO_ReadData(uint16_t Reg); 00213 void LCD_Delay (uint32_t delay); 00214 #endif /*HAL_SRAM_MODULE_ENABLED*/ 00215 00216 /* I2Cx bus function */ 00217 #ifdef HAL_I2C_MODULE_ENABLED 00218 /* Link function for I2C EEPROM peripheral */ 00219 static void I2Cx_Init(void); 00220 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value); 00221 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00222 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg); 00223 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00224 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00225 static void I2Cx_Error (void); 00226 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c); 00227 00228 /* Link function for EEPROM peripheral over I2C */ 00229 void EEPROM_I2C_IO_Init(void); 00230 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00231 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00232 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00233 00234 /* Link functions for Temperature Sensor peripheral */ 00235 void TSENSOR_IO_Init(void); 00236 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length); 00237 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length); 00238 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00239 00240 /* Link function for Audio peripheral */ 00241 void AUDIO_IO_Init(void); 00242 void AUDIO_IO_DeInit(void); 00243 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00244 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00245 00246 #endif /* HAL_I2C_MODULE_ENABLED */ 00247 00248 /* SPIx bus function */ 00249 #ifdef HAL_SPI_MODULE_ENABLED 00250 static void SPIx_Init(void); 00251 static void SPIx_Write(uint8_t Value); 00252 static uint32_t SPIx_Read(void); 00253 static void SPIx_Error (void); 00254 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00255 00256 /* Link function for EEPROM peripheral over SPI */ 00257 void EEPROM_SPI_IO_Init(void); 00258 void EEPROM_SPI_IO_WriteByte(uint8_t Data); 00259 uint8_t EEPROM_SPI_IO_ReadByte(void); 00260 HAL_StatusTypeDef EEPROM_SPI_IO_WriteData(uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00261 HAL_StatusTypeDef EEPROM_SPI_IO_ReadData(uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00262 HAL_StatusTypeDef EEPROM_SPI_IO_WaitEepromStandbyState(void); 00263 00264 #endif /* HAL_SPI_MODULE_ENABLED */ 00265 00266 00267 /** @defgroup STM32L152D_EVAL_Exported_Functions Exported Functions 00268 * @{ 00269 */ 00270 00271 /** 00272 * @brief This method returns the STM32L152D EVAL BSP Driver revision 00273 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00274 */ 00275 uint32_t BSP_GetVersion(void) 00276 { 00277 return __STM32L152D_EVAL_BSP_VERSION; 00278 } 00279 00280 /** 00281 * @brief Configures LED GPIO. 00282 * @param Led: Specifies the Led to be configured. 00283 * This parameter can be one of following parameters: 00284 * @arg LED1 00285 * @arg LED2 00286 * @arg LED3 00287 * @arg LED4 00288 * @retval None 00289 */ 00290 void BSP_LED_Init(Led_TypeDef Led) 00291 { 00292 GPIO_InitTypeDef gpioinitstruct = {0}; 00293 00294 /* Enable the GPIO_LED clock */ 00295 LEDx_GPIO_CLK_ENABLE(Led); 00296 00297 /* Configure the GPIO_LED pin */ 00298 gpioinitstruct.Pin = LED_PIN[Led]; 00299 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00300 gpioinitstruct.Pull = GPIO_NOPULL; 00301 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00302 00303 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00304 00305 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00306 } 00307 00308 /** 00309 * @brief Turns selected LED On. 00310 * @param Led: Specifies the Led to be set on. 00311 * This parameter can be one of following parameters: 00312 * @arg LED1 00313 * @arg LED2 00314 * @arg LED3 00315 * @arg LED4 00316 * @retval None 00317 */ 00318 void BSP_LED_On(Led_TypeDef Led) 00319 { 00320 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00321 } 00322 00323 /** 00324 * @brief Turns selected LED Off. 00325 * @param Led: Specifies the Led to be set off. 00326 * This parameter can be one of following parameters: 00327 * @arg LED1 00328 * @arg LED2 00329 * @arg LED3 00330 * @arg LED4 00331 * @retval None 00332 */ 00333 void BSP_LED_Off(Led_TypeDef Led) 00334 { 00335 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00336 } 00337 00338 /** 00339 * @brief Toggles the selected LED. 00340 * @param Led: Specifies the Led to be toggled. 00341 * This parameter can be one of following parameters: 00342 * @arg LED1 00343 * @arg LED2 00344 * @arg LED3 00345 * @arg LED4 00346 * @retval None 00347 */ 00348 void BSP_LED_Toggle(Led_TypeDef Led) 00349 { 00350 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00351 } 00352 00353 /** 00354 * @brief Configures push button GPIO and EXTI Line. 00355 * @param Button: Button to be configured. 00356 * This parameter can be one of the following values: 00357 * @arg BUTTON_TAMPER: Key/Tamper Push Button 00358 * @arg BUTTON_SEL : Sel Push Button on Joystick 00359 * @arg BUTTON_LEFT : Left Push Button on Joystick 00360 * @arg BUTTON_RIGHT : Right Push Button on Joystick 00361 * @arg BUTTON_DOWN : Down Push Button on Joystick 00362 * @arg BUTTON_UP : Up Push Button on Joystick 00363 * @param Button_Mode: Button mode requested. 00364 * This parameter can be one of the following values: 00365 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00366 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00367 * with interrupt generation capability 00368 * @retval None 00369 */ 00370 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00371 { 00372 GPIO_InitTypeDef gpioinitstruct = {0}; 00373 00374 /* Enable the corresponding Push Button clock */ 00375 BUTTONx_GPIO_CLK_ENABLE(Button); 00376 00377 /* Configure Push Button pin as input */ 00378 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00379 gpioinitstruct.Pull = GPIO_NOPULL; 00380 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00381 00382 if (Button_Mode == BUTTON_MODE_GPIO) 00383 { 00384 /* Configure Button pin as input */ 00385 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00386 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00387 } 00388 else if (Button_Mode == BUTTON_MODE_EXTI) 00389 { 00390 if(Button != BUTTON_TAMPER) 00391 { 00392 /* Configure Joystick Button pin as input with External interrupt, falling edge */ 00393 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00394 } 00395 else 00396 { 00397 /* Configure Key Push Button pin as input with External interrupt, rising edge */ 00398 gpioinitstruct.Mode = GPIO_MODE_IT_RISING; 00399 } 00400 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00401 00402 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00403 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00404 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00405 } 00406 } 00407 00408 /** 00409 * @brief Returns the selected button state. 00410 * @param Button: Button to be checked. 00411 * This parameter can be one of the following values: 00412 * @arg BUTTON_TAMPER: Key/Tamper Push Button 00413 * @retval Button state 00414 */ 00415 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00416 { 00417 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00418 } 00419 00420 /** 00421 * @brief Configures all button of the joystick in GPIO or EXTI modes. 00422 * @param Joy_Mode: Joystick mode. 00423 * This parameter can be one of the following values: 00424 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00425 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00426 * with interrupt generation capability 00427 * @retval HAL_OK: if all initializations are OK. Other value if error. 00428 */ 00429 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00430 { 00431 JOYState_TypeDef joykey = JOY_NONE; 00432 GPIO_InitTypeDef gpioinitstruct = {0}; 00433 00434 /* Initialized the Joystick. */ 00435 for(joykey = JOY_SEL; joykey < (JOY_SEL+JOYn) ; joykey++) 00436 { 00437 /* Enable the JOY clock */ 00438 JOYx_GPIO_CLK_ENABLE(joykey); 00439 00440 gpioinitstruct.Pin = JOY_PIN[joykey]; 00441 gpioinitstruct.Pull = GPIO_NOPULL; 00442 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00443 00444 if (Joy_Mode == JOY_MODE_GPIO) 00445 { 00446 /* Configure Joy pin as input */ 00447 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00448 HAL_GPIO_Init(JOY_PORT[joykey], &gpioinitstruct); 00449 } 00450 00451 if (Joy_Mode == JOY_MODE_EXTI) 00452 { 00453 /* Configure Joy pin as input with External interrupt */ 00454 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00455 HAL_GPIO_Init(JOY_PORT[joykey], &gpioinitstruct); 00456 00457 /* Enable and set Joy EXTI Interrupt to the lowest priority */ 00458 HAL_NVIC_SetPriority((IRQn_Type)(JOY_IRQn[joykey]), 0x0F, 0); 00459 HAL_NVIC_EnableIRQ((IRQn_Type)(JOY_IRQn[joykey])); 00460 } 00461 } 00462 00463 return HAL_OK; 00464 } 00465 00466 /** 00467 * @brief Returns the current joystick status. 00468 * @retval Code of the joystick key pressed 00469 * This code can be one of the following values: 00470 * @arg JOY_SEL 00471 * @arg JOY_DOWN 00472 * @arg JOY_LEFT 00473 * @arg JOY_RIGHT 00474 * @arg JOY_UP 00475 * @arg JOY_NONE 00476 */ 00477 JOYState_TypeDef BSP_JOY_GetState(void) 00478 { 00479 JOYState_TypeDef joykey = JOY_NONE; 00480 00481 for(joykey = JOY_SEL; joykey < (JOY_SEL+JOYn) ; joykey++) 00482 { 00483 if(HAL_GPIO_ReadPin(JOY_PORT[joykey], JOY_PIN[joykey]) == GPIO_PIN_RESET) 00484 { 00485 /* Return Code Joystick key pressed */ 00486 return joykey; 00487 } 00488 } 00489 00490 /* No Joystick key pressed */ 00491 return JOY_NONE; 00492 } 00493 00494 #ifdef HAL_UART_MODULE_ENABLED 00495 /** 00496 * @brief Configures COM port. 00497 * @param COM: Specifies the COM port to be configured. 00498 * This parameter can be one of following parameters: 00499 * @arg COM1 00500 * @param huart: pointer to a UART_HandleTypeDef structure that 00501 * contains the configuration information for the specified UART peripheral. 00502 * @retval None 00503 */ 00504 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00505 { 00506 GPIO_InitTypeDef gpioinitstruct = {0}; 00507 00508 /* Enable GPIO clock */ 00509 COMx_TX_GPIO_CLK_ENABLE(COM); 00510 COMx_RX_GPIO_CLK_ENABLE(COM); 00511 00512 /* Enable USART clock */ 00513 COMx_CLK_ENABLE(COM); 00514 00515 /* Configure USART Tx as alternate function push-pull */ 00516 gpioinitstruct.Pin = COM_TX_PIN[COM]; 00517 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00518 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00519 gpioinitstruct.Pull = GPIO_PULLUP; 00520 gpioinitstruct.Alternate = COM_TX_AF[COM]; 00521 HAL_GPIO_Init(COM_TX_PORT[COM], &gpioinitstruct); 00522 00523 /* Configure USART Rx as alternate function push-pull */ 00524 gpioinitstruct.Pin = COM_RX_PIN[COM]; 00525 gpioinitstruct.Alternate = COM_RX_AF[COM]; 00526 HAL_GPIO_Init(COM_RX_PORT[COM], &gpioinitstruct); 00527 00528 /* USART configuration */ 00529 huart->Instance = COM_USART[COM]; 00530 HAL_UART_Init(huart); 00531 } 00532 #endif /* HAL_UART_MODULE_ENABLED */ 00533 00534 /** 00535 * @} 00536 */ 00537 00538 /** @defgroup STM32L152D_EVAL_BusOperations_Functions Bus Operations Functions 00539 * @{ 00540 */ 00541 00542 /******************************************************************************* 00543 BUS OPERATIONS 00544 *******************************************************************************/ 00545 00546 /*************************** FSMC Routines ************************************/ 00547 #if defined(HAL_SRAM_MODULE_ENABLED) 00548 /** 00549 * @brief Initializes FSMC_BANK4 MSP. 00550 * @retval None 00551 */ 00552 static void FSMC_BANK4_MspInit(void) 00553 { 00554 GPIO_InitTypeDef gpioinitstruct = {0}; 00555 00556 /* Enable FMC clock */ 00557 __HAL_RCC_FSMC_CLK_ENABLE(); 00558 00559 /* Enable GPIOs clock */ 00560 __HAL_RCC_GPIOD_CLK_ENABLE(); 00561 __HAL_RCC_GPIOE_CLK_ENABLE(); 00562 __HAL_RCC_GPIOF_CLK_ENABLE(); 00563 __HAL_RCC_GPIOG_CLK_ENABLE(); 00564 00565 /* Common GPIO configuration */ 00566 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00567 gpioinitstruct.Pull = GPIO_PULLUP; 00568 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00569 gpioinitstruct.Alternate = GPIO_AF12_FSMC; 00570 00571 /* GPIOD configuration */ 00572 /* Set PD.00(D2), PD.01(D3), PD.04(NOE), PD.05(NWE), PD.08(D13), PD.09(D14), 00573 PD.10(D15), PD.14(D0), PD.15(D1) as alternate function push pull */ 00574 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | 00575 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_14 | 00576 GPIO_PIN_15; 00577 00578 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00579 00580 /* GPIOE configuration */ 00581 /* Set PE.07(D4), PE.08(D5), PE.09(D6), PE.10(D7), PE.11(D8), PE.12(D9), PE.13(D10), 00582 PE.14(D11), PE.15(D12) as alternate function push pull */ 00583 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00584 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | 00585 GPIO_PIN_15; 00586 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00587 00588 /* GPIOF configuration */ 00589 /* Set PF.00(A0 (RS)) and PF.01 (A1 (Level Shifter Direction) as alternate 00590 function push pull */ 00591 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1; 00592 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00593 00594 /* GPIOG configuration */ 00595 /* Set PG.12(NE4 (LCD/CS)) as alternate function push pull - NE4(LCD /CS) */ 00596 gpioinitstruct.Pin = GPIO_PIN_12; 00597 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00598 } 00599 00600 /** 00601 * @brief Initializes LCD IO. 00602 * @retval None 00603 */ 00604 static void FSMC_BANK4_Init(void) 00605 { 00606 SRAM_HandleTypeDef hsram; 00607 FSMC_NORSRAM_TimingTypeDef sramtiming = {0}; 00608 00609 /*** Configure the SRAM Bank 4 ***/ 00610 /* Configure IPs */ 00611 hsram.Instance = FSMC_NORSRAM_DEVICE; 00612 hsram.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00613 00614 sramtiming.AddressSetupTime = 4; 00615 sramtiming.AddressHoldTime = 3; 00616 sramtiming.DataSetupTime = 7; 00617 sramtiming.BusTurnAroundDuration = 1; 00618 sramtiming.CLKDivision = 2; 00619 sramtiming.DataLatency = 2; 00620 sramtiming.AccessMode = FSMC_ACCESS_MODE_A; 00621 00622 hsram.Init.NSBank = FSMC_NORSRAM_BANK4; 00623 hsram.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00624 hsram.Init.MemoryType = FSMC_MEMORY_TYPE_SRAM; 00625 hsram.Init.MemoryDataWidth = FSMC_NORSRAM_MEM_BUS_WIDTH_16; 00626 hsram.Init.BurstAccessMode = FSMC_BURST_ACCESS_MODE_DISABLE; 00627 hsram.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00628 hsram.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00629 hsram.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00630 hsram.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00631 hsram.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00632 hsram.Init.ExtendedMode = FSMC_EXTENDED_MODE_DISABLE; 00633 hsram.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00634 hsram.Init.WriteBurst = FSMC_WRITE_BURST_DISABLE; 00635 00636 /* Initialize the SRAM controller */ 00637 FSMC_BANK4_MspInit(); 00638 HAL_SRAM_Init(&hsram, &sramtiming, &sramtiming); 00639 } 00640 00641 /** 00642 * @brief Writes register value. 00643 * @param Data: 00644 * @retval None 00645 */ 00646 static void FSMC_BANK4_WriteData(uint16_t Data) 00647 { 00648 /* Write 16-bit Data */ 00649 TFT_LCD->LCD_RAM_W = Data; 00650 } 00651 00652 /** 00653 * @brief Writes register address. 00654 * @param Reg: 00655 * @retval None 00656 */ 00657 static void FSMC_BANK4_WriteReg(uint8_t Reg) 00658 { 00659 /* Write 16-bit Index, then Write Reg */ 00660 TFT_LCD->LCD_REG_W = Reg; 00661 } 00662 00663 /** 00664 * @brief Reads register value. 00665 * @retval Read value 00666 */ 00667 static uint16_t FSMC_BANK4_ReadData(void) 00668 { 00669 /* Read 16-bit Reg */ 00670 return (TFT_LCD->LCD_RAM_R); 00671 } 00672 #endif /*HAL_SRAM_MODULE_ENABLED*/ 00673 00674 #ifdef HAL_I2C_MODULE_ENABLED 00675 /******************************* I2C Routines**********************************/ 00676 00677 /** 00678 * @brief Eval I2Cx MSP Initialization 00679 * @param hi2c: I2C handle 00680 * @retval None 00681 */ 00682 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c) 00683 { 00684 GPIO_InitTypeDef gpioinitstruct = {0}; 00685 00686 if (hi2c->Instance == EVAL_I2Cx) 00687 { 00688 /*## Configure the GPIOs ################################################*/ 00689 00690 /* Enable GPIO clock */ 00691 EVAL_I2Cx_SDA_GPIO_CLK_ENABLE(); 00692 EVAL_I2Cx_SCL_GPIO_CLK_ENABLE(); 00693 00694 /* Configure I2C Tx as alternate function */ 00695 gpioinitstruct.Pin = EVAL_I2Cx_SCL_PIN; 00696 gpioinitstruct.Mode = GPIO_MODE_AF_OD; 00697 gpioinitstruct.Pull = GPIO_NOPULL; 00698 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00699 gpioinitstruct.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00700 HAL_GPIO_Init(EVAL_I2Cx_SCL_GPIO_PORT, &gpioinitstruct); 00701 00702 /* Configure I2C Rx as alternate function */ 00703 gpioinitstruct.Pin = EVAL_I2Cx_SDA_PIN; 00704 HAL_GPIO_Init(EVAL_I2Cx_SDA_GPIO_PORT, &gpioinitstruct); 00705 00706 00707 /*## Configure the Eval I2Cx peripheral #######################################*/ 00708 /* Enable Eval_I2Cx clock */ 00709 EVAL_I2Cx_CLK_ENABLE(); 00710 00711 /* Force the I2C Periheral Clock Reset */ 00712 EVAL_I2Cx_FORCE_RESET(); 00713 00714 /* Release the I2C Periheral Clock Reset */ 00715 EVAL_I2Cx_RELEASE_RESET(); 00716 00717 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00718 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 5, 0); 00719 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00720 00721 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00722 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 5, 0); 00723 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00724 } 00725 } 00726 00727 /** 00728 * @brief Eval I2Cx Bus initialization 00729 * @retval None 00730 */ 00731 static void I2Cx_Init(void) 00732 { 00733 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00734 { 00735 heval_I2c.Instance = EVAL_I2Cx; 00736 heval_I2c.Init.ClockSpeed = EVAL_I2C_SPEED; 00737 heval_I2c.Init.DutyCycle = I2C_DUTYCYCLE_2; 00738 heval_I2c.Init.OwnAddress1 = 0; 00739 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00740 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00741 heval_I2c.Init.OwnAddress2 = 0; 00742 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00743 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00744 00745 /* Init the I2C */ 00746 I2Cx_MspInit(&heval_I2c); 00747 HAL_I2C_Init(&heval_I2c); 00748 } 00749 } 00750 00751 /** 00752 * @brief Write a value in a register of the device through BUS. 00753 * @param Addr: Device address on BUS Bus. 00754 * @param Reg: The target register address to write 00755 * @param Value: The target register value to be written 00756 * @retval None 00757 */ 00758 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value) 00759 { 00760 HAL_StatusTypeDef status = HAL_OK; 00761 00762 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2cxTimeout); 00763 00764 /* Check the communication status */ 00765 if(status != HAL_OK) 00766 { 00767 /* Execute user timeout callback */ 00768 I2Cx_Error(); 00769 } 00770 } 00771 00772 /** 00773 * @brief Write a value in a register of the device through BUS. 00774 * @param Addr: Device address on BUS Bus. 00775 * @param Reg: The target register address to write 00776 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00777 * @param pBuffer: The target register value to be written 00778 * @param Length: buffer size to be written 00779 * @retval None 00780 */ 00781 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00782 { 00783 HAL_StatusTypeDef status = HAL_OK; 00784 00785 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00786 00787 /* Check the communication status */ 00788 if(status != HAL_OK) 00789 { 00790 /* Re-Initiaize the BUS */ 00791 I2Cx_Error(); 00792 } 00793 return status; 00794 } 00795 00796 /** 00797 * @brief Read a value in a register of the device through BUS. 00798 * @param Addr: Device address on BUS Bus. 00799 * @param Reg: The target register address to write 00800 * @retval Data read at register @ 00801 */ 00802 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg) 00803 { 00804 HAL_StatusTypeDef status = HAL_OK; 00805 uint8_t value = 0; 00806 00807 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &value, 1, I2cxTimeout); 00808 00809 /* Check the communication status */ 00810 if(status != HAL_OK) 00811 { 00812 /* Execute user timeout callback */ 00813 I2Cx_Error(); 00814 00815 } 00816 return value; 00817 } 00818 00819 /** 00820 * @brief Reads multiple data on the BUS. 00821 * @param Addr: I2C Address 00822 * @param Reg: Reg Address 00823 * @param RegSize : The target register size (can be 8BIT or 16BIT) 00824 * @param pBuffer: pointer to read data buffer 00825 * @param Length: length of the data 00826 * @retval 0 if no problems to read multiple data 00827 */ 00828 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00829 { 00830 HAL_StatusTypeDef status = HAL_OK; 00831 00832 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00833 00834 /* Check the communication status */ 00835 if(status != HAL_OK) 00836 { 00837 /* Re-Initiaize the BUS */ 00838 I2Cx_Error(); 00839 } 00840 return status; 00841 } 00842 00843 /** 00844 * @brief Checks if target device is ready for communication. 00845 * @note This function is used with Memory devices 00846 * @param DevAddress: Target device address 00847 * @param Trials: Number of trials 00848 * @retval HAL status 00849 */ 00850 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00851 { 00852 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, I2cxTimeout)); 00853 } 00854 00855 00856 /** 00857 * @brief Eval I2Cx error treatment function 00858 * @retval None 00859 */ 00860 static void I2Cx_Error (void) 00861 { 00862 /* De-initialize the I2C communication BUS */ 00863 HAL_I2C_DeInit(&heval_I2c); 00864 00865 /* Re- Initiaize the I2C communication BUS */ 00866 I2Cx_Init(); 00867 } 00868 00869 #endif /* HAL_I2C_MODULE_ENABLED */ 00870 00871 /******************************* SPI Routines**********************************/ 00872 #ifdef HAL_SPI_MODULE_ENABLED 00873 /** 00874 * @brief Initializes SPI MSP. 00875 * @retval None 00876 */ 00877 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00878 { 00879 GPIO_InitTypeDef gpioinitstruct = {0}; 00880 00881 /*** Configure the GPIOs ***/ 00882 /* Enable GPIO clock */ 00883 EVAL_SPIx_SCK_GPIO_CLK_ENABLE(); 00884 EVAL_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00885 00886 /* configure SPI SCK */ 00887 gpioinitstruct.Pin = EVAL_SPIx_SCK_PIN; 00888 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00889 gpioinitstruct.Pull = GPIO_NOPULL; 00890 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00891 gpioinitstruct.Alternate = EVAL_SPIx_SCK_AF; 00892 HAL_GPIO_Init(EVAL_SPIx_SCK_GPIO_PORT, &gpioinitstruct); 00893 00894 /* configure SPI MISO and MOSI */ 00895 gpioinitstruct.Pin = (EVAL_SPIx_MISO_PIN | EVAL_SPIx_MOSI_PIN); 00896 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00897 gpioinitstruct.Pull = GPIO_NOPULL; 00898 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00899 gpioinitstruct.Alternate = EVAL_SPIx_MISO_MOSI_AF; 00900 HAL_GPIO_Init(EVAL_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00901 00902 /*** Configure the SPI peripheral ***/ 00903 /* Enable SPI clock */ 00904 EVAL_SPIx_CLK_ENABLE(); 00905 } 00906 00907 /** 00908 * @brief Initializes SPI HAL. 00909 * @retval None 00910 */ 00911 static void SPIx_Init(void) 00912 { 00913 /* DeInitializes the SPI peripheral */ 00914 heval_Spi.Instance = EVAL_SPIx; 00915 HAL_SPI_DeInit(&heval_Spi); 00916 00917 /* SPI Config */ 00918 /* SPI baudrate is set to 32 MHz (PCLK2/SPI_BaudRatePrescaler = 32/2 = 16 MHz) */ 00919 heval_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_2; 00920 heval_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00921 heval_Spi.Init.CLKPhase = SPI_PHASE_1EDGE; 00922 heval_Spi.Init.CLKPolarity = SPI_POLARITY_LOW; 00923 heval_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00924 heval_Spi.Init.CRCPolynomial = 7; 00925 heval_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00926 heval_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00927 heval_Spi.Init.NSS = SPI_NSS_SOFT; 00928 heval_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00929 heval_Spi.Init.Mode = SPI_MODE_MASTER; 00930 00931 SPIx_MspInit(&heval_Spi); 00932 if (HAL_SPI_Init(&heval_Spi) != HAL_OK) 00933 { 00934 /* Should not occur */ 00935 while(1) {}; 00936 } 00937 } 00938 00939 /** 00940 * @brief SPI Read 4 bytes from device 00941 * @retval Read data 00942 */ 00943 static uint32_t SPIx_Read(void) 00944 { 00945 HAL_StatusTypeDef status = HAL_OK; 00946 uint32_t readvalue = 0; 00947 uint32_t writevalue = 0xFFFFFFFF; 00948 00949 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &writevalue, (uint8_t*) &readvalue, 1, SpixTimeout); 00950 00951 /* Check the communication status */ 00952 if(status != HAL_OK) 00953 { 00954 /* Execute user timeout callback */ 00955 SPIx_Error(); 00956 } 00957 00958 return readvalue; 00959 } 00960 00961 /** 00962 * @brief SPI Write a byte to device 00963 * @param Value: value to be written 00964 * @retval None 00965 */ 00966 static void SPIx_Write(uint8_t Value) 00967 { 00968 HAL_StatusTypeDef status = HAL_OK; 00969 00970 status = HAL_SPI_Transmit(&heval_Spi, (uint8_t*) &Value, 1, SpixTimeout); 00971 00972 /* Check the communication status */ 00973 if(status != HAL_OK) 00974 { 00975 /* Execute user timeout callback */ 00976 SPIx_Error(); 00977 } 00978 } 00979 00980 /** 00981 * @brief SPI error treatment function 00982 * @retval None 00983 */ 00984 static void SPIx_Error (void) 00985 { 00986 /* De-initialize the SPI communication BUS */ 00987 HAL_SPI_DeInit(&heval_Spi); 00988 00989 /* Re- Initiaize the SPI communication BUS */ 00990 SPIx_Init(); 00991 } 00992 #endif /* HAL_SPI_MODULE_ENABLED */ 00993 00994 /** 00995 * @} 00996 */ 00997 00998 /** @defgroup STM32L152D_EVAL_LinkOperations_Functions Link Operations Functions 00999 * @{ 01000 */ 01001 01002 /******************************************************************************* 01003 LINK OPERATIONS 01004 *******************************************************************************/ 01005 01006 #if defined(HAL_SRAM_MODULE_ENABLED) 01007 /********************************* LINK LCD ***********************************/ 01008 01009 /** 01010 * @brief Initializes LCD low level. 01011 * @retval None 01012 */ 01013 void LCD_IO_Init(void) 01014 { 01015 FSMC_BANK4_Init(); 01016 } 01017 01018 /** 01019 * @brief Writes data on LCD data register. 01020 * @param RegValue: Data to be written 01021 * @retval None 01022 */ 01023 void LCD_IO_WriteData(uint16_t RegValue) 01024 { 01025 FSMC_BANK4_WriteData(RegValue); 01026 } 01027 01028 /** 01029 * @brief Writes multiple data on LCD data register. 01030 * @param pData: Data to be written 01031 * @param Size: number of data to write 01032 * @retval None 01033 */ 01034 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 01035 { 01036 uint32_t index = 0; 01037 uint16_t data = 0; 01038 01039 for(index = 0; index < (Size / 2); index++) 01040 { 01041 data = *pData; 01042 pData++; 01043 data |= (*pData++ << 8); 01044 FSMC_BANK4_WriteData(data); 01045 } 01046 } 01047 01048 /** 01049 * @brief Writes register on LCD register. 01050 * @param Reg: Register to be written 01051 * @retval None 01052 */ 01053 void LCD_IO_WriteReg(uint8_t Reg) 01054 { 01055 FSMC_BANK4_WriteReg(Reg); 01056 } 01057 01058 /** 01059 * @brief Reads data from LCD data register. 01060 * @param Reg: Register to be read 01061 * @retval Read data. 01062 */ 01063 uint16_t LCD_IO_ReadData(uint16_t Reg) 01064 { 01065 FSMC_BANK4_WriteReg(Reg); 01066 01067 /* Read 16-bit Reg */ 01068 return (FSMC_BANK4_ReadData()); 01069 } 01070 01071 /** 01072 * @brief Wait for loop in ms. 01073 * @param Delay in ms. 01074 * @retval None 01075 */ 01076 void LCD_Delay (uint32_t Delay) 01077 { 01078 HAL_Delay(Delay); 01079 } 01080 01081 #endif /*HAL_SRAM_MODULE_ENABLED*/ 01082 01083 #ifdef HAL_SPI_MODULE_ENABLED 01084 /******************************** LINK EEPROM SPI ********************************/ 01085 01086 /** 01087 * @brief Initializes the EEPROM SPI and put it into StandBy State (Ready for 01088 * data transfer). 01089 * @retval None 01090 */ 01091 void EEPROM_SPI_IO_Init(void) 01092 { 01093 GPIO_InitTypeDef gpioinitstruct = {0}; 01094 01095 /* EEPROM_CS_GPIO Periph clock enable */ 01096 EEPROM_CS_GPIO_CLK_ENABLE(); 01097 01098 /* Configure EEPROM_CS_PIN pin: EEPROM SPI CS pin */ 01099 gpioinitstruct.Pin = EEPROM_CS_PIN; 01100 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 01101 gpioinitstruct.Pull = GPIO_PULLUP; 01102 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 01103 HAL_GPIO_Init(EEPROM_CS_GPIO_PORT, &gpioinitstruct); 01104 01105 /*------------Put EEPROM in SPI mode--------------*/ 01106 /* EEPROM SPI Config */ 01107 SPIx_Init(); 01108 01109 /* EEPROM chip select high */ 01110 EEPROM_CS_HIGH(); 01111 } 01112 01113 /** 01114 * @brief Write a byte on the EEPROM. 01115 * @param Data: byte to send. 01116 * @retval None 01117 */ 01118 void EEPROM_SPI_IO_WriteByte(uint8_t Data) 01119 { 01120 /* Send the byte */ 01121 SPIx_Write(Data); 01122 } 01123 01124 /** 01125 * @brief Read a byte from the EEPROM. 01126 * @retval uint8_t (The received byte). 01127 */ 01128 uint8_t EEPROM_SPI_IO_ReadByte(void) 01129 { 01130 uint8_t data = 0; 01131 01132 /* Get the received data */ 01133 data = SPIx_Read(); 01134 01135 /* Return the shifted data */ 01136 return data; 01137 } 01138 01139 /** 01140 * @brief Write data to SPI EEPROM driver 01141 * @param MemAddress: Internal memory address 01142 * @param pBuffer: Pointer to data buffer 01143 * @param BufferSize: Amount of data to be read 01144 * @retval HAL_StatusTypeDef HAL Status 01145 */ 01146 HAL_StatusTypeDef EEPROM_SPI_IO_WriteData(uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01147 { 01148 /*!< Enable the write access to the EEPROM */ 01149 /*!< Select the EEPROM: Chip Select low */ 01150 EEPROM_CS_LOW(); 01151 01152 /*!< Send "Write Enable" instruction */ 01153 SPIx_Write(EEPROM_CMD_WREN); 01154 01155 /*!< Deselect the EEPROM: Chip Select high */ 01156 EEPROM_CS_HIGH(); 01157 01158 /*!< Select the EEPROM: Chip Select low */ 01159 EEPROM_CS_LOW(); 01160 01161 /*!< Send "Write to Memory" instruction and MSB of MemAddress */ 01162 SPIx_Write(EEPROM_CMD_WRITE | (uint8_t)((MemAddress & 0x0100)>>5)); 01163 01164 /*!< Send MemAddress address byte to write to */ 01165 SPIx_Write(MemAddress & 0xFF); 01166 01167 /*!< while there is data to be written on the EEPROM */ 01168 while ((BufferSize)--) 01169 { 01170 /*!< Send the current byte */ 01171 SPIx_Write(*pBuffer); 01172 /*!< Point on the next byte to be written */ 01173 pBuffer++; 01174 } 01175 01176 /*!< Deselect the EEPROM: Chip Select high */ 01177 EEPROM_CS_HIGH(); 01178 01179 /*!< Wait the end of EEPROM writing */ 01180 EEPROM_SPI_IO_WaitEepromStandbyState(); 01181 01182 /*!< Disable the write access to the EEROM */ 01183 EEPROM_CS_LOW(); 01184 01185 /*!< Send "Write Disable" instruction */ 01186 SPIx_Write(EEPROM_CMD_WRDI); 01187 01188 /*!< Deselect the EEPROM: Chip Select high */ 01189 EEPROM_CS_HIGH(); 01190 01191 return HAL_OK; 01192 } 01193 01194 /** 01195 * @brief Read data from SPI EEPROM driver 01196 * @param MemAddress: Internal memory address 01197 * @param pBuffer: Pointer to data buffer 01198 * @param BufferSize: Amount of data to be read 01199 * @retval HAL_StatusTypeDef HAL Status 01200 */ 01201 HAL_StatusTypeDef EEPROM_SPI_IO_ReadData(uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01202 { 01203 /*!< Select the EEPROM: Chip Select low */ 01204 EEPROM_CS_LOW(); 01205 01206 /*!< Send "Read from Memory" instruction and MSB of MemAddress */ 01207 SPIx_Write(EEPROM_CMD_READ | (uint8_t)((MemAddress & 0x100)>>5)); 01208 01209 /*!< Send MemAddress address byte to read to*/ 01210 SPIx_Write(MemAddress & 0xFF); 01211 01212 while ((BufferSize)--) /*!< while there is data to be read */ 01213 { 01214 /*!< Read a byte from the EEPROM */ 01215 *pBuffer = SPIx_Read(); 01216 /*!< Point to the next location where the byte read will be saved */ 01217 pBuffer++; 01218 } 01219 01220 /*!< Deselect the EEPROM: Chip Select high */ 01221 EEPROM_CS_HIGH(); 01222 01223 return HAL_OK; 01224 } 01225 01226 /** 01227 * @brief Wait response from the SPI EEPROM 01228 * @retval HAL_StatusTypeDef HAL Status 01229 */ 01230 HAL_StatusTypeDef EEPROM_SPI_IO_WaitEepromStandbyState(void) 01231 { 01232 uint32_t timeout = 0xFFFF; 01233 uint32_t eepromstatus = 0; 01234 01235 /*!< Select the EEPROM: Chip Select low */ 01236 EEPROM_CS_LOW(); 01237 01238 /*!< Send "Read Status Register" instruction */ 01239 SPIx_Write(EEPROM_CMD_RDSR); 01240 01241 /*!< Loop as long as the memory is busy with a write cycle */ 01242 do 01243 { 01244 /*!< Send a dummy byte to generate the clock needed by the EEPROM 01245 and put the value of the status register in EEPROM Status variable */ 01246 eepromstatus = SPIx_Read(); 01247 timeout --; 01248 } 01249 while (((eepromstatus & EEPROM_WIP_FLAG) == SET) && timeout); /* Write in progress */ 01250 01251 /*!< Deselect the EEPROM: Chip Select high */ 01252 EEPROM_CS_HIGH(); 01253 01254 if ((eepromstatus & EEPROM_WIP_FLAG) != SET) 01255 { 01256 /* Right response got */ 01257 return HAL_OK; 01258 } 01259 else 01260 { 01261 /* After time out */ 01262 return HAL_TIMEOUT; 01263 } 01264 } 01265 #endif /* HAL_SPI_MODULE_ENABLED */ 01266 01267 #ifdef HAL_I2C_MODULE_ENABLED 01268 /********************************* LINK I2C EEPROM *****************************/ 01269 /** 01270 * @brief Initializes peripherals used by the I2C EEPROM driver. 01271 * @retval None 01272 */ 01273 void EEPROM_I2C_IO_Init(void) 01274 { 01275 I2Cx_Init(); 01276 } 01277 01278 /** 01279 * @brief Write data to I2C EEPROM driver 01280 * @param DevAddress: Target device address 01281 * @param MemAddress: Internal memory address 01282 * @param pBuffer: Pointer to data buffer 01283 * @param BufferSize: Amount of data to be sent 01284 * @retval HAL status 01285 */ 01286 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01287 { 01288 return (I2Cx_WriteBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01289 } 01290 01291 /** 01292 * @brief Read data from I2C EEPROM driver 01293 * @param DevAddress: Target device address 01294 * @param MemAddress: Internal memory address 01295 * @param pBuffer: Pointer to data buffer 01296 * @param BufferSize: Amount of data to be read 01297 * @retval HAL status 01298 */ 01299 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01300 { 01301 return (I2Cx_ReadBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01302 } 01303 01304 /** 01305 * @brief Checks if target device is ready for communication. 01306 * @note This function is used with Memory devices 01307 * @param DevAddress: Target device address 01308 * @param Trials: Number of trials 01309 * @retval HAL status 01310 */ 01311 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01312 { 01313 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01314 } 01315 01316 /********************************* LINK I2C TEMPERATURE SENSOR *****************************/ 01317 /** 01318 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 01319 * @retval None 01320 */ 01321 void TSENSOR_IO_Init(void) 01322 { 01323 I2Cx_Init(); 01324 } 01325 01326 /** 01327 * @brief Writes one byte to the TSENSOR. 01328 * @param DevAddress: Target device address 01329 * @param pBuffer: Pointer to data buffer 01330 * @param WriteAddr: TSENSOR's internal address to write to. 01331 * @param Length: Number of data to write 01332 * @retval None 01333 */ 01334 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length) 01335 { 01336 I2Cx_WriteBuffer(DevAddress, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01337 } 01338 01339 /** 01340 * @brief Reads one byte from the TSENSOR. 01341 * @param DevAddress: Target device address 01342 * @param pBuffer : pointer to the buffer that receives the data read from the TSENSOR. 01343 * @param ReadAddr : TSENSOR's internal address to read from. 01344 * @param Length: Number of data to read 01345 * @retval None 01346 */ 01347 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length) 01348 { 01349 I2Cx_ReadBuffer(DevAddress, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01350 } 01351 01352 /** 01353 * @brief Checks if Temperature Sensor is ready for communication. 01354 * @param DevAddress: Target device address 01355 * @param Trials: Number of trials 01356 * @retval HAL status 01357 */ 01358 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01359 { 01360 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01361 } 01362 01363 /********************************* LINK AUDIO ***********************************/ 01364 01365 /** 01366 * @brief Initializes Audio low level. 01367 * @retval None 01368 */ 01369 void AUDIO_IO_Init (void) 01370 { 01371 GPIO_InitTypeDef gpioinitstruct = {0}; 01372 01373 /* Enable Reset GPIO Clock */ 01374 AUDIO_RESET_GPIO_CLK_ENABLE(); 01375 01376 /* Audio reset pin configuration -------------------------------------------------*/ 01377 gpioinitstruct.Pin = AUDIO_RESET_PIN; 01378 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 01379 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 01380 gpioinitstruct.Pull = GPIO_NOPULL; 01381 HAL_GPIO_Init(AUDIO_RESET_GPIO, &gpioinitstruct); 01382 01383 I2Cx_Init(); 01384 01385 /* Power Down the codec */ 01386 HAL_GPIO_WritePin(AUDIO_RESET_GPIO, AUDIO_RESET_PIN, GPIO_PIN_RESET); 01387 01388 /* wait for a delay to insure registers erasing */ 01389 HAL_Delay(5); 01390 01391 /* Power on the codec */ 01392 HAL_GPIO_WritePin(AUDIO_RESET_GPIO, AUDIO_RESET_PIN, GPIO_PIN_SET); 01393 01394 /* wait for a delay to insure registers erasing */ 01395 HAL_Delay(5); 01396 } 01397 01398 /** 01399 * @brief DeInitializes Audio low level. 01400 * @note This function is intentionally kept empty, user should define it. 01401 */ 01402 void AUDIO_IO_DeInit(void) 01403 { 01404 01405 } 01406 01407 /** 01408 * @brief Writes a single data. 01409 * @param Addr: I2C address 01410 * @param Reg: Reg address 01411 * @param Value: Data to be written 01412 * @retval None 01413 */ 01414 void AUDIO_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) 01415 { 01416 I2Cx_WriteData(Addr, Reg, Value); 01417 } 01418 01419 /** 01420 * @brief Reads a single data. 01421 * @param Addr: I2C address 01422 * @param Reg: Reg address 01423 * @retval Data to be read 01424 */ 01425 uint8_t AUDIO_IO_Read (uint8_t Addr, uint8_t Reg) 01426 { 01427 return I2Cx_ReadData(Addr, Reg); 01428 } 01429 01430 #endif /* HAL_I2C_MODULE_ENABLED */ 01431 01432 /** 01433 * @} 01434 */ 01435 01436 /** 01437 * @} 01438 */ 01439 01440 /** 01441 * @} 01442 */ 01443 01444 /** 01445 * @} 01446 */ 01447 01448 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01449
Generated on Thu Aug 24 2017 17:57:47 for STM32L152D_EVAL BSP User Manual by
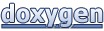