STM32F769I-Discovery BSP User Manual
|
stm32f769i_discovery.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_discovery.h 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file contains definitions for STM32F769I-Discovery LEDs, 00008 * push-buttons hardware resources. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32F769I_DISCOVERY_H 00041 #define __STM32F769I_DISCOVERY_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32f7xx_hal.h" 00050 00051 /** @addtogroup BSP 00052 * @{ 00053 */ 00054 00055 /** @addtogroup STM32F769I_DISCOVERY 00056 * @{ 00057 */ 00058 00059 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL STM32F769I-Discovery LOW LEVEL 00060 * @{ 00061 */ 00062 00063 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL_Exported_Types STM32F769I Discovery Low Level Exported Types 00064 * @{ 00065 */ 00066 00067 /** 00068 * @brief Define for STM32F769I_DISCOVERY board 00069 */ 00070 #if !defined (USE_STM32F769I_DISCO) 00071 #define USE_STM32F769I_DISCO 00072 #endif 00073 00074 /** @brief Led_TypeDef 00075 * STM32F769I_DISCOVERY board leds definitions. 00076 */ 00077 typedef enum 00078 { 00079 LED1 = 0, 00080 LED_RED = LED1, 00081 LED2 = 1, 00082 LED_GREEN = LED2 00083 } Led_TypeDef; 00084 00085 /** @brief Button_TypeDef 00086 * STM32F769I_DISCOVERY board Buttons definitions. 00087 */ 00088 typedef enum 00089 { 00090 BUTTON_WAKEUP = 0, 00091 } Button_TypeDef; 00092 00093 #define BUTTON_USER BUTTON_WAKEUP 00094 00095 /** @brief ButtonMode_TypeDef 00096 * STM32F769I_DISCOVERY board Buttons Modes definitions. 00097 */ 00098 typedef enum 00099 { 00100 BUTTON_MODE_GPIO = 0, 00101 BUTTON_MODE_EXTI = 1 00102 00103 } ButtonMode_TypeDef; 00104 00105 /** @addtogroup Exported_types 00106 * @{ 00107 */ 00108 typedef enum 00109 { 00110 PB_SET = 0, 00111 PB_RESET = !PB_SET 00112 } ButtonValue_TypeDef; 00113 00114 00115 /** @brief DISCO_Status_TypeDef 00116 * STM32F769I_DISCO board Status return possible values. 00117 */ 00118 typedef enum 00119 { 00120 DISCO_OK = 0, 00121 DISCO_ERROR = 1 00122 00123 } DISCO_Status_TypeDef; 00124 00125 /** 00126 * @} 00127 */ 00128 00129 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL_Exported_Constants STM32F769I Discovery Low Level Exported Constants 00130 * @{ 00131 */ 00132 00133 00134 /** @addtogroup STM32F769I_DISCOVERY_LOW_LEVEL_LED STM32F769I Discovery Low Level Led 00135 * @{ 00136 */ 00137 /* Always four leds for all revisions of Discovery boards */ 00138 #define LEDn ((uint8_t)2) 00139 00140 00141 /* 2 Leds are connected to MCU directly on PJ13 and PJ5 */ 00142 #define LED1_GPIO_PORT ((GPIO_TypeDef*)GPIOJ) 00143 #define LED2_GPIO_PORT ((GPIO_TypeDef*)GPIOJ) 00144 00145 #define LEDx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOJ_CLK_ENABLE() 00146 #define LEDx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOJ_CLK_DISABLE() 00147 00148 #define LED1_PIN ((uint32_t)GPIO_PIN_13) 00149 #define LED2_PIN ((uint32_t)GPIO_PIN_5) 00150 00151 /** 00152 * @} 00153 */ 00154 00155 /** @addtogroup STM32F769I_DISCOVERY_LOW_LEVEL_BUTTON STM32F769I Discovery Low Level Button 00156 * @{ 00157 */ 00158 /* Only one User/Wakeup button */ 00159 #define BUTTONn ((uint8_t)1) 00160 00161 /** 00162 * @brief Wakeup push-button 00163 */ 00164 #define WAKEUP_BUTTON_PIN GPIO_PIN_0 00165 #define WAKEUP_BUTTON_GPIO_PORT GPIOA 00166 #define WAKEUP_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00167 #define WAKEUP_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00168 #define WAKEUP_BUTTON_EXTI_IRQn EXTI0_IRQn 00169 00170 /* Define the USER button as an alias of the Wakeup button */ 00171 #define USER_BUTTON_PIN WAKEUP_BUTTON_PIN 00172 #define USER_BUTTON_GPIO_PORT WAKEUP_BUTTON_GPIO_PORT 00173 #define USER_BUTTON_GPIO_CLK_ENABLE() WAKEUP_BUTTON_GPIO_CLK_ENABLE() 00174 #define USER_BUTTON_GPIO_CLK_DISABLE() WAKEUP_BUTTON_GPIO_CLK_DISABLE() 00175 #define USER_BUTTON_EXTI_IRQn WAKEUP_BUTTON_EXTI_IRQn 00176 00177 #define BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00178 00179 /** 00180 * @} 00181 */ 00182 00183 /** 00184 * @brief USB OTG HS Over Current signal 00185 */ 00186 #define OTG_HS_OVER_CURRENT_PIN GPIO_PIN_4 00187 #define OTG_HS_OVER_CURRENT_PORT GPIOD 00188 #define OTG_HS_OVER_CURRENT_PORT_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00189 00190 /** 00191 * @brief SD-detect signal 00192 */ 00193 #define SD_DETECT_PIN ((uint32_t)GPIO_PIN_15) 00194 #define SD_DETECT_GPIO_PORT ((GPIO_TypeDef*)GPIOI) 00195 #define SD_DETECT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOI_CLK_ENABLE() 00196 #define SD_DETECT_GPIO_CLK_DISABLE() __HAL_RCC_GPIOI_CLK_DISABLE() 00197 #define SD_DETECT_EXTI_IRQn EXTI15_10_IRQn 00198 00199 /** 00200 * @brief Touch screen interrupt signal 00201 */ 00202 #define TS_INT_PIN ((uint32_t)GPIO_PIN_13) 00203 #define TS_INT_GPIO_PORT ((GPIO_TypeDef*)GPIOI) 00204 #define TS_INT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOI_CLK_ENABLE() 00205 #define TS_INT_GPIO_CLK_DISABLE() __HAL_RCC_GPIOI_CLK_DISABLE() 00206 #define TS_INT_EXTI_IRQn EXTI15_10_IRQn 00207 00208 /** 00209 * @brief TouchScreen FT6206 Slave I2C address 1 00210 */ 00211 #define TS_I2C_ADDRESS ((uint16_t)0x54) 00212 00213 /** 00214 * @brief TouchScreen FT6336G Slave I2C address 2 00215 */ 00216 #define TS_I2C_ADDRESS_A02 ((uint16_t)0x70) 00217 00218 /** 00219 * @brief Audio I2C Slave address 00220 */ 00221 #define AUDIO_I2C_ADDRESS ((uint16_t)0x34) 00222 00223 /** 00224 * @brief EEPROM I2C Slave address 1 00225 */ 00226 #define EEPROM_I2C_ADDRESS_A01 ((uint16_t)0xA0) 00227 00228 /** 00229 * @brief EEPROM I2C Slave address 2 00230 */ 00231 #define EEPROM_I2C_ADDRESS_A02 ((uint16_t)0xA6) 00232 00233 /** 00234 * @brief User can use this section to tailor I2C4/I2C4 instance used and associated 00235 * resources (audio codec). 00236 * Definition for I2C4 clock resources 00237 */ 00238 #define DISCOVERY_AUDIO_I2Cx I2C4 00239 #define DISCOVERY_AUDIO_I2Cx_CLK_ENABLE() __HAL_RCC_I2C4_CLK_ENABLE() 00240 #define DISCOVERY_AUDIO_I2Cx_SCL_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00241 #define DISCOVERY_AUDIO_I2Cx_SDA_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00242 00243 #define DISCOVERY_AUDIO_I2Cx_FORCE_RESET() __HAL_RCC_I2C4_FORCE_RESET() 00244 #define DISCOVERY_AUDIO_I2Cx_RELEASE_RESET() __HAL_RCC_I2C4_RELEASE_RESET() 00245 00246 /** @brief Definition for I2C4 Pins 00247 */ 00248 #define DISCOVERY_AUDIO_I2Cx_SCL_PIN GPIO_PIN_12 /*!< PD12 */ 00249 #define DISCOVERY_AUDIO_I2Cx_SCL_AF GPIO_AF4_I2C4 00250 #define DISCOVERY_AUDIO_I2Cx_SCL_GPIO_PORT GPIOD 00251 #define DISCOVERY_AUDIO_I2Cx_SDA_PIN GPIO_PIN_7 /*!< PB7 */ 00252 #define DISCOVERY_AUDIO_I2Cx_SDA_AF GPIO_AF11_I2C4 00253 #define DISCOVERY_AUDIO_I2Cx_SDA_GPIO_PORT GPIOB 00254 /** @brief Definition of I2C4 interrupt requests 00255 */ 00256 #define DISCOVERY_AUDIO_I2Cx_EV_IRQn I2C4_EV_IRQn 00257 #define DISCOVERY_AUDIO_I2Cx_ER_IRQn I2C4_ER_IRQn 00258 00259 /** 00260 * @brief User can use this section to tailor I2C1/I2C1 instance used and associated 00261 * resources. 00262 * Definition for I2C1 clock resources 00263 */ 00264 #define DISCOVERY_EXT_I2Cx I2C1 00265 #define DISCOVERY_EXT_I2Cx_CLK_ENABLE() __HAL_RCC_I2C1_CLK_ENABLE() 00266 #define DISCOVERY_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00267 #define DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00268 00269 #define DISCOVERY_EXT_I2Cx_FORCE_RESET() __HAL_RCC_I2C1_FORCE_RESET() 00270 #define DISCOVERY_EXT_I2Cx_RELEASE_RESET() __HAL_RCC_I2C1_RELEASE_RESET() 00271 00272 /** @brief Definition for I2C1 Pins 00273 */ 00274 #define DISCOVERY_EXT_I2Cx_SCL_PIN GPIO_PIN_8 /*!< PB8 */ 00275 #define DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT GPIOB 00276 #define DISCOVERY_EXT_I2Cx_SCL_SDA_AF GPIO_AF4_I2C1 00277 #define DISCOVERY_EXT_I2Cx_SDA_PIN GPIO_PIN_9 /*!< PB9 */ 00278 00279 /** @brief Definition of I2C interrupt requests 00280 */ 00281 #define DISCOVERY_EXT_I2Cx_EV_IRQn I2C1_EV_IRQn 00282 #define DISCOVERY_EXT_I2Cx_ER_IRQn I2C1_ER_IRQn 00283 00284 /* I2C TIMING Register define when I2C clock source is SYSCLK */ 00285 /* I2C TIMING is calculated from APB1 source clock = 50 MHz */ 00286 /* Due to the big MOFSET capacity for adapting the camera level the rising time is very large (>1us) */ 00287 /* 0x40912732 takes in account the big rising and aims a clock of 100khz */ 00288 #ifndef DISCOVERY_I2Cx_TIMING 00289 #define DISCOVERY_I2Cx_TIMING ((uint32_t)0x40912732) 00290 #endif /* DISCOVERY_I2Cx_TIMING */ 00291 00292 00293 /** 00294 * @} 00295 */ 00296 00297 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL_Exported_Macros STM32F769I Discovery Low Level Exported Macros 00298 * @{ 00299 */ 00300 /** 00301 * @} 00302 */ 00303 00304 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL_Exported_Functions STM32F769I Discovery Low Level Exported Functions 00305 * @{ 00306 */ 00307 uint32_t BSP_GetVersion(void); 00308 void BSP_LED_Init(Led_TypeDef Led); 00309 void BSP_LED_DeInit(Led_TypeDef Led); 00310 void BSP_LED_On(Led_TypeDef Led); 00311 void BSP_LED_Off(Led_TypeDef Led); 00312 void BSP_LED_Toggle(Led_TypeDef Led); 00313 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode); 00314 void BSP_PB_DeInit(Button_TypeDef Button); 00315 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00316 00317 /** 00318 * @} 00319 */ 00320 00321 /** 00322 * @} 00323 */ 00324 00325 /** 00326 * @} 00327 */ 00328 00329 /** 00330 * @} 00331 */ 00332 00333 /** 00334 * @} 00335 */ 00336 00337 00338 #ifdef __cplusplus 00339 } 00340 #endif 00341 00342 #endif /* __STM32F769I_DISCOVERY_H */ 00343 00344 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 18:30:07 for STM32F769I-Discovery BSP User Manual by
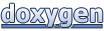