STM32F769I-Discovery BSP User Manual
|
stm32f769i_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_discovery.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons, external SDRAM, external QSPI Flash, RF EEPROM, 00009 * available on STM32F769I-Discovery board (MB1225) from 00010 * STMicroelectronics. 00011 ****************************************************************************** 00012 * @attention 00013 * 00014 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00015 * 00016 * Redistribution and use in source and binary forms, with or without modification, 00017 * are permitted provided that the following conditions are met: 00018 * 1. Redistributions of source code must retain the above copyright notice, 00019 * this list of conditions and the following disclaimer. 00020 * 2. Redistributions in binary form must reproduce the above copyright notice, 00021 * this list of conditions and the following disclaimer in the documentation 00022 * and/or other materials provided with the distribution. 00023 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00024 * may be used to endorse or promote products derived from this software 00025 * without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00028 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00029 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00030 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00031 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00032 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00033 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00034 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00035 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00036 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00037 * 00038 ****************************************************************************** 00039 */ 00040 00041 /* Includes ------------------------------------------------------------------*/ 00042 #include "stm32f769i_discovery.h" 00043 00044 /** @addtogroup BSP 00045 * @{ 00046 */ 00047 00048 /** @addtogroup STM32F769I_DISCOVERY 00049 * @{ 00050 */ 00051 00052 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL STM32F769I_DISCOVERY LOW LEVEL 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL_Private_TypesDefinitions STM32F769I Discovery Low Level Private Typedef 00057 * @{ 00058 */ 00059 /** 00060 * @} 00061 */ 00062 00063 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL_Private_Defines LOW_LEVEL Private Defines 00064 * @{ 00065 */ 00066 /** 00067 * @brief STM32F769I Discovery BSP Driver version number V2.0.0 00068 */ 00069 #define __STM32F769I_DISCOVERY_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00070 #define __STM32F769I_DISCOVERY_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00071 #define __STM32F769I_DISCOVERY_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00072 #define __STM32F769I_DISCOVERY_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00073 #define __STM32F769I_DISCOVERY_BSP_VERSION ((__STM32F769I_DISCOVERY_BSP_VERSION_MAIN << 24)\ 00074 |(__STM32F769I_DISCOVERY_BSP_VERSION_SUB1 << 16)\ 00075 |(__STM32F769I_DISCOVERY_BSP_VERSION_SUB2 << 8 )\ 00076 |(__STM32F769I_DISCOVERY_BSP_VERSION_RC)) 00077 /** 00078 * @} 00079 */ 00080 00081 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL_Private_Macros LOW_LEVEL Private Macros 00082 * @{ 00083 */ 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL_Private_Variables LOW_LEVEL Private Variables 00089 * @{ 00090 */ 00091 uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00092 LED2_PIN}; 00093 00094 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED1_GPIO_PORT, 00095 LED2_GPIO_PORT}; 00096 00097 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT }; 00098 00099 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN }; 00100 00101 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn }; 00102 00103 00104 static I2C_HandleTypeDef hI2cAudioHandler = {0}; 00105 static I2C_HandleTypeDef hI2cExtHandler = {0}; 00106 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL_Private_FunctionPrototypes LOW_LEVEL Private FunctionPrototypes 00112 * @{ 00113 */ 00114 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler); 00115 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler); 00116 00117 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00118 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00119 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials); 00120 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr); 00121 00122 /* AUDIO IO functions */ 00123 void AUDIO_IO_Init(void); 00124 void AUDIO_IO_DeInit(void); 00125 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00126 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00127 void AUDIO_IO_Delay(uint32_t Delay); 00128 00129 /* HDMI IO functions */ 00130 void HDMI_IO_Init(void); 00131 void HDMI_IO_Delay(uint32_t Delay); 00132 void HDMI_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00133 uint8_t HDMI_IO_Read(uint8_t Addr, uint8_t Reg); 00134 00135 /* I2C EEPROM IO function */ 00136 void EEPROM_IO_Init(void); 00137 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00138 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00139 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00140 00141 /* TouchScreen (TS) IO functions */ 00142 void TS_IO_Init(void); 00143 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00144 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00145 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00146 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00147 void TS_IO_Delay(uint32_t Delay); 00148 00149 /* LCD Display IO functions */ 00150 void OTM8009A_IO_Delay(uint32_t Delay); 00151 /** 00152 * @} 00153 */ 00154 00155 /** @defgroup STM32F769I_DISCOVERY_BSP_Public_Functions BSP Public Functions 00156 * @{ 00157 */ 00158 00159 /** 00160 * @brief This method returns the STM32F769I Discovery BSP Driver revision 00161 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00162 */ 00163 uint32_t BSP_GetVersion(void) 00164 { 00165 return __STM32F769I_DISCOVERY_BSP_VERSION; 00166 } 00167 00168 /** 00169 * @brief Configures LED GPIO. 00170 * @param Led: LED to be configured. 00171 * This parameter can be one of the following values: 00172 * @arg LED1 00173 * @arg LED2 00174 * @retval None 00175 */ 00176 void BSP_LED_Init(Led_TypeDef Led) 00177 { 00178 GPIO_InitTypeDef gpio_init_structure; 00179 00180 LEDx_GPIO_CLK_ENABLE(); 00181 /* Configure the GPIO_LED pin */ 00182 gpio_init_structure.Pin = GPIO_PIN[Led]; 00183 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00184 gpio_init_structure.Pull = GPIO_PULLUP; 00185 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00186 00187 HAL_GPIO_Init(GPIO_PORT[Led], &gpio_init_structure); 00188 00189 } 00190 00191 00192 /** 00193 * @brief DeInit LEDs. 00194 * @param Led: LED to be configured. 00195 * This parameter can be one of the following values: 00196 * @arg LED1 00197 * @arg LED2 00198 * @note Led DeInit does not disable the GPIO clock 00199 * @retval None 00200 */ 00201 void BSP_LED_DeInit(Led_TypeDef Led) 00202 { 00203 GPIO_InitTypeDef gpio_init_structure; 00204 00205 /* DeInit the GPIO_LED pin */ 00206 gpio_init_structure.Pin = GPIO_PIN[Led]; 00207 00208 /* Turn off LED */ 00209 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00210 HAL_GPIO_DeInit(GPIO_PORT[Led], gpio_init_structure.Pin); 00211 } 00212 00213 /** 00214 * @brief Turns selected LED On. 00215 * @param Led: LED to be set on 00216 * This parameter can be one of the following values: 00217 * @arg LED1 00218 * @arg LED2 00219 * @retval None 00220 */ 00221 void BSP_LED_On(Led_TypeDef Led) 00222 { 00223 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00224 } 00225 00226 /** 00227 * @brief Turns selected LED Off. 00228 * @param Led: LED to be set off 00229 * This parameter can be one of the following values: 00230 * @arg LED1 00231 * @arg LED2 00232 * @retval None 00233 */ 00234 void BSP_LED_Off(Led_TypeDef Led) 00235 { 00236 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00237 } 00238 00239 /** 00240 * @brief Toggles the selected LED. 00241 * @param Led: LED to be toggled 00242 * This parameter can be one of the following values: 00243 * @arg LED1 00244 * @arg LED2 00245 * @retval None 00246 */ 00247 void BSP_LED_Toggle(Led_TypeDef Led) 00248 { 00249 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00250 } 00251 00252 /** 00253 * @brief Configures button GPIO and EXTI Line. 00254 * @param Button: Button to be configured 00255 * This parameter can be one of the following values: 00256 * @arg BUTTON_WAKEUP: Wakeup Push Button 00257 * @arg BUTTON_USER: User Push Button 00258 * @param Button_Mode: Button mode 00259 * This parameter can be one of the following values: 00260 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00261 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00262 * with interrupt generation capability 00263 * @retval None 00264 */ 00265 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00266 { 00267 GPIO_InitTypeDef gpio_init_structure; 00268 00269 /* Enable the BUTTON clock */ 00270 BUTTON_GPIO_CLK_ENABLE(); 00271 00272 if(Button_Mode == BUTTON_MODE_GPIO) 00273 { 00274 /* Configure Button pin as input */ 00275 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00276 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00277 gpio_init_structure.Pull = GPIO_NOPULL; 00278 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00279 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00280 } 00281 00282 if(Button_Mode == BUTTON_MODE_EXTI) 00283 { 00284 /* Configure Button pin as input with External interrupt */ 00285 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00286 gpio_init_structure.Pull = GPIO_NOPULL; 00287 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00288 00289 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00290 00291 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00292 00293 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00294 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00295 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00296 } 00297 } 00298 00299 /** 00300 * @brief Push Button DeInit. 00301 * @param Button: Button to be configured 00302 * This parameter can be one of the following values: 00303 * @arg BUTTON_WAKEUP: Wakeup Push Button 00304 * @arg BUTTON_USER: User Push Button 00305 * @note PB DeInit does not disable the GPIO clock 00306 * @retval None 00307 */ 00308 void BSP_PB_DeInit(Button_TypeDef Button) 00309 { 00310 GPIO_InitTypeDef gpio_init_structure; 00311 00312 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00313 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00314 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00315 } 00316 00317 00318 /** 00319 * @brief Returns the selected button state. 00320 * @param Button: Button to be checked 00321 * This parameter can be one of the following values: 00322 * @arg BUTTON_WAKEUP: Wakeup Push Button 00323 * @arg BUTTON_USER: User Push Button 00324 * @retval The Button GPIO pin value 00325 */ 00326 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00327 { 00328 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00329 } 00330 00331 /** 00332 * @} 00333 */ 00334 00335 /** @defgroup STM32F769I_DISCOVERY_LOW_LEVEL_Private_Functions STM32F769I_DISCOVERY_LOW_LEVEL Private Functions 00336 * @{ 00337 */ 00338 00339 00340 /******************************************************************************* 00341 BUS OPERATIONS 00342 *******************************************************************************/ 00343 00344 /******************************* I2C Routines *********************************/ 00345 /** 00346 * @brief Initializes I2C MSP. 00347 * @param i2c_handler : I2C handler 00348 * @retval None 00349 */ 00350 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler) 00351 { 00352 GPIO_InitTypeDef gpio_init_structure; 00353 00354 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00355 { 00356 /*** Configure the GPIOs ***/ 00357 /* Enable GPIO clock */ 00358 DISCOVERY_AUDIO_I2Cx_SCL_GPIO_CLK_ENABLE(); 00359 DISCOVERY_AUDIO_I2Cx_SDA_GPIO_CLK_ENABLE(); 00360 /* Configure I2C Tx as alternate function */ 00361 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SCL_PIN; 00362 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00363 gpio_init_structure.Pull = GPIO_NOPULL; 00364 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00365 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SCL_AF; 00366 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_GPIO_PORT, &gpio_init_structure); 00367 00368 /* Configure I2C Rx as alternate function */ 00369 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SDA_PIN; 00370 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SDA_AF; 00371 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SDA_GPIO_PORT, &gpio_init_structure); 00372 00373 /*** Configure the I2C peripheral ***/ 00374 /* Enable I2C clock */ 00375 DISCOVERY_AUDIO_I2Cx_CLK_ENABLE(); 00376 00377 /* Force the I2C peripheral clock reset */ 00378 DISCOVERY_AUDIO_I2Cx_FORCE_RESET(); 00379 00380 /* Release the I2C peripheral clock reset */ 00381 DISCOVERY_AUDIO_I2Cx_RELEASE_RESET(); 00382 00383 /* Enable and set I2C1 Interrupt to a lower priority */ 00384 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_EV_IRQn, 0x0F, 0); 00385 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_EV_IRQn); 00386 00387 /* Enable and set I2C1 Interrupt to a lower priority */ 00388 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_ER_IRQn, 0x0F, 0); 00389 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_ER_IRQn); 00390 00391 } 00392 else 00393 { 00394 /*** Configure the GPIOs ***/ 00395 /* Enable GPIO clock */ 00396 DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00397 00398 /* Configure I2C Tx as alternate function */ 00399 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SCL_PIN; 00400 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00401 gpio_init_structure.Pull = GPIO_NOPULL; 00402 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00403 gpio_init_structure.Alternate = DISCOVERY_EXT_I2Cx_SCL_SDA_AF; 00404 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00405 00406 /* Configure I2C Rx as alternate function */ 00407 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SDA_PIN; 00408 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00409 00410 /*** Configure the I2C peripheral ***/ 00411 /* Enable I2C clock */ 00412 DISCOVERY_EXT_I2Cx_CLK_ENABLE(); 00413 00414 /* Force the I2C peripheral clock reset */ 00415 DISCOVERY_EXT_I2Cx_FORCE_RESET(); 00416 00417 /* Release the I2C peripheral clock reset */ 00418 DISCOVERY_EXT_I2Cx_RELEASE_RESET(); 00419 00420 /* Enable and set I2C1 Interrupt to a lower priority */ 00421 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_EV_IRQn, 0x0F, 0); 00422 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_EV_IRQn); 00423 00424 /* Enable and set I2C1 Interrupt to a lower priority */ 00425 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_ER_IRQn, 0x0F, 0); 00426 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_ER_IRQn); 00427 } 00428 } 00429 00430 /** 00431 * @brief Initializes I2C HAL. 00432 * @param i2c_handler : I2C handler 00433 * @retval None 00434 */ 00435 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler) 00436 { 00437 if(HAL_I2C_GetState(i2c_handler) == HAL_I2C_STATE_RESET) 00438 { 00439 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00440 { 00441 /* Audio and LCD I2C configuration */ 00442 i2c_handler->Instance = DISCOVERY_AUDIO_I2Cx; 00443 } 00444 else 00445 { 00446 /* External, camera and Arduino connector I2C configuration */ 00447 i2c_handler->Instance = DISCOVERY_EXT_I2Cx; 00448 } 00449 i2c_handler->Init.Timing = DISCOVERY_I2Cx_TIMING; 00450 i2c_handler->Init.OwnAddress1 = 0; 00451 i2c_handler->Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00452 i2c_handler->Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00453 i2c_handler->Init.OwnAddress2 = 0; 00454 i2c_handler->Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00455 i2c_handler->Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00456 00457 /* Init the I2C */ 00458 I2Cx_MspInit(i2c_handler); 00459 HAL_I2C_Init(i2c_handler); 00460 } 00461 } 00462 00463 /** 00464 * @brief Reads multiple data. 00465 * @param i2c_handler : I2C handler 00466 * @param Addr: I2C address 00467 * @param Reg: Reg address 00468 * @param MemAddress: memory address 00469 * @param Buffer: Pointer to data buffer 00470 * @param Length: Length of the data 00471 * @retval HAL status 00472 */ 00473 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00474 { 00475 HAL_StatusTypeDef status = HAL_OK; 00476 00477 status = HAL_I2C_Mem_Read(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00478 00479 /* Check the communication status */ 00480 if(status != HAL_OK) 00481 { 00482 /* I2C error occured */ 00483 I2Cx_Error(i2c_handler, Addr); 00484 } 00485 return status; 00486 } 00487 00488 00489 /** 00490 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00491 * @param i2c_handler : I2C handler 00492 * @param Addr: Device address on BUS Bus. 00493 * @param Reg: The target register address to write 00494 * @param MemAddress: memory address 00495 * @param Buffer: The target register value to be written 00496 * @param Length: buffer size to be written 00497 * @retval HAL status 00498 */ 00499 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00500 { 00501 HAL_StatusTypeDef status = HAL_OK; 00502 00503 status = HAL_I2C_Mem_Write(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00504 00505 /* Check the communication status */ 00506 if(status != HAL_OK) 00507 { 00508 /* Re-Initiaize the I2C Bus */ 00509 I2Cx_Error(i2c_handler, Addr); 00510 } 00511 return status; 00512 } 00513 00514 /** 00515 * @brief Checks if target device is ready for communication. 00516 * @note This function is used with Memory devices 00517 * @param i2c_handler : I2C handler 00518 * @param DevAddress: Target device address 00519 * @param Trials: Number of trials 00520 * @retval HAL status 00521 */ 00522 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials) 00523 { 00524 return (HAL_I2C_IsDeviceReady(i2c_handler, DevAddress, Trials, 1000)); 00525 } 00526 00527 /** 00528 * @brief Manages error callback by re-initializing I2C. 00529 * @param i2c_handler : I2C handler 00530 * @param Addr: I2C Address 00531 * @retval None 00532 */ 00533 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr) 00534 { 00535 /* De-initialize the I2C communication bus */ 00536 HAL_I2C_DeInit(i2c_handler); 00537 00538 /* Re-Initialize the I2C communication bus */ 00539 I2Cx_Init(i2c_handler); 00540 } 00541 00542 /** 00543 * @} 00544 */ 00545 00546 /******************************************************************************* 00547 LINK OPERATIONS 00548 *******************************************************************************/ 00549 00550 /********************************* LINK AUDIO *********************************/ 00551 00552 /** 00553 * @brief Initializes Audio low level. 00554 */ 00555 void AUDIO_IO_Init(void) 00556 { 00557 I2Cx_Init(&hI2cAudioHandler); 00558 } 00559 00560 /** 00561 * @brief DeInitializes Audio low level. 00562 */ 00563 void AUDIO_IO_DeInit(void) 00564 { 00565 00566 } 00567 00568 /** 00569 * @brief Writes a single data. 00570 * @param Addr: I2C address 00571 * @param Reg: Reg address 00572 * @param Value: Data to be written 00573 * @retval None 00574 */ 00575 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 00576 { 00577 uint16_t tmp = Value; 00578 00579 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 00580 00581 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 00582 00583 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 00584 } 00585 00586 /** 00587 * @brief Reads a single data. 00588 * @param Addr: I2C address 00589 * @param Reg: Reg address 00590 * @retval Data to be read 00591 */ 00592 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 00593 { 00594 uint16_t read_value = 0, tmp = 0; 00595 00596 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 00597 00598 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 00599 00600 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 00601 00602 read_value = tmp; 00603 00604 return read_value; 00605 } 00606 00607 /** 00608 * @brief AUDIO Codec delay 00609 * @param Delay: Delay in ms 00610 */ 00611 void AUDIO_IO_Delay(uint32_t Delay) 00612 { 00613 HAL_Delay(Delay); 00614 } 00615 00616 /******************************** LINK I2C EEPROM *****************************/ 00617 00618 /** 00619 * @brief Initializes peripherals used by the I2C EEPROM driver. 00620 */ 00621 void EEPROM_IO_Init(void) 00622 { 00623 I2Cx_Init(&hI2cExtHandler); 00624 } 00625 00626 /** 00627 * @brief Write data to I2C EEPROM driver in using DMA channel. 00628 * @param DevAddress: Target device address 00629 * @param MemAddress: Internal memory address 00630 * @param pBuffer: Pointer to data buffer 00631 * @param BufferSize: Amount of data to be sent 00632 * @retval HAL status 00633 */ 00634 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00635 { 00636 return (I2Cx_WriteMultiple(&hI2cExtHandler, DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00637 } 00638 00639 /** 00640 * @brief Read data from I2C EEPROM driver in using DMA channel. 00641 * @param DevAddress: Target device address 00642 * @param MemAddress: Internal memory address 00643 * @param pBuffer: Pointer to data buffer 00644 * @param BufferSize: Amount of data to be read 00645 * @retval HAL status 00646 */ 00647 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00648 { 00649 return (I2Cx_ReadMultiple(&hI2cExtHandler, DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00650 } 00651 00652 /** 00653 * @brief Checks if target device is ready for communication. 00654 * @note This function is used with Memory devices 00655 * @param DevAddress: Target device address 00656 * @param Trials: Number of trials 00657 * @retval HAL status 00658 */ 00659 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00660 { 00661 return (I2Cx_IsDeviceReady(&hI2cExtHandler, DevAddress, Trials)); 00662 } 00663 00664 /******************************** LINK TS (TouchScreen) ***********************/ 00665 00666 /** 00667 * @brief Initializes Touchscreen low level. 00668 * @retval None 00669 */ 00670 void TS_IO_Init(void) 00671 { 00672 I2Cx_Init(&hI2cAudioHandler); 00673 } 00674 00675 /** 00676 * @brief Writes a single data. 00677 * @param Addr: I2C address 00678 * @param Reg: Reg address 00679 * @param Value: Data to be written 00680 * @retval None 00681 */ 00682 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00683 { 00684 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00685 } 00686 00687 /** 00688 * @brief Reads a single data. 00689 * @param Addr: I2C address 00690 * @param Reg: Reg address 00691 * @retval Data to be read 00692 */ 00693 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 00694 { 00695 uint8_t read_value = 0; 00696 00697 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00698 00699 return read_value; 00700 } 00701 00702 /** 00703 * @brief Reads multiple data with I2C communication 00704 * channel from TouchScreen. 00705 * @param Addr: I2C address 00706 * @param Reg: Register address 00707 * @param Buffer: Pointer to data buffer 00708 * @param Length: Length of the data 00709 * @retval Number of read data 00710 */ 00711 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00712 { 00713 return I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00714 } 00715 00716 /** 00717 * @brief Writes multiple data with I2C communication 00718 * channel from MCU to TouchScreen. 00719 * @param Addr: I2C address 00720 * @param Reg: Register address 00721 * @param Buffer: Pointer to data buffer 00722 * @param Length: Length of the data 00723 * @retval None 00724 */ 00725 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00726 { 00727 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00728 } 00729 00730 /** 00731 * @brief Delay function used in TouchScreen low level driver. 00732 * @param Delay: Delay in ms 00733 * @retval None 00734 */ 00735 void TS_IO_Delay(uint32_t Delay) 00736 { 00737 HAL_Delay(Delay); 00738 } 00739 00740 /**************************** LINK OTM8009A (Display driver) ******************/ 00741 /** 00742 * @brief OTM8009A delay 00743 * @param Delay: Delay in ms 00744 */ 00745 void OTM8009A_IO_Delay(uint32_t Delay) 00746 { 00747 HAL_Delay(Delay); 00748 } 00749 00750 /**************************** LINK ADV7533 DSI-HDMI (Display driver) **********/ 00751 /** 00752 * @brief Initializes HDMI IO low level. 00753 * @retval None 00754 */ 00755 void HDMI_IO_Init(void) 00756 { 00757 I2Cx_Init(&hI2cAudioHandler); 00758 } 00759 00760 /** 00761 * @brief HDMI writes single data. 00762 * @param Addr: I2C address 00763 * @param Reg: Register address 00764 * @param Value: Data to be written 00765 * @retval None 00766 */ 00767 void HDMI_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00768 { 00769 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1); 00770 } 00771 00772 /** 00773 * @brief Reads single data with I2C communication 00774 * channel from HDMI bridge. 00775 * @param Addr: I2C address 00776 * @param Reg: Register address 00777 * @retval Read data 00778 */ 00779 uint8_t HDMI_IO_Read(uint8_t Addr, uint8_t Reg) 00780 { 00781 uint8_t value = 0x00; 00782 00783 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &value, 1); 00784 00785 return value; 00786 } 00787 00788 /** 00789 * @brief HDMI delay 00790 * @param Delay: Delay in ms 00791 * @retval None 00792 */ 00793 void HDMI_IO_Delay(uint32_t Delay) 00794 { 00795 HAL_Delay(Delay); 00796 } 00797 /** 00798 * @} 00799 */ 00800 00801 /** 00802 * @} 00803 */ 00804 00805 /** 00806 * @} 00807 */ 00808 00809 /** 00810 * @} 00811 */ 00812 00813 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 18:30:07 for STM32F769I-Discovery BSP User Manual by
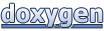