STM32F723E-Discovery BSP User Manual
|
stm32f723e_discovery_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f723e_discovery_ts.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 30-December-2016 00007 * @brief This file provides a set of functions needed to manage the Touch 00008 * Screen on STM32F723E-DISCOVERY evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the touch screen module of the STM32F723E-DISCOVERY 00044 evaluation board on the FRIDA LCD mounted on MB1260 discovery board. 00045 The touch screen driver IC is a FT6x36 type which share the same register naming 00046 with FT6206 type. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the TS module using the BSP_TS_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 communication layer configuration to start the TS use. The LCD size properties 00054 (x and y) are passed as parameters. 00055 Note: The FT6x36 requires a calibration. This should be done at application level. 00056 Refer to BSP example to correctly calibrate the touch screen. 00057 o If TS interrupt mode is desired, you must configure the TS interrupt mode 00058 by calling the function BSP_TS_ITConfig(). The TS interrupt mode is generated 00059 as an external interrupt whenever a touch is detected. 00060 00061 + Touch screen use 00062 o The touch screen state is captured whenever the function BSP_TS_GetState() is 00063 used. This function returns information about the last LCD touch occurred 00064 in the TS_StateTypeDef structure. 00065 ------------------------------------------------------------------------------*/ 00066 00067 /* Includes ------------------------------------------------------------------*/ 00068 #include "stm32f723e_discovery_ts.h" 00069 00070 /** @addtogroup BSP 00071 * @{ 00072 */ 00073 00074 /** @addtogroup STM32F723E_DISCOVERY 00075 * @{ 00076 */ 00077 00078 /** @defgroup STM32F723E_DISCOVERY_TS STM32F723E-DISCOVERY TS 00079 * @{ 00080 */ 00081 00082 /** @defgroup STM32F723E-DISCOVERY_TS_Private_Types_Definitions TS Private Types Definitions 00083 * @{ 00084 */ 00085 /** 00086 * @} 00087 */ 00088 00089 /** @defgroup STM32F723E-DISCOVERY_TS_Private_Defines TS Private Types Defines 00090 * @{ 00091 */ 00092 /** 00093 * @} 00094 */ 00095 00096 /** @defgroup STM32F723E-DISCOVERY_TS_Private_Macros TS Private Macros 00097 * @{ 00098 */ 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup STM32F723E-DISCOVERY_TS_Imported_Variables TS Imported Variables 00104 * @{ 00105 */ 00106 /** 00107 * @} 00108 */ 00109 00110 /** @defgroup STM32F723E-DISCOVERY_TS_Private_Variables TS Private Variables 00111 * @{ 00112 */ 00113 static TS_DrvTypeDef *tsDriver; 00114 static uint8_t I2C_Address = 0; 00115 static uint8_t tsOrientation = TS_SWAP_NONE; 00116 00117 /* Table for touchscreen event information display on LCD : table indexed on enum @ref TS_TouchEventTypeDef information */ 00118 char * ts_event_string_tab[TOUCH_EVENT_NB_MAX] = { "None", 00119 "Press down", 00120 "Lift up", 00121 "Contact" 00122 }; 00123 00124 /* Table for touchscreen gesture Id information display on LCD : table indexed on enum @ref TS_GestureIdTypeDef information */ 00125 char * ts_gesture_id_string_tab[GEST_ID_NB_MAX] = { "None", 00126 "Move Up", 00127 "Move Right", 00128 "Move Down", 00129 "Move Left", 00130 "Zoom In", 00131 "Zoom Out" 00132 }; 00133 /** 00134 * @} 00135 */ 00136 00137 /** @defgroup STM32F723E-DISCOVERY_TS_Private_Function_Prototypes TS Private Function Prototypes 00138 * @{ 00139 */ 00140 /** 00141 * @} 00142 */ 00143 00144 /** @defgroup STM32F723E-DISCOVERY_TS_Public_Functions TS Public Functions 00145 * @{ 00146 */ 00147 /** 00148 * @brief Initializes and configures the touch screen functionalities and 00149 * configures all necessary hardware resources (GPIOs, I2C, clocks..). 00150 * @param ts_SizeX : Maximum X size of the TS area on LCD 00151 * @param ts_SizeY : Maximum Y size of the TS area on LCD 00152 * @retval TS_OK if all initializations are OK. Other value if error. 00153 */ 00154 uint8_t BSP_TS_Init(uint16_t ts_SizeX, uint16_t ts_SizeY) 00155 { 00156 return (BSP_TS_InitEx(ts_SizeX, ts_SizeY, TS_ORIENTATION_LANDSCAPE)); 00157 } 00158 00159 /** 00160 * @brief Initializes and configures the touch screen functionalities and 00161 * configures all necessary hardware resources (GPIOs, I2C, clocks..) 00162 * with a given orientation 00163 * @param ts_SizeX : Maximum X size of the TS area on LCD 00164 * @param ts_SizeY : Maximum Y size of the TS area on LCD 00165 * @param orientation : TS_ORIENTATION_LANDSCAPE or TS_ORIENTATION_PORTRAIT 00166 * @retval TS_OK if all initializations are OK. Other value if error. 00167 */ 00168 uint8_t BSP_TS_InitEx(uint16_t ts_SizeX, uint16_t ts_SizeY, uint8_t orientation) 00169 { 00170 uint8_t ts_status = TS_OK; 00171 00172 /* Note : I2C_Address is un-initialized here, but is not used at all in init function */ 00173 /* but the prototype of Init() is like that in template and should be respected */ 00174 00175 /* Initialize the communication channel to sensor (I2C) if necessary */ 00176 /* that is initialization is done only once after a power up */ 00177 ft6x06_ts_drv.Init(I2C_Address); 00178 00179 /* Scan FT6x36 TouchScreen IC controller ID register by I2C Read */ 00180 /* Verify this is a FT6x36, otherwise this is an error case */ 00181 if(ft6x06_ts_drv.ReadID(TS_I2C_ADDRESS) == FT6x36_ID_VALUE) 00182 { 00183 /* Found FT6x36 : Initialize the TS driver structure */ 00184 tsDriver = &ft6x06_ts_drv; 00185 00186 I2C_Address = TS_I2C_ADDRESS; 00187 00188 /* Get LCD chosen orientation */ 00189 if(orientation == TS_ORIENTATION_PORTRAIT) 00190 { 00191 tsOrientation = TS_SWAP_X | TS_SWAP_Y; 00192 } 00193 else if(orientation == TS_ORIENTATION_LANDSCAPE_ROT180) 00194 { 00195 tsOrientation = TS_SWAP_XY; 00196 } 00197 else 00198 { 00199 tsOrientation = TS_SWAP_XY | TS_SWAP_Y; 00200 } 00201 00202 if(ts_status == TS_OK) 00203 { 00204 /* Software reset the TouchScreen */ 00205 tsDriver->Reset(I2C_Address); 00206 00207 /* Calibrate, Configure and Start the TouchScreen driver */ 00208 tsDriver->Start(I2C_Address); 00209 00210 } /* of if(ts_status == TS_OK) */ 00211 } 00212 else 00213 { 00214 ts_status = TS_DEVICE_NOT_FOUND; 00215 } 00216 00217 return (ts_status); 00218 } 00219 00220 /** 00221 * @brief Configures and enables the touch screen interrupts. 00222 * @retval TS_OK if all initializations are OK. Other value if error. 00223 */ 00224 uint8_t BSP_TS_ITConfig(void) 00225 { 00226 uint8_t ts_status = TS_OK; 00227 GPIO_InitTypeDef gpio_init_structure; 00228 00229 /* Msp Init of GPIO used for TS_INT pin coming from TouchScreen driver IC FT6x36 */ 00230 /* When touchscreen is operated in interrupt mode */ 00231 BSP_TS_INT_MspInit(); 00232 00233 /* Configure Interrupt mode for TS_INT pin falling edge : when a new touch is available */ 00234 /* TS_INT pin is active on low level on new touch available */ 00235 gpio_init_structure.Pin = TS_INT_PIN; 00236 gpio_init_structure.Pull = GPIO_PULLUP; 00237 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00238 gpio_init_structure.Mode = GPIO_MODE_IT_FALLING; 00239 HAL_GPIO_Init(TS_INT_GPIO_PORT, &gpio_init_structure); 00240 00241 /* Enable and set the TS_INT EXTI Interrupt to an intermediate priority */ 00242 HAL_NVIC_SetPriority((IRQn_Type)(TS_INT_EXTI_IRQn), 0x0F, 0x00); 00243 HAL_NVIC_EnableIRQ((IRQn_Type)(TS_INT_EXTI_IRQn)); 00244 00245 /* Enable the TS in interrupt mode */ 00246 /* In that case the INT output of FT6206 when new touch is available */ 00247 /* is active on low level and directed on EXTI */ 00248 tsDriver->EnableIT(I2C_Address); 00249 00250 return (ts_status); 00251 } 00252 00253 /** 00254 * @brief Returns status and positions of the touch screen. 00255 * @param TS_State: Pointer to touch screen current state structure 00256 * @retval TS_OK if all initializations are OK. Other value if error. 00257 */ 00258 uint8_t BSP_TS_GetState(TS_StateTypeDef *TS_State) 00259 { 00260 static uint32_t _x[TS_MAX_NB_TOUCH] = {0, 0}; 00261 static uint32_t _y[TS_MAX_NB_TOUCH] = {0, 0}; 00262 uint8_t ts_status = TS_OK; 00263 uint16_t tmp; 00264 uint16_t Raw_x[TS_MAX_NB_TOUCH]; 00265 uint16_t Raw_y[TS_MAX_NB_TOUCH]; 00266 uint16_t xDiff; 00267 uint16_t yDiff; 00268 uint32_t index; 00269 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00270 uint32_t weight = 0; 00271 uint32_t area = 0; 00272 uint32_t event = 0; 00273 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00274 00275 /* Check and update the number of touches active detected */ 00276 TS_State->touchDetected = tsDriver->DetectTouch(I2C_Address); 00277 if(TS_State->touchDetected) 00278 { 00279 for(index=0; index < TS_State->touchDetected; index++) 00280 { 00281 /* Get each touch coordinates */ 00282 tsDriver->GetXY(I2C_Address, &(Raw_x[index]), &(Raw_y[index])); 00283 00284 if(tsOrientation & TS_SWAP_XY) 00285 { 00286 tmp = Raw_x[index]; 00287 Raw_x[index] = Raw_y[index]; 00288 Raw_y[index] = tmp; 00289 } 00290 00291 if(tsOrientation & TS_SWAP_X) 00292 { 00293 Raw_x[index] = FT_6206_MAX_WIDTH_HEIGHT - 1 - Raw_x[index]; 00294 } 00295 00296 if(tsOrientation & TS_SWAP_Y) 00297 { 00298 Raw_y[index] = FT_6206_MAX_WIDTH_HEIGHT - 1 - Raw_y[index]; 00299 } 00300 00301 xDiff = Raw_x[index] > _x[index]? (Raw_x[index] - _x[index]): (_x[index] - Raw_x[index]); 00302 yDiff = Raw_y[index] > _y[index]? (Raw_y[index] - _y[index]): (_y[index] - Raw_y[index]); 00303 00304 if ((xDiff + yDiff) > 5) 00305 { 00306 _x[index] = Raw_x[index]; 00307 _y[index] = Raw_y[index]; 00308 } 00309 00310 00311 TS_State->touchX[index] = _x[index]; 00312 TS_State->touchY[index] = _y[index]; 00313 00314 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00315 00316 /* Get touch info related to the current touch */ 00317 ft6x06_TS_GetTouchInfo(I2C_Address, index, &weight, &area, &event); 00318 00319 /* Update TS_State structure */ 00320 TS_State->touchWeight[index] = weight; 00321 TS_State->touchArea[index] = area; 00322 00323 /* Remap touch event */ 00324 switch(event) 00325 { 00326 case FT6206_TOUCH_EVT_FLAG_PRESS_DOWN : 00327 TS_State->touchEventId[index] = TOUCH_EVENT_PRESS_DOWN; 00328 break; 00329 case FT6206_TOUCH_EVT_FLAG_LIFT_UP : 00330 TS_State->touchEventId[index] = TOUCH_EVENT_LIFT_UP; 00331 break; 00332 case FT6206_TOUCH_EVT_FLAG_CONTACT : 00333 TS_State->touchEventId[index] = TOUCH_EVENT_CONTACT; 00334 break; 00335 case FT6206_TOUCH_EVT_FLAG_NO_EVENT : 00336 TS_State->touchEventId[index] = TOUCH_EVENT_NO_EVT; 00337 break; 00338 default : 00339 ts_status = TS_ERROR; 00340 break; 00341 } /* of switch(event) */ 00342 00343 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00344 00345 } /* of for(index=0; index < TS_State->touchDetected; index++) */ 00346 00347 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00348 /* Get gesture Id */ 00349 ts_status = BSP_TS_Get_GestureId(TS_State); 00350 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00351 00352 } /* end of if(TS_State->touchDetected != 0) */ 00353 00354 return (ts_status); 00355 } 00356 00357 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00358 /** 00359 * @brief Update gesture Id following a touch detected. 00360 * @param TS_State: Pointer to touch screen current state structure 00361 * @retval TS_OK if all initializations are OK. Other value if error. 00362 */ 00363 uint8_t BSP_TS_Get_GestureId(TS_StateTypeDef *TS_State) 00364 { 00365 uint32_t gestureId = 0; 00366 uint8_t ts_status = TS_OK; 00367 00368 /* Get gesture Id */ 00369 ft6x06_TS_GetGestureID(I2C_Address, &gestureId); 00370 00371 /* Remap gesture Id to a TS_GestureIdTypeDef value */ 00372 switch(gestureId) 00373 { 00374 case FT6206_GEST_ID_NO_GESTURE : 00375 TS_State->gestureId = GEST_ID_NO_GESTURE; 00376 break; 00377 case FT6206_GEST_ID_MOVE_UP : 00378 TS_State->gestureId = GEST_ID_MOVE_UP; 00379 break; 00380 case FT6206_GEST_ID_MOVE_RIGHT : 00381 TS_State->gestureId = GEST_ID_MOVE_RIGHT; 00382 break; 00383 case FT6206_GEST_ID_MOVE_DOWN : 00384 TS_State->gestureId = GEST_ID_MOVE_DOWN; 00385 break; 00386 case FT6206_GEST_ID_MOVE_LEFT : 00387 TS_State->gestureId = GEST_ID_MOVE_LEFT; 00388 break; 00389 case FT6206_GEST_ID_ZOOM_IN : 00390 TS_State->gestureId = GEST_ID_ZOOM_IN; 00391 break; 00392 case FT6206_GEST_ID_ZOOM_OUT : 00393 TS_State->gestureId = GEST_ID_ZOOM_OUT; 00394 break; 00395 default : 00396 ts_status = TS_ERROR; 00397 break; 00398 } /* of switch(gestureId) */ 00399 00400 return(ts_status); 00401 } 00402 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00403 00404 00405 /** @defgroup STM32F723E-DISCOVERY_TS_Private_Functions TS Private Functions 00406 * @{ 00407 */ 00408 00409 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00410 /** 00411 * @brief Function used to reset all touch data before a new acquisition 00412 * of touch information. 00413 * @param TS_State: Pointer to touch screen current state structure 00414 * @retval TS_OK if OK, TE_ERROR if problem found. 00415 */ 00416 uint8_t BSP_TS_ResetTouchData(TS_StateTypeDef *TS_State) 00417 { 00418 uint8_t ts_status = TS_ERROR; 00419 uint32_t index; 00420 00421 if (TS_State != (TS_StateTypeDef *)NULL) 00422 { 00423 TS_State->gestureId = GEST_ID_NO_GESTURE; 00424 TS_State->touchDetected = 0; 00425 00426 for(index = 0; index < TS_MAX_NB_TOUCH; index++) 00427 { 00428 TS_State->touchX[index] = 0; 00429 TS_State->touchY[index] = 0; 00430 TS_State->touchArea[index] = 0; 00431 TS_State->touchEventId[index] = TOUCH_EVENT_NO_EVT; 00432 TS_State->touchWeight[index] = 0; 00433 } 00434 00435 ts_status = TS_OK; 00436 00437 } /* of if (TS_State != (TS_StateTypeDef *)NULL) */ 00438 00439 return (ts_status); 00440 } 00441 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00442 00443 /** 00444 * @brief Initializes the TS_INT pin MSP. 00445 * @retval None 00446 */ 00447 __weak void BSP_TS_INT_MspInit(void) 00448 { 00449 GPIO_InitTypeDef gpio_init_structure; 00450 00451 TS_INT_GPIO_CLK_ENABLE(); 00452 00453 /* GPIO configuration in input for TouchScreen interrupt signal on TS_INT pin */ 00454 gpio_init_structure.Pin = TS_INT_PIN; 00455 00456 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00457 gpio_init_structure.Pull = GPIO_PULLUP; 00458 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00459 HAL_GPIO_Init(TS_INT_GPIO_PORT, &gpio_init_structure); 00460 } 00461 00462 /** 00463 * @} 00464 */ 00465 00466 /** 00467 * @} 00468 */ 00469 00470 /** 00471 * @} 00472 */ 00473 00474 /** 00475 * @} 00476 */ 00477 00478 /** 00479 * @} 00480 */ 00481 00482 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Jan 2 2017 09:52:50 for STM32F723E-Discovery BSP User Manual by
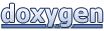