STM32F723E-Discovery BSP User Manual
|
stm32f723e_discovery_psram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f723e_discovery_psram.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 30-December-2016 00007 * @brief This file includes the PSRAM driver for the IS61WV51216BLL-10MLI memory 00008 * device mounted on STM32F723E-DISCOVERY boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the IS61WV51216BLL-10M PSRAM external memory mounted 00013 on STM32F723E discovery board. 00014 - This driver does not need a specific component driver for the PSRAM device 00015 to be included with. 00016 00017 Driver description: 00018 ------------------ 00019 + Initialization steps: 00020 o Initialize the PSRAM external memory using the BSP_PSRAM_Init() function. This 00021 function includes the MSP layer hardware resources initialization and the 00022 FMC controller configuration to interface with the external PSRAM memory. 00023 00024 + PSRAM read/write operations 00025 o PSRAM external memory can be accessed with read/write operations once it is 00026 initialized. 00027 Read/write operation can be performed with AHB access using the functions 00028 BSP_PSRAM_ReadData()/BSP_PSRAM_WriteData(), or by DMA transfer using the functions 00029 BSP_PSRAM_ReadData_DMA()/BSP_PSRAM_WriteData_DMA(). 00030 o The AHB access is performed with 16-bit width transaction, the DMA transfer 00031 configuration is fixed at single (no burst) halfword transfer 00032 (see the PSRAM_MspInit() static function). 00033 o User can implement his own functions for read/write access with his desired 00034 configurations. 00035 o If interrupt mode is used for DMA transfer, the function BSP_PSRAM_DMA_IRQHandler() 00036 is called in IRQ handler file, to serve the generated interrupt once the DMA 00037 transfer is complete. 00038 @endverbatim 00039 ****************************************************************************** 00040 * @attention 00041 * 00042 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00043 * 00044 * Redistribution and use in source and binary forms, with or without modification, 00045 * are permitted provided that the following conditions are met: 00046 * 1. Redistributions of source code must retain the above copyright notice, 00047 * this list of conditions and the following disclaimer. 00048 * 2. Redistributions in binary form must reproduce the above copyright notice, 00049 * this list of conditions and the following disclaimer in the documentation 00050 * and/or other materials provided with the distribution. 00051 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00052 * may be used to endorse or promote products derived from this software 00053 * without specific prior written permission. 00054 * 00055 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00056 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00057 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00058 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00059 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00060 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00061 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00062 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00063 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00064 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00065 * 00066 ****************************************************************************** 00067 */ 00068 00069 /* Includes ------------------------------------------------------------------*/ 00070 #include "stm32f723e_discovery_psram.h" 00071 00072 /** @addtogroup BSP 00073 * @{ 00074 */ 00075 00076 /** @addtogroup STM32F723E_DISCOVERY 00077 * @{ 00078 */ 00079 00080 /** @defgroup STM32F723E_DISCOVERY_PSRAM STM32F723E-DISCOVERY PSRAM 00081 * @{ 00082 */ 00083 00084 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Types_Definitions PSRAM Private Types Definitions 00085 * @{ 00086 */ 00087 /** 00088 * @} 00089 */ 00090 00091 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Defines PSRAM Private Defines 00092 * @{ 00093 */ 00094 /** 00095 * @} 00096 */ 00097 00098 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Macros PSRAM Private Macros 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Variables PSRAM Private Variables 00106 * @{ 00107 */ 00108 SRAM_HandleTypeDef psramHandle; 00109 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Function_Prototypes PSRAM Private Function Prototypes 00115 * @{ 00116 */ 00117 /** 00118 * @} 00119 */ 00120 00121 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Functions PSRAM Private Functions 00122 * @{ 00123 */ 00124 00125 /** 00126 * @brief Initializes the PSRAM device. 00127 * @retval PSRAM status 00128 */ 00129 uint8_t BSP_PSRAM_Init(void) 00130 { 00131 static FMC_NORSRAM_TimingTypeDef Timing; 00132 static uint8_t psram_status = PSRAM_ERROR; 00133 00134 /* PSRAM device configuration */ 00135 psramHandle.Instance = FMC_NORSRAM_DEVICE; 00136 psramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00137 00138 /* PSRAM device configuration */ 00139 /* Timing configuration derived from system clock (up to 216Mhz) 00140 for 108Mhz as PSRAM clock frequency */ 00141 Timing.AddressSetupTime = 9; 00142 Timing.AddressHoldTime = 2; 00143 Timing.DataSetupTime = 6; 00144 Timing.BusTurnAroundDuration = 1; 00145 Timing.CLKDivision = 2; 00146 Timing.DataLatency = 2; 00147 Timing.AccessMode = FMC_ACCESS_MODE_A; 00148 00149 psramHandle.Init.NSBank = FMC_NORSRAM_BANK1; 00150 psramHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00151 psramHandle.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00152 psramHandle.Init.MemoryDataWidth = PSRAM_MEMORY_WIDTH; 00153 psramHandle.Init.BurstAccessMode = PSRAM_BURSTACCESS; 00154 psramHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00155 psramHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00156 psramHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00157 psramHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00158 psramHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00159 psramHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00160 psramHandle.Init.WriteBurst = PSRAM_WRITEBURST; 00161 psramHandle.Init.WriteFifo = FMC_WRITE_FIFO_DISABLE; 00162 psramHandle.Init.PageSize = FMC_PAGE_SIZE_NONE; 00163 psramHandle.Init.ContinuousClock = CONTINUOUSCLOCK_FEATURE; 00164 00165 /* PSRAM controller initialization */ 00166 BSP_PSRAM_MspInit(&psramHandle, NULL); /* __weak function can be rewritten by the application */ 00167 if(HAL_SRAM_Init(&psramHandle, &Timing, &Timing) != HAL_OK) 00168 { 00169 psram_status = PSRAM_ERROR; 00170 } 00171 else 00172 { 00173 psram_status = PSRAM_OK; 00174 } 00175 return psram_status; 00176 } 00177 00178 /** 00179 * @brief DeInitializes the PSRAM device. 00180 * @retval PSRAM status 00181 */ 00182 uint8_t BSP_PSRAM_DeInit(void) 00183 { 00184 static uint8_t psram_status = PSRAM_ERROR; 00185 /* PSRAM device de-initialization */ 00186 psramHandle.Instance = FMC_NORSRAM_DEVICE; 00187 psramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00188 00189 if(HAL_SRAM_DeInit(&psramHandle) != HAL_OK) 00190 { 00191 psram_status = PSRAM_ERROR; 00192 } 00193 else 00194 { 00195 psram_status = PSRAM_OK; 00196 } 00197 00198 /* PSRAM controller de-initialization */ 00199 BSP_PSRAM_MspDeInit(&psramHandle, NULL); 00200 00201 return psram_status; 00202 } 00203 00204 /** 00205 * @brief Reads an amount of data from the PSRAM device in polling mode. 00206 * @param uwStartAddress: Read start address 00207 * @param pData: Pointer to data to be read 00208 * @param uwDataSize: Size of read data from the memory 00209 * @retval PSRAM status 00210 */ 00211 uint8_t BSP_PSRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00212 { 00213 if(HAL_SRAM_Read_16b(&psramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00214 { 00215 return PSRAM_ERROR; 00216 } 00217 else 00218 { 00219 return PSRAM_OK; 00220 } 00221 } 00222 00223 /** 00224 * @brief Reads an amount of data from the PSRAM device in DMA mode. 00225 * @param uwStartAddress: Read start address 00226 * @param pData: Pointer to data to be read 00227 * @param uwDataSize: Size of read data from the memory 00228 * @retval PSRAM status 00229 */ 00230 uint8_t BSP_PSRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00231 { 00232 if(HAL_SRAM_Read_DMA(&psramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00233 { 00234 return PSRAM_ERROR; 00235 } 00236 else 00237 { 00238 return PSRAM_OK; 00239 } 00240 } 00241 00242 /** 00243 * @brief Writes an amount of data from the PSRAM device in polling mode. 00244 * @param uwStartAddress: Write start address 00245 * @param pData: Pointer to data to be written 00246 * @param uwDataSize: Size of written data from the memory 00247 * @retval PSRAM status 00248 */ 00249 uint8_t BSP_PSRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00250 { 00251 if(HAL_SRAM_Write_16b(&psramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00252 { 00253 return PSRAM_ERROR; 00254 } 00255 else 00256 { 00257 return PSRAM_OK; 00258 } 00259 } 00260 00261 /** 00262 * @brief Writes an amount of data from the PSRAM device in DMA mode. 00263 * @param uwStartAddress: Write start address 00264 * @param pData: Pointer to data to be written 00265 * @param uwDataSize: Size of written data from the memory 00266 * @retval PSRAM status 00267 */ 00268 uint8_t BSP_PSRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00269 { 00270 if(HAL_SRAM_Write_DMA(&psramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00271 { 00272 return PSRAM_ERROR; 00273 } 00274 else 00275 { 00276 return PSRAM_OK; 00277 } 00278 } 00279 00280 /** 00281 * @brief Initializes PSRAM MSP. 00282 * @param hsram: PSRAM handle 00283 * @param Params 00284 * @retval None 00285 */ 00286 __weak void BSP_PSRAM_MspInit(SRAM_HandleTypeDef *hsram, void *Params) 00287 { 00288 static DMA_HandleTypeDef dma_handle; 00289 GPIO_InitTypeDef gpio_init_structure; 00290 00291 /* Enable FMC clock */ 00292 __HAL_RCC_FMC_CLK_ENABLE(); 00293 00294 /* Enable chosen DMAx clock */ 00295 __PSRAM_DMAx_CLK_ENABLE(); 00296 00297 /* Enable GPIOs clock */ 00298 __HAL_RCC_GPIOF_CLK_ENABLE(); 00299 __HAL_RCC_GPIOG_CLK_ENABLE(); 00300 __HAL_RCC_GPIOD_CLK_ENABLE(); 00301 __HAL_RCC_GPIOE_CLK_ENABLE(); 00302 00303 /* Common GPIO configuration */ 00304 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00305 gpio_init_structure.Pull = GPIO_PULLUP; 00306 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00307 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00308 00309 /* GPIOD configuration: GPIO_PIN_7 is FMC_NE1 , GPIO_PIN_11 ans GPIO_PIN_12 are PSRAM_A16 and PSRAM_A17 */ 00310 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_7 | GPIO_PIN_8 |\ 00311 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_14 | GPIO_PIN_15; 00312 00313 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00314 00315 /* GPIOE configuration */ 00316 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00317 GPIO_PIN_12 |GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00318 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00319 00320 /* GPIOF configuration */ 00321 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 |\ 00322 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00323 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00324 00325 /* GPIOG configuration */ 00326 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 |\ 00327 GPIO_PIN_5; 00328 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00329 00330 /* Configure common DMA parameters */ 00331 dma_handle.Init.Channel = PSRAM_DMAx_CHANNEL; 00332 dma_handle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00333 dma_handle.Init.PeriphInc = DMA_PINC_ENABLE; 00334 dma_handle.Init.MemInc = DMA_MINC_ENABLE; 00335 dma_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00336 dma_handle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00337 dma_handle.Init.Mode = DMA_NORMAL; 00338 dma_handle.Init.Priority = DMA_PRIORITY_HIGH; 00339 dma_handle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00340 dma_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00341 dma_handle.Init.MemBurst = DMA_MBURST_SINGLE; 00342 dma_handle.Init.PeriphBurst = DMA_PBURST_SINGLE; 00343 00344 dma_handle.Instance = PSRAM_DMAx_STREAM; 00345 00346 /* Associate the DMA handle */ 00347 __HAL_LINKDMA(hsram, hdma, dma_handle); 00348 00349 /* Deinitialize the Stream for new transfer */ 00350 HAL_DMA_DeInit(&dma_handle); 00351 00352 /* Configure the DMA Stream */ 00353 HAL_DMA_Init(&dma_handle); 00354 00355 /* NVIC configuration for DMA transfer complete interrupt */ 00356 HAL_NVIC_SetPriority(PSRAM_DMAx_IRQn, 0x0F, 0); 00357 HAL_NVIC_EnableIRQ(PSRAM_DMAx_IRQn); 00358 } 00359 00360 00361 /** 00362 * @brief DeInitializes SRAM MSP. 00363 * @param hsram: SRAM handle 00364 * @param Params 00365 * @retval None 00366 */ 00367 __weak void BSP_PSRAM_MspDeInit(SRAM_HandleTypeDef *hsram, void *Params) 00368 { 00369 static DMA_HandleTypeDef dma_handle; 00370 00371 /* Disable NVIC configuration for DMA interrupt */ 00372 HAL_NVIC_DisableIRQ(PSRAM_DMAx_IRQn); 00373 00374 /* Deinitialize the stream for new transfer */ 00375 dma_handle.Instance = PSRAM_DMAx_STREAM; 00376 HAL_DMA_DeInit(&dma_handle); 00377 00378 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the applications 00379 by surcharging this __weak function */ 00380 } 00381 /** 00382 * @} 00383 */ 00384 00385 /** 00386 * @} 00387 */ 00388 00389 /** 00390 * @} 00391 */ 00392 00393 /** 00394 * @} 00395 */ 00396 00397 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Jan 2 2017 09:52:50 for STM32F723E-Discovery BSP User Manual by
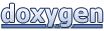