STM32F723E-Discovery BSP User Manual
|
stm32f723e_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f723e_discovery.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 30-December-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons, external PSRAM, external QSPI Flash, TS available on 00009 * STM32F723E-Discovery board (MB1260) from STMicroelectronics. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "stm32f723e_discovery.h" 00042 00043 /** @addtogroup BSP 00044 * @{ 00045 */ 00046 00047 /** @addtogroup STM32F723E_DISCOVERY 00048 * @{ 00049 */ 00050 00051 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL STM32F723E-DISCOVERY LOW LEVEL 00052 * @{ 00053 */ 00054 00055 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_TypesDefinitions STM32F723E Discovery Low Level Private Typedef 00056 * @{ 00057 */ 00058 typedef struct 00059 { 00060 __IO uint16_t REG; 00061 __IO uint16_t RAM; 00062 }LCD_CONTROLLER_TypeDef; 00063 /** 00064 * @} 00065 */ 00066 00067 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_Defines LOW_LEVEL Private Defines 00068 * @{ 00069 */ 00070 /** 00071 * @brief STM32F723E Discovery BSP Driver version number V1.0.0 00072 */ 00073 #define __STM32F723E_DISCOVERY_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00074 #define __STM32F723E_DISCOVERY_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00075 #define __STM32F723E_DISCOVERY_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00076 #define __STM32F723E_DISCOVERY_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00077 #define __STM32F723E_DISCOVERY_BSP_VERSION ((__STM32F723E_DISCOVERY_BSP_VERSION_MAIN << 24)\ 00078 |(__STM32F723E_DISCOVERY_BSP_VERSION_SUB1 << 16)\ 00079 |(__STM32F723E_DISCOVERY_BSP_VERSION_SUB2 << 8 )\ 00080 |(__STM32F723E_DISCOVERY_BSP_VERSION_RC)) 00081 00082 /* We use BANK2 as we use FMC_NE2 signal */ 00083 #define FMC_BANK2_BASE ((uint32_t)(0x60000000 | 0x04000000)) 00084 #define FMC_BANK2 ((LCD_CONTROLLER_TypeDef *) FMC_BANK2_BASE) 00085 /** 00086 * @} 00087 */ 00088 00089 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_Macros LOW_LEVEL Private Macros 00090 * @{ 00091 */ 00092 /** 00093 * @} 00094 */ 00095 00096 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_Variables LOW_LEVEL Private Variables 00097 * @{ 00098 */ 00099 uint32_t GPIO_PIN[LEDn] = {LED5_PIN, 00100 LED6_PIN}; 00101 00102 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED5_GPIO_PORT, 00103 LED6_GPIO_PORT}; 00104 00105 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT }; 00106 00107 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN }; 00108 00109 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn }; 00110 00111 USART_TypeDef* COM_USART[COMn] = {DISCOVERY_COM1}; 00112 00113 GPIO_TypeDef* COM_TX_PORT[COMn] = {DISCOVERY_COM1_TX_GPIO_PORT}; 00114 00115 GPIO_TypeDef* COM_RX_PORT[COMn] = {DISCOVERY_COM1_RX_GPIO_PORT}; 00116 00117 const uint16_t COM_TX_PIN[COMn] = {DISCOVERY_COM1_TX_PIN}; 00118 00119 const uint16_t COM_RX_PIN[COMn] = {DISCOVERY_COM1_RX_PIN}; 00120 00121 const uint16_t COM_TX_AF[COMn] = {DISCOVERY_COM1_TX_AF}; 00122 00123 const uint16_t COM_RX_AF[COMn] = {DISCOVERY_COM1_RX_AF}; 00124 00125 static I2C_HandleTypeDef hI2cAudioHandler = {0}; 00126 static I2C_HandleTypeDef hI2cTsHandler = {0}; 00127 00128 /** 00129 * @} 00130 */ 00131 00132 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_FunctionPrototypes LOW_LEVEL Private FunctionPrototypes 00133 * @{ 00134 */ 00135 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler); 00136 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler); 00137 00138 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00139 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00140 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr); 00141 00142 static void FMC_BANK2_WriteData(uint16_t Data); 00143 static void FMC_BANK2_WriteReg(uint8_t Reg); 00144 static uint16_t FMC_BANK2_ReadData(void); 00145 static void FMC_BANK2_Init(void); 00146 static void FMC_BANK2_MspInit(void); 00147 00148 /* LCD IO functions */ 00149 void LCD_IO_Init(void); 00150 void LCD_IO_WriteData(uint16_t RegValue); 00151 void LCD_IO_WriteReg(uint8_t Reg); 00152 void LCD_IO_WriteMultipleData(uint16_t *pData, uint32_t Size); 00153 uint16_t LCD_IO_ReadData(void); 00154 void LCD_IO_Delay(uint32_t Delay); 00155 00156 /* AUDIO IO functions */ 00157 void AUDIO_IO_Init(void); 00158 void AUDIO_IO_DeInit(void); 00159 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00160 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00161 void AUDIO_IO_Delay(uint32_t Delay); 00162 00163 /* TouchScreen (TS) IO functions */ 00164 void TS_IO_Init(void); 00165 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00166 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00167 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00168 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00169 void TS_IO_Delay(uint32_t Delay); 00170 00171 /** 00172 * @} 00173 */ 00174 00175 /** @defgroup STM32F723E_DISCOVERY_BSP_Public_Functions BSP Public Functions 00176 * @{ 00177 */ 00178 00179 /** 00180 * @brief This method returns the STM32F723E Discovery BSP Driver revision 00181 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00182 */ 00183 uint32_t BSP_GetVersion(void) 00184 { 00185 return __STM32F723E_DISCOVERY_BSP_VERSION; 00186 } 00187 00188 /** 00189 * @brief Configures LED GPIO. 00190 * @param Led: LED to be configured. 00191 * This parameter can be one of the following values: 00192 * @arg LED5 00193 * @arg LED6 00194 * @retval None 00195 */ 00196 void BSP_LED_Init(Led_TypeDef Led) 00197 { 00198 GPIO_InitTypeDef gpio_init_structure; 00199 00200 LEDx_GPIO_CLK_ENABLE(Led); 00201 /* Configure the GPIO_LED pin */ 00202 gpio_init_structure.Pin = GPIO_PIN[Led]; 00203 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00204 gpio_init_structure.Pull = GPIO_PULLUP; 00205 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00206 00207 HAL_GPIO_Init(GPIO_PORT[Led], &gpio_init_structure); 00208 00209 } 00210 00211 00212 /** 00213 * @brief DeInit LEDs. 00214 * @param Led: LED to be configured. 00215 * This parameter can be one of the following values: 00216 * @arg LED5 00217 * @arg LED6 00218 * @note Led DeInit does not disable the GPIO clock 00219 * @retval None 00220 */ 00221 void BSP_LED_DeInit(Led_TypeDef Led) 00222 { 00223 GPIO_InitTypeDef gpio_init_structure; 00224 00225 /* DeInit the GPIO_LED pin */ 00226 gpio_init_structure.Pin = GPIO_PIN[Led]; 00227 00228 /* Turn off LED */ 00229 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00230 HAL_GPIO_DeInit(GPIO_PORT[Led], gpio_init_structure.Pin); 00231 } 00232 00233 /** 00234 * @brief Turns selected LED On. 00235 * @param Led: LED to be set on 00236 * This parameter can be one of the following values: 00237 * @arg LED5 00238 * @arg LED6 00239 * @retval None 00240 */ 00241 void BSP_LED_On(Led_TypeDef Led) 00242 { 00243 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00244 } 00245 00246 /** 00247 * @brief Turns selected LED Off. 00248 * @param Led: LED to be set off 00249 * This parameter can be one of the following values: 00250 * @arg LED5 00251 * @arg LED6 00252 * @retval None 00253 */ 00254 void BSP_LED_Off(Led_TypeDef Led) 00255 { 00256 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00257 } 00258 00259 /** 00260 * @brief Toggles the selected LED. 00261 * @param Led: LED to be toggled 00262 * This parameter can be one of the following values: 00263 * @arg LED5 00264 * @arg LED6 00265 * @retval None 00266 */ 00267 void BSP_LED_Toggle(Led_TypeDef Led) 00268 { 00269 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00270 } 00271 00272 /** 00273 * @brief Configures button GPIO and EXTI Line. 00274 * @param Button: Button to be configured 00275 * This parameter can be one of the following values: 00276 * @arg BUTTON_WAKEUP: Wakeup Push Button 00277 * @arg BUTTON_USER: User Push Button 00278 * @param Button_Mode: Button mode 00279 * This parameter can be one of the following values: 00280 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00281 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00282 * with interrupt generation capability 00283 * @retval None 00284 */ 00285 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00286 { 00287 GPIO_InitTypeDef gpio_init_structure; 00288 00289 /* Enable the BUTTON clock */ 00290 BUTTON_GPIO_CLK_ENABLE(); 00291 00292 if(Button_Mode == BUTTON_MODE_GPIO) 00293 { 00294 /* Configure Button pin as input */ 00295 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00296 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00297 gpio_init_structure.Pull = GPIO_NOPULL; 00298 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00299 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00300 } 00301 00302 if(Button_Mode == BUTTON_MODE_EXTI) 00303 { 00304 /* Configure Button pin as input with External interrupt */ 00305 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00306 gpio_init_structure.Pull = GPIO_NOPULL; 00307 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00308 00309 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00310 00311 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00312 00313 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00314 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00315 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00316 } 00317 } 00318 00319 /** 00320 * @brief Push Button DeInit. 00321 * @param Button: Button to be configured 00322 * This parameter can be one of the following values: 00323 * @arg BUTTON_WAKEUP: Wakeup Push Button 00324 * @arg BUTTON_USER: User Push Button 00325 * @note PB DeInit does not disable the GPIO clock 00326 * @retval None 00327 */ 00328 void BSP_PB_DeInit(Button_TypeDef Button) 00329 { 00330 GPIO_InitTypeDef gpio_init_structure; 00331 00332 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00333 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00334 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00335 } 00336 00337 00338 /** 00339 * @brief Returns the selected button state. 00340 * @param Button: Button to be checked 00341 * This parameter can be one of the following values: 00342 * @arg BUTTON_WAKEUP: Wakeup Push Button 00343 * @arg BUTTON_USER: User Push Button 00344 * @retval The Button GPIO pin value 00345 */ 00346 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00347 { 00348 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00349 } 00350 00351 /** 00352 * @} 00353 */ 00354 00355 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_Functions STM32F723E_DISCOVERY_LOW_LEVEL Private Functions 00356 * @{ 00357 */ 00358 /** 00359 * @brief Configures COM port. 00360 * @param COM: COM port to be configured. 00361 * This parameter can be one of the following values: 00362 * @arg COM1 00363 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00364 * configuration information for the specified USART peripheral. 00365 * @retval None 00366 */ 00367 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00368 { 00369 GPIO_InitTypeDef gpio_init_structure; 00370 00371 /* Enable GPIO clock */ 00372 DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(COM); 00373 DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(COM); 00374 00375 /* Enable USART clock */ 00376 DISCOVERY_COMx_CLK_ENABLE(COM); 00377 00378 /* Configure USART Tx as alternate function */ 00379 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00380 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00381 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00382 gpio_init_structure.Pull = GPIO_PULLUP; 00383 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00384 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00385 00386 /* Configure USART Rx as alternate function */ 00387 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00388 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00389 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00390 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00391 00392 /* USART configuration */ 00393 huart->Instance = COM_USART[COM]; 00394 HAL_UART_Init(huart); 00395 } 00396 00397 /** 00398 * @brief DeInit COM port. 00399 * @param COM: COM port to be configured. 00400 * This parameter can be one of the following values: 00401 * @arg COM1 00402 * @arg COM2 00403 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00404 * configuration information for the specified USART peripheral. 00405 * @retval None 00406 */ 00407 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00408 { 00409 /* USART configuration */ 00410 huart->Instance = COM_USART[COM]; 00411 HAL_UART_DeInit(huart); 00412 00413 /* Enable USART clock */ 00414 DISCOVERY_COMx_CLK_DISABLE(COM); 00415 00416 /* DeInit GPIO pins can be done in the application 00417 (by surcharging this __weak function) */ 00418 00419 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the application 00420 by surcharging this __weak function */ 00421 } 00422 00423 /******************************************************************************* 00424 BUS OPERATIONS 00425 *******************************************************************************/ 00426 00427 /******************************* I2C Routines *********************************/ 00428 /** 00429 * @brief Initializes I2C MSP. 00430 * @param i2c_handler : I2C handler 00431 * @retval None 00432 */ 00433 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler) 00434 { 00435 GPIO_InitTypeDef gpio_init_structure; 00436 00437 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00438 { 00439 /*** Configure the GPIOs ***/ 00440 /* Enable GPIO clock */ 00441 DISCOVERY_AUDIO_I2Cx_SCL_GPIO_CLK_ENABLE(); 00442 DISCOVERY_AUDIO_I2Cx_SDA_GPIO_CLK_ENABLE(); 00443 /* Configure I2C Tx as alternate function */ 00444 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SCL_PIN; 00445 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00446 gpio_init_structure.Pull = GPIO_NOPULL; 00447 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00448 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SCL_AF; 00449 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_GPIO_PORT, &gpio_init_structure); 00450 00451 /* Configure I2C Rx as alternate function */ 00452 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SDA_PIN; 00453 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SDA_AF; 00454 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SDA_GPIO_PORT, &gpio_init_structure); 00455 00456 /*** Configure the I2C peripheral ***/ 00457 /* Enable I2C clock */ 00458 DISCOVERY_AUDIO_I2Cx_CLK_ENABLE(); 00459 00460 /* Force the I2C peripheral clock reset */ 00461 DISCOVERY_AUDIO_I2Cx_FORCE_RESET(); 00462 00463 /* Release the I2C peripheral clock reset */ 00464 DISCOVERY_AUDIO_I2Cx_RELEASE_RESET(); 00465 00466 /* Enable and set I2C1 Interrupt to a lower priority */ 00467 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_EV_IRQn, 0x0F, 0); 00468 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_EV_IRQn); 00469 00470 /* Enable and set I2C1 Interrupt to a lower priority */ 00471 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_ER_IRQn, 0x0F, 0); 00472 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_ER_IRQn); 00473 00474 } 00475 else if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cTsHandler)) 00476 { 00477 /*** Configure the GPIOs ***/ 00478 /* Enable GPIO clock */ 00479 TS_I2Cx_SCL_GPIO_CLK_ENABLE(); 00480 TS_I2Cx_SDA_GPIO_CLK_ENABLE(); 00481 /* Configure I2C SCL as alternate function */ 00482 gpio_init_structure.Pin = TS_I2Cx_SCL_PIN; 00483 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00484 gpio_init_structure.Pull = GPIO_NOPULL; 00485 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00486 gpio_init_structure.Alternate = TS_I2Cx_SCL_AF; 00487 HAL_GPIO_Init(TS_I2Cx_SCL_GPIO_PORT, &gpio_init_structure); 00488 00489 /* Configure I2C SDA as alternate function */ 00490 gpio_init_structure.Pin = TS_I2Cx_SDA_PIN; 00491 gpio_init_structure.Alternate = TS_I2Cx_SDA_AF; 00492 HAL_GPIO_Init(TS_I2Cx_SDA_GPIO_PORT, &gpio_init_structure); 00493 00494 /*** Configure the I2C peripheral ***/ 00495 /* Enable I2C clock */ 00496 TS_I2Cx_CLK_ENABLE(); 00497 00498 /* Force the I2C peripheral clock reset */ 00499 TS_I2Cx_FORCE_RESET(); 00500 00501 /* Release the I2C peripheral clock reset */ 00502 TS_I2Cx_RELEASE_RESET(); 00503 00504 /* Enable and set I2C Interrupt to a lower priority */ 00505 HAL_NVIC_SetPriority(TS_I2Cx_EV_IRQn, 0x0F, 0); 00506 HAL_NVIC_EnableIRQ(TS_I2Cx_EV_IRQn); 00507 00508 /* Enable and set I2C Interrupt to a lower priority */ 00509 HAL_NVIC_SetPriority(TS_I2Cx_ER_IRQn, 0x0F, 0); 00510 HAL_NVIC_EnableIRQ(TS_I2Cx_ER_IRQn); 00511 00512 } 00513 else 00514 { 00515 /*** Configure the GPIOs ***/ 00516 /* Enable GPIO clock */ 00517 DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00518 00519 /* Configure I2C Tx as alternate function */ 00520 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SCL_PIN; 00521 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00522 gpio_init_structure.Pull = GPIO_NOPULL; 00523 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00524 gpio_init_structure.Alternate = DISCOVERY_EXT_I2Cx_SCL_SDA_AF; 00525 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00526 00527 /* Configure I2C Rx as alternate function */ 00528 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SDA_PIN; 00529 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00530 00531 /*** Configure the I2C peripheral ***/ 00532 /* Enable I2C clock */ 00533 DISCOVERY_EXT_I2Cx_CLK_ENABLE(); 00534 00535 /* Force the I2C peripheral clock reset */ 00536 DISCOVERY_EXT_I2Cx_FORCE_RESET(); 00537 00538 /* Release the I2C peripheral clock reset */ 00539 DISCOVERY_EXT_I2Cx_RELEASE_RESET(); 00540 00541 /* Enable and set I2C1 Interrupt to a lower priority */ 00542 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_EV_IRQn, 0x0F, 0); 00543 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_EV_IRQn); 00544 00545 /* Enable and set I2C1 Interrupt to a lower priority */ 00546 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_ER_IRQn, 0x0F, 0); 00547 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_ER_IRQn); 00548 } 00549 } 00550 00551 /** 00552 * @brief Initializes I2C HAL. 00553 * @param i2c_handler : I2C handler 00554 * @retval None 00555 */ 00556 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler) 00557 { 00558 if(HAL_I2C_GetState(i2c_handler) == HAL_I2C_STATE_RESET) 00559 { 00560 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00561 { 00562 /* Audio and LCD I2C configuration */ 00563 i2c_handler->Instance = DISCOVERY_AUDIO_I2Cx; 00564 } 00565 else if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cTsHandler)) 00566 { 00567 /* TouchScreen I2C configuration */ 00568 i2c_handler->Instance = TS_I2Cx; 00569 } 00570 else 00571 { 00572 /* External, camera and Arduino connector I2C configuration */ 00573 i2c_handler->Instance = DISCOVERY_EXT_I2Cx; 00574 } 00575 i2c_handler->Init.Timing = DISCOVERY_I2Cx_TIMING; 00576 i2c_handler->Init.OwnAddress1 = 0; 00577 i2c_handler->Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00578 i2c_handler->Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00579 i2c_handler->Init.OwnAddress2 = 0; 00580 i2c_handler->Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00581 i2c_handler->Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00582 00583 /* Init the I2C */ 00584 I2Cx_MspInit(i2c_handler); 00585 HAL_I2C_Init(i2c_handler); 00586 } 00587 } 00588 00589 /** 00590 * @brief Reads multiple data. 00591 * @param i2c_handler : I2C handler 00592 * @param Addr: I2C address 00593 * @param Reg: Reg address 00594 * @param MemAddress: memory address 00595 * @param Buffer: Pointer to data buffer 00596 * @param Length: Length of the data 00597 * @retval HAL status 00598 */ 00599 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00600 { 00601 HAL_StatusTypeDef status = HAL_OK; 00602 00603 status = HAL_I2C_Mem_Read(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00604 00605 /* Check the communication status */ 00606 if(status != HAL_OK) 00607 { 00608 /* I2C error occured */ 00609 I2Cx_Error(i2c_handler, Addr); 00610 } 00611 return status; 00612 } 00613 00614 00615 /** 00616 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00617 * @param i2c_handler : I2C handler 00618 * @param Addr: Device address on BUS Bus. 00619 * @param Reg: The target register address to write 00620 * @param MemAddress: memory address 00621 * @param Buffer: The target register value to be written 00622 * @param Length: buffer size to be written 00623 * @retval HAL status 00624 */ 00625 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00626 { 00627 HAL_StatusTypeDef status = HAL_OK; 00628 00629 status = HAL_I2C_Mem_Write(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00630 00631 /* Check the communication status */ 00632 if(status != HAL_OK) 00633 { 00634 /* Re-Initiaize the I2C Bus */ 00635 I2Cx_Error(i2c_handler, Addr); 00636 } 00637 return status; 00638 } 00639 00640 00641 /** 00642 * @brief Manages error callback by re-initializing I2C. 00643 * @param i2c_handler : I2C handler 00644 * @param Addr: I2C Address 00645 * @retval None 00646 */ 00647 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr) 00648 { 00649 /* De-initialize the I2C communication bus */ 00650 HAL_I2C_DeInit(i2c_handler); 00651 00652 /* Re-Initialize the I2C communication bus */ 00653 I2Cx_Init(i2c_handler); 00654 } 00655 00656 /** 00657 * @} 00658 */ 00659 00660 /******************************************************************************* 00661 LINK OPERATIONS 00662 *******************************************************************************/ 00663 /*************************** FMC Routines *************************************/ 00664 /** 00665 * @brief Initializes FMC_BANK2 MSP. 00666 * @retval None 00667 */ 00668 static void FMC_BANK2_MspInit(void) 00669 { 00670 GPIO_InitTypeDef gpio_init_structure; 00671 00672 /* Enable FMC clock */ 00673 __HAL_RCC_FMC_CLK_ENABLE(); 00674 00675 /* Enable FSMC clock */ 00676 __HAL_RCC_FMC_CLK_ENABLE(); 00677 __HAL_RCC_FMC_FORCE_RESET(); 00678 __HAL_RCC_FMC_RELEASE_RESET(); 00679 00680 /* Enable GPIOs clock */ 00681 __HAL_RCC_GPIOD_CLK_ENABLE(); 00682 __HAL_RCC_GPIOE_CLK_ENABLE(); 00683 __HAL_RCC_GPIOF_CLK_ENABLE(); 00684 __HAL_RCC_GPIOG_CLK_ENABLE(); 00685 00686 /* Common GPIO configuration */ 00687 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00688 gpio_init_structure.Pull = GPIO_PULLUP; 00689 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00690 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00691 00692 /* GPIOD configuration */ 00693 /* LCD_PSRAM_D2, LCD_PSRAM_D3, LCD_PSRAM_NOE, LCD_PSRAM_NWE, PSRAM_NE1, LCD_PSRAM_D13, 00694 LCD_PSRAM_D14, LCD_PSRAM_D15, PSRAM_A16, PSRAM_A17, LCD_PSRAM_D0, LCD_PSRAM_D1 */ 00695 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_7 | GPIO_PIN_8 |\ 00696 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_14 | GPIO_PIN_15; 00697 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00698 00699 /* GPIOE configuration */ 00700 /* PSRAM_NBL0, PSRAM_NBL1, LCD_PSRAM_D4, LCD_PSRAM_D5, LCD_PSRAM_D6, LCD_PSRAM_D7, 00701 LCD_PSRAM_D8, LCD_PSRAM_D9, LCD_PSRAM_D10, LCD_PSRAM_D11, LCD_PSRAM_D12 */ 00702 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 |GPIO_PIN_10 |\ 00703 GPIO_PIN_11 | GPIO_PIN_12 |GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00704 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00705 00706 /* GPIOF configuration */ 00707 /* PSRAM_A0, PSRAM_A1, PSRAM_A2, PSRAM_A3, PSRAM_A4, PSRAM_A5, 00708 PSRAM_A6, PSRAM_A7, PSRAM_A8, PSRAM_A9 */ 00709 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 |\ 00710 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00711 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00712 00713 /* GPIOG configuration */ 00714 /* PSRAM_A10, PSRAM_A11, PSRAM_A12, PSRAM_A13, PSRAM_A14, PSRAM_A15, LCD_NE */ 00715 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 |\ 00716 GPIO_PIN_9 ; 00717 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00718 } 00719 00720 00721 /** 00722 * @brief Initializes LCD IO. 00723 * @retval None 00724 */ 00725 static void FMC_BANK2_Init(void) 00726 { 00727 SRAM_HandleTypeDef hsram; 00728 FMC_NORSRAM_TimingTypeDef sram_timing; 00729 00730 /* GPIO configuration for FMC signals (LCD) */ 00731 FMC_BANK2_MspInit(); 00732 00733 /* PSRAM device configuration */ 00734 hsram.Instance = FMC_NORSRAM_DEVICE; 00735 hsram.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00736 00737 /* Set parameters for LCD access (FMC_NORSRAM_BANK2) */ 00738 hsram.Init.NSBank = FMC_NORSRAM_BANK2; 00739 hsram.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00740 hsram.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00741 hsram.Init.MemoryDataWidth = FMC_NORSRAM_MEM_BUS_WIDTH_16; 00742 hsram.Init.BurstAccessMode = FMC_BURST_ACCESS_MODE_DISABLE; 00743 hsram.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00744 hsram.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00745 hsram.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00746 hsram.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00747 hsram.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00748 hsram.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00749 hsram.Init.WriteBurst = FMC_WRITE_BURST_DISABLE; 00750 hsram.Init.WriteFifo = FMC_WRITE_FIFO_DISABLE; 00751 hsram.Init.PageSize = FMC_PAGE_SIZE_NONE; 00752 hsram.Init.ContinuousClock = FMC_CONTINUOUS_CLOCK_SYNC_ONLY; 00753 00754 /* PSRAM device configuration */ 00755 /* Timing configuration derived from system clock (up to 216Mhz) 00756 for 108Mhz as PSRAM clock frequency */ 00757 sram_timing.AddressSetupTime = 9; 00758 sram_timing.AddressHoldTime = 2; 00759 sram_timing.DataSetupTime = 6; 00760 sram_timing.BusTurnAroundDuration = 1; 00761 sram_timing.CLKDivision = 2; 00762 sram_timing.DataLatency = 2; 00763 sram_timing.AccessMode = FMC_ACCESS_MODE_A; 00764 00765 /* Initialize the FMC controller for LCD (FMC_NORSRAM_BANK2) */ 00766 HAL_SRAM_Init(&hsram, &sram_timing, &sram_timing); 00767 } 00768 00769 /** 00770 * @brief Writes register value. 00771 * @param Data: Data to be written 00772 * @retval None 00773 */ 00774 static void FMC_BANK2_WriteData(uint16_t Data) 00775 { 00776 /* Write 16-bit Reg */ 00777 FMC_BANK2->RAM = Data; 00778 __DSB(); 00779 } 00780 00781 /** 00782 * @brief Writes register address. 00783 * @param Reg: Register to be written 00784 * @retval None 00785 */ 00786 static void FMC_BANK2_WriteReg(uint8_t Reg) 00787 { 00788 /* Write 16-bit Index, then write register */ 00789 FMC_BANK2->REG = Reg; 00790 __DSB(); 00791 } 00792 00793 /** 00794 * @brief Reads register value. 00795 * @retval Read value 00796 */ 00797 static uint16_t FMC_BANK2_ReadData(void) 00798 { 00799 return FMC_BANK2->RAM; 00800 } 00801 00802 /******************************************************************************* 00803 LINK OPERATIONS 00804 *******************************************************************************/ 00805 00806 /********************************* LINK LCD ***********************************/ 00807 00808 /** 00809 * @brief Initializes LCD low level. 00810 * @retval None 00811 */ 00812 void LCD_IO_Init(void) 00813 { 00814 FMC_BANK2_Init(); 00815 } 00816 00817 /** 00818 * @brief Writes data on LCD data register. 00819 * @param RegValue: Register value to be written 00820 * @retval None 00821 */ 00822 void LCD_IO_WriteData(uint16_t RegValue) 00823 { 00824 /* Write 16-bit Reg */ 00825 FMC_BANK2_WriteData(RegValue); 00826 __DSB(); 00827 } 00828 00829 /** 00830 * @brief Writes several data on LCD data register. 00831 * @param pData: pointer on data to be written 00832 * @param Size: data amount in 16bits short unit 00833 * @retval None 00834 */ 00835 void LCD_IO_WriteMultipleData(uint16_t *pData, uint32_t Size) 00836 { 00837 uint32_t i; 00838 00839 for (i = 0; i < Size; i++) 00840 { 00841 FMC_BANK2_WriteData(pData[i]); 00842 __DSB(); 00843 } 00844 } 00845 00846 /** 00847 * @brief Writes register on LCD register. 00848 * @param Reg: Register to be written 00849 * @retval None 00850 */ 00851 void LCD_IO_WriteReg(uint8_t Reg) 00852 { 00853 /* Write 16-bit Index, then Write Reg */ 00854 FMC_BANK2_WriteReg(Reg); 00855 __DSB(); 00856 } 00857 00858 /** 00859 * @brief Reads data from LCD data register. 00860 * @retval Read data. 00861 */ 00862 uint16_t LCD_IO_ReadData(void) 00863 { 00864 return FMC_BANK2_ReadData(); 00865 } 00866 00867 /** 00868 * @brief LCD delay 00869 * @param Delay: Delay in ms 00870 * @retval None 00871 */ 00872 void LCD_IO_Delay(uint32_t Delay) 00873 { 00874 HAL_Delay(Delay); 00875 } 00876 00877 /********************************* LINK AUDIO *********************************/ 00878 00879 /** 00880 * @brief Initializes Audio low level. 00881 */ 00882 void AUDIO_IO_Init(void) 00883 { 00884 I2Cx_Init(&hI2cAudioHandler); 00885 } 00886 00887 /** 00888 * @brief DeInitializes Audio low level. 00889 */ 00890 void AUDIO_IO_DeInit(void) 00891 { 00892 00893 } 00894 00895 /** 00896 * @brief Writes a single data. 00897 * @param Addr: I2C address 00898 * @param Reg: Reg address 00899 * @param Value: Data to be written 00900 * @retval None 00901 */ 00902 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 00903 { 00904 uint16_t tmp = Value; 00905 00906 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 00907 00908 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 00909 00910 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 00911 } 00912 00913 /** 00914 * @brief Reads a single data. 00915 * @param Addr: I2C address 00916 * @param Reg: Reg address 00917 * @retval Data to be read 00918 */ 00919 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 00920 { 00921 uint16_t read_value = 0, tmp = 0; 00922 00923 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 00924 00925 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 00926 00927 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 00928 00929 read_value = tmp; 00930 00931 return read_value; 00932 } 00933 00934 /** 00935 * @brief AUDIO Codec delay 00936 * @param Delay: Delay in ms 00937 */ 00938 void AUDIO_IO_Delay(uint32_t Delay) 00939 { 00940 HAL_Delay(Delay); 00941 } 00942 00943 /******************************** LINK TS (TouchScreen) ***********************/ 00944 00945 /** 00946 * @brief Initializes Touchscreen low level. 00947 * @retval None 00948 */ 00949 void TS_IO_Init(void) 00950 { 00951 TS_RESET_GPIO_CLK_ENABLE(); 00952 GPIO_InitTypeDef gpio_init_structure; 00953 /* Configure Button pin as input */ 00954 gpio_init_structure.Pin = TS_RESET_PIN; 00955 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00956 gpio_init_structure.Pull = GPIO_NOPULL; 00957 gpio_init_structure.Speed = GPIO_SPEED_FREQ_LOW; 00958 HAL_GPIO_Init(TS_RESET_GPIO_PORT, &gpio_init_structure); 00959 HAL_GPIO_WritePin(TS_RESET_GPIO_PORT, TS_RESET_PIN, GPIO_PIN_SET); 00960 I2Cx_Init(&hI2cTsHandler); 00961 } 00962 00963 /** 00964 * @brief Writes a single data. 00965 * @param Addr: I2C address 00966 * @param Reg: Reg address 00967 * @param Value: Data to be written 00968 * @retval None 00969 */ 00970 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00971 { 00972 I2Cx_WriteMultiple(&hI2cTsHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00973 } 00974 00975 /** 00976 * @brief Reads a single data. 00977 * @param Addr: I2C address 00978 * @param Reg: Reg address 00979 * @retval Data to be read 00980 */ 00981 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 00982 { 00983 uint8_t read_value = 0; 00984 00985 I2Cx_ReadMultiple(&hI2cTsHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00986 00987 return read_value; 00988 } 00989 00990 /** 00991 * @brief Reads multiple data with I2C communication 00992 * channel from TouchScreen. 00993 * @param Addr: I2C address 00994 * @param Reg: Register address 00995 * @param Buffer: Pointer to data buffer 00996 * @param Length: Length of the data 00997 * @retval Number of read data 00998 */ 00999 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01000 { 01001 return I2Cx_ReadMultiple(&hI2cTsHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01002 } 01003 01004 /** 01005 * @brief Writes multiple data with I2C communication 01006 * channel from MCU to TouchScreen. 01007 * @param Addr: I2C address 01008 * @param Reg: Register address 01009 * @param Buffer: Pointer to data buffer 01010 * @param Length: Length of the data 01011 * @retval None 01012 */ 01013 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01014 { 01015 I2Cx_WriteMultiple(&hI2cTsHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01016 } 01017 01018 /** 01019 * @brief Delay function used in TouchScreen low level driver. 01020 * @param Delay: Delay in ms 01021 * @retval None 01022 */ 01023 void TS_IO_Delay(uint32_t Delay) 01024 { 01025 HAL_Delay(Delay); 01026 } 01027 01028 /** 01029 * @} 01030 */ 01031 01032 /** 01033 * @} 01034 */ 01035 01036 /** 01037 * @} 01038 */ 01039 01040 /** 01041 * @} 01042 */ 01043 01044 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Jan 2 2017 09:52:50 for STM32F723E-Discovery BSP User Manual by
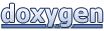