STM32F469I-Discovery BSP User Manual
|
stm32469i_discovery_sdram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_discovery_sdram.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 13-January-2016 00007 * @brief This file includes the SDRAM driver for the MT48LC4M32B2B5-7 memory 00008 * device mounted on STM32469I-Discovery board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the MT48LC4M32B2B5-7 SDRAM external memory mounted 00044 on STM32469I-Discovery board. 00045 - This driver does not need a specific component driver for the SDRAM device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the SDRAM external memory using the BSP_SDRAM_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 FMC controller configuration to interface with the external SDRAM memory. 00054 o It contains the SDRAM initialization sequence to program the SDRAM external 00055 device using the function BSP_SDRAM_Initialization_sequence(). Note that this 00056 sequence is standard for all SDRAM devices, but can include some differences 00057 from a device to another. If it is the case, the right sequence should be 00058 implemented separately. 00059 00060 + SDRAM read/write operations 00061 o SDRAM external memory can be accessed with read/write operations once it is 00062 initialized. 00063 Read/write operation can be performed with AHB access using the functions 00064 BSP_SDRAM_ReadData()/BSP_SDRAM_WriteData(), or by DMA transfer using the functions 00065 BSP_SDRAM_ReadData_DMA()/BSP_SDRAM_WriteData_DMA(). 00066 o The AHB access is performed with 32-bit width transaction, the DMA transfer 00067 configuration is fixed at single (no burst) word transfer (see the 00068 BSP_SDRAM_MspInit() weak function). 00069 o User can implement his own functions for read/write access with his desired 00070 configurations. 00071 o If interrupt mode is used for DMA transfer, the function BSP_SDRAM_DMA_IRQHandler() 00072 is called in IRQ handler file, to serve the generated interrupt once the DMA 00073 transfer is complete. 00074 o You can send a command to the SDRAM device in runtime using the function 00075 BSP_SDRAM_Sendcmd(), and giving the desired command as parameter chosen between 00076 the predefined commands of the "FMC_SDRAM_CommandTypeDef" structure. 00077 00078 ------------------------------------------------------------------------------*/ 00079 00080 /* Includes ------------------------------------------------------------------*/ 00081 #include "stm32469i_discovery_sdram.h" 00082 00083 /** @addtogroup BSP 00084 * @{ 00085 */ 00086 00087 /** @addtogroup STM32469I_Discovery 00088 * @{ 00089 */ 00090 00091 /** @defgroup STM32469I-Discovery_SDRAM STM32469I Discovery SDRAM 00092 * @{ 00093 */ 00094 00095 /** @defgroup STM32469I-Discovery_SDRAM_Private_Types_Definitions STM32469I Discovery SDRAM Private TypesDef 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM32469I-Discovery_SDRAM_Private_Defines STM32469I Discovery SDRAM Private Defines 00103 * @{ 00104 */ 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM32469I-Discovery_SDRAM_Private_Macros STM32469I Discovery SDRAM Private Macros 00110 * @{ 00111 */ 00112 /** 00113 * @} 00114 */ 00115 00116 /** @defgroup STM32469I-Discovery_SDRAM_Private_Variables STM32469I Discovery SDRAM Private Variables 00117 * @{ 00118 */ 00119 static SDRAM_HandleTypeDef sdramHandle; 00120 static FMC_SDRAM_TimingTypeDef Timing; 00121 static FMC_SDRAM_CommandTypeDef Command; 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM32469I-Discovery_SDRAM_Private_Function_Prototypes STM32469I Discovery SDRAM Private Prototypes 00127 * @{ 00128 */ 00129 00130 /** 00131 * @} 00132 */ 00133 00134 /** @defgroup STM32469I-Discovery_SDRAM_Private_Functions STM32469I Discovery SDRAM Private Functions 00135 * @{ 00136 */ 00137 00138 /** 00139 * @} 00140 */ 00141 00142 /** @defgroup STM32469I_Discovery_SDRAM_Exported_Functions STM32469I Discovery SDRAM Exported Functions 00143 * @{ 00144 */ 00145 00146 /** 00147 * @brief Initializes the SDRAM device. 00148 * @retval SDRAM status 00149 */ 00150 uint8_t BSP_SDRAM_Init(void) 00151 { 00152 static uint8_t sdramstatus = SDRAM_ERROR; 00153 00154 /* SDRAM device configuration */ 00155 sdramHandle.Instance = FMC_SDRAM_DEVICE; 00156 00157 /* Timing configuration for 90 MHz as SD clock frequency (System clock is up to 180 MHz) */ 00158 Timing.LoadToActiveDelay = 2; 00159 Timing.ExitSelfRefreshDelay = 7; 00160 Timing.SelfRefreshTime = 4; 00161 Timing.RowCycleDelay = 7; 00162 Timing.WriteRecoveryTime = 2; 00163 Timing.RPDelay = 2; 00164 Timing.RCDDelay = 2; 00165 00166 sdramHandle.Init.SDBank = FMC_SDRAM_BANK1; 00167 sdramHandle.Init.ColumnBitsNumber = FMC_SDRAM_COLUMN_BITS_NUM_8; 00168 sdramHandle.Init.RowBitsNumber = FMC_SDRAM_ROW_BITS_NUM_11; 00169 sdramHandle.Init.MemoryDataWidth = SDRAM_MEMORY_WIDTH; 00170 sdramHandle.Init.InternalBankNumber = FMC_SDRAM_INTERN_BANKS_NUM_4; 00171 sdramHandle.Init.CASLatency = FMC_SDRAM_CAS_LATENCY_3; 00172 sdramHandle.Init.WriteProtection = FMC_SDRAM_WRITE_PROTECTION_DISABLE; 00173 sdramHandle.Init.SDClockPeriod = SDCLOCK_PERIOD; 00174 sdramHandle.Init.ReadBurst = FMC_SDRAM_RBURST_ENABLE; 00175 sdramHandle.Init.ReadPipeDelay = FMC_SDRAM_RPIPE_DELAY_0; 00176 00177 /* SDRAM controller initialization */ 00178 /* __weak function can be surcharged by the application code */ 00179 BSP_SDRAM_MspInit(&sdramHandle, (void *)NULL); 00180 if(HAL_SDRAM_Init(&sdramHandle, &Timing) != HAL_OK) 00181 { 00182 sdramstatus = SDRAM_ERROR; 00183 } 00184 else 00185 { 00186 sdramstatus = SDRAM_OK; 00187 } 00188 00189 /* SDRAM initialization sequence */ 00190 BSP_SDRAM_Initialization_sequence(REFRESH_COUNT); 00191 00192 return sdramstatus; 00193 } 00194 00195 /** 00196 * @brief DeInitializes the SDRAM device. 00197 * @retval SDRAM status : SDRAM_OK or SDRAM_ERROR. 00198 */ 00199 uint8_t BSP_SDRAM_DeInit(void) 00200 { 00201 static uint8_t sdramstatus = SDRAM_ERROR; 00202 00203 /* SDRAM device configuration */ 00204 sdramHandle.Instance = FMC_SDRAM_DEVICE; 00205 00206 if(HAL_SDRAM_DeInit(&sdramHandle) == HAL_OK) 00207 { 00208 sdramstatus = SDRAM_OK; 00209 00210 /* SDRAM controller De-initialization */ 00211 BSP_SDRAM_MspDeInit(&sdramHandle, (void *)NULL); 00212 } 00213 00214 return sdramstatus; 00215 } 00216 00217 00218 /** 00219 * @brief Programs the SDRAM device. 00220 * @param RefreshCount: SDRAM refresh counter value 00221 */ 00222 void BSP_SDRAM_Initialization_sequence(uint32_t RefreshCount) 00223 { 00224 __IO uint32_t tmpmrd = 0; 00225 00226 /* Step 1: Configure a clock configuration enable command */ 00227 Command.CommandMode = FMC_SDRAM_CMD_CLK_ENABLE; 00228 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00229 Command.AutoRefreshNumber = 1; 00230 Command.ModeRegisterDefinition = 0; 00231 00232 /* Send the command */ 00233 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00234 00235 /* Step 2: Insert 100 us minimum delay */ 00236 /* Inserted delay is equal to 1 ms due to systick time base unit (ms) */ 00237 HAL_Delay(1); 00238 00239 /* Step 3: Configure a PALL (precharge all) command */ 00240 Command.CommandMode = FMC_SDRAM_CMD_PALL; 00241 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00242 Command.AutoRefreshNumber = 1; 00243 Command.ModeRegisterDefinition = 0; 00244 00245 /* Send the command */ 00246 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00247 00248 /* Step 4: Configure an Auto Refresh command */ 00249 Command.CommandMode = FMC_SDRAM_CMD_AUTOREFRESH_MODE; 00250 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00251 Command.AutoRefreshNumber = 8; 00252 Command.ModeRegisterDefinition = 0; 00253 00254 /* Send the command */ 00255 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00256 00257 /* Step 5: Program the external memory mode register */ 00258 tmpmrd = (uint32_t)SDRAM_MODEREG_BURST_LENGTH_1 |\ 00259 SDRAM_MODEREG_BURST_TYPE_SEQUENTIAL |\ 00260 SDRAM_MODEREG_CAS_LATENCY_3 |\ 00261 SDRAM_MODEREG_OPERATING_MODE_STANDARD |\ 00262 SDRAM_MODEREG_WRITEBURST_MODE_SINGLE; 00263 00264 Command.CommandMode = FMC_SDRAM_CMD_LOAD_MODE; 00265 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00266 Command.AutoRefreshNumber = 1; 00267 Command.ModeRegisterDefinition = tmpmrd; 00268 00269 /* Send the command */ 00270 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00271 00272 /* Step 6: Set the refresh rate counter */ 00273 /* Set the device refresh rate */ 00274 HAL_SDRAM_ProgramRefreshRate(&sdramHandle, RefreshCount); 00275 } 00276 00277 /** 00278 * @brief Reads an mount of data from the SDRAM memory in polling mode. 00279 * @param uwStartAddress: Read start address 00280 * @param pData: Pointer to data to be read 00281 * @param uwDataSize: Size of read data from the memory 00282 * @retval SDRAM status : SDRAM_OK or SDRAM_ERROR. 00283 */ 00284 uint8_t BSP_SDRAM_ReadData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00285 { 00286 if(HAL_SDRAM_Read_32b(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00287 { 00288 return SDRAM_ERROR; 00289 } 00290 else 00291 { 00292 return SDRAM_OK; 00293 } 00294 } 00295 00296 /** 00297 * @brief Reads an mount of data from the SDRAM memory in DMA mode. 00298 * @param uwStartAddress: Read start address 00299 * @param pData: Pointer to data to be read 00300 * @param uwDataSize: Size of read data from the memory 00301 * @retval SDRAM status : SDRAM_OK or SDRAM_ERROR. 00302 */ 00303 uint8_t BSP_SDRAM_ReadData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00304 { 00305 if(HAL_SDRAM_Read_DMA(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00306 { 00307 return SDRAM_ERROR; 00308 } 00309 else 00310 { 00311 return SDRAM_OK; 00312 } 00313 } 00314 00315 /** 00316 * @brief Writes an mount of data to the SDRAM memory in polling mode. 00317 * @param uwStartAddress: Write start address 00318 * @param pData: Pointer to data to be written 00319 * @param uwDataSize: Size of written data from the memory 00320 * @retval SDRAM status : SDRAM_OK or SDRAM_ERROR. 00321 */ 00322 uint8_t BSP_SDRAM_WriteData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00323 { 00324 if(HAL_SDRAM_Write_32b(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00325 { 00326 return SDRAM_ERROR; 00327 } 00328 else 00329 { 00330 return SDRAM_OK; 00331 } 00332 } 00333 00334 /** 00335 * @brief Writes an mount of data to the SDRAM memory in DMA mode. 00336 * @param uwStartAddress: Write start address 00337 * @param pData: Pointer to data to be written 00338 * @param uwDataSize: Size of written data from the memory 00339 * @retval SDRAM status : SDRAM_OK or SDRAM_ERROR. 00340 */ 00341 uint8_t BSP_SDRAM_WriteData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00342 { 00343 if(HAL_SDRAM_Write_DMA(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00344 { 00345 return SDRAM_ERROR; 00346 } 00347 else 00348 { 00349 return SDRAM_OK; 00350 } 00351 } 00352 00353 /** 00354 * @brief Sends command to the SDRAM bank. 00355 * @param SdramCmd: Pointer to SDRAM command structure 00356 * @retval HAL status : SDRAM_OK or SDRAM_ERROR. 00357 */ 00358 uint8_t BSP_SDRAM_Sendcmd(FMC_SDRAM_CommandTypeDef *SdramCmd) 00359 { 00360 if(HAL_SDRAM_SendCommand(&sdramHandle, SdramCmd, SDRAM_TIMEOUT) != HAL_OK) 00361 { 00362 return SDRAM_ERROR; 00363 } 00364 else 00365 { 00366 return SDRAM_OK; 00367 } 00368 } 00369 00370 /** 00371 * @brief Handles SDRAM DMA transfer interrupt request. 00372 */ 00373 void BSP_SDRAM_DMA_IRQHandler(void) 00374 { 00375 HAL_DMA_IRQHandler(sdramHandle.hdma); 00376 } 00377 00378 /** 00379 * @brief Initializes SDRAM MSP. 00380 * @note This function can be surcharged by application code. 00381 * @param hsdram: pointer on SDRAM handle 00382 * @param Params: pointer on additional configuration parameters, can be NULL. 00383 */ 00384 __weak void BSP_SDRAM_MspInit(SDRAM_HandleTypeDef *hsdram, void *Params) 00385 { 00386 static DMA_HandleTypeDef dma_handle; 00387 GPIO_InitTypeDef gpio_init_structure; 00388 00389 if(hsdram != (SDRAM_HandleTypeDef *)NULL) 00390 { 00391 /* Enable FMC clock */ 00392 __HAL_RCC_FMC_CLK_ENABLE(); 00393 00394 /* Enable chosen DMAx clock */ 00395 __DMAx_CLK_ENABLE(); 00396 00397 /* Enable GPIOs clock */ 00398 __HAL_RCC_GPIOC_CLK_ENABLE(); 00399 __HAL_RCC_GPIOD_CLK_ENABLE(); 00400 __HAL_RCC_GPIOE_CLK_ENABLE(); 00401 __HAL_RCC_GPIOF_CLK_ENABLE(); 00402 __HAL_RCC_GPIOG_CLK_ENABLE(); 00403 __HAL_RCC_GPIOH_CLK_ENABLE(); 00404 __HAL_RCC_GPIOI_CLK_ENABLE(); 00405 00406 /* Common GPIO configuration */ 00407 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00408 gpio_init_structure.Pull = GPIO_PULLUP; 00409 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00410 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00411 00412 /* GPIOC configuration : PC0 is SDNWE */ 00413 gpio_init_structure.Pin = GPIO_PIN_0; 00414 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00415 00416 /* GPIOD configuration */ 00417 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8| GPIO_PIN_9 | GPIO_PIN_10 |\ 00418 GPIO_PIN_14 | GPIO_PIN_15; 00419 00420 00421 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00422 00423 /* GPIOE configuration */ 00424 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7| GPIO_PIN_8 | GPIO_PIN_9 |\ 00425 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00426 GPIO_PIN_15; 00427 00428 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00429 00430 /* GPIOF configuration */ 00431 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00432 GPIO_PIN_5 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00433 GPIO_PIN_15; 00434 00435 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00436 00437 /* GPIOG configuration */ 00438 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4| GPIO_PIN_5 | GPIO_PIN_8 |\ 00439 GPIO_PIN_15; 00440 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00441 00442 /* GPIOH configuration */ 00443 gpio_init_structure.Pin = GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_8 | GPIO_PIN_9 |\ 00444 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00445 GPIO_PIN_15; 00446 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00447 00448 /* GPIOI configuration */ 00449 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 |\ 00450 GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_9 | GPIO_PIN_10; 00451 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 00452 00453 /* Configure common DMA parameters */ 00454 dma_handle.Init.Channel = SDRAM_DMAx_CHANNEL; 00455 dma_handle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00456 dma_handle.Init.PeriphInc = DMA_PINC_ENABLE; 00457 dma_handle.Init.MemInc = DMA_MINC_ENABLE; 00458 dma_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00459 dma_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00460 dma_handle.Init.Mode = DMA_NORMAL; 00461 dma_handle.Init.Priority = DMA_PRIORITY_HIGH; 00462 dma_handle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00463 dma_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00464 dma_handle.Init.MemBurst = DMA_MBURST_SINGLE; 00465 dma_handle.Init.PeriphBurst = DMA_PBURST_SINGLE; 00466 00467 dma_handle.Instance = SDRAM_DMAx_STREAM; 00468 00469 /* Associate the DMA handle */ 00470 __HAL_LINKDMA(hsdram, hdma, dma_handle); 00471 00472 /* Deinitialize the stream for new transfer */ 00473 HAL_DMA_DeInit(&dma_handle); 00474 00475 /* Configure the DMA stream */ 00476 HAL_DMA_Init(&dma_handle); 00477 00478 /* NVIC configuration for DMA transfer complete interrupt */ 00479 HAL_NVIC_SetPriority(SDRAM_DMAx_IRQn, 5, 0); 00480 HAL_NVIC_EnableIRQ(SDRAM_DMAx_IRQn); 00481 00482 } /* of if(hsdram != (SDRAM_HandleTypeDef *)NULL) */ 00483 } 00484 00485 /** 00486 * @brief DeInitializes SDRAM MSP. 00487 * @note This function can be surcharged by application code. 00488 * @param hsdram: pointer on SDRAM handle 00489 * @param Params: pointer on additional configuration parameters, can be NULL. 00490 */ 00491 __weak void BSP_SDRAM_MspDeInit(SDRAM_HandleTypeDef *hsdram, void *Params) 00492 { 00493 static DMA_HandleTypeDef dma_handle; 00494 00495 if(hsdram != (SDRAM_HandleTypeDef *)NULL) 00496 { 00497 /* Disable NVIC configuration for DMA interrupt */ 00498 HAL_NVIC_DisableIRQ(SDRAM_DMAx_IRQn); 00499 00500 /* Deinitialize the stream for new transfer */ 00501 dma_handle.Instance = SDRAM_DMAx_STREAM; 00502 HAL_DMA_DeInit(&dma_handle); 00503 00504 /* DeInit GPIO pins can be done in the application 00505 (by surcharging this __weak function) */ 00506 00507 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the application 00508 by surcharging this __weak function */ 00509 00510 } /* of if(hsdram != (SDRAM_HandleTypeDef *)NULL) */ 00511 } 00512 00513 /** 00514 * @} 00515 */ 00516 00517 /** 00518 * @} 00519 */ 00520 00521 /** 00522 * @} 00523 */ 00524 00525 /** 00526 * @} 00527 */ 00528 00529 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 10:58:46 for STM32F469I-Discovery BSP User Manual by
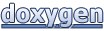