STM32F469I-Discovery BSP User Manual
|
stm32469i_discovery_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_discovery_sd.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 13-January-2016 00007 * @brief This file includes the uSD card driver mounted on STM32469I-Discovery 00008 * board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the micro SD external card mounted on STM32469I-Discovery 00044 board. 00045 - This driver does not need a specific component driver for the micro SD device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the micro SD card using the BSP_SD_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 SDIO interface configuration to interface with the external micro SD. It 00054 also includes the micro SD initialization sequence. 00055 o To check the SD card presence you can use the function BSP_SD_IsDetected() which 00056 returns the detection status 00057 o If SD presence detection interrupt mode is desired, you must configure the 00058 SD detection interrupt mode by calling the function BSP_SD_ITConfig(). The interrupt 00059 is generated as an external interrupt whenever the micro SD card is 00060 plugged/unplugged in/from the discovery board. The SD detection interrupt 00061 is handled by calling the function BSP_SD_DetectIT() which is called in the IRQ 00062 handler file, the user callback is implemented in the function BSP_SD_DetectCallback(). 00063 o The function BSP_SD_GetCardInfo() is used to get the micro SD card information 00064 which is stored in the structure "HAL_SD_CardInfoTypedef". 00065 00066 + Micro SD card operations 00067 o The micro SD card can be accessed with read/write block(s) operations once 00068 it is reay for access. The access cand be performed whether using the polling 00069 mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), or by DMA 00070 transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA() 00071 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00072 is complete, the SD interrupt is handeled using the function BSP_SD_IRQHandler(), 00073 the DMA Tx/Rx transfer complete are handeled using the functions 00074 BSP_SD_DMA_Tx_IRQHandler()/BSP_SD_DMA_Rx_IRQHandler(). The corresponding user callbacks 00075 are implemented by the user at application level. 00076 o The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying 00077 the number of blocks to erase. 00078 o The SD runtime status is returned when calling the function BSP_SD_GetStatus(). 00079 00080 ------------------------------------------------------------------------------*/ 00081 00082 /* Includes ------------------------------------------------------------------*/ 00083 #include "stm32469i_discovery_sd.h" 00084 00085 /** @addtogroup BSP 00086 * @{ 00087 */ 00088 00089 /** @addtogroup STM32469I_Discovery 00090 * @{ 00091 */ 00092 00093 /** @defgroup STM32469I-Discovery_SD STM32469I Discovery SD 00094 * @{ 00095 */ 00096 00097 00098 /** @defgroup STM32469I-Discovery_SD_Private_TypesDefinitions STM32469I Discovery SD Private TypesDef 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32469I-Discovery_SD_Private_Defines STM32469I Discovery SD Private Defines 00106 * @{ 00107 */ 00108 /** 00109 * @} 00110 */ 00111 00112 /** @defgroup STM32469I-Discovery_SD_Private_Macros STM32469I Discovery SD Private Macro 00113 * @{ 00114 */ 00115 /** 00116 * @} 00117 */ 00118 00119 /** @defgroup STM32469I-Discovery_SD_Private_Variables STM32469I Discovery SD Private Variables 00120 * @{ 00121 */ 00122 static SD_HandleTypeDef uSdHandle; 00123 static SD_CardInfo uSdCardInfo; 00124 00125 /** 00126 * @} 00127 */ 00128 00129 /** @defgroup STM32469I-Discovery_SD_Private_FunctionPrototypes STM32469I Discovery SD Private Prototypes 00130 * @{ 00131 */ 00132 /** 00133 * @} 00134 */ 00135 00136 /** @defgroup STM32469I-Discovery_SD_Private_Functions STM32469I Discovery SD Private Functions 00137 * @{ 00138 */ 00139 00140 /** 00141 * @brief Initializes the SD card device. 00142 * @retval SD status 00143 */ 00144 uint8_t BSP_SD_Init(void) 00145 { 00146 uint8_t sd_state = MSD_OK; 00147 00148 /* PLLSAI is dedicated to LCD periph. Do not use it to get 48MHz*/ 00149 00150 /* uSD device interface configuration */ 00151 uSdHandle.Instance = SDIO; 00152 00153 uSdHandle.Init.ClockEdge = SDIO_CLOCK_EDGE_RISING; 00154 uSdHandle.Init.ClockBypass = SDIO_CLOCK_BYPASS_DISABLE; 00155 uSdHandle.Init.ClockPowerSave = SDIO_CLOCK_POWER_SAVE_DISABLE; 00156 uSdHandle.Init.BusWide = SDIO_BUS_WIDE_1B; 00157 uSdHandle.Init.HardwareFlowControl = SDIO_HARDWARE_FLOW_CONTROL_ENABLE; 00158 uSdHandle.Init.ClockDiv = SDIO_TRANSFER_CLK_DIV; 00159 00160 /* Msp SD Detect pin initialization */ 00161 BSP_SD_Detect_MspInit(&uSdHandle, NULL); 00162 if(BSP_SD_IsDetected() != SD_PRESENT) /* Check if SD card is present */ 00163 { 00164 return MSD_ERROR_SD_NOT_PRESENT; 00165 } 00166 00167 /* Msp SD initialization */ 00168 BSP_SD_MspInit(&uSdHandle, NULL); 00169 00170 /* HAL SD initialization */ 00171 if(HAL_SD_Init(&uSdHandle, &uSdCardInfo) != SD_OK) 00172 { 00173 sd_state = MSD_ERROR; 00174 } 00175 00176 /* Configure SD Bus width */ 00177 if(sd_state == MSD_OK) 00178 { 00179 /* Enable wide operation */ 00180 if(HAL_SD_WideBusOperation_Config(&uSdHandle, SDIO_BUS_WIDE_4B) != SD_OK) 00181 { 00182 sd_state = MSD_ERROR; 00183 } 00184 else 00185 { 00186 sd_state = MSD_OK; 00187 } 00188 } 00189 return sd_state; 00190 } 00191 00192 /** 00193 * @brief DeInitializes the SD card device. 00194 * @retval SD status 00195 */ 00196 uint8_t BSP_SD_DeInit(void) 00197 { 00198 uint8_t sd_state = MSD_OK; 00199 00200 uSdHandle.Instance = SDIO; 00201 00202 /* HAL SD deinitialization */ 00203 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00204 { 00205 sd_state = MSD_ERROR; 00206 } 00207 00208 /* Msp SD deinitialization */ 00209 uSdHandle.Instance = SDIO; 00210 BSP_SD_MspDeInit(&uSdHandle, NULL); 00211 00212 return sd_state; 00213 } 00214 00215 /** 00216 * @brief Configures Interrupt mode for SD detection pin. 00217 * @retval Returns 0 00218 */ 00219 uint8_t BSP_SD_ITConfig(void) 00220 { 00221 GPIO_InitTypeDef gpio_init_structure; 00222 00223 /* Configure Interrupt mode for SD detection pin */ 00224 gpio_init_structure.Pin = SD_DETECT_PIN; 00225 gpio_init_structure.Pull = GPIO_PULLUP; 00226 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00227 gpio_init_structure.Mode = GPIO_MODE_IT_RISING_FALLING; 00228 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00229 00230 /* Enable and set SD detect EXTI Interrupt to the lowest priority */ 00231 HAL_NVIC_SetPriority((IRQn_Type)(SD_DETECT_EXTI_IRQn), 0x0F, 0x00); 00232 HAL_NVIC_EnableIRQ((IRQn_Type)(SD_DETECT_EXTI_IRQn)); 00233 00234 return MSD_OK; 00235 } 00236 00237 /** 00238 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00239 * @retval Returns if SD is detected or not 00240 */ 00241 uint8_t BSP_SD_IsDetected(void) 00242 { 00243 __IO uint8_t status = SD_PRESENT; 00244 00245 /* Check SD card detect pin */ 00246 if (HAL_GPIO_ReadPin(SD_DETECT_GPIO_PORT, SD_DETECT_PIN) == GPIO_PIN_SET) 00247 { 00248 status = SD_NOT_PRESENT; 00249 } 00250 00251 return status; 00252 } 00253 00254 00255 00256 /** 00257 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00258 * @param pData: Pointer to the buffer that will contain the data to transmit 00259 * @param ReadAddr: Address from where data is to be read 00260 * @param BlockSize: SD card data block size, that should be 512 00261 * @param NumOfBlocks: Number of SD blocks to read 00262 * @retval SD status 00263 */ 00264 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00265 { 00266 if(HAL_SD_ReadBlocks(&uSdHandle, pData, ReadAddr, BlockSize, NumOfBlocks) != SD_OK) 00267 { 00268 return MSD_ERROR; 00269 } 00270 else 00271 { 00272 return MSD_OK; 00273 } 00274 } 00275 00276 /** 00277 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00278 * @param pData: Pointer to the buffer that will contain the data to transmit 00279 * @param WriteAddr: Address from where data is to be written 00280 * @param BlockSize: SD card data block size, that should be 512 00281 * @param NumOfBlocks: Number of SD blocks to write 00282 * @retval SD status 00283 */ 00284 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00285 { 00286 if(HAL_SD_WriteBlocks(&uSdHandle, pData, WriteAddr, BlockSize, NumOfBlocks) != SD_OK) 00287 { 00288 return MSD_ERROR; 00289 } 00290 else 00291 { 00292 return MSD_OK; 00293 } 00294 } 00295 00296 /** 00297 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00298 * @param pData: Pointer to the buffer that will contain the data to transmit 00299 * @param ReadAddr: Address from where data is to be read 00300 * @param BlockSize: SD card data block size, that should be 512 00301 * @param NumOfBlocks: Number of SD blocks to read 00302 * @retval SD status 00303 */ 00304 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00305 { 00306 uint8_t sd_state = MSD_OK; 00307 00308 /* Read block(s) in DMA transfer mode */ 00309 if(HAL_SD_ReadBlocks_DMA(&uSdHandle, pData, ReadAddr, BlockSize, NumOfBlocks) != SD_OK) 00310 { 00311 sd_state = MSD_ERROR; 00312 } 00313 00314 /* Wait until transfer is complete */ 00315 if(sd_state == MSD_OK) 00316 { 00317 if(HAL_SD_CheckReadOperation(&uSdHandle, (uint32_t)SD_DATATIMEOUT) != SD_OK) 00318 { 00319 sd_state = MSD_ERROR; 00320 } 00321 else 00322 { 00323 sd_state = MSD_OK; 00324 } 00325 } 00326 00327 return sd_state; 00328 } 00329 00330 /** 00331 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00332 * @param pData: Pointer to the buffer that will contain the data to transmit 00333 * @param WriteAddr: Address from where data is to be written 00334 * @param BlockSize: SD card data block size, that should be 512 00335 * @param NumOfBlocks: Number of SD blocks to write 00336 * @retval SD status 00337 */ 00338 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00339 { 00340 uint8_t sd_state = MSD_OK; 00341 00342 /* Write block(s) in DMA transfer mode */ 00343 if(HAL_SD_WriteBlocks_DMA(&uSdHandle, pData, WriteAddr, BlockSize, NumOfBlocks) != SD_OK) 00344 { 00345 sd_state = MSD_ERROR; 00346 } 00347 00348 /* Wait until transfer is complete */ 00349 if(sd_state == MSD_OK) 00350 { 00351 if(HAL_SD_CheckWriteOperation(&uSdHandle, (uint32_t)SD_DATATIMEOUT) != SD_OK) 00352 { 00353 sd_state = MSD_ERROR; 00354 } 00355 else 00356 { 00357 sd_state = MSD_OK; 00358 } 00359 } 00360 00361 return sd_state; 00362 } 00363 00364 /** 00365 * @brief Erases the specified memory area of the given SD card. 00366 * @param StartAddr: Start byte address 00367 * @param EndAddr: End byte address 00368 * @retval SD status 00369 */ 00370 uint8_t BSP_SD_Erase(uint64_t StartAddr, uint64_t EndAddr) 00371 { 00372 if(HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) != SD_OK) 00373 { 00374 return MSD_ERROR; 00375 } 00376 else 00377 { 00378 return MSD_OK; 00379 } 00380 } 00381 00382 /** 00383 * @brief Initializes the SD MSP. 00384 * @param hsd: SD handle 00385 * @param Params : pointer on additional configuration parameters, can be NULL. 00386 */ 00387 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00388 { 00389 static DMA_HandleTypeDef dma_rx_handle; 00390 static DMA_HandleTypeDef dma_tx_handle; 00391 GPIO_InitTypeDef gpio_init_structure; 00392 00393 /* Enable SDIO clock */ 00394 __HAL_RCC_SDIO_CLK_ENABLE(); 00395 00396 /* Enable DMA2 clocks */ 00397 __DMAx_TxRx_CLK_ENABLE(); 00398 00399 /* Enable GPIOs clock */ 00400 __HAL_RCC_GPIOC_CLK_ENABLE(); 00401 __HAL_RCC_GPIOD_CLK_ENABLE(); 00402 00403 /* Common GPIO configuration */ 00404 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00405 gpio_init_structure.Pull = GPIO_PULLUP; 00406 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00407 gpio_init_structure.Alternate = GPIO_AF12_SDIO; 00408 00409 /* GPIOC configuration */ 00410 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12; 00411 00412 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00413 00414 /* GPIOD configuration */ 00415 gpio_init_structure.Pin = GPIO_PIN_2; 00416 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00417 00418 /* NVIC configuration for SDIO interrupts */ 00419 HAL_NVIC_SetPriority(SDIO_IRQn, 5, 0); 00420 HAL_NVIC_EnableIRQ(SDIO_IRQn); 00421 00422 /* Configure DMA Rx parameters */ 00423 dma_rx_handle.Init.Channel = SD_DMAx_Rx_CHANNEL; 00424 dma_rx_handle.Init.Direction = DMA_PERIPH_TO_MEMORY; 00425 dma_rx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00426 dma_rx_handle.Init.MemInc = DMA_MINC_ENABLE; 00427 dma_rx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00428 dma_rx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00429 dma_rx_handle.Init.Mode = DMA_PFCTRL; 00430 dma_rx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00431 dma_rx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00432 dma_rx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00433 dma_rx_handle.Init.MemBurst = DMA_MBURST_INC4; 00434 dma_rx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00435 00436 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00437 00438 /* Associate the DMA handle */ 00439 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle); 00440 00441 /* Deinitialize the stream for new transfer */ 00442 HAL_DMA_DeInit(&dma_rx_handle); 00443 00444 /* Configure the DMA stream */ 00445 HAL_DMA_Init(&dma_rx_handle); 00446 00447 /* Configure DMA Tx parameters */ 00448 dma_tx_handle.Init.Channel = SD_DMAx_Tx_CHANNEL; 00449 dma_tx_handle.Init.Direction = DMA_MEMORY_TO_PERIPH; 00450 dma_tx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00451 dma_tx_handle.Init.MemInc = DMA_MINC_ENABLE; 00452 dma_tx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00453 dma_tx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00454 dma_tx_handle.Init.Mode = DMA_PFCTRL; 00455 dma_tx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00456 dma_tx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00457 dma_tx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00458 dma_tx_handle.Init.MemBurst = DMA_MBURST_INC4; 00459 dma_tx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00460 00461 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00462 00463 /* Associate the DMA handle */ 00464 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle); 00465 00466 /* Deinitialize the stream for new transfer */ 00467 HAL_DMA_DeInit(&dma_tx_handle); 00468 00469 /* Configure the DMA stream */ 00470 HAL_DMA_Init(&dma_tx_handle); 00471 00472 /* NVIC configuration for DMA transfer complete interrupt */ 00473 HAL_NVIC_SetPriority(SD_DMAx_Rx_IRQn, 6, 0); 00474 HAL_NVIC_EnableIRQ(SD_DMAx_Rx_IRQn); 00475 00476 /* NVIC configuration for DMA transfer complete interrupt */ 00477 HAL_NVIC_SetPriority(SD_DMAx_Tx_IRQn, 6, 0); 00478 HAL_NVIC_EnableIRQ(SD_DMAx_Tx_IRQn); 00479 } 00480 00481 /** 00482 * @brief Initializes the SD Detect pin MSP. 00483 * @param hsd: SD handle 00484 * @param Params : pointer on additional configuration parameters, can be NULL. 00485 */ 00486 __weak void BSP_SD_Detect_MspInit(SD_HandleTypeDef *hsd, void *Params) 00487 { 00488 GPIO_InitTypeDef gpio_init_structure; 00489 00490 SD_DETECT_GPIO_CLK_ENABLE(); 00491 00492 /* GPIO configuration in input for uSD_Detect signal */ 00493 gpio_init_structure.Pin = SD_DETECT_PIN; 00494 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00495 gpio_init_structure.Pull = GPIO_PULLUP; 00496 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00497 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00498 } 00499 00500 /** 00501 * @brief DeInitializes the SD MSP. 00502 * @param hsd: SD handle 00503 * @param Params : pointer on additional configuration parameters, can be NULL. 00504 */ 00505 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00506 { 00507 static DMA_HandleTypeDef dma_rx_handle; 00508 static DMA_HandleTypeDef dma_tx_handle; 00509 00510 /* Disable NVIC for DMA transfer complete interrupts */ 00511 HAL_NVIC_DisableIRQ(SD_DMAx_Rx_IRQn); 00512 HAL_NVIC_DisableIRQ(SD_DMAx_Tx_IRQn); 00513 00514 /* Deinitialize the stream for new transfer */ 00515 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00516 HAL_DMA_DeInit(&dma_rx_handle); 00517 00518 /* Deinitialize the stream for new transfer */ 00519 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00520 HAL_DMA_DeInit(&dma_tx_handle); 00521 00522 /* Disable NVIC for SDIO interrupts */ 00523 HAL_NVIC_DisableIRQ(SDIO_IRQn); 00524 00525 /* DeInit GPIO pins can be done in the application 00526 (by surcharging this __weak function) */ 00527 00528 /* Disable SDIO clock */ 00529 __HAL_RCC_SDIO_CLK_DISABLE(); 00530 00531 /* GPOI pins clock and DMA cloks can be shut down in the applic 00532 by surcgarging this __weak function */ 00533 } 00534 00535 /** 00536 * @brief Handles SD card interrupt request. 00537 */ 00538 void BSP_SD_IRQHandler(void) 00539 { 00540 HAL_SD_IRQHandler(&uSdHandle); 00541 } 00542 00543 /** 00544 * @brief Handles SD DMA Tx transfer interrupt request. 00545 */ 00546 void BSP_SD_DMA_Tx_IRQHandler(void) 00547 { 00548 HAL_DMA_IRQHandler(uSdHandle.hdmatx); 00549 } 00550 00551 /** 00552 * @brief Handles SD DMA Rx transfer interrupt request. 00553 */ 00554 void BSP_SD_DMA_Rx_IRQHandler(void) 00555 { 00556 HAL_DMA_IRQHandler(uSdHandle.hdmarx); 00557 } 00558 00559 /** 00560 * @brief Gets the current SD card data status. 00561 * @retval Data transfer state. 00562 * This value can be one of the following values: 00563 * @arg SD_TRANSFER_OK: No data transfer is acting 00564 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00565 * @arg SD_TRANSFER_ERROR: Data transfer error 00566 */ 00567 HAL_SD_TransferStateTypedef BSP_SD_GetStatus(void) 00568 { 00569 return(HAL_SD_GetStatus(&uSdHandle)); 00570 } 00571 00572 /** 00573 * @brief Get SD information about specific SD card. 00574 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00575 */ 00576 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypedef *CardInfo) 00577 { 00578 /* Get SD card Information */ 00579 HAL_SD_Get_CardInfo(&uSdHandle, CardInfo); 00580 } 00581 00582 /** 00583 * @} 00584 */ 00585 00586 /** 00587 * @} 00588 */ 00589 00590 /** 00591 * @} 00592 */ 00593 00594 /** 00595 * @} 00596 */ 00597 00598 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 10:58:46 for STM32F469I-Discovery BSP User Manual by
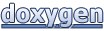