STM32F469I-Discovery BSP User Manual
|
stm32469i_discovery_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_discovery_lcd.h 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 13-January-2016 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32469i_discovery_lcd.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32469I_DISCOVERY_LCD_H 00041 #define __STM32469I_DISCOVERY_LCD_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 /* Include LCD component Driver */ 00049 00050 /* Include OTM8009A LCD Driver IC driver code */ 00051 #include "../Components/otm8009a/otm8009a.h" 00052 00053 /* Include SDRAM Driver */ 00054 #include "stm32469i_discovery_sdram.h" 00055 #include "stm32469i_discovery.h" 00056 #include "../../../Utilities/Fonts/fonts.h" 00057 00058 #include <string.h> /* use of memset() */ 00059 00060 /** @addtogroup BSP 00061 * @{ 00062 */ 00063 00064 /** @addtogroup STM32469I_Discovery 00065 * @{ 00066 */ 00067 00068 /** @addtogroup STM32469I-Discovery_LCD 00069 * @{ 00070 */ 00071 00072 /** @defgroup STM32469I-Discovery_LCD_Exported_Constants STM32469I Discovery LCD Exported Constants 00073 * @{ 00074 */ 00075 00076 #define LCD_LayerCfgTypeDef LTDC_LayerCfgTypeDef 00077 /** 00078 * @brief LCD FB_StartAddress 00079 */ 00080 #define LCD_FB_START_ADDRESS ((uint32_t)0xC0000000) 00081 00082 /** @brief Maximum number of LTDC layers 00083 */ 00084 #define LTDC_MAX_LAYER_NUMBER ((uint32_t) 2) 00085 00086 /** @brief LTDC Background layer index 00087 */ 00088 #define LTDC_ACTIVE_LAYER_BACKGROUND ((uint32_t) 0) 00089 00090 /** @brief LTDC Foreground layer index 00091 */ 00092 #define LTDC_ACTIVE_LAYER_FOREGROUND ((uint32_t) 1) 00093 00094 /** @brief Number of LTDC layers 00095 */ 00096 #define LTDC_NB_OF_LAYERS ((uint32_t) 2) 00097 00098 /** @brief LTDC Default used layer index 00099 */ 00100 #define LTDC_DEFAULT_ACTIVE_LAYER LTDC_ACTIVE_LAYER_FOREGROUND 00101 00102 /** 00103 * @brief LCD status structure definition 00104 */ 00105 #define LCD_OK 0x00 00106 #define LCD_ERROR 0x01 00107 #define LCD_TIMEOUT 0x02 00108 00109 /** 00110 * @brief LCD Display OTM8009A ID 00111 */ 00112 #define LCD_OTM8009A_ID ((uint32_t) 0) 00113 00114 /** 00115 * @brief LCD color definitions values 00116 * in ARGB8888 format. 00117 */ 00118 00119 /** @brief Blue value in ARGB8888 format 00120 */ 00121 #define LCD_COLOR_BLUE ((uint32_t) 0xFF0000FF) 00122 00123 /** @brief Green value in ARGB8888 format 00124 */ 00125 #define LCD_COLOR_GREEN ((uint32_t) 0xFF00FF00) 00126 00127 /** @brief Red value in ARGB8888 format 00128 */ 00129 #define LCD_COLOR_RED ((uint32_t) 0xFFFF0000) 00130 00131 /** @brief Cyan value in ARGB8888 format 00132 */ 00133 #define LCD_COLOR_CYAN ((uint32_t) 0xFF00FFFF) 00134 00135 /** @brief Magenta value in ARGB8888 format 00136 */ 00137 #define LCD_COLOR_MAGENTA ((uint32_t) 0xFFFF00FF) 00138 00139 /** @brief Yellow value in ARGB8888 format 00140 */ 00141 #define LCD_COLOR_YELLOW ((uint32_t) 0xFFFFFF00) 00142 00143 /** @brief Light Blue value in ARGB8888 format 00144 */ 00145 #define LCD_COLOR_LIGHTBLUE ((uint32_t) 0xFF8080FF) 00146 00147 /** @brief Light Green value in ARGB8888 format 00148 */ 00149 #define LCD_COLOR_LIGHTGREEN ((uint32_t) 0xFF80FF80) 00150 00151 /** @brief Light Red value in ARGB8888 format 00152 */ 00153 #define LCD_COLOR_LIGHTRED ((uint32_t) 0xFFFF8080) 00154 00155 /** @brief Light Cyan value in ARGB8888 format 00156 */ 00157 #define LCD_COLOR_LIGHTCYAN ((uint32_t) 0xFF80FFFF) 00158 00159 /** @brief Light Magenta value in ARGB8888 format 00160 */ 00161 #define LCD_COLOR_LIGHTMAGENTA ((uint32_t) 0xFFFF80FF) 00162 00163 /** @brief Light Yellow value in ARGB8888 format 00164 */ 00165 #define LCD_COLOR_LIGHTYELLOW ((uint32_t) 0xFFFFFF80) 00166 00167 /** @brief Dark Blue value in ARGB8888 format 00168 */ 00169 #define LCD_COLOR_DARKBLUE ((uint32_t) 0xFF000080) 00170 00171 /** @brief Light Dark Green value in ARGB8888 format 00172 */ 00173 #define LCD_COLOR_DARKGREEN ((uint32_t) 0xFF008000) 00174 00175 /** @brief Light Dark Red value in ARGB8888 format 00176 */ 00177 #define LCD_COLOR_DARKRED ((uint32_t) 0xFF800000) 00178 00179 /** @brief Dark Cyan value in ARGB8888 format 00180 */ 00181 #define LCD_COLOR_DARKCYAN ((uint32_t) 0xFF008080) 00182 00183 /** @brief Dark Magenta value in ARGB8888 format 00184 */ 00185 #define LCD_COLOR_DARKMAGENTA ((uint32_t) 0xFF800080) 00186 00187 /** @brief Dark Yellow value in ARGB8888 format 00188 */ 00189 #define LCD_COLOR_DARKYELLOW ((uint32_t) 0xFF808000) 00190 00191 /** @brief White value in ARGB8888 format 00192 */ 00193 #define LCD_COLOR_WHITE ((uint32_t) 0xFFFFFFFF) 00194 00195 /** @brief Light Gray value in ARGB8888 format 00196 */ 00197 #define LCD_COLOR_LIGHTGRAY ((uint32_t) 0xFFD3D3D3) 00198 00199 /** @brief Gray value in ARGB8888 format 00200 */ 00201 #define LCD_COLOR_GRAY ((uint32_t) 0xFF808080) 00202 00203 /** @brief Dark Gray value in ARGB8888 format 00204 */ 00205 #define LCD_COLOR_DARKGRAY ((uint32_t) 0xFF404040) 00206 00207 /** @brief Black value in ARGB8888 format 00208 */ 00209 #define LCD_COLOR_BLACK ((uint32_t) 0xFF000000) 00210 00211 /** @brief Brown value in ARGB8888 format 00212 */ 00213 #define LCD_COLOR_BROWN ((uint32_t) 0xFFA52A2A) 00214 00215 /** @brief Orange value in ARGB8888 format 00216 */ 00217 #define LCD_COLOR_ORANGE ((uint32_t) 0xFFFFA500) 00218 00219 /** @brief Transparent value in ARGB8888 format 00220 */ 00221 #define LCD_COLOR_TRANSPARENT ((uint32_t) 0xFF000000) 00222 00223 /** 00224 * @brief LCD default font 00225 */ 00226 #define LCD_DEFAULT_FONT Font24 00227 /** 00228 * @} 00229 */ 00230 00231 /** @defgroup STM32469I-Discovery_LCD_Exported_Types STM32469I Discovery LCD Exported Types 00232 * @{ 00233 */ 00234 00235 /** 00236 * @brief LCD Drawing main properties 00237 */ 00238 typedef struct 00239 { 00240 uint32_t TextColor; /*!< Specifies the color of text */ 00241 uint32_t BackColor; /*!< Specifies the background color below the text */ 00242 sFONT *pFont; /*!< Specifies the font used for the text */ 00243 00244 } LCD_DrawPropTypeDef; 00245 00246 /** 00247 * @brief LCD Drawing point (pixel) geometric definition 00248 */ 00249 typedef struct 00250 { 00251 int16_t X; /*!< geometric X position of drawing */ 00252 int16_t Y; /*!< geometric Y position of drawing */ 00253 00254 } Point; 00255 00256 /** 00257 * @brief Pointer on LCD Drawing point (pixel) geometric definition 00258 */ 00259 typedef Point * pPoint; 00260 00261 /** 00262 * @brief LCD drawing Line alignment mode definitions 00263 */ 00264 typedef enum 00265 { 00266 CENTER_MODE = 0x01, /*!< Center mode */ 00267 RIGHT_MODE = 0x02, /*!< Right mode */ 00268 LEFT_MODE = 0x03 /*!< Left mode */ 00269 00270 } Text_AlignModeTypdef; 00271 00272 00273 /** 00274 * @brief LCD_OrientationTypeDef 00275 * Possible values of Display Orientation 00276 */ 00277 typedef enum 00278 { 00279 LCD_ORIENTATION_PORTRAIT = 0x00, /*!< Portrait orientation choice of LCD screen */ 00280 LCD_ORIENTATION_LANDSCAPE = 0x01, /*!< Landscape orientation choice of LCD screen */ 00281 LCD_ORIENTATION_INVALID = 0x02 /*!< Invalid orientation choice of LCD screen */ 00282 } LCD_OrientationTypeDef; 00283 00284 /** 00285 * @brief Possible values of 00286 * pixel data format (ie color coding) transmitted on DSI Data lane in DSI packets 00287 */ 00288 typedef enum 00289 { 00290 LCD_DSI_PIXEL_DATA_FMT_RBG888 = 0x00, /*!< DSI packet pixel format chosen is RGB888 : 24 bpp */ 00291 LCD_DSI_PIXEL_DATA_FMT_RBG565 = 0x02, /*!< DSI packet pixel format chosen is RGB565 : 16 bpp */ 00292 LCD_DSI_PIXEL_DATA_FMT_INVALID = 0x03 /*!< Invalid DSI packet pixel format */ 00293 00294 } LCD_DsiPixelDataFmtTypeDef; 00295 00296 /** 00297 * @} 00298 */ 00299 00300 /** @defgroup STM32469I-Discovery_LCD_Exported_Macro STM32469I Discovery LCD Exported Macro 00301 * @{ 00302 */ 00303 00304 /** 00305 * @} 00306 */ 00307 00308 /** @defgroup STM32469I-Discovery_LCD_Exported_Functions STM32469I Discovery LCD Exported Functions 00309 * @{ 00310 */ 00311 void BSP_LCD_DMA2D_IRQHandler(void); 00312 void BSP_LCD_DSI_IRQHandler(void); 00313 void BSP_LCD_LTDC_IRQHandler(void); 00314 void BSP_LCD_LTDC_ER_IRQHandler(void); 00315 00316 uint8_t BSP_LCD_Init(void); 00317 uint8_t BSP_LCD_InitEx(LCD_OrientationTypeDef orientation); 00318 00319 void BSP_LCD_MspDeInit(void); 00320 void BSP_LCD_MspInit(void); 00321 void BSP_LCD_Reset(void); 00322 00323 uint32_t BSP_LCD_GetXSize(void); 00324 uint32_t BSP_LCD_GetYSize(void); 00325 void BSP_LCD_SetXSize(uint32_t imageWidthPixels); 00326 void BSP_LCD_SetYSize(uint32_t imageHeightPixels); 00327 00328 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address); 00329 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency); 00330 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address); 00331 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue); 00332 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex); 00333 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00334 00335 void BSP_LCD_SelectLayer(uint32_t LayerIndex); 00336 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State); 00337 00338 void BSP_LCD_SetTextColor(uint32_t Color); 00339 uint32_t BSP_LCD_GetTextColor(void); 00340 void BSP_LCD_SetBackColor(uint32_t Color); 00341 uint32_t BSP_LCD_GetBackColor(void); 00342 void BSP_LCD_SetFont(sFONT *fonts); 00343 sFONT *BSP_LCD_GetFont(void); 00344 00345 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos); 00346 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t pixel); 00347 void BSP_LCD_Clear(uint32_t Color); 00348 void BSP_LCD_ClearStringLine(uint32_t Line); 00349 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00350 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode); 00351 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00352 00353 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00354 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00355 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); 00356 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00357 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00358 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount); 00359 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00360 void BSP_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp); 00361 00362 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00363 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00364 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount); 00365 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00366 00367 void BSP_LCD_DisplayOff(void); 00368 void BSP_LCD_DisplayOn(void); 00369 00370 /** 00371 * @} 00372 */ 00373 00374 /** @defgroup STM32469I-EVAL_LCD_Exported_Variables STM32469I EVAL LCD Exported Variables 00375 * @{ 00376 */ 00377 00378 /* @brief DMA2D handle variable */ 00379 extern DMA2D_HandleTypeDef hdma2d_eval; 00380 00381 /** 00382 * @} 00383 */ 00384 00385 /** @defgroup STM32469I-Discovery_LCD_Exported_Variables STM32469I Discovery LCD Exported Variables 00386 * @{ 00387 */ 00388 00389 /** 00390 * @} 00391 */ 00392 00393 /** 00394 * @} 00395 */ 00396 00397 /** 00398 * @} 00399 */ 00400 00401 /** 00402 * @} 00403 */ 00404 00405 #ifdef __cplusplus 00406 } 00407 #endif 00408 00409 #endif /* __STM32469I_DISCOVERY_LCD_H */ 00410 00411 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 10:58:45 for STM32F469I-Discovery BSP User Manual by
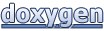