STM32F469I-Discovery BSP User Manual
|
stm32469i_discovery_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_discovery_lcd.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 13-January-2016 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM32469I-Discovery evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info: ------------------------------------------------------------------ 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive directly in video mode a LCD TFT using the DSI interface. 00044 The following IPs are implied : DSI Host IP block working 00045 in conjunction to the LTDC controller. 00046 - This driver is linked by construction to LCD KoD mounted on board MB1166. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the LCD using the BSP_LCD_Init() function. 00052 o Select the LCD layer to be used using the BSP_LCD_SelectLayer() function. 00053 o Enable the LCD display using the BSP_LCD_DisplayOn() function. 00054 00055 + Options 00056 o Configure and enable the color keying functionality using the 00057 BSP_LCD_SetColorKeying() function. 00058 o Modify in the fly the transparency and/or the frame buffer address 00059 using the following functions: 00060 - BSP_LCD_SetTransparency() 00061 - BSP_LCD_SetLayerAddress() 00062 00063 + Display on LCD 00064 o Clear the whole LCD using BSP_LCD_Clear() function or only one specified string 00065 line using the BSP_LCD_ClearStringLine() function. 00066 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00067 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00068 o Display a string line on the specified position (x,y in pixel) and align mode 00069 using the BSP_LCD_DisplayStringAtLine() function. 00070 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00071 on LCD using the available set of functions. 00072 00073 ------------------------------------------------------------------------------*/ 00074 00075 /* Includes ------------------------------------------------------------------*/ 00076 #include "stm32469i_discovery_lcd.h" 00077 #include "../../../Utilities/Fonts/fonts.h" 00078 #include "../../../Utilities/Fonts/font24.c" 00079 #include "../../../Utilities/Fonts/font20.c" 00080 #include "../../../Utilities/Fonts/font16.c" 00081 #include "../../../Utilities/Fonts/font12.c" 00082 #include "../../../Utilities/Fonts/font8.c" 00083 00084 /** @addtogroup BSP 00085 * @{ 00086 */ 00087 00088 /** @addtogroup STM32469I_Discovery 00089 * @{ 00090 */ 00091 00092 /** @defgroup STM32469I-Discovery_LCD STM32469I Discovery LCD 00093 * @{ 00094 */ 00095 00096 /** @defgroup STM32469I-Discovery_LCD_Private_TypesDefinitions STM32469I Discovery LCD Private TypesDefinitions 00097 * @{ 00098 */ 00099 static DSI_VidCfgTypeDef hdsivideo_handle; 00100 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32469I-Discovery_LCD_Private_Defines STM32469I Discovery LCD Private Defines 00106 * @{ 00107 */ 00108 /** 00109 * @} 00110 */ 00111 00112 /** @defgroup STM32469I-Discovery_LCD_Private_Macros STM32469I Discovery LCD Private Macros 00113 * @{ 00114 */ 00115 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00116 00117 #define POLY_X(Z) ((int32_t)((Points + (Z))->X)) 00118 #define POLY_Y(Z) ((int32_t)((Points + (Z))->Y)) 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup STM32469I-Discovery_LCD_Exported_Variables STM32469I Discovery LCD Exported Variables 00124 * @{ 00125 */ 00126 00127 /** 00128 * @} 00129 */ 00130 00131 00132 /** @defgroup STM32469I-Discovery_LCD_Private_Variables STM32469I Discovery LCD Private Variables 00133 * @{ 00134 */ 00135 00136 DMA2D_HandleTypeDef hdma2d_eval; 00137 LTDC_HandleTypeDef hltdc_eval; 00138 DSI_HandleTypeDef hdsi_eval; 00139 uint32_t lcd_x_size = OTM8009A_800X480_WIDTH; 00140 uint32_t lcd_y_size = OTM8009A_800X480_HEIGHT; 00141 00142 /** 00143 * @} 00144 */ 00145 00146 00147 /** @defgroup STM32469I-Discovery_LCD_Private_Variables STM32469I Discovery LCD Private Variables 00148 * @{ 00149 */ 00150 00151 /** 00152 * @brief Default Active LTDC Layer in which drawing is made is LTDC Layer Background 00153 */ 00154 static uint32_t ActiveLayer = LTDC_ACTIVE_LAYER_BACKGROUND; 00155 00156 /** 00157 * @brief Current Drawing Layer properties variable 00158 */ 00159 static LCD_DrawPropTypeDef DrawProp[LTDC_MAX_LAYER_NUMBER]; 00160 /** 00161 * @} 00162 */ 00163 00164 /** @defgroup STM32469I-Discovery_LCD_Private_FunctionPrototypes STM32469I Discovery LCD Private FunctionPrototypes 00165 * @{ 00166 */ 00167 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00168 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00169 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex); 00170 static void LL_ConvertLineToARGB8888(void * pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode); 00171 /** 00172 * @} 00173 */ 00174 00175 /** @defgroup STM32469I-Discovery_LCD_Exported_Functions STM32469I Discovery LCD Exported Functions 00176 * @{ 00177 */ 00178 00179 /** 00180 * @brief Initializes the DSI LCD. 00181 * @retval LCD state 00182 */ 00183 uint8_t BSP_LCD_Init(void) 00184 { 00185 return (BSP_LCD_InitEx(LCD_ORIENTATION_LANDSCAPE)); 00186 } 00187 00188 /** 00189 * @brief Initializes the DSI LCD. 00190 * The ititialization is done as below: 00191 * - DSI PLL ititialization 00192 * - DSI ititialization 00193 * - LTDC ititialization 00194 * - OTM8009A LCD Display IC Driver ititialization 00195 * @retval LCD state 00196 */ 00197 uint8_t BSP_LCD_InitEx(LCD_OrientationTypeDef orientation) 00198 { 00199 DSI_PLLInitTypeDef dsiPllInit; 00200 static RCC_PeriphCLKInitTypeDef PeriphClkInitStruct; 00201 uint32_t LcdClock = 27429; /*!< LcdClk = 27429 kHz */ 00202 uint32_t Clockratio = 0; 00203 uint32_t laneByteClk_kHz = 0; 00204 uint32_t VSA; /*!< Vertical start active time in units of lines */ 00205 uint32_t VBP; /*!< Vertical Back Porch time in units of lines */ 00206 uint32_t VFP; /*!< Vertical Front Porch time in units of lines */ 00207 uint32_t VACT; /*!< Vertical Active time in units of lines = imageSize Y in pixels to display */ 00208 uint32_t HSA; /*!< Horizontal start active time in units of lcdClk */ 00209 uint32_t HBP; /*!< Horizontal Back Porch time in units of lcdClk */ 00210 uint32_t HFP; /*!< Horizontal Front Porch time in units of lcdClk */ 00211 uint32_t HACT; /*!< Horizontal Active time in units of lcdClk = imageSize X in pixels to display */ 00212 00213 00214 /* Toggle Hardware Reset of the DSI LCD using 00215 * its XRES signal (active low) */ 00216 BSP_LCD_Reset(); 00217 00218 /* Call first MSP Initialize only in case of first initialization 00219 * This will set IP blocks LTDC, DSI and DMA2D 00220 * - out of reset 00221 * - clocked 00222 * - NVIC IRQ related to IP blocks enabled 00223 */ 00224 BSP_LCD_MspInit(); 00225 00226 /*************************DSI Initialization***********************************/ 00227 00228 /* Base address of DSI Host/Wrapper registers to be set before calling De-Init */ 00229 hdsi_eval.Instance = DSI; 00230 00231 HAL_DSI_DeInit(&(hdsi_eval)); 00232 00233 #if !defined(USE_STM32469I_DISCO_REVA) 00234 dsiPllInit.PLLNDIV = 125; 00235 dsiPllInit.PLLIDF = DSI_PLL_IN_DIV2; 00236 dsiPllInit.PLLODF = DSI_PLL_OUT_DIV1; 00237 #else 00238 dsiPllInit.PLLNDIV = 100; 00239 dsiPllInit.PLLIDF = DSI_PLL_IN_DIV5; 00240 dsiPllInit.PLLODF = DSI_PLL_OUT_DIV1; 00241 #endif 00242 laneByteClk_kHz = 62500; /* 500 MHz / 8 = 62.5 MHz = 62500 kHz */ 00243 00244 /* Set number of Lanes */ 00245 hdsi_eval.Init.NumberOfLanes = DSI_TWO_DATA_LANES; 00246 00247 /* TXEscapeCkdiv = f(LaneByteClk)/15.62 = 4 */ 00248 hdsi_eval.Init.TXEscapeCkdiv = laneByteClk_kHz/15620; 00249 00250 HAL_DSI_Init(&(hdsi_eval), &(dsiPllInit)); 00251 Clockratio = laneByteClk_kHz/LcdClock; 00252 /* Timing parameters for all Video modes 00253 * Set Timing parameters of LTDC depending on its chosen orientation 00254 */ 00255 if(orientation == LCD_ORIENTATION_PORTRAIT) 00256 { 00257 VSA = OTM8009A_480X800_VSYNC; /* 12 */ 00258 VBP = OTM8009A_480X800_VBP; /* 12 */ 00259 VFP = OTM8009A_480X800_VFP; /* 12 */ 00260 HSA = OTM8009A_480X800_HSYNC; /* 120 */ 00261 HBP = OTM8009A_480X800_HBP; /* 120 */ 00262 HFP = OTM8009A_480X800_HFP; /* 120 */ 00263 lcd_x_size = OTM8009A_480X800_WIDTH; /* 480 */ 00264 lcd_y_size = OTM8009A_480X800_HEIGHT; /* 800 */ 00265 } 00266 else 00267 { 00268 /* lcd_orientation == LCD_ORIENTATION_LANDSCAPE */ 00269 VSA = OTM8009A_800X480_VSYNC; /* 12 */ 00270 VBP = OTM8009A_800X480_VBP; /* 12 */ 00271 VFP = OTM8009A_800X480_VFP; /* 12 */ 00272 HSA = OTM8009A_800X480_HSYNC; /* 120 */ 00273 HBP = OTM8009A_800X480_HBP; /* 120 */ 00274 HFP = OTM8009A_800X480_HFP; /* 120 */ 00275 lcd_x_size = OTM8009A_800X480_WIDTH; /* 800 */ 00276 lcd_y_size = OTM8009A_800X480_HEIGHT; /* 480 */ 00277 } 00278 00279 HACT = lcd_x_size; 00280 VACT = lcd_y_size; 00281 00282 00283 hdsivideo_handle.VirtualChannelID = LCD_OTM8009A_ID; 00284 hdsivideo_handle.ColorCoding = LCD_DSI_PIXEL_DATA_FMT_RBG888; 00285 hdsivideo_handle.VSPolarity = DSI_VSYNC_ACTIVE_HIGH; 00286 hdsivideo_handle.HSPolarity = DSI_HSYNC_ACTIVE_HIGH; 00287 hdsivideo_handle.DEPolarity = DSI_DATA_ENABLE_ACTIVE_HIGH; 00288 hdsivideo_handle.Mode = DSI_VID_MODE_BURST; /* Mode Video burst ie : one LgP per line */ 00289 hdsivideo_handle.NullPacketSize = 0xFFF; 00290 hdsivideo_handle.NumberOfChunks = 0; 00291 hdsivideo_handle.PacketSize = HACT; /* Value depending on display orientation choice portrait/landscape */ 00292 hdsivideo_handle.HorizontalSyncActive = HSA*Clockratio; 00293 hdsivideo_handle.HorizontalBackPorch = HBP*Clockratio; 00294 hdsivideo_handle.HorizontalLine = (HACT + HSA + HBP + HFP)*Clockratio; /* Value depending on display orientation choice portrait/landscape */ 00295 hdsivideo_handle.VerticalSyncActive = VSA; 00296 hdsivideo_handle.VerticalBackPorch = VBP; 00297 hdsivideo_handle.VerticalFrontPorch = VFP; 00298 hdsivideo_handle.VerticalActive = VACT; /* Value depending on display orientation choice portrait/landscape */ 00299 00300 /* Enable or disable sending LP command while streaming is active in video mode */ 00301 hdsivideo_handle.LPCommandEnable = DSI_LP_COMMAND_ENABLE; /* Enable sending commands in mode LP (Low Power) */ 00302 00303 /* Largest packet size possible to transmit in LP mode in VSA, VBP, VFP regions */ 00304 /* Only useful when sending LP packets is allowed while streaming is active in video mode */ 00305 hdsivideo_handle.LPLargestPacketSize = 64; 00306 00307 /* Largest packet size possible to transmit in LP mode in HFP region during VACT period */ 00308 /* Only useful when sending LP packets is allowed while streaming is active in video mode */ 00309 hdsivideo_handle.LPVACTLargestPacketSize = 64; 00310 00311 00312 /* Specify for each region of the video frame, if the transmission of command in LP mode is allowed in this region */ 00313 /* while streaming is active in video mode */ 00314 hdsivideo_handle.LPHorizontalFrontPorchEnable = DSI_LP_HFP_ENABLE; /* Allow sending LP commands during HFP period */ 00315 hdsivideo_handle.LPHorizontalBackPorchEnable = DSI_LP_HBP_ENABLE; /* Allow sending LP commands during HBP period */ 00316 hdsivideo_handle.LPVerticalActiveEnable = DSI_LP_VACT_ENABLE; /* Allow sending LP commands during VACT period */ 00317 hdsivideo_handle.LPVerticalFrontPorchEnable = DSI_LP_VFP_ENABLE; /* Allow sending LP commands during VFP period */ 00318 hdsivideo_handle.LPVerticalBackPorchEnable = DSI_LP_VBP_ENABLE; /* Allow sending LP commands during VBP period */ 00319 hdsivideo_handle.LPVerticalSyncActiveEnable = DSI_LP_VSYNC_ENABLE; /* Allow sending LP commands during VSync = VSA period */ 00320 00321 /* Configure DSI Video mode timings with settings set above */ 00322 HAL_DSI_ConfigVideoMode(&(hdsi_eval), &(hdsivideo_handle)); 00323 00324 /* Enable the DSI host and wrapper : but LTDC is not started yet at this stage */ 00325 HAL_DSI_Start(&(hdsi_eval)); 00326 /*************************End DSI Initialization*******************************/ 00327 00328 00329 /************************LTDC Initialization***********************************/ 00330 00331 /* Timing Configuration */ 00332 hltdc_eval.Init.HorizontalSync = (HSA - 1); 00333 hltdc_eval.Init.AccumulatedHBP = (HSA + HBP - 1); 00334 hltdc_eval.Init.AccumulatedActiveW = (lcd_x_size + HSA + HBP - 1); 00335 hltdc_eval.Init.TotalWidth = (lcd_x_size + HSA + HBP + HFP - 1); 00336 00337 /* Initialize the LCD pixel width and pixel height */ 00338 hltdc_eval.LayerCfg->ImageWidth = lcd_x_size; 00339 hltdc_eval.LayerCfg->ImageHeight = lcd_y_size; 00340 00341 00342 /* LCD clock configuration */ 00343 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 00344 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 384 Mhz */ 00345 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 384 MHz / 7 = 54.857 MHz */ 00346 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_2 = 54.857 MHz / 2 = 27.429 MHz */ 00347 PeriphClkInitStruct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 00348 PeriphClkInitStruct.PLLSAI.PLLSAIN = 384; 00349 PeriphClkInitStruct.PLLSAI.PLLSAIR = 7; 00350 PeriphClkInitStruct.PLLSAIDivR = RCC_PLLSAIDIVR_2; 00351 HAL_RCCEx_PeriphCLKConfig(&PeriphClkInitStruct); 00352 00353 /* Background value */ 00354 hltdc_eval.Init.Backcolor.Blue = 0; 00355 hltdc_eval.Init.Backcolor.Green = 0; 00356 hltdc_eval.Init.Backcolor.Red = 0; 00357 hltdc_eval.Init.PCPolarity = LTDC_PCPOLARITY_IPC; 00358 hltdc_eval.Instance = LTDC; 00359 00360 /* Get LTDC Configuration from DSI Configuration */ 00361 HAL_LTDC_StructInitFromVideoConfig(&(hltdc_eval), &(hdsivideo_handle)); 00362 00363 /* Initialize the LTDC */ 00364 HAL_LTDC_Init(&hltdc_eval); 00365 00366 #if !defined(DATA_IN_ExtSDRAM) 00367 /* Initialize the SDRAM */ 00368 BSP_SDRAM_Init(); 00369 #endif /* DATA_IN_ExtSDRAM */ 00370 00371 /* Initialize the font */ 00372 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00373 00374 /************************End LTDC Initialization*******************************/ 00375 00376 00377 /***********************OTM8009A Initialization********************************/ 00378 00379 /* Initialize the OTM8009A LCD Display IC Driver (KoD LCD IC Driver) 00380 * depending on configuration set in 'hdsivideo_handle'. 00381 */ 00382 OTM8009A_Init(hdsivideo_handle.ColorCoding, orientation); 00383 00384 /***********************End OTM8009A Initialization****************************/ 00385 00386 return LCD_OK; 00387 } 00388 00389 /** 00390 * @brief BSP LCD Reset 00391 * Hw reset the LCD DSI activating its XRES signal (active low for some time) 00392 * and desactivating it later. 00393 * This signal is only cabled on Discovery Rev B and beyond. 00394 */ 00395 void BSP_LCD_Reset(void) 00396 { 00397 #if !defined(USE_STM32469I_DISCO_REVA) 00398 /* EVAL Rev B and beyond : reset the LCD by activation of XRES (active low) connected to PH7 */ 00399 GPIO_InitTypeDef gpio_init_structure; 00400 00401 __HAL_RCC_GPIOH_CLK_ENABLE(); 00402 00403 /* Configure the GPIO on PH7 */ 00404 gpio_init_structure.Pin = GPIO_PIN_7; 00405 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_OD; 00406 gpio_init_structure.Pull = GPIO_NOPULL; 00407 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00408 00409 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00410 00411 /* Activate XRES active low */ 00412 HAL_GPIO_WritePin(GPIOH, GPIO_PIN_7, GPIO_PIN_RESET); 00413 00414 HAL_Delay(20); /* wait 20 ms */ 00415 00416 /* Desactivate XRES */ 00417 HAL_GPIO_WritePin(GPIOH, GPIO_PIN_7, GPIO_PIN_SET); 00418 00419 /* Wait for 10ms after releasing XRES before sending commands */ 00420 HAL_Delay(10); 00421 #else 00422 00423 #endif /* USE_STM32469I_DISCO_REVA == 0 */ 00424 } 00425 00426 /** 00427 * @brief Gets the LCD X size. 00428 * @retval Used LCD X size 00429 */ 00430 uint32_t BSP_LCD_GetXSize(void) 00431 { 00432 return (lcd_x_size); 00433 } 00434 00435 /** 00436 * @brief Gets the LCD Y size. 00437 * @retval Used LCD Y size 00438 */ 00439 uint32_t BSP_LCD_GetYSize(void) 00440 { 00441 return (lcd_y_size); 00442 } 00443 00444 /** 00445 * @brief Set the LCD X size. 00446 * @param imageWidthPixels : uint32_t image width in pixels unit 00447 */ 00448 void BSP_LCD_SetXSize(uint32_t imageWidthPixels) 00449 { 00450 hltdc_eval.LayerCfg[ActiveLayer].ImageWidth = imageWidthPixels; 00451 } 00452 00453 /** 00454 * @brief Set the LCD Y size. 00455 * @param imageHeightPixels : uint32_t image height in lines unit 00456 */ 00457 void BSP_LCD_SetYSize(uint32_t imageHeightPixels) 00458 { 00459 hltdc_eval.LayerCfg[ActiveLayer].ImageHeight = imageHeightPixels; 00460 } 00461 00462 00463 /** 00464 * @brief Initializes the LCD layers. 00465 * @param LayerIndex: Layer foreground or background 00466 * @param FB_Address: Layer frame buffer 00467 */ 00468 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address) 00469 { 00470 LCD_LayerCfgTypeDef Layercfg; 00471 00472 /* Layer Init */ 00473 Layercfg.WindowX0 = 0; 00474 Layercfg.WindowX1 = BSP_LCD_GetXSize(); 00475 Layercfg.WindowY0 = 0; 00476 Layercfg.WindowY1 = BSP_LCD_GetYSize(); 00477 Layercfg.PixelFormat = LTDC_PIXEL_FORMAT_ARGB8888; 00478 Layercfg.FBStartAdress = FB_Address; 00479 Layercfg.Alpha = 255; 00480 Layercfg.Alpha0 = 0; 00481 Layercfg.Backcolor.Blue = 0; 00482 Layercfg.Backcolor.Green = 0; 00483 Layercfg.Backcolor.Red = 0; 00484 Layercfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00485 Layercfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00486 Layercfg.ImageWidth = BSP_LCD_GetXSize(); 00487 Layercfg.ImageHeight = BSP_LCD_GetYSize(); 00488 00489 HAL_LTDC_ConfigLayer(&hltdc_eval, &Layercfg, LayerIndex); 00490 00491 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00492 DrawProp[LayerIndex].pFont = &Font24; 00493 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00494 } 00495 00496 00497 /** 00498 * @brief Selects the LCD Layer. 00499 * @param LayerIndex: Layer foreground or background 00500 */ 00501 void BSP_LCD_SelectLayer(uint32_t LayerIndex) 00502 { 00503 ActiveLayer = LayerIndex; 00504 } 00505 00506 /** 00507 * @brief Sets an LCD Layer visible 00508 * @param LayerIndex: Visible Layer 00509 * @param State: New state of the specified layer 00510 * This parameter can be one of the following values: 00511 * @arg ENABLE 00512 * @arg DISABLE 00513 */ 00514 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State) 00515 { 00516 if(State == ENABLE) 00517 { 00518 __HAL_LTDC_LAYER_ENABLE(&(hltdc_eval), LayerIndex); 00519 } 00520 else 00521 { 00522 __HAL_LTDC_LAYER_DISABLE(&(hltdc_eval), LayerIndex); 00523 } 00524 __HAL_LTDC_RELOAD_CONFIG(&(hltdc_eval)); 00525 00526 } 00527 00528 /** 00529 * @brief Configures the transparency. 00530 * @param LayerIndex: Layer foreground or background. 00531 * @param Transparency: Transparency 00532 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00533 */ 00534 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency) 00535 { 00536 00537 HAL_LTDC_SetAlpha(&(hltdc_eval), Transparency, LayerIndex); 00538 00539 } 00540 00541 /** 00542 * @brief Sets an LCD layer frame buffer address. 00543 * @param LayerIndex: Layer foreground or background 00544 * @param Address: New LCD frame buffer value 00545 */ 00546 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address) 00547 { 00548 00549 HAL_LTDC_SetAddress(&(hltdc_eval), Address, LayerIndex); 00550 00551 } 00552 00553 /** 00554 * @brief Sets display window. 00555 * @param LayerIndex: Layer index 00556 * @param Xpos: LCD X position 00557 * @param Ypos: LCD Y position 00558 * @param Width: LCD window width 00559 * @param Height: LCD window height 00560 */ 00561 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00562 { 00563 /* Reconfigure the layer size */ 00564 HAL_LTDC_SetWindowSize(&(hltdc_eval), Width, Height, LayerIndex); 00565 00566 /* Reconfigure the layer position */ 00567 HAL_LTDC_SetWindowPosition(&(hltdc_eval), Xpos, Ypos, LayerIndex); 00568 00569 } 00570 00571 /** 00572 * @brief Configures and sets the color keying. 00573 * @param LayerIndex: Layer foreground or background 00574 * @param RGBValue: Color reference 00575 */ 00576 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue) 00577 { 00578 /* Configure and Enable the color Keying for LCD Layer */ 00579 HAL_LTDC_ConfigColorKeying(&(hltdc_eval), RGBValue, LayerIndex); 00580 HAL_LTDC_EnableColorKeying(&(hltdc_eval), LayerIndex); 00581 } 00582 00583 /** 00584 * @brief Disables the color keying. 00585 * @param LayerIndex: Layer foreground or background 00586 */ 00587 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex) 00588 { 00589 /* Disable the color Keying for LCD Layer */ 00590 HAL_LTDC_DisableColorKeying(&(hltdc_eval), LayerIndex); 00591 } 00592 00593 /** 00594 * @brief Sets the LCD text color. 00595 * @param Color: Text color code ARGB(8-8-8-8) 00596 */ 00597 void BSP_LCD_SetTextColor(uint32_t Color) 00598 { 00599 DrawProp[ActiveLayer].TextColor = Color; 00600 } 00601 00602 /** 00603 * @brief Gets the LCD text color. 00604 * @retval Used text color. 00605 */ 00606 uint32_t BSP_LCD_GetTextColor(void) 00607 { 00608 return DrawProp[ActiveLayer].TextColor; 00609 } 00610 00611 /** 00612 * @brief Sets the LCD background color. 00613 * @param Color: Layer background color code ARGB(8-8-8-8) 00614 */ 00615 void BSP_LCD_SetBackColor(uint32_t Color) 00616 { 00617 DrawProp[ActiveLayer].BackColor = Color; 00618 } 00619 00620 /** 00621 * @brief Gets the LCD background color. 00622 * @retval Used background color 00623 */ 00624 uint32_t BSP_LCD_GetBackColor(void) 00625 { 00626 return DrawProp[ActiveLayer].BackColor; 00627 } 00628 00629 /** 00630 * @brief Sets the LCD text font. 00631 * @param fonts: Layer font to be used 00632 */ 00633 void BSP_LCD_SetFont(sFONT *fonts) 00634 { 00635 DrawProp[ActiveLayer].pFont = fonts; 00636 } 00637 00638 /** 00639 * @brief Gets the LCD text font. 00640 * @retval Used layer font 00641 */ 00642 sFONT *BSP_LCD_GetFont(void) 00643 { 00644 return DrawProp[ActiveLayer].pFont; 00645 } 00646 00647 /** 00648 * @brief Reads an LCD pixel. 00649 * @param Xpos: X position 00650 * @param Ypos: Y position 00651 * @retval RGB pixel color 00652 */ 00653 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00654 { 00655 uint32_t ret = 0; 00656 00657 if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB8888) 00658 { 00659 /* Read data value from SDRAM memory */ 00660 ret = *(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00661 } 00662 else if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB888) 00663 { 00664 /* Read data value from SDRAM memory */ 00665 ret = (*(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))) & 0x00FFFFFF); 00666 } 00667 else if((hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) || \ 00668 (hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB4444) || \ 00669 (hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_AL88)) 00670 { 00671 /* Read data value from SDRAM memory */ 00672 ret = *(__IO uint16_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00673 } 00674 else 00675 { 00676 /* Read data value from SDRAM memory */ 00677 ret = *(__IO uint8_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00678 } 00679 00680 return ret; 00681 } 00682 00683 /** 00684 * @brief Clears the whole currently active layer of LTDC. 00685 * @param Color: Color of the background 00686 */ 00687 void BSP_LCD_Clear(uint32_t Color) 00688 { 00689 /* Clear the LCD */ 00690 LL_FillBuffer(ActiveLayer, (uint32_t *)(hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress), BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), 0, Color); 00691 } 00692 00693 /** 00694 * @brief Clears the selected line in currently active layer. 00695 * @param Line: Line to be cleared 00696 */ 00697 void BSP_LCD_ClearStringLine(uint32_t Line) 00698 { 00699 uint32_t color_backup = DrawProp[ActiveLayer].TextColor; 00700 DrawProp[ActiveLayer].TextColor = DrawProp[ActiveLayer].BackColor; 00701 00702 /* Draw rectangle with background color */ 00703 BSP_LCD_FillRect(0, (Line * DrawProp[ActiveLayer].pFont->Height), BSP_LCD_GetXSize(), DrawProp[ActiveLayer].pFont->Height); 00704 00705 DrawProp[ActiveLayer].TextColor = color_backup; 00706 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00707 } 00708 00709 /** 00710 * @brief Displays one character in currently active layer. 00711 * @param Xpos: Start column address 00712 * @param Ypos: Line where to display the character shape. 00713 * @param Ascii: Character ascii code 00714 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00715 */ 00716 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00717 { 00718 DrawChar(Xpos, Ypos, &DrawProp[ActiveLayer].pFont->table[(Ascii-' ') *\ 00719 DrawProp[ActiveLayer].pFont->Height * ((DrawProp[ActiveLayer].pFont->Width + 7) / 8)]); 00720 } 00721 00722 /** 00723 * @brief Displays characters in currently active layer. 00724 * @param Xpos: X position (in pixel) 00725 * @param Ypos: Y position (in pixel) 00726 * @param Text: Pointer to string to display on LCD 00727 * @param Mode: Display mode 00728 * This parameter can be one of the following values: 00729 * @arg CENTER_MODE 00730 * @arg RIGHT_MODE 00731 * @arg LEFT_MODE 00732 */ 00733 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) 00734 { 00735 uint16_t refcolumn = 1, i = 0; 00736 uint32_t size = 0, xsize = 0; 00737 uint8_t *ptr = Text; 00738 00739 /* Get the text size */ 00740 while (*ptr++) size ++ ; 00741 00742 /* Characters number per line */ 00743 xsize = (BSP_LCD_GetXSize()/DrawProp[ActiveLayer].pFont->Width); 00744 00745 switch (Mode) 00746 { 00747 case CENTER_MODE: 00748 { 00749 refcolumn = Xpos + ((xsize - size)* DrawProp[ActiveLayer].pFont->Width) / 2; 00750 break; 00751 } 00752 case LEFT_MODE: 00753 { 00754 refcolumn = Xpos; 00755 break; 00756 } 00757 case RIGHT_MODE: 00758 { 00759 refcolumn = - Xpos + ((xsize - size)*DrawProp[ActiveLayer].pFont->Width); 00760 break; 00761 } 00762 default: 00763 { 00764 refcolumn = Xpos; 00765 break; 00766 } 00767 } 00768 00769 /* Check that the Start column is located in the screen */ 00770 if ((refcolumn < 1) || (refcolumn >= 0x8000)) 00771 { 00772 refcolumn = 1; 00773 } 00774 00775 /* Send the string character by character on LCD */ 00776 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp[ActiveLayer].pFont->Width)) & 0xFFFF) >= DrawProp[ActiveLayer].pFont->Width)) 00777 { 00778 /* Display one character on LCD */ 00779 BSP_LCD_DisplayChar(refcolumn, Ypos, *Text); 00780 /* Decrement the column position by 16 */ 00781 refcolumn += DrawProp[ActiveLayer].pFont->Width; 00782 00783 /* Point on the next character */ 00784 Text++; 00785 i++; 00786 } 00787 00788 } 00789 00790 /** 00791 * @brief Displays a maximum of 60 characters on the LCD. 00792 * @param Line: Line where to display the character shape 00793 * @param ptr: Pointer to string to display on LCD 00794 */ 00795 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00796 { 00797 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00798 } 00799 00800 /** 00801 * @brief Draws an horizontal line in currently active layer. 00802 * @param Xpos: X position 00803 * @param Ypos: Y position 00804 * @param Length: Line length 00805 */ 00806 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00807 { 00808 uint32_t Xaddress = 0; 00809 00810 /* Get the line address */ 00811 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00812 00813 /* Write line */ 00814 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Length, 1, 0, DrawProp[ActiveLayer].TextColor); 00815 } 00816 00817 /** 00818 * @brief Draws a vertical line in currently active layer. 00819 * @param Xpos: X position 00820 * @param Ypos: Y position 00821 * @param Length: Line length 00822 */ 00823 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00824 { 00825 uint32_t Xaddress = 0; 00826 00827 /* Get the line address */ 00828 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00829 00830 /* Write line */ 00831 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, 1, Length, (BSP_LCD_GetXSize() - 1), DrawProp[ActiveLayer].TextColor); 00832 } 00833 00834 /** 00835 * @brief Draws an uni-line (between two points) in currently active layer. 00836 * @param x1: Point 1 X position 00837 * @param y1: Point 1 Y position 00838 * @param x2: Point 2 X position 00839 * @param y2: Point 2 Y position 00840 */ 00841 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00842 { 00843 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00844 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00845 curpixel = 0; 00846 00847 deltax = ABS(x2 - x1); /* The difference between the x's */ 00848 deltay = ABS(y2 - y1); /* The difference between the y's */ 00849 x = x1; /* Start x off at the first pixel */ 00850 y = y1; /* Start y off at the first pixel */ 00851 00852 if (x2 >= x1) /* The x-values are increasing */ 00853 { 00854 xinc1 = 1; 00855 xinc2 = 1; 00856 } 00857 else /* The x-values are decreasing */ 00858 { 00859 xinc1 = -1; 00860 xinc2 = -1; 00861 } 00862 00863 if (y2 >= y1) /* The y-values are increasing */ 00864 { 00865 yinc1 = 1; 00866 yinc2 = 1; 00867 } 00868 else /* The y-values are decreasing */ 00869 { 00870 yinc1 = -1; 00871 yinc2 = -1; 00872 } 00873 00874 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00875 { 00876 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00877 yinc2 = 0; /* Don't change the y for every iteration */ 00878 den = deltax; 00879 num = deltax / 2; 00880 numadd = deltay; 00881 numpixels = deltax; /* There are more x-values than y-values */ 00882 } 00883 else /* There is at least one y-value for every x-value */ 00884 { 00885 xinc2 = 0; /* Don't change the x for every iteration */ 00886 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00887 den = deltay; 00888 num = deltay / 2; 00889 numadd = deltax; 00890 numpixels = deltay; /* There are more y-values than x-values */ 00891 } 00892 00893 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00894 { 00895 BSP_LCD_DrawPixel(x, y, DrawProp[ActiveLayer].TextColor); /* Draw the current pixel */ 00896 num += numadd; /* Increase the numerator by the top of the fraction */ 00897 if (num >= den) /* Check if numerator >= denominator */ 00898 { 00899 num -= den; /* Calculate the new numerator value */ 00900 x += xinc1; /* Change the x as appropriate */ 00901 y += yinc1; /* Change the y as appropriate */ 00902 } 00903 x += xinc2; /* Change the x as appropriate */ 00904 y += yinc2; /* Change the y as appropriate */ 00905 } 00906 } 00907 00908 /** 00909 * @brief Draws a rectangle in currently active layer. 00910 * @param Xpos: X position 00911 * @param Ypos: Y position 00912 * @param Width: Rectangle width 00913 * @param Height: Rectangle height 00914 */ 00915 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00916 { 00917 /* Draw horizontal lines */ 00918 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00919 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00920 00921 /* Draw vertical lines */ 00922 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00923 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00924 } 00925 00926 /** 00927 * @brief Draws a circle in currently active layer. 00928 * @param Xpos: X position 00929 * @param Ypos: Y position 00930 * @param Radius: Circle radius 00931 */ 00932 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00933 { 00934 int32_t D; /* Decision Variable */ 00935 uint32_t CurX; /* Current X Value */ 00936 uint32_t CurY; /* Current Y Value */ 00937 00938 D = 3 - (Radius << 1); 00939 CurX = 0; 00940 CurY = Radius; 00941 00942 while (CurX <= CurY) 00943 { 00944 BSP_LCD_DrawPixel((Xpos + CurX), (Ypos - CurY), DrawProp[ActiveLayer].TextColor); 00945 00946 BSP_LCD_DrawPixel((Xpos - CurX), (Ypos - CurY), DrawProp[ActiveLayer].TextColor); 00947 00948 BSP_LCD_DrawPixel((Xpos + CurY), (Ypos - CurX), DrawProp[ActiveLayer].TextColor); 00949 00950 BSP_LCD_DrawPixel((Xpos - CurY), (Ypos - CurX), DrawProp[ActiveLayer].TextColor); 00951 00952 BSP_LCD_DrawPixel((Xpos + CurX), (Ypos + CurY), DrawProp[ActiveLayer].TextColor); 00953 00954 BSP_LCD_DrawPixel((Xpos - CurX), (Ypos + CurY), DrawProp[ActiveLayer].TextColor); 00955 00956 BSP_LCD_DrawPixel((Xpos + CurY), (Ypos + CurX), DrawProp[ActiveLayer].TextColor); 00957 00958 BSP_LCD_DrawPixel((Xpos - CurY), (Ypos + CurX), DrawProp[ActiveLayer].TextColor); 00959 00960 if (D < 0) 00961 { 00962 D += (CurX << 2) + 6; 00963 } 00964 else 00965 { 00966 D += ((CurX - CurY) << 2) + 10; 00967 CurY--; 00968 } 00969 CurX++; 00970 } 00971 } 00972 00973 /** 00974 * @brief Draws an poly-line (between many points) in currently active layer. 00975 * @param Points: Pointer to the points array 00976 * @param PointCount: Number of points 00977 */ 00978 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00979 { 00980 int16_t X = 0, Y = 0; 00981 00982 if(PointCount < 2) 00983 { 00984 return; 00985 } 00986 00987 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00988 00989 while(--PointCount) 00990 { 00991 X = Points->X; 00992 Y = Points->Y; 00993 Points++; 00994 BSP_LCD_DrawLine(X, Y, Points->X, Points->Y); 00995 } 00996 } 00997 00998 /** 00999 * @brief Draws an ellipse on LCD in currently active layer. 01000 * @param Xpos: X position 01001 * @param Ypos: Y position 01002 * @param XRadius: Ellipse X radius 01003 * @param YRadius: Ellipse Y radius 01004 */ 01005 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01006 { 01007 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01008 float K = 0, rad1 = 0, rad2 = 0; 01009 01010 rad1 = XRadius; 01011 rad2 = YRadius; 01012 01013 K = (float)(rad2/rad1); 01014 01015 do { 01016 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 01017 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 01018 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 01019 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 01020 01021 e2 = err; 01022 if (e2 <= x) { 01023 err += ++x*2+1; 01024 if (-y == x && e2 <= y) e2 = 0; 01025 } 01026 if (e2 > y) err += ++y*2+1; 01027 } 01028 while (y <= 0); 01029 } 01030 01031 /** 01032 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp) in currently active layer. 01033 * @param Xpos: Bmp X position in the LCD 01034 * @param Ypos: Bmp Y position in the LCD 01035 * @param pbmp: Pointer to Bmp picture address in the internal Flash 01036 */ 01037 void BSP_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) 01038 { 01039 uint32_t index = 0, width = 0, height = 0, bit_pixel = 0; 01040 uint32_t Address; 01041 uint32_t InputColorMode = 0; 01042 01043 /* Get bitmap data address offset */ 01044 index = *(__IO uint16_t *) (pbmp + 10); 01045 index |= (*(__IO uint16_t *) (pbmp + 12)) << 16; 01046 01047 /* Read bitmap width */ 01048 width = *(uint16_t *) (pbmp + 18); 01049 width |= (*(uint16_t *) (pbmp + 20)) << 16; 01050 01051 /* Read bitmap height */ 01052 height = *(uint16_t *) (pbmp + 22); 01053 height |= (*(uint16_t *) (pbmp + 24)) << 16; 01054 01055 /* Read bit/pixel */ 01056 bit_pixel = *(uint16_t *) (pbmp + 28); 01057 01058 /* Set the address */ 01059 Address = hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (((BSP_LCD_GetXSize()*Ypos) + Xpos)*(4)); 01060 01061 /* Get the layer pixel format */ 01062 if ((bit_pixel/8) == 4) 01063 { 01064 InputColorMode = CM_ARGB8888; 01065 } 01066 else if ((bit_pixel/8) == 2) 01067 { 01068 InputColorMode = CM_RGB565; 01069 } 01070 else 01071 { 01072 InputColorMode = CM_RGB888; 01073 } 01074 01075 /* Bypass the bitmap header */ 01076 pbmp += (index + (width * (height - 1) * (bit_pixel/8))); 01077 01078 /* Convert picture to ARGB8888 pixel format */ 01079 for(index=0; index < height; index++) 01080 { 01081 /* Pixel format conversion */ 01082 LL_ConvertLineToARGB8888((uint32_t *)pbmp, (uint32_t *)Address, width, InputColorMode); 01083 01084 /* Increment the source and destination buffers */ 01085 Address+= (BSP_LCD_GetXSize()*4); 01086 pbmp -= width*(bit_pixel/8); 01087 } 01088 } 01089 01090 /** 01091 * @brief Draws a full rectangle in currently active layer. 01092 * @param Xpos: X position 01093 * @param Ypos: Y position 01094 * @param Width: Rectangle width 01095 * @param Height: Rectangle height 01096 */ 01097 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01098 { 01099 uint32_t Xaddress = 0; 01100 01101 /* Set the text color */ 01102 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01103 01104 /* Get the rectangle start address */ 01105 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 01106 01107 /* Fill the rectangle */ 01108 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Width, Height, (BSP_LCD_GetXSize() - Width), DrawProp[ActiveLayer].TextColor); 01109 } 01110 01111 /** 01112 * @brief Draws a full circle in currently active layer. 01113 * @param Xpos: X position 01114 * @param Ypos: Y position 01115 * @param Radius: Circle radius 01116 */ 01117 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 01118 { 01119 int32_t D; /* Decision Variable */ 01120 uint32_t CurX; /* Current X Value */ 01121 uint32_t CurY; /* Current Y Value */ 01122 01123 D = 3 - (Radius << 1); 01124 01125 CurX = 0; 01126 CurY = Radius; 01127 01128 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01129 01130 while (CurX <= CurY) 01131 { 01132 if(CurY > 0) 01133 { 01134 BSP_LCD_DrawHLine(Xpos - CurY, Ypos + CurX, 2*CurY); 01135 BSP_LCD_DrawHLine(Xpos - CurY, Ypos - CurX, 2*CurY); 01136 } 01137 01138 if(CurX > 0) 01139 { 01140 BSP_LCD_DrawHLine(Xpos - CurX, Ypos - CurY, 2*CurX); 01141 BSP_LCD_DrawHLine(Xpos - CurX, Ypos + CurY, 2*CurX); 01142 } 01143 if (D < 0) 01144 { 01145 D += (CurX << 2) + 6; 01146 } 01147 else 01148 { 01149 D += ((CurX - CurY) << 2) + 10; 01150 CurY--; 01151 } 01152 CurX++; 01153 } 01154 01155 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01156 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 01157 } 01158 01159 /** 01160 * @brief Draws a full poly-line (between many points) in currently active layer. 01161 * @param Points: Pointer to the points array 01162 * @param PointCount: Number of points 01163 */ 01164 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 01165 { 01166 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 01167 uint16_t IMAGE_LEFT = 0, IMAGE_RIGHT = 0, IMAGE_TOP = 0, IMAGE_BOTTOM = 0; 01168 01169 IMAGE_LEFT = IMAGE_RIGHT = Points->X; 01170 IMAGE_TOP= IMAGE_BOTTOM = Points->Y; 01171 01172 for(counter = 1; counter < PointCount; counter++) 01173 { 01174 pixelX = POLY_X(counter); 01175 if(pixelX < IMAGE_LEFT) 01176 { 01177 IMAGE_LEFT = pixelX; 01178 } 01179 if(pixelX > IMAGE_RIGHT) 01180 { 01181 IMAGE_RIGHT = pixelX; 01182 } 01183 01184 pixelY = POLY_Y(counter); 01185 if(pixelY < IMAGE_TOP) 01186 { 01187 IMAGE_TOP = pixelY; 01188 } 01189 if(pixelY > IMAGE_BOTTOM) 01190 { 01191 IMAGE_BOTTOM = pixelY; 01192 } 01193 } 01194 01195 if(PointCount < 2) 01196 { 01197 return; 01198 } 01199 01200 X_center = (IMAGE_LEFT + IMAGE_RIGHT)/2; 01201 Y_center = (IMAGE_BOTTOM + IMAGE_TOP)/2; 01202 01203 X_first = Points->X; 01204 Y_first = Points->Y; 01205 01206 while(--PointCount) 01207 { 01208 X = Points->X; 01209 Y = Points->Y; 01210 Points++; 01211 X2 = Points->X; 01212 Y2 = Points->Y; 01213 01214 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 01215 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 01216 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 01217 } 01218 01219 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 01220 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 01221 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 01222 } 01223 01224 /** 01225 * @brief Draws a full ellipse in currently active layer. 01226 * @param Xpos: X position 01227 * @param Ypos: Y position 01228 * @param XRadius: Ellipse X radius 01229 * @param YRadius: Ellipse Y radius 01230 */ 01231 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01232 { 01233 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01234 float K = 0, rad1 = 0, rad2 = 0; 01235 01236 rad1 = XRadius; 01237 rad2 = YRadius; 01238 01239 K = (float)(rad2/rad1); 01240 01241 do 01242 { 01243 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos+y), (2*(uint16_t)(x/K) + 1)); 01244 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos-y), (2*(uint16_t)(x/K) + 1)); 01245 01246 e2 = err; 01247 if (e2 <= x) 01248 { 01249 err += ++x*2+1; 01250 if (-y == x && e2 <= y) e2 = 0; 01251 } 01252 if (e2 > y) err += ++y*2+1; 01253 } 01254 while (y <= 0); 01255 } 01256 01257 /** 01258 * @brief Switch back on the display if was switched off by previous call of BSP_LCD_DisplayOff(). 01259 * Exit DSI ULPM mode if was allowed and configured in Dsi Configuration. 01260 */ 01261 void BSP_LCD_DisplayOn(void) 01262 { 01263 /* Send Display on DCS command to display */ 01264 HAL_DSI_ShortWrite(&(hdsi_eval), 01265 hdsivideo_handle.VirtualChannelID, 01266 DSI_DCS_SHORT_PKT_WRITE_P1, 01267 OTM8009A_CMD_DISPON, 01268 0x00); 01269 01270 } 01271 01272 /** 01273 * @brief Switch Off the display. 01274 * Enter DSI ULPM mode if was allowed and configured in Dsi Configuration. 01275 */ 01276 void BSP_LCD_DisplayOff(void) 01277 { 01278 /* Send Display off DCS Command to display */ 01279 HAL_DSI_ShortWrite(&(hdsi_eval), 01280 hdsivideo_handle.VirtualChannelID, 01281 DSI_DCS_SHORT_PKT_WRITE_P1, 01282 OTM8009A_CMD_DISPOFF, 01283 0x00); 01284 01285 } 01286 01287 /** 01288 * @brief DCS or Generic short/long write command 01289 * @param NbrParams: Number of parameters. It indicates the write command mode: 01290 * If inferior to 2, a long write command is performed else short. 01291 * @param pParams: Pointer to parameter values table. 01292 * @retval HAL status 01293 */ 01294 void DSI_IO_WriteCmd(uint32_t NbrParams, uint8_t *pParams) 01295 { 01296 if(NbrParams <= 1) 01297 { 01298 HAL_DSI_ShortWrite(&hdsi_eval, LCD_OTM8009A_ID, DSI_DCS_SHORT_PKT_WRITE_P1, pParams[0], pParams[1]); 01299 } 01300 else 01301 { 01302 HAL_DSI_LongWrite(&hdsi_eval, LCD_OTM8009A_ID, DSI_DCS_LONG_PKT_WRITE, NbrParams, pParams[NbrParams], pParams); 01303 } 01304 } 01305 01306 /******************************************************************************* 01307 LTDC, DMA2D and DSI BSP Routines 01308 *******************************************************************************/ 01309 /** 01310 * @brief Handles DMA2D interrupt request. 01311 * @note : Can be surcharged by application code implementation of the function. 01312 */ 01313 __weak void BSP_LCD_DMA2D_IRQHandler(void) 01314 { 01315 HAL_DMA2D_IRQHandler(&hdma2d_eval); 01316 } 01317 01318 /** 01319 * @brief Handles DSI interrupt request. 01320 * @note : Can be surcharged by application code implementation of the function. 01321 */ 01322 __weak void BSP_LCD_DSI_IRQHandler(void) 01323 { 01324 HAL_DSI_IRQHandler(&(hdsi_eval)); 01325 } 01326 01327 01328 /** 01329 * @brief Handles LTDC interrupt request. 01330 * @note : Can be surcharged by application code implementation of the function. 01331 */ 01332 __weak void BSP_LCD_LTDC_IRQHandler(void) 01333 { 01334 HAL_LTDC_IRQHandler(&(hltdc_eval)); 01335 } 01336 01337 /** 01338 * @brief De-Initializes the BSP LCD Msp 01339 * Application can surcharge if needed this function implementation. 01340 */ 01341 __weak void BSP_LCD_MspDeInit(void) 01342 { 01343 /** @brief Disable IRQ of LTDC IP */ 01344 HAL_NVIC_DisableIRQ(LTDC_IRQn); 01345 01346 /** @brief Disable IRQ of DMA2D IP */ 01347 HAL_NVIC_DisableIRQ(DMA2D_IRQn); 01348 01349 /** @brief Disable IRQ of DSI IP */ 01350 HAL_NVIC_DisableIRQ(DSI_IRQn); 01351 01352 /** @brief Force and let in reset state LTDC, DMA2D and DSI Host + Wrapper IPs */ 01353 __HAL_RCC_LTDC_FORCE_RESET(); 01354 __HAL_RCC_DMA2D_FORCE_RESET(); 01355 __HAL_RCC_DSI_FORCE_RESET(); 01356 01357 /** @brief Disable the LTDC, DMA2D and DSI Host and Wrapper clocks */ 01358 __HAL_RCC_LTDC_CLK_DISABLE(); 01359 __HAL_RCC_DMA2D_CLK_DISABLE(); 01360 __HAL_RCC_DSI_CLK_DISABLE(); 01361 } 01362 01363 /** 01364 * @brief Initialize the BSP LCD Msp. 01365 * Application can surcharge if needed this function implementation 01366 */ 01367 __weak void BSP_LCD_MspInit(void) 01368 { 01369 /** @brief Enable the LTDC clock */ 01370 __HAL_RCC_LTDC_CLK_ENABLE(); 01371 01372 /** @brief Toggle Sw reset of LTDC IP */ 01373 __HAL_RCC_LTDC_FORCE_RESET(); 01374 __HAL_RCC_LTDC_RELEASE_RESET(); 01375 01376 /** @brief Enable the DMA2D clock */ 01377 __HAL_RCC_DMA2D_CLK_ENABLE(); 01378 01379 /** @brief Toggle Sw reset of DMA2D IP */ 01380 __HAL_RCC_DMA2D_FORCE_RESET(); 01381 __HAL_RCC_DMA2D_RELEASE_RESET(); 01382 01383 /** @brief Enable DSI Host and wrapper clocks */ 01384 __HAL_RCC_DSI_CLK_ENABLE(); 01385 01386 /** @brief Soft Reset the DSI Host and wrapper */ 01387 __HAL_RCC_DSI_FORCE_RESET(); 01388 __HAL_RCC_DSI_RELEASE_RESET(); 01389 01390 /** @brief NVIC configuration for LTDC interrupt that is now enabled */ 01391 HAL_NVIC_SetPriority(LTDC_IRQn, 3, 0); 01392 HAL_NVIC_EnableIRQ(LTDC_IRQn); 01393 01394 /** @brief NVIC configuration for DMA2D interrupt that is now enabled */ 01395 HAL_NVIC_SetPriority(DMA2D_IRQn, 3, 0); 01396 HAL_NVIC_EnableIRQ(DMA2D_IRQn); 01397 01398 /** @brief NVIC configuration for DSI interrupt that is now enabled */ 01399 HAL_NVIC_SetPriority(DSI_IRQn, 3, 0); 01400 HAL_NVIC_EnableIRQ(DSI_IRQn); 01401 } 01402 01403 /** 01404 * @brief This function handles LTDC Error interrupt Handler. 01405 * @note : Can be surcharged by application code implementation of the function. 01406 */ 01407 01408 __weak void BSP_LCD_LTDC_ER_IRQHandler(void) 01409 { 01410 HAL_LTDC_IRQHandler(&(hltdc_eval)); 01411 } 01412 01413 01414 /** 01415 * @brief Draws a pixel on LCD. 01416 * @param Xpos: X position 01417 * @param Ypos: Y position 01418 * @param RGB_Code: Pixel color in ARGB mode (8-8-8-8) 01419 */ 01420 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) 01421 { 01422 /* Write data value to all SDRAM memory */ 01423 *(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) = RGB_Code; 01424 } 01425 01426 01427 /** 01428 * @brief Draws a character on LCD. 01429 * @param Xpos: Line where to display the character shape 01430 * @param Ypos: Start column address 01431 * @param c: Pointer to the character data 01432 */ 01433 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01434 { 01435 uint32_t i = 0, j = 0; 01436 uint16_t height, width; 01437 uint8_t offset; 01438 uint8_t *pchar; 01439 uint32_t line; 01440 01441 height = DrawProp[ActiveLayer].pFont->Height; 01442 width = DrawProp[ActiveLayer].pFont->Width; 01443 01444 offset = 8 *((width + 7)/8) - width ; 01445 01446 for(i = 0; i < height; i++) 01447 { 01448 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01449 01450 switch(((width + 7)/8)) 01451 { 01452 01453 case 1: 01454 line = pchar[0]; 01455 break; 01456 01457 case 2: 01458 line = (pchar[0]<< 8) | pchar[1]; 01459 break; 01460 01461 case 3: 01462 default: 01463 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01464 break; 01465 } 01466 01467 for (j = 0; j < width; j++) 01468 { 01469 if(line & (1 << (width- j + offset- 1))) 01470 { 01471 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].TextColor); 01472 } 01473 else 01474 { 01475 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].BackColor); 01476 } 01477 } 01478 Ypos++; 01479 } 01480 } 01481 01482 /** 01483 * @brief Fills a triangle (between 3 points). 01484 * @param x1: Point 1 X position 01485 * @param y1: Point 1 Y position 01486 * @param x2: Point 2 X position 01487 * @param y2: Point 2 Y position 01488 * @param x3: Point 3 X position 01489 * @param y3: Point 3 Y position 01490 */ 01491 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01492 { 01493 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01494 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 01495 curpixel = 0; 01496 01497 deltax = ABS(x2 - x1); /* The difference between the x's */ 01498 deltay = ABS(y2 - y1); /* The difference between the y's */ 01499 x = x1; /* Start x off at the first pixel */ 01500 y = y1; /* Start y off at the first pixel */ 01501 01502 if (x2 >= x1) /* The x-values are increasing */ 01503 { 01504 xinc1 = 1; 01505 xinc2 = 1; 01506 } 01507 else /* The x-values are decreasing */ 01508 { 01509 xinc1 = -1; 01510 xinc2 = -1; 01511 } 01512 01513 if (y2 >= y1) /* The y-values are increasing */ 01514 { 01515 yinc1 = 1; 01516 yinc2 = 1; 01517 } 01518 else /* The y-values are decreasing */ 01519 { 01520 yinc1 = -1; 01521 yinc2 = -1; 01522 } 01523 01524 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01525 { 01526 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01527 yinc2 = 0; /* Don't change the y for every iteration */ 01528 den = deltax; 01529 num = deltax / 2; 01530 numadd = deltay; 01531 numpixels = deltax; /* There are more x-values than y-values */ 01532 } 01533 else /* There is at least one y-value for every x-value */ 01534 { 01535 xinc2 = 0; /* Don't change the x for every iteration */ 01536 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01537 den = deltay; 01538 num = deltay / 2; 01539 numadd = deltax; 01540 numpixels = deltay; /* There are more y-values than x-values */ 01541 } 01542 01543 for (curpixel = 0; curpixel <= numpixels; curpixel++) 01544 { 01545 BSP_LCD_DrawLine(x, y, x3, y3); 01546 01547 num += numadd; /* Increase the numerator by the top of the fraction */ 01548 if (num >= den) /* Check if numerator >= denominator */ 01549 { 01550 num -= den; /* Calculate the new numerator value */ 01551 x += xinc1; /* Change the x as appropriate */ 01552 y += yinc1; /* Change the y as appropriate */ 01553 } 01554 x += xinc2; /* Change the x as appropriate */ 01555 y += yinc2; /* Change the y as appropriate */ 01556 } 01557 } 01558 01559 /** 01560 * @brief Fills a buffer. 01561 * @param LayerIndex: Layer index 01562 * @param pDst: Pointer to destination buffer 01563 * @param xSize: Buffer width 01564 * @param ySize: Buffer height 01565 * @param OffLine: Offset 01566 * @param ColorIndex: Color index 01567 */ 01568 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) 01569 { 01570 /* Register to memory mode with ARGB8888 as color Mode */ 01571 hdma2d_eval.Init.Mode = DMA2D_R2M; 01572 hdma2d_eval.Init.ColorMode = DMA2D_ARGB8888; 01573 hdma2d_eval.Init.OutputOffset = OffLine; 01574 01575 hdma2d_eval.Instance = DMA2D; 01576 01577 /* DMA2D Initialization */ 01578 if(HAL_DMA2D_Init(&hdma2d_eval) == HAL_OK) 01579 { 01580 if(HAL_DMA2D_ConfigLayer(&hdma2d_eval, LayerIndex) == HAL_OK) 01581 { 01582 if (HAL_DMA2D_Start(&hdma2d_eval, ColorIndex, (uint32_t)pDst, xSize, ySize) == HAL_OK) 01583 { 01584 /* Polling For DMA transfer */ 01585 HAL_DMA2D_PollForTransfer(&hdma2d_eval, 10); 01586 } 01587 } 01588 } 01589 } 01590 01591 /** 01592 * @brief Converts a line to an ARGB8888 pixel format. 01593 * @param pSrc: Pointer to source buffer 01594 * @param pDst: Output color 01595 * @param xSize: Buffer width 01596 * @param ColorMode: Input color mode 01597 */ 01598 static void LL_ConvertLineToARGB8888(void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode) 01599 { 01600 /* Configure the DMA2D Mode, Color Mode and output offset */ 01601 hdma2d_eval.Init.Mode = DMA2D_M2M_PFC; 01602 hdma2d_eval.Init.ColorMode = DMA2D_ARGB8888; 01603 hdma2d_eval.Init.OutputOffset = 0; 01604 01605 /* Foreground Configuration */ 01606 hdma2d_eval.LayerCfg[1].AlphaMode = DMA2D_NO_MODIF_ALPHA; 01607 hdma2d_eval.LayerCfg[1].InputAlpha = 0xFF; 01608 hdma2d_eval.LayerCfg[1].InputColorMode = ColorMode; 01609 hdma2d_eval.LayerCfg[1].InputOffset = 0; 01610 01611 hdma2d_eval.Instance = DMA2D; 01612 01613 /* DMA2D Initialization */ 01614 if(HAL_DMA2D_Init(&hdma2d_eval) == HAL_OK) 01615 { 01616 if(HAL_DMA2D_ConfigLayer(&hdma2d_eval, 1) == HAL_OK) 01617 { 01618 if (HAL_DMA2D_Start(&hdma2d_eval, (uint32_t)pSrc, (uint32_t)pDst, xSize, 1) == HAL_OK) 01619 { 01620 /* Polling For DMA transfer */ 01621 HAL_DMA2D_PollForTransfer(&hdma2d_eval, 10); 01622 } 01623 } 01624 } 01625 } 01626 01627 /** 01628 * @} 01629 */ 01630 01631 /** 01632 * @} 01633 */ 01634 01635 /** 01636 * @} 01637 */ 01638 01639 /** 01640 * @} 01641 */ 01642 01643 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 10:58:45 for STM32F469I-Discovery BSP User Manual by
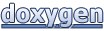