STM32F469I-Discovery BSP User Manual
|
stm32469i_discovery.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_discovery.h 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 13-January-2016 00007 * @brief This file contains definitions for STM32469I-Discovery LEDs, 00008 * push-buttons hardware resources. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32469I_DISCOVERY_H 00041 #define __STM32469I_DISCOVERY_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32f4xx_hal.h" 00050 00051 /** @addtogroup BSP 00052 * @{ 00053 */ 00054 00055 /** @addtogroup STM32469I_Discovery 00056 * @{ 00057 */ 00058 00059 /** @addtogroup STM32469I_Discovery_LOW_LEVEL 00060 * @{ 00061 */ 00062 00063 /** @defgroup STM32469I_Discovery_LOW_LEVEL_Exported_Types STM32469I Discovery LOW LEVEL Exported Types 00064 * @{ 00065 */ 00066 00067 /** @brief Led_TypeDef 00068 * STM32469I_Discovery board leds definitions. 00069 */ 00070 typedef enum 00071 { 00072 LED1 = 0, 00073 LED_GREEN = LED1, 00074 LED2 = 1, 00075 LED_ORANGE = LED2, 00076 LED3 = 2, 00077 LED_RED = LED3, 00078 LED4 = 3, 00079 LED_BLUE = LED4 00080 00081 } Led_TypeDef; 00082 00083 /** @brief Button_TypeDef 00084 * STM32469I_Discovery board Buttons definitions. 00085 */ 00086 typedef enum 00087 { 00088 BUTTON_WAKEUP = 0 00089 } Button_TypeDef; 00090 00091 #define BUTTON_USER BUTTON_WAKEUP 00092 00093 /** @brief ButtonMode_TypeDef 00094 * STM32469I_Discovery board Buttons Modes definitions. 00095 */ 00096 typedef enum 00097 { 00098 BUTTON_MODE_GPIO = 0, 00099 BUTTON_MODE_EXTI = 1 00100 00101 } ButtonMode_TypeDef; 00102 00103 /** @addtogroup Exported_types 00104 * @{ 00105 */ 00106 typedef enum 00107 { 00108 PB_SET = 0, 00109 PB_RESET = !PB_SET 00110 } ButtonValue_TypeDef; 00111 00112 00113 /** @brief DISCO_Status_TypeDef 00114 * STM32469I_DISCO board Status return possible values. 00115 */ 00116 typedef enum 00117 { 00118 DISCO_OK = 0, 00119 DISCO_ERROR = 1 00120 00121 } DISCO_Status_TypeDef; 00122 00123 /** 00124 * @} 00125 */ 00126 00127 /** 00128 * @} 00129 */ 00130 00131 /** @defgroup STM32469I_Discovery_LOW_LEVEL_Exported_Constants STM32469I Discovery LOW LEVEL Exported Constants 00132 * @{ 00133 */ 00134 00135 00136 /** @defgroup STM32469I_Discovery_LOW_LEVEL_LED STM32469I Discovery LOW LEVEL LED 00137 * @{ 00138 */ 00139 /* Always four leds for all revisions of Discovery boards */ 00140 #define LEDn ((uint8_t)4) 00141 00142 00143 /* 4 Leds are connected to MCU directly on PG6, PD4, PD5, PK3 */ 00144 #define LED1_GPIO_PORT ((GPIO_TypeDef*)GPIOG) 00145 #define LED2_GPIO_PORT ((GPIO_TypeDef*)GPIOD) 00146 #define LED3_GPIO_PORT ((GPIO_TypeDef*)GPIOD) 00147 #define LED4_GPIO_PORT ((GPIO_TypeDef*)GPIOK) 00148 00149 #define LED1_GPIO_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00150 #define LED1_GPIO_CLK_DISABLE() __HAL_RCC_GPIOG_CLK_DISABLE() 00151 #define LED2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00152 #define LED2_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00153 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00154 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00155 #define LED4_GPIO_CLK_ENABLE() __HAL_RCC_GPIOK_CLK_ENABLE() 00156 #define LED4_GPIO_CLK_DISABLE() __HAL_RCC_GPIOK_CLK_DISABLE() 00157 00158 #define LED1_PIN ((uint32_t)GPIO_PIN_6) 00159 #define LED2_PIN ((uint32_t)GPIO_PIN_4) 00160 #define LED3_PIN ((uint32_t)GPIO_PIN_5) 00161 #define LED4_PIN ((uint32_t)GPIO_PIN_3) 00162 /** 00163 * @} 00164 */ 00165 00166 /** @addtogroup STM32469I_Discovery_LOW_LEVEL_BUTTON STM32469I Discovery LOW LEVEL BUTTON 00167 * @{ 00168 */ 00169 /* Only one User/Wakeup button */ 00170 #define BUTTONn ((uint8_t)1) 00171 00172 /** 00173 * @brief Wakeup push-button 00174 */ 00175 #define WAKEUP_BUTTON_PIN GPIO_PIN_0 00176 #define WAKEUP_BUTTON_GPIO_PORT GPIOA 00177 #define WAKEUP_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00178 #define WAKEUP_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00179 #define WAKEUP_BUTTON_EXTI_IRQn EXTI0_IRQn 00180 00181 /* Define the USER button as an alias of the Wakeup button */ 00182 #define USER_BUTTON_PIN WAKEUP_BUTTON_PIN 00183 #define USER_BUTTON_GPIO_PORT WAKEUP_BUTTON_GPIO_PORT 00184 #define USER_BUTTON_GPIO_CLK_ENABLE() WAKEUP_BUTTON_GPIO_CLK_ENABLE() 00185 #define USER_BUTTON_GPIO_CLK_DISABLE() WAKEUP_BUTTON_GPIO_CLK_DISABLE() 00186 #define USER_BUTTON_EXTI_IRQn WAKEUP_BUTTON_EXTI_IRQn 00187 00188 #define BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00189 00190 /** 00191 * @} 00192 */ 00193 00194 /** 00195 * @brief Discovery Pins definition 00196 * TODO : to be modified/reviewed 00197 */ 00198 #define AUDIO_INT_PIN GPIO_PIN_7 00199 #define AUDIO_INT_PORT GPIOB 00200 #define AUDIO_INT_PORT_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00201 00202 #define OTG_FS1_OVER_CURRENT_PIN GPIO_PIN_7 00203 #define OTG_FS1_OVER_CURRENT_PORT GPIOB 00204 #define OTG_FS1_OVER_CURRENT_PORT_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00205 00206 #define OTG_FS1_POWER_SWITCH_PIN GPIO_PIN_2 00207 #define OTG_FS1_POWER_SWITCH_PORT GPIOB 00208 #define OTG_FS1_POWER_SWITCH_PORT_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00209 00210 /** 00211 * @brief SD-detect signal 00212 */ 00213 #define SD_DETECT_PIN ((uint32_t)GPIO_PIN_2) 00214 #define SD_DETECT_GPIO_PORT ((GPIO_TypeDef*)GPIOG) 00215 #define SD_DETECT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00216 #define SD_DETECT_GPIO_CLK_DISABLE() __HAL_RCC_GPIOG_CLK_DISABLE() 00217 #define SD_DETECT_EXTI_IRQn EXTI2_IRQn 00218 00219 /** 00220 * @brief TS_INT signal from TouchScreen when it is configured in interrupt mode 00221 * GPIOJ5 is used for that purpose on Manta Dragon Discovery board 00222 */ 00223 #define TS_INT_PIN ((uint32_t)GPIO_PIN_5) 00224 #define TS_INT_GPIO_PORT ((GPIO_TypeDef*)GPIOJ) 00225 #define TS_INT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOJ_CLK_ENABLE() 00226 #define TS_INT_GPIO_CLK_DISABLE() __HAL_RCC_GPIOJ_CLK_DISABLE() 00227 #define TS_INT_EXTI_IRQn EXTI9_5_IRQn 00228 00229 /** 00230 * @brief TouchScreen FT6206 Slave I2C address 00231 */ 00232 #define TS_I2C_ADDRESS ((uint16_t)0x54) 00233 00234 00235 /** 00236 * @brief Audio I2C Slave address 00237 */ 00238 #define AUDIO_I2C_ADDRESS ((uint16_t)0x94) 00239 00240 /** 00241 * @brief EEPROM I2C Slave address 1 00242 */ 00243 #define EEPROM_I2C_ADDRESS_A01 ((uint16_t)0xA0) 00244 00245 /** 00246 * @brief EEPROM I2C Slave address 2 00247 */ 00248 #define EEPROM_I2C_ADDRESS_A02 ((uint16_t)0xA6) 00249 00250 /** 00251 * @brief I2C clock speed configuration (in Hz) 00252 * WARNING: 00253 * Make sure that this define is not already declared in other files 00254 * It can be used in parallel by other modules. 00255 */ 00256 #ifndef I2C1_SCL_FREQ_KHZ 00257 #define I2C1_SCL_FREQ_KHZ 400000 /*!< f(I2C_SCL) = 400 kHz */ 00258 #endif /* I2C1_SCL_FREQ_KHZ */ 00259 00260 /** 00261 * @brief User can use this section to tailor I2C1/I2C1 instance used and associated 00262 * resources. 00263 * Definition for I2C1 clock resources 00264 */ 00265 #define DISCO_I2C1 I2C1 00266 #define DISCO_I2C1_CLK_ENABLE() __HAL_RCC_I2C1_CLK_ENABLE() 00267 #define DISCO_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00268 #define DISCO_I2C1_SCL_SDA_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00269 00270 #define DISCO_I2C1_FORCE_RESET() __HAL_RCC_I2C1_FORCE_RESET() 00271 #define DISCO_I2C1_RELEASE_RESET() __HAL_RCC_I2C1_RELEASE_RESET() 00272 00273 /** @brief Definition for I2C1 Pins 00274 */ 00275 #define DISCO_I2C1_SCL_PIN GPIO_PIN_8 /*!< PB8 */ 00276 #define DISCO_I2C1_SCL_SDA_GPIO_PORT GPIOB 00277 #define DISCO_I2C1_SCL_SDA_AF GPIO_AF4_I2C1 00278 #define DISCO_I2C1_SDA_PIN GPIO_PIN_9 /*!< PB9 */ 00279 00280 /** @brief Definition of I2C interrupt requests 00281 */ 00282 #define DISCO_I2C1_EV_IRQn I2C1_EV_IRQn 00283 #define DISCO_I2C1_ER_IRQn I2C1_ER_IRQn 00284 00285 00286 00287 /** 00288 * @brief I2C2 clock speed configuration (in Hz) 00289 * WARNING: 00290 * Make sure that this define is not already declared in other files 00291 * It can be used in parallel by other modules. 00292 */ 00293 #ifndef I2C2_SCL_FREQ_KHZ 00294 #define I2C2_SCL_FREQ_KHZ 100000 /*!< f(I2C2_SCL) < 100 kHz */ 00295 #endif /* I2C2_SCL_FREQ_KHZ */ 00296 00297 /** 00298 * @brief User can use this section to tailor I2C2/I2C2 instance used and associated 00299 * resources (audio codec). 00300 * Definition for I2C2 clock resources 00301 */ 00302 #define DISCO_I2C2 I2C2 00303 #define DISCO_I2C2_CLK_ENABLE() __HAL_RCC_I2C2_CLK_ENABLE() 00304 #define DISCO_I2C2_SCL_SDA_GPIO_CLK_ENABLE() __HAL_RCC_GPIOH_CLK_ENABLE() 00305 00306 #define DISCO_I2C2_FORCE_RESET() __HAL_RCC_I2C2_FORCE_RESET() 00307 #define DISCO_I2C2_RELEASE_RESET() __HAL_RCC_I2C2_RELEASE_RESET() 00308 00309 /** @brief Definition for I2C2 Pins 00310 */ 00311 #define DISCO_I2C2_SCL_PIN GPIO_PIN_4 /*!< PH4 */ 00312 #define DISCO_I2C2_SCL_SDA_GPIO_PORT GPIOH 00313 #define DISCO_I2C2_SCL_SDA_AF GPIO_AF4_I2C2 00314 #define DISCO_I2C2_SDA_PIN GPIO_PIN_5 /*!< PH5 */ 00315 00316 /** @brief Definition of I2C2 interrupt requests 00317 */ 00318 #define DISCO_I2C2_EV_IRQn I2C2_EV_IRQn 00319 #define DISCO_I2C2_ER_IRQn I2C2_ER_IRQn 00320 00321 00322 /** 00323 * @} 00324 */ 00325 00326 /** @defgroup STM32469I_Discovery_LOW_LEVEL_Exported_Macros STM32469I Discovery LOW LEVEL Exported Macros 00327 * @{ 00328 */ 00329 /** 00330 * @} 00331 */ 00332 00333 /** @defgroup STM32469I_Discovery_LOW_LEVEL_Exported_Functions STM32469I Discovery LOW LEVEL Exported Functions 00334 * @{ 00335 */ 00336 uint32_t BSP_GetVersion(void); 00337 void BSP_LED_Init(Led_TypeDef Led); 00338 void BSP_LED_DeInit(Led_TypeDef Led); 00339 void BSP_LED_On(Led_TypeDef Led); 00340 void BSP_LED_Off(Led_TypeDef Led); 00341 void BSP_LED_Toggle(Led_TypeDef Led); 00342 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode); 00343 void BSP_PB_DeInit(Button_TypeDef Button); 00344 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00345 00346 /** 00347 * @} 00348 */ 00349 00350 /** 00351 * @} 00352 */ 00353 00354 /** 00355 * @} 00356 */ 00357 00358 /** 00359 * @} 00360 */ 00361 00362 00363 #ifdef __cplusplus 00364 } 00365 #endif 00366 00367 #endif /* __STM32469I_DISCOVERY_H */ 00368 00369 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 10:58:45 for STM32F469I-Discovery BSP User Manual by
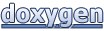