STM32F469I-Discovery BSP User Manual
|
stm32469i_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_discovery.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 13-January-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons, external SDRAM, external QSPI Flash, RF EEPROM, 00009 * available on STM32469I-Discovery 00010 * board (MB1189) RevA/B from STMicroelectronics. 00011 ****************************************************************************** 00012 * @attention 00013 * 00014 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00015 * 00016 * Redistribution and use in source and binary forms, with or without modification, 00017 * are permitted provided that the following conditions are met: 00018 * 1. Redistributions of source code must retain the above copyright notice, 00019 * this list of conditions and the following disclaimer. 00020 * 2. Redistributions in binary form must reproduce the above copyright notice, 00021 * this list of conditions and the following disclaimer in the documentation 00022 * and/or other materials provided with the distribution. 00023 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00024 * may be used to endorse or promote products derived from this software 00025 * without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00028 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00029 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00030 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00031 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00032 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00033 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00034 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00035 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00036 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00037 * 00038 ****************************************************************************** 00039 */ 00040 00041 /* Includes ------------------------------------------------------------------*/ 00042 #include "stm32469i_discovery.h" 00043 00044 /** @defgroup BSP BSP 00045 * @{ 00046 */ 00047 00048 /** @defgroup STM32469I_Discovery STM32469I Discovery 00049 * @{ 00050 */ 00051 00052 /** @defgroup STM32469I_Discovery_LOW_LEVEL STM32469I Discovery LOW LEVEL 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32469I_Discovery_LOW_LEVEL_Private_TypesDefinitions STM32469I Discovery LOW LEVEL Private TypesDefinitions 00057 * @{ 00058 */ 00059 /** 00060 * @} 00061 */ 00062 00063 /** @defgroup STM32469I_Discovery_LOW_LEVEL_Private_Defines STM32469I Discovery LOW LEVEL Private Defines 00064 * @{ 00065 */ 00066 /** 00067 * @brief STM32469I Discovery BSP Driver version number V1.0.2 00068 */ 00069 #define __STM32469I_DISCOVERY_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00070 #define __STM32469I_DISCOVERY_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00071 #define __STM32469I_DISCOVERY_BSP_VERSION_SUB2 (0x02) /*!< [15:8] sub2 version */ 00072 #define __STM32469I_DISCOVERY_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00073 #define __STM32469I_DISCOVERY_BSP_VERSION ((__STM32469I_DISCOVERY_BSP_VERSION_MAIN << 24)\ 00074 |(__STM32469I_DISCOVERY_BSP_VERSION_SUB1 << 16)\ 00075 |(__STM32469I_DISCOVERY_BSP_VERSION_SUB2 << 8 )\ 00076 |(__STM32469I_DISCOVERY_BSP_VERSION_RC)) 00077 /** 00078 * @} 00079 */ 00080 00081 /** @defgroup STM32469I_Discovery_LOW_LEVEL_Private_Macros STM32469I Discovery LOW LEVEL Private Macros 00082 * @{ 00083 */ 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM32469I_Discovery_LOW_LEVEL_Private_Variables STM32469I Discovery LOW LEVEL Private Variables 00089 * @{ 00090 */ 00091 uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00092 LED2_PIN, 00093 LED3_PIN, 00094 LED4_PIN}; 00095 00096 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED1_GPIO_PORT, 00097 LED2_GPIO_PORT, 00098 LED3_GPIO_PORT, 00099 LED4_GPIO_PORT}; 00100 00101 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT }; 00102 00103 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN }; 00104 00105 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn }; 00106 00107 00108 static I2C_HandleTypeDef heval_I2c1; 00109 static I2C_HandleTypeDef heval_I2c2; 00110 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup STM32469I_Discovery_LOW_LEVEL_Private_FunctionPrototypes STM32469I Discovery LOW LEVEL Private FunctionPrototypes 00116 * @{ 00117 */ 00118 static void I2C1_MspInit(void); 00119 static void I2C2_MspInit(void); 00120 static void I2C1_Init(void); 00121 static void I2C2_Init(void); 00122 00123 #if defined(USE_IOEXPANDER) 00124 static void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00125 static uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg); 00126 #endif /* USE_IOEXPANDER */ 00127 static HAL_StatusTypeDef I2C1_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00128 static HAL_StatusTypeDef I2C2_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00129 static HAL_StatusTypeDef I2C1_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00130 static HAL_StatusTypeDef I2C2_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00131 static HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00132 static void I2C1_Error(uint8_t Addr); 00133 static void I2C2_Error(uint8_t Addr); 00134 00135 /* AUDIO IO functions */ 00136 void AUDIO_IO_Init(void); 00137 void AUDIO_IO_DeInit(void); 00138 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00139 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00140 void AUDIO_IO_Delay(uint32_t Delay); 00141 00142 00143 /* I2C EEPROM IO function */ 00144 void EEPROM_IO_Init(void); 00145 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00146 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00147 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00148 00149 /* TouchScreen (TS) IO functions */ 00150 void TS_IO_Init(void); 00151 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00152 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00153 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00154 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00155 void TS_IO_Delay(uint32_t Delay); 00156 void OTM8009A_IO_Delay(uint32_t Delay); 00157 /** 00158 * @} 00159 */ 00160 00161 /** @defgroup STM32469I_Discovery_BSP_Public_Functions STM32469I Discovery BSP Public Functions 00162 * @{ 00163 */ 00164 00165 /** 00166 * @brief This method returns the STM32469I Discovery BSP Driver revision 00167 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00168 */ 00169 uint32_t BSP_GetVersion(void) 00170 { 00171 return __STM32469I_DISCOVERY_BSP_VERSION; 00172 } 00173 00174 /** 00175 * @brief Configures LED GPIO. 00176 * @param Led: LED to be configured. 00177 * This parameter can be one of the following values: 00178 * @arg LED1 00179 * @arg LED2 00180 * @arg LED3 00181 * @arg LED4 00182 */ 00183 void BSP_LED_Init(Led_TypeDef Led) 00184 { 00185 GPIO_InitTypeDef gpio_init_structure; 00186 00187 if (Led <= LED4) 00188 { 00189 /* Configure the GPIO_LED pin */ 00190 gpio_init_structure.Pin = GPIO_PIN[Led]; 00191 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00192 gpio_init_structure.Pull = GPIO_PULLUP; 00193 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00194 00195 switch(Led) 00196 { 00197 case LED1 : 00198 LED1_GPIO_CLK_ENABLE(); 00199 break; 00200 case LED2 : 00201 LED2_GPIO_CLK_ENABLE(); 00202 break; 00203 case LED3 : 00204 LED3_GPIO_CLK_ENABLE(); 00205 break; 00206 case LED4 : 00207 LED4_GPIO_CLK_ENABLE(); 00208 break; 00209 default : 00210 break; 00211 00212 } /* end switch */ 00213 00214 HAL_GPIO_Init(GPIO_PORT[Led], &gpio_init_structure); 00215 00216 /* By default, turn off LED by setting a high level on corresponding GPIO */ 00217 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00218 00219 } /* of if (Led <= LED4) */ 00220 00221 } 00222 00223 00224 /** 00225 * @brief DeInit LEDs. 00226 * @param Led: LED to be configured. 00227 * This parameter can be one of the following values: 00228 * @arg LED1 00229 * @arg LED2 00230 * @arg LED3 00231 * @arg LED4 00232 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00233 */ 00234 void BSP_LED_DeInit(Led_TypeDef Led) 00235 { 00236 GPIO_InitTypeDef gpio_init_structure; 00237 00238 if (Led <= LED4) 00239 { 00240 /* DeInit the GPIO_LED pin */ 00241 gpio_init_structure.Pin = GPIO_PIN[Led]; 00242 00243 /* Turn off LED */ 00244 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00245 HAL_GPIO_DeInit(GPIO_PORT[Led], gpio_init_structure.Pin); 00246 } 00247 00248 } 00249 00250 /** 00251 * @brief Turns selected LED On. 00252 * @param Led: LED to be set on 00253 * This parameter can be one of the following values: 00254 * @arg LED1 00255 * @arg LED2 00256 * @arg LED3 00257 * @arg LED4 00258 */ 00259 void BSP_LED_On(Led_TypeDef Led) 00260 { 00261 if (Led <= LED4) 00262 { 00263 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00264 } 00265 00266 } 00267 00268 /** 00269 * @brief Turns selected LED Off. 00270 * @param Led: LED to be set off 00271 * This parameter can be one of the following values: 00272 * @arg LED1 00273 * @arg LED2 00274 * @arg LED3 00275 * @arg LED4 00276 */ 00277 void BSP_LED_Off(Led_TypeDef Led) 00278 { 00279 if (Led <= LED4) 00280 { 00281 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00282 } 00283 } 00284 00285 /** 00286 * @brief Toggles the selected LED. 00287 * @param Led: LED to be toggled 00288 * This parameter can be one of the following values: 00289 * @arg LED1 00290 * @arg LED2 00291 * @arg LED3 00292 * @arg LED4 00293 */ 00294 void BSP_LED_Toggle(Led_TypeDef Led) 00295 { 00296 if (Led <= LED4) 00297 { 00298 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00299 } 00300 } 00301 00302 /** 00303 * @brief Configures button GPIO and EXTI Line. 00304 * @param Button: Button to be configured 00305 * This parameter can be one of the following values: 00306 * @arg BUTTON_WAKEUP: Wakeup Push Button 00307 * @arg BUTTON_USER: User Push Button 00308 * @param Button_Mode: Button mode 00309 * This parameter can be one of the following values: 00310 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00311 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00312 * with interrupt generation capability 00313 */ 00314 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00315 { 00316 GPIO_InitTypeDef gpio_init_structure; 00317 00318 /* Enable the BUTTON clock */ 00319 BUTTON_GPIO_CLK_ENABLE(); 00320 00321 if(Button_Mode == BUTTON_MODE_GPIO) 00322 { 00323 /* Configure Button pin as input */ 00324 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00325 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00326 gpio_init_structure.Pull = GPIO_NOPULL; 00327 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00328 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00329 } 00330 00331 if(Button_Mode == BUTTON_MODE_EXTI) 00332 { 00333 /* Configure Button pin as input with External interrupt */ 00334 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00335 gpio_init_structure.Pull = GPIO_NOPULL; 00336 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00337 00338 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00339 00340 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00341 00342 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00343 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00344 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00345 } 00346 } 00347 00348 /** 00349 * @brief Push Button DeInit. 00350 * @param Button: Button to be configured 00351 * This parameter can be one of the following values: 00352 * @arg BUTTON_WAKEUP: Wakeup Push Button 00353 * @arg BUTTON_USER: User Push Button 00354 * @note PB DeInit does not disable the GPIO clock 00355 */ 00356 void BSP_PB_DeInit(Button_TypeDef Button) 00357 { 00358 GPIO_InitTypeDef gpio_init_structure; 00359 00360 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00361 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00362 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00363 } 00364 00365 00366 /** 00367 * @brief Returns the selected button state. 00368 * @param Button: Button to be checked 00369 * This parameter can be one of the following values: 00370 * @arg BUTTON_WAKEUP: Wakeup Push Button 00371 * @arg BUTTON_USER: User Push Button 00372 * @retval The Button GPIO pin value 00373 */ 00374 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00375 { 00376 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00377 } 00378 00379 /** 00380 * @} 00381 */ 00382 00383 /** @defgroup STM32469I_Discovery_LOW_LEVEL_Private_Functions STM32469I Discovery LOW LEVEL Private Functions 00384 * @{ 00385 */ 00386 00387 00388 /******************************************************************************* 00389 BUS OPERATIONS 00390 *******************************************************************************/ 00391 00392 /******************************* I2C Routines *********************************/ 00393 /** 00394 * @brief Initializes I2C MSP. 00395 */ 00396 static void I2C1_MspInit(void) 00397 { 00398 GPIO_InitTypeDef gpio_init_structure; 00399 00400 /*** Configure the GPIOs ***/ 00401 /* Enable GPIO clock */ 00402 DISCO_I2C1_SCL_SDA_GPIO_CLK_ENABLE(); 00403 00404 /* Configure I2C Tx as alternate function */ 00405 gpio_init_structure.Pin = DISCO_I2C1_SCL_PIN; 00406 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00407 gpio_init_structure.Pull = GPIO_NOPULL; 00408 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00409 gpio_init_structure.Alternate = DISCO_I2C1_SCL_SDA_AF; 00410 HAL_GPIO_Init(DISCO_I2C1_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00411 00412 /* Configure I2C Rx as alternate function */ 00413 gpio_init_structure.Pin = DISCO_I2C1_SDA_PIN; 00414 HAL_GPIO_Init(DISCO_I2C1_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00415 00416 /*** Configure the I2C peripheral ***/ 00417 /* Enable I2C clock */ 00418 DISCO_I2C1_CLK_ENABLE(); 00419 00420 /* Force the I2C peripheral clock reset */ 00421 DISCO_I2C1_FORCE_RESET(); 00422 00423 /* Release the I2C peripheral clock reset */ 00424 DISCO_I2C1_RELEASE_RESET(); 00425 00426 /* Enable and set I2C1 Interrupt to a lower priority */ 00427 HAL_NVIC_SetPriority(DISCO_I2C1_EV_IRQn, 0x05, 0); 00428 HAL_NVIC_EnableIRQ(DISCO_I2C1_EV_IRQn); 00429 00430 /* Enable and set I2C1 Interrupt to a lower priority */ 00431 HAL_NVIC_SetPriority(DISCO_I2C1_ER_IRQn, 0x05, 0); 00432 HAL_NVIC_EnableIRQ(DISCO_I2C1_ER_IRQn); 00433 } 00434 00435 /** 00436 * @brief Initializes I2C MSP. 00437 */ 00438 static void I2C2_MspInit(void) 00439 { 00440 GPIO_InitTypeDef gpio_init_structure; 00441 00442 /*** Configure the GPIOs ***/ 00443 /* Enable GPIO clock */ 00444 DISCO_I2C2_SCL_SDA_GPIO_CLK_ENABLE(); 00445 00446 /* Configure I2C Tx as alternate function */ 00447 gpio_init_structure.Pin = DISCO_I2C2_SCL_PIN; 00448 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00449 gpio_init_structure.Pull = GPIO_NOPULL; 00450 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00451 gpio_init_structure.Alternate = DISCO_I2C2_SCL_SDA_AF; 00452 HAL_GPIO_Init(DISCO_I2C2_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00453 00454 /* Configure I2C Rx as alternate function */ 00455 gpio_init_structure.Pin = DISCO_I2C2_SDA_PIN; 00456 HAL_GPIO_Init(DISCO_I2C2_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00457 00458 /*** Configure the I2C peripheral ***/ 00459 /* Enable I2C clock */ 00460 DISCO_I2C2_CLK_ENABLE(); 00461 00462 /* Force the I2C peripheral clock reset */ 00463 DISCO_I2C2_FORCE_RESET(); 00464 00465 /* Release the I2C peripheral clock reset */ 00466 DISCO_I2C2_RELEASE_RESET(); 00467 00468 /* Enable and set I2C1 Interrupt to a lower priority */ 00469 HAL_NVIC_SetPriority(DISCO_I2C2_EV_IRQn, 0x05, 0); 00470 HAL_NVIC_EnableIRQ(DISCO_I2C2_EV_IRQn); 00471 00472 /* Enable and set I2C1 Interrupt to a lower priority */ 00473 HAL_NVIC_SetPriority(DISCO_I2C2_ER_IRQn, 0x05, 0); 00474 HAL_NVIC_EnableIRQ(DISCO_I2C2_ER_IRQn); 00475 } 00476 00477 /** 00478 * @brief Initializes I2C HAL. 00479 */ 00480 static void I2C1_Init(void) 00481 { 00482 if(HAL_I2C_GetState(&heval_I2c1) == HAL_I2C_STATE_RESET) 00483 { 00484 heval_I2c1.Instance = I2C1; 00485 heval_I2c1.Init.ClockSpeed = I2C1_SCL_FREQ_KHZ; 00486 heval_I2c1.Init.DutyCycle = I2C_DUTYCYCLE_2; 00487 heval_I2c1.Init.OwnAddress1 = 0; 00488 heval_I2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00489 heval_I2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00490 heval_I2c1.Init.OwnAddress2 = 0; 00491 heval_I2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00492 heval_I2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00493 00494 /* Init the I2C */ 00495 I2C1_MspInit(); 00496 HAL_I2C_Init(&heval_I2c1); 00497 } 00498 } 00499 00500 /** 00501 * @brief Initializes I2C HAL. 00502 */ 00503 static void I2C2_Init(void) 00504 { 00505 if(HAL_I2C_GetState(&heval_I2c2) == HAL_I2C_STATE_RESET) 00506 { 00507 heval_I2c2.Instance = I2C2; 00508 heval_I2c2.Init.ClockSpeed = I2C2_SCL_FREQ_KHZ; 00509 heval_I2c2.Init.DutyCycle = I2C_DUTYCYCLE_2; 00510 heval_I2c2.Init.OwnAddress1 = 0; 00511 heval_I2c2.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00512 heval_I2c2.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00513 heval_I2c2.Init.OwnAddress2 = 0; 00514 heval_I2c2.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00515 heval_I2c2.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00516 00517 /* Init the I2C */ 00518 I2C2_MspInit(); 00519 HAL_I2C_Init(&heval_I2c2); 00520 } 00521 } 00522 00523 /** 00524 * @brief Writes a single data. 00525 * @param Addr: I2C address 00526 * @param Reg: Register address 00527 * @param Value: Data to be written 00528 */ 00529 static void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00530 { 00531 HAL_StatusTypeDef status = HAL_OK; 00532 00533 status = HAL_I2C_Mem_Write(&heval_I2c1, 00534 Addr, 00535 (uint16_t)Reg, 00536 I2C_MEMADD_SIZE_8BIT, 00537 &Value, 00538 1, 00539 100); 00540 00541 /* Check the communication status */ 00542 if(status != HAL_OK) 00543 { 00544 /* Execute user timeout callback */ 00545 I2C1_Error(Addr); 00546 } 00547 } 00548 00549 /** 00550 * @brief Reads a single data. 00551 * @param Addr: I2C address 00552 * @param Reg: Register address 00553 * @retval Read data 00554 */ 00555 static uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg) 00556 { 00557 HAL_StatusTypeDef status = HAL_OK; 00558 uint8_t Value = 0; 00559 00560 status = HAL_I2C_Mem_Read(&heval_I2c1, 00561 Addr, 00562 Reg, 00563 I2C_MEMADD_SIZE_8BIT, 00564 &Value, 00565 1, 00566 1000); 00567 00568 /* Check the communication status */ 00569 if(status != HAL_OK) 00570 { 00571 /* Execute user timeout callback */ 00572 I2C1_Error(Addr); 00573 } 00574 return Value; 00575 } 00576 00577 /** 00578 * @brief Reads multiple data. 00579 * @param Addr: I2C address 00580 * @param Reg: Reg address 00581 * @param MemAddress: memory address 00582 * @param Buffer: Pointer to data buffer 00583 * @param Length: Length of the data 00584 * @retval HAL status 00585 */ 00586 static HAL_StatusTypeDef I2C1_ReadMultiple(uint8_t Addr, 00587 uint16_t Reg, 00588 uint16_t MemAddress, 00589 uint8_t *Buffer, 00590 uint16_t Length) 00591 { 00592 HAL_StatusTypeDef status = HAL_OK; 00593 00594 status = HAL_I2C_Mem_Read(&heval_I2c1, 00595 Addr, 00596 (uint16_t)Reg, 00597 MemAddress, 00598 Buffer, 00599 Length, 00600 1000); 00601 00602 /* Check the communication status */ 00603 if(status != HAL_OK) 00604 { 00605 /* I2C error occured */ 00606 I2C1_Error(Addr); 00607 } 00608 return status; 00609 } 00610 00611 static HAL_StatusTypeDef I2C2_ReadMultiple(uint8_t Addr, 00612 uint16_t Reg, 00613 uint16_t MemAddress, 00614 uint8_t *Buffer, 00615 uint16_t Length) 00616 { 00617 HAL_StatusTypeDef status = HAL_OK; 00618 00619 status = HAL_I2C_Mem_Read(&heval_I2c2, 00620 Addr, 00621 (uint16_t)Reg, 00622 MemAddress, 00623 Buffer, 00624 Length, 00625 1000); 00626 00627 /* Check the communication status */ 00628 if(status != HAL_OK) 00629 { 00630 /* I2C2 error occured */ 00631 I2C2_Error(Addr); 00632 } 00633 return status; 00634 } 00635 00636 /** 00637 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00638 * @param Addr: Device address on BUS Bus. 00639 * @param Reg: The target register address to write 00640 * @param MemAddress: memory address 00641 * @param Buffer: The target register value to be written 00642 * @param Length: buffer size to be written 00643 * @retval HAL status 00644 */ 00645 static HAL_StatusTypeDef I2C1_WriteMultiple(uint8_t Addr, 00646 uint16_t Reg, 00647 uint16_t MemAddress, 00648 uint8_t *Buffer, 00649 uint16_t Length) 00650 { 00651 HAL_StatusTypeDef status = HAL_OK; 00652 00653 status = HAL_I2C_Mem_Write(&heval_I2c1, 00654 Addr, 00655 (uint16_t)Reg, 00656 MemAddress, 00657 Buffer, 00658 Length, 00659 1000); 00660 00661 /* Check the communication status */ 00662 if(status != HAL_OK) 00663 { 00664 /* Re-Initiaize the I2C Bus */ 00665 I2C1_Error(Addr); 00666 } 00667 return status; 00668 } 00669 00670 static HAL_StatusTypeDef I2C2_WriteMultiple(uint8_t Addr, 00671 uint16_t Reg, 00672 uint16_t MemAddress, 00673 uint8_t *Buffer, 00674 uint16_t Length) 00675 { 00676 HAL_StatusTypeDef status = HAL_OK; 00677 00678 status = HAL_I2C_Mem_Write(&heval_I2c2, 00679 Addr, 00680 (uint16_t)Reg, 00681 MemAddress, 00682 Buffer, 00683 Length, 00684 1000); 00685 00686 /* Check the communication status */ 00687 if(status != HAL_OK) 00688 { 00689 /* Re-Initiaize the I2C2 Bus */ 00690 I2C2_Error(Addr); 00691 } 00692 return status; 00693 } 00694 00695 /** 00696 * @brief Checks if target device is ready for communication. 00697 * @note This function is used with Memory devices 00698 * @param DevAddress: Target device address 00699 * @param Trials: Number of trials 00700 * @retval HAL status 00701 */ 00702 static HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00703 { 00704 return (HAL_I2C_IsDeviceReady(&heval_I2c1, DevAddress, Trials, 1000)); 00705 } 00706 00707 /** 00708 * @brief Manages error callback by re-initializing I2C. 00709 * @param Addr: I2C Address 00710 */ 00711 static void I2C1_Error(uint8_t Addr) 00712 { 00713 /* De-initialize the I2C comunication bus */ 00714 HAL_I2C_DeInit(&heval_I2c1); 00715 00716 /* Re-Initiaize the I2C comunication bus */ 00717 I2C1_Init(); 00718 } 00719 00720 static void I2C2_Error(uint8_t Addr) 00721 { 00722 /* De-initialize the I2C2 comunication bus */ 00723 HAL_I2C_DeInit(&heval_I2c2); 00724 00725 /* Re-Initiaize the I2C2 comunication bus */ 00726 I2C2_Init(); 00727 } 00728 00729 /** 00730 * @} 00731 */ 00732 00733 /******************************************************************************* 00734 LINK OPERATIONS 00735 *******************************************************************************/ 00736 00737 /********************************* LINK AUDIO *********************************/ 00738 00739 /** 00740 * @brief Initializes Audio low level. 00741 */ 00742 void AUDIO_IO_Init(void) 00743 { 00744 I2C2_Init(); 00745 } 00746 00747 /** 00748 * @brief DeInitializes Audio low level. 00749 */ 00750 void AUDIO_IO_DeInit(void) 00751 { 00752 00753 } 00754 00755 /** 00756 * @brief Writes a single data. 00757 * @param Addr: I2C address 00758 * @param Reg: Reg address 00759 * @param Value: Data to be written 00760 */ 00761 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00762 { 00763 I2C2_WriteMultiple(Addr, (uint16_t) Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&Value, 1); 00764 } 00765 00766 /** 00767 * @brief Reads a single data. 00768 * @param Addr: I2C address 00769 * @param Reg: Reg address 00770 * @retval Data to be read 00771 */ 00772 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg) 00773 { 00774 uint8_t read_value = 0; 00775 00776 I2C2_ReadMultiple(Addr, (uint16_t) Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00777 00778 return read_value; 00779 } 00780 00781 /** 00782 * @brief AUDIO Codec delay 00783 * @param Delay: Delay in ms 00784 */ 00785 void AUDIO_IO_Delay(uint32_t Delay) 00786 { 00787 HAL_Delay(Delay); 00788 } 00789 00790 /******************************** LINK I2C EEPROM *****************************/ 00791 00792 /** 00793 * @brief Initializes peripherals used by the I2C EEPROM driver. 00794 */ 00795 void EEPROM_IO_Init(void) 00796 { 00797 I2C1_Init(); 00798 } 00799 00800 /** 00801 * @brief Write data to I2C EEPROM driver in using DMA channel. 00802 * @param DevAddress: Target device address 00803 * @param MemAddress: Internal memory address 00804 * @param pBuffer: Pointer to data buffer 00805 * @param BufferSize: Amount of data to be sent 00806 * @retval HAL status 00807 */ 00808 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00809 { 00810 return (I2C1_WriteMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00811 } 00812 00813 /** 00814 * @brief Read data from I2C EEPROM driver in using DMA channel. 00815 * @param DevAddress: Target device address 00816 * @param MemAddress: Internal memory address 00817 * @param pBuffer: Pointer to data buffer 00818 * @param BufferSize: Amount of data to be read 00819 * @retval HAL status 00820 */ 00821 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00822 { 00823 return (I2C1_ReadMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00824 } 00825 00826 /** 00827 * @brief Checks if target device is ready for communication. 00828 * @note This function is used with Memory devices 00829 * @param DevAddress: Target device address 00830 * @param Trials: Number of trials 00831 * @retval HAL status 00832 */ 00833 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00834 { 00835 return (I2C1_IsDeviceReady(DevAddress, Trials)); 00836 } 00837 00838 /******************************** LINK TS (TouchScreen) ***********************/ 00839 00840 /** 00841 * @brief Initialize I2C communication 00842 * channel from MCU to TouchScreen (TS). 00843 */ 00844 void TS_IO_Init(void) 00845 { 00846 I2C1_Init(); 00847 } 00848 00849 /** 00850 * @brief Writes single data with I2C communication 00851 * channel from MCU to TouchScreen. 00852 * @param Addr: I2C address 00853 * @param Reg: Register address 00854 * @param Value: Data to be written 00855 */ 00856 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00857 { 00858 I2C1_Write(Addr, Reg, Value); 00859 } 00860 00861 /** 00862 * @brief Reads single data with I2C communication 00863 * channel from TouchScreen. 00864 * @param Addr: I2C address 00865 * @param Reg: Register address 00866 * @retval Read data 00867 */ 00868 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 00869 { 00870 return I2C1_Read(Addr, Reg); 00871 } 00872 00873 /** 00874 * @brief Reads multiple data with I2C communication 00875 * channel from TouchScreen. 00876 * @param Addr: I2C address 00877 * @param Reg: Register address 00878 * @param Buffer: Pointer to data buffer 00879 * @param Length: Length of the data 00880 * @retval Number of read data 00881 */ 00882 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00883 { 00884 return I2C1_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00885 } 00886 00887 /** 00888 * @brief Writes multiple data with I2C communication 00889 * channel from MCU to TouchScreen. 00890 * @param Addr: I2C address 00891 * @param Reg: Register address 00892 * @param Buffer: Pointer to data buffer 00893 * @param Length: Length of the data 00894 */ 00895 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00896 { 00897 I2C1_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00898 } 00899 00900 /** 00901 * @brief Delay function used in TouchScreen low level driver. 00902 * @param Delay: Delay in ms 00903 */ 00904 void TS_IO_Delay(uint32_t Delay) 00905 { 00906 HAL_Delay(Delay); 00907 } 00908 00909 /**************************** LINK OTM8009A (Display driver) ******************/ 00910 /** 00911 * @brief OTM8009A delay 00912 * @param Delay: Delay in ms 00913 */ 00914 void OTM8009A_IO_Delay(uint32_t Delay) 00915 { 00916 HAL_Delay(Delay); 00917 } 00918 00919 /** 00920 * @} 00921 */ 00922 00923 /** 00924 * @} 00925 */ 00926 00927 /** 00928 * @} 00929 */ 00930 00931 /** 00932 * @} 00933 */ 00934 00935 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 10:58:45 for STM32F469I-Discovery BSP User Manual by
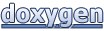