STM32F413H-Discovery BSP User Manual
|
stm32f413h_discovery_psram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f413h_discovery_psram.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 27-January-2017 00007 * @brief This file includes the PSRAM driver for the IS61WV51216BLL-10MLI memory 00008 * device mounted on STM32F413H-DISCOVERY boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the IS61WV51216BLL-10M PSRAM external memory mounted 00013 on STM32F413H-DisCovERYevaluation board. 00014 - This driver does not need a specific component driver for the PSRAM device 00015 to be included with. 00016 00017 Driver description: 00018 ------------------ 00019 + Initialization steps: 00020 o Initialize the PSRAM external memory using the BSP_PSRAM_Init() function. This 00021 function includes the MSP layer hardware resources initialization and the 00022 FSMC controller configuration to interface with the external PSRAM memory. 00023 00024 + PSRAM read/write operations 00025 o PSRAM external memory can be accessed with read/write operations once it is 00026 initialized. 00027 Read/write operation can be performed with AHB access using the functions 00028 BSP_PSRAM_ReadData()/BSP_PSRAM_WriteData(), or by DMA transfer using the functions 00029 BSP_PSRAM_ReadData_DMA()/BSP_PSRAM_WriteData_DMA(). 00030 o The AHB access is performed with 16-bit width transaction, the DMA transfer 00031 configuration is fixed at single (no burst) halfword transfer 00032 (see the PSRAM_MspInit() static function). 00033 o User can implement his own functions for read/write access with his desired 00034 configurations. 00035 o If interrupt mode is used for DMA transfer, the function BSP_PSRAM_DMA_IRQHandler() 00036 is called in IRQ handler file, to serve the generated interrupt once the DMA 00037 transfer is complete. 00038 @endverbatim 00039 ****************************************************************************** 00040 * @attention 00041 * 00042 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00043 * 00044 * Redistribution and use in source and binary forms, with or without modification, 00045 * are permitted provided that the following conditions are met: 00046 * 1. Redistributions of source code must retain the above copyright notice, 00047 * this list of conditions and the following disclaimer. 00048 * 2. Redistributions in binary form must reproduce the above copyright notice, 00049 * this list of conditions and the following disclaimer in the documentation 00050 * and/or other materials provided with the distribution. 00051 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00052 * may be used to endorse or promote products derived from this software 00053 * without specific prior written permission. 00054 * 00055 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00056 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00057 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00058 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00059 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00060 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00061 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00062 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00063 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00064 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00065 * 00066 ****************************************************************************** 00067 */ 00068 00069 /* Includes ------------------------------------------------------------------*/ 00070 #include "stm32f413h_discovery_psram.h" 00071 00072 /** @addtogroup BSP 00073 * @{ 00074 */ 00075 00076 /** @addtogroup STM32F413H_DISCOVERY 00077 * @{ 00078 */ 00079 00080 /** @defgroup STM32F413H_DISCOVERY_PSRAM STM32F413H_DISCOVERY PSRAM 00081 * @{ 00082 */ 00083 00084 /** @defgroup STM32F413H_DISCOVERY_PSRAM_Private_Variables STM32F413H DISCOVERY PSRAM Private Variables 00085 * @{ 00086 */ 00087 SRAM_HandleTypeDef psramHandle; 00088 static FSMC_NORSRAM_TimingTypeDef Timing; 00089 00090 /** 00091 * @} 00092 */ 00093 00094 /** @defgroup STM32F413H_DISCOVERY_PSRAM_Private_Functions STM32F413H DISCOVERY PSRAM Private Functions 00095 * @{ 00096 */ 00097 00098 /** 00099 * @brief Initializes the PSRAM device. 00100 * @retval PSRAM status 00101 */ 00102 uint8_t BSP_PSRAM_Init(void) 00103 { 00104 static uint8_t psram_status = PSRAM_ERROR; 00105 /* SRAM device configuration */ 00106 psramHandle.Instance = FSMC_NORSRAM_DEVICE; 00107 psramHandle.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00108 00109 /* PSRAM device configuration */ 00110 /* Timing configuration derived from system clock (up to 100Mhz)*/ 00111 Timing.AddressSetupTime = 3; 00112 Timing.AddressHoldTime = 1; 00113 Timing.DataSetupTime = 4; 00114 Timing.BusTurnAroundDuration = 1; 00115 Timing.CLKDivision = 2; 00116 Timing.DataLatency = 2; 00117 Timing.AccessMode = FSMC_ACCESS_MODE_A; 00118 00119 psramHandle.Init.NSBank = FSMC_NORSRAM_BANK1; 00120 psramHandle.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00121 psramHandle.Init.MemoryType = FSMC_MEMORY_TYPE_SRAM; 00122 psramHandle.Init.MemoryDataWidth = PSRAM_MEMORY_WIDTH; 00123 psramHandle.Init.BurstAccessMode = PSRAM_BURSTACCESS; 00124 psramHandle.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00125 psramHandle.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00126 psramHandle.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00127 psramHandle.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00128 psramHandle.Init.ExtendedMode = FSMC_EXTENDED_MODE_DISABLE; 00129 psramHandle.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00130 psramHandle.Init.WriteBurst = PSRAM_WRITEBURST; 00131 psramHandle.Init.ContinuousClock = CONTINUOUSCLOCK_FEATURE; 00132 00133 /* PSRAM controller initialization */ 00134 BSP_PSRAM_MspInit(&psramHandle, NULL); /* __weak function can be rewritten by the application */ 00135 if(HAL_SRAM_Init(&psramHandle, &Timing, &Timing) != HAL_OK) 00136 { 00137 psram_status = PSRAM_ERROR; 00138 } 00139 else 00140 { 00141 psram_status = PSRAM_OK; 00142 } 00143 return psram_status; 00144 } 00145 00146 /** 00147 * @brief DeInitializes the PSRAM device. 00148 * @retval PSRAM status 00149 */ 00150 uint8_t BSP_PSRAM_DeInit(void) 00151 { 00152 static uint8_t psram_status = PSRAM_ERROR; 00153 /* PSRAM device de-initialization */ 00154 psramHandle.Instance = FSMC_NORSRAM_DEVICE; 00155 psramHandle.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00156 00157 if(HAL_SRAM_DeInit(&psramHandle) != HAL_OK) 00158 { 00159 psram_status = PSRAM_ERROR; 00160 } 00161 else 00162 { 00163 psram_status = PSRAM_OK; 00164 } 00165 00166 /* PSRAM controller de-initialization */ 00167 BSP_PSRAM_MspDeInit(&psramHandle, NULL); 00168 00169 return psram_status; 00170 } 00171 00172 /** 00173 * @brief Reads an amount of data from the PSRAM device in polling mode. 00174 * @param uwStartAddress: Read start address 00175 * @param pData: Pointer to data to be read 00176 * @param uwDataSize: Size of read data from the memory 00177 * @retval PSRAM status 00178 */ 00179 uint8_t BSP_PSRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00180 { 00181 if(HAL_SRAM_Read_16b(&psramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00182 { 00183 return PSRAM_ERROR; 00184 } 00185 else 00186 { 00187 return PSRAM_OK; 00188 } 00189 } 00190 00191 /** 00192 * @brief Reads an amount of data from the PSRAM device in DMA mode. 00193 * @param uwStartAddress: Read start address 00194 * @param pData: Pointer to data to be read 00195 * @param uwDataSize: Size of read data from the memory 00196 * @retval PSRAM status 00197 */ 00198 uint8_t BSP_PSRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00199 { 00200 if(HAL_SRAM_Read_DMA(&psramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00201 { 00202 return PSRAM_ERROR; 00203 } 00204 else 00205 { 00206 return PSRAM_OK; 00207 } 00208 } 00209 00210 /** 00211 * @brief Writes an amount of data from the PSRAM device in polling mode. 00212 * @param uwStartAddress: Write start address 00213 * @param pData: Pointer to data to be written 00214 * @param uwDataSize: Size of written data from the memory 00215 * @retval PSRAM status 00216 */ 00217 uint8_t BSP_PSRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00218 { 00219 if(HAL_SRAM_Write_16b(&psramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00220 { 00221 return PSRAM_ERROR; 00222 } 00223 else 00224 { 00225 return PSRAM_OK; 00226 } 00227 } 00228 00229 /** 00230 * @brief Writes an amount of data from the PSRAM device in DMA mode. 00231 * @param uwStartAddress: Write start address 00232 * @param pData: Pointer to data to be written 00233 * @param uwDataSize: Size of written data from the memory 00234 * @retval PSRAM status 00235 */ 00236 uint8_t BSP_PSRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00237 { 00238 if(HAL_SRAM_Write_DMA(&psramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00239 { 00240 return PSRAM_ERROR; 00241 } 00242 else 00243 { 00244 return PSRAM_OK; 00245 } 00246 } 00247 00248 /** 00249 * @brief Initializes PSRAM MSP. 00250 * @param hsram: PSRAM handle 00251 * @param Params : pointer on additional configuration parameters, can be NULL. 00252 */ 00253 __weak void BSP_PSRAM_MspInit(SRAM_HandleTypeDef *hsram, void *Params) 00254 { 00255 static DMA_HandleTypeDef dma_handle; 00256 GPIO_InitTypeDef gpio_init_structure; 00257 00258 /* Prevent unused argument(s) compilation warning */ 00259 UNUSED(Params); 00260 00261 /* Enable FSMC clock */ 00262 __HAL_RCC_FSMC_CLK_ENABLE(); 00263 00264 /* Enable chosen DMAx clock */ 00265 PSRAM_DMAx_CLK_ENABLE(); 00266 00267 /* Enable GPIOs clock */ 00268 __HAL_RCC_GPIOD_CLK_ENABLE(); 00269 __HAL_RCC_GPIOE_CLK_ENABLE(); 00270 __HAL_RCC_GPIOF_CLK_ENABLE(); 00271 __HAL_RCC_GPIOG_CLK_ENABLE(); 00272 00273 /* Common GPIO configuration */ 00274 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00275 gpio_init_structure.Pull = GPIO_PULLUP; 00276 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00277 gpio_init_structure.Alternate = GPIO_AF12_FSMC; 00278 00279 /* GPIOD configuration */ 00280 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_7 |\ 00281 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00282 GPIO_PIN_14 | GPIO_PIN_15; 00283 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00284 00285 /* GPIOE configuration */ 00286 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 |\ 00287 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00288 GPIO_PIN_15; 00289 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00290 00291 /* GPIOF configuration */ 00292 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00293 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00294 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00295 00296 /* GPIOG configuration */ 00297 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00298 GPIO_PIN_5; 00299 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00300 00301 /* Configure common DMA parameters */ 00302 dma_handle.Init.Channel = PSRAM_DMAx_CHANNEL; 00303 dma_handle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00304 dma_handle.Init.PeriphInc = DMA_PINC_ENABLE; 00305 dma_handle.Init.MemInc = DMA_MINC_ENABLE; 00306 dma_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00307 dma_handle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00308 dma_handle.Init.Mode = DMA_NORMAL; 00309 dma_handle.Init.Priority = DMA_PRIORITY_HIGH; 00310 dma_handle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00311 dma_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00312 dma_handle.Init.MemBurst = DMA_MBURST_SINGLE; 00313 dma_handle.Init.PeriphBurst = DMA_PBURST_SINGLE; 00314 00315 dma_handle.Instance = PSRAM_DMAx_STREAM; 00316 00317 /* Associate the DMA handle */ 00318 __HAL_LINKDMA(hsram, hdma, dma_handle); 00319 00320 /* Deinitialize the Stream for new transfer */ 00321 HAL_DMA_DeInit(&dma_handle); 00322 00323 /* Configure the DMA Stream */ 00324 HAL_DMA_Init(&dma_handle); 00325 00326 /* NVIC configuration for DMA transfer complete interrupt */ 00327 HAL_NVIC_SetPriority(PSRAM_DMAx_IRQn, 0x0F, 0); 00328 HAL_NVIC_EnableIRQ(PSRAM_DMAx_IRQn); 00329 } 00330 00331 /** 00332 * @brief DeInitializes SRAM MSP. 00333 * @param hsram: SRAM handle 00334 * @param Params : pointer on additional configuration parameters, can be NULL. 00335 * @retval None 00336 */ 00337 __weak void BSP_PSRAM_MspDeInit(SRAM_HandleTypeDef *hsram, void *Params) 00338 { 00339 /* Prevent unused argument(s) compilation warning */ 00340 UNUSED(Params); 00341 00342 /* Disable NVIC configuration for DMA interrupt */ 00343 HAL_NVIC_DisableIRQ(PSRAM_DMAx_IRQn); 00344 00345 if(hsram->Instance != NULL) 00346 { 00347 /* Deinitialize the stream for new transfer */ 00348 HAL_DMA_DeInit(hsram->hdma); 00349 } 00350 00351 /* GPIO pins clock, FSMC clock and DMA clock can be shut down in the applications 00352 by surcharging this __weak function */ 00353 } 00354 /** 00355 * @} 00356 */ 00357 00358 /** 00359 * @} 00360 */ 00361 00362 /** 00363 * @} 00364 */ 00365 00366 /** 00367 * @} 00368 */ 00369 00370 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jan 26 2017 16:30:37 for STM32F413H-Discovery BSP User Manual by
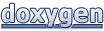