STM32F413H-Discovery BSP User Manual
|
Functions | |
uint8_t | BSP_SD_Init (void) |
Initializes the SD card device. | |
uint8_t | BSP_SD_DeInit (void) |
DeInitializes the SD card device. | |
uint8_t | BSP_SD_ITConfig (void) |
Configures Interrupt mode for SD detection pin. | |
uint8_t | BSP_SD_IsDetected (void) |
Detects if SD card is correctly plugged in the memory slot or not. | |
uint8_t | BSP_SD_ReadBlocks (uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) |
Reads block(s) from a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocks (uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) |
Writes block(s) to a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_ReadBlocks_DMA (uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) |
Reads block(s) from a specified address in an SD card, in DMA mode. | |
uint8_t | BSP_SD_WriteBlocks_DMA (uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) |
Writes block(s) to a specified address in an SD card, in DMA mode. | |
uint8_t | BSP_SD_Erase (uint32_t StartAddr, uint32_t EndAddr) |
Erases the specified memory area of the given SD card. | |
__weak void | BSP_SD_MspInit (SD_HandleTypeDef *hsd, void *Params) |
Initializes the SD MSP. | |
__weak void | BSP_SD_Detect_MspInit (SD_HandleTypeDef *hsd, void *Params) |
Initializes the SD Detect pin MSP. | |
__weak void | BSP_SD_MspDeInit (SD_HandleTypeDef *hsd, void *Params) |
DeInitializes the SD MSP. | |
uint8_t | BSP_SD_GetCardState (void) |
Gets the current SD card data status. | |
void | BSP_SD_GetCardInfo (HAL_SD_CardInfoTypeDef *CardInfo) |
Get SD information about specific SD card. | |
void | HAL_SD_AbortCallback (SD_HandleTypeDef *hsd) |
SD Abort callbacks. | |
void | HAL_SD_TxCpltCallback (SD_HandleTypeDef *hsd) |
Tx Transfer completed callbacks. | |
void | HAL_SD_RxCpltCallback (SD_HandleTypeDef *hsd) |
Rx Transfer completed callbacks. | |
__weak void | BSP_SD_AbortCallback (void) |
BSP SD Abort callbacks. | |
__weak void | BSP_SD_WriteCpltCallback (void) |
BSP Tx Transfer completed callbacks. | |
__weak void | BSP_SD_ReadCpltCallback (void) |
BSP Rx Transfer completed callbacks. |
Function Documentation
__weak void BSP_SD_AbortCallback | ( | void | ) |
BSP SD Abort callbacks.
Definition at line 536 of file stm32f413h_discovery_sd.c.
Referenced by HAL_SD_AbortCallback().
uint8_t BSP_SD_DeInit | ( | void | ) |
DeInitializes the SD card device.
- Return values:
-
SD status
Definition at line 165 of file stm32f413h_discovery_sd.c.
References BSP_SD_MspDeInit(), MSD_ERROR, MSD_OK, and uSdHandle.
__weak void BSP_SD_Detect_MspInit | ( | SD_HandleTypeDef * | hsd, |
void * | Params | ||
) |
Initializes the SD Detect pin MSP.
- Parameters:
-
hsd,: SD handle Params : pointer on additional configuration parameters, can be NULL.
Definition at line 428 of file stm32f413h_discovery_sd.c.
References SD_DETECT_GPIO_CLK_ENABLE, SD_DETECT_GPIO_PORT, and SD_DETECT_PIN.
Referenced by BSP_SD_Init().
uint8_t BSP_SD_Erase | ( | uint32_t | StartAddr, |
uint32_t | EndAddr | ||
) |
Erases the specified memory area of the given SD card.
- Parameters:
-
StartAddr,: Start byte address EndAddr,: End byte address
- Return values:
-
SD status
Definition at line 309 of file stm32f413h_discovery_sd.c.
void BSP_SD_GetCardInfo | ( | HAL_SD_CardInfoTypeDef * | CardInfo | ) |
Get SD information about specific SD card.
- Parameters:
-
CardInfo,: Pointer to HAL_SD_CardInfoTypedef structure
Definition at line 500 of file stm32f413h_discovery_sd.c.
References uSdHandle.
uint8_t BSP_SD_GetCardState | ( | void | ) |
Gets the current SD card data status.
- Return values:
-
Data transfer state. This value can be one of the following values: - SD_TRANSFER_OK: No data transfer is acting
- SD_TRANSFER_BUSY: Data transfer is acting
Definition at line 490 of file stm32f413h_discovery_sd.c.
References SD_TRANSFER_BUSY, SD_TRANSFER_OK, and uSdHandle.
uint8_t BSP_SD_Init | ( | void | ) |
Initializes the SD card device.
- Return values:
-
SD status
Definition at line 112 of file stm32f413h_discovery_sd.c.
References BSP_SD_Detect_MspInit(), BSP_SD_IsDetected(), BSP_SD_MspInit(), MSD_ERROR, MSD_ERROR_SD_NOT_PRESENT, MSD_OK, SD_PRESENT, and uSdHandle.
uint8_t BSP_SD_IsDetected | ( | void | ) |
Detects if SD card is correctly plugged in the memory slot or not.
- Return values:
-
Returns if SD is detected or not
Definition at line 210 of file stm32f413h_discovery_sd.c.
References SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_NOT_PRESENT, and SD_PRESENT.
Referenced by BSP_SD_Init().
uint8_t BSP_SD_ITConfig | ( | void | ) |
Configures Interrupt mode for SD detection pin.
- Return values:
-
Returns MSD_OK
Definition at line 188 of file stm32f413h_discovery_sd.c.
References MSD_OK, SD_DETECT_EXTI_IRQn, SD_DETECT_GPIO_PORT, and SD_DETECT_PIN.
__weak void BSP_SD_MspDeInit | ( | SD_HandleTypeDef * | hsd, |
void * | Params | ||
) |
DeInitializes the SD MSP.
- Parameters:
-
hsd,: SD handle Params : pointer on additional configuration parameters, can be NULL.
Definition at line 450 of file stm32f413h_discovery_sd.c.
References SD_DMAx_Rx_IRQn, SD_DMAx_Rx_STREAM, SD_DMAx_Tx_IRQn, and SD_DMAx_Tx_STREAM.
Referenced by BSP_SD_DeInit().
__weak void BSP_SD_MspInit | ( | SD_HandleTypeDef * | hsd, |
void * | Params | ||
) |
Initializes the SD MSP.
- Parameters:
-
hsd,: SD handle Params : pointer on additional configuration parameters, can be NULL.
Definition at line 326 of file stm32f413h_discovery_sd.c.
References __DMAx_TxRx_CLK_ENABLE, SD_DMAx_Rx_CHANNEL, SD_DMAx_Rx_IRQn, SD_DMAx_Rx_STREAM, SD_DMAx_Tx_CHANNEL, SD_DMAx_Tx_IRQn, and SD_DMAx_Tx_STREAM.
Referenced by BSP_SD_Init().
uint8_t BSP_SD_ReadBlocks | ( | uint32_t * | pData, |
uint32_t | ReadAddr, | ||
uint32_t | NumOfBlocks, | ||
uint32_t | Timeout | ||
) |
Reads block(s) from a specified address in an SD card, in polling mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit ReadAddr,: Address from where data is to be read NumOfBlocks,: Number of SD blocks to read Timeout,: Timeout for read operation
- Return values:
-
SD status
Definition at line 231 of file stm32f413h_discovery_sd.c.
uint8_t BSP_SD_ReadBlocks_DMA | ( | uint32_t * | pData, |
uint32_t | ReadAddr, | ||
uint32_t | NumOfBlocks | ||
) |
Reads block(s) from a specified address in an SD card, in DMA mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit ReadAddr,: Address from where data is to be read NumOfBlocks,: Number of SD blocks to read
- Return values:
-
SD status
Definition at line 270 of file stm32f413h_discovery_sd.c.
__weak void BSP_SD_ReadCpltCallback | ( | void | ) |
BSP Rx Transfer completed callbacks.
Definition at line 552 of file stm32f413h_discovery_sd.c.
Referenced by HAL_SD_RxCpltCallback().
uint8_t BSP_SD_WriteBlocks | ( | uint32_t * | pData, |
uint32_t | WriteAddr, | ||
uint32_t | NumOfBlocks, | ||
uint32_t | Timeout | ||
) |
Writes block(s) to a specified address in an SD card, in polling mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit WriteAddr,: Address from where data is to be written NumOfBlocks,: Number of SD blocks to write Timeout,: Timeout for write operation
- Return values:
-
SD status
Definition at line 251 of file stm32f413h_discovery_sd.c.
uint8_t BSP_SD_WriteBlocks_DMA | ( | uint32_t * | pData, |
uint32_t | WriteAddr, | ||
uint32_t | NumOfBlocks | ||
) |
Writes block(s) to a specified address in an SD card, in DMA mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit WriteAddr,: Address from where data is to be written NumOfBlocks,: Number of SD blocks to write
- Return values:
-
SD status
Definition at line 290 of file stm32f413h_discovery_sd.c.
__weak void BSP_SD_WriteCpltCallback | ( | void | ) |
BSP Tx Transfer completed callbacks.
Definition at line 544 of file stm32f413h_discovery_sd.c.
Referenced by HAL_SD_TxCpltCallback().
void HAL_SD_AbortCallback | ( | SD_HandleTypeDef * | hsd | ) |
SD Abort callbacks.
- Parameters:
-
hsd,: SD handle
Definition at line 510 of file stm32f413h_discovery_sd.c.
References BSP_SD_AbortCallback().
void HAL_SD_RxCpltCallback | ( | SD_HandleTypeDef * | hsd | ) |
Rx Transfer completed callbacks.
- Parameters:
-
hsd,: SD handle
Definition at line 528 of file stm32f413h_discovery_sd.c.
References BSP_SD_ReadCpltCallback().
void HAL_SD_TxCpltCallback | ( | SD_HandleTypeDef * | hsd | ) |
Tx Transfer completed callbacks.
- Parameters:
-
hsd,: SD handle
Definition at line 519 of file stm32f413h_discovery_sd.c.
References BSP_SD_WriteCpltCallback().
Generated on Thu Jan 26 2017 16:30:37 for STM32F413H-Discovery BSP User Manual by
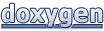