STM32F413H-Discovery BSP User Manual
|
stm32f413h_discovery_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f413h_discovery_lcd.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 27-January-2017 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM32F413H-DISCOVERY board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive indirectly an LCD TFT. 00044 - This driver supports the LS016B8UY LCD. 00045 - The LS016B8UY component driver MUST be included with this driver. 00046 00047 2. Driver description: 00048 --------------------- 00049 + Initialization steps: 00050 o Initialize the LCD using the BSP_LCD_Init() function. 00051 00052 + Display on LCD 00053 o Clear the hole LCD using BSP_LCD_Clear() function or only one specified string 00054 line using the BSP_LCD_ClearStringLine() function. 00055 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00056 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00057 o Display a string line on the specified position (x,y in pixel) and align mode 00058 using the BSP_LCD_DisplayStringAtLine() function. 00059 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00060 on LCD using the available set of functions. 00061 00062 ------------------------------------------------------------------------------*/ 00063 00064 /* Includes ------------------------------------------------------------------*/ 00065 #include "stm32f413h_discovery_lcd.h" 00066 #include "../../../Utilities/Fonts/fonts.h" 00067 #include "../../../Utilities/Fonts/font24.c" 00068 #include "../../../Utilities/Fonts/font20.c" 00069 #include "../../../Utilities/Fonts/font16.c" 00070 #include "../../../Utilities/Fonts/font12.c" 00071 #include "../../../Utilities/Fonts/font8.c" 00072 00073 /** @addtogroup BSP 00074 * @{ 00075 */ 00076 00077 /** @addtogroup STM32F413H_DISCOVERY 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM32F413H_DISCOVERY_LCD STM32F413H_DISCOVERY LCD 00082 * @{ 00083 */ 00084 00085 /** @defgroup STM32F413H_DISCOVERY_LCD_Private_Macros STM32F413H DISCOVERY LCD Private Macros 00086 * @{ 00087 */ 00088 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00089 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00090 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM32F413H_DISCOVERY_LCD_Private_Variables STM32F413H DISCOVERY LCD Private Variables 00096 * @{ 00097 */ 00098 LCD_DrawPropTypeDef DrawProp; 00099 static LCD_DrvTypeDef *LcdDrv; 00100 /** 00101 * @} 00102 */ 00103 00104 /** @defgroup STM32F413H_DISCOVERY_LCD_Private_FunctionPrototypes STM32F413H DISCOVERY LCD Private Functions Prototypes 00105 * @{ 00106 */ 00107 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00108 static void SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00109 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32F413H_DISCOVERY_LCD_Private_Functions STM32F413H DISCOVERY LCD Private Functions 00115 * @{ 00116 */ 00117 /** 00118 * @brief Initializes the LCD. 00119 * @retval LCD state 00120 */ 00121 uint8_t BSP_LCD_Init(void) 00122 { 00123 return (BSP_LCD_InitEx(LCD_ORIENTATION_LANDSCAPE)); 00124 } 00125 /** 00126 * @brief Initializes the LCD with a given orientation. 00127 * @param orientation: LCD_ORIENTATION_PORTRAIT or LCD_ORIENTATION_LANDSCAPE 00128 * or LCD_ORIENTATION_LANDSCAPE_ROT180 00129 * @retval LCD state 00130 */ 00131 uint8_t BSP_LCD_InitEx(uint32_t orientation) 00132 { 00133 uint8_t ret = LCD_ERROR; 00134 00135 /* Default value for draw propriety */ 00136 DrawProp.BackColor = 0xFFFF; 00137 DrawProp.pFont = &Font24; 00138 DrawProp.TextColor = 0x0000; 00139 00140 /* Initialize LCD special pins GPIOs */ 00141 BSP_LCD_MspInit(); 00142 00143 /* Backlight control signal assertion */ 00144 HAL_GPIO_WritePin(LCD_BL_CTRL_GPIO_PORT, LCD_BL_CTRL_PIN, GPIO_PIN_SET); 00145 00146 /* Apply hardware reset according to procedure indicated in FRD154BP2901 documentation */ 00147 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_RESET); 00148 HAL_Delay(5); /* Reset signal asserted during 5ms */ 00149 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_SET); 00150 HAL_Delay(10); /* Reset signal released during 10ms */ 00151 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_RESET); 00152 HAL_Delay(20); /* Reset signal asserted during 20ms */ 00153 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_SET); 00154 HAL_Delay(10); /* Reset signal released during 10ms */ 00155 00156 if(ST7789H2_drv.ReadID() == ST7789H2_ID) 00157 { 00158 LcdDrv = &ST7789H2_drv; 00159 00160 /* LCD Init */ 00161 LcdDrv->Init(); 00162 00163 if(orientation == LCD_ORIENTATION_PORTRAIT) 00164 { 00165 ST7789H2_SetOrientation(ST7789H2_ORIENTATION_PORTRAIT); 00166 } 00167 else if(orientation == LCD_ORIENTATION_LANDSCAPE_ROT180) 00168 { 00169 ST7789H2_SetOrientation(ST7789H2_ORIENTATION_LANDSCAPE_ROT180); 00170 } 00171 else 00172 { 00173 /* Default landscape orientation is selected */ 00174 } 00175 /* Initialize the font */ 00176 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00177 00178 ret = LCD_OK; 00179 } 00180 00181 return ret; 00182 } 00183 00184 /** 00185 * @brief DeInitializes the LCD. 00186 * @retval LCD state 00187 */ 00188 uint8_t BSP_LCD_DeInit(void) 00189 { 00190 /* Actually LcdDrv does not provide a DeInit function */ 00191 return LCD_OK; 00192 } 00193 00194 /** 00195 * @brief Gets the LCD X size. 00196 * @retval Used LCD X size 00197 */ 00198 uint32_t BSP_LCD_GetXSize(void) 00199 { 00200 return(LcdDrv->GetLcdPixelWidth()); 00201 } 00202 00203 /** 00204 * @brief Gets the LCD Y size. 00205 * @retval Used LCD Y size 00206 */ 00207 uint32_t BSP_LCD_GetYSize(void) 00208 { 00209 return(LcdDrv->GetLcdPixelHeight()); 00210 } 00211 00212 /** 00213 * @brief Gets the LCD text color. 00214 * @retval Used text color. 00215 */ 00216 uint16_t BSP_LCD_GetTextColor(void) 00217 { 00218 return DrawProp.TextColor; 00219 } 00220 00221 /** 00222 * @brief Gets the LCD background color. 00223 * @retval Used background color 00224 */ 00225 uint16_t BSP_LCD_GetBackColor(void) 00226 { 00227 return DrawProp.BackColor; 00228 } 00229 00230 /** 00231 * @brief Sets the LCD text color. 00232 * @param Color: Text color code 00233 */ 00234 void BSP_LCD_SetTextColor(uint16_t Color) 00235 { 00236 DrawProp.TextColor = Color; 00237 } 00238 00239 /** 00240 * @brief Sets the LCD background color. 00241 * @param Color: Background color code 00242 */ 00243 void BSP_LCD_SetBackColor(uint16_t Color) 00244 { 00245 DrawProp.BackColor = Color; 00246 } 00247 00248 /** 00249 * @brief Sets the LCD text font. 00250 * @param fonts: Font to be used 00251 */ 00252 void BSP_LCD_SetFont(sFONT *fonts) 00253 { 00254 DrawProp.pFont = fonts; 00255 } 00256 00257 /** 00258 * @brief Gets the LCD text font. 00259 * @retval Used font 00260 */ 00261 sFONT *BSP_LCD_GetFont(void) 00262 { 00263 return DrawProp.pFont; 00264 } 00265 00266 /** 00267 * @brief Clears the hole LCD. 00268 * @param Color: Color of the background 00269 */ 00270 void BSP_LCD_Clear(uint16_t Color) 00271 { 00272 uint32_t counter = 0; 00273 uint32_t y_size = 0; 00274 uint32_t color_backup = DrawProp.TextColor; 00275 00276 DrawProp.TextColor = Color; 00277 y_size = BSP_LCD_GetYSize(); 00278 00279 for(counter = 0; counter < y_size; counter++) 00280 { 00281 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00282 } 00283 DrawProp.TextColor = color_backup; 00284 BSP_LCD_SetTextColor(DrawProp.TextColor); 00285 } 00286 00287 /** 00288 * @brief Clears the selected line. 00289 * @param Line: Line to be cleared 00290 * This parameter can be one of the following values: 00291 * @arg 0..9: if the Current fonts is Font16x24 00292 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00293 * @arg 0..29: if the Current fonts is Font8x8 00294 */ 00295 void BSP_LCD_ClearStringLine(uint16_t Line) 00296 { 00297 uint32_t color_backup = DrawProp.TextColor; 00298 00299 DrawProp.TextColor = DrawProp.BackColor;; 00300 00301 /* Draw a rectangle with background color */ 00302 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00303 00304 DrawProp.TextColor = color_backup; 00305 BSP_LCD_SetTextColor(DrawProp.TextColor); 00306 } 00307 00308 /** 00309 * @brief Displays one character. 00310 * @param Xpos: Start column address 00311 * @param Ypos: Line where to display the character shape. 00312 * @param Ascii: Character ascii code 00313 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00314 */ 00315 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00316 { 00317 DrawChar(Xpos, Ypos, &DrawProp.pFont->table[(Ascii-' ') *\ 00318 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00319 } 00320 00321 /** 00322 * @brief Displays characters on the LCD. 00323 * @param Xpos: X position (in pixel) 00324 * @param Ypos: Y position (in pixel) 00325 * @param Text: Pointer to string to display on LCD 00326 * @param Mode: Display mode 00327 * This parameter can be one of the following values: 00328 * @arg CENTER_MODE 00329 * @arg RIGHT_MODE 00330 * @arg LEFT_MODE 00331 */ 00332 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Line_ModeTypdef Mode) 00333 { 00334 uint16_t refcolumn = 1, i = 0; 00335 uint32_t size = 0, xsize = 0; 00336 uint8_t *ptr = Text; 00337 00338 /* Get the text size */ 00339 while (*ptr++) size ++ ; 00340 00341 /* Characters number per line */ 00342 xsize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00343 00344 switch (Mode) 00345 { 00346 case CENTER_MODE: 00347 { 00348 refcolumn = Xpos + ((xsize - size)* DrawProp.pFont->Width) / 2; 00349 break; 00350 } 00351 case LEFT_MODE: 00352 { 00353 refcolumn = Xpos; 00354 break; 00355 } 00356 case RIGHT_MODE: 00357 { 00358 refcolumn = - Xpos + ((xsize - size)*DrawProp.pFont->Width); 00359 break; 00360 } 00361 default: 00362 { 00363 refcolumn = Xpos; 00364 break; 00365 } 00366 } 00367 00368 /* Check that the Start column is located in the screen */ 00369 if ((refcolumn < 1) || (refcolumn >= 0x8000)) 00370 { 00371 refcolumn = 1; 00372 } 00373 00374 /* Send the string character by character on lCD */ 00375 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00376 { 00377 /* Display one character on LCD */ 00378 BSP_LCD_DisplayChar(refcolumn, Ypos, *Text); 00379 /* Decrement the column position by 16 */ 00380 refcolumn += DrawProp.pFont->Width; 00381 /* Point on the next character */ 00382 Text++; 00383 i++; 00384 } 00385 } 00386 00387 /** 00388 * @brief Displays a character on the LCD. 00389 * @param Line: Line where to display the character shape 00390 * This parameter can be one of the following values: 00391 * @arg 0..9: if the Current fonts is Font16x24 00392 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00393 * @arg 0..29: if the Current fonts is Font8x8 00394 * @param ptr: Pointer to string to display on LCD 00395 */ 00396 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00397 { 00398 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00399 } 00400 00401 /** 00402 * @brief Reads an LCD pixel. 00403 * @param Xpos: X position 00404 * @param Ypos: Y position 00405 * @retval RGB pixel color 00406 */ 00407 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00408 { 00409 uint16_t ret = 0; 00410 00411 if(LcdDrv->ReadPixel != NULL) 00412 { 00413 ret = LcdDrv->ReadPixel(Xpos, Ypos); 00414 } 00415 00416 return ret; 00417 } 00418 00419 /** 00420 * @brief Draws a pixel on LCD. 00421 * @param Xpos: X position 00422 * @param Ypos: Y position 00423 * @param RGB_Code: Pixel color in RGB mode (5-6-5) 00424 */ 00425 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGB_Code) 00426 { 00427 if(LcdDrv->WritePixel != NULL) 00428 { 00429 LcdDrv->WritePixel(Xpos, Ypos, RGB_Code); 00430 } 00431 } 00432 00433 /** 00434 * @brief Draws an horizontal line. 00435 * @param Xpos: X position 00436 * @param Ypos: Y position 00437 * @param Length: Line length 00438 */ 00439 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00440 { 00441 uint32_t index = 0; 00442 00443 if(LcdDrv->DrawHLine != NULL) 00444 { 00445 LcdDrv->DrawHLine(DrawProp.TextColor, Xpos, Ypos, Length); 00446 } 00447 else 00448 { 00449 for(index = 0; index < Length; index++) 00450 { 00451 BSP_LCD_DrawPixel((Xpos + index), Ypos, DrawProp.TextColor); 00452 } 00453 } 00454 } 00455 00456 /** 00457 * @brief Draws a vertical line. 00458 * @param Xpos: X position 00459 * @param Ypos: Y position 00460 * @param Length: Line length 00461 */ 00462 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00463 { 00464 uint32_t index = 0; 00465 00466 if(LcdDrv->DrawVLine != NULL) 00467 { 00468 LcdDrv->DrawVLine(DrawProp.TextColor, Xpos, Ypos, Length); 00469 } 00470 else 00471 { 00472 for(index = 0; index < Length; index++) 00473 { 00474 BSP_LCD_DrawPixel(Xpos, Ypos + index, DrawProp.TextColor); 00475 } 00476 } 00477 } 00478 00479 /** 00480 * @brief Draws an uni-line (between two points). 00481 * @param x1: Point 1 X position 00482 * @param y1: Point 1 Y position 00483 * @param x2: Point 2 X position 00484 * @param y2: Point 2 Y position 00485 */ 00486 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00487 { 00488 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00489 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00490 curpixel = 0; 00491 00492 deltax = ABS(x2 - x1); /* The difference between the x's */ 00493 deltay = ABS(y2 - y1); /* The difference between the y's */ 00494 x = x1; /* Start x off at the first pixel */ 00495 y = y1; /* Start y off at the first pixel */ 00496 00497 if (x2 >= x1) /* The x-values are increasing */ 00498 { 00499 xinc1 = 1; 00500 xinc2 = 1; 00501 } 00502 else /* The x-values are decreasing */ 00503 { 00504 xinc1 = -1; 00505 xinc2 = -1; 00506 } 00507 00508 if (y2 >= y1) /* The y-values are increasing */ 00509 { 00510 yinc1 = 1; 00511 yinc2 = 1; 00512 } 00513 else /* The y-values are decreasing */ 00514 { 00515 yinc1 = -1; 00516 yinc2 = -1; 00517 } 00518 00519 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00520 { 00521 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00522 yinc2 = 0; /* Don't change the y for every iteration */ 00523 den = deltax; 00524 num = deltax / 2; 00525 numadd = deltay; 00526 numpixels = deltax; /* There are more x-values than y-values */ 00527 } 00528 else /* There is at least one y-value for every x-value */ 00529 { 00530 xinc2 = 0; /* Don't change the x for every iteration */ 00531 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00532 den = deltay; 00533 num = deltay / 2; 00534 numadd = deltax; 00535 numpixels = deltay; /* There are more y-values than x-values */ 00536 } 00537 00538 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00539 { 00540 BSP_LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00541 num += numadd; /* Increase the numerator by the top of the fraction */ 00542 if (num >= den) /* Check if numerator >= denominator */ 00543 { 00544 num -= den; /* Calculate the new numerator value */ 00545 x += xinc1; /* Change the x as appropriate */ 00546 y += yinc1; /* Change the y as appropriate */ 00547 } 00548 x += xinc2; /* Change the x as appropriate */ 00549 y += yinc2; /* Change the y as appropriate */ 00550 } 00551 } 00552 00553 /** 00554 * @brief Draws a rectangle. 00555 * @param Xpos: X position 00556 * @param Ypos: Y position 00557 * @param Width: Rectangle width 00558 * @param Height: Rectangle height 00559 */ 00560 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00561 { 00562 /* Draw horizontal lines */ 00563 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00564 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00565 00566 /* Draw vertical lines */ 00567 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00568 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00569 } 00570 00571 /** 00572 * @brief Draws a circle. 00573 * @param Xpos: X position 00574 * @param Ypos: Y position 00575 * @param Radius: Circle radius 00576 */ 00577 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00578 { 00579 int32_t decision; /* Decision Variable */ 00580 uint32_t current_x; /* Current X Value */ 00581 uint32_t current_y; /* Current Y Value */ 00582 00583 decision = 3 - (Radius << 1); 00584 current_x = 0; 00585 current_y = Radius; 00586 00587 while (current_x <= current_y) 00588 { 00589 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos - current_y), DrawProp.TextColor); 00590 00591 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos - current_y), DrawProp.TextColor); 00592 00593 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos - current_x), DrawProp.TextColor); 00594 00595 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos - current_x), DrawProp.TextColor); 00596 00597 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos + current_y), DrawProp.TextColor); 00598 00599 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos + current_y), DrawProp.TextColor); 00600 00601 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos + current_x), DrawProp.TextColor); 00602 00603 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos + current_x), DrawProp.TextColor); 00604 00605 /* Initialize the font */ 00606 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00607 00608 if (decision < 0) 00609 { 00610 decision += (current_x << 2) + 6; 00611 } 00612 else 00613 { 00614 decision += ((current_x - current_y) << 2) + 10; 00615 current_y--; 00616 } 00617 current_x++; 00618 } 00619 } 00620 00621 /** 00622 * @brief Draws an poly-line (between many points). 00623 * @param Points: Pointer to the points array 00624 * @param PointCount: Number of points 00625 */ 00626 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00627 { 00628 int16_t x = 0, y = 0; 00629 00630 if(PointCount < 2) 00631 { 00632 return; 00633 } 00634 00635 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00636 00637 while(--PointCount) 00638 { 00639 x = Points->X; 00640 y = Points->Y; 00641 Points++; 00642 BSP_LCD_DrawLine(x, y, Points->X, Points->Y); 00643 } 00644 } 00645 00646 /** 00647 * @brief Draws an ellipse on LCD. 00648 * @param Xpos: X position 00649 * @param Ypos: Y position 00650 * @param XRadius: Ellipse X radius 00651 * @param YRadius: Ellipse Y radius 00652 */ 00653 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00654 { 00655 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00656 float k = 0, rad1 = 0, rad2 = 0; 00657 00658 rad1 = XRadius; 00659 rad2 = YRadius; 00660 00661 k = (float)(rad2/rad1); 00662 00663 do { 00664 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp.TextColor); 00665 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp.TextColor); 00666 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp.TextColor); 00667 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp.TextColor); 00668 00669 e2 = err; 00670 if (e2 <= x) { 00671 err += ++x*2+1; 00672 if (-y == x && e2 <= y) e2 = 0; 00673 } 00674 if (e2 > y) err += ++y*2+1; 00675 } 00676 while (y <= 0); 00677 } 00678 00679 /** 00680 * @brief Draws a bitmap picture (16 bpp). 00681 * @param Xpos: Bmp X position in the LCD 00682 * @param Ypos: Bmp Y position in the LCD 00683 * @param pbmp: Pointer to Bmp picture address. 00684 */ 00685 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp) 00686 { 00687 uint32_t height = 0; 00688 uint32_t width = 0; 00689 00690 /* Read bitmap width */ 00691 width = *(uint16_t *) (pbmp + 18); 00692 width |= (*(uint16_t *) (pbmp + 20)) << 16; 00693 00694 /* Read bitmap height */ 00695 height = *(uint16_t *) (pbmp + 22); 00696 height |= (*(uint16_t *) (pbmp + 24)) << 16; 00697 00698 SetDisplayWindow(Xpos, Ypos, width, height); 00699 00700 if(LcdDrv->DrawBitmap != NULL) 00701 { 00702 LcdDrv->DrawBitmap(Xpos, Ypos, pbmp); 00703 } 00704 SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00705 } 00706 00707 /** 00708 * @brief Draws RGB Image (16 bpp). 00709 * @param Xpos: X position in the LCD 00710 * @param Ypos: Y position in the LCD 00711 * @param Xsize: X size in the LCD 00712 * @param Ysize: Y size in the LCD 00713 * @param pdata: Pointer to the RGB Image address. 00714 */ 00715 void BSP_LCD_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pdata) 00716 { 00717 00718 SetDisplayWindow(Xpos, Ypos, Xsize, Ysize); 00719 00720 if(LcdDrv->DrawRGBImage != NULL) 00721 { 00722 LcdDrv->DrawRGBImage(Xpos, Ypos, Xsize, Ysize, pdata); 00723 } 00724 SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00725 } 00726 00727 /** 00728 * @brief Draws a full rectangle. 00729 * @param Xpos: X position 00730 * @param Ypos: Y position 00731 * @param Width: Rectangle width 00732 * @param Height: Rectangle height 00733 */ 00734 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00735 { 00736 BSP_LCD_SetTextColor(DrawProp.TextColor); 00737 do 00738 { 00739 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00740 } 00741 while(Height--); 00742 } 00743 00744 /** 00745 * @brief Draws a full circle. 00746 * @param Xpos: X position 00747 * @param Ypos: Y position 00748 * @param Radius: Circle radius 00749 */ 00750 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00751 { 00752 int32_t decision; /* Decision Variable */ 00753 uint32_t current_x; /* Current X Value */ 00754 uint32_t current_y; /* Current Y Value */ 00755 00756 decision = 3 - (Radius << 1); 00757 00758 current_x = 0; 00759 current_y = Radius; 00760 00761 BSP_LCD_SetTextColor(DrawProp.TextColor); 00762 00763 while (current_x <= current_y) 00764 { 00765 if(current_y > 0) 00766 { 00767 BSP_LCD_DrawHLine(Xpos - current_y, Ypos + current_x, 2*current_y); 00768 BSP_LCD_DrawHLine(Xpos - current_y, Ypos - current_x, 2*current_y); 00769 } 00770 00771 if(current_x > 0) 00772 { 00773 BSP_LCD_DrawHLine(Xpos - current_x, Ypos - current_y, 2*current_x); 00774 BSP_LCD_DrawHLine(Xpos - current_x, Ypos + current_y, 2*current_x); 00775 } 00776 if (decision < 0) 00777 { 00778 decision += (current_x << 2) + 6; 00779 } 00780 else 00781 { 00782 decision += ((current_x - current_y) << 2) + 10; 00783 current_y--; 00784 } 00785 current_x++; 00786 } 00787 00788 BSP_LCD_SetTextColor(DrawProp.TextColor); 00789 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00790 } 00791 00792 /** 00793 * @brief Draws a full poly-line (between many points). 00794 * @param Points: Pointer to the points array 00795 * @param PointCount: Number of points 00796 */ 00797 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 00798 { 00799 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 00800 uint16_t IMAGE_LEFT = 0, IMAGE_RIGHT = 0, IMAGE_TOP = 0, IMAGE_BOTTOM = 0; 00801 00802 IMAGE_LEFT = IMAGE_RIGHT = Points->X; 00803 IMAGE_TOP= IMAGE_BOTTOM = Points->Y; 00804 00805 for(counter = 1; counter < PointCount; counter++) 00806 { 00807 pixelX = POLY_X(counter); 00808 if(pixelX < IMAGE_LEFT) 00809 { 00810 IMAGE_LEFT = pixelX; 00811 } 00812 if(pixelX > IMAGE_RIGHT) 00813 { 00814 IMAGE_RIGHT = pixelX; 00815 } 00816 00817 pixelY = POLY_Y(counter); 00818 if(pixelY < IMAGE_TOP) 00819 { 00820 IMAGE_TOP = pixelY; 00821 } 00822 if(pixelY > IMAGE_BOTTOM) 00823 { 00824 IMAGE_BOTTOM = pixelY; 00825 } 00826 } 00827 00828 if(PointCount < 2) 00829 { 00830 return; 00831 } 00832 00833 X_center = (IMAGE_LEFT + IMAGE_RIGHT)/2; 00834 Y_center = (IMAGE_BOTTOM + IMAGE_TOP)/2; 00835 00836 X_first = Points->X; 00837 Y_first = Points->Y; 00838 00839 while(--PointCount) 00840 { 00841 X = Points->X; 00842 Y = Points->Y; 00843 Points++; 00844 X2 = Points->X; 00845 Y2 = Points->Y; 00846 00847 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 00848 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 00849 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 00850 } 00851 00852 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 00853 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 00854 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 00855 } 00856 00857 /** 00858 * @brief Draws a full ellipse. 00859 * @param Xpos: X position 00860 * @param Ypos: Y position 00861 * @param XRadius: Ellipse X radius 00862 * @param YRadius: Ellipse Y radius 00863 */ 00864 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00865 { 00866 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00867 float k = 0, rad1 = 0, rad2 = 0; 00868 00869 rad1 = XRadius; 00870 rad2 = YRadius; 00871 00872 k = (float)(rad2/rad1); 00873 00874 do 00875 { 00876 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos+y), (2*(uint16_t)(x/k) + 1)); 00877 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos-y), (2*(uint16_t)(x/k) + 1)); 00878 00879 e2 = err; 00880 if (e2 <= x) 00881 { 00882 err += ++x*2+1; 00883 if (-y == x && e2 <= y) e2 = 0; 00884 } 00885 if (e2 > y) err += ++y*2+1; 00886 } 00887 while (y <= 0); 00888 } 00889 00890 /** 00891 * @brief Enables the display. 00892 */ 00893 void BSP_LCD_DisplayOn(void) 00894 { 00895 LcdDrv->DisplayOn(); 00896 } 00897 00898 /** 00899 * @brief Disables the display. 00900 */ 00901 void BSP_LCD_DisplayOff(void) 00902 { 00903 LcdDrv->DisplayOff(); 00904 } 00905 00906 /** 00907 * @brief Initializes the LCD GPIO special pins MSP. 00908 */ 00909 __weak void BSP_LCD_MspInit(void) 00910 { 00911 GPIO_InitTypeDef gpio_init_structure; 00912 00913 /* Enable GPIOs clock */ 00914 LCD_RESET_GPIO_CLK_ENABLE(); 00915 LCD_TE_GPIO_CLK_ENABLE(); 00916 LCD_BL_CTRL_GPIO_CLK_ENABLE(); 00917 00918 /* LCD_RESET GPIO configuration */ 00919 gpio_init_structure.Pin = LCD_RESET_PIN; /* LCD_RESET pin has to be manually controlled */ 00920 gpio_init_structure.Pull = GPIO_NOPULL; 00921 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00922 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00923 HAL_GPIO_Init(LCD_RESET_GPIO_PORT, &gpio_init_structure); 00924 00925 /* LCD_TE GPIO configuration */ 00926 gpio_init_structure.Pin = LCD_TE_PIN; /* LCD_TE pin has to be manually managed */ 00927 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00928 HAL_GPIO_Init(LCD_TE_GPIO_PORT, &gpio_init_structure); 00929 00930 /* LCD_BL_CTRL GPIO configuration */ 00931 gpio_init_structure.Pin = LCD_BL_CTRL_PIN; /* LCD_BL_CTRL pin has to be manually controlled */ 00932 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00933 HAL_GPIO_Init(LCD_BL_CTRL_GPIO_PORT, &gpio_init_structure); 00934 } 00935 00936 /** 00937 * @brief DeInitializes LCD GPIO special pins MSP. 00938 */ 00939 __weak void BSP_LCD_MspDeInit(void) 00940 { 00941 GPIO_InitTypeDef gpio_init_structure; 00942 00943 /* LCD_RESET GPIO deactivation */ 00944 gpio_init_structure.Pin = LCD_RESET_PIN; 00945 HAL_GPIO_DeInit(LCD_RESET_GPIO_PORT, gpio_init_structure.Pin); 00946 00947 /* LCD_TE GPIO deactivation */ 00948 gpio_init_structure.Pin = LCD_TE_PIN; 00949 HAL_GPIO_DeInit(LCD_TE_GPIO_PORT, gpio_init_structure.Pin); 00950 00951 /* LCD_BL_CTRL GPIO deactivation */ 00952 gpio_init_structure.Pin = LCD_BL_CTRL_PIN; 00953 HAL_GPIO_DeInit(LCD_BL_CTRL_GPIO_PORT, gpio_init_structure.Pin); 00954 00955 /* GPIO pins clock can be shut down in the application 00956 by surcharging this __weak function */ 00957 } 00958 00959 /** 00960 * @} 00961 */ 00962 /****************************************************************************** 00963 Static Functions 00964 *******************************************************************************/ 00965 /** @addtogroup STM32F413H_DISCOVERY_LCD_Private_FunctionPrototypes 00966 * @{ 00967 */ 00968 00969 /** 00970 * @brief Draws a character on LCD. 00971 * @param Xpos: Line where to display the character shape 00972 * @param Ypos: Start column address 00973 * @param c: Pointer to the character data 00974 */ 00975 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 00976 { 00977 uint32_t i = 0, j = 0; 00978 uint16_t height, width; 00979 uint8_t offset; 00980 uint8_t *pchar; 00981 uint32_t line; 00982 00983 height = DrawProp.pFont->Height; 00984 width = DrawProp.pFont->Width; 00985 00986 offset = 8 *((width + 7)/8) - width ; 00987 00988 for(i = 0; i < height; i++) 00989 { 00990 pchar = ((uint8_t *)c + (width + 7)/8 * i); 00991 00992 switch(((width + 7)/8)) 00993 { 00994 case 1: 00995 line = pchar[0]; 00996 break; 00997 00998 case 2: 00999 line = (pchar[0]<< 8) | pchar[1]; 01000 break; 01001 01002 case 3: 01003 default: 01004 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01005 break; 01006 } 01007 01008 for (j = 0; j < width; j++) 01009 { 01010 if(line & (1 << (width- j + offset- 1))) 01011 { 01012 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp.TextColor); 01013 } 01014 else 01015 { 01016 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp.BackColor); 01017 } 01018 } 01019 Ypos++; 01020 } 01021 } 01022 01023 /** 01024 * @brief Sets display window. 01025 * @param Xpos: LCD X position 01026 * @param Ypos: LCD Y position 01027 * @param Width: LCD window width 01028 * @param Height: LCD window height 01029 */ 01030 static void SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01031 { 01032 if(LcdDrv->SetDisplayWindow != NULL) 01033 { 01034 LcdDrv->SetDisplayWindow(Xpos, Ypos, Width, Height); 01035 } 01036 } 01037 01038 /** 01039 * @brief Fills a triangle (between 3 points). 01040 * @param x1: Point 1 X position 01041 * @param y1: Point 1 Y position 01042 * @param x2: Point 2 X position 01043 * @param y2: Point 2 Y position 01044 * @param x3: Point 3 X position 01045 * @param y3: Point 3 Y position 01046 */ 01047 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01048 { 01049 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01050 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 01051 curpixel = 0; 01052 01053 deltax = ABS(x2 - x1); /* The difference between the x's */ 01054 deltay = ABS(y2 - y1); /* The difference between the y's */ 01055 x = x1; /* Start x off at the first pixel */ 01056 y = y1; /* Start y off at the first pixel */ 01057 01058 if (x2 >= x1) /* The x-values are increasing */ 01059 { 01060 xinc1 = 1; 01061 xinc2 = 1; 01062 } 01063 else /* The x-values are decreasing */ 01064 { 01065 xinc1 = -1; 01066 xinc2 = -1; 01067 } 01068 01069 if (y2 >= y1) /* The y-values are increasing */ 01070 { 01071 yinc1 = 1; 01072 yinc2 = 1; 01073 } 01074 else /* The y-values are decreasing */ 01075 { 01076 yinc1 = -1; 01077 yinc2 = -1; 01078 } 01079 01080 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01081 { 01082 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01083 yinc2 = 0; /* Don't change the y for every iteration */ 01084 den = deltax; 01085 num = deltax / 2; 01086 numadd = deltay; 01087 numpixels = deltax; /* There are more x-values than y-values */ 01088 } 01089 else /* There is at least one y-value for every x-value */ 01090 { 01091 xinc2 = 0; /* Don't change the x for every iteration */ 01092 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01093 den = deltay; 01094 num = deltay / 2; 01095 numadd = deltax; 01096 numpixels = deltay; /* There are more y-values than x-values */ 01097 } 01098 01099 for (curpixel = 0; curpixel <= numpixels; curpixel++) 01100 { 01101 BSP_LCD_DrawLine(x, y, x3, y3); 01102 01103 num += numadd; /* Increase the numerator by the top of the fraction */ 01104 if (num >= den) /* Check if numerator >= denominator */ 01105 { 01106 num -= den; /* Calculate the new numerator value */ 01107 x += xinc1; /* Change the x as appropriate */ 01108 y += yinc1; /* Change the y as appropriate */ 01109 } 01110 x += xinc2; /* Change the x as appropriate */ 01111 y += yinc2; /* Change the y as appropriate */ 01112 } 01113 } 01114 01115 /** 01116 * @} 01117 */ 01118 01119 /** 01120 * @} 01121 */ 01122 01123 /** 01124 * @} 01125 */ 01126 01127 /** 01128 * @} 01129 */ 01130 01131 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jan 26 2017 16:30:37 for STM32F413H-Discovery BSP User Manual by
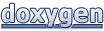