STM32F413H-Discovery BSP User Manual
|
stm32f413h_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f413h_discovery.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 27-January-2017 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM32F413H-DISCOVERY board 00009 * (MB1209) from STMicroelectronics. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "stm32f413h_discovery.h" 00042 00043 00044 /** @addtogroup BSP 00045 * @{ 00046 */ 00047 00048 /** @defgroup STM32F413H_DISCOVERY STM32F413H_DISCOVERY 00049 * @{ 00050 */ 00051 00052 /** @defgroup STM32F413H_DISCOVERY_LOW_LEVEL STM32F413H-DISCOVERY LOW LEVEL 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32F413H_DISCOVERY_LOW_LEVEL_Private_TypesDefinitions STM32F413H Discovery Low Level Private Typedef 00057 * @{ 00058 */ 00059 typedef struct 00060 { 00061 __IO uint16_t REG; 00062 __IO uint16_t RAM; 00063 }LCD_CONTROLLER_TypeDef; 00064 /** 00065 * @} 00066 */ 00067 00068 /** @defgroup STM32F413H_DISCOVERY_LOW_LEVEL_Private_Defines STM32F413H Discovery Low Level Private Def 00069 * @{ 00070 */ 00071 /** 00072 * @brief STM32F413H DISCOVERY BSP Driver version number V1.0.0 00073 */ 00074 #define __STM32F413H_DISCOVERY_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00075 #define __STM32F413H_DISCOVERY_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00076 #define __STM32F413H_DISCOVERY_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00077 #define __STM32F413H_DISCOVERY_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00078 #define __STM32F413H_DISCOVERY_BSP_VERSION ((__STM32F413H_DISCOVERY_BSP_VERSION_MAIN << 24)\ 00079 |(__STM32F413H_DISCOVERY_BSP_VERSION_SUB1 << 16)\ 00080 |(__STM32F413H_DISCOVERY_BSP_VERSION_SUB2 << 8 )\ 00081 |(__STM32F413H_DISCOVERY_BSP_VERSION_RC)) 00082 00083 /* We use BANK3 as we use FMC_NE3 signal */ 00084 #define FMC_BANK3_BASE ((uint32_t)(0x60000000 | 0x08000000)) 00085 #define FMC_BANK3 ((LCD_CONTROLLER_TypeDef *) FMC_BANK3_BASE) 00086 00087 /** 00088 * @} 00089 */ 00090 00091 /** @defgroup STM32F413H_DISCOVERY_LOW_LEVEL_Private_Variables STM32F413H Discovery Low Level Variables 00092 * @{ 00093 */ 00094 00095 const uint32_t GPIO_PIN[LEDn] = {LED3_PIN, 00096 LED4_PIN}; 00097 00098 00099 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED3_GPIO_PORT, 00100 LED4_GPIO_PORT}; 00101 00102 00103 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT}; 00104 00105 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN}; 00106 00107 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn}; 00108 00109 USART_TypeDef* COM_USART[COMn] = {DISCOVERY_COM1}; 00110 00111 GPIO_TypeDef* COM_TX_PORT[COMn] = {DISCOVERY_COM1_TX_GPIO_PORT}; 00112 00113 GPIO_TypeDef* COM_RX_PORT[COMn] = {DISCOVERY_COM1_RX_GPIO_PORT}; 00114 00115 const uint16_t COM_TX_PIN[COMn] = {DISCOVERY_COM1_TX_PIN}; 00116 00117 const uint16_t COM_RX_PIN[COMn] = {DISCOVERY_COM1_RX_PIN}; 00118 00119 const uint16_t COM_TX_AF[COMn] = {DISCOVERY_COM1_TX_AF}; 00120 00121 const uint16_t COM_RX_AF[COMn] = {DISCOVERY_COM1_RX_AF}; 00122 00123 static FMPI2C_HandleTypeDef hI2cAudioHandler; 00124 00125 /** 00126 * @} 00127 */ 00128 00129 /** @defgroup STM32F413H_DISCOVERY_LOW_LEVEL_Private_FunctionPrototypes STM32F413H Discovery Low Level Private Prototypes 00130 * @{ 00131 */ 00132 static void FMPI2Cx_Init(FMPI2C_HandleTypeDef *i2c_handler); 00133 static void FMPI2Cx_DeInit(FMPI2C_HandleTypeDef *i2c_handler); 00134 00135 static HAL_StatusTypeDef FMPI2Cx_ReadMultiple(FMPI2C_HandleTypeDef *fmpi2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00136 static HAL_StatusTypeDef FMPI2Cx_WriteMultiple(FMPI2C_HandleTypeDef *fmpi2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00137 static void FMPI2Cx_Error(FMPI2C_HandleTypeDef *fmpi2c_handler, uint8_t Addr); 00138 00139 static void FMC_BANK3_WriteData(uint16_t Data); 00140 static void FMC_BANK3_WriteReg(uint8_t Reg); 00141 static uint16_t FMC_BANK3_ReadData(void); 00142 static void FMC_BANK3_Init(void); 00143 static void FMC_BANK3_MspInit(void); 00144 00145 /* LCD IO functions */ 00146 void LCD_IO_Init(void); 00147 void LCD_IO_WriteData(uint16_t RegValue); 00148 void LCD_IO_WriteReg(uint8_t Reg); 00149 void LCD_IO_WriteMultipleData(uint16_t *pData, uint32_t Size); 00150 uint16_t LCD_IO_ReadData(void); 00151 void LCD_IO_Delay(uint32_t Delay); 00152 00153 /* AUDIO IO functions */ 00154 void AUDIO_IO_Init(void); 00155 void AUDIO_IO_DeInit(void); 00156 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00157 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00158 void AUDIO_IO_Delay(uint32_t Delay); 00159 00160 /* TouchScreen (TS) IO functions */ 00161 void TS_IO_Init(void); 00162 void TS_IO_DeInit(void); 00163 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00164 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00165 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00166 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00167 void TS_IO_Delay(uint32_t Delay); 00168 00169 /** 00170 * @} 00171 */ 00172 00173 /** @defgroup STM32F413H_DISCOVERY_LOW_LEVEL_Private_Functions STM32F413H Discovery Low Level Private Functions 00174 * @{ 00175 */ 00176 00177 /** 00178 * @brief This method returns the STM32F413H DISCOVERY BSP Driver revision 00179 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00180 */ 00181 uint32_t BSP_GetVersion(void) 00182 { 00183 return __STM32F413H_DISCOVERY_BSP_VERSION; 00184 } 00185 00186 /** 00187 * @brief Configures LEDs. 00188 * @param Led: LED to be configured. 00189 * This parameter can be one of the following values: 00190 * @arg LED3 00191 * @arg LED4 00192 */ 00193 void BSP_LED_Init(Led_TypeDef Led) 00194 { 00195 GPIO_InitTypeDef gpio_init_structure; 00196 00197 LEDx_GPIO_CLK_ENABLE(Led); 00198 /* Configure the GPIO_LED pin */ 00199 gpio_init_structure.Pin = GPIO_PIN[Led]; 00200 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00201 gpio_init_structure.Pull = GPIO_PULLUP; 00202 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00203 00204 HAL_GPIO_Init(GPIO_PORT[Led], &gpio_init_structure); 00205 } 00206 00207 /** 00208 * @brief DeInit LEDs. 00209 * @param Led: LED to be configured. 00210 * This parameter can be one of the following values: 00211 * @arg LED3 00212 * @arg LED4 00213 */ 00214 void BSP_LED_DeInit(Led_TypeDef Led) 00215 { 00216 GPIO_InitTypeDef gpio_init_structure; 00217 00218 /* DeInit the GPIO_LED pin */ 00219 gpio_init_structure.Pin = GPIO_PIN[Led]; 00220 00221 /* Turn off LED */ 00222 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00223 HAL_GPIO_DeInit(GPIO_PORT[Led], gpio_init_structure.Pin); 00224 } 00225 00226 /** 00227 * @brief Turns selected LED On. 00228 * @param Led: LED to be set on 00229 * This parameter can be one of the following values: 00230 * @arg LED3 00231 * @arg LED4 00232 */ 00233 void BSP_LED_On(Led_TypeDef Led) 00234 { 00235 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00236 } 00237 00238 /** 00239 * @brief Turns selected LED Off. 00240 * @param Led: LED to be set off 00241 * This parameter can be one of the following values: 00242 * @arg LED3 00243 * @arg LED4 00244 */ 00245 void BSP_LED_Off(Led_TypeDef Led) 00246 { 00247 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00248 } 00249 00250 /** 00251 * @brief Toggles the selected LED. 00252 * @param Led: LED to be toggled 00253 * This parameter can be one of the following values: 00254 * @arg LED3 00255 * @arg LED4 00256 */ 00257 void BSP_LED_Toggle(Led_TypeDef Led) 00258 { 00259 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00260 } 00261 00262 /** 00263 * @brief Configures button GPIO and EXTI Line. 00264 * @param Button: Button to be configured 00265 * This parameter can be one of the following values: 00266 * @arg BUTTON_WAKEUP: Wakeup Push Button 00267 * @param ButtonMode: Button mode 00268 * This parameter can be one of the following values: 00269 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00270 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00271 * with interrupt generation capability 00272 */ 00273 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00274 { 00275 GPIO_InitTypeDef gpio_init_structure; 00276 00277 /* Enable the BUTTON clock */ 00278 WAKEUP_BUTTON_GPIO_CLK_ENABLE(); 00279 00280 if(ButtonMode == BUTTON_MODE_GPIO) 00281 { 00282 /* Configure Button pin as input */ 00283 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00284 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00285 gpio_init_structure.Pull = GPIO_PULLDOWN; 00286 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00287 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00288 } 00289 00290 if(ButtonMode == BUTTON_MODE_EXTI) 00291 { 00292 /* Configure Button pin as input with External interrupt */ 00293 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00294 gpio_init_structure.Pull = GPIO_PULLDOWN; 00295 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00296 00297 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00298 00299 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00300 00301 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00302 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00303 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00304 } 00305 } 00306 00307 /** 00308 * @brief Push Button DeInit. 00309 * @param Button: Button to be configured 00310 * This parameter can be one of the following values: 00311 * @arg BUTTON_WAKEUP: Wakeup Push Button 00312 * @note PB DeInit does not disable the GPIO clock 00313 */ 00314 void BSP_PB_DeInit(Button_TypeDef Button) 00315 { 00316 GPIO_InitTypeDef gpio_init_structure; 00317 00318 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00319 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00320 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00321 } 00322 00323 00324 /** 00325 * @brief Returns the selected button state. 00326 * @param Button: Button to be checked 00327 * This parameter can be one of the following values: 00328 * @arg BUTTON_WAKEUP: Wakeup Push Button 00329 * @retval The Button GPIO pin value (GPIO_PIN_RESET = button pressed) 00330 */ 00331 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00332 { 00333 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00334 } 00335 00336 /** 00337 * @brief Configures COM port. 00338 * @param COM: COM port to be configured. 00339 * This parameter can be one of the following values: 00340 * @arg COM1 00341 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00342 * configuration information for the specified USART peripheral. 00343 */ 00344 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00345 { 00346 GPIO_InitTypeDef gpio_init_structure; 00347 00348 /* Enable GPIO clock */ 00349 DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(COM); 00350 DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(COM); 00351 00352 /* Enable USART clock */ 00353 DISCOVERY_COMx_CLK_ENABLE(COM); 00354 00355 /* Configure USART Tx as alternate function */ 00356 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00357 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00358 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00359 gpio_init_structure.Pull = GPIO_PULLUP; 00360 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00361 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00362 00363 /* Configure USART Rx as alternate function */ 00364 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00365 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00366 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00367 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00368 00369 /* USART configuration */ 00370 huart->Instance = COM_USART[COM]; 00371 HAL_UART_Init(huart); 00372 } 00373 00374 /** 00375 * @brief DeInit COM port. 00376 * @param COM: COM port to be configured. 00377 * This parameter can be one of the following values: 00378 * @arg COM1 00379 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00380 * configuration information for the specified USART peripheral. 00381 */ 00382 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00383 { 00384 /* USART configuration */ 00385 huart->Instance = COM_USART[COM]; 00386 HAL_UART_DeInit(huart); 00387 00388 /* Enable USART clock */ 00389 DISCOVERY_COMx_CLK_DISABLE(COM); 00390 00391 /* DeInit GPIO pins can be done in the application 00392 (by surcharging this __weak function) */ 00393 00394 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the application 00395 by surcharging this __weak function */ 00396 } 00397 00398 00399 /******************************************************************************* 00400 BUS OPERATIONS 00401 *******************************************************************************/ 00402 00403 /******************************* I2C Routines *********************************/ 00404 /** 00405 * @brief Initializes FMPI2C MSP. 00406 * @param fmpi2c_handler : FMPI2C handler 00407 */ 00408 static void FMPI2Cx_MspInit(FMPI2C_HandleTypeDef *fmpi2c_handler) 00409 { 00410 GPIO_InitTypeDef gpio_init_structure; 00411 00412 /* AUDIO FMPI2C MSP init */ 00413 00414 /*** Configure the GPIOs ***/ 00415 /* Enable GPIO clock */ 00416 DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00417 00418 /* Configure I2C Tx as alternate function */ 00419 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SCL_PIN; 00420 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00421 gpio_init_structure.Pull = GPIO_NOPULL; 00422 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00423 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SCL_SDA_AF; 00424 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00425 00426 /* Configure I2C Rx as alternate function */ 00427 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SDA_PIN; 00428 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SCL_SDA_AF; 00429 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00430 00431 /*** Configure the I2C peripheral ***/ 00432 /* Enable I2C clock */ 00433 DISCOVERY_AUDIO_I2Cx_CLK_ENABLE(); 00434 00435 /* Force the I2C peripheral clock reset */ 00436 DISCOVERY_AUDIO_I2Cx_FORCE_RESET(); 00437 00438 /* Release the I2C peripheral clock reset */ 00439 DISCOVERY_AUDIO_I2Cx_RELEASE_RESET(); 00440 00441 /* Enable and set I2Cx Interrupt to a lower priority */ 00442 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_EV_IRQn, 0x0F, 0x00); 00443 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_EV_IRQn); 00444 00445 /* Enable and set I2Cx Interrupt to a lower priority */ 00446 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_ER_IRQn, 0x0F, 0x00); 00447 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_ER_IRQn); 00448 } 00449 00450 /** 00451 * @brief Initializes FMPI2C HAL. 00452 * @param fmpi2c_handler : FMPI2C handler 00453 */ 00454 static void FMPI2Cx_Init(FMPI2C_HandleTypeDef *fmpi2c_handler) 00455 { 00456 if(HAL_FMPI2C_GetState(fmpi2c_handler) == HAL_FMPI2C_STATE_RESET) 00457 { 00458 /* Audio FMPI2C configuration */ 00459 fmpi2c_handler->Instance = DISCOVERY_AUDIO_I2Cx; 00460 fmpi2c_handler->Init.Timing = DISCOVERY_I2Cx_TIMING; 00461 fmpi2c_handler->Init.OwnAddress1 = 0; 00462 fmpi2c_handler->Init.AddressingMode = FMPI2C_ADDRESSINGMODE_7BIT; 00463 fmpi2c_handler->Init.DualAddressMode = FMPI2C_DUALADDRESS_DISABLE; 00464 fmpi2c_handler->Init.OwnAddress2 = 0; 00465 fmpi2c_handler->Init.OwnAddress2Masks = FMPI2C_OA2_NOMASK; 00466 fmpi2c_handler->Init.GeneralCallMode = FMPI2C_GENERALCALL_DISABLE; 00467 fmpi2c_handler->Init.NoStretchMode = FMPI2C_NOSTRETCH_DISABLE; 00468 /* Init the FMPI2C */ 00469 FMPI2Cx_MspInit(fmpi2c_handler); 00470 HAL_FMPI2C_Init(fmpi2c_handler); 00471 } 00472 } 00473 00474 /** 00475 * @brief Reads multiple data. 00476 * @param fmpi2c_handler : FMPI2C handler 00477 * @param Addr: I2C address 00478 * @param Reg: Reg address 00479 * @param MemAddress: Memory address 00480 * @param Buffer: Pointer to data buffer 00481 * @param Length: Length of the data 00482 * @retval Number of read data 00483 */ 00484 static HAL_StatusTypeDef FMPI2Cx_ReadMultiple(FMPI2C_HandleTypeDef *fmpi2c_handler, 00485 uint8_t Addr, 00486 uint16_t Reg, 00487 uint16_t MemAddress, 00488 uint8_t *Buffer, 00489 uint16_t Length) 00490 { 00491 HAL_StatusTypeDef status = HAL_OK; 00492 00493 status = HAL_FMPI2C_Mem_Read(fmpi2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00494 00495 /* Check the communication status */ 00496 if(status != HAL_OK) 00497 { 00498 /* FMPI2C error occurred */ 00499 FMPI2Cx_Error(fmpi2c_handler, Addr); 00500 } 00501 return status; 00502 } 00503 00504 /** 00505 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00506 * @param fmpi2c_handler : FMPI2C handler 00507 * @param Addr: Device address on BUS Bus. 00508 * @param Reg: The target register address to write 00509 * @param MemAddress: Memory address 00510 * @param Buffer: The target register value to be written 00511 * @param Length: buffer size to be written 00512 * @retval HAL status 00513 */ 00514 static HAL_StatusTypeDef FMPI2Cx_WriteMultiple(FMPI2C_HandleTypeDef *fmpi2c_handler, 00515 uint8_t Addr, 00516 uint16_t Reg, 00517 uint16_t MemAddress, 00518 uint8_t *Buffer, 00519 uint16_t Length) 00520 { 00521 HAL_StatusTypeDef status = HAL_OK; 00522 00523 status = HAL_FMPI2C_Mem_Write(fmpi2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00524 00525 /* Check the communication status */ 00526 if(status != HAL_OK) 00527 { 00528 /* Re-Initialize the FMPI2C Bus */ 00529 FMPI2Cx_Error(fmpi2c_handler, Addr); 00530 } 00531 return status; 00532 } 00533 00534 /** 00535 * @brief Manages error callback by re-initializing I2C. 00536 * @param fmpi2c_handler : FMPI2C handler 00537 * @param Addr: I2C Address 00538 * @retval None 00539 */ 00540 static void FMPI2Cx_Error(FMPI2C_HandleTypeDef *fmpi2c_handler, uint8_t Addr) 00541 { 00542 /* De-initialize the FMPI2C communication bus */ 00543 HAL_FMPI2C_DeInit(fmpi2c_handler); 00544 00545 /* Re-Initialize the FMPI2C communication bus */ 00546 FMPI2Cx_Init(fmpi2c_handler); 00547 } 00548 00549 /** 00550 * @brief Deinitializes FMPI2C interface 00551 * @param fmpi2c_handler : FMPI2C handler 00552 */ 00553 static void FMPI2Cx_DeInit(FMPI2C_HandleTypeDef *fmpi2c_handler) 00554 { 00555 /* Audio and LCD I2C configuration */ 00556 fmpi2c_handler->Instance = DISCOVERY_AUDIO_I2Cx; 00557 /* Disable FMPI2C block */ 00558 __HAL_FMPI2C_DISABLE(fmpi2c_handler); 00559 00560 /* DeInit the FMPI2C */ 00561 HAL_FMPI2C_DeInit(fmpi2c_handler); 00562 } 00563 /*************************** FMC Routines ************************************/ 00564 /** 00565 * @brief Initializes FMC_BANK3 MSP. 00566 */ 00567 static void FMC_BANK3_MspInit(void) 00568 { 00569 GPIO_InitTypeDef gpio_init_structure; 00570 00571 /* Enable FSMC clock */ 00572 __HAL_RCC_FSMC_CLK_ENABLE(); 00573 00574 /* Enable GPIOs clock */ 00575 __HAL_RCC_GPIOD_CLK_ENABLE(); 00576 __HAL_RCC_GPIOE_CLK_ENABLE(); 00577 __HAL_RCC_GPIOF_CLK_ENABLE(); 00578 __HAL_RCC_GPIOG_CLK_ENABLE(); 00579 00580 /* Common GPIO configuration */ 00581 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00582 gpio_init_structure.Pull = GPIO_PULLUP; 00583 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00584 gpio_init_structure.Alternate = GPIO_AF12_FSMC; 00585 00586 /* GPIOD configuration: GPIO_PIN_7 is FMC_NE1 , GPIO_PIN_11 ans GPIO_PIN_12 are PSRAM_A16 and PSRAM_A17 */ 00587 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_8 |\ 00588 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_14 | GPIO_PIN_15 | GPIO_PIN_7|\ 00589 GPIO_PIN_11 | GPIO_PIN_12; 00590 00591 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00592 00593 /* GPIOE configuration */ 00594 gpio_init_structure.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00595 GPIO_PIN_12 |GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00596 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00597 00598 /* GPIOF configuration */ 00599 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 |\ 00600 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00601 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00602 00603 /* GPIOG configuration */ 00604 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 |\ 00605 GPIO_PIN_5 | GPIO_PIN_10 ; 00606 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00607 } 00608 00609 /** 00610 * @brief Initializes LCD IOs. 00611 */ 00612 static void FMC_BANK3_Init(void) 00613 { 00614 SRAM_HandleTypeDef hsram; 00615 FSMC_NORSRAM_TimingTypeDef sram_timing; 00616 00617 /* Initialize the SRAM controller */ 00618 FMC_BANK3_MspInit(); 00619 00620 /*** Configure the SRAM Bank 1 ***/ 00621 /* Configure IPs */ 00622 hsram.Instance = FSMC_NORSRAM_DEVICE; 00623 hsram.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00624 00625 /* Timing config */ 00626 sram_timing.AddressSetupTime = 3; 00627 sram_timing.AddressHoldTime = 1; 00628 sram_timing.DataSetupTime = 4; 00629 sram_timing.BusTurnAroundDuration = 1; 00630 sram_timing.CLKDivision = 2; 00631 sram_timing.DataLatency = 2; 00632 sram_timing.AccessMode = FSMC_ACCESS_MODE_A; 00633 00634 hsram.Init.NSBank = FSMC_NORSRAM_BANK3; 00635 hsram.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00636 hsram.Init.MemoryType = FSMC_MEMORY_TYPE_SRAM; 00637 hsram.Init.MemoryDataWidth = FSMC_NORSRAM_MEM_BUS_WIDTH_16; 00638 hsram.Init.BurstAccessMode = FSMC_BURST_ACCESS_MODE_DISABLE; 00639 hsram.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00640 hsram.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00641 hsram.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00642 hsram.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00643 hsram.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00644 hsram.Init.ExtendedMode = FSMC_EXTENDED_MODE_ENABLE; 00645 hsram.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00646 hsram.Init.WriteBurst = FSMC_WRITE_BURST_DISABLE; 00647 hsram.Init.WriteFifo = FSMC_WRITE_FIFO_DISABLE; 00648 hsram.Init.PageSize = FSMC_PAGE_SIZE_NONE; 00649 hsram.Init.ContinuousClock = FSMC_CONTINUOUS_CLOCK_SYNC_ONLY; 00650 00651 HAL_SRAM_Init(&hsram, &sram_timing, &sram_timing); 00652 } 00653 00654 /** 00655 * @brief Writes register value. 00656 * @param Data: Data to be written 00657 */ 00658 static void FMC_BANK3_WriteData(uint16_t Data) 00659 { 00660 /* Write 16-bit Reg */ 00661 FMC_BANK3->RAM = Data; 00662 __DSB(); 00663 } 00664 00665 /** 00666 * @brief Writes register address. 00667 * @param Reg: Register to be written 00668 */ 00669 static void FMC_BANK3_WriteReg(uint8_t Reg) 00670 { 00671 /* Write 16-bit Index, then write register */ 00672 FMC_BANK3->REG = Reg; 00673 __DSB(); 00674 } 00675 00676 /** 00677 * @brief Reads register value. 00678 * @retval Read value 00679 */ 00680 static uint16_t FMC_BANK3_ReadData(void) 00681 { 00682 return FMC_BANK3->RAM; 00683 } 00684 00685 /******************************************************************************* 00686 LINK OPERATIONS 00687 *******************************************************************************/ 00688 00689 /********************************* LINK LCD ***********************************/ 00690 /** 00691 * @brief Initializes LCD low level. 00692 */ 00693 void LCD_IO_Init(void) 00694 { 00695 FMC_BANK3_Init(); 00696 } 00697 00698 /** 00699 * @brief Writes data on LCD data register. 00700 * @param RegValue: Data to be written 00701 */ 00702 void LCD_IO_WriteData(uint16_t RegValue) 00703 { 00704 /* Write 16-bit Reg */ 00705 FMC_BANK3_WriteData(RegValue); 00706 } 00707 00708 /** 00709 * @brief Writes several data on LCD data register. 00710 * @param pData: pointer on data to be written 00711 * @param Size: data amount in 16bits short unit 00712 */ 00713 void LCD_IO_WriteMultipleData(uint16_t *pData, uint32_t Size) 00714 { 00715 uint32_t i; 00716 00717 for (i = 0; i < Size; i++) 00718 { 00719 FMC_BANK3_WriteData(pData[i]); 00720 } 00721 } 00722 00723 /** 00724 * @brief Writes register on LCD register. 00725 * @param Reg: Register to be written 00726 */ 00727 void LCD_IO_WriteReg(uint8_t Reg) 00728 { 00729 /* Write 16-bit Index, then Write Reg */ 00730 FMC_BANK3_WriteReg(Reg); 00731 } 00732 00733 /** 00734 * @brief Reads data from LCD data register. 00735 * @retval Read data. 00736 */ 00737 uint16_t LCD_IO_ReadData(void) 00738 { 00739 return FMC_BANK3_ReadData(); 00740 } 00741 00742 /** 00743 * @brief LCD delay 00744 * @param Delay: Delay in ms 00745 */ 00746 void LCD_IO_Delay(uint32_t Delay) 00747 { 00748 HAL_Delay(Delay); 00749 } 00750 00751 /********************************* LINK AUDIO *********************************/ 00752 00753 /** 00754 * @brief Initializes Audio low level. 00755 */ 00756 void AUDIO_IO_Init(void) 00757 { 00758 FMPI2Cx_Init(&hI2cAudioHandler); 00759 } 00760 00761 /** 00762 * @brief Deinitializes Audio low level. 00763 */ 00764 void AUDIO_IO_DeInit(void) 00765 { 00766 FMPI2Cx_DeInit(&hI2cAudioHandler); 00767 } 00768 00769 /** 00770 * @brief Writes a single data. 00771 * @param Addr: I2C address 00772 * @param Reg: Reg address 00773 * @param Value: Data to be written 00774 */ 00775 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 00776 { 00777 uint16_t tmp = Value; 00778 00779 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 00780 00781 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 00782 00783 FMPI2Cx_WriteMultiple(&hI2cAudioHandler, Addr, Reg, FMPI2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 00784 } 00785 00786 /** 00787 * @brief Reads a single data. 00788 * @param Addr: I2C address 00789 * @param Reg: Reg address 00790 * @retval Data to be read 00791 */ 00792 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 00793 { 00794 uint16_t read_value = 0, tmp = 0; 00795 00796 FMPI2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, FMPI2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 00797 00798 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 00799 00800 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 00801 00802 read_value = tmp; 00803 00804 return read_value; 00805 } 00806 00807 /** 00808 * @brief Reads multiple data with I2C communication 00809 * channel from TouchScreen. 00810 * @param Addr: I2C address 00811 * @param Reg: Register address 00812 * @param Buffer: Pointer to data buffer 00813 * @param Length: Length of the data 00814 * @retval Number of read data 00815 */ 00816 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00817 { 00818 return FMPI2Cx_ReadMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00819 } 00820 00821 /** 00822 * @brief Writes multiple data with I2C communication 00823 * channel from MCU to TouchScreen. 00824 * @param Addr: I2C address 00825 * @param Reg: Register address 00826 * @param Buffer: Pointer to data buffer 00827 * @param Length: Length of the data 00828 */ 00829 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00830 { 00831 FMPI2Cx_WriteMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00832 } 00833 00834 /** 00835 * @brief AUDIO Codec delay 00836 * @param Delay: Delay in ms 00837 */ 00838 void AUDIO_IO_Delay(uint32_t Delay) 00839 { 00840 HAL_Delay(Delay); 00841 } 00842 00843 /******************************** LINK TS (TouchScreen) *****************************/ 00844 00845 /** 00846 * @brief Initializes TS low level. 00847 */ 00848 void TS_IO_Init(void) 00849 { 00850 GPIO_InitTypeDef gpio_init_structure; 00851 00852 TS_RESET_GPIO_CLK_ENABLE(); 00853 00854 /* Configure Button pin as input */ 00855 gpio_init_structure.Pin = TS_RESET_PIN; 00856 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00857 gpio_init_structure.Pull = GPIO_NOPULL; 00858 gpio_init_structure.Speed = GPIO_SPEED_FREQ_LOW; 00859 HAL_GPIO_Init(TS_RESET_GPIO_PORT, &gpio_init_structure); 00860 00861 FMPI2Cx_Init(&hI2cAudioHandler); 00862 } 00863 00864 /** 00865 * @brief Deinitializes TS low level. 00866 */ 00867 void TS_IO_DeInit(void) 00868 { 00869 FMPI2Cx_DeInit(&hI2cAudioHandler); 00870 } 00871 00872 /** 00873 * @brief Reads a single data. 00874 * @param Addr: I2C address 00875 * @param Reg: Register address 00876 * @retval Data to be read 00877 */ 00878 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 00879 { 00880 uint8_t read_value = 0; 00881 00882 FMPI2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00883 00884 return read_value; 00885 } 00886 00887 /** 00888 * @brief Writes a single data. 00889 * @param Addr: I2C address 00890 * @param Reg: Reg address 00891 * @param Value: Data to be written 00892 */ 00893 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00894 { 00895 FMPI2Cx_WriteMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00896 } 00897 00898 /** 00899 * @brief Delay function used in TouchScreen low level driver. 00900 * @param Delay: Delay in ms 00901 */ 00902 void TS_IO_Delay(uint32_t Delay) 00903 { 00904 HAL_Delay(Delay); 00905 } 00906 00907 /** 00908 * @} 00909 */ 00910 00911 /** 00912 * @} 00913 */ 00914 00915 /** 00916 * @} 00917 */ 00918 00919 /** 00920 * @} 00921 */ 00922 00923 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jan 26 2017 16:30:37 for STM32F413H-Discovery BSP User Manual by
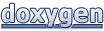