STM32F401-Discovery BSP User Manual
|
stm32f401_discovery_gyroscope.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f401_discovery_gyroscope.c 00004 * @author MCD Application Team 00005 * @version V2.2.2 00006 * @date 31-January-2017 00007 * @brief This file provides a set of functions needed to manage the 00008 * MEMS gyroscope available on STM32F401-Discovery Kit. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 /* Includes ------------------------------------------------------------------*/ 00039 #include "stm32f401_discovery_gyroscope.h" 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup STM32F401_DISCOVERY 00046 * @{ 00047 */ 00048 00049 /** @defgroup STM32F401_DISCOVERY_GYROSCOPE STM32F401 DISCOVERY GYROSCOPE 00050 * @{ 00051 */ 00052 00053 00054 /** @defgroup STM32F401_DISCOVERY_GYROSCOPE_Private_TypesDefinitions STM32F401 DISCOVERY GYROSCOPE Private TypesDefinitions 00055 * @{ 00056 */ 00057 /** 00058 * @} 00059 */ 00060 00061 /** @defgroup STM32F401_DISCOVERY_GYROSCOPE_Private_Defines STM32F401 DISCOVERY GYROSCOPE Private Defines 00062 * @{ 00063 */ 00064 /** 00065 * @} 00066 */ 00067 00068 /** @defgroup STM32F401_DISCOVERY_GYROSCOPE_Private_Macros STM32F401 DISCOVERY GYROSCOPE Private Macros 00069 * @{ 00070 */ 00071 /** 00072 * @} 00073 */ 00074 00075 /** @defgroup STM32F401_DISCOVERY_GYROSCOPE_Private_Variables STM32F401 DISCOVERY GYROSCOPE Private Variables 00076 * @{ 00077 */ 00078 static GYRO_DrvTypeDef *GyroscopeDrv; 00079 /** 00080 * @} 00081 */ 00082 00083 /** @defgroup STM32F401_DISCOVERY_GYROSCOPE_Private_FunctionPrototypes STM32F401 DISCOVERY GYROSCOPE Private FunctionPrototypes 00084 * @{ 00085 */ 00086 /** 00087 * @} 00088 */ 00089 00090 /** @defgroup STM32F401_DISCOVERY_GYROSCOPE_Private_Functions STM32F401 DISCOVERY GYROSCOPE Private Functions 00091 * @{ 00092 */ 00093 00094 /** 00095 * @brief Set Gyroscope Initialization. 00096 * @retval GYRO_OK if no problem during initialization 00097 */ 00098 uint8_t BSP_GYRO_Init(void) 00099 { 00100 uint8_t ret = GYRO_ERROR; 00101 uint16_t ctrl = 0x0000; 00102 GYRO_InitTypeDef L3GD20_InitStructure; 00103 GYRO_FilterConfigTypeDef L3GD20_FilterStructure = {0,0}; 00104 00105 if((L3gd20Drv.ReadID() == I_AM_L3GD20) || (L3gd20Drv.ReadID() == I_AM_L3GD20_TR)) 00106 { 00107 /* Initialize the Gyroscope driver structure */ 00108 GyroscopeDrv = &L3gd20Drv; 00109 00110 /* MEMS configuration ----------------------------------------------------*/ 00111 /* Fill the Gyroscope structure */ 00112 L3GD20_InitStructure.Power_Mode = L3GD20_MODE_ACTIVE; 00113 L3GD20_InitStructure.Output_DataRate = L3GD20_OUTPUT_DATARATE_1; 00114 L3GD20_InitStructure.Axes_Enable = L3GD20_AXES_ENABLE; 00115 L3GD20_InitStructure.Band_Width = L3GD20_BANDWIDTH_4; 00116 L3GD20_InitStructure.BlockData_Update = L3GD20_BlockDataUpdate_Continous; 00117 L3GD20_InitStructure.Endianness = L3GD20_BLE_LSB; 00118 L3GD20_InitStructure.Full_Scale = L3GD20_FULLSCALE_500; 00119 00120 /* Configure MEMS: data rate, power mode, full scale and axes */ 00121 ctrl = (uint16_t) (L3GD20_InitStructure.Power_Mode | L3GD20_InitStructure.Output_DataRate | \ 00122 L3GD20_InitStructure.Axes_Enable | L3GD20_InitStructure.Band_Width); 00123 00124 ctrl |= (uint16_t) ((L3GD20_InitStructure.BlockData_Update | L3GD20_InitStructure.Endianness | \ 00125 L3GD20_InitStructure.Full_Scale) << 8); 00126 00127 /* Configure the Gyroscope main parameters */ 00128 GyroscopeDrv->Init(ctrl); 00129 00130 L3GD20_FilterStructure.HighPassFilter_Mode_Selection =L3GD20_HPM_NORMAL_MODE_RES; 00131 L3GD20_FilterStructure.HighPassFilter_CutOff_Frequency = L3GD20_HPFCF_0; 00132 00133 ctrl = (uint8_t) ((L3GD20_FilterStructure.HighPassFilter_Mode_Selection |\ 00134 L3GD20_FilterStructure.HighPassFilter_CutOff_Frequency)); 00135 00136 /* Configure the Gyroscope main parameters */ 00137 GyroscopeDrv->FilterConfig(ctrl) ; 00138 00139 GyroscopeDrv->FilterCmd(L3GD20_HIGHPASSFILTER_ENABLE); 00140 00141 ret = GYRO_OK; 00142 } 00143 return ret; 00144 } 00145 00146 /** 00147 * @brief Read ID of Gyroscope component. 00148 * @retval ID 00149 */ 00150 uint8_t BSP_GYRO_ReadID(void) 00151 { 00152 uint8_t id = 0x00; 00153 00154 if(GyroscopeDrv->ReadID != NULL) 00155 { 00156 id = GyroscopeDrv->ReadID(); 00157 } 00158 return id; 00159 } 00160 00161 /** 00162 * @brief Reboot memory content of Gyroscope. 00163 */ 00164 void BSP_GYRO_Reset(void) 00165 { 00166 if(GyroscopeDrv->Reset != NULL) 00167 { 00168 GyroscopeDrv->Reset(); 00169 } 00170 } 00171 00172 /** 00173 * @brief Configures INT1 interrupt. 00174 * @param pIntConfig: pointer to a L3GD20_InterruptConfig_TypeDef 00175 * structure that contains the configuration setting for the L3GD20 Interrupt. 00176 */ 00177 void BSP_GYRO_ITConfig(GYRO_InterruptConfigTypeDef *pIntConfig) 00178 { 00179 uint16_t interruptconfig = 0x0000; 00180 00181 if(GyroscopeDrv->ConfigIT != NULL) 00182 { 00183 /* Configure latch Interrupt request and axe interrupts */ 00184 interruptconfig |= ((uint8_t)(pIntConfig->Latch_Request| \ 00185 pIntConfig->Interrupt_Axes) << 8); 00186 00187 interruptconfig |= (uint8_t)(pIntConfig->Interrupt_ActiveEdge); 00188 00189 GyroscopeDrv->ConfigIT(interruptconfig); 00190 } 00191 } 00192 00193 /** 00194 * @brief Enables INT1 or INT2 interrupt. 00195 * @param IntPin: Interrupt pin 00196 * This parameter can be: 00197 * @arg L3GD20_INT1 00198 * @arg L3GD20_INT2 00199 */ 00200 void BSP_GYRO_EnableIT(uint8_t IntPin) 00201 { 00202 if(GyroscopeDrv->EnableIT != NULL) 00203 { 00204 GyroscopeDrv->EnableIT(IntPin); 00205 } 00206 } 00207 00208 /** 00209 * @brief Disables INT1 or INT2 interrupt. 00210 * @param IntPin: Interrupt pin 00211 * This parameter can be: 00212 * @arg L3GD20_INT1 00213 * @arg L3GD20_INT2 00214 */ 00215 void BSP_GYRO_DisableIT(uint8_t IntPin) 00216 { 00217 if(GyroscopeDrv->DisableIT != NULL) 00218 { 00219 GyroscopeDrv->DisableIT(IntPin); 00220 } 00221 } 00222 00223 /** 00224 * @brief Get XYZ angular acceleration. 00225 * @param pfData: pointer on floating array 00226 */ 00227 void BSP_GYRO_GetXYZ(float *pfData) 00228 { 00229 if(GyroscopeDrv->GetXYZ!= NULL) 00230 { 00231 GyroscopeDrv->GetXYZ(pfData); 00232 } 00233 } 00234 00235 /** 00236 * @} 00237 */ 00238 00239 /** 00240 * @} 00241 */ 00242 00243 /** 00244 * @} 00245 */ 00246 00247 /** 00248 * @} 00249 */ 00250 00251 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jan 19 2017 16:53:47 for STM32F401-Discovery BSP User Manual by
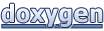