STM32F401-Discovery BSP User Manual
|
stm32f401_discovery_audio.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f401_discovery_audio.h 00004 * @author MCD Application Team 00005 * @version V2.2.2 00006 * @date 31-January-2017 00007 * @brief This file contains the common defines and functions prototypes for 00008 * stm32f401_discovery_audio.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32F401_DISCOVERY_AUDIO_H 00041 #define __STM32F401_DISCOVERY_AUDIO_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 /* Include audio component Driver */ 00049 #include "../Components/cs43l22/cs43l22.h" 00050 #include "stm32f401_discovery.h" 00051 #include "../../../Middlewares/ST/STM32_Audio/Addons/PDM/pdm_filter.h" 00052 00053 /** @addtogroup BSP 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM32F401_DISCOVERY 00058 * @{ 00059 */ 00060 00061 /** @addtogroup STM32F401_DISCOVERY_AUDIO 00062 * @{ 00063 */ 00064 00065 /** @defgroup STM32F401_DISCOVERY_AUDIO_Exported_Types STM32F401 DISCOVERY AUDIO Exported Types 00066 * @{ 00067 */ 00068 /** 00069 * @} 00070 */ 00071 00072 /** @defgroup STM32F401_DISCOVERY_AUDIO_OUT_Exported_Constants STM32F401 DISCOVERY AUDIO OUT Exported Constants 00073 * @{ 00074 */ 00075 00076 /*------------------------------------------------------------------------------ 00077 AUDIO OUT CONFIGURATION 00078 ------------------------------------------------------------------------------*/ 00079 00080 /* I2S peripheral configuration defines */ 00081 #define I2S3 SPI3 00082 #define I2S3_CLK_ENABLE() __HAL_RCC_SPI3_CLK_ENABLE() 00083 #define I2S3_CLK_DISABLE() __HAL_RCC_SPI3_CLK_DISABLE() 00084 #define I2S3_SCK_SD_WS_AF GPIO_AF6_SPI3 00085 #define I2S3_SCK_SD_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00086 #define I2S3_MCK_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00087 #define I2S3_WS_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00088 #define I2S3_WS_PIN GPIO_PIN_4 00089 #define I2S3_SCK_PIN GPIO_PIN_10 00090 #define I2S3_SD_PIN GPIO_PIN_12 00091 #define I2S3_MCK_PIN GPIO_PIN_7 00092 #define I2S3_SCK_SD_GPIO_PORT GPIOC 00093 #define I2S3_WS_GPIO_PORT GPIOA 00094 #define I2S3_MCK_GPIO_PORT GPIOC 00095 00096 /* I2S DMA Stream definitions */ 00097 #define I2S3_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00098 #define I2S3_DMAx_CLK_DISABLE() __HAL_RCC_DMA1_CLK_DISABLE() 00099 #define I2S3_DMAx_STREAM DMA1_Stream7 00100 #define I2S3_DMAx_CHANNEL DMA_CHANNEL_0 00101 #define I2S3_DMAx_IRQ DMA1_Stream7_IRQn 00102 #define I2S3_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00103 #define I2S3_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00104 #define DMA_MAX_SZE 0xFFFF 00105 00106 #define I2S3_IRQHandler DMA1_Stream7_IRQHandler 00107 00108 /* Select the interrupt preemption priority and subpriority for the DMA interrupt */ 00109 #define AUDIO_OUT_IRQ_PREPRIO 0x0E /* Select the preemption priority level(0 is the highest) */ 00110 00111 /*------------------------------------------------------------------------------ 00112 AUDIO IN CONFIGURATION 00113 ------------------------------------------------------------------------------*/ 00114 /* SPI Configuration defines */ 00115 #define I2S2 SPI2 00116 #define I2S2_CLK_ENABLE() __HAL_RCC_SPI2_CLK_ENABLE() 00117 #define I2S2_CLK_DISABLE() __HAL_RCC_SPI2_CLK_DISABLE() 00118 #define I2S2_SCK_PIN GPIO_PIN_10 00119 #define I2S2_SCK_GPIO_PORT GPIOB 00120 #define I2S2_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00121 #define I2S2_SCK_AF GPIO_AF5_SPI2 00122 00123 #define I2S2_MOSI_PIN GPIO_PIN_3 00124 #define I2S2_MOSI_GPIO_PORT GPIOC 00125 #define I2S2_MOSI_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00126 #define I2S2_MOSI_AF GPIO_AF5_SPI2 00127 00128 /* I2S DMA Stream Rx definitions */ 00129 #define I2S2_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00130 #define I2S2_DMAx_CLK_DISABLE() __HAL_RCC_DMA1_CLK_DISABLE() 00131 #define I2S2_DMAx_STREAM DMA1_Stream3 00132 #define I2S2_DMAx_CHANNEL DMA_CHANNEL_0 00133 #define I2S2_DMAx_IRQ DMA1_Stream3_IRQn 00134 #define I2S2_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00135 #define I2S2_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00136 00137 #define I2S2_IRQHandler DMA1_Stream3_IRQHandler 00138 00139 /* Select the interrupt preemption priority and subpriority for the IT/DMA interrupt */ 00140 #define AUDIO_IN_IRQ_PREPRIO 0x0F /* Select the preemption priority level(0 is the highest) */ 00141 00142 /*------------------------------------------------------------------------------ 00143 CONFIGURATION: Audio Driver Configuration parameters 00144 ------------------------------------------------------------------------------*/ 00145 00146 #define AUDIODATA_SIZE 2 /* 16-bits audio data size */ 00147 00148 /* Audio status definition */ 00149 #define AUDIO_OK 0 00150 #define AUDIO_ERROR 1 00151 #define AUDIO_TIMEOUT 2 00152 00153 /* AudioFreq * DataSize (2 bytes) * NumChannels (Stereo: 2) */ 00154 #define DEFAULT_AUDIO_IN_FREQ I2S_AUDIOFREQ_16K 00155 #define DEFAULT_AUDIO_IN_BIT_RESOLUTION 16 00156 #define DEFAULT_AUDIO_IN_CHANNEL_NBR 1 /* Mono = 1, Stereo = 2 */ 00157 #define DEFAULT_AUDIO_IN_VOLUME 64 00158 00159 /* PDM buffer input size */ 00160 #define INTERNAL_BUFF_SIZE 128*DEFAULT_AUDIO_IN_FREQ/16000*DEFAULT_AUDIO_IN_CHANNEL_NBR 00161 /* PCM buffer output size */ 00162 #define PCM_OUT_SIZE DEFAULT_AUDIO_IN_FREQ/1000 00163 #define CHANNEL_DEMUX_MASK 0x55 00164 00165 /*------------------------------------------------------------------------------ 00166 OPTIONAL Configuration defines parameters 00167 ------------------------------------------------------------------------------*/ 00168 00169 /** 00170 * @} 00171 */ 00172 00173 /** @defgroup STM32F401_DISCOVERY_AUDIO_Exported_Variables STM32F401 DISCOVERY AUDIO Exported Variables 00174 * @{ 00175 */ 00176 extern __IO uint16_t AudioInVolume; 00177 /** 00178 * @} 00179 */ 00180 00181 /** @defgroup STM32F401_DISCOVERY_AUDIO_Exported_Macros STM32F401 DISCOVERY AUDIO Exported Macros 00182 * @{ 00183 */ 00184 #define DMA_MAX(_X_) (((_X_) <= DMA_MAX_SZE)? (_X_):DMA_MAX_SZE) 00185 /** 00186 * @} 00187 */ 00188 00189 /** @defgroup STM32F401_DISCOVERY_AUDIO_OUT_Exported_Functions STM32F401 DISCOVERY AUDIO OUT Exported Functions 00190 * @{ 00191 */ 00192 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00193 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size); 00194 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00195 uint8_t BSP_AUDIO_OUT_Pause(void); 00196 uint8_t BSP_AUDIO_OUT_Resume(void); 00197 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option); 00198 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume); 00199 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq); 00200 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd); 00201 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output); 00202 00203 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00204 /* This function is called when the requested data has been completely transferred. */ 00205 void BSP_AUDIO_OUT_TransferComplete_CallBack(void); 00206 00207 /* This function is called when half of the requested buffer has been transferred. */ 00208 void BSP_AUDIO_OUT_HalfTransfer_CallBack(void); 00209 00210 /* This function is called when an Interrupt due to transfer error on or peripheral 00211 error occurs. */ 00212 void BSP_AUDIO_OUT_Error_CallBack(void); 00213 00214 /* These function can be modified in case the current settings (e.g. DMA stream) 00215 need to be changed for specific application needs */ 00216 void BSP_AUDIO_OUT_ClockConfig(I2S_HandleTypeDef *hi2s, uint32_t AudioFreq, void *Params); 00217 void BSP_AUDIO_OUT_MspInit(I2S_HandleTypeDef *hi2s, void *Params); 00218 void BSP_AUDIO_OUT_MspDeInit(I2S_HandleTypeDef *hi2s, void *Params); 00219 00220 /** 00221 * @} 00222 */ 00223 00224 /** @defgroup STM32F401_DISCOVERY_AUDIO_IN_Exported_Functions STM32F401 DISCOVERY AUDIO IN Exported Functions 00225 * @{ 00226 */ 00227 uint8_t BSP_AUDIO_IN_Init(uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr); 00228 uint8_t BSP_AUDIO_IN_Record(uint16_t *pData, uint32_t Size); 00229 uint8_t BSP_AUDIO_IN_Stop(void); 00230 uint8_t BSP_AUDIO_IN_Pause(void); 00231 uint8_t BSP_AUDIO_IN_Resume(void); 00232 uint8_t BSP_AUDIO_IN_SetVolume(uint8_t Volume); 00233 uint8_t BSP_AUDIO_IN_PDMToPCM(uint16_t *PDMBuf, uint16_t *PCMBuf); 00234 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00235 /* This function should be implemented by the user application. 00236 It is called into this driver when the current buffer is filled to prepare the next 00237 buffer pointer and its size. */ 00238 void BSP_AUDIO_IN_TransferComplete_CallBack(void); 00239 void BSP_AUDIO_IN_HalfTransfer_CallBack(void); 00240 00241 /* This function is called when an Interrupt due to transfer error on or peripheral 00242 error occurs. */ 00243 void BSP_AUDIO_IN_Error_Callback(void); 00244 00245 /* These function can be modified in case the current settings (e.g. DMA stream) 00246 need to be changed for specific application needs */ 00247 void BSP_AUDIO_IN_ClockConfig(I2S_HandleTypeDef *hi2s, uint32_t AudioFreq, void *Params); 00248 void BSP_AUDIO_IN_MspInit(I2S_HandleTypeDef *hi2s, void *Params); 00249 void BSP_AUDIO_IN_MspDeInit(I2S_HandleTypeDef *hi2s, void *Params); 00250 00251 /** 00252 * @} 00253 */ 00254 00255 /** 00256 * @} 00257 */ 00258 00259 /** 00260 * @} 00261 */ 00262 00263 /** 00264 * @} 00265 */ 00266 00267 #ifdef __cplusplus 00268 } 00269 #endif 00270 00271 #endif /* __STM32F401_DISCOVERY_AUDIO_H */ 00272 00273 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jan 19 2017 16:53:47 for STM32F401-Discovery BSP User Manual by
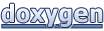