STM32F4-Discovery BSP User Manual
|
stm32f4_discovery.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f4_discovery.h 00004 * @author MCD Application Team 00005 * @version V2.1.2 00006 * @date 31-January-2017 00007 * @brief This file contains definitions for STM32F4-Discovery Kit's Leds and 00008 * push-button hardware resources. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32F4_DISCOVERY_H 00041 #define __STM32F4_DISCOVERY_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32f4xx_hal.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32F4_DISCOVERY 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM32F4_DISCOVERY_LOW_LEVEL 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_Exported_Types STM32F4 DISCOVERY LOW LEVEL_Exported_Types 00063 * @{ 00064 */ 00065 typedef enum 00066 { 00067 LED4 = 0, 00068 LED3 = 1, 00069 LED5 = 2, 00070 LED6 = 3 00071 } Led_TypeDef; 00072 00073 typedef enum 00074 { 00075 BUTTON_KEY = 0, 00076 } Button_TypeDef; 00077 00078 typedef enum 00079 { 00080 BUTTON_MODE_GPIO = 0, 00081 BUTTON_MODE_EXTI = 1 00082 } ButtonMode_TypeDef; 00083 /** 00084 * @} 00085 */ 00086 00087 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_Exported_Constants STM32F4 DISCOVERY LOW LEVEL Exported Constants 00088 * @{ 00089 */ 00090 00091 /** 00092 * @brief Define for STM32F4_DISCOVERY board 00093 */ 00094 #if !defined (USE_STM32F4_DISCO) 00095 #define USE_STM32F4_DISCO 00096 #endif 00097 00098 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_LED STM32F4 DISCOVERY LOW LEVEL LED 00099 * @{ 00100 */ 00101 #define LEDn 4 00102 00103 #define LED4_PIN GPIO_PIN_12 00104 #define LED4_GPIO_PORT GPIOD 00105 #define LED4_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00106 #define LED4_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00107 00108 #define LED3_PIN GPIO_PIN_13 00109 #define LED3_GPIO_PORT GPIOD 00110 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00111 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00112 00113 #define LED5_PIN GPIO_PIN_14 00114 #define LED5_GPIO_PORT GPIOD 00115 #define LED5_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00116 #define LED5_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00117 00118 #define LED6_PIN GPIO_PIN_15 00119 #define LED6_GPIO_PORT GPIOD 00120 #define LED6_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00121 #define LED6_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00122 00123 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do{if((__INDEX__) == 0) LED4_GPIO_CLK_ENABLE(); else \ 00124 if((__INDEX__) == 1) LED3_GPIO_CLK_ENABLE(); else \ 00125 if((__INDEX__) == 2) LED5_GPIO_CLK_ENABLE(); else \ 00126 if((__INDEX__) == 3) LED6_GPIO_CLK_ENABLE(); \ 00127 }while(0) 00128 00129 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) do{if((__INDEX__) == 0) LED4_GPIO_CLK_DISABLE(); else \ 00130 if((__INDEX__) == 1) LED3_GPIO_CLK_DISABLE(); else \ 00131 if((__INDEX__) == 2) LED5_GPIO_CLK_DISABLE(); else \ 00132 if((__INDEX__) == 3) LED6_GPIO_CLK_DISABLE(); \ 00133 }while(0) 00134 /** 00135 * @} 00136 */ 00137 00138 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_BUTTON STM32F4 DISCOVERY LOW LEVEL BUTTON 00139 * @{ 00140 */ 00141 #define BUTTONn 1 00142 00143 /** 00144 * @brief Wakeup push-button 00145 */ 00146 #define KEY_BUTTON_PIN GPIO_PIN_0 00147 #define KEY_BUTTON_GPIO_PORT GPIOA 00148 #define KEY_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00149 #define KEY_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00150 #define KEY_BUTTON_EXTI_IRQn EXTI0_IRQn 00151 00152 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) do{if((__INDEX__) == 0) KEY_BUTTON_GPIO_CLK_ENABLE(); \ 00153 }while(0) 00154 00155 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) do{if((__INDEX__) == 0) KEY_BUTTON_GPIO_CLK_DISABLE(); \ 00156 }while(0) 00157 /** 00158 * @} 00159 */ 00160 00161 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_BUS STM32F4 DISCOVERY LOW LEVEL BUS 00162 * @{ 00163 */ 00164 00165 /*############################### SPI1 #######################################*/ 00166 #define DISCOVERY_SPIx SPI1 00167 #define DISCOVERY_SPIx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00168 #define DISCOVERY_SPIx_GPIO_PORT GPIOA /* GPIOA */ 00169 #define DISCOVERY_SPIx_AF GPIO_AF5_SPI1 00170 #define DISCOVERY_SPIx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00171 #define DISCOVERY_SPIx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00172 #define DISCOVERY_SPIx_SCK_PIN GPIO_PIN_5 /* PA.05 */ 00173 #define DISCOVERY_SPIx_MISO_PIN GPIO_PIN_6 /* PA.06 */ 00174 #define DISCOVERY_SPIx_MOSI_PIN GPIO_PIN_7 /* PA.07 */ 00175 00176 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00177 on accurate values, they just guarantee that the application will not remain 00178 stuck if the SPI communication is corrupted. 00179 You may modify these timeout values depending on CPU frequency and application 00180 conditions (interrupts routines ...). */ 00181 #define SPIx_TIMEOUT_MAX 0x1000 /*<! The value of the maximal timeout for BUS waiting loops */ 00182 00183 00184 /*############################# I2C1 #########################################*/ 00185 /* I2C clock speed configuration (in Hz) */ 00186 #ifndef BSP_I2C_SPEED 00187 #define BSP_I2C_SPEED 100000 00188 #endif /* BSP_I2C_SPEED */ 00189 00190 /* I2C peripheral configuration defines (control interface of the audio codec) */ 00191 #define DISCOVERY_I2Cx I2C1 00192 #define DISCOVERY_I2Cx_CLK_ENABLE() __HAL_RCC_I2C1_CLK_ENABLE() 00193 #define DISCOVERY_I2Cx_SCL_SDA_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00194 #define DISCOVERY_I2Cx_SCL_SDA_AF GPIO_AF4_I2C1 00195 #define DISCOVERY_I2Cx_SCL_SDA_GPIO_PORT GPIOB 00196 #define DISCOVERY_I2Cx_SCL_PIN GPIO_PIN_6 00197 #define DISCOVERY_I2Cx_SDA_PIN GPIO_PIN_9 00198 00199 #define DISCOVERY_I2Cx_FORCE_RESET() __HAL_RCC_I2C1_FORCE_RESET() 00200 #define DISCOVERY_I2Cx_RELEASE_RESET() __HAL_RCC_I2C1_RELEASE_RESET() 00201 00202 /* I2C interrupt requests */ 00203 #define DISCOVERY_I2Cx_EV_IRQn I2C1_EV_IRQn 00204 #define DISCOVERY_I2Cx_ER_IRQn I2C1_ER_IRQn 00205 00206 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00207 on accurate values, they just guarantee that the application will not remain 00208 stuck if the SPI communication is corrupted. 00209 You may modify these timeout values depending on CPU frequency and application 00210 conditions (interrupts routines ...). */ 00211 #define I2Cx_TIMEOUT_MAX 0x1000 /*<! The value of the maximal timeout for BUS waiting loops */ 00212 00213 00214 /*############################# ACCELEROMETER ################################*/ 00215 /* Read/Write command */ 00216 #define READWRITE_CMD ((uint8_t)0x80) 00217 /* Multiple byte read/write command */ 00218 #define MULTIPLEBYTE_CMD ((uint8_t)0x40) 00219 /* Dummy Byte Send by the SPI Master device in order to generate the Clock to the Slave device */ 00220 #define DUMMY_BYTE ((uint8_t)0x00) 00221 00222 /* Chip Select macro definition */ 00223 #define ACCELERO_CS_LOW() HAL_GPIO_WritePin(ACCELERO_CS_GPIO_PORT, ACCELERO_CS_PIN, GPIO_PIN_RESET) 00224 #define ACCELERO_CS_HIGH() HAL_GPIO_WritePin(ACCELERO_CS_GPIO_PORT, ACCELERO_CS_PIN, GPIO_PIN_SET) 00225 00226 /** 00227 * @brief ACCELEROMETER Interface pins 00228 */ 00229 #define ACCELERO_CS_PIN GPIO_PIN_3 /* PE.03 */ 00230 #define ACCELERO_CS_GPIO_PORT GPIOE /* GPIOE */ 00231 #define ACCELERO_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOE_CLK_ENABLE() 00232 #define ACCELERO_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOE_CLK_DISABLE() 00233 #define ACCELERO_INT_GPIO_PORT GPIOE /* GPIOE */ 00234 #define ACCELERO_INT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOE_CLK_ENABLE() 00235 #define ACCELERO_INT_GPIO_CLK_DISABLE() __HAL_RCC_GPIOE_CLK_DISABLE() 00236 #define ACCELERO_INT1_PIN GPIO_PIN_0 /* PE.00 */ 00237 #define ACCELERO_INT1_EXTI_IRQn EXTI0_IRQn 00238 #define ACCELERO_INT2_PIN GPIO_PIN_1 /* PE.01 */ 00239 #define ACCELERO_INT2_EXTI_IRQn EXTI1_IRQn 00240 /** 00241 * @} 00242 */ 00243 00244 00245 /*############################### AUDIO ######################################*/ 00246 /** 00247 * @brief AUDIO I2C Interface pins 00248 */ 00249 #define AUDIO_I2C_ADDRESS 0x94 00250 00251 /* Audio Reset Pin definition */ 00252 #define AUDIO_RESET_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00253 #define AUDIO_RESET_PIN GPIO_PIN_4 00254 #define AUDIO_RESET_GPIO GPIOD 00255 /** 00256 * @} 00257 */ 00258 00259 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_Exported_Macros STM32F4 DISCOVERY LOW LEVEL Exported Macros 00260 * @{ 00261 */ 00262 /** 00263 * @} 00264 */ 00265 00266 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_Exported_Functions STM32F4 DISCOVERY LOW LEVEL Exported Functions 00267 * @{ 00268 */ 00269 uint32_t BSP_GetVersion(void); 00270 void BSP_LED_Init(Led_TypeDef Led); 00271 void BSP_LED_On(Led_TypeDef Led); 00272 void BSP_LED_Off(Led_TypeDef Led); 00273 void BSP_LED_Toggle(Led_TypeDef Led); 00274 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode); 00275 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00276 00277 /** 00278 * @} 00279 */ 00280 00281 /** 00282 * @} 00283 */ 00284 00285 /** 00286 * @} 00287 */ 00288 00289 /** 00290 * @} 00291 */ 00292 00293 /** 00294 * @} 00295 */ 00296 00297 #ifdef __cplusplus 00298 } 00299 #endif 00300 00301 #endif /* __STM32F4_DISCOVERY_H */ 00302 00303 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jan 19 2017 15:34:14 for STM32F4-Discovery BSP User Manual by
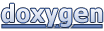