STM32F4-Discovery BSP User Manual
|
stm32f4_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f4_discovery.c 00004 * @author MCD Application Team 00005 * @version V2.1.2 00006 * @date 31-January-2017 00007 * @brief This file provides set of firmware functions to manage Leds and 00008 * push-button available on STM32F4-Discovery Kit from STMicroelectronics. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 /* Includes ------------------------------------------------------------------*/ 00039 #include "stm32f4_discovery.h" 00040 00041 /** @defgroup BSP BSP 00042 * @{ 00043 */ 00044 00045 /** @defgroup STM32F4_DISCOVERY STM32F4 DISCOVERY 00046 * @{ 00047 */ 00048 00049 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL STM32F4 DISCOVERY LOW LEVEL 00050 * @brief This file provides set of firmware functions to manage Leds and push-button 00051 * available on STM32F4-Discovery Kit from STMicroelectronics. 00052 * @{ 00053 */ 00054 00055 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_Private_TypesDefinitions STM32F4 DISCOVERY LOW LEVEL Private TypesDefinitions 00056 * @{ 00057 */ 00058 /** 00059 * @} 00060 */ 00061 00062 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_Private_Defines STM32F4 DISCOVERY LOW LEVEL Private Defines 00063 * @{ 00064 */ 00065 00066 /** 00067 * @brief STM32F4 DISCO BSP Driver version number V2.1.2 00068 */ 00069 #define __STM32F4_DISCO_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00070 #define __STM32F4_DISCO_BSP_VERSION_SUB1 (0x01) /*!< [23:16] sub1 version */ 00071 #define __STM32F4_DISCO_BSP_VERSION_SUB2 (0x02) /*!< [15:8] sub2 version */ 00072 #define __STM32F4_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00073 #define __STM32F4_DISCO_BSP_VERSION ((__STM32F4_DISCO_BSP_VERSION_MAIN << 24)\ 00074 |(__STM32F4_DISCO_BSP_VERSION_SUB1 << 16)\ 00075 |(__STM32F4_DISCO_BSP_VERSION_SUB2 << 8 )\ 00076 |(__STM32F4_DISCO_BSP_VERSION_RC)) 00077 /** 00078 * @} 00079 */ 00080 00081 00082 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_Private_Macros STM32F4 DISCOVERY LOW LEVEL Private Macros 00083 * @{ 00084 */ 00085 /** 00086 * @} 00087 */ 00088 00089 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_Private_Variables STM32F4 DISCOVERY LOW LEVEL Private Variables 00090 * @{ 00091 */ 00092 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED4_GPIO_PORT, 00093 LED3_GPIO_PORT, 00094 LED5_GPIO_PORT, 00095 LED6_GPIO_PORT}; 00096 const uint16_t GPIO_PIN[LEDn] = {LED4_PIN, 00097 LED3_PIN, 00098 LED5_PIN, 00099 LED6_PIN}; 00100 00101 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {KEY_BUTTON_GPIO_PORT}; 00102 const uint16_t BUTTON_PIN[BUTTONn] = {KEY_BUTTON_PIN}; 00103 const uint8_t BUTTON_IRQn[BUTTONn] = {KEY_BUTTON_EXTI_IRQn}; 00104 00105 uint32_t I2cxTimeout = I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00106 uint32_t SpixTimeout = SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00107 00108 static SPI_HandleTypeDef SpiHandle; 00109 static I2C_HandleTypeDef I2cHandle; 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_Private_FunctionPrototypes STM32F4 DISCOVERY LOW LEVEL Private FunctionPrototypes 00115 * @{ 00116 */ 00117 /** 00118 * @} 00119 */ 00120 00121 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_Private_Functions STM32F4 DISCOVERY LOW LEVEL Private Functions 00122 * @{ 00123 */ 00124 static void I2Cx_Init(void); 00125 static void I2Cx_WriteData(uint8_t Addr, uint8_t Reg, uint8_t Value); 00126 static uint8_t I2Cx_ReadData(uint8_t Addr, uint8_t Reg); 00127 static void I2Cx_MspInit(void); 00128 static void I2Cx_Error(uint8_t Addr); 00129 00130 static void SPIx_Init(void); 00131 static void SPIx_MspInit(void); 00132 static uint8_t SPIx_WriteRead(uint8_t Byte); 00133 static void SPIx_Error(void); 00134 00135 /* Link functions for Accelerometer peripheral */ 00136 void ACCELERO_IO_Init(void); 00137 void ACCELERO_IO_ITConfig(void); 00138 void ACCELERO_IO_Write(uint8_t *pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite); 00139 void ACCELERO_IO_Read(uint8_t *pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead); 00140 00141 /* Link functions for Audio peripheral */ 00142 void AUDIO_IO_Init(void); 00143 void AUDIO_IO_DeInit(void); 00144 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00145 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00146 /** 00147 * @} 00148 */ 00149 00150 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_LED_Functions STM32F4 DISCOVERY LOW LEVEL LED Functions 00151 * @{ 00152 */ 00153 00154 /** 00155 * @brief This method returns the STM32F4 DISCO BSP Driver revision 00156 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00157 */ 00158 uint32_t BSP_GetVersion(void) 00159 { 00160 return __STM32F4_DISCO_BSP_VERSION; 00161 } 00162 00163 /** 00164 * @brief Configures LED GPIO. 00165 * @param Led: Specifies the Led to be configured. 00166 * This parameter can be one of following parameters: 00167 * @arg LED4 00168 * @arg LED3 00169 * @arg LED5 00170 * @arg LED6 00171 */ 00172 void BSP_LED_Init(Led_TypeDef Led) 00173 { 00174 GPIO_InitTypeDef GPIO_InitStruct; 00175 00176 /* Enable the GPIO_LED Clock */ 00177 LEDx_GPIO_CLK_ENABLE(Led); 00178 00179 /* Configure the GPIO_LED pin */ 00180 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00181 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00182 GPIO_InitStruct.Pull = GPIO_PULLUP; 00183 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00184 00185 HAL_GPIO_Init(GPIO_PORT[Led], &GPIO_InitStruct); 00186 00187 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00188 } 00189 00190 /** 00191 * @brief Turns selected LED On. 00192 * @param Led: Specifies the Led to be set on. 00193 * This parameter can be one of following parameters: 00194 * @arg LED4 00195 * @arg LED3 00196 * @arg LED5 00197 * @arg LED6 00198 */ 00199 void BSP_LED_On(Led_TypeDef Led) 00200 { 00201 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00202 } 00203 00204 /** 00205 * @brief Turns selected LED Off. 00206 * @param Led: Specifies the Led to be set off. 00207 * This parameter can be one of following parameters: 00208 * @arg LED4 00209 * @arg LED3 00210 * @arg LED5 00211 * @arg LED6 00212 */ 00213 void BSP_LED_Off(Led_TypeDef Led) 00214 { 00215 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00216 } 00217 00218 /** 00219 * @brief Toggles the selected LED. 00220 * @param Led: Specifies the Led to be toggled. 00221 * This parameter can be one of following parameters: 00222 * @arg LED4 00223 * @arg LED3 00224 * @arg LED5 00225 * @arg LED6 00226 */ 00227 void BSP_LED_Toggle(Led_TypeDef Led) 00228 { 00229 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00230 } 00231 00232 /** 00233 * @} 00234 */ 00235 00236 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_BUTTON_Functions STM32F4 DISCOVERY LOW LEVEL BUTTON Functions 00237 * @{ 00238 */ 00239 00240 /** 00241 * @brief Configures Button GPIO and EXTI Line. 00242 * @param Button: Specifies the Button to be configured. 00243 * This parameter should be: BUTTON_KEY 00244 * @param Mode: Specifies Button mode. 00245 * This parameter can be one of following parameters: 00246 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00247 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00248 * generation capability 00249 */ 00250 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode) 00251 { 00252 GPIO_InitTypeDef GPIO_InitStruct; 00253 00254 /* Enable the BUTTON Clock */ 00255 BUTTONx_GPIO_CLK_ENABLE(Button); 00256 00257 if (Mode == BUTTON_MODE_GPIO) 00258 { 00259 /* Configure Button pin as input */ 00260 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00261 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00262 GPIO_InitStruct.Pull = GPIO_NOPULL; 00263 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00264 00265 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00266 } 00267 00268 if (Mode == BUTTON_MODE_EXTI) 00269 { 00270 /* Configure Button pin as input with External interrupt */ 00271 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00272 GPIO_InitStruct.Pull = GPIO_NOPULL; 00273 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00274 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00275 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00276 00277 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00278 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00279 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00280 } 00281 } 00282 00283 /** 00284 * @brief Returns the selected Button state. 00285 * @param Button: Specifies the Button to be checked. 00286 * This parameter should be: BUTTON_KEY 00287 * @retval The Button GPIO pin value. 00288 */ 00289 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00290 { 00291 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00292 } 00293 00294 /** 00295 * @} 00296 */ 00297 00298 /** @defgroup STM32F4_DISCOVERY_LOW_LEVEL_BUS_Functions STM32F4 DISCOVERY LOW LEVEL BUS Functions 00299 * @{ 00300 */ 00301 00302 /******************************************************************************* 00303 BUS OPERATIONS 00304 *******************************************************************************/ 00305 00306 /******************************* SPI Routines *********************************/ 00307 00308 /** 00309 * @brief SPIx Bus initialization 00310 */ 00311 static void SPIx_Init(void) 00312 { 00313 if(HAL_SPI_GetState(&SpiHandle) == HAL_SPI_STATE_RESET) 00314 { 00315 /* SPI configuration -----------------------------------------------------*/ 00316 SpiHandle.Instance = DISCOVERY_SPIx; 00317 SpiHandle.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_16; 00318 SpiHandle.Init.Direction = SPI_DIRECTION_2LINES; 00319 SpiHandle.Init.CLKPhase = SPI_PHASE_1EDGE; 00320 SpiHandle.Init.CLKPolarity = SPI_POLARITY_LOW; 00321 SpiHandle.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLED; 00322 SpiHandle.Init.CRCPolynomial = 7; 00323 SpiHandle.Init.DataSize = SPI_DATASIZE_8BIT; 00324 SpiHandle.Init.FirstBit = SPI_FIRSTBIT_MSB; 00325 SpiHandle.Init.NSS = SPI_NSS_SOFT; 00326 SpiHandle.Init.TIMode = SPI_TIMODE_DISABLED; 00327 SpiHandle.Init.Mode = SPI_MODE_MASTER; 00328 00329 SPIx_MspInit(); 00330 HAL_SPI_Init(&SpiHandle); 00331 } 00332 } 00333 00334 /** 00335 * @brief Sends a Byte through the SPI interface and return the Byte received 00336 * from the SPI bus. 00337 * @param Byte: Byte send. 00338 * @retval The received byte value 00339 */ 00340 static uint8_t SPIx_WriteRead(uint8_t Byte) 00341 { 00342 uint8_t receivedbyte = 0; 00343 00344 /* Send a Byte through the SPI peripheral */ 00345 /* Read byte from the SPI bus */ 00346 if(HAL_SPI_TransmitReceive(&SpiHandle, (uint8_t*) &Byte, (uint8_t*) &receivedbyte, 1, SpixTimeout) != HAL_OK) 00347 { 00348 SPIx_Error(); 00349 } 00350 00351 return receivedbyte; 00352 } 00353 00354 /** 00355 * @brief SPIx error treatment function. 00356 */ 00357 static void SPIx_Error(void) 00358 { 00359 /* De-initialize the SPI communication bus */ 00360 HAL_SPI_DeInit(&SpiHandle); 00361 00362 /* Re-Initialize the SPI communication bus */ 00363 SPIx_Init(); 00364 } 00365 00366 /** 00367 * @brief SPI MSP Init. 00368 */ 00369 static void SPIx_MspInit(void) 00370 { 00371 GPIO_InitTypeDef GPIO_InitStructure; 00372 00373 /* Enable the SPI peripheral */ 00374 DISCOVERY_SPIx_CLK_ENABLE(); 00375 00376 /* Enable SCK, MOSI and MISO GPIO clocks */ 00377 DISCOVERY_SPIx_GPIO_CLK_ENABLE(); 00378 00379 /* SPI SCK, MOSI, MISO pin configuration */ 00380 GPIO_InitStructure.Pin = (DISCOVERY_SPIx_SCK_PIN | DISCOVERY_SPIx_MISO_PIN | DISCOVERY_SPIx_MOSI_PIN); 00381 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 00382 GPIO_InitStructure.Pull = GPIO_PULLDOWN; 00383 GPIO_InitStructure.Speed = GPIO_SPEED_MEDIUM; 00384 GPIO_InitStructure.Alternate = DISCOVERY_SPIx_AF; 00385 HAL_GPIO_Init(DISCOVERY_SPIx_GPIO_PORT, &GPIO_InitStructure); 00386 } 00387 00388 /******************************* I2C Routines**********************************/ 00389 /** 00390 * @brief Configures I2C interface. 00391 */ 00392 static void I2Cx_Init(void) 00393 { 00394 if(HAL_I2C_GetState(&I2cHandle) == HAL_I2C_STATE_RESET) 00395 { 00396 /* DISCOVERY_I2Cx peripheral configuration */ 00397 I2cHandle.Init.ClockSpeed = BSP_I2C_SPEED; 00398 I2cHandle.Init.DutyCycle = I2C_DUTYCYCLE_2; 00399 I2cHandle.Init.OwnAddress1 = 0x33; 00400 I2cHandle.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00401 I2cHandle.Instance = DISCOVERY_I2Cx; 00402 00403 /* Init the I2C */ 00404 I2Cx_MspInit(); 00405 HAL_I2C_Init(&I2cHandle); 00406 } 00407 } 00408 00409 /** 00410 * @brief Write a value in a register of the device through BUS. 00411 * @param Addr: Device address on BUS Bus. 00412 * @param Reg: The target register address to write 00413 * @param Value: The target register value to be written 00414 * @retval HAL status 00415 */ 00416 static void I2Cx_WriteData(uint8_t Addr, uint8_t Reg, uint8_t Value) 00417 { 00418 HAL_StatusTypeDef status = HAL_OK; 00419 00420 status = HAL_I2C_Mem_Write(&I2cHandle, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2cxTimeout); 00421 00422 /* Check the communication status */ 00423 if(status != HAL_OK) 00424 { 00425 /* Execute user timeout callback */ 00426 I2Cx_Error(Addr); 00427 } 00428 } 00429 00430 /** 00431 * @brief Read a register of the device through BUS 00432 * @param Addr: Device address on BUS 00433 * @param Reg: The target register address to read 00434 * @retval HAL status 00435 */ 00436 static uint8_t I2Cx_ReadData(uint8_t Addr, uint8_t Reg) 00437 { 00438 HAL_StatusTypeDef status = HAL_OK; 00439 uint8_t value = 0; 00440 00441 status = HAL_I2C_Mem_Read(&I2cHandle, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &value, 1,I2cxTimeout); 00442 00443 /* Check the communication status */ 00444 if(status != HAL_OK) 00445 { 00446 /* Execute user timeout callback */ 00447 I2Cx_Error(Addr); 00448 } 00449 return value; 00450 } 00451 00452 /** 00453 * @brief Manages error callback by re-initializing I2C. 00454 * @param Addr: I2C Address 00455 */ 00456 static void I2Cx_Error(uint8_t Addr) 00457 { 00458 /* De-initialize the I2C communication bus */ 00459 HAL_I2C_DeInit(&I2cHandle); 00460 00461 /* Re-Initialize the I2C communication bus */ 00462 I2Cx_Init(); 00463 } 00464 00465 /** 00466 * @brief I2C MSP Initialization 00467 */ 00468 static void I2Cx_MspInit(void) 00469 { 00470 GPIO_InitTypeDef GPIO_InitStruct; 00471 00472 /* Enable I2C GPIO clocks */ 00473 DISCOVERY_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00474 00475 /* DISCOVERY_I2Cx SCL and SDA pins configuration ---------------------------*/ 00476 GPIO_InitStruct.Pin = DISCOVERY_I2Cx_SCL_PIN | DISCOVERY_I2Cx_SDA_PIN; 00477 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00478 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00479 GPIO_InitStruct.Pull = GPIO_NOPULL; 00480 GPIO_InitStruct.Alternate = DISCOVERY_I2Cx_SCL_SDA_AF; 00481 HAL_GPIO_Init(DISCOVERY_I2Cx_SCL_SDA_GPIO_PORT, &GPIO_InitStruct); 00482 00483 /* Enable the DISCOVERY_I2Cx peripheral clock */ 00484 DISCOVERY_I2Cx_CLK_ENABLE(); 00485 00486 /* Force the I2C peripheral clock reset */ 00487 DISCOVERY_I2Cx_FORCE_RESET(); 00488 00489 /* Release the I2C peripheral clock reset */ 00490 DISCOVERY_I2Cx_RELEASE_RESET(); 00491 00492 /* Enable and set I2Cx Interrupt to the highest priority */ 00493 HAL_NVIC_SetPriority(DISCOVERY_I2Cx_EV_IRQn, 0, 0); 00494 HAL_NVIC_EnableIRQ(DISCOVERY_I2Cx_EV_IRQn); 00495 00496 /* Enable and set I2Cx Interrupt to the highest priority */ 00497 HAL_NVIC_SetPriority(DISCOVERY_I2Cx_ER_IRQn, 0, 0); 00498 HAL_NVIC_EnableIRQ(DISCOVERY_I2Cx_ER_IRQn); 00499 } 00500 00501 /******************************************************************************* 00502 LINK OPERATIONS 00503 *******************************************************************************/ 00504 00505 /***************************** LINK ACCELEROMETER *****************************/ 00506 00507 /** 00508 * @brief Configures the Accelerometer SPI interface. 00509 */ 00510 void ACCELERO_IO_Init(void) 00511 { 00512 GPIO_InitTypeDef GPIO_InitStructure; 00513 00514 /* Configure the Accelerometer Control pins --------------------------------*/ 00515 /* Enable CS GPIO clock and configure GPIO pin for Accelerometer Chip select */ 00516 ACCELERO_CS_GPIO_CLK_ENABLE(); 00517 00518 /* Configure GPIO PIN for LIS Chip select */ 00519 GPIO_InitStructure.Pin = ACCELERO_CS_PIN; 00520 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 00521 GPIO_InitStructure.Pull = GPIO_NOPULL; 00522 GPIO_InitStructure.Speed = GPIO_SPEED_MEDIUM; 00523 HAL_GPIO_Init(ACCELERO_CS_GPIO_PORT, &GPIO_InitStructure); 00524 00525 /* Deselect: Chip Select high */ 00526 ACCELERO_CS_HIGH(); 00527 00528 SPIx_Init(); 00529 } 00530 00531 /** 00532 * @brief Configures the Accelerometer INT2. 00533 * EXTI0 is already used by user button so INT1 is not configured here. 00534 */ 00535 void ACCELERO_IO_ITConfig(void) 00536 { 00537 GPIO_InitTypeDef GPIO_InitStructure; 00538 00539 /* Enable INT2 GPIO clock and configure GPIO PINs to detect Interrupts */ 00540 ACCELERO_INT_GPIO_CLK_ENABLE(); 00541 00542 /* Configure GPIO PINs to detect Interrupts */ 00543 GPIO_InitStructure.Pin = ACCELERO_INT2_PIN; 00544 GPIO_InitStructure.Mode = GPIO_MODE_IT_RISING; 00545 GPIO_InitStructure.Speed = GPIO_SPEED_FAST; 00546 GPIO_InitStructure.Pull = GPIO_NOPULL; 00547 HAL_GPIO_Init(ACCELERO_INT_GPIO_PORT, &GPIO_InitStructure); 00548 00549 /* Enable and set Accelerometer INT2 to the lowest priority */ 00550 HAL_NVIC_SetPriority((IRQn_Type)ACCELERO_INT2_EXTI_IRQn, 0x0F, 0); 00551 HAL_NVIC_EnableIRQ((IRQn_Type)ACCELERO_INT2_EXTI_IRQn); 00552 } 00553 00554 /** 00555 * @brief Writes one byte to the Accelerometer. 00556 * @param pBuffer: pointer to the buffer containing the data to be written to the Accelerometer. 00557 * @param WriteAddr: Accelerometer's internal address to write to. 00558 * @param NumByteToWrite: Number of bytes to write. 00559 */ 00560 void ACCELERO_IO_Write(uint8_t *pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite) 00561 { 00562 /* Configure the MS bit: 00563 - When 0, the address will remain unchanged in multiple read/write commands. 00564 - When 1, the address will be auto incremented in multiple read/write commands. 00565 */ 00566 if(NumByteToWrite > 0x01) 00567 { 00568 WriteAddr |= (uint8_t)MULTIPLEBYTE_CMD; 00569 } 00570 /* Set chip select Low at the start of the transmission */ 00571 ACCELERO_CS_LOW(); 00572 00573 /* Send the Address of the indexed register */ 00574 SPIx_WriteRead(WriteAddr); 00575 00576 /* Send the data that will be written into the device (MSB First) */ 00577 while(NumByteToWrite >= 0x01) 00578 { 00579 SPIx_WriteRead(*pBuffer); 00580 NumByteToWrite--; 00581 pBuffer++; 00582 } 00583 00584 /* Set chip select High at the end of the transmission */ 00585 ACCELERO_CS_HIGH(); 00586 } 00587 00588 /** 00589 * @brief Reads a block of data from the Accelerometer. 00590 * @param pBuffer: pointer to the buffer that receives the data read from the Accelerometer. 00591 * @param ReadAddr: Accelerometer's internal address to read from. 00592 * @param NumByteToRead: number of bytes to read from the Accelerometer. 00593 */ 00594 void ACCELERO_IO_Read(uint8_t *pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead) 00595 { 00596 if(NumByteToRead > 0x01) 00597 { 00598 ReadAddr |= (uint8_t)(READWRITE_CMD | MULTIPLEBYTE_CMD); 00599 } 00600 else 00601 { 00602 ReadAddr |= (uint8_t)READWRITE_CMD; 00603 } 00604 /* Set chip select Low at the start of the transmission */ 00605 ACCELERO_CS_LOW(); 00606 00607 /* Send the Address of the indexed register */ 00608 SPIx_WriteRead(ReadAddr); 00609 00610 /* Receive the data that will be read from the device (MSB First) */ 00611 while(NumByteToRead > 0x00) 00612 { 00613 /* Send dummy byte (0x00) to generate the SPI clock to ACCELEROMETER (Slave device) */ 00614 *pBuffer = SPIx_WriteRead(DUMMY_BYTE); 00615 NumByteToRead--; 00616 pBuffer++; 00617 } 00618 00619 /* Set chip select High at the end of the transmission */ 00620 ACCELERO_CS_HIGH(); 00621 } 00622 00623 /********************************* LINK AUDIO *********************************/ 00624 00625 /** 00626 * @brief Initializes Audio low level. 00627 */ 00628 void AUDIO_IO_Init(void) 00629 { 00630 GPIO_InitTypeDef GPIO_InitStruct; 00631 00632 /* Enable Reset GPIO Clock */ 00633 AUDIO_RESET_GPIO_CLK_ENABLE(); 00634 00635 /* Audio reset pin configuration */ 00636 GPIO_InitStruct.Pin = AUDIO_RESET_PIN; 00637 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00638 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00639 GPIO_InitStruct.Pull = GPIO_NOPULL; 00640 HAL_GPIO_Init(AUDIO_RESET_GPIO, &GPIO_InitStruct); 00641 00642 I2Cx_Init(); 00643 00644 /* Power Down the codec */ 00645 HAL_GPIO_WritePin(AUDIO_RESET_GPIO, AUDIO_RESET_PIN, GPIO_PIN_RESET); 00646 00647 /* Wait for a delay to insure registers erasing */ 00648 HAL_Delay(5); 00649 00650 /* Power on the codec */ 00651 HAL_GPIO_WritePin(AUDIO_RESET_GPIO, AUDIO_RESET_PIN, GPIO_PIN_SET); 00652 00653 /* Wait for a delay to insure registers erasing */ 00654 HAL_Delay(5); 00655 } 00656 00657 /** 00658 * @brief DeInitializes Audio low level. 00659 */ 00660 void AUDIO_IO_DeInit(void) 00661 { 00662 00663 } 00664 00665 /** 00666 * @brief Writes a single data. 00667 * @param Addr: I2C address 00668 * @param Reg: Reg address 00669 * @param Value: Data to be written 00670 */ 00671 void AUDIO_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) 00672 { 00673 I2Cx_WriteData(Addr, Reg, Value); 00674 } 00675 00676 /** 00677 * @brief Reads a single data. 00678 * @param Addr: I2C address 00679 * @param Reg: Reg address 00680 * @retval Data to be read 00681 */ 00682 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg) 00683 { 00684 return I2Cx_ReadData(Addr, Reg); 00685 } 00686 00687 /** 00688 * @} 00689 */ 00690 00691 /** 00692 * @} 00693 */ 00694 00695 /** 00696 * @} 00697 */ 00698 00699 /** 00700 * @} 00701 */ 00702 00703 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jan 19 2017 15:34:14 for STM32F4-Discovery BSP User Manual by
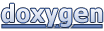