STM32F3xx_Nucleo_144 BSP User Manual
|
Functions | |
uint8_t | BSP_JOY_Init (void) |
Configures joystick available on adafruit 1.8" TFT shield managed through ADC to detect motion. | |
void | BSP_JOY_DeInit (void) |
DeInit joystick GPIOs. | |
JOYState_TypeDef | BSP_JOY_GetState (void) |
Returns the Joystick key pressed. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_CSState (uint8_t val) |
Set the SD_CS pin. | |
void | SD_IO_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) |
Write a byte on the SD. | |
uint8_t | SD_IO_WriteByte (uint8_t Data) |
Writes a byte on the SD. | |
void | LCD_IO_Init (void) |
Initializes the LCD. | |
void | LCD_IO_WriteReg (uint8_t LCDReg) |
Writes command to select the LCD register. | |
void | LCD_IO_WriteData (uint8_t Data) |
Writes data to select the LCD register. | |
void | LCD_IO_WriteMultipleData (uint8_t *pData, uint32_t Size) |
Write register value. | |
void | LCD_Delay (uint32_t Delay) |
Wait for loop in ms. | |
static void | ADCx_MspInit (ADC_HandleTypeDef *hadc) |
Initializes ADC MSP. | |
static void | ADCx_MspDeInit (ADC_HandleTypeDef *hadc) |
DeInitializes ADC MSP. | |
static void | ADCx_Init (void) |
Initializes ADC HAL. | |
static void | ADCx_DeInit (void) |
Initializes ADC HAL. |
Function Documentation
static void ADCx_DeInit | ( | void | ) | [static] |
Initializes ADC HAL.
- Return values:
-
None
Definition at line 784 of file stm32f3xx_nucleo_144.c.
References ADCx_MspDeInit(), hnucleo_Adc, and NUCLEO_ADCx.
Referenced by BSP_JOY_DeInit().
static void ADCx_Init | ( | void | ) | [static] |
Initializes ADC HAL.
- Return values:
-
None
Definition at line 746 of file stm32f3xx_nucleo_144.c.
References ADCx_MspInit(), hnucleo_Adc, and NUCLEO_ADCx.
Referenced by BSP_JOY_Init().
static void ADCx_MspDeInit | ( | ADC_HandleTypeDef * | hadc | ) | [static] |
DeInitializes ADC MSP.
- Parameters:
-
hadc
- Note:
- ADC DeInit does not disable the GPIO clock
- Return values:
-
None
Definition at line 726 of file stm32f3xx_nucleo_144.c.
References NUCLEO_ADCx_CLK_DISABLE, NUCLEO_ADCx_GPIO_PIN, and NUCLEO_ADCx_GPIO_PORT.
Referenced by ADCx_DeInit().
static void ADCx_MspInit | ( | ADC_HandleTypeDef * | hadc | ) | [static] |
Initializes ADC MSP.
- Parameters:
-
hadc
- Return values:
-
None
Definition at line 701 of file stm32f3xx_nucleo_144.c.
References NUCLEO_ADCx_CLK_ENABLE, NUCLEO_ADCx_GPIO_CLK_ENABLE, NUCLEO_ADCx_GPIO_PIN, and NUCLEO_ADCx_GPIO_PORT.
Referenced by ADCx_Init().
void BSP_JOY_DeInit | ( | void | ) |
DeInit joystick GPIOs.
- Note:
- JOY DeInit does not disable the Mfx, just set the Mfx pins in Off mode
- Return values:
-
None.
Definition at line 820 of file stm32f3xx_nucleo_144.c.
References ADCx_DeInit().
JOYState_TypeDef BSP_JOY_GetState | ( | void | ) |
Returns the Joystick key pressed.
- Note:
- To know which Joystick key is pressed we need to detect the voltage level on each key output
- None : 3.3 V / 4095
- SEL : 1.055 V / 1308
- DOWN : 0.71 V / 88
- LEFT : 3.0 V / 3720
- RIGHT : 0.595 V / 737
- UP : 1.65 V / 2046
- Return values:
-
JOYState_TypeDef,: Code of the Joystick key pressed.
Definition at line 837 of file stm32f3xx_nucleo_144.c.
References hnucleo_Adc, JOY_DOWN, JOY_LEFT, JOY_NONE, JOY_RIGHT, JOY_SEL, and JOY_UP.
uint8_t BSP_JOY_Init | ( | void | ) |
Configures joystick available on adafruit 1.8" TFT shield managed through ADC to detect motion.
- Return values:
-
Joystickstatus (0=> success, 1=> fail)
Definition at line 799 of file stm32f3xx_nucleo_144.c.
References ADCx_Init(), hnucleo_Adc, and sConfig.
void LCD_Delay | ( | uint32_t | Delay | ) |
Wait for loop in ms.
- Parameters:
-
Delay in ms.
- Return values:
-
None
Definition at line 688 of file stm32f3xx_nucleo_144.c.
void LCD_IO_Init | ( | void | ) |
Initializes the LCD.
- Return values:
-
None
Definition at line 559 of file stm32f3xx_nucleo_144.c.
References LCD_CS_GPIO_CLK_ENABLE, LCD_CS_GPIO_PORT, LCD_CS_HIGH, LCD_CS_PIN, LCD_DC_GPIO_CLK_ENABLE, LCD_DC_GPIO_PORT, LCD_DC_PIN, and SPIx_Init().
void LCD_IO_WriteData | ( | uint8_t | Data | ) |
Writes data to select the LCD register.
This function must be used after st7735_WriteReg() function
- Parameters:
-
Data data to write to the selected register.
- Return values:
-
None
Definition at line 611 of file stm32f3xx_nucleo_144.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_DC_HIGH, and SPIx_Write().
void LCD_IO_WriteMultipleData | ( | uint8_t * | pData, |
uint32_t | Size | ||
) |
Write register value.
- Parameters:
-
pData Pointer on the register value Size Size of byte to transmit to the register
- Return values:
-
None
Definition at line 632 of file stm32f3xx_nucleo_144.c.
References hnucleo_Spi, LCD_CS_HIGH, LCD_CS_LOW, LCD_DC_HIGH, and SPIx_Write().
void LCD_IO_WriteReg | ( | uint8_t | LCDReg | ) |
Writes command to select the LCD register.
- Parameters:
-
LCDReg Address of the selected register.
- Return values:
-
None
Definition at line 590 of file stm32f3xx_nucleo_144.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_DC_LOW, and SPIx_Write().
void SD_IO_CSState | ( | uint8_t | val | ) |
Set the SD_CS pin.
- Parameters:
-
val pin value.
- Return values:
-
None
Definition at line 514 of file stm32f3xx_nucleo_144.c.
References SD_CS_HIGH, and SD_CS_LOW.
void SD_IO_Init | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
- Return values:
-
None
Definition at line 478 of file stm32f3xx_nucleo_144.c.
References SD_CS_GPIO_CLK_ENABLE, SD_CS_GPIO_PORT, SD_CS_HIGH, SD_CS_PIN, SD_DUMMY_BYTE, SD_IO_WriteByte(), and SPIx_Init().
uint8_t SD_IO_WriteByte | ( | uint8_t | Data | ) |
Writes a byte on the SD.
- Parameters:
-
Data byte to send.
- Return values:
-
None
Definition at line 546 of file stm32f3xx_nucleo_144.c.
References SPIx_WriteReadData().
Referenced by SD_IO_Init().
void SD_IO_WriteReadData | ( | const uint8_t * | DataIn, |
uint8_t * | DataOut, | ||
uint16_t | DataLength | ||
) |
Write a byte on the SD.
- Parameters:
-
DataIn byte to send DataOut data out DataLength data length
- Return values:
-
None
Definition at line 533 of file stm32f3xx_nucleo_144.c.
References SPIx_WriteReadData().
Generated on Wed May 31 2017 11:02:21 for STM32F3xx_Nucleo_144 BSP User Manual by
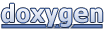