STM32F3xx_Nucleo_144 BSP User Manual
|
stm32f3xx_nucleo_144.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f3xx_nucleo_144.c 00004 * @author MCD Application Team 00005 * @brief This file provides set of firmware functions to manage: 00006 * - LEDs and push-button available on STM32F3XX-Nucleo-144 Kit 00007 * from STMicroelectronics 00008 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00009 * shield (reference ID 802) 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "stm32f3xx_nucleo_144.h" 00042 00043 /** @addtogroup BSP 00044 * @{ 00045 */ 00046 00047 /** @addtogroup STM32F3XX_NUCLEO_144 00048 * @brief This file provides set of firmware functions to manage Leds and push-button 00049 * available on STM32F3XX-NUCLEO Kit from STMicroelectronics. 00050 * @{ 00051 */ 00052 /** @addtogroup STM32F3XX_NUCLEO_144_Common 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM32F3XX_NUCLEO_144_Private_Constants 00057 * @{ 00058 */ 00059 00060 /** 00061 * @brief STM32F3xx NUCLEO BSP Driver version number V1.0.3 00062 */ 00063 #define __STM32F3xx_NUCLEO_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00064 #define __STM32F3xx_NUCLEO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00065 #define __STM32F3xx_NUCLEO_BSP_VERSION_SUB2 (0x03) /*!< [15:8] sub2 version */ 00066 #define __STM32F3xx_NUCLEO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00067 #define __STM32F3xx_NUCLEO_BSP_VERSION ((__STM32F3xx_NUCLEO_BSP_VERSION_MAIN << 24)\ 00068 |(__STM32F3xx_NUCLEO_BSP_VERSION_SUB1 << 16)\ 00069 |(__STM32F3xx_NUCLEO_BSP_VERSION_SUB2 << 8 )\ 00070 |(__STM32F3xx_NUCLEO_BSP_VERSION_RC)) 00071 00072 /** 00073 * @brief LINK SD Card 00074 */ 00075 #define SD_DUMMY_BYTE 0xFF 00076 #define SD_NO_RESPONSE_EXPECTED 0x80 00077 00078 /** 00079 * @} 00080 */ 00081 00082 /** @addtogroup STM32F3XX_NUCLEO_144_Private_Variables 00083 * @{ 00084 */ 00085 /** 00086 * @brief LED variables 00087 */ 00088 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED1_GPIO_PORT, LED2_GPIO_PORT, LED3_GPIO_PORT}; 00089 00090 const uint16_t GPIO_PIN[LEDn] = {LED1_PIN, LED2_PIN, LED3_PIN}; 00091 00092 /** 00093 * @brief BUTTON variables 00094 */ 00095 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00096 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00097 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn}; 00098 00099 /** 00100 * @brief BUS variables 00101 */ 00102 00103 #ifdef HAL_SPI_MODULE_ENABLED 00104 uint32_t SpixTimeout = NUCLEO_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00105 static SPI_HandleTypeDef hnucleo_Spi; 00106 #endif /* HAL_SPI_MODULE_ENABLED */ 00107 00108 #ifdef HAL_ADC_MODULE_ENABLED 00109 static ADC_HandleTypeDef hnucleo_Adc; 00110 /* ADC channel configuration structure declaration */ 00111 static ADC_ChannelConfTypeDef sConfig; 00112 #endif /* HAL_ADC_MODULE_ENABLED */ 00113 /** 00114 * @} 00115 */ 00116 00117 /** @defgroup STM32F3XX_NUCLEO_144_BUS Bus Operation functions 00118 * @{ 00119 */ 00120 #ifdef HAL_SPI_MODULE_ENABLED 00121 static void SPIx_Init(void); 00122 static void SPIx_Write(uint8_t Value); 00123 static void SPIx_Error(void); 00124 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00125 00126 /* SD IO functions */ 00127 void SD_IO_Init(void); 00128 void SD_IO_CSState(uint8_t state); 00129 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00130 uint8_t SD_IO_WriteByte(uint8_t Data); 00131 00132 /* LCD IO functions */ 00133 void LCD_IO_Init(void); 00134 void LCD_IO_WriteData(uint8_t Data); 00135 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00136 void LCD_IO_WriteReg(uint8_t LCDReg); 00137 void LCD_Delay(uint32_t delay); 00138 #endif /* HAL_SPI_MODULE_ENABLED */ 00139 00140 #ifdef HAL_ADC_MODULE_ENABLED 00141 static void ADCx_Init(void); 00142 static void ADCx_DeInit(void); 00143 static void ADCx_MspInit(ADC_HandleTypeDef *hadc); 00144 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc); 00145 #endif /* HAL_ADC_MODULE_ENABLED */ 00146 /** 00147 * @} 00148 */ 00149 00150 /** @addtogroup STM32F3XX_NUCLEO_144_Exported_Functions 00151 * @{ 00152 */ 00153 00154 /** 00155 * @brief This method returns the STM32F3XX NUCLEO BSP Driver revision 00156 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00157 */ 00158 00159 uint32_t BSP_GetVersion(void) 00160 { 00161 return __STM32F3xx_NUCLEO_BSP_VERSION; 00162 } 00163 00164 /** 00165 * @brief Configures LED GPIO. 00166 * @param Led Specifies the Led to be configured. 00167 * This parameter can be one of following parameters: 00168 * @arg LED1 00169 * @arg LED2 00170 * @arg LED3 00171 * @retval None 00172 */ 00173 void BSP_LED_Init(Led_TypeDef Led) 00174 { 00175 GPIO_InitTypeDef GPIO_InitStruct; 00176 00177 /* Enable the GPIO_LED Clock */ 00178 LEDx_GPIO_CLK_ENABLE(Led); 00179 00180 /* Configure the GPIO_LED pin */ 00181 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00182 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00183 GPIO_InitStruct.Pull = GPIO_NOPULL; 00184 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00185 00186 HAL_GPIO_Init(GPIO_PORT[Led], &GPIO_InitStruct); 00187 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00188 } 00189 00190 /** 00191 * @brief DeInit LEDs. 00192 * @param Led LED to be de-init. 00193 * This parameter can be one of the following values: 00194 * @arg LED1 00195 * @arg LED2 00196 * @arg LED3 00197 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00198 * @retval None 00199 */ 00200 void BSP_LED_DeInit(Led_TypeDef Led) 00201 { 00202 GPIO_InitTypeDef gpio_init_structure; 00203 00204 /* Turn off LED */ 00205 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00206 /* DeInit the GPIO_LED pin */ 00207 gpio_init_structure.Pin = GPIO_PIN[Led]; 00208 HAL_GPIO_DeInit(GPIO_PORT[Led], gpio_init_structure.Pin); 00209 } 00210 00211 /** 00212 * @brief Turns selected LED On. 00213 * @param Led Specifies the Led to be set on. 00214 * This parameter can be one of following parameters: 00215 * @arg LED2 00216 * @retval None 00217 */ 00218 void BSP_LED_On(Led_TypeDef Led) 00219 { 00220 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00221 } 00222 00223 /** 00224 * @brief Turns selected LED Off. 00225 * @param Led Specifies the Led to be set off. 00226 * This parameter can be one of following parameters: 00227 * @arg LED1 00228 * @arg LED2 00229 * @arg LED3 00230 * @retval None 00231 */ 00232 void BSP_LED_Off(Led_TypeDef Led) 00233 { 00234 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00235 } 00236 00237 /** 00238 * @brief Toggles the selected LED. 00239 * @param Led Specifies the Led to be toggled. 00240 * This parameter can be one of following parameters: 00241 * @arg LED1 00242 * @arg LED2 00243 * @arg LED3 00244 * @retval None 00245 */ 00246 void BSP_LED_Toggle(Led_TypeDef Led) 00247 { 00248 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00249 } 00250 00251 /** 00252 * @brief Configures Button GPIO and EXTI Line. 00253 * @param Button Specifies the Button to be configured. 00254 * This parameter should be: BUTTON_USER 00255 * @param ButtonMode Specifies Button mode. 00256 * This parameter can be one of following parameters: 00257 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00258 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00259 * generation capability 00260 * @retval None 00261 */ 00262 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00263 { 00264 GPIO_InitTypeDef GPIO_InitStruct; 00265 00266 /* Enable the BUTTON Clock */ 00267 BUTTONx_GPIO_CLK_ENABLE(Button); 00268 00269 if(ButtonMode == BUTTON_MODE_GPIO) 00270 { 00271 /* Configure Button pin as input */ 00272 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00273 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00274 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00275 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00276 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00277 } 00278 00279 if(ButtonMode == BUTTON_MODE_EXTI) 00280 { 00281 /* Configure Button pin as input with External interrupt */ 00282 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00283 GPIO_InitStruct.Pull = GPIO_NOPULL; 00284 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00285 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00286 00287 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00288 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00289 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00290 } 00291 } 00292 00293 /** 00294 * @brief Push Button DeInit. 00295 * @param Button Button to be configured 00296 * This parameter should be: BUTTON_USER 00297 * @note PB DeInit does not disable the GPIO clock 00298 * @retval None 00299 */ 00300 void BSP_PB_DeInit(Button_TypeDef Button) 00301 { 00302 GPIO_InitTypeDef gpio_init_structure; 00303 00304 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00305 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00306 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00307 } 00308 00309 /** 00310 * @brief Returns the selected Button state. 00311 * @param Button Specifies the Button to be checked. 00312 * This parameter should be: BUTTON_USER 00313 * @retval The Button GPIO pin value. 00314 */ 00315 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00316 { 00317 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00318 } 00319 00320 /** 00321 * @} 00322 */ 00323 00324 /** @addtogroup STM32F3XX_NUCLEO_144_BUS 00325 * @{ 00326 */ 00327 00328 /****************************************************************************** 00329 BUS OPERATIONS 00330 *******************************************************************************/ 00331 00332 /******************************* SPI ********************************/ 00333 #ifdef HAL_SPI_MODULE_ENABLED 00334 00335 /** 00336 * @brief Initializes SPI MSP. 00337 * @retval None 00338 */ 00339 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00340 { 00341 GPIO_InitTypeDef GPIO_InitStruct; 00342 00343 /*** Configure the GPIOs ***/ 00344 /* Enable GPIO clock */ 00345 NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE(); 00346 NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00347 00348 /* Configure SPI SCK */ 00349 GPIO_InitStruct.Pin = NUCLEO_SPIx_SCK_PIN; 00350 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00351 GPIO_InitStruct.Pull = GPIO_PULLUP; 00352 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00353 GPIO_InitStruct.Alternate = NUCLEO_SPIx_SCK_AF; 00354 HAL_GPIO_Init(NUCLEO_SPIx_SCK_GPIO_PORT, &GPIO_InitStruct); 00355 00356 /* Configure SPI MISO and MOSI */ 00357 GPIO_InitStruct.Pin = NUCLEO_SPIx_MOSI_PIN; 00358 GPIO_InitStruct.Alternate = NUCLEO_SPIx_MISO_MOSI_AF; 00359 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00360 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &GPIO_InitStruct); 00361 00362 GPIO_InitStruct.Pin = NUCLEO_SPIx_MISO_PIN; 00363 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00364 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &GPIO_InitStruct); 00365 00366 /*** Configure the SPI peripheral ***/ 00367 /* Enable SPI clock */ 00368 NUCLEO_SPIx_CLK_ENABLE(); 00369 } 00370 00371 00372 /** 00373 * @brief Initializes SPI HAL. 00374 * @retval None 00375 */ 00376 static void SPIx_Init(void) 00377 { 00378 if(HAL_SPI_GetState(&hnucleo_Spi) == HAL_SPI_STATE_RESET) 00379 { 00380 /* SPI Config */ 00381 hnucleo_Spi.Instance = NUCLEO_SPIx; 00382 /* SPI baudrate is set to 9 MHz maximum (APB1/SPI_BaudRatePrescaler = 72/8 = 9 MHz) 00383 to verify these constraints: 00384 - ST7735 LCD SPI interface max baudrate is 15MHz for write and 6.66MHz for read 00385 Since the provided driver doesn't use read capability from LCD, only constraint 00386 on write baudrate is considered. 00387 - SD card SPI interface max baudrate is 25MHz for write/read 00388 - PCLK2 max frequency is 72 MHz 00389 */ 00390 hnucleo_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_8; 00391 hnucleo_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00392 hnucleo_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00393 hnucleo_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00394 hnucleo_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00395 hnucleo_Spi.Init.CRCPolynomial = 7; 00396 hnucleo_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00397 hnucleo_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00398 hnucleo_Spi.Init.NSS = SPI_NSS_SOFT; 00399 hnucleo_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00400 hnucleo_Spi.Init.Mode = SPI_MODE_MASTER; 00401 00402 SPIx_MspInit(&hnucleo_Spi); 00403 HAL_SPI_Init(&hnucleo_Spi); 00404 } 00405 } 00406 00407 /** 00408 * @brief SPI Write a byte to device 00409 * @param DataIn value to be written 00410 * @param DataOut data out 00411 * @param DataLegnth data length 00412 * @retval None 00413 */ 00414 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth) 00415 { 00416 HAL_StatusTypeDef status = HAL_OK; 00417 00418 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) DataIn, DataOut, DataLegnth, SpixTimeout); 00419 00420 /* Check the communication status */ 00421 if(status != HAL_OK) 00422 { 00423 /* Execute user timeout callback */ 00424 SPIx_Error(); 00425 } 00426 } 00427 00428 /** 00429 * @brief SPI Write a byte to device. 00430 * @param Value value to be written 00431 * @retval None 00432 */ 00433 static void SPIx_Write(uint8_t Value) 00434 { 00435 HAL_StatusTypeDef status = HAL_OK; 00436 uint8_t data; 00437 00438 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) &Value, &data, 1, SpixTimeout); 00439 00440 /* Check the communication status */ 00441 if(status != HAL_OK) 00442 { 00443 /* Execute user timeout callback */ 00444 SPIx_Error(); 00445 } 00446 } 00447 00448 /** 00449 * @brief SPI error treatment function 00450 * @retval None 00451 */ 00452 static void SPIx_Error (void) 00453 { 00454 /* De-initialize the SPI communication BUS */ 00455 HAL_SPI_DeInit(&hnucleo_Spi); 00456 00457 /* Re-Initiaize the SPI communication BUS */ 00458 SPIx_Init(); 00459 } 00460 /** 00461 * @} 00462 */ 00463 00464 /** @defgroup STM32F3XX_NUCLEO_144_LINK_OPERATIONS Link Operation functions 00465 * @{ 00466 */ 00467 00468 /****************************************************************************** 00469 LINK OPERATIONS 00470 *******************************************************************************/ 00471 00472 /********************************* LINK SD ************************************/ 00473 /** 00474 * @brief Initializes the SD Card and put it into StandBy State (Ready for 00475 * data transfer). 00476 * @retval None 00477 */ 00478 void SD_IO_Init(void) 00479 { 00480 GPIO_InitTypeDef GPIO_InitStruct; 00481 uint8_t counter; 00482 00483 /* SD_CS_GPIO Periph clock enable */ 00484 SD_CS_GPIO_CLK_ENABLE(); 00485 00486 /* Configure SD_CS_PIN pin: SD Card CS pin */ 00487 GPIO_InitStruct.Pin = SD_CS_PIN; 00488 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00489 GPIO_InitStruct.Pull = GPIO_PULLUP; 00490 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00491 HAL_GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStruct); 00492 00493 /*------------Put SD in SPI mode--------------*/ 00494 /* SD SPI Config */ 00495 SPIx_Init(); 00496 00497 /* SD chip select high */ 00498 SD_CS_HIGH(); 00499 00500 /* Send dummy byte 0xFF, 10 times with CS high */ 00501 /* Rise CS and MOSI for 80 clocks cycles */ 00502 for (counter = 0; counter <= 9; counter++) 00503 { 00504 /* Send dummy byte 0xFF */ 00505 SD_IO_WriteByte(SD_DUMMY_BYTE); 00506 } 00507 } 00508 00509 /** 00510 * @brief Set the SD_CS pin. 00511 * @param val pin value. 00512 * @retval None 00513 */ 00514 void SD_IO_CSState(uint8_t val) 00515 { 00516 if(val == 1) 00517 { 00518 SD_CS_HIGH(); 00519 } 00520 else 00521 { 00522 SD_CS_LOW(); 00523 } 00524 } 00525 00526 /** 00527 * @brief Write a byte on the SD. 00528 * @param DataIn byte to send 00529 * @param DataOut data out 00530 * @param DataLength data length 00531 * @retval None 00532 */ 00533 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00534 { 00535 // /* SD chip select low */ 00536 // SD_CS_LOW(); 00537 /* Send the byte */ 00538 SPIx_WriteReadData(DataIn, DataOut, DataLength); 00539 } 00540 00541 /** 00542 * @brief Writes a byte on the SD. 00543 * @param Data byte to send. 00544 * @retval None 00545 */ 00546 uint8_t SD_IO_WriteByte(uint8_t Data) 00547 { 00548 uint8_t tmp; 00549 /* Send the byte */ 00550 SPIx_WriteReadData(&Data,&tmp,1); 00551 return tmp; 00552 } 00553 00554 /********************************* LINK LCD ***********************************/ 00555 /** 00556 * @brief Initializes the LCD 00557 * @retval None 00558 */ 00559 void LCD_IO_Init(void) 00560 { 00561 GPIO_InitTypeDef GPIO_InitStruct; 00562 00563 /* LCD_CS_GPIO and LCD_DC_GPIO Periph clock enable */ 00564 LCD_CS_GPIO_CLK_ENABLE(); 00565 LCD_DC_GPIO_CLK_ENABLE(); 00566 00567 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00568 GPIO_InitStruct.Pin = LCD_CS_PIN; 00569 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00570 GPIO_InitStruct.Pull = GPIO_NOPULL; 00571 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00572 HAL_GPIO_Init(LCD_CS_GPIO_PORT, &GPIO_InitStruct); 00573 00574 /* Configure LCD_DC_PIN pin: LCD Card DC pin */ 00575 GPIO_InitStruct.Pin = LCD_DC_PIN; 00576 HAL_GPIO_Init(LCD_DC_GPIO_PORT, &GPIO_InitStruct); 00577 00578 /* LCD chip select high */ 00579 LCD_CS_HIGH(); 00580 00581 /* LCD SPI Config */ 00582 SPIx_Init(); 00583 } 00584 00585 /** 00586 * @brief Writes command to select the LCD register. 00587 * @param LCDReg Address of the selected register. 00588 * @retval None 00589 */ 00590 void LCD_IO_WriteReg(uint8_t LCDReg) 00591 { 00592 /* Reset LCD control line CS */ 00593 LCD_CS_LOW(); 00594 00595 /* Set LCD data/command line DC to Low */ 00596 LCD_DC_LOW(); 00597 00598 /* Send Command */ 00599 SPIx_Write(LCDReg); 00600 00601 /* Deselect : Chip Select high */ 00602 LCD_CS_HIGH(); 00603 } 00604 00605 /** 00606 * @brief Writes data to select the LCD register. 00607 * This function must be used after st7735_WriteReg() function 00608 * @param Data data to write to the selected register. 00609 * @retval None 00610 */ 00611 void LCD_IO_WriteData(uint8_t Data) 00612 { 00613 /* Reset LCD control line CS */ 00614 LCD_CS_LOW(); 00615 00616 /* Set LCD data/command line DC to High */ 00617 LCD_DC_HIGH(); 00618 00619 /* Send Data */ 00620 SPIx_Write(Data); 00621 00622 /* Deselect : Chip Select high */ 00623 LCD_CS_HIGH(); 00624 } 00625 00626 /** 00627 * @brief Write register value. 00628 * @param pData Pointer on the register value 00629 * @param Size Size of byte to transmit to the register 00630 * @retval None 00631 */ 00632 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00633 { 00634 uint32_t counter = 0; 00635 __IO uint32_t data = 0; 00636 00637 /* Reset LCD control line CS */ 00638 LCD_CS_LOW(); 00639 00640 /* Set LCD data/command line DC to High */ 00641 LCD_DC_HIGH(); 00642 00643 if (Size == 1) 00644 { 00645 /* Only 1 byte to be sent to LCD - general interface can be used */ 00646 /* Send Data */ 00647 SPIx_Write(*pData); 00648 } 00649 else 00650 { 00651 /* Several data should be sent in a raw */ 00652 /* Direct SPI accesses for optimization */ 00653 for (counter = Size; counter != 0; counter--) 00654 { 00655 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00656 { 00657 } 00658 /* Need to invert bytes for LCD*/ 00659 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *(pData+1); 00660 00661 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00662 { 00663 } 00664 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *pData; 00665 counter--; 00666 pData += 2; 00667 } 00668 00669 /* Wait until the bus is ready before releasing Chip select */ 00670 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 00671 { 00672 } 00673 } 00674 00675 /* Empty the Rx fifo */ 00676 data = *(&hnucleo_Spi.Instance->DR); 00677 UNUSED(data); 00678 00679 /* Deselect : Chip Select high */ 00680 LCD_CS_HIGH(); 00681 } 00682 00683 /** 00684 * @brief Wait for loop in ms. 00685 * @param Delay in ms. 00686 * @retval None 00687 */ 00688 void LCD_Delay(uint32_t Delay) 00689 { 00690 HAL_Delay(Delay); 00691 } 00692 #endif /* HAL_SPI_MODULE_ENABLED */ 00693 00694 /******************************* ADC driver ********************************/ 00695 #ifdef HAL_ADC_MODULE_ENABLED 00696 /** 00697 * @brief Initializes ADC MSP. 00698 * @param hadc 00699 * @retval None 00700 */ 00701 static void ADCx_MspInit(ADC_HandleTypeDef *hadc) 00702 { 00703 GPIO_InitTypeDef GPIO_InitStruct; 00704 00705 /*** Configure the GPIOs ***/ 00706 /* Enable GPIO clock */ 00707 NUCLEO_ADCx_GPIO_CLK_ENABLE(); 00708 00709 /* Configure the selected ADC Channel as analog input */ 00710 GPIO_InitStruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00711 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 00712 GPIO_InitStruct.Pull = GPIO_NOPULL; 00713 HAL_GPIO_Init(NUCLEO_ADCx_GPIO_PORT, &GPIO_InitStruct); 00714 00715 /*** Configure the ADC peripheral ***/ 00716 /* Enable ADC clock */ 00717 NUCLEO_ADCx_CLK_ENABLE(); 00718 } 00719 00720 /** 00721 * @brief DeInitializes ADC MSP. 00722 * @param hadc 00723 * @note ADC DeInit does not disable the GPIO clock 00724 * @retval None 00725 */ 00726 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc) 00727 { 00728 GPIO_InitTypeDef GPIO_InitStruct; 00729 00730 /*** DeInit the ADC peripheral ***/ 00731 /* Disable ADC clock */ 00732 NUCLEO_ADCx_CLK_DISABLE(); 00733 00734 /* Configure the selected ADC Channel as analog input */ 00735 GPIO_InitStruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00736 HAL_GPIO_DeInit(NUCLEO_ADCx_GPIO_PORT, GPIO_InitStruct.Pin); 00737 00738 /* Disable GPIO clock has to be done by the application*/ 00739 /* NUCLEO_ADCx_GPIO_CLK_DISABLE(); */ 00740 } 00741 00742 /** 00743 * @brief Initializes ADC HAL. 00744 * @retval None 00745 */ 00746 static void ADCx_Init(void) 00747 { 00748 /* Set ADC instance */ 00749 hnucleo_Adc.Instance = NUCLEO_ADCx; 00750 00751 if(HAL_ADC_GetState(&hnucleo_Adc) == HAL_ADC_STATE_RESET) 00752 { 00753 /* ADC Config */ 00754 hnucleo_Adc.Init.ClockPrescaler = ADC_CLOCK_SYNC_PCLK_DIV4; /* ADC clock of STM32F37x devices must not exceed 16MHz (ADC clock of other STMF3 devices can be set up to 72MHz) */ 00755 hnucleo_Adc.Init.Resolution = ADC_RESOLUTION_12B; 00756 hnucleo_Adc.Init.DataAlign = ADC_DATAALIGN_RIGHT; 00757 hnucleo_Adc.Init.ScanConvMode = ADC_SCAN_DISABLE; /* Sequencer disabled (ADC conversion on only 1 channel: channel set on rank 1) */ 00758 hnucleo_Adc.Init.EOCSelection = ADC_EOC_SINGLE_CONV; 00759 hnucleo_Adc.Init.LowPowerAutoWait = DISABLE; 00760 hnucleo_Adc.Init.ContinuousConvMode = DISABLE; /* Continuous mode disabled to have only 1 conversion at each conversion trig */ 00761 hnucleo_Adc.Init.NbrOfConversion = 1; /* Parameter discarded because sequencer is disabled */ 00762 hnucleo_Adc.Init.DiscontinuousConvMode = DISABLE; /* Parameter discarded because sequencer is disabled */ 00763 hnucleo_Adc.Init.NbrOfDiscConversion = 1; /* Parameter discarded because sequencer is disabled */ 00764 hnucleo_Adc.Init.ExternalTrigConv = ADC_SOFTWARE_START; /* Software start to trig the 1st conversion manually, without external event */ 00765 hnucleo_Adc.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; /* Parameter discarded because trig by software start */ 00766 hnucleo_Adc.Init.DMAContinuousRequests = DISABLE; 00767 hnucleo_Adc.Init.Overrun = ADC_OVR_DATA_OVERWRITTEN; 00768 00769 /* Initialize MSP related to ADC */ 00770 ADCx_MspInit(&hnucleo_Adc); 00771 00772 /* Initialize ADC */ 00773 HAL_ADC_Init(&hnucleo_Adc); 00774 00775 /* Run ADC calibration */ 00776 HAL_ADCEx_Calibration_Start(&hnucleo_Adc, ADC_SINGLE_ENDED); 00777 } 00778 } 00779 00780 /** 00781 * @brief Initializes ADC HAL. 00782 * @retval None 00783 */ 00784 static void ADCx_DeInit(void) 00785 { 00786 hnucleo_Adc.Instance = NUCLEO_ADCx; 00787 00788 HAL_ADC_DeInit(&hnucleo_Adc); 00789 ADCx_MspDeInit(&hnucleo_Adc); 00790 } 00791 00792 /******************************* LINK JOYSTICK ********************************/ 00793 00794 /** 00795 * @brief Configures joystick available on adafruit 1.8" TFT shield 00796 * managed through ADC to detect motion. 00797 * @retval Joystickstatus (0=> success, 1=> fail) 00798 */ 00799 uint8_t BSP_JOY_Init(void) 00800 { 00801 ADCx_Init(); 00802 00803 /* Select the ADC Channel to be converted */ 00804 sConfig.Channel = NUCLEO_ADCx_CHANNEL; 00805 sConfig.Rank = ADC_REGULAR_RANK_1; 00806 sConfig.SamplingTime = ADC_SAMPLETIME_19CYCLES_5; 00807 sConfig.SingleDiff = ADC_SINGLE_ENDED; 00808 sConfig.OffsetNumber = ADC_OFFSET_NONE; 00809 sConfig.Offset = 0; 00810 00811 /* Return Joystick initialization status */ 00812 return (uint8_t)HAL_ADC_ConfigChannel(&hnucleo_Adc, &sConfig); 00813 } 00814 00815 /** 00816 * @brief DeInit joystick GPIOs. 00817 * @note JOY DeInit does not disable the Mfx, just set the Mfx pins in Off mode 00818 * @retval None. 00819 */ 00820 void BSP_JOY_DeInit(void) 00821 { 00822 ADCx_DeInit(); 00823 } 00824 00825 /** 00826 * @brief Returns the Joystick key pressed. 00827 * @note To know which Joystick key is pressed we need to detect the voltage 00828 * level on each key output 00829 * - None : 3.3 V / 4095 00830 * - SEL : 1.055 V / 1308 00831 * - DOWN : 0.71 V / 88 00832 * - LEFT : 3.0 V / 3720 00833 * - RIGHT : 0.595 V / 737 00834 * - UP : 1.65 V / 2046 00835 * @retval JOYState_TypeDef: Code of the Joystick key pressed. 00836 */ 00837 JOYState_TypeDef BSP_JOY_GetState(void) 00838 { 00839 JOYState_TypeDef state; 00840 uint16_t keyconvertedvalue = 0; 00841 00842 /* Start the conversion process */ 00843 HAL_ADC_Start(&hnucleo_Adc); 00844 00845 /* Wait for the end of conversion */ 00846 if (HAL_ADC_PollForConversion(&hnucleo_Adc, 10) != HAL_TIMEOUT) 00847 { 00848 /* Get the converted value of regular channel */ 00849 keyconvertedvalue = HAL_ADC_GetValue(&hnucleo_Adc); 00850 } 00851 00852 if((keyconvertedvalue > 2010) && (keyconvertedvalue < 2090)) 00853 { 00854 state = JOY_UP; 00855 } 00856 else if((keyconvertedvalue > 680) && (keyconvertedvalue < 780)) 00857 { 00858 state = JOY_RIGHT; 00859 } 00860 else if((keyconvertedvalue > 1270) && (keyconvertedvalue < 1350)) 00861 { 00862 state = JOY_SEL; 00863 } 00864 else if((keyconvertedvalue > 50) && (keyconvertedvalue < 130)) 00865 { 00866 state = JOY_DOWN; 00867 } 00868 else if((keyconvertedvalue > 3680) && (keyconvertedvalue < 3760)) 00869 { 00870 state = JOY_LEFT; 00871 } 00872 else 00873 { 00874 state = JOY_NONE; 00875 } 00876 00877 /* Loop while a key is pressed */ 00878 if(state != JOY_NONE) 00879 { 00880 keyconvertedvalue = HAL_ADC_GetValue(&hnucleo_Adc); 00881 } 00882 /* Return the code of the Joystick key pressed */ 00883 return state; 00884 } 00885 #endif /* HAL_ADC_MODULE_ENABLED */ 00886 00887 /** 00888 * @} 00889 */ 00890 00891 /** 00892 * @} 00893 */ 00894 00895 /** 00896 * @} 00897 */ 00898 00899 /** 00900 * @} 00901 */ 00902 00903 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 11:02:21 for STM32F3xx_Nucleo_144 BSP User Manual by
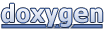