STM32F0308-Discovery BSP User Manual
|
stm32f0308_discovery.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f0308_discovery.h 00004 * @author MCD Application Team 00005 * @brief This file contains definitions for STM32F0308-Discovery's Leds, push- 00006 * buttons hardware resources. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32F0308_DISCOVERY_H 00039 #define __STM32F0308_DISCOVERY_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32f0xx_hal.h" 00047 00048 /** @addtogroup BSP 00049 * @{ 00050 */ 00051 00052 /** @defgroup STM32F0308_DISCOVERY STM32F0308_DISCOVERY 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32F0308_DISCOVERY_Common STM32F0308_DISCOVERY Common 00057 * @{ 00058 */ 00059 00060 /** @defgroup STM32F0308_DISCOVERY_Exported_Types Exported Types 00061 * @{ 00062 */ 00063 00064 /** 00065 * @brief LED Types Definition 00066 */ 00067 typedef enum 00068 { 00069 LED3 = 0, 00070 LED4 = 1, 00071 /* Color led aliases */ 00072 LED_GREEN = LED3, 00073 LED_BLUE = LED4 00074 }Led_TypeDef; 00075 00076 /** 00077 * @brief BUTTON Types Definition 00078 */ 00079 typedef enum 00080 { 00081 BUTTON_USER = 0 00082 }Button_TypeDef; 00083 00084 typedef enum 00085 { 00086 BUTTON_MODE_GPIO = 0, 00087 BUTTON_MODE_EXTI = 1 00088 }ButtonMode_TypeDef; 00089 00090 /** 00091 * @} 00092 */ 00093 00094 /** @defgroup STM32F0308_DISCOVERY_Exported_Constants Exported Constants 00095 * @{ 00096 */ 00097 /** 00098 * @brief Define for STM32F0308_DISCOVERY board 00099 */ 00100 #if !defined (USE_STM320308_DISCO) 00101 #define USE_STM320308_DISCO 00102 #endif 00103 00104 /** @defgroup STM32F0308_DISCOVERY_LED STM32F0308_DISCOVERY LED 00105 * @{ 00106 */ 00107 #define LEDn 2 00108 00109 #define LED3_PIN GPIO_PIN_9 00110 #define LED3_GPIO_PORT GPIOC 00111 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00112 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00113 00114 #define LED4_PIN GPIO_PIN_8 00115 #define LED4_GPIO_PORT GPIOC 00116 #define LED4_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00117 #define LED4_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00118 00119 #define LEDx_GPIO_CLK_ENABLE(__LED__) do { if((__LED__) == LED3) LED3_GPIO_CLK_ENABLE(); else \ 00120 if((__LED__) == LED4) LED4_GPIO_CLK_ENABLE();} while(0) 00121 00122 #define LEDx_GPIO_CLK_DISABLE(__LED__) (((__LED__) == LED3) ? LED3_GPIO_CLK_DISABLE() :\ 00123 ((__LED__) == LED4) ? LED4_GPIO_CLK_DISABLE() : 0 ) 00124 /** 00125 * @} 00126 */ 00127 00128 /** @defgroup STM32F0308_DISCOVERY_BUTTON STM32F0308_DISCOVERY BUTTON 00129 * @{ 00130 */ 00131 #define BUTTONn 1 00132 00133 /** 00134 * @brief USER push-button 00135 */ 00136 #define USER_BUTTON_PIN GPIO_PIN_0 /* PA0 */ 00137 #define USER_BUTTON_GPIO_PORT GPIOA 00138 #define USER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00139 #define USER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00140 #define USER_BUTTON_EXTI_IRQn EXTI0_1_IRQn 00141 00142 #define BUTTONx_GPIO_CLK_ENABLE(__BUTTON__) do { if((__BUTTON__) == BUTTON_USER) USER_BUTTON_GPIO_CLK_ENABLE();} while(0) 00143 00144 #define BUTTONx_GPIO_CLK_DISABLE(__BUTTON__) (((__BUTTON__) == BUTTON_USER) ? USER_BUTTON_GPIO_CLK_DISABLE() : 0 ) 00145 /** 00146 * @} 00147 */ 00148 00149 /** 00150 * @} 00151 */ 00152 00153 /** @defgroup STM32F0308_DISCOVERY_Exported_Functions Exported Functions 00154 * @{ 00155 */ 00156 uint32_t BSP_GetVersion(void); 00157 void BSP_LED_Init(Led_TypeDef Led); 00158 void BSP_LED_On(Led_TypeDef Led); 00159 void BSP_LED_Off(Led_TypeDef Led); 00160 void BSP_LED_Toggle(Led_TypeDef Led); 00161 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode); 00162 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00163 /** 00164 * @} 00165 */ 00166 00167 /** 00168 * @} 00169 */ 00170 00171 /** 00172 * @} 00173 */ 00174 00175 /** 00176 * @} 00177 */ 00178 00179 #ifdef __cplusplus 00180 } 00181 #endif 00182 00183 #endif /* __STM32F0308_DISCOVERY_H */ 00184 00185 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jul 5 2017 09:26:24 for STM32F0308-Discovery BSP User Manual by
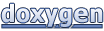