STM32F0308-Discovery BSP User Manual
|
stm32f0308_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f0308_discovery.c 00004 * @author MCD Application Team 00005 * @brief This file provides set of firmware functions to manage Leds and 00006 * push-button available on STM32F0308-DISCOVERY Kit from STMicroelectronics. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32f0308_discovery.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32F0308_DISCOVERY 00045 * @{ 00046 */ 00047 00048 /** @addtogroup STM32F0308_DISCOVERY_Common 00049 * @brief This file provides firmware functions to manage Leds and push-buttons, 00050 * available on STM32F0308_DISCOVERY evaluation board from STMicroelectronics. 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32F0308_DISCOVERY_Private_Constants Private Constants 00055 * @{ 00056 */ 00057 00058 /** 00059 * @brief STM32F0308 DISCO BSP Driver version number V2.0.5 00060 */ 00061 #define __STM32F0308_DISCO_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00062 #define __STM32F0308_DISCO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00063 #define __STM32F0308_DISCO_BSP_VERSION_SUB2 (0x05) /*!< [15:8] sub2 version */ 00064 #define __STM32F0308_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00065 #define __STM32F0308_DISCO_BSP_VERSION ((__STM32F0308_DISCO_BSP_VERSION_MAIN << 24)|\ 00066 (__STM32F0308_DISCO_BSP_VERSION_SUB1 << 16)|\ 00067 (__STM32F0308_DISCO_BSP_VERSION_SUB2 << 8 )|\ 00068 (__STM32F0308_DISCO_BSP_VERSION_RC)) 00069 00070 /** 00071 * @} 00072 */ 00073 00074 /** @defgroup STM32F0308_DISCOVERY_Private_Variables Private Variables 00075 * @{ 00076 */ 00077 GPIO_TypeDef* LED_PORT[LEDn] = {LED3_GPIO_PORT, LED4_GPIO_PORT}; 00078 const uint16_t LED_PIN[LEDn] = {LED3_PIN, LED4_PIN}; 00079 00080 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00081 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00082 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn}; 00083 00084 /** 00085 * @} 00086 */ 00087 00088 /** @addtogroup STM32F0308_DISCOVERY_Exported_Functions 00089 * @{ 00090 */ 00091 00092 /** 00093 * @brief This method returns the STM32F0308 DISCO BSP Driver revision 00094 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00095 */ 00096 uint32_t BSP_GetVersion(void) 00097 { 00098 return __STM32F0308_DISCO_BSP_VERSION; 00099 } 00100 00101 /** 00102 * @brief Configures LED GPIO. 00103 * @param Led Specifies the Led to be configured. 00104 * This parameter can be one of following parameters: 00105 * @arg LED3 00106 * @arg LED4 00107 * @retval None 00108 */ 00109 void BSP_LED_Init(Led_TypeDef Led) 00110 { 00111 GPIO_InitTypeDef GPIO_InitStruct; 00112 00113 /* Enable the GPIO_LED clock */ 00114 LEDx_GPIO_CLK_ENABLE(Led); 00115 00116 /* Configure the GPIO_LED pin */ 00117 GPIO_InitStruct.Pin = LED_PIN[Led]; 00118 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00119 GPIO_InitStruct.Pull = GPIO_NOPULL; 00120 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00121 00122 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00123 00124 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00125 } 00126 00127 /** 00128 * @brief Turns selected LED On. 00129 * @param Led Specifies the Led to be set on. 00130 * This parameter can be one of following parameters: 00131 * @arg LED3 00132 * @arg LED4 00133 * @retval None 00134 */ 00135 void BSP_LED_On(Led_TypeDef Led) 00136 { 00137 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00138 } 00139 00140 /** 00141 * @brief Turns selected LED Off. 00142 * @param Led Specifies the Led to be set off. 00143 * This parameter can be one of following parameters: 00144 * @arg LED3 00145 * @arg LED4 00146 * @retval None 00147 */ 00148 void BSP_LED_Off(Led_TypeDef Led) 00149 { 00150 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00151 } 00152 00153 /** 00154 * @brief Toggles the selected LED. 00155 * @param Led Specifies the Led to be toggled. 00156 * This parameter can be one of following parameters: 00157 * @arg LED3 00158 * @arg LED4 00159 * @retval None 00160 */ 00161 void BSP_LED_Toggle(Led_TypeDef Led) 00162 { 00163 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00164 } 00165 00166 /** 00167 * @brief Configures Button GPIO and EXTI Line. 00168 * @param Button Specifies the Button to be configured. 00169 * This parameter should be: BUTTON_USER 00170 * @param Mode Specifies Button mode. 00171 * This parameter can be one of the following values: 00172 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00173 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00174 * with interrupt generation capability 00175 * @retval None 00176 */ 00177 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode) 00178 { 00179 GPIO_InitTypeDef GPIO_InitStruct; 00180 00181 /* Enable the BUTTON Clock */ 00182 BUTTONx_GPIO_CLK_ENABLE(Button); 00183 __HAL_RCC_SYSCFG_CLK_ENABLE(); 00184 00185 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00186 GPIO_InitStruct.Pull = GPIO_NOPULL; 00187 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00188 00189 if(Mode == BUTTON_MODE_GPIO) 00190 { 00191 /* Configure Button pin as input */ 00192 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00193 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00194 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00195 } 00196 00197 if(Mode == BUTTON_MODE_EXTI) 00198 { 00199 /* Configure Button pin as input with External interrupt */ 00200 GPIO_InitStruct.Pull = GPIO_NOPULL; 00201 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00202 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00203 00204 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00205 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x03, 0x00); 00206 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00207 } 00208 } 00209 00210 00211 /** 00212 * @brief Returns the selected Button state. 00213 * @param Button Specifies the Button to be checked. 00214 * This parameter should be: BUTTON_USER 00215 * @retval The Button GPIO pin value. 00216 */ 00217 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00218 { 00219 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00220 } 00221 00222 /** 00223 * @} 00224 */ 00225 00226 /** 00227 * @} 00228 */ 00229 00230 /** 00231 * @} 00232 */ 00233 00234 /** 00235 * @} 00236 */ 00237 00238 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jul 5 2017 09:26:24 for STM32F0308-Discovery BSP User Manual by
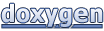