STM32769I_EVAL BSP User Manual
|
stm32f769i_eval_audio.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_eval_audio.h 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32f769i_eval_audio.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32F769I_EVAL_AUDIO_H 00041 #define __STM32F769I_EVAL_AUDIO_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 /* Include audio component Driver */ 00049 #include "../Components/wm8994/wm8994.h" 00050 00051 #if defined(USE_LCD_HDMI) 00052 /* Include ADV7533 HDMI Driver IC driver code */ 00053 #include "../Components/adv7533/adv7533.h" 00054 #endif /* USE_LCD_HDMI */ 00055 00056 #include "stm32f769i_eval.h" 00057 #include <stdlib.h> 00058 00059 /** @addtogroup BSP 00060 * @{ 00061 */ 00062 00063 /** @addtogroup STM32F769I_EVAL 00064 * @{ 00065 */ 00066 00067 /** @addtogroup STM32F769I_EVAL_AUDIO 00068 * @{ 00069 */ 00070 00071 /** @defgroup STM32F769I_EVAL_AUDIO_Exported_Types STM32F769I_EVAL_AUDIO Exported Types 00072 * @{ 00073 */ 00074 /** 00075 * @} 00076 */ 00077 00078 /** @defgroup STM32F769I_EVAL_AUDIO_Exported_Constants STM32F769I_EVAL_AUDIO Exported Constants 00079 * @{ 00080 */ 00081 00082 /** @defgroup BSP_Audio_Out_Option BSP Audio Out Option 00083 * @{ 00084 */ 00085 #define BSP_AUDIO_OUT_CIRCULARMODE ((uint32_t)0x00000001) /* BUFFER CIRCULAR MODE */ 00086 #define BSP_AUDIO_OUT_NORMALMODE ((uint32_t)0x00000002) /* BUFFER NORMAL MODE */ 00087 #define BSP_AUDIO_OUT_STEREOMODE ((uint32_t)0x00000004) /* STEREO MODE */ 00088 #define BSP_AUDIO_OUT_MONOMODE ((uint32_t)0x00000008) /* MONO MODE */ 00089 /** 00090 * @} 00091 */ 00092 /** @defgroup BSP_Audio_Sample_Rate BSP Audio Sample Rate 00093 * @{ 00094 */ 00095 #define BSP_AUDIO_FREQUENCY_96K SAI_AUDIO_FREQUENCY_96K 00096 #define BSP_AUDIO_FREQUENCY_48K SAI_AUDIO_FREQUENCY_48K 00097 #define BSP_AUDIO_FREQUENCY_44K SAI_AUDIO_FREQUENCY_44K 00098 #define BSP_AUDIO_FREQUENCY_32K SAI_AUDIO_FREQUENCY_32K 00099 #define BSP_AUDIO_FREQUENCY_22K SAI_AUDIO_FREQUENCY_22K 00100 #define BSP_AUDIO_FREQUENCY_16K SAI_AUDIO_FREQUENCY_16K 00101 #define BSP_AUDIO_FREQUENCY_11K SAI_AUDIO_FREQUENCY_11K 00102 #define BSP_AUDIO_FREQUENCY_8K SAI_AUDIO_FREQUENCY_8K 00103 /** 00104 * @} 00105 */ 00106 00107 /*------------------------------------------------------------------------------ 00108 USER SAI defines parameters 00109 -----------------------------------------------------------------------------*/ 00110 /** CODEC_AudioFrame_SLOT_TDMMode In W8994 codec the Audio frame contains 4 slots : TDM Mode 00111 * TDM format : 00112 * +------------------|------------------|--------------------|-------------------+ 00113 * | CODEC_SLOT0 Left | CODEC_SLOT1 Left | CODEC_SLOT0 Right | CODEC_SLOT1 Right | 00114 * +------------------------------------------------------------------------------+ 00115 */ 00116 /* To have 2 separate audio stream in Both headphone and speaker the 4 slot must be activated */ 00117 #define CODEC_AUDIOFRAME_SLOT_0123 SAI_SLOTACTIVE_0 | SAI_SLOTACTIVE_1 | SAI_SLOTACTIVE_2 | SAI_SLOTACTIVE_3 00118 /* To have an audio stream in headphone only SAI Slot 0 and Slot 2 must be activated */ 00119 #define CODEC_AUDIOFRAME_SLOT_02 SAI_SLOTACTIVE_0 | SAI_SLOTACTIVE_2 00120 /* To have an audio stream in speaker only SAI Slot 1 and Slot 3 must be activated */ 00121 #define CODEC_AUDIOFRAME_SLOT_13 SAI_SLOTACTIVE_1 | SAI_SLOTACTIVE_3 00122 00123 /* SAI peripheral configuration defines */ 00124 #define AUDIO_SAIx SAI2_Block_B 00125 #define AUDIO_SAIx_CLK_ENABLE() __HAL_RCC_SAI2_CLK_ENABLE() 00126 #define AUDIO_SAIx_CLK_DISABLE() __HAL_RCC_SAI2_CLK_DISABLE() 00127 #define AUDIO_SAIx_SCK_AF GPIO_AF8_SAI2 00128 #define AUDIO_SAIx_FS_SD_MCLK_AF GPIO_AF10_SAI2 00129 00130 #define AUDIO_SAIx_MCLK_ENABLE() __HAL_RCC_GPIOE_CLK_ENABLE() 00131 #define AUDIO_SAIx_MCLK_GPIO_PORT GPIOE 00132 #define AUDIO_SAIx_MCLK_PIN GPIO_PIN_6 00133 #define AUDIO_SAIx_SCK_SD_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00134 #define AUDIO_SAIx_SCK_SD_GPIO_PORT GPIOA 00135 #define AUDIO_SAIx_SCK_PIN GPIO_PIN_2 00136 #define AUDIO_SAIx_SD_PIN GPIO_PIN_0 00137 #define AUDIO_SAIx_FS_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00138 #define AUDIO_SAIx_FS_GPIO_PORT GPIOG 00139 #define AUDIO_SAIx_FS_PIN GPIO_PIN_9 00140 00141 00142 /* SAI DMA Stream definitions */ 00143 #define AUDIO_SAIx_DMAx_CLK_ENABLE() __HAL_RCC_DMA2_CLK_ENABLE() 00144 #define AUDIO_SAIx_DMAx_STREAM DMA2_Stream6 00145 #define AUDIO_SAIx_DMAx_CHANNEL DMA_CHANNEL_3 00146 #define AUDIO_SAIx_DMAx_IRQ DMA2_Stream6_IRQn 00147 #define AUDIO_SAIx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00148 #define AUDIO_SAIx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00149 #define DMA_MAX_SZE 0xFFFF 00150 00151 #define AUDIO_SAIx_DMAx_IRQHandler DMA2_Stream6_IRQHandler 00152 00153 /* Select the interrupt preemption priority for the DMA interrupt */ 00154 #define AUDIO_OUT_IRQ_PREPRIO ((uint32_t)0x0E) 00155 00156 /*------------------------------------------------------------------------------ 00157 AUDIO IN CONFIGURATION 00158 ------------------------------------------------------------------------------*/ 00159 /* DFSDM Configuration defines */ 00160 #define AUDIO_DFSDMx_LEFT_CHANNEL DFSDM_CHANNEL_1 00161 #define AUDIO_DFSDMx_RIGHT_CHANNEL DFSDM_CHANNEL_0 00162 #define AUDIO_DFSDMx_LEFT_FILTER DFSDM1_Filter0 00163 #define AUDIO_DFSDMx_RIGHT_FILTER DFSDM1_Filter1 00164 #define AUDIO_DFSDMx_CLK_ENABLE() __HAL_RCC_DFSDM_CLK_ENABLE() 00165 #define AUDIO_DFSDMx_CKOUT_PIN GPIO_PIN_3 00166 #define AUDIO_DFSDMx_DMIC_DATIN_PIN GPIO_PIN_6 00167 #define AUDIO_DFSDMx_CKOUT_DMIC_DATIN_GPIO_PORT GPIOD 00168 #define AUDIO_DFSDMx_CKOUT_DMIC_DATIN_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00169 #define AUDIO_DFSDMx_DMIC_DATIN_AF GPIO_AF10_DFSDM1 00170 #define AUDIO_DFSDMx_CKOUT_AF GPIO_AF3_DFSDM1 00171 00172 /* DFSDM DMA Right and Left channels definitions */ 00173 #define AUDIO_DFSDMx_DMAx_CLK_ENABLE() __HAL_RCC_DMA2_CLK_ENABLE() 00174 #define AUDIO_DFSDMx_DMAx_LEFT_CHANNEL DMA_CHANNEL_8 00175 #define AUDIO_DFSDMx_DMAx_RIGHT_CHANNEL DMA_CHANNEL_8 00176 #define AUDIO_DFSDMx_DMAx_LEFT_IRQ DMA2_Stream0_IRQn 00177 #define AUDIO_DFSDMx_DMAx_RIGHT_IRQ DMA2_Stream5_IRQn 00178 #define AUDIO_DFSDMx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_WORD 00179 #define AUDIO_DFSDMx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_WORD 00180 00181 #define AUDIO_DFSDM_DMAx_LEFT_IRQHandler DMA2_Stream0_IRQHandler 00182 #define AUDIO_DFSDM_DMAx_RIGHT_IRQHandler DMA2_Stream5_IRQHandler 00183 00184 /* Select the interrupt preemption priority and subpriority for the IT/DMA interrupt */ 00185 #define AUDIO_IN_IRQ_PREPRIO ((uint32_t)0x0F) 00186 00187 00188 /*------------------------------------------------------------------------------ 00189 CONFIGURATION: Audio Driver Configuration parameters 00190 ------------------------------------------------------------------------------*/ 00191 00192 #define AUDIODATA_SIZE 2 /* 16-bits audio data size */ 00193 00194 /* Audio status definition */ 00195 #define AUDIO_OK ((uint8_t)0) 00196 #define AUDIO_ERROR ((uint8_t)1) 00197 #define AUDIO_TIMEOUT ((uint8_t)2) 00198 00199 /* AudioFreq * DataSize (2 bytes) * NumChannels (Stereo: 2) */ 00200 #define DEFAULT_AUDIO_IN_FREQ I2S_AUDIOFREQ_16K 00201 #define DEFAULT_AUDIO_IN_BIT_RESOLUTION ((uint8_t)16) 00202 #define DEFAULT_AUDIO_IN_CHANNEL_NBR ((uint8_t)2) /* Mono = 1, Stereo = 2 */ 00203 #define DEFAULT_AUDIO_IN_VOLUME ((uint16_t)64) 00204 00205 /*------------------------------------------------------------------------------ 00206 OPTIONAL Configuration defines parameters 00207 ------------------------------------------------------------------------------*/ 00208 00209 /* Delay for the Codec to be correctly reset */ 00210 #define CODEC_RESET_DELAY ((uint8_t)5) 00211 00212 00213 /*------------------------------------------------------------------------------ 00214 OUTPUT DEVICES definition 00215 ------------------------------------------------------------------------------*/ 00216 /* Alias on existing output devices to adapt for 2 headphones output */ 00217 #define OUTPUT_DEVICE_HEADPHONE1 OUTPUT_DEVICE_HEADPHONE 00218 #define OUTPUT_DEVICE_HEADPHONE2 OUTPUT_DEVICE_SPEAKER /* Headphone2 is connected to Speaker output of the wm8994 */ 00219 #define OUTPUT_DEVICE_HDMI OUTPUT_DEVICE_ADV7533_HDMI 00220 /** 00221 * @} 00222 */ 00223 00224 /** @defgroup STM32F769I_EVAL_AUDIO_Exported_Variables STM32F769I_EVAL_AUDIO Exported Variables 00225 * @{ 00226 */ 00227 /** 00228 * @} 00229 */ 00230 00231 /** @defgroup STM32F769I_EVAL_AUDIO_Exported_Macros STM32F769I_EVAL_AUDIO Exported Macros 00232 * @{ 00233 */ 00234 #define DMA_MAX(x) (((x) <= DMA_MAX_SZE)? (x):DMA_MAX_SZE) 00235 /** 00236 * @} 00237 */ 00238 00239 /** @defgroup STM32F769I_EVAL_AUDIO_OUT_Exported_Functions STM32F769I_EVAL_AUDIO_OUT Exported Functions 00240 * @{ 00241 */ 00242 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00243 void BSP_AUDIO_OUT_DeInit(void); 00244 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size); 00245 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00246 uint8_t BSP_AUDIO_OUT_Pause(void); 00247 uint8_t BSP_AUDIO_OUT_Resume(void); 00248 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option); 00249 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume); 00250 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq); 00251 void BSP_AUDIO_OUT_SetAudioFrameSlot(uint32_t AudioFrameSlot); 00252 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd); 00253 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output); 00254 00255 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00256 /* This function is called when the requested data has been completely transferred.*/ 00257 void BSP_AUDIO_OUT_TransferComplete_CallBack(void); 00258 00259 /* This function is called when half of the requested buffer has been transferred. */ 00260 void BSP_AUDIO_OUT_HalfTransfer_CallBack(void); 00261 00262 /* This function is called when an Interrupt due to transfer error on or peripheral 00263 error occurs. */ 00264 void BSP_AUDIO_OUT_Error_CallBack(void); 00265 00266 /* These function can be modified in case the current settings (e.g. DMA stream) 00267 need to be changed for specific application needs */ 00268 void BSP_AUDIO_OUT_ClockConfig(SAI_HandleTypeDef *hsai, uint32_t AudioFreq, void *Params); 00269 void BSP_AUDIO_OUT_MspInit(SAI_HandleTypeDef *hsai, void *Params); 00270 void BSP_AUDIO_OUT_MspDeInit(SAI_HandleTypeDef *hsai, void *Params); 00271 00272 /** 00273 * @} 00274 */ 00275 00276 /** @defgroup STM32F769I_EVAL_AUDIO_IN_Exported_Functions STM32F769I_EVAL_AUDIO_IN Exported Functions 00277 * @{ 00278 */ 00279 uint8_t BSP_AUDIO_IN_Init(uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr); 00280 uint8_t BSP_AUDIO_IN_AllocScratch (int32_t *pScratch, uint32_t size); 00281 void BSP_AUDIO_IN_DeInit(void); 00282 uint8_t BSP_AUDIO_IN_Record(uint16_t *pData, uint32_t Size); 00283 uint8_t BSP_AUDIO_IN_Stop(void); 00284 uint8_t BSP_AUDIO_IN_Pause(void); 00285 uint8_t BSP_AUDIO_IN_Resume(void); 00286 00287 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00288 /* This function should be implemented by the user application. 00289 It is called into this driver when the current buffer is filled to prepare the next 00290 buffer pointer and its size. */ 00291 void BSP_AUDIO_IN_TransferComplete_CallBack(void); 00292 void BSP_AUDIO_IN_HalfTransfer_CallBack(void); 00293 00294 /* This function is called when an Interrupt due to transfer error on or peripheral 00295 error occurs. */ 00296 void BSP_AUDIO_IN_Error_Callback(void); 00297 00298 /* These function can be modified in case the current settings (e.g. DMA stream) 00299 need to be changed for specific application needs */ 00300 void BSP_AUDIO_IN_ClockConfig(DFSDM_Filter_HandleTypeDef *hdfsdm_filter, uint32_t AudioFreq, void *Params); 00301 void BSP_AUDIO_IN_MspInit(void); 00302 void BSP_AUDIO_IN_MspDeInit(void); 00303 /** 00304 * @} 00305 */ 00306 00307 /** 00308 * @} 00309 */ 00310 00311 /** 00312 * @} 00313 */ 00314 00315 /** 00316 * @} 00317 */ 00318 00319 #ifdef __cplusplus 00320 } 00321 #endif 00322 00323 #endif /* __STM32F769I_EVAL_AUDIO_H */ 00324 00325 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 20:55:35 for STM32769I_EVAL BSP User Manual by
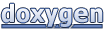