STM32769I_EVAL BSP User Manual
|
stm32f769i_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_eval.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM32F769I-EVAL 00009 * evaluation board(MB1219) from STMicroelectronics. 00010 * 00011 @verbatim 00012 This driver requires the stm32f769i_eval_io.c/.h files to manage the 00013 IO module resources mapped on the MFX IO expander. 00014 These resources are mainly LEDs, Joystick push buttons, SD detect pin, 00015 USB OTG power switch/over current drive pins, Camera plug pin, Audio 00016 INT pin 00017 @endverbatim 00018 ****************************************************************************** 00019 * @attention 00020 * 00021 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00022 * 00023 * Redistribution and use in source and binary forms, with or without modification, 00024 * are permitted provided that the following conditions are met: 00025 * 1. Redistributions of source code must retain the above copyright notice, 00026 * this list of conditions and the following disclaimer. 00027 * 2. Redistributions in binary form must reproduce the above copyright notice, 00028 * this list of conditions and the following disclaimer in the documentation 00029 * and/or other materials provided with the distribution. 00030 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00031 * may be used to endorse or promote products derived from this software 00032 * without specific prior written permission. 00033 * 00034 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00035 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00036 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00037 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00038 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00039 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00040 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00041 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00042 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00043 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00044 * 00045 ****************************************************************************** 00046 */ 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32f769i_eval.h" 00050 #if defined(USE_IOEXPANDER) 00051 #include "stm32f769i_eval_io.h" 00052 #endif /* USE_IOEXPANDER */ 00053 00054 /** @addtogroup BSP 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM32F769I_EVAL 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32F769I_EVAL_LOW_LEVEL STM32F769I_EVAL LOW LEVEL 00063 * @{ 00064 */ 00065 00066 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_TypesDefinitions STM32F769I-EVAL LOW LEVEL Private Types Definitions 00067 * @{ 00068 */ 00069 /** 00070 * @} 00071 */ 00072 00073 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_Defines STM32F769I-EVAL LOW LEVEL Private Defines 00074 * @{ 00075 */ 00076 /** 00077 * @brief STM32F769I EVAL BSP Driver version number V2.0.0 00078 */ 00079 #define __STM32F769I_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00080 #define __STM32F769I_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00081 #define __STM32F769I_EVAL_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00082 #define __STM32F769I_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00083 #define __STM32F769I_EVAL_BSP_VERSION ((__STM32F769I_EVAL_BSP_VERSION_MAIN << 24)\ 00084 |(__STM32F769I_EVAL_BSP_VERSION_SUB1 << 16)\ 00085 |(__STM32F769I_EVAL_BSP_VERSION_SUB2 << 8 )\ 00086 |(__STM32F769I_EVAL_BSP_VERSION_RC)) 00087 /** 00088 * @} 00089 */ 00090 00091 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_Macros STM32F769I-EVAL LOW LEVEL Private Macros 00092 * @{ 00093 */ 00094 /** 00095 * @} 00096 */ 00097 00098 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_Variables STM32F769I-EVAL LOW LEVEL Private Variables 00099 * @{ 00100 */ 00101 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED1_GPIO_PORT, 00102 LED2_GPIO_PORT, 00103 LED3_GPIO_PORT, 00104 LED4_GPIO_PORT}; 00105 00106 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00107 LED2_PIN, 00108 LED3_PIN, 00109 LED4_PIN}; 00110 00111 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00112 TAMPER_BUTTON_GPIO_PORT, 00113 KEY_BUTTON_GPIO_PORT}; 00114 00115 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00116 TAMPER_BUTTON_PIN, 00117 KEY_BUTTON_PIN}; 00118 00119 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00120 TAMPER_BUTTON_EXTI_IRQn, 00121 KEY_BUTTON_EXTI_IRQn}; 00122 00123 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00124 00125 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00126 00127 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00128 00129 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00130 00131 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00132 00133 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00134 00135 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00136 00137 static I2C_HandleTypeDef hEvalI2c; 00138 static ADC_HandleTypeDef hEvalADC; 00139 00140 /** 00141 * @} 00142 */ 00143 00144 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_FunctionPrototypes STM32F769I_EVAL LOW LEVEL Private Function Prototypes 00145 * @{ 00146 */ 00147 static void I2Cx_MspInit(void); 00148 static void I2Cx_Init(void); 00149 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00150 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg); 00151 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00152 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00153 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00154 static void I2Cx_Error(uint8_t Addr); 00155 00156 #if defined(USE_IOEXPANDER) 00157 /* IOExpander IO functions */ 00158 void IOE_Init(void); 00159 void IOE_ITConfig(void); 00160 void IOE_Delay(uint32_t Delay); 00161 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00162 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00163 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00164 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00165 00166 /* MFX IO functions */ 00167 void MFX_IO_Init(void); 00168 void MFX_IO_DeInit(void); 00169 void MFX_IO_ITConfig(void); 00170 void MFX_IO_Delay(uint32_t Delay); 00171 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value); 00172 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg); 00173 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00174 void MFX_IO_Wakeup(void); 00175 void MFX_IO_EnableWakeupPin(void); 00176 #endif /* USE_IOEXPANDER */ 00177 00178 /* AUDIO IO functions */ 00179 void AUDIO_IO_Init(void); 00180 void AUDIO_IO_DeInit(void); 00181 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00182 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00183 void AUDIO_IO_Delay(uint32_t Delay); 00184 00185 /* CAMERA IO functions */ 00186 void CAMERA_IO_Init(void); 00187 void CAMERA_Delay(uint32_t Delay); 00188 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00189 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg); 00190 00191 /* I2C EEPROM IO function */ 00192 void EEPROM_IO_Init(void); 00193 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00194 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00195 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00196 00197 /* TouchScreen (TS) IO functions */ 00198 void TS_IO_Init(void); 00199 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00200 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00201 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00202 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00203 void TS_IO_Delay(uint32_t Delay); 00204 00205 #if defined(USE_LCD_HDMI) 00206 /* HDMI IO functions */ 00207 void HDMI_IO_Init(void); 00208 void HDMI_IO_Delay(uint32_t Delay); 00209 void HDMI_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00210 uint8_t HDMI_IO_Read(uint8_t Addr, uint8_t Reg); 00211 #endif /* USE_LCD_HDMI */ 00212 00213 void OTM8009A_IO_Delay(uint32_t Delay); 00214 /** 00215 * @} 00216 */ 00217 00218 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_Functions STM32F769I_EVAL LOW LEVEL Private Functions 00219 * @{ 00220 */ 00221 00222 /** 00223 * @brief This method returns the STM32F769I EVAL BSP Driver revision 00224 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00225 */ 00226 uint32_t BSP_GetVersion(void) 00227 { 00228 return __STM32F769I_EVAL_BSP_VERSION; 00229 } 00230 00231 /** 00232 * @brief Configures LED on GPIO and/or on MFX. 00233 * @param Led: LED to be configured. 00234 * This parameter can be one of the following values: 00235 * @arg LED1 00236 * @arg LED2 00237 * @arg LED3 00238 * @arg LED4 00239 * @retval None 00240 */ 00241 void BSP_LED_Init(Led_TypeDef Led) 00242 { 00243 GPIO_InitTypeDef GPIO_InitStruct; 00244 00245 /* Enable the GPIO_LED clock */ 00246 LEDx_GPIO_CLK_ENABLE(Led); 00247 00248 /* Configure the GPIO_LED pin */ 00249 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00250 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00251 GPIO_InitStruct.Pull = GPIO_PULLUP; 00252 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00253 00254 HAL_GPIO_Init(GPIO_PORT[Led], &GPIO_InitStruct); 00255 00256 /* By default, turn off LED */ 00257 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00258 } 00259 00260 00261 /** 00262 * @brief DeInit LEDs. 00263 * @param Led: LED to be configured. 00264 * This parameter can be one of the following values: 00265 * @arg LED1 00266 * @arg LED2 00267 * @arg LED3 00268 * @arg LED4 00269 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00270 * @retval None 00271 */ 00272 void BSP_LED_DeInit(Led_TypeDef Led) 00273 { 00274 /* Turn off LED */ 00275 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00276 /* Configure the GPIO_LED pin */ 00277 HAL_GPIO_DeInit(GPIO_PORT[Led], GPIO_PIN[Led]); 00278 } 00279 00280 /** 00281 * @brief Turns selected LED On. 00282 * @param Led: LED to be set on 00283 * This parameter can be one of the following values: 00284 * @arg LED1 00285 * @arg LED2 00286 * @arg LED3 00287 * @arg LED4 00288 * @retval None 00289 */ 00290 void BSP_LED_On(Led_TypeDef Led) 00291 { 00292 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00293 } 00294 00295 /** 00296 * @brief Turns selected LED Off. 00297 * @param Led: LED to be set off 00298 * This parameter can be one of the following values: 00299 * @arg LED1 00300 * @arg LED2 00301 * @arg LED3 00302 * @arg LED4 00303 * @retval None 00304 */ 00305 void BSP_LED_Off(Led_TypeDef Led) 00306 { 00307 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00308 } 00309 00310 /** 00311 * @brief Toggles the selected LED. 00312 * @param Led: LED to be toggled 00313 * This parameter can be one of the following values: 00314 * @arg LED1 00315 * @arg LED2 00316 * @arg LED3 00317 * @arg LED4 00318 * @retval None 00319 */ 00320 void BSP_LED_Toggle(Led_TypeDef Led) 00321 { 00322 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00323 } 00324 00325 /** 00326 * @brief Configures button GPIO and EXTI Line. 00327 * @param Button: Button to be configured 00328 * This parameter can be one of the following values: 00329 * @arg BUTTON_WAKEUP: Wakeup Push Button 00330 * @arg BUTTON_TAMPER: Tamper Push Button 00331 * @arg BUTTON_KEY: Key Push Button 00332 * @param ButtonMode: Button mode 00333 * This parameter can be one of the following values: 00334 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00335 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00336 * with interrupt generation capability 00337 * @note On STM32F769I-EVAL evaluation board, the three buttons (Wakeup, Tamper 00338 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00339 * on the board serigraphy. 00340 * @retval None 00341 */ 00342 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00343 { 00344 GPIO_InitTypeDef GPIO_InitStruct; 00345 00346 /* Enable the BUTTON clock */ 00347 BUTTONx_GPIO_CLK_ENABLE(Button); 00348 00349 if(ButtonMode == BUTTON_MODE_GPIO) 00350 { 00351 /* Configure Button pin as input */ 00352 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00353 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00354 GPIO_InitStruct.Pull = GPIO_NOPULL; 00355 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00356 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00357 } 00358 00359 if(ButtonMode == BUTTON_MODE_EXTI) 00360 { 00361 /* Configure Button pin as input with External interrupt */ 00362 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00363 GPIO_InitStruct.Pull = GPIO_NOPULL; 00364 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00365 00366 if(Button != BUTTON_WAKEUP) 00367 { 00368 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00369 } 00370 else 00371 { 00372 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00373 } 00374 00375 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00376 00377 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00378 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00379 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00380 } 00381 } 00382 00383 /** 00384 * @brief Push Button DeInit. 00385 * @param Button: Button to be configured 00386 * This parameter can be one of the following values: 00387 * @arg BUTTON_WAKEUP: Wakeup Push Button 00388 * @arg BUTTON_TAMPER: Tamper Push Button 00389 * @arg BUTTON_KEY: Key Push Button 00390 * @note On STM32F769I-EVAL evaluation board, the three buttons (Wakeup, Tamper 00391 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00392 * on the board serigraphy. 00393 * @note PB DeInit does not disable the GPIO clock 00394 * @retval None 00395 */ 00396 void BSP_PB_DeInit(Button_TypeDef Button) 00397 { 00398 GPIO_InitTypeDef gpio_init_structure; 00399 00400 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00401 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00402 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00403 } 00404 00405 00406 /** 00407 * @brief Returns the selected button state. 00408 * @param Button: Button to be checked 00409 * This parameter can be one of the following values: 00410 * @arg BUTTON_WAKEUP: Wakeup Push Button 00411 * @arg BUTTON_TAMPER: Tamper Push Button 00412 * @arg BUTTON_KEY: Key Push Button 00413 * @note On STM32F769I-EVAL evaluation board, the three buttons (Wakeup, Tamper 00414 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00415 * on the board serigraphy. 00416 * @retval The Button GPIO pin value 00417 */ 00418 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00419 { 00420 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00421 } 00422 00423 /** 00424 * @brief Configures COM port. 00425 * @param COM: COM port to be configured. 00426 * This parameter can be one of the following values: 00427 * @arg COM1 00428 * @arg COM2 00429 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00430 * configuration information for the specified USART peripheral. 00431 * @retval None 00432 */ 00433 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00434 { 00435 GPIO_InitTypeDef gpio_init_structure; 00436 00437 /* Enable GPIO clock */ 00438 EVAL_COMx_TX_GPIO_CLK_ENABLE(COM); 00439 EVAL_COMx_RX_GPIO_CLK_ENABLE(COM); 00440 00441 /* Enable USART clock */ 00442 EVAL_COMx_CLK_ENABLE(COM); 00443 00444 /* Configure USART Tx as alternate function */ 00445 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00446 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00447 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00448 gpio_init_structure.Pull = GPIO_PULLUP; 00449 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00450 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00451 00452 /* Configure USART Rx as alternate function */ 00453 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00454 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00455 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00456 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00457 00458 /* USART configuration */ 00459 huart->Instance = COM_USART[COM]; 00460 HAL_UART_Init(huart); 00461 } 00462 00463 /** 00464 * @brief DeInit COM port. 00465 * @param COM: COM port to be configured. 00466 * This parameter can be one of the following values: 00467 * @arg COM1 00468 * @arg COM2 00469 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00470 * configuration information for the specified USART peripheral. 00471 * @retval None 00472 */ 00473 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00474 { 00475 /* USART configuration */ 00476 huart->Instance = COM_USART[COM]; 00477 HAL_UART_DeInit(huart); 00478 00479 /* Enable USART clock */ 00480 EVAL_COMx_CLK_DISABLE(COM); 00481 00482 /* DeInit GPIO pins can be done in the application 00483 (by surcharging this __weak function) */ 00484 00485 /* GPIO pins clock, DMA clock can be shut down in the application 00486 by surcharging this __weak function */ 00487 } 00488 00489 /** 00490 * @brief Init Potentiometer. 00491 * @retval None 00492 */ 00493 void BSP_POTENTIOMETER_Init(void) 00494 { 00495 GPIO_InitTypeDef GPIO_InitStruct; 00496 ADC_ChannelConfTypeDef ADC_Config; 00497 00498 /* ADC an GPIO Periph clock enable */ 00499 ADCx_CLK_ENABLE(); 00500 ADCx_CHANNEL_GPIO_CLK_ENABLE(); 00501 00502 /* ADC Channel GPIO pin configuration */ 00503 GPIO_InitStruct.Pin = ADCx_CHANNEL_PIN; 00504 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 00505 GPIO_InitStruct.Pull = GPIO_NOPULL; 00506 HAL_GPIO_Init(ADCx_CHANNEL_GPIO_PORT, &GPIO_InitStruct); 00507 00508 /* Configure the ADC peripheral */ 00509 hEvalADC.Instance = ADCx; 00510 00511 HAL_ADC_DeInit(&hEvalADC); 00512 00513 hEvalADC.Init.ClockPrescaler = ADC_CLOCKPRESCALER_PCLK_DIV4; /* Asynchronous clock mode, input ADC clock not divided */ 00514 hEvalADC.Init.Resolution = ADC_RESOLUTION_12B; /* 12-bit resolution for converted data */ 00515 hEvalADC.Init.DataAlign = ADC_DATAALIGN_RIGHT; /* Right-alignment for converted data */ 00516 hEvalADC.Init.ScanConvMode = DISABLE; /* Sequencer disabled (ADC conversion on only 1 channel: channel set on rank 1) */ 00517 hEvalADC.Init.EOCSelection = DISABLE; /* EOC flag picked-up to indicate conversion end */ 00518 hEvalADC.Init.ContinuousConvMode = DISABLE; /* Continuous mode disabled to have only 1 conversion at each conversion trig */ 00519 hEvalADC.Init.NbrOfConversion = 1; /* Parameter discarded because sequencer is disabled */ 00520 hEvalADC.Init.DiscontinuousConvMode = DISABLE; /* Parameter discarded because sequencer is disabled */ 00521 hEvalADC.Init.NbrOfDiscConversion = 0; /* Parameter discarded because sequencer is disabled */ 00522 hEvalADC.Init.ExternalTrigConv = ADC_EXTERNALTRIGCONV_T1_CC1; /* Software start to trig the 1st conversion manually, without external event */ 00523 hEvalADC.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; /* Parameter discarded because software trigger chosen */ 00524 hEvalADC.Init.DMAContinuousRequests = DISABLE; /* DMA one-shot mode selected */ 00525 00526 00527 HAL_ADC_Init(&hEvalADC); 00528 00529 /* Configure ADC regular channel */ 00530 ADC_Config.Channel = ADCx_CHANNEL; /* Sampled channel number */ 00531 ADC_Config.Rank = 1; /* Rank of sampled channel number ADCx_CHANNEL */ 00532 ADC_Config.SamplingTime = ADC_SAMPLETIME_3CYCLES; /* Sampling time (number of clock cycles unit) */ 00533 ADC_Config.Offset = 0; /* Parameter discarded because offset correction is disabled */ 00534 00535 HAL_ADC_ConfigChannel(&hEvalADC, &ADC_Config); 00536 } 00537 00538 /** 00539 * @brief Get Potentiometer level in 12 bits. 00540 * @retval Potentiometer level(0..0xFFF) / 0xFFFFFFFF : Error 00541 */ 00542 uint32_t BSP_POTENTIOMETER_GetLevel(void) 00543 { 00544 if(HAL_ADC_Start(&hEvalADC) == HAL_OK) 00545 { 00546 if(HAL_ADC_PollForConversion(&hEvalADC, ADCx_POLL_TIMEOUT)== HAL_OK) 00547 { 00548 return (HAL_ADC_GetValue(&hEvalADC)); 00549 } 00550 } 00551 return 0xFFFFFFFF; 00552 } 00553 00554 #if defined(USE_IOEXPANDER) 00555 /** 00556 * @brief Configures joystick GPIO and EXTI modes. 00557 * @param JoyMode: Button mode. 00558 * This parameter can be one of the following values: 00559 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00560 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00561 * with interrupt generation capability 00562 * @retval IO_OK: if all initializations are OK. Other value if error. 00563 */ 00564 uint8_t BSP_JOY_Init(JOYMode_TypeDef JoyMode) 00565 { 00566 uint8_t ret = 0; 00567 00568 /* Initialize the IO functionalities */ 00569 ret = BSP_IO_Init(); 00570 00571 /* Configure joystick pins in IT mode */ 00572 if(JoyMode == JOY_MODE_EXTI) 00573 { 00574 /* Configure IO interrupt acquisition mode */ 00575 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_LOW_LEVEL_PU); 00576 } 00577 else 00578 { 00579 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_INPUT_PU); 00580 } 00581 00582 return ret; 00583 } 00584 00585 00586 /** 00587 * @brief DeInit joystick GPIOs. 00588 * @note JOY DeInit does not disable the MFX, just set the MFX pins in Off mode 00589 * @retval None. 00590 */ 00591 void BSP_JOY_DeInit() 00592 { 00593 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_OFF); 00594 } 00595 00596 /** 00597 * @brief Returns the current joystick status. 00598 * @retval Code of the joystick key pressed 00599 * This code can be one of the following values: 00600 * @arg JOY_NONE 00601 * @arg JOY_SEL 00602 * @arg JOY_DOWN 00603 * @arg JOY_LEFT 00604 * @arg JOY_RIGHT 00605 * @arg JOY_UP 00606 */ 00607 JOYState_TypeDef BSP_JOY_GetState(void) 00608 { 00609 uint16_t pin_status = 0; 00610 00611 /* Read the status joystick pins */ 00612 pin_status = BSP_IO_ReadPin(JOY_ALL_PINS); 00613 00614 /* Check the pressed keys */ 00615 if((pin_status & JOY_NONE_PIN) == JOY_NONE) 00616 { 00617 return(JOYState_TypeDef) JOY_NONE; 00618 } 00619 else if(!(pin_status & JOY_SEL_PIN)) 00620 { 00621 return(JOYState_TypeDef) JOY_SEL; 00622 } 00623 else if(!(pin_status & JOY_DOWN_PIN)) 00624 { 00625 return(JOYState_TypeDef) JOY_DOWN; 00626 } 00627 else if(!(pin_status & JOY_LEFT_PIN)) 00628 { 00629 return(JOYState_TypeDef) JOY_LEFT; 00630 } 00631 else if(!(pin_status & JOY_RIGHT_PIN)) 00632 { 00633 return(JOYState_TypeDef) JOY_RIGHT; 00634 } 00635 else if(!(pin_status & JOY_UP_PIN)) 00636 { 00637 return(JOYState_TypeDef) JOY_UP; 00638 } 00639 else 00640 { 00641 return(JOYState_TypeDef) JOY_NONE; 00642 } 00643 } 00644 00645 #endif /* USE_IOEXPANDER */ 00646 00647 /******************************************************************************* 00648 BUS OPERATIONS 00649 *******************************************************************************/ 00650 00651 /******************************* I2C Routines *********************************/ 00652 /** 00653 * @brief Initializes I2C MSP. 00654 * @retval None 00655 */ 00656 static void I2Cx_MspInit(void) 00657 { 00658 GPIO_InitTypeDef gpio_init_structure; 00659 00660 /*** Configure the GPIOs ***/ 00661 /* Enable GPIO clock */ 00662 EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00663 00664 /* Configure I2C Tx as alternate function */ 00665 gpio_init_structure.Pin = EVAL_I2Cx_SCL_PIN; 00666 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00667 gpio_init_structure.Pull = GPIO_NOPULL; 00668 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00669 gpio_init_structure.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00670 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00671 00672 /* Configure I2C Rx as alternate function */ 00673 gpio_init_structure.Pin = EVAL_I2Cx_SDA_PIN; 00674 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00675 00676 /*** Configure the I2C peripheral ***/ 00677 /* Enable I2C clock */ 00678 EVAL_I2Cx_CLK_ENABLE(); 00679 00680 /* Force the I2C peripheral clock reset */ 00681 EVAL_I2Cx_FORCE_RESET(); 00682 00683 /* Release the I2C peripheral clock reset */ 00684 EVAL_I2Cx_RELEASE_RESET(); 00685 00686 /* Enable and set I2Cx Interrupt to a lower priority */ 00687 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x0F, 0); 00688 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00689 00690 /* Enable and set I2Cx Interrupt to a lower priority */ 00691 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x0F, 0); 00692 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00693 } 00694 00695 /** 00696 * @brief Initializes I2C HAL. 00697 * @retval None 00698 */ 00699 static void I2Cx_Init(void) 00700 { 00701 if(HAL_I2C_GetState(&hEvalI2c) == HAL_I2C_STATE_RESET) 00702 { 00703 hEvalI2c.Instance = EVAL_I2Cx; 00704 hEvalI2c.Init.Timing = EVAL_I2Cx_TIMING; 00705 hEvalI2c.Init.OwnAddress1 = 0; 00706 hEvalI2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00707 hEvalI2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00708 hEvalI2c.Init.OwnAddress2 = 0; 00709 hEvalI2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00710 hEvalI2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00711 00712 /* Init the I2C */ 00713 I2Cx_MspInit(); 00714 HAL_I2C_Init(&hEvalI2c); 00715 } 00716 } 00717 00718 /** 00719 * @brief Writes a single data. 00720 * @param Addr: I2C address 00721 * @param Reg: Register address 00722 * @param Value: Data to be written 00723 * @retval None 00724 */ 00725 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00726 { 00727 HAL_StatusTypeDef status = HAL_OK; 00728 00729 status = HAL_I2C_Mem_Write(&hEvalI2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00730 00731 /* Check the communication status */ 00732 if(status != HAL_OK) 00733 { 00734 /* Execute user timeout callback */ 00735 I2Cx_Error(Addr); 00736 } 00737 } 00738 00739 /** 00740 * @brief Reads a single data. 00741 * @param Addr: I2C address 00742 * @param Reg: Register address 00743 * @retval Read data 00744 */ 00745 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg) 00746 { 00747 HAL_StatusTypeDef status = HAL_OK; 00748 uint8_t Value = 0; 00749 00750 status = HAL_I2C_Mem_Read(&hEvalI2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00751 00752 /* Check the communication status */ 00753 if(status != HAL_OK) 00754 { 00755 /* Execute user timeout callback */ 00756 I2Cx_Error(Addr); 00757 } 00758 return Value; 00759 } 00760 00761 /** 00762 * @brief Reads multiple data. 00763 * @param Addr: I2C address 00764 * @param Reg: Reg address 00765 * @param MemAddress: internal memory address 00766 * @param Buffer: Pointer to data buffer 00767 * @param Length: Length of the data 00768 * @retval Number of read data 00769 */ 00770 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00771 { 00772 HAL_StatusTypeDef status = HAL_OK; 00773 00774 status = HAL_I2C_Mem_Read(&hEvalI2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00775 00776 /* Check the communication status */ 00777 if(status != HAL_OK) 00778 { 00779 /* I2C error occurred */ 00780 I2Cx_Error(Addr); 00781 } 00782 return status; 00783 } 00784 00785 /** 00786 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00787 * @param Addr: Device address on BUS Bus. 00788 * @param Reg: The target register address to write 00789 * @param MemAddress: internal memory address 00790 * @param Buffer: The target register value to be written 00791 * @param Length: buffer size to be written 00792 * @retval HAL status 00793 */ 00794 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00795 { 00796 HAL_StatusTypeDef status = HAL_OK; 00797 00798 status = HAL_I2C_Mem_Write(&hEvalI2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00799 00800 /* Check the communication status */ 00801 if(status != HAL_OK) 00802 { 00803 /* Re-Initiaize the I2C Bus */ 00804 I2Cx_Error(Addr); 00805 } 00806 return status; 00807 } 00808 00809 /** 00810 * @brief Checks if target device is ready for communication. 00811 * @note This function is used with Memory devices 00812 * @param DevAddress: Target device address 00813 * @param Trials: Number of trials 00814 * @retval HAL status 00815 */ 00816 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00817 { 00818 return (HAL_I2C_IsDeviceReady(&hEvalI2c, DevAddress, Trials, 1000)); 00819 } 00820 00821 /** 00822 * @brief Manages error callback by re-initializing I2C. 00823 * @param Addr: I2C Address 00824 * @retval None 00825 */ 00826 static void I2Cx_Error(uint8_t Addr) 00827 { 00828 /* De-initialize the I2C communication bus */ 00829 HAL_I2C_DeInit(&hEvalI2c); 00830 00831 /* Re-Initialize the I2C communication bus */ 00832 I2Cx_Init(); 00833 } 00834 00835 /******************************************************************************* 00836 LINK OPERATIONS 00837 *******************************************************************************/ 00838 00839 /********************************* LINK IOE ***********************************/ 00840 #if defined(USE_IOEXPANDER) 00841 /** 00842 * @brief Initializes IOE low level. 00843 * @retval None 00844 */ 00845 void IOE_Init(void) 00846 { 00847 I2Cx_Init(); 00848 } 00849 00850 /** 00851 * @brief Configures IOE low level interrupt. 00852 * @retval None 00853 */ 00854 void IOE_ITConfig(void) 00855 { 00856 /* IO expander IT config done by BSP_TS_ITConfig function */ 00857 } 00858 00859 /** 00860 * @brief IOE writes single data. 00861 * @param Addr: I2C address 00862 * @param Reg: Register address 00863 * @param Value: Data to be written 00864 * @retval None 00865 */ 00866 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00867 { 00868 I2Cx_Write(Addr, Reg, Value); 00869 } 00870 00871 /** 00872 * @brief IOE reads single data. 00873 * @param Addr: I2C address 00874 * @param Reg: Register address 00875 * @retval Read data 00876 */ 00877 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 00878 { 00879 return I2Cx_Read(Addr, Reg); 00880 } 00881 00882 /** 00883 * @brief IOE reads multiple data. 00884 * @param Addr: I2C address 00885 * @param Reg: Register address 00886 * @param Buffer: Pointer to data buffer 00887 * @param Length: Length of the data 00888 * @retval Number of read data 00889 */ 00890 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00891 { 00892 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00893 } 00894 00895 /** 00896 * @brief IOE writes multiple data. 00897 * @param Addr: I2C address 00898 * @param Reg: Register address 00899 * @param Buffer: Pointer to data buffer 00900 * @param Length: Length of the data 00901 * @retval None 00902 */ 00903 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00904 { 00905 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00906 } 00907 00908 /** 00909 * @brief IOE delay 00910 * @param Delay: Delay in ms 00911 * @retval None 00912 */ 00913 void IOE_Delay(uint32_t Delay) 00914 { 00915 HAL_Delay(Delay); 00916 } 00917 #endif /* USE_IOEXPANDER */ 00918 00919 /********************************* LINK MFX ***********************************/ 00920 00921 #if defined(USE_IOEXPANDER) 00922 /** 00923 * @brief Initializes MFX low level. 00924 * @retval None 00925 */ 00926 void MFX_IO_Init(void) 00927 { 00928 I2Cx_Init(); 00929 } 00930 00931 /** 00932 * @brief DeInitializes MFX low level. 00933 * @retval None 00934 */ 00935 void MFX_IO_DeInit(void) 00936 { 00937 } 00938 00939 /** 00940 * @brief Configures MFX low level interrupt. 00941 * @retval None 00942 */ 00943 void MFX_IO_ITConfig(void) 00944 { 00945 static uint8_t mfx_io_it_enabled = 0; 00946 GPIO_InitTypeDef gpio_init_structure; 00947 00948 if(mfx_io_it_enabled == 0) 00949 { 00950 mfx_io_it_enabled = 1; 00951 /* Enable the GPIO EXTI clock */ 00952 __HAL_RCC_GPIOI_CLK_ENABLE(); 00953 __HAL_RCC_SYSCFG_CLK_ENABLE(); 00954 00955 gpio_init_structure.Pin = GPIO_PIN_8; 00956 gpio_init_structure.Pull = GPIO_NOPULL; 00957 gpio_init_structure.Speed = GPIO_SPEED_FREQ_LOW; 00958 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00959 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 00960 00961 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 00962 HAL_NVIC_SetPriority((IRQn_Type)(EXTI9_5_IRQn), 0x0F, 0x0F); 00963 HAL_NVIC_EnableIRQ((IRQn_Type)(EXTI9_5_IRQn)); 00964 } 00965 } 00966 00967 /** 00968 * @brief MFX writes single data. 00969 * @param Addr: I2C address 00970 * @param Reg: Register address 00971 * @param Value: Data to be written 00972 * @retval None 00973 */ 00974 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 00975 { 00976 I2Cx_Write((uint8_t) Addr, Reg, Value); 00977 } 00978 00979 /** 00980 * @brief MFX reads single data. 00981 * @param Addr: I2C address 00982 * @param Reg: Register address 00983 * @retval Read data 00984 */ 00985 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 00986 { 00987 return I2Cx_Read((uint8_t) Addr, Reg); 00988 } 00989 00990 /** 00991 * @brief MFX reads multiple data. 00992 * @param Addr: I2C address 00993 * @param Reg: Register address 00994 * @param Buffer: Pointer to data buffer 00995 * @param Length: Length of the data 00996 * @retval Number of read data 00997 */ 00998 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00999 { 01000 return I2Cx_ReadMultiple((uint8_t)Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01001 } 01002 01003 /** 01004 * @brief MFX delay 01005 * @param Delay: Delay in ms 01006 * @retval None 01007 */ 01008 void MFX_IO_Delay(uint32_t Delay) 01009 { 01010 HAL_Delay(Delay); 01011 } 01012 01013 /** 01014 * @brief Used by Lx family but requested for MFX component compatibility. 01015 * @retval None 01016 */ 01017 void MFX_IO_Wakeup(void) 01018 { 01019 } 01020 01021 /** 01022 * @brief Used by Lx family but requested for MXF component compatibility. 01023 * @retval None 01024 */ 01025 void MFX_IO_EnableWakeupPin(void) 01026 { 01027 } 01028 01029 #endif /* USE_IOEXPANDER */ 01030 01031 /********************************* LINK AUDIO *********************************/ 01032 01033 /** 01034 * @brief Initializes Audio low level. 01035 * @retval None 01036 */ 01037 void AUDIO_IO_Init(void) 01038 { 01039 I2Cx_Init(); 01040 } 01041 01042 /** 01043 * @brief Deinitializes Audio low level. 01044 * @retval None 01045 */ 01046 void AUDIO_IO_DeInit(void) 01047 { 01048 } 01049 01050 /** 01051 * @brief Writes a single data. 01052 * @param Addr: I2C address 01053 * @param Reg: Reg address 01054 * @param Value: Data to be written 01055 * @retval None 01056 */ 01057 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01058 { 01059 uint16_t tmp = Value; 01060 01061 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01062 01063 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01064 01065 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01066 } 01067 01068 /** 01069 * @brief Reads a single data. 01070 * @param Addr: I2C address 01071 * @param Reg: Reg address 01072 * @retval Data to be read 01073 */ 01074 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 01075 { 01076 uint16_t read_value = 0, tmp = 0; 01077 01078 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01079 01080 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01081 01082 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01083 01084 read_value = tmp; 01085 01086 return read_value; 01087 } 01088 01089 /** 01090 * @brief AUDIO Codec delay 01091 * @param Delay: Delay in ms 01092 * @retval None 01093 */ 01094 void AUDIO_IO_Delay(uint32_t Delay) 01095 { 01096 HAL_Delay(Delay); 01097 } 01098 01099 /********************************* LINK CAMERA ********************************/ 01100 01101 /** 01102 * @brief Initializes Camera low level. 01103 * @retval None 01104 */ 01105 void CAMERA_IO_Init(void) 01106 { 01107 I2Cx_Init(); 01108 } 01109 01110 /** 01111 * @brief Camera writes single data. 01112 * @param Addr: I2C address 01113 * @param Reg: Register address 01114 * @param Value: Data to be written 01115 * @retval None 01116 */ 01117 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01118 { 01119 uint16_t tmp = Value; 01120 /* For S5K5CAG sensor, 16 bits accesses are used */ 01121 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01122 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01123 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01124 } 01125 01126 /** 01127 * @brief Camera reads single data. 01128 * @param Addr: I2C address 01129 * @param Reg: Register address 01130 * @retval Read data 01131 */ 01132 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg) 01133 { 01134 uint16_t read_value = 0, tmp = 0; 01135 /* For S5K5CAG sensor, 16 bits accesses are used */ 01136 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01137 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01138 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01139 read_value = tmp; 01140 return read_value; 01141 } 01142 01143 /** 01144 * @brief Camera delay 01145 * @param Delay: Delay in ms 01146 * @retval None 01147 */ 01148 void CAMERA_Delay(uint32_t Delay) 01149 { 01150 HAL_Delay(Delay); 01151 } 01152 01153 /******************************** LINK I2C EEPROM *****************************/ 01154 01155 /** 01156 * @brief Initializes peripherals used by the I2C EEPROM driver. 01157 * @retval None 01158 */ 01159 void EEPROM_IO_Init(void) 01160 { 01161 I2Cx_Init(); 01162 } 01163 01164 /** 01165 * @brief Write data to I2C EEPROM driver in using DMA channel. 01166 * @param DevAddress: Target device address 01167 * @param MemAddress: Internal memory address 01168 * @param pBuffer: Pointer to data buffer 01169 * @param BufferSize: Amount of data to be sent 01170 * @retval HAL status 01171 */ 01172 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01173 { 01174 return (I2Cx_WriteMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01175 } 01176 01177 /** 01178 * @brief Read data from I2C EEPROM driver in using DMA channel. 01179 * @param DevAddress: Target device address 01180 * @param MemAddress: Internal memory address 01181 * @param pBuffer: Pointer to data buffer 01182 * @param BufferSize: Amount of data to be read 01183 * @retval HAL status 01184 */ 01185 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01186 { 01187 return (I2Cx_ReadMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01188 } 01189 01190 /** 01191 * @brief Checks if target device is ready for communication. 01192 * @note This function is used with Memory devices 01193 * @param DevAddress: Target device address 01194 * @param Trials: Number of trials 01195 * @retval HAL status 01196 */ 01197 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01198 { 01199 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01200 } 01201 01202 /******************************** LINK TS (TouchScreen) *****************************/ 01203 01204 /** 01205 * @brief Initialize I2C communication 01206 * channel from MCU to TouchScreen (TS). 01207 */ 01208 void TS_IO_Init(void) 01209 { 01210 I2Cx_Init(); 01211 } 01212 01213 /** 01214 * @brief Writes single data with I2C communication 01215 * channel from MCU to TouchScreen. 01216 * @param Addr: I2C address 01217 * @param Reg: Register address 01218 * @param Value: Data to be written 01219 */ 01220 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01221 { 01222 I2Cx_Write(Addr, Reg, Value); 01223 } 01224 01225 /** 01226 * @brief Reads single data with I2C communication 01227 * channel from TouchScreen. 01228 * @param Addr: I2C address 01229 * @param Reg: Register address 01230 * @retval Read data 01231 */ 01232 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 01233 { 01234 return I2Cx_Read(Addr, Reg); 01235 } 01236 01237 /** 01238 * @brief Reads multiple data with I2C communication 01239 * channel from TouchScreen. 01240 * @param Addr: I2C address 01241 * @param Reg: Register address 01242 * @param Buffer: Pointer to data buffer 01243 * @param Length: Length of the data 01244 * @retval Number of read data 01245 */ 01246 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01247 { 01248 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01249 } 01250 01251 /** 01252 * @brief Writes multiple data with I2C communication 01253 * channel from MCU to TouchScreen. 01254 * @param Addr: I2C address 01255 * @param Reg: Register address 01256 * @param Buffer: Pointer to data buffer 01257 * @param Length: Length of the data 01258 * @retval None 01259 */ 01260 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01261 { 01262 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01263 } 01264 01265 /** 01266 * @brief Delay function used in TouchScreen low level driver. 01267 * @param Delay: Delay in ms 01268 * @retval None 01269 */ 01270 void TS_IO_Delay(uint32_t Delay) 01271 { 01272 HAL_Delay(Delay); 01273 } 01274 01275 /**************************** LINK OTM8009A (Display driver) ******************/ 01276 /** 01277 * @brief OTM8009A delay 01278 * @param Delay: Delay in ms 01279 */ 01280 void OTM8009A_IO_Delay(uint32_t Delay) 01281 { 01282 HAL_Delay(Delay); 01283 } 01284 01285 #if defined(USE_LCD_HDMI) 01286 /**************************** LINK ADV7533 DSI-HDMI (Display driver) **********/ 01287 /** 01288 * @brief Initializes HDMI IO low level. 01289 * @retval None 01290 */ 01291 void HDMI_IO_Init(void) 01292 { 01293 I2Cx_Init(); 01294 } 01295 01296 /** 01297 * @brief HDMI writes single data. 01298 * @param Addr: I2C address 01299 * @param Reg: Register address 01300 * @param Value: Data to be written 01301 * @retval None 01302 */ 01303 void HDMI_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01304 { 01305 I2Cx_Write(Addr, Reg, Value); 01306 } 01307 01308 /** 01309 * @brief Reads single data with I2C communication 01310 * channel from HDMI bridge. 01311 * @param Addr: I2C address 01312 * @param Reg: Register address 01313 * @retval Read data 01314 */ 01315 uint8_t HDMI_IO_Read(uint8_t Addr, uint8_t Reg) 01316 { 01317 return I2Cx_Read(Addr, Reg); 01318 } 01319 01320 /** 01321 * @brief HDMI delay 01322 * @param Delay: Delay in ms 01323 * @retval None 01324 */ 01325 void HDMI_IO_Delay(uint32_t Delay) 01326 { 01327 HAL_Delay(Delay); 01328 } 01329 #endif /* USE_LCD_HDMI */ 01330 01331 /** 01332 * @} 01333 */ 01334 01335 /** 01336 * @} 01337 */ 01338 01339 /** 01340 * @} 01341 */ 01342 01343 /** 01344 * @} 01345 */ 01346 01347 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 20:55:35 for STM32769I_EVAL BSP User Manual by
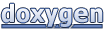