STM324xG_EVAL BSP User Manual
|
stm324xg_eval_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324xg_eval_ts.c 00004 * @author MCD Application Team 00005 * @version V2.2.1 00006 * @date 15-January-2016 00007 * @brief This file provides a set of functions needed to manage the touch 00008 * screen on STM324xG-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the touch screen module of the STM324xG-EVAL 00044 evaluation board on the ILI9325 LCD mounted on MB785 daughter board . 00045 - The STMPE811 IO expander device component driver must be included with this 00046 driver in order to run the TS module commanded by the IO expander device 00047 mounted on the evaluation board. 00048 00049 2. Driver description: 00050 --------------------- 00051 + Initialization steps: 00052 o Initialize the TS module using the BSP_TS_Init() function. This 00053 function includes the MSP layer hardware resources initialization and the 00054 communication layer configuration to start the TS use. The LCD size properties 00055 (x and y) are passed as parameters. 00056 o If TS interrupt mode is desired, you must configure the TS interrupt mode 00057 by calling the function BSP_TS_ITConfig(). The TS interrupt mode is generated 00058 as an external interrupt whenever a touch is detected. 00059 00060 + Touch screen use 00061 o The touch screen state is captured whenever the function BSP_TS_GetState() is 00062 used. This function returns information about the last LCD touch occurred 00063 in the TS_StateTypeDef structure. 00064 o If TS interrupt mode is used, the function BSP_TS_ITGetStatus() is needed to get 00065 the interrupt status. To clear the IT pending bits, you should call the 00066 function BSP_TS_ITClear(). 00067 o The IT is handled using the corresponding external interrupt IRQ handler, 00068 the user IT callback treatment is implemented on the same external interrupt 00069 callback. 00070 00071 ------------------------------------------------------------------------------*/ 00072 00073 /* Includes ------------------------------------------------------------------*/ 00074 #include "stm324xg_eval_ts.h" 00075 00076 /** @addtogroup BSP 00077 * @{ 00078 */ 00079 00080 /** @addtogroup STM324xG_EVAL 00081 * @{ 00082 */ 00083 00084 /** @defgroup STM324xG_EVAL_TS STM324xG EVAL TS 00085 * @{ 00086 */ 00087 00088 /** @defgroup STM324xG_EVAL_TS_Private_Types_Definitions STM324xG EVAL TS Private Types Definitions 00089 * @{ 00090 */ 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM324xG_EVAL_TS_Private_Defines STM324xG EVAL TS Private Defines 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM324xG_EVAL_TS_Private_Macros STM324xG EVAL TS Private Macros 00103 * @{ 00104 */ 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM324xG_EVAL_TS_Private_Variables STM324xG EVAL TS Private Variables 00110 * @{ 00111 */ 00112 static TS_DrvTypeDef *ts_driver; 00113 static uint16_t ts_x_boundary, ts_y_boundary; 00114 static uint8_t ts_orientation; 00115 /** 00116 * @} 00117 */ 00118 00119 /** @defgroup STM324xG_EVAL_TS_Private_Function_Prototypes STM324xG EVAL TS Private Function Prototypes 00120 * @{ 00121 */ 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM324xG_EVAL_TS_Private_Functions STM324xG EVAL TS Private Functions 00127 * @{ 00128 */ 00129 00130 /** 00131 * @brief Initializes and configures the touch screen functionalities and 00132 * configures all necessary hardware resources (GPIOs, clocks..). 00133 * @param xSize: Maximum X size of the TS area on LCD 00134 * @param ySize: Maximum Y size of the TS area on LCD 00135 * @retval TS_OK if all initializations are OK. Other value if error. 00136 */ 00137 uint8_t BSP_TS_Init(uint16_t xSize, uint16_t ySize) 00138 { 00139 uint8_t ret = TS_ERROR; 00140 00141 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 00142 { 00143 /* Initialize the TS driver structure */ 00144 ts_driver = &stmpe811_ts_drv; 00145 00146 /* Initialize x and y positions boundaries */ 00147 ts_x_boundary = xSize; 00148 ts_y_boundary = ySize; 00149 ts_orientation = TS_SWAP_XY; 00150 ret = TS_OK; 00151 } 00152 00153 if(ret == TS_OK) 00154 { 00155 /* Initialize the LL TS Driver */ 00156 ts_driver->Init(TS_I2C_ADDRESS); 00157 ts_driver->Start(TS_I2C_ADDRESS); 00158 } 00159 00160 return ret; 00161 } 00162 00163 /** 00164 * @brief Configures and enables the touch screen interrupts. 00165 * @retval TS_OK if all initializations are OK. Other value if error. 00166 */ 00167 uint8_t BSP_TS_ITConfig(void) 00168 { 00169 /* Call component driver to enable TS ITs */ 00170 ts_driver->EnableIT(TS_I2C_ADDRESS); 00171 00172 return TS_OK; 00173 } 00174 00175 /** 00176 * @brief Gets the touch screen interrupt status. 00177 * @retval TS_OK if all initializations are OK. Other value if error. 00178 */ 00179 uint8_t BSP_TS_ITGetStatus(void) 00180 { 00181 /* Call component driver to enable TS ITs */ 00182 return (ts_driver->GetITStatus(TS_I2C_ADDRESS)); 00183 } 00184 00185 /** 00186 * @brief Returns status and positions of the touch screen. 00187 * @param TS_State: Pointer to touch screen current state structure 00188 * @retval TS_OK if all initializations are OK. Other value if error. 00189 */ 00190 uint8_t BSP_TS_GetState(TS_StateTypeDef *TS_State) 00191 { 00192 static uint32_t _x = 0, _y = 0; 00193 uint16_t xDiff, yDiff , x , y; 00194 uint16_t swap; 00195 00196 TS_State->TouchDetected = ts_driver->DetectTouch(TS_I2C_ADDRESS); 00197 00198 if(TS_State->TouchDetected) 00199 { 00200 ts_driver->GetXY(TS_I2C_ADDRESS, &x, &y); 00201 00202 if(ts_orientation & TS_SWAP_X) 00203 { 00204 x = 4096 - x; 00205 } 00206 00207 if(ts_orientation & TS_SWAP_Y) 00208 { 00209 y = 4096 - y; 00210 } 00211 00212 if(ts_orientation & TS_SWAP_XY) 00213 { 00214 swap = y; 00215 y = x; 00216 x = swap; 00217 } 00218 00219 xDiff = x > _x? (x - _x): (_x - x); 00220 yDiff = y > _y? (y - _y): (_y - y); 00221 00222 if (xDiff + yDiff > 5) 00223 { 00224 _x = x; 00225 _y = y; 00226 } 00227 00228 TS_State->x = (ts_x_boundary * _x) >> 12; 00229 TS_State->y = (ts_y_boundary * _y) >> 12; 00230 } 00231 00232 return TS_OK; 00233 } 00234 00235 /** 00236 * @brief Clears all touch screen interrupts. 00237 */ 00238 void BSP_TS_ITClear(void) 00239 { 00240 ts_driver->ClearIT(TS_I2C_ADDRESS); 00241 } 00242 00243 /** 00244 * @} 00245 */ 00246 00247 /** 00248 * @} 00249 */ 00250 00251 /** 00252 * @} 00253 */ 00254 00255 /** 00256 * @} 00257 */ 00258 00259 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 14:22:29 for STM324xG_EVAL BSP User Manual by
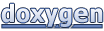