STM324xG_EVAL BSP User Manual
|
stm324xg_eval_sram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324xg_eval_sram.c 00004 * @author MCD Application Team 00005 * @version V2.2.1 00006 * @date 15-January-2016 00007 * @brief This file includes the SRAM driver for the IS61WV102416BLL-10MLI memory 00008 * device mounted on STM324xG-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the IS61WV102416BLL-10MLI SRAM external memory mounted 00044 on STM324xG-EVAL evaluation board. 00045 - This driver does not need a specific component driver for the SRAM device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the SRAM external memory using the BSP_SRAM_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 FMC controller configuration to interface with the external SRAM memory. 00054 00055 + SRAM read/write operations 00056 o SRAM external memory can be accessed with read/write operations once it is 00057 initialized. 00058 Read/write operation can be performed with AHB access using the functions 00059 BSP_SRAM_ReadData()/BSP_SRAM_WriteData(), or by DMA transfer using the functions 00060 BSP_SRAM_ReadData_DMA()/BSP_SRAM_WriteData_DMA(). 00061 o The AHB access is performed with 16-bit width transaction, the DMA transfer 00062 configuration is fixed at single (no burst) halfword transfer 00063 (see the SRAM_MspInit() static function). 00064 o User can implement his own functions for read/write access with his desired 00065 configurations. 00066 o If interrupt mode is used for DMA transfer, the function BSP_SRAM_DMA_IRQHandler() 00067 is called in IRQ handler file, to serve the generated interrupt once the DMA 00068 transfer is complete. 00069 00070 ------------------------------------------------------------------------------*/ 00071 00072 /* Includes ------------------------------------------------------------------*/ 00073 #include "stm324xg_eval_sram.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM324xG_EVAL 00080 * @{ 00081 */ 00082 00083 /** @defgroup STM324xG_EVAL_SRAM STM324xG EVAL SRAM 00084 * @{ 00085 */ 00086 00087 /** @defgroup STM324xG_EVAL_SRAM_Private_Types_Definitions STM324xG EVAL SRAM Private Types Definitions 00088 * @{ 00089 */ 00090 /** 00091 * @} 00092 */ 00093 00094 /** @defgroup STM324xG_EVAL_SRAM_Private_Defines STM324xG EVAL SRAM Private Defines 00095 * @{ 00096 */ 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM324xG_EVAL_SRAM_Private_Macros STM324xG EVAL SRAM Private Macros 00102 * @{ 00103 */ 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM324xG_EVAL_SRAM_Private_Variables STM324xG EVAL SRAM Private Variables 00109 * @{ 00110 */ 00111 static SRAM_HandleTypeDef sramHandle; 00112 static FMC_NORSRAM_TimingTypeDef Timing; 00113 /** 00114 * @} 00115 */ 00116 00117 /** @defgroup STM324xG_EVAL_SRAM_Private_Function_Prototypes STM324xG EVAL SRAM Private Function Prototypes 00118 * @{ 00119 */ 00120 static void SRAM_MspInit(void); 00121 /** 00122 * @} 00123 */ 00124 00125 /** @defgroup STM324xG_EVAL_SRAM_Private_Functions STM324xG EVAL SRAM Private Functions 00126 * @{ 00127 */ 00128 00129 /** 00130 * @brief Initializes the SRAM device. 00131 * @retval SRAM status 00132 */ 00133 uint8_t BSP_SRAM_Init(void) 00134 { 00135 sramHandle.Instance = FMC_NORSRAM_DEVICE; 00136 sramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00137 00138 /* SRAM device configuration */ 00139 Timing.AddressSetupTime = 2; 00140 Timing.AddressHoldTime = 1; 00141 Timing.DataSetupTime = 2; 00142 Timing.BusTurnAroundDuration = 1; 00143 Timing.CLKDivision = 2; 00144 Timing.DataLatency = 2; 00145 Timing.AccessMode = FSMC_ACCESS_MODE_A; 00146 00147 sramHandle.Init.NSBank = FSMC_NORSRAM_BANK2; 00148 sramHandle.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00149 sramHandle.Init.MemoryType = FSMC_MEMORY_TYPE_SRAM; 00150 sramHandle.Init.MemoryDataWidth = SRAM_MEMORY_WIDTH; 00151 sramHandle.Init.BurstAccessMode = SRAM_BURSTACCESS; 00152 sramHandle.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00153 sramHandle.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00154 sramHandle.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00155 sramHandle.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00156 sramHandle.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00157 sramHandle.Init.ExtendedMode = FSMC_EXTENDED_MODE_DISABLE; 00158 sramHandle.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00159 sramHandle.Init.WriteBurst = SRAM_WRITEBURST; 00160 00161 /* SRAM controller initialization */ 00162 SRAM_MspInit(); 00163 if(HAL_SRAM_Init(&sramHandle, &Timing, &Timing) != HAL_OK) 00164 { 00165 return SRAM_ERROR; 00166 } 00167 else 00168 { 00169 return SRAM_OK; 00170 } 00171 } 00172 00173 /** 00174 * @brief Reads an amount of data from the SRAM device in polling mode. 00175 * @param uwStartAddress : Read start address 00176 * @param pData: Pointer to data to be read 00177 * @param uwDataSize: Size of read data from the memory 00178 * @retval SRAM status 00179 */ 00180 uint8_t BSP_SRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00181 { 00182 if(HAL_SRAM_Read_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00183 { 00184 return SRAM_ERROR; 00185 } 00186 else 00187 { 00188 return SRAM_OK; 00189 } 00190 } 00191 00192 /** 00193 * @brief Reads an amount of data from the SRAM device in DMA mode. 00194 * @param uwStartAddress : Read start address 00195 * @param pData: Pointer to data to be read 00196 * @param uwDataSize: Size of read data from the memory 00197 * @retval SRAM status 00198 */ 00199 uint8_t BSP_SRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00200 { 00201 if(HAL_SRAM_Read_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00202 { 00203 return SRAM_ERROR; 00204 } 00205 else 00206 { 00207 return SRAM_OK; 00208 } 00209 } 00210 00211 /** 00212 * @brief Writes an amount of data from the SRAM device in polling mode. 00213 * @param uwStartAddress: Write start address 00214 * @param pData: Pointer to data to be written 00215 * @param uwDataSize: Size of written data from the memory 00216 * @retval SRAM status 00217 */ 00218 uint8_t BSP_SRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00219 { 00220 if(HAL_SRAM_Write_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00221 { 00222 return SRAM_ERROR; 00223 } 00224 else 00225 { 00226 return SRAM_OK; 00227 } 00228 } 00229 00230 /** 00231 * @brief Writes an amount of data from the SRAM device in DMA mode. 00232 * @param uwStartAddress: Write start address 00233 * @param pData: Pointer to data to be written 00234 * @param uwDataSize: Size of written data from the memory 00235 * @retval SRAM status 00236 */ 00237 uint8_t BSP_SRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00238 { 00239 if(HAL_SRAM_Write_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00240 { 00241 return SRAM_ERROR; 00242 } 00243 else 00244 { 00245 return SRAM_OK; 00246 } 00247 } 00248 00249 /** 00250 * @brief Handles SRAM DMA transfer interrupt request. 00251 */ 00252 void BSP_SRAM_DMA_IRQHandler(void) 00253 { 00254 HAL_DMA_IRQHandler(sramHandle.hdma); 00255 } 00256 00257 /** 00258 * @brief Initializes SRAM MSP. 00259 */ 00260 static void SRAM_MspInit(void) 00261 { 00262 static DMA_HandleTypeDef dmaHandle; 00263 GPIO_InitTypeDef GPIO_Init_Structure; 00264 SRAM_HandleTypeDef *hsram = &sramHandle; 00265 00266 /* Enable FMC clock */ 00267 __FSMC_CLK_ENABLE(); 00268 00269 /* Enable chosen DMAx clock */ 00270 __SRAM_DMAx_CLK_ENABLE(); 00271 00272 /* Enable GPIOs clock */ 00273 __GPIOD_CLK_ENABLE(); 00274 __GPIOE_CLK_ENABLE(); 00275 __GPIOF_CLK_ENABLE(); 00276 __GPIOG_CLK_ENABLE(); 00277 00278 /* Common GPIO configuration */ 00279 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00280 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00281 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00282 GPIO_Init_Structure.Alternate = GPIO_AF12_FSMC; 00283 00284 /* GPIOD configuration */ 00285 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_8 |\ 00286 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 |\ 00287 GPIO_PIN_14 | GPIO_PIN_15; 00288 00289 HAL_GPIO_Init(GPIOD, &GPIO_Init_Structure); 00290 00291 /* GPIOE configuration */ 00292 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3| GPIO_PIN_4 | GPIO_PIN_7 |\ 00293 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00294 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00295 HAL_GPIO_Init(GPIOE, &GPIO_Init_Structure); 00296 00297 /* GPIOF configuration */ 00298 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00299 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00300 HAL_GPIO_Init(GPIOF, &GPIO_Init_Structure); 00301 00302 /* GPIOG configuration */ 00303 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00304 GPIO_PIN_5 | GPIO_PIN_9; 00305 00306 HAL_GPIO_Init(GPIOG, &GPIO_Init_Structure); 00307 00308 00309 /* Configure common DMA parameters */ 00310 dmaHandle.Init.Channel = SRAM_DMAx_CHANNEL; 00311 dmaHandle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00312 dmaHandle.Init.PeriphInc = DMA_PINC_ENABLE; 00313 dmaHandle.Init.MemInc = DMA_MINC_ENABLE; 00314 dmaHandle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00315 dmaHandle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00316 dmaHandle.Init.Mode = DMA_NORMAL; 00317 dmaHandle.Init.Priority = DMA_PRIORITY_HIGH; 00318 dmaHandle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00319 dmaHandle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00320 dmaHandle.Init.MemBurst = DMA_MBURST_INC8; 00321 dmaHandle.Init.PeriphBurst = DMA_PBURST_INC8; 00322 00323 dmaHandle.Instance = SRAM_DMAx_STREAM; 00324 00325 /* Associate the DMA handle */ 00326 __HAL_LINKDMA(hsram, hdma, dmaHandle); 00327 00328 /* Deinitialize the stream for new transfer */ 00329 HAL_DMA_DeInit(&dmaHandle); 00330 00331 /* Configure the DMA stream */ 00332 HAL_DMA_Init(&dmaHandle); 00333 00334 /* NVIC configuration for DMA transfer complete interrupt */ 00335 HAL_NVIC_SetPriority(SRAM_DMAx_IRQn, 5, 0); 00336 HAL_NVIC_EnableIRQ(SRAM_DMAx_IRQn); 00337 } 00338 00339 /** 00340 * @} 00341 */ 00342 00343 /** 00344 * @} 00345 */ 00346 00347 /** 00348 * @} 00349 */ 00350 00351 /** 00352 * @} 00353 */ 00354 00355 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 14:22:29 for STM324xG_EVAL BSP User Manual by
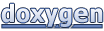