STM324xG_EVAL BSP User Manual
|
stm324xg_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324xg_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version V2.2.1 00006 * @date 15-January-2016 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM324xG-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive indirectly an LCD TFT. 00044 - This driver supports the ILI9325 LCD mounted on MB785 daughter board 00045 - The ILI9325 component driver MUST be included with this driver. 00046 00047 2. Driver description: 00048 --------------------- 00049 + Initialization steps: 00050 o Initialize the LCD using the BSP_LCD_Init() function. 00051 00052 + Display on LCD 00053 o Clear the hole LCD using yhe BSP_LCD_Clear() function or only one specified 00054 string line using the BSP_LCD_ClearStringLine() function. 00055 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00056 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00057 o Display a string line on the specified position (x,y in pixel) and align mode 00058 using the BSP_LCD_DisplayStringAtLine() function. 00059 o Draw and fill basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap, raw picture) 00060 on LCD using a set of functions. 00061 00062 ------------------------------------------------------------------------------*/ 00063 00064 /* Includes ------------------------------------------------------------------*/ 00065 #include "stm324xg_eval_lcd.h" 00066 #include "../../../Utilities/Fonts/fonts.h" 00067 #include "../../../Utilities/Fonts/font24.c" 00068 #include "../../../Utilities/Fonts/font20.c" 00069 #include "../../../Utilities/Fonts/font16.c" 00070 #include "../../../Utilities/Fonts/font12.c" 00071 #include "../../../Utilities/Fonts/font8.c" 00072 00073 /** @addtogroup BSP 00074 * @{ 00075 */ 00076 00077 /** @addtogroup STM324xG_EVAL 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM324xG_EVAL_LCD STM324xG EVAL LCD 00082 * @{ 00083 */ 00084 00085 /** @defgroup STM324xG_EVAL_LCD_Private_TypesDefinitions STM324xG EVAL LCD Private TypesDefinitions 00086 * @{ 00087 */ 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup STM324xG_EVAL_LCD_Private_Defines STM324xG EVAL LCD Private Defines 00093 * @{ 00094 */ 00095 #define POLY_X(Z) ((int32_t)((Points + (Z))->X)) 00096 #define POLY_Y(Z) ((int32_t)((Points + (Z))->Y)) 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM324xG_EVAL_LCD_Private_Macros STM324xG EVAL LCD Private Macros 00102 * @{ 00103 */ 00104 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM324xG_EVAL_LCD_Private_Variables STM324xG EVAL LCD Private Variables 00110 * @{ 00111 */ 00112 LCD_DrawPropTypeDef DrawProp; 00113 static LCD_DrvTypeDef *lcd_drv; 00114 /** 00115 * @} 00116 */ 00117 00118 /** @defgroup STM324xG_EVAL_LCD_Private_FunctionPrototypes STM324xG EVAL LCD Private FunctionPrototypes 00119 * @{ 00120 */ 00121 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00122 static void SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00123 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00124 /** 00125 * @} 00126 */ 00127 00128 /** @defgroup STM324xG_EVAL_LCD_Private_Functions STM324xG EVAL LCD Private Functions 00129 * @{ 00130 */ 00131 00132 /** 00133 * @brief Initializes the LCD. 00134 * @retval LCD state 00135 */ 00136 uint8_t BSP_LCD_Init(void) 00137 { 00138 uint8_t ret = LCD_ERROR; 00139 00140 /* Default value for draw propriety */ 00141 DrawProp.BackColor = 0xFFFF; 00142 DrawProp.pFont = &Font24; 00143 DrawProp.TextColor = 0x0000; 00144 00145 if(ili9325_drv.ReadID() == ILI9325_ID) 00146 { 00147 lcd_drv = &ili9325_drv; 00148 00149 /* LCD Init */ 00150 lcd_drv->Init(); 00151 00152 /* Initialize the font */ 00153 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00154 00155 ret = LCD_OK; 00156 } 00157 00158 return ret; 00159 } 00160 00161 /** 00162 * @brief Gets the LCD X size. 00163 * @retval Used LCD X size 00164 */ 00165 uint32_t BSP_LCD_GetXSize(void) 00166 { 00167 return(lcd_drv->GetLcdPixelWidth()); 00168 } 00169 00170 /** 00171 * @brief Gets the LCD Y size. 00172 * @retval Used LCD Y size 00173 */ 00174 uint32_t BSP_LCD_GetYSize(void) 00175 { 00176 return(lcd_drv->GetLcdPixelHeight()); 00177 } 00178 00179 /** 00180 * @brief Gets the LCD text color. 00181 * @retval Used text color. 00182 */ 00183 uint16_t BSP_LCD_GetTextColor(void) 00184 { 00185 return DrawProp.TextColor; 00186 } 00187 00188 /** 00189 * @brief Gets the LCD background color. 00190 * @retval Used background color 00191 */ 00192 uint16_t BSP_LCD_GetBackColor(void) 00193 { 00194 return DrawProp.BackColor; 00195 } 00196 00197 /** 00198 * @brief Sets the LCD text color. 00199 * @param Color: Text color code RGB(5-6-5) 00200 */ 00201 void BSP_LCD_SetTextColor(uint16_t Color) 00202 { 00203 DrawProp.TextColor = Color; 00204 } 00205 00206 /** 00207 * @brief Sets the LCD background color. 00208 * @param Color: Background color code RGB(5-6-5) 00209 */ 00210 void BSP_LCD_SetBackColor(uint16_t Color) 00211 { 00212 DrawProp.BackColor = Color; 00213 } 00214 00215 /** 00216 * @brief Sets the LCD text font. 00217 * @param fonts: Font to be used 00218 */ 00219 void BSP_LCD_SetFont(sFONT *fonts) 00220 { 00221 DrawProp.pFont = fonts; 00222 } 00223 00224 /** 00225 * @brief Gets the LCD text font. 00226 * @retval Used font 00227 */ 00228 sFONT *BSP_LCD_GetFont(void) 00229 { 00230 return DrawProp.pFont; 00231 } 00232 00233 /** 00234 * @brief Clears the hole LCD. 00235 * @param Color: Color of the background 00236 */ 00237 void BSP_LCD_Clear(uint16_t Color) 00238 { 00239 uint32_t counter = 0; 00240 uint32_t color_backup = DrawProp.TextColor; 00241 DrawProp.TextColor = Color; 00242 00243 for(counter = 0; counter < BSP_LCD_GetYSize(); counter++) 00244 { 00245 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00246 } 00247 DrawProp.TextColor = color_backup; 00248 BSP_LCD_SetTextColor(DrawProp.TextColor); 00249 } 00250 00251 /** 00252 * @brief Clears the selected line. 00253 * @param Line: Line to be cleared 00254 * This parameter can be one of the following values: 00255 * @arg 0..9: if the Current fonts is Font16x24 00256 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00257 * @arg 0..29: if the Current fonts is Font8x8 00258 */ 00259 void BSP_LCD_ClearStringLine(uint16_t Line) 00260 { 00261 uint32_t color_backup = DrawProp.TextColor; 00262 DrawProp.TextColor = DrawProp.BackColor;; 00263 00264 /* Draw a rectangle with background color */ 00265 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00266 00267 DrawProp.TextColor = color_backup; 00268 BSP_LCD_SetTextColor(DrawProp.TextColor); 00269 } 00270 00271 /** 00272 * @brief Displays one character. 00273 * @param Xpos: Start column address 00274 * @param Ypos: Line where to display the character shape. 00275 * @param Ascii: Character ascii code 00276 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00277 */ 00278 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00279 { 00280 DrawChar(Xpos, Ypos, &DrawProp.pFont->table[(Ascii-' ') *\ 00281 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00282 } 00283 00284 /** 00285 * @brief Displays characters on the LCD. 00286 * @param Xpos: X position (in pixel) 00287 * @param Ypos: Y position (in pixel) 00288 * @param Text: Pointer to string to display on LCD 00289 * @param Mode: Display mode 00290 * This parameter can be one of the following values: 00291 * @arg CENTER_MODE 00292 * @arg RIGHT_MODE 00293 * @arg LEFT_MODE 00294 */ 00295 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Line_ModeTypdef Mode) 00296 { 00297 uint16_t refcolumn = 1, i = 0; 00298 uint32_t size = 0, xsize = 0; 00299 uint8_t *ptr = Text; 00300 00301 /* Get the text size */ 00302 while (*ptr++) size ++ ; 00303 00304 /* Characters number per line */ 00305 xsize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00306 00307 switch (Mode) 00308 { 00309 case CENTER_MODE: 00310 { 00311 refcolumn = Xpos + ((xsize - size)* DrawProp.pFont->Width) / 2; 00312 break; 00313 } 00314 case LEFT_MODE: 00315 { 00316 refcolumn = Xpos; 00317 break; 00318 } 00319 case RIGHT_MODE: 00320 { 00321 refcolumn = - Xpos + ((xsize - size)*DrawProp.pFont->Width); 00322 break; 00323 } 00324 default: 00325 { 00326 refcolumn = Xpos; 00327 break; 00328 } 00329 } 00330 00331 /* Send the string character by character on lCD */ 00332 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00333 { 00334 /* Display one character on LCD */ 00335 BSP_LCD_DisplayChar(refcolumn, Ypos, *Text); 00336 /* Decrement the column position by 16 */ 00337 refcolumn += DrawProp.pFont->Width; 00338 /* Point on the next character */ 00339 Text++; 00340 i++; 00341 } 00342 } 00343 00344 /** 00345 * @brief Displays a character on the LCD. 00346 * @param Line: Line where to display the character shape 00347 * This parameter can be one of the following values: 00348 * @arg 0..9: if the Current fonts is Font16x24 00349 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00350 * @arg 0..29: if the Current fonts is Font8x8 00351 * @param ptr: Pointer to string to display on LCD 00352 */ 00353 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00354 { 00355 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00356 } 00357 00358 /** 00359 * @brief Reads an LCD pixel. 00360 * @param Xpos: X position 00361 * @param Ypos: Y position 00362 * @retval RGB pixel color 00363 */ 00364 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00365 { 00366 uint16_t ret = 0; 00367 00368 if(lcd_drv->ReadPixel != NULL) 00369 { 00370 ret = lcd_drv->ReadPixel(Xpos, Ypos); 00371 } 00372 00373 return ret; 00374 } 00375 00376 /** 00377 * @brief Draws a pixel on LCD. 00378 * @param Xpos: X position 00379 * @param Ypos: Y position 00380 * @param RGB_Code: Pixel color in RGB mode (5-6-5) 00381 */ 00382 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGB_Code) 00383 { 00384 if(lcd_drv->WritePixel != NULL) 00385 { 00386 lcd_drv->WritePixel(Xpos, Ypos, RGB_Code); 00387 } 00388 } 00389 00390 /** 00391 * @brief Draws an horizontal line. 00392 * @param Xpos: X position 00393 * @param Ypos: Y position 00394 * @param Length: Line length 00395 */ 00396 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00397 { 00398 uint32_t index = 0; 00399 00400 if(lcd_drv->DrawHLine != NULL) 00401 { 00402 lcd_drv->DrawHLine(DrawProp.TextColor, Xpos, Ypos, Length); 00403 } 00404 else 00405 { 00406 for(index = 0; index < Length; index++) 00407 { 00408 BSP_LCD_DrawPixel((Xpos + index), Ypos, DrawProp.TextColor); 00409 } 00410 } 00411 } 00412 00413 /** 00414 * @brief Draws a vertical line. 00415 * @param Xpos: X position 00416 * @param Ypos: Y position 00417 * @param Length: Line length 00418 */ 00419 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00420 { 00421 uint32_t index = 0; 00422 00423 if(lcd_drv->DrawVLine != NULL) 00424 { 00425 lcd_drv->DrawVLine(DrawProp.TextColor, Xpos, Ypos, Length); 00426 } 00427 else 00428 { 00429 for(index = 0; index < Length; index++) 00430 { 00431 BSP_LCD_DrawPixel(Xpos, Ypos + index, DrawProp.TextColor); 00432 } 00433 } 00434 } 00435 00436 /** 00437 * @brief Draws an uni-line (between two points). 00438 * @param x1: Point 1 X position 00439 * @param y1: Point 1 Y position 00440 * @param x2: Point 2 X position 00441 * @param y2: Point 2 Y position 00442 */ 00443 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00444 { 00445 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00446 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00447 curpixel = 0; 00448 00449 deltax = ABS(x2 - x1); /* The difference between the x's */ 00450 deltay = ABS(y2 - y1); /* The difference between the y's */ 00451 x = x1; /* Start x off at the first pixel */ 00452 y = y1; /* Start y off at the first pixel */ 00453 00454 if (x2 >= x1) /* The x-values are increasing */ 00455 { 00456 xinc1 = 1; 00457 xinc2 = 1; 00458 } 00459 else /* The x-values are decreasing */ 00460 { 00461 xinc1 = -1; 00462 xinc2 = -1; 00463 } 00464 00465 if (y2 >= y1) /* The y-values are increasing */ 00466 { 00467 yinc1 = 1; 00468 yinc2 = 1; 00469 } 00470 else /* The y-values are decreasing */ 00471 { 00472 yinc1 = -1; 00473 yinc2 = -1; 00474 } 00475 00476 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00477 { 00478 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00479 yinc2 = 0; /* Don't change the y for every iteration */ 00480 den = deltax; 00481 num = deltax / 2; 00482 numadd = deltay; 00483 numpixels = deltax; /* There are more x-values than y-values */ 00484 } 00485 else /* There is at least one y-value for every x-value */ 00486 { 00487 xinc2 = 0; /* Don't change the x for every iteration */ 00488 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00489 den = deltay; 00490 num = deltay / 2; 00491 numadd = deltax; 00492 numpixels = deltay; /* There are more y-values than x-values */ 00493 } 00494 00495 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00496 { 00497 BSP_LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00498 num += numadd; /* Increase the numerator by the top of the fraction */ 00499 if (num >= den) /* Check if numerator >= denominator */ 00500 { 00501 num -= den; /* Calculate the new numerator value */ 00502 x += xinc1; /* Change the x as appropriate */ 00503 y += yinc1; /* Change the y as appropriate */ 00504 } 00505 x += xinc2; /* Change the x as appropriate */ 00506 y += yinc2; /* Change the y as appropriate */ 00507 } 00508 } 00509 00510 /** 00511 * @brief Draws a rectangle. 00512 * @param Xpos: X position 00513 * @param Ypos: Y position 00514 * @param Width: Rectangle width 00515 * @param Height: Rectangle height 00516 */ 00517 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00518 { 00519 /* Draw horizontal lines */ 00520 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00521 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00522 00523 /* Draw vertical lines */ 00524 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00525 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00526 } 00527 00528 /** 00529 * @brief Draws a circle. 00530 * @param Xpos: X position 00531 * @param Ypos: Y position 00532 * @param Radius: Circle radius 00533 */ 00534 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00535 { 00536 int32_t D; /* Decision Variable */ 00537 uint32_t CurX; /* Current X Value */ 00538 uint32_t CurY; /* Current Y Value */ 00539 00540 D = 3 - (Radius << 1); 00541 CurX = 0; 00542 CurY = Radius; 00543 00544 while (CurX <= CurY) 00545 { 00546 BSP_LCD_DrawPixel((Xpos + CurX), (Ypos - CurY), DrawProp.TextColor); 00547 00548 BSP_LCD_DrawPixel((Xpos - CurX), (Ypos - CurY), DrawProp.TextColor); 00549 00550 BSP_LCD_DrawPixel((Xpos + CurY), (Ypos - CurX), DrawProp.TextColor); 00551 00552 BSP_LCD_DrawPixel((Xpos - CurY), (Ypos - CurX), DrawProp.TextColor); 00553 00554 BSP_LCD_DrawPixel((Xpos + CurX), (Ypos + CurY), DrawProp.TextColor); 00555 00556 BSP_LCD_DrawPixel((Xpos - CurX), (Ypos + CurY), DrawProp.TextColor); 00557 00558 BSP_LCD_DrawPixel((Xpos + CurY), (Ypos + CurX), DrawProp.TextColor); 00559 00560 BSP_LCD_DrawPixel((Xpos - CurY), (Ypos + CurX), DrawProp.TextColor); 00561 00562 /* Initialize the font */ 00563 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00564 00565 if (D < 0) 00566 { 00567 D += (CurX << 2) + 6; 00568 } 00569 else 00570 { 00571 D += ((CurX - CurY) << 2) + 10; 00572 CurY--; 00573 } 00574 CurX++; 00575 } 00576 } 00577 00578 /** 00579 * @brief Draws an poly-line (between many points). 00580 * @param Points: Pointer to the points array 00581 * @param PointCount: Number of points 00582 */ 00583 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00584 { 00585 int16_t X = 0, Y = 0; 00586 00587 if(PointCount < 2) 00588 { 00589 return; 00590 } 00591 00592 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00593 00594 while(--PointCount) 00595 { 00596 X = Points->X; 00597 Y = Points->Y; 00598 Points++; 00599 BSP_LCD_DrawLine(X, Y, Points->X, Points->Y); 00600 } 00601 00602 } 00603 00604 /** 00605 * @brief Draws an ellipse on LCD. 00606 * @param Xpos: X position 00607 * @param Ypos: Y position 00608 * @param XRadius: Ellipse X radius 00609 * @param YRadius: Ellipse Y radius 00610 */ 00611 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00612 { 00613 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00614 float K = 0, rad1 = 0, rad2 = 0; 00615 00616 rad1 = XRadius; 00617 rad2 = YRadius; 00618 00619 K = (float)(rad2/rad1); 00620 00621 do { 00622 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos+y), DrawProp.TextColor); 00623 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos+y), DrawProp.TextColor); 00624 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos-y), DrawProp.TextColor); 00625 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos-y), DrawProp.TextColor); 00626 00627 e2 = err; 00628 if (e2 <= x) { 00629 err += ++x*2+1; 00630 if (-y == x && e2 <= y) e2 = 0; 00631 } 00632 if (e2 > y) err += ++y*2+1; 00633 } 00634 while (y <= 0); 00635 } 00636 00637 /** 00638 * @brief Draws a bitmap picture (16 bpp). 00639 * @param Xpos: Bmp X position in the LCD 00640 * @param Ypos: Bmp Y position in the LCD 00641 * @param pbmp: Pointer to Bmp picture address. 00642 */ 00643 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp) 00644 { 00645 uint32_t height = 0; 00646 uint32_t width = 0; 00647 00648 00649 /* Read bitmap width */ 00650 width = *(uint16_t *) (pbmp + 18); 00651 width |= (*(uint16_t *) (pbmp + 20)) << 16; 00652 00653 /* Read bitmap height */ 00654 height = *(uint16_t *) (pbmp + 22); 00655 height |= (*(uint16_t *) (pbmp + 24)) << 16; 00656 00657 SetDisplayWindow(Xpos, Ypos, width, height); 00658 00659 if(lcd_drv->DrawBitmap != NULL) 00660 { 00661 lcd_drv->DrawBitmap(Xpos, Ypos, pbmp); 00662 } 00663 SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00664 } 00665 00666 /** 00667 * @brief Draws RGB Image (16 bpp). 00668 * @param Xpos: X position in the LCD 00669 * @param Ypos: Y position in the LCD 00670 * @param Xsize: X size in the LCD 00671 * @param Ysize: Y size in the LCD 00672 * @param pdata: Pointer to the RGB Image address. 00673 */ 00674 void BSP_LCD_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pdata) 00675 { 00676 00677 SetDisplayWindow(Xpos, Ypos, Xsize, Ysize); 00678 00679 if(lcd_drv->DrawRGBImage != NULL) 00680 { 00681 lcd_drv->DrawRGBImage(Xpos, Ypos, Xsize, Ysize, pdata); 00682 } 00683 SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00684 } 00685 00686 /** 00687 * @brief Draws a full rectangle. 00688 * @param Xpos: X position 00689 * @param Ypos: Y position 00690 * @param Width: Rectangle width 00691 * @param Height: Rectangle height 00692 */ 00693 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00694 { 00695 BSP_LCD_SetTextColor(DrawProp.TextColor); 00696 do 00697 { 00698 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00699 } 00700 while(Height--); 00701 } 00702 00703 /** 00704 * @brief Draws a full circle. 00705 * @param Xpos: X position 00706 * @param Ypos: Y position 00707 * @param Radius: Circle radius 00708 */ 00709 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00710 { 00711 int32_t D; /* Decision Variable */ 00712 uint32_t CurX; /* Current X Value */ 00713 uint32_t CurY; /* Current Y Value */ 00714 00715 D = 3 - (Radius << 1); 00716 00717 CurX = 0; 00718 CurY = Radius; 00719 00720 BSP_LCD_SetTextColor(DrawProp.TextColor); 00721 00722 while (CurX <= CurY) 00723 { 00724 if(CurY > 0) 00725 { 00726 BSP_LCD_DrawHLine(Xpos - CurY, Ypos + CurX, 2*CurY); 00727 BSP_LCD_DrawHLine(Xpos - CurY, Ypos - CurX, 2*CurY); 00728 } 00729 00730 if(CurX > 0) 00731 { 00732 BSP_LCD_DrawHLine(Xpos - CurX, Ypos - CurY, 2*CurX); 00733 BSP_LCD_DrawHLine(Xpos - CurX, Ypos + CurY, 2*CurX); 00734 } 00735 if (D < 0) 00736 { 00737 D += (CurX << 2) + 6; 00738 } 00739 else 00740 { 00741 D += ((CurX - CurY) << 2) + 10; 00742 CurY--; 00743 } 00744 CurX++; 00745 } 00746 00747 BSP_LCD_SetTextColor(DrawProp.TextColor); 00748 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00749 } 00750 00751 /** 00752 * @brief Draws a full poly-line (between many points). 00753 * @param Points: Pointer to the points array 00754 * @param PointCount: Number of points 00755 */ 00756 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 00757 { 00758 00759 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 00760 uint16_t IMAGE_LEFT = 0, IMAGE_RIGHT = 0, IMAGE_TOP = 0, IMAGE_BOTTOM = 0; 00761 00762 IMAGE_LEFT = IMAGE_RIGHT = Points->X; 00763 IMAGE_TOP= IMAGE_BOTTOM = Points->Y; 00764 00765 for(counter = 1; counter < PointCount; counter++) 00766 { 00767 pixelX = POLY_X(counter); 00768 if(pixelX < IMAGE_LEFT) 00769 { 00770 IMAGE_LEFT = pixelX; 00771 } 00772 if(pixelX > IMAGE_RIGHT) 00773 { 00774 IMAGE_RIGHT = pixelX; 00775 } 00776 00777 pixelY = POLY_Y(counter); 00778 if(pixelY < IMAGE_TOP) 00779 { 00780 IMAGE_TOP = pixelY; 00781 } 00782 if(pixelY > IMAGE_BOTTOM) 00783 { 00784 IMAGE_BOTTOM = pixelY; 00785 } 00786 } 00787 00788 if(PointCount < 2) 00789 { 00790 return; 00791 } 00792 00793 X_center = (IMAGE_LEFT + IMAGE_RIGHT)/2; 00794 Y_center = (IMAGE_BOTTOM + IMAGE_TOP)/2; 00795 00796 X_first = Points->X; 00797 Y_first = Points->Y; 00798 00799 while(--PointCount) 00800 { 00801 X = Points->X; 00802 Y = Points->Y; 00803 Points++; 00804 X2 = Points->X; 00805 Y2 = Points->Y; 00806 00807 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 00808 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 00809 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 00810 } 00811 00812 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 00813 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 00814 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 00815 } 00816 00817 /** 00818 * @brief Draws a full ellipse. 00819 * @param Xpos: X position 00820 * @param Ypos: Y position 00821 * @param XRadius: Ellipse X radius 00822 * @param YRadius: Ellipse Y radius 00823 */ 00824 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00825 { 00826 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00827 float K = 0, rad1 = 0, rad2 = 0; 00828 00829 rad1 = XRadius; 00830 rad2 = YRadius; 00831 00832 K = (float)(rad2/rad1); 00833 00834 do 00835 { 00836 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos+y), (2*(uint16_t)(x/K) + 1)); 00837 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos-y), (2*(uint16_t)(x/K) + 1)); 00838 00839 e2 = err; 00840 if (e2 <= x) 00841 { 00842 err += ++x*2+1; 00843 if (-y == x && e2 <= y) e2 = 0; 00844 } 00845 if (e2 > y) err += ++y*2+1; 00846 } 00847 while (y <= 0); 00848 } 00849 00850 /** 00851 * @brief Enables the display. 00852 */ 00853 void BSP_LCD_DisplayOn(void) 00854 { 00855 lcd_drv->DisplayOn(); 00856 } 00857 00858 /** 00859 * @brief Disables the display. 00860 */ 00861 void BSP_LCD_DisplayOff(void) 00862 { 00863 lcd_drv->DisplayOff(); 00864 } 00865 00866 /****************************************************************************** 00867 Static Function 00868 *******************************************************************************/ 00869 00870 /** 00871 * @brief Draws a character on LCD. 00872 * @param Xpos: Line where to display the character shape 00873 * @param Ypos: Start column address 00874 * @param c: Pointer to the character data 00875 */ 00876 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 00877 { 00878 uint32_t i = 0, j = 0; 00879 uint16_t height, width; 00880 uint8_t offset; 00881 uint8_t *pchar; 00882 uint32_t line; 00883 00884 height = DrawProp.pFont->Height; 00885 width = DrawProp.pFont->Width; 00886 00887 offset = 8 *((width + 7)/8) - width ; 00888 00889 for(i = 0; i < height; i++) 00890 { 00891 pchar = ((uint8_t *)c + (width + 7)/8 * i); 00892 00893 switch(((width + 7)/8)) 00894 { 00895 case 1: 00896 line = pchar[0]; 00897 break; 00898 00899 case 2: 00900 line = (pchar[0]<< 8) | pchar[1]; 00901 break; 00902 00903 case 3: 00904 default: 00905 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 00906 break; 00907 } 00908 00909 for (j = 0; j < width; j++) 00910 { 00911 if(line & (1 << (width- j + offset- 1))) 00912 { 00913 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp.TextColor); 00914 } 00915 else 00916 { 00917 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp.BackColor); 00918 } 00919 } 00920 Ypos++; 00921 } 00922 } 00923 00924 /** 00925 * @brief Sets display window. 00926 * @param Xpos: LCD X position 00927 * @param Ypos: LCD Y position 00928 * @param Width: LCD window width 00929 * @param Height: LCD window height 00930 */ 00931 static void SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00932 { 00933 if(lcd_drv->SetDisplayWindow != NULL) 00934 { 00935 lcd_drv->SetDisplayWindow(Xpos, Ypos, Width, Height); 00936 } 00937 } 00938 00939 /** 00940 * @brief Fills a triangle (between 3 points). 00941 * @param x1: Point 1 X position 00942 * @param y1: Point 1 Y position 00943 * @param x2: Point 2 X position 00944 * @param y2: Point 2 Y position 00945 * @param x3: Point 3 X position 00946 * @param y3: Point 3 Y position 00947 */ 00948 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 00949 { 00950 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00951 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00952 curpixel = 0; 00953 00954 deltax = ABS(x2 - x1); /* The difference between the x's */ 00955 deltay = ABS(y2 - y1); /* The difference between the y's */ 00956 x = x1; /* Start x off at the first pixel */ 00957 y = y1; /* Start y off at the first pixel */ 00958 00959 if (x2 >= x1) /* The x-values are increasing */ 00960 { 00961 xinc1 = 1; 00962 xinc2 = 1; 00963 } 00964 else /* The x-values are decreasing */ 00965 { 00966 xinc1 = -1; 00967 xinc2 = -1; 00968 } 00969 00970 if (y2 >= y1) /* The y-values are increasing */ 00971 { 00972 yinc1 = 1; 00973 yinc2 = 1; 00974 } 00975 else /* The y-values are decreasing */ 00976 { 00977 yinc1 = -1; 00978 yinc2 = -1; 00979 } 00980 00981 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00982 { 00983 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00984 yinc2 = 0; /* Don't change the y for every iteration */ 00985 den = deltax; 00986 num = deltax / 2; 00987 numadd = deltay; 00988 numpixels = deltax; /* There are more x-values than y-values */ 00989 } 00990 else /* There is at least one y-value for every x-value */ 00991 { 00992 xinc2 = 0; /* Don't change the x for every iteration */ 00993 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00994 den = deltay; 00995 num = deltay / 2; 00996 numadd = deltax; 00997 numpixels = deltay; /* There are more y-values than x-values */ 00998 } 00999 01000 for (curpixel = 0; curpixel <= numpixels; curpixel++) 01001 { 01002 BSP_LCD_DrawLine(x, y, x3, y3); 01003 01004 num += numadd; /* Increase the numerator by the top of the fraction */ 01005 if (num >= den) /* Check if numerator >= denominator */ 01006 { 01007 num -= den; /* Calculate the new numerator value */ 01008 x += xinc1; /* Change the x as appropriate */ 01009 y += yinc1; /* Change the y as appropriate */ 01010 } 01011 x += xinc2; /* Change the x as appropriate */ 01012 y += yinc2; /* Change the y as appropriate */ 01013 } 01014 } 01015 01016 /** 01017 * @} 01018 */ 01019 01020 /** 01021 * @} 01022 */ 01023 01024 /** 01025 * @} 01026 */ 01027 01028 /** 01029 * @} 01030 */ 01031 01032 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 14:22:29 for STM324xG_EVAL BSP User Manual by
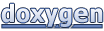