STM324xG_EVAL BSP User Manual
|
stm324xg_eval_camera.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324xg_eval_camera.c 00004 * @author MCD Application Team 00005 * @version V2.2.1 00006 * @date 15-January-2016 00007 * @brief This file includes the driver for Camera module mounted on 00008 * STM324xG-EVAL evaluation board(MB786). 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info: ------------------------------------------------------------------ 00040 User NOTES 00041 1. How to use this driver: 00042 -------------------------- 00043 - This driver is used to drive the Camera. 00044 - The OV2640 component driver MUST be included with this driver. 00045 00046 2. Driver description: 00047 --------------------- 00048 + Initialization steps: 00049 o Initialize the Camera using the BSP_CAMERA_Init() function. 00050 o Start the Camera capture or snapshot using CAMERA_Start() function. 00051 o Suspend, resume or stop the Camera capture using the following functions: 00052 - BSP_CAMERA_Suspend() 00053 - BSP_CAMERA_Resume() 00054 - BSP_CAMERA_Stop() 00055 00056 + Options 00057 o Increase or decrease on the fly the brightness and/or contrast 00058 using the following function: 00059 - BSP_CAMERA_ContrastBrightnessConfig 00060 o Add a special effect on the fly using the following functions: 00061 - BSP_CAMERA_BlackWhiteConfig() 00062 - BSP_CAMERA_ColorEffectConfig() 00063 00064 ------------------------------------------------------------------------------*/ 00065 00066 /* Includes ------------------------------------------------------------------*/ 00067 #include "stm324xg_eval_camera.h" 00068 00069 /** @addtogroup BSP 00070 * @{ 00071 */ 00072 00073 /** @addtogroup STM324xG_EVAL 00074 * @{ 00075 */ 00076 00077 /** @defgroup STM324xG_EVAL_CAMERA STM324xG EVAL CAMERA 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM324xG_EVAL_CAMERA_Private_TypesDefinitions STM324xG EVAL CAMERA Private TypesDefinitions 00082 * @{ 00083 */ 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM324xG_EVAL_CAMERA_Private_Defines STM324xG EVAL CAMERA Private Defines 00089 * @{ 00090 */ 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM324xG_EVAL_CAMERA_Private_Macros STM324xG EVAL CAMERA Private Macros 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM324xG_EVAL_CAMERA_Private_Variables STM324xG EVAL CAMERA Private Variables 00103 * @{ 00104 */ 00105 static DCMI_HandleTypeDef hdcmi_eval; 00106 CAMERA_DrvTypeDef *camera_drv; 00107 uint32_t current_resolution; 00108 /** 00109 * @} 00110 */ 00111 00112 /** @defgroup STM324xG_EVAL_CAMERA_Private_FunctionPrototypes STM324xG EVAL CAMERA Private FunctionPrototypes 00113 * @{ 00114 */ 00115 static void DCMI_MspInit(void); 00116 static uint32_t GetSize(uint32_t resolution); 00117 /** 00118 * @} 00119 */ 00120 00121 /** @defgroup STM324xG_EVAL_CAMERA_Private_Functions STM324xG EVAL CAMERA Private Functions 00122 * @{ 00123 */ 00124 00125 /** 00126 * @brief Initializes the Camera. 00127 * @param Resolution: Camera resolution 00128 * @retval Camera status 00129 */ 00130 uint8_t BSP_CAMERA_Init(uint32_t Resolution) 00131 { 00132 DCMI_HandleTypeDef *phdcmi; 00133 uint8_t ret = CAMERA_ERROR; 00134 00135 /* Get the DCMI handle structure */ 00136 phdcmi = &hdcmi_eval; 00137 00138 /*** Configures the DCMI to interface with the Camera module ***/ 00139 /* DCMI configuration */ 00140 phdcmi->Init.CaptureRate = DCMI_CR_ALL_FRAME; 00141 phdcmi->Init.HSPolarity = DCMI_HSPOLARITY_LOW; 00142 phdcmi->Init.SynchroMode = DCMI_SYNCHRO_HARDWARE; 00143 phdcmi->Init.VSPolarity = DCMI_VSPOLARITY_LOW; 00144 phdcmi->Init.ExtendedDataMode = DCMI_EXTEND_DATA_8B; 00145 phdcmi->Init.PCKPolarity = DCMI_PCKPOLARITY_RISING; 00146 phdcmi->Instance = DCMI; 00147 00148 /* DCMI Initialization */ 00149 DCMI_MspInit(); 00150 HAL_DCMI_Init(phdcmi); 00151 00152 if(ov2640_drv.ReadID(CAMERA_I2C_ADDRESS) == OV2640_ID) 00153 { 00154 /* Initialize the Camera driver structure */ 00155 camera_drv = &ov2640_drv; 00156 00157 /* Camera Init */ 00158 camera_drv->Init(CAMERA_I2C_ADDRESS, Resolution); 00159 00160 /* Return CAMERA_OK status */ 00161 ret = CAMERA_OK; 00162 } 00163 00164 current_resolution = Resolution; 00165 00166 return ret; 00167 } 00168 00169 /** 00170 * @brief Starts the Camera capture in continuous mode. 00171 * @param buff: pointer to the Camera output buffer 00172 */ 00173 void BSP_CAMERA_ContinuousStart(uint8_t *buff) 00174 { 00175 /* Start the Camera capture */ 00176 HAL_DCMI_Start_DMA(&hdcmi_eval, DCMI_MODE_CONTINUOUS, (uint32_t)buff, GetSize(current_resolution)); 00177 } 00178 00179 /** 00180 * @brief Starts the Camera capture in snapshot mode. 00181 * @param buff: pointer to the Camera output buffer 00182 */ 00183 void BSP_CAMERA_SnapshotStart(uint8_t *buff) 00184 { 00185 /* Start the Camera capture */ 00186 HAL_DCMI_Start_DMA(&hdcmi_eval, DCMI_MODE_SNAPSHOT, (uint32_t)buff, GetSize(current_resolution)); 00187 } 00188 00189 /** 00190 * @brief Suspends the Camera capture. 00191 */ 00192 void BSP_CAMERA_Suspend(void) 00193 { 00194 /* Disable the DMA */ 00195 __HAL_DMA_DISABLE(hdcmi_eval.DMA_Handle); 00196 /* Disable the DCMI */ 00197 __HAL_DCMI_DISABLE(&hdcmi_eval); 00198 } 00199 00200 /** 00201 * @brief Resumes the Camera capture. 00202 */ 00203 void BSP_CAMERA_Resume(void) 00204 { 00205 /* Enable the DCMI */ 00206 __HAL_DCMI_ENABLE(&hdcmi_eval); 00207 /* Enable the DMA */ 00208 __HAL_DMA_ENABLE(hdcmi_eval.DMA_Handle); 00209 } 00210 00211 /** 00212 * @brief Stops the Camera capture. 00213 * @retval Camera status 00214 */ 00215 uint8_t BSP_CAMERA_Stop(void) 00216 { 00217 DCMI_HandleTypeDef *phdcmi; 00218 00219 uint8_t ret = CAMERA_ERROR; 00220 00221 /* Get the DCMI handle structure */ 00222 phdcmi = &hdcmi_eval; 00223 00224 if(HAL_DCMI_Stop(phdcmi) == HAL_OK) 00225 { 00226 ret = CAMERA_OK; 00227 } 00228 00229 return ret; 00230 } 00231 00232 /** 00233 * @brief Configures the Camera contrast and brightness. 00234 * @param contrast_level: Contrast level 00235 * This parameter can be one of the following values: 00236 * @arg CAMERA_CONTRAST_LEVEL4: for contrast +2 00237 * @arg CAMERA_CONTRAST_LEVEL3: for contrast +1 00238 * @arg CAMERA_CONTRAST_LEVEL2: for contrast 0 00239 * @arg CAMERA_CONTRAST_LEVEL1: for contrast -1 00240 * @arg CAMERA_CONTRAST_LEVEL0: for contrast -2 00241 * @param brightness_level: Brightness level 00242 * This parameter can be one of the following values: 00243 * @arg CAMERA_BRIGHTNESS_LEVEL4: for brightness +2 00244 * @arg CAMERA_BRIGHTNESS_LEVEL3: for brightness +1 00245 * @arg CAMERA_BRIGHTNESS_LEVEL2: for brightness 0 00246 * @arg CAMERA_BRIGHTNESS_LEVEL1: for brightness -1 00247 * @arg CAMERA_BRIGHTNESS_LEVEL0: for brightness -2 00248 */ 00249 void BSP_CAMERA_ContrastBrightnessConfig(uint32_t contrast_level, uint32_t brightness_level) 00250 { 00251 if(camera_drv->Config != NULL) 00252 { 00253 camera_drv->Config(CAMERA_I2C_ADDRESS, CAMERA_CONTRAST_BRIGHTNESS, contrast_level, brightness_level); 00254 } 00255 } 00256 00257 /** 00258 * @brief Configures the Camera white balance. 00259 * @param Mode: black_white mode 00260 * This parameter can be one of the following values: 00261 * @arg CAMERA_BLACK_WHITE_BW 00262 * @arg CAMERA_BLACK_WHITE_NEGATIVE 00263 * @arg CAMERA_BLACK_WHITE_BW_NEGATIVE 00264 * @arg CAMERA_BLACK_WHITE_NORMAL 00265 */ 00266 void BSP_CAMERA_BlackWhiteConfig(uint32_t Mode) 00267 { 00268 if(camera_drv->Config != NULL) 00269 { 00270 camera_drv->Config(CAMERA_I2C_ADDRESS, CAMERA_BLACK_WHITE, Mode, 0); 00271 } 00272 } 00273 00274 /** 00275 * @brief Configures the Camera color effect. 00276 * @param Effect: Color effect 00277 * This parameter can be one of the following values: 00278 * @arg CAMERA_COLOR_EFFECT_ANTIQUE 00279 * @arg CAMERA_COLOR_EFFECT_BLUE 00280 * @arg CAMERA_COLOR_EFFECT_GREEN 00281 * @arg CAMERA_COLOR_EFFECT_RED 00282 */ 00283 void BSP_CAMERA_ColorEffectConfig(uint32_t Effect) 00284 { 00285 if(camera_drv->Config != NULL) 00286 { 00287 camera_drv->Config(CAMERA_I2C_ADDRESS, CAMERA_COLOR_EFFECT, Effect, 0); 00288 } 00289 } 00290 00291 /** 00292 * @brief Handles DCMI interrupt request. 00293 */ 00294 void BSP_CAMERA_IRQHandler(void) 00295 { 00296 HAL_DCMI_IRQHandler(&hdcmi_eval); 00297 } 00298 00299 /** 00300 * @brief Handles DMA interrupt request. 00301 */ 00302 void BSP_CAMERA_DMA_IRQHandler(void) 00303 { 00304 HAL_DMA_IRQHandler(hdcmi_eval.DMA_Handle); 00305 } 00306 00307 /** 00308 * @brief Get the capture size. 00309 * @param resolution: the current resolution. 00310 * @retval cpature size 00311 */ 00312 static uint32_t GetSize(uint32_t resolution) 00313 { 00314 uint32_t size = 0; 00315 00316 /* Get capture size */ 00317 switch (resolution) 00318 { 00319 case CAMERA_R160x120: 00320 { 00321 size = 0x2580; 00322 } 00323 break; 00324 case CAMERA_R320x240: 00325 { 00326 size = 0x9600; 00327 } 00328 break; 00329 default: 00330 { 00331 break; 00332 } 00333 } 00334 00335 return size; 00336 } 00337 00338 /** 00339 * @brief Initializes the DCMI MSP. 00340 */ 00341 static void DCMI_MspInit(void) 00342 { 00343 static DMA_HandleTypeDef hdma; 00344 GPIO_InitTypeDef GPIO_Init_Structure; 00345 DCMI_HandleTypeDef *hdcmi = &hdcmi_eval; 00346 00347 /*** Enable peripherals and GPIO clocks ***/ 00348 /* Enable DCMI clock */ 00349 __DCMI_CLK_ENABLE(); 00350 00351 /* Enable DMA2 clock */ 00352 __DMA2_CLK_ENABLE(); 00353 00354 /* Enable GPIO clocks */ 00355 __GPIOA_CLK_ENABLE(); 00356 __GPIOH_CLK_ENABLE(); 00357 __GPIOI_CLK_ENABLE(); 00358 00359 /*** Configure the GPIO ***/ 00360 /* Configure DCMI GPIO as alternate function */ 00361 GPIO_Init_Structure.Pin = GPIO_PIN_6; 00362 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00363 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00364 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00365 GPIO_Init_Structure.Alternate = GPIO_AF13_DCMI; 00366 HAL_GPIO_Init(GPIOA, &GPIO_Init_Structure); 00367 00368 GPIO_Init_Structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 |\ 00369 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_14; 00370 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00371 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00372 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00373 GPIO_Init_Structure.Alternate = GPIO_AF13_DCMI; 00374 HAL_GPIO_Init(GPIOH, &GPIO_Init_Structure); 00375 00376 GPIO_Init_Structure.Pin = GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 |\ 00377 GPIO_PIN_7; 00378 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00379 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00380 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00381 GPIO_Init_Structure.Alternate = GPIO_AF13_DCMI; 00382 HAL_GPIO_Init(GPIOI, &GPIO_Init_Structure); 00383 00384 /*** Configure the DMA streams ***/ 00385 /* Configure the DMA handler for Transmission process */ 00386 hdma.Init.Channel = DMA_CHANNEL_1; 00387 hdma.Init.Direction = DMA_PERIPH_TO_MEMORY; 00388 hdma.Init.PeriphInc = DMA_PINC_DISABLE; 00389 hdma.Init.MemInc = DMA_MINC_ENABLE; 00390 hdma.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00391 hdma.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00392 hdma.Init.Mode = DMA_CIRCULAR; 00393 hdma.Init.Priority = DMA_PRIORITY_HIGH; 00394 hdma.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00395 hdma.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00396 hdma.Init.MemBurst = DMA_MBURST_SINGLE; 00397 hdma.Init.PeriphBurst = DMA_PBURST_SINGLE; 00398 00399 hdma.Instance = DMA2_Stream1; 00400 00401 /* Associate the initialized DMA handle to the DCMI handle */ 00402 __HAL_LINKDMA(hdcmi, DMA_Handle, hdma); 00403 00404 /*** Configure the NVIC for DCMI and DMA ***/ 00405 /* NVIC configuration for DCMI transfer complete interrupt */ 00406 HAL_NVIC_SetPriority(DCMI_IRQn, 5, 0); 00407 HAL_NVIC_EnableIRQ(DCMI_IRQn); 00408 00409 /* NVIC configuration for DMA2 transfer complete interrupt */ 00410 HAL_NVIC_SetPriority(DMA2_Stream1_IRQn, 5, 0); 00411 HAL_NVIC_EnableIRQ(DMA2_Stream1_IRQn); 00412 00413 /* Configure the DMA stream */ 00414 HAL_DMA_Init(hdcmi->DMA_Handle); 00415 } 00416 00417 /** 00418 * @brief Line event callback 00419 * @param hdcmi: pointer to the DCMI handle 00420 */ 00421 void HAL_DCMI_LineEventCallback(DCMI_HandleTypeDef *hdcmi) 00422 { 00423 BSP_CAMERA_LineEventCallback(); 00424 } 00425 00426 /** 00427 * @brief Line Event callback. 00428 */ 00429 __weak void BSP_CAMERA_LineEventCallback(void) 00430 { 00431 /* NOTE : This function Should not be modified, when the callback is needed, 00432 the HAL_DCMI_LineEventCallback could be implemented in the user file 00433 */ 00434 } 00435 00436 /** 00437 * @brief VSYNC event callback 00438 * @param hdcmi: pointer to the DCMI handle 00439 */ 00440 void HAL_DCMI_VsyncEventCallback(DCMI_HandleTypeDef *hdcmi) 00441 { 00442 BSP_CAMERA_VsyncEventCallback(); 00443 } 00444 00445 /** 00446 * @brief VSYNC Event callback. 00447 */ 00448 __weak void BSP_CAMERA_VsyncEventCallback(void) 00449 { 00450 /* NOTE : This function Should not be modified, when the callback is needed, 00451 the HAL_DCMI_VsyncEventCallback could be implemented in the user file 00452 */ 00453 } 00454 00455 /** 00456 * @brief Frame event callback 00457 * @param hdcmi: pointer to the DCMI handle 00458 */ 00459 void HAL_DCMI_FrameEventCallback(DCMI_HandleTypeDef *hdcmi) 00460 { 00461 BSP_CAMERA_FrameEventCallback(); 00462 } 00463 00464 /** 00465 * @brief Frame Event callback. 00466 */ 00467 __weak void BSP_CAMERA_FrameEventCallback(void) 00468 { 00469 /* NOTE : This function Should not be modified, when the callback is needed, 00470 the HAL_DCMI_FrameEventCallback could be implemented in the user file 00471 */ 00472 } 00473 00474 /** 00475 * @brief Error callback 00476 * @param hdcmi: pointer to the DCMI handle 00477 */ 00478 void HAL_DCMI_ErrorCallback(DCMI_HandleTypeDef *hdcmi) 00479 { 00480 BSP_CAMERA_ErrorCallback(); 00481 } 00482 00483 /** 00484 * @brief Error callback. 00485 */ 00486 __weak void BSP_CAMERA_ErrorCallback(void) 00487 { 00488 /* NOTE : This function Should not be modified, when the callback is needed, 00489 the HAL_DCMI_ErrorCallback could be implemented in the user file 00490 */ 00491 } 00492 00493 /** 00494 * @} 00495 */ 00496 00497 /** 00498 * @} 00499 */ 00500 00501 /** 00502 * @} 00503 */ 00504 00505 /** 00506 * @} 00507 */ 00508 00509 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 14:22:29 for STM324xG_EVAL BSP User Manual by
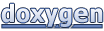