STM324xG_EVAL BSP User Manual
|
stm324xg_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324xg_eval.c 00004 * @author MCD Application Team 00005 * @version V2.2.1 00006 * @date 15-January-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM324xG-EVAL evaluation 00009 * board(MB786) RevB from STMicroelectronics. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* File Info: ------------------------------------------------------------------ 00041 User NOTE 00042 00043 This driver requires the stm324xG_eval_io.c driver to manage the joystick 00044 00045 ------------------------------------------------------------------------------*/ 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm324xg_eval.h" 00049 #include "stm324xg_eval_io.h" 00050 00051 /** @defgroup BSP BSP 00052 * @{ 00053 */ 00054 00055 /** @defgroup STM324xG_EVAL STM324xG EVAL 00056 * @{ 00057 */ 00058 00059 /** @defgroup STM324xG_EVAL_LOW_LEVEL STM324xG EVAL LOW LEVEL 00060 * @{ 00061 */ 00062 00063 /** @defgroup STM324xG_EVAL_LOW_LEVEL_Private_TypesDefinitions STM324xG EVAL LOW LEVEL Private TypesDefinitions 00064 * @{ 00065 */ 00066 typedef struct 00067 { 00068 __IO uint16_t REG; 00069 __IO uint16_t RAM; 00070 }LCD_CONTROLLER_TypeDef; 00071 /** 00072 * @} 00073 */ 00074 00075 /** @defgroup STM324xG_EVAL_LOW_LEVEL_Private_Defines STM324xG EVAL LOW LEVEL Private Defines 00076 * @{ 00077 */ 00078 00079 /** 00080 * @brief STM324xG EVAL BSP Driver version number V2.2.1 00081 */ 00082 #define __STM324xG_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00083 #define __STM324xG_EVAL_BSP_VERSION_SUB1 (0x02) /*!< [23:16] sub1 version */ 00084 #define __STM324xG_EVAL_BSP_VERSION_SUB2 (0x01) /*!< [15:8] sub2 version */ 00085 #define __STM324xG_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00086 #define __STM324xG_EVAL_BSP_VERSION ((__STM324xG_EVAL_BSP_VERSION_MAIN << 24)\ 00087 |(__STM324xG_EVAL_BSP_VERSION_SUB1 << 16)\ 00088 |(__STM324xG_EVAL_BSP_VERSION_SUB2 << 8 )\ 00089 |(__STM324xG_EVAL_BSP_VERSION_RC)) 00090 00091 #define FMC_BANK3_BASE ((uint32_t)(0x60000000 | 0x08000000)) 00092 #define FMC_BANK3 ((LCD_CONTROLLER_TypeDef *) FMC_BANK3_BASE) 00093 00094 #define I2C_TIMEOUT 100 /*<! Value of Timeout when I2C communication fails */ 00095 00096 /** 00097 * @} 00098 */ 00099 00100 /** @defgroup STM324xG_EVAL_LOW_LEVEL_Private_Macros STM324xG EVAL LOW LEVEL Private Macros 00101 * @{ 00102 */ 00103 /** 00104 * @} 00105 */ 00106 00107 /** @defgroup STM324xG_EVAL_LOW_LEVEL_Private_Variables STM324xG EVAL LOW LEVEL Private Variables 00108 * @{ 00109 */ 00110 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED1_GPIO_PORT, 00111 LED2_GPIO_PORT, 00112 LED3_GPIO_PORT, 00113 LED4_GPIO_PORT}; 00114 00115 const uint16_t GPIO_PIN[LEDn] = {LED1_PIN, 00116 LED2_PIN, 00117 LED3_PIN, 00118 LED4_PIN}; 00119 00120 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00121 TAMPER_BUTTON_GPIO_PORT, 00122 KEY_BUTTON_GPIO_PORT}; 00123 00124 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00125 TAMPER_BUTTON_PIN, 00126 KEY_BUTTON_PIN}; 00127 00128 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00129 TAMPER_BUTTON_EXTI_IRQn, 00130 KEY_BUTTON_EXTI_IRQn}; 00131 00132 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00133 00134 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00135 00136 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00137 00138 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00139 00140 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00141 00142 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00143 00144 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00145 00146 I2C_HandleTypeDef heval_I2c; 00147 00148 static uint8_t Is_LCD_IO_Initialized = 0; 00149 00150 /** 00151 * @} 00152 */ 00153 00154 /** @defgroup STM324xG_EVAL_LOW_LEVEL_Private_FunctionPrototypes STM324xG EVAL LOW LEVEL Private FunctionPrototypes 00155 * @{ 00156 */ 00157 static void I2Cx_Init(void); 00158 static void I2Cx_ITConfig(void); 00159 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00160 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg); 00161 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length); 00162 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length); 00163 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00164 static void I2Cx_Error(uint8_t Addr); 00165 static void I2Cx_MspInit(void); 00166 00167 static void FSMC_BANK3_WriteData(uint16_t Data); 00168 static void FSMC_BANK3_WriteReg(uint8_t Reg); 00169 static uint16_t FSMC_BANK3_ReadData(void); 00170 static void FSMC_BANK3_Init(void); 00171 static void FSMC_BANK3_MspInit(void); 00172 00173 /* IOExpander IO functions */ 00174 void IOE_Init(void); 00175 void IOE_ITConfig(void); 00176 void IOE_Delay(uint32_t Delay); 00177 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00178 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00179 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00180 00181 /* LCD IO functions */ 00182 void LCD_IO_Init(void); 00183 void LCD_IO_WriteData(uint16_t Data); 00184 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00185 void LCD_IO_WriteReg(uint8_t Reg); 00186 uint16_t LCD_IO_ReadData(uint16_t Reg); 00187 00188 /* AUDIO IO functions */ 00189 void AUDIO_IO_Init(void); 00190 void AUDIO_IO_DeInit(void); 00191 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00192 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00193 00194 /* Camera IO functions */ 00195 void CAMERA_IO_Init(void); 00196 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00197 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg); 00198 void CAMERA_Delay(uint32_t Delay); 00199 00200 /* I2C EEPROM IO function */ 00201 void EEPROM_IO_Init(void); 00202 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00203 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00204 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00205 /** 00206 * @} 00207 */ 00208 00209 /** @defgroup STM324xG_EVAL_LOW_LEVEL_Private_Functions STM324xG EVAL LOW LEVEL Private Functions 00210 * @{ 00211 */ 00212 00213 /** 00214 * @brief This method returns the STM324xG EVAL BSP Driver revision 00215 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00216 */ 00217 uint32_t BSP_GetVersion(void) 00218 { 00219 return __STM324xG_EVAL_BSP_VERSION; 00220 } 00221 00222 /** 00223 * @brief Configures LED GPIO. 00224 * @param Led: LED to be configured. 00225 * This parameter can be one of the following values: 00226 * @arg LED1 00227 * @arg LED2 00228 * @arg LED3 00229 * @arg LED4 00230 */ 00231 void BSP_LED_Init(Led_TypeDef Led) 00232 { 00233 GPIO_InitTypeDef GPIO_InitStruct; 00234 00235 /* Enable the GPIO_LED clock */ 00236 LEDx_GPIO_CLK_ENABLE(Led); 00237 00238 /* Configure the GPIO_LED pin */ 00239 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00240 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00241 GPIO_InitStruct.Pull = GPIO_PULLUP; 00242 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00243 00244 HAL_GPIO_Init(GPIO_PORT[Led], &GPIO_InitStruct); 00245 } 00246 00247 /** 00248 * @brief Turns selected LED On. 00249 * @param Led: LED to be set on 00250 * This parameter can be one of the following values: 00251 * @arg LED1 00252 * @arg LED2 00253 * @arg LED3 00254 * @arg LED4 00255 */ 00256 void BSP_LED_On(Led_TypeDef Led) 00257 { 00258 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00259 } 00260 00261 /** 00262 * @brief Turns selected LED Off. 00263 * @param Led: LED to be set off 00264 * This parameter can be one of the following values: 00265 * @arg LED1 00266 * @arg LED2 00267 * @arg LED3 00268 * @arg LED4 00269 */ 00270 void BSP_LED_Off(Led_TypeDef Led) 00271 { 00272 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00273 } 00274 00275 /** 00276 * @brief Toggles the selected LED. 00277 * @param Led: LED to be toggled 00278 * This parameter can be one of the following values: 00279 * @arg LED1 00280 * @arg LED2 00281 * @arg LED3 00282 * @arg LED4 00283 */ 00284 void BSP_LED_Toggle(Led_TypeDef Led) 00285 { 00286 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00287 } 00288 00289 /** 00290 * @brief Configures button GPIO and EXTI Line. 00291 * @param Button: Button to be configured 00292 * This parameter can be one of the following values: 00293 * @arg BUTTON_WAKEUP: Wakeup Push Button 00294 * @arg BUTTON_TAMPER: Tamper Push Button 00295 * @arg BUTTON_KEY: Key Push Button 00296 * @arg BUTTON_RIGHT: Joystick Right Push Button 00297 * @arg BUTTON_LEFT: Joystick Left Push Button 00298 * @arg BUTTON_UP: Joystick Up Push Button 00299 * @arg BUTTON_DOWN: Joystick Down Push Button 00300 * @arg BUTTON_SEL: Joystick Sel Push Button 00301 * @param Button_Mode: Button mode 00302 * This parameter can be one of the following values: 00303 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00304 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00305 * with interrupt generation capability 00306 */ 00307 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00308 { 00309 GPIO_InitTypeDef GPIO_InitStruct; 00310 00311 /* Enable the BUTTON clock */ 00312 BUTTONx_GPIO_CLK_ENABLE(Button); 00313 00314 if(Button_Mode == BUTTON_MODE_GPIO) 00315 { 00316 /* Configure Button pin as input */ 00317 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00318 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00319 GPIO_InitStruct.Pull = GPIO_NOPULL; 00320 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00321 00322 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00323 } 00324 00325 if(Button_Mode == BUTTON_MODE_EXTI) 00326 { 00327 /* Configure Button pin as input with External interrupt */ 00328 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00329 GPIO_InitStruct.Pull = GPIO_NOPULL; 00330 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00331 00332 if(Button != BUTTON_WAKEUP) 00333 { 00334 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00335 } 00336 else 00337 { 00338 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00339 } 00340 00341 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00342 00343 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00344 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x0); 00345 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00346 } 00347 } 00348 00349 /** 00350 * @brief Returns the selected button state. 00351 * @param Button: Button to be checked 00352 * This parameter can be one of the following values: 00353 * @arg BUTTON_WAKEUP: Wakeup Push Button 00354 * @arg BUTTON_TAMPER: Tamper Push Button 00355 * @arg BUTTON_KEY: Key Push Button 00356 * @arg BUTTON_RIGHT: Joystick Right Push Button 00357 * @arg BUTTON_LEFT: Joystick Left Push Button 00358 * @arg BUTTON_UP: Joystick Up Push Button 00359 * @arg BUTTON_DOWN: Joystick Down Push Button 00360 * @arg BUTTON_SEL: Joystick Sel Push Button 00361 * @retval The Button GPIO pin value 00362 */ 00363 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00364 { 00365 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00366 } 00367 00368 /** 00369 * @brief Configures COM port. 00370 * @param COM: COM port to be configured. 00371 * This parameter can be one of the following values: 00372 * @arg COM1 00373 * @arg COM2 00374 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00375 * configuration information for the specified USART peripheral. 00376 */ 00377 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00378 { 00379 GPIO_InitTypeDef GPIO_InitStruct; 00380 00381 /* Enable GPIO clock */ 00382 EVAL_COMx_TX_GPIO_CLK_ENABLE(COM); 00383 EVAL_COMx_RX_GPIO_CLK_ENABLE(COM); 00384 00385 /* Enable USART clock */ 00386 EVAL_COMx_CLK_ENABLE(COM); 00387 00388 /* Configure USART Tx as alternate function */ 00389 GPIO_InitStruct.Pin = COM_TX_PIN[COM]; 00390 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00391 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00392 GPIO_InitStruct.Pull = GPIO_PULLUP; 00393 GPIO_InitStruct.Alternate = COM_TX_AF[COM]; 00394 HAL_GPIO_Init(COM_TX_PORT[COM], &GPIO_InitStruct); 00395 00396 /* Configure USART Rx as alternate function */ 00397 GPIO_InitStruct.Pin = COM_RX_PIN[COM]; 00398 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00399 GPIO_InitStruct.Alternate = COM_RX_AF[COM]; 00400 HAL_GPIO_Init(COM_RX_PORT[COM], &GPIO_InitStruct); 00401 00402 /* USART configuration */ 00403 huart->Instance = COM_USART[COM]; 00404 HAL_UART_Init(huart); 00405 } 00406 00407 /** 00408 * @brief Configures joystick GPIO and EXTI modes. 00409 * @param Joy_Mode: Button mode. 00410 * This parameter can be one of the following values: 00411 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00412 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00413 * with interrupt generation capability 00414 * @retval IO_OK: if all initializations are OK. Other value if error. 00415 */ 00416 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00417 { 00418 uint8_t ret = 0; 00419 00420 /* Initialize the IO functionalities */ 00421 ret = BSP_IO_Init(); 00422 00423 /* Configure joystick pins in IT mode */ 00424 if(Joy_Mode == JOY_MODE_EXTI) 00425 { 00426 /* Configure joystick pins in IT mode */ 00427 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_FALLING_EDGE); 00428 } 00429 00430 return ret; 00431 } 00432 00433 /** 00434 * @brief Returns the current joystick status. 00435 * @retval Code of the joystick key pressed 00436 * This code can be one of the following values: 00437 * @arg JOY_NONE 00438 * @arg JOY_SEL 00439 * @arg JOY_DOWN 00440 * @arg JOY_LEFT 00441 * @arg JOY_RIGHT 00442 * @arg JOY_UP 00443 */ 00444 JOYState_TypeDef BSP_JOY_GetState(void) 00445 { 00446 uint8_t tmp = 0; 00447 00448 /* Read the status joystick pins */ 00449 tmp = (uint8_t)BSP_IO_ReadPin(JOY_ALL_PINS); 00450 00451 /* Check the pressed keys */ 00452 if((tmp & JOY_NONE_PIN) == JOY_NONE) 00453 { 00454 return(JOYState_TypeDef) JOY_NONE; 00455 } 00456 else if(!(tmp & JOY_SEL_PIN)) 00457 { 00458 return(JOYState_TypeDef) JOY_SEL; 00459 } 00460 else if(!(tmp & JOY_DOWN_PIN)) 00461 { 00462 return(JOYState_TypeDef) JOY_DOWN; 00463 } 00464 else if(!(tmp & JOY_LEFT_PIN)) 00465 { 00466 return(JOYState_TypeDef) JOY_LEFT; 00467 } 00468 else if(!(tmp & JOY_RIGHT_PIN)) 00469 { 00470 return(JOYState_TypeDef) JOY_RIGHT; 00471 } 00472 else if(!(tmp & JOY_UP_PIN)) 00473 { 00474 return(JOYState_TypeDef) JOY_UP; 00475 } 00476 else 00477 { 00478 return(JOYState_TypeDef) JOY_NONE; 00479 } 00480 } 00481 00482 /******************************************************************************* 00483 BUS OPERATIONS 00484 *******************************************************************************/ 00485 00486 /**************************** I2C Routines ************************************/ 00487 00488 /** 00489 * @brief Initializes I2C MSP. 00490 */ 00491 static void I2Cx_MspInit(void) 00492 { 00493 GPIO_InitTypeDef GPIO_InitStruct; 00494 00495 /*** Configure the GPIOs ***/ 00496 /* Enable GPIO clock */ 00497 EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00498 00499 /* Configure I2C Tx as alternate function */ 00500 GPIO_InitStruct.Pin = EVAL_I2Cx_SCL_PIN; 00501 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00502 GPIO_InitStruct.Pull = GPIO_NOPULL; 00503 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00504 GPIO_InitStruct.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00505 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &GPIO_InitStruct); 00506 00507 /* Configure I2C Rx as alternate function */ 00508 GPIO_InitStruct.Pin = EVAL_I2Cx_SDA_PIN; 00509 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &GPIO_InitStruct); 00510 00511 /*** Configure the I2C peripheral ***/ 00512 /* Enable I2C clock */ 00513 EVAL_I2Cx_CLK_ENABLE(); 00514 00515 /* Force the I2C peripheral clock reset */ 00516 EVAL_I2Cx_FORCE_RESET(); 00517 00518 /* Release the I2C peripheral clock reset */ 00519 EVAL_I2Cx_RELEASE_RESET(); 00520 00521 /* Set priority and enable I2Cx event Interrupt */ 00522 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 5, 0); 00523 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00524 00525 /* Set priority and enable I2Cx error Interrupt */ 00526 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 5, 0); 00527 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00528 } 00529 00530 /** 00531 * @brief Initializes I2C HAL. 00532 */ 00533 static void I2Cx_Init(void) 00534 { 00535 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00536 { 00537 heval_I2c.Instance = EVAL_I2Cx; 00538 heval_I2c.Init.ClockSpeed = BSP_I2C_SPEED; 00539 heval_I2c.Init.DutyCycle = I2C_DUTYCYCLE_2; 00540 heval_I2c.Init.OwnAddress1 = 0; 00541 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00542 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLED; 00543 heval_I2c.Init.OwnAddress2 = 0; 00544 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLED; 00545 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLED; 00546 00547 /* Init the I2C */ 00548 I2Cx_MspInit(); 00549 HAL_I2C_Init(&heval_I2c); 00550 } 00551 } 00552 00553 /** 00554 * @brief Configures I2C Interrupt. 00555 */ 00556 static void I2Cx_ITConfig(void) 00557 { 00558 static uint8_t I2C_IT_Enabled = 0; 00559 GPIO_InitTypeDef GPIO_InitStruct; 00560 00561 if(I2C_IT_Enabled == 0) 00562 { 00563 I2C_IT_Enabled = 1; 00564 00565 /* Enable the GPIO EXTI clock */ 00566 __GPIOI_CLK_ENABLE(); 00567 __SYSCFG_CLK_ENABLE(); 00568 00569 GPIO_InitStruct.Pin = GPIO_PIN_2; 00570 GPIO_InitStruct.Pull = GPIO_NOPULL; 00571 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00572 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00573 HAL_GPIO_Init(GPIOI, &GPIO_InitStruct); 00574 00575 /* Set priority and Enable GPIO EXTI Interrupt */ 00576 HAL_NVIC_SetPriority((IRQn_Type)(EXTI2_IRQn), 5, 0); 00577 HAL_NVIC_EnableIRQ((IRQn_Type)(EXTI2_IRQn)); 00578 } 00579 } 00580 00581 /** 00582 * @brief Reads a single data. 00583 * @param Addr: I2C address 00584 * @param Reg: Reg address 00585 * @retval Data to be read 00586 */ 00587 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg) 00588 { 00589 HAL_StatusTypeDef status = HAL_OK; 00590 uint8_t Value = 0; 00591 00592 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2C_TIMEOUT); 00593 00594 /* Check the communication status */ 00595 if(status != HAL_OK) 00596 { 00597 /* Execute user timeout callback */ 00598 I2Cx_Error(Addr); 00599 } 00600 00601 return Value; 00602 } 00603 00604 /** 00605 * @brief Writes a single data. 00606 * @param Addr: I2C address 00607 * @param Reg: Reg address 00608 * @param Value: Data to be written 00609 */ 00610 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00611 { 00612 HAL_StatusTypeDef status = HAL_OK; 00613 00614 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2C_TIMEOUT); 00615 00616 /* Check the communication status */ 00617 if(status != HAL_OK) 00618 { 00619 /* I2C error occured */ 00620 I2Cx_Error(Addr); 00621 } 00622 } 00623 00624 /** 00625 * @brief Reads multiple data. 00626 * @param Addr: I2C address 00627 * @param Reg: Reg address 00628 * @param MemAddress Internal memory address 00629 * @param Buffer: Pointer to data buffer 00630 * @param Length: Length of the data 00631 * @retval Number of read data 00632 */ 00633 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00634 { 00635 HAL_StatusTypeDef status = HAL_OK; 00636 00637 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, I2C_TIMEOUT); 00638 00639 /* Check the communication status */ 00640 if(status != HAL_OK) 00641 { 00642 /* I2C error occured */ 00643 I2Cx_Error(Addr); 00644 } 00645 return status; 00646 } 00647 00648 /** 00649 * @brief Write a value in a register of the device through BUS in using DMA mode 00650 * @param Addr: Device address on BUS Bus. 00651 * @param Reg: The target register address to write 00652 * @param MemAddress Internal memory address 00653 * @param Buffer: The target register value to be written 00654 * @param Length: buffer size to be written 00655 * @retval HAL status 00656 */ 00657 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00658 { 00659 HAL_StatusTypeDef status = HAL_OK; 00660 00661 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, I2C_TIMEOUT); 00662 00663 /* Check the communication status */ 00664 if(status != HAL_OK) 00665 { 00666 /* Re-Initiaize the I2C Bus */ 00667 I2Cx_Error(Addr); 00668 } 00669 return status; 00670 } 00671 00672 /** 00673 * @brief Checks if target device is ready for communication. 00674 * @note This function is used with Memory devices 00675 * @param DevAddress: Target device address 00676 * @param Trials: Number of trials 00677 * @retval HAL status 00678 */ 00679 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00680 { 00681 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, I2C_TIMEOUT)); 00682 } 00683 00684 /** 00685 * @brief Manages error callback by re-initializing I2C. 00686 * @param Addr: I2C Address 00687 */ 00688 static void I2Cx_Error(uint8_t Addr) 00689 { 00690 /* De-initialize the IOE comunication BUS */ 00691 HAL_I2C_DeInit(&heval_I2c); 00692 00693 /* Re-Initiaize the IOE comunication BUS */ 00694 I2Cx_Init(); 00695 } 00696 00697 /*************************** FSMC Routines ************************************/ 00698 /** 00699 * @brief Initializes FSMC_BANK3 MSP. 00700 */ 00701 static void FSMC_BANK3_MspInit(void) 00702 { 00703 GPIO_InitTypeDef GPIO_Init_Structure; 00704 00705 /* Enable FSMC clock */ 00706 __FSMC_CLK_ENABLE(); 00707 00708 /* Enable GPIOs clock */ 00709 __GPIOD_CLK_ENABLE(); 00710 __GPIOE_CLK_ENABLE(); 00711 __GPIOF_CLK_ENABLE(); 00712 __GPIOG_CLK_ENABLE(); 00713 00714 /* Common GPIO configuration */ 00715 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00716 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00717 GPIO_Init_Structure.Speed = GPIO_SPEED_HIGH; 00718 GPIO_Init_Structure.Alternate = GPIO_AF12_FSMC; 00719 00720 /* GPIOD configuration */ 00721 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_8 |\ 00722 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 |\ 00723 GPIO_PIN_14 | GPIO_PIN_15; 00724 00725 HAL_GPIO_Init(GPIOD, &GPIO_Init_Structure); 00726 00727 /* GPIOE configuration */ 00728 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3| GPIO_PIN_4 | GPIO_PIN_7 |\ 00729 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00730 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00731 HAL_GPIO_Init(GPIOE, &GPIO_Init_Structure); 00732 00733 /* GPIOF configuration */ 00734 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00735 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00736 HAL_GPIO_Init(GPIOF, &GPIO_Init_Structure); 00737 00738 /* GPIOG configuration */ 00739 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00740 GPIO_PIN_5 | GPIO_PIN_10; 00741 00742 HAL_GPIO_Init(GPIOG, &GPIO_Init_Structure); 00743 } 00744 00745 /** 00746 * @brief Initializes LCD IO. 00747 */ 00748 static void FSMC_BANK3_Init(void) 00749 { 00750 SRAM_HandleTypeDef hsram; 00751 FSMC_NORSRAM_TimingTypeDef SRAM_Timing; 00752 00753 /*** Configure the SRAM Bank 3 ***/ 00754 /* Configure IPs */ 00755 hsram.Instance = FMC_NORSRAM_DEVICE; 00756 hsram.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00757 00758 SRAM_Timing.AddressSetupTime = 5; 00759 SRAM_Timing.AddressHoldTime = 1; 00760 SRAM_Timing.DataSetupTime = 9; 00761 SRAM_Timing.BusTurnAroundDuration = 0; 00762 SRAM_Timing.CLKDivision = 2; 00763 SRAM_Timing.DataLatency = 2; 00764 SRAM_Timing.AccessMode = FSMC_ACCESS_MODE_A; 00765 00766 hsram.Init.NSBank = FSMC_NORSRAM_BANK3; 00767 hsram.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00768 hsram.Init.MemoryType = FSMC_MEMORY_TYPE_SRAM; 00769 hsram.Init.MemoryDataWidth = FSMC_NORSRAM_MEM_BUS_WIDTH_16; 00770 hsram.Init.BurstAccessMode = FSMC_BURST_ACCESS_MODE_DISABLE; 00771 hsram.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00772 hsram.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00773 hsram.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00774 hsram.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00775 hsram.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00776 hsram.Init.ExtendedMode = FSMC_EXTENDED_MODE_DISABLE; 00777 hsram.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00778 hsram.Init.WriteBurst = FSMC_WRITE_BURST_DISABLE; 00779 00780 /* Initialize the SRAM controller */ 00781 FSMC_BANK3_MspInit(); 00782 HAL_SRAM_Init(&hsram, &SRAM_Timing, &SRAM_Timing); 00783 } 00784 00785 /** 00786 * @brief Writes register value. 00787 * @param Data: Data to be written 00788 */ 00789 static void FSMC_BANK3_WriteData(uint16_t Data) 00790 { 00791 /* Write 16-bit Reg */ 00792 FMC_BANK3->RAM = Data; 00793 } 00794 00795 /** 00796 * @brief Writes register address. 00797 * @param Reg: Register to be written 00798 */ 00799 static void FSMC_BANK3_WriteReg(uint8_t Reg) 00800 { 00801 /* Write 16-bit Index, then write register */ 00802 FMC_BANK3->REG = Reg; 00803 } 00804 00805 /** 00806 * @brief Reads register value. 00807 * @retval Read value 00808 */ 00809 static uint16_t FSMC_BANK3_ReadData(void) 00810 { 00811 return FMC_BANK3->RAM; 00812 } 00813 00814 /******************************************************************************* 00815 LINK OPERATIONS 00816 *******************************************************************************/ 00817 00818 /***************************** LINK IOE ***************************************/ 00819 00820 /** 00821 * @brief Initializes IOE low level. 00822 */ 00823 void IOE_Init(void) 00824 { 00825 I2Cx_Init(); 00826 } 00827 00828 /** 00829 * @brief Configures IOE low level Interrupt. 00830 */ 00831 void IOE_ITConfig(void) 00832 { 00833 I2Cx_ITConfig(); 00834 } 00835 00836 /** 00837 * @brief IOE writes single data. 00838 * @param Addr: I2C address 00839 * @param Reg: Reg address 00840 * @param Value: Data to be written 00841 */ 00842 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00843 { 00844 I2Cx_Write(Addr, Reg, Value); 00845 } 00846 00847 /** 00848 * @brief IOE reads single data. 00849 * @param Addr: I2C address 00850 * @param Reg: Reg address 00851 * @retval Read data 00852 */ 00853 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 00854 { 00855 return I2Cx_Read(Addr, Reg); 00856 } 00857 00858 /** 00859 * @brief IOE reads multiple data. 00860 * @param Addr: I2C address 00861 * @param Reg: Reg address 00862 * @param Buffer: Pointer to data buffer 00863 * @param Length: Length of the data 00864 * @retval Number of read data 00865 */ 00866 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00867 { 00868 return I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00869 } 00870 00871 /** 00872 * @brief IOE delay. 00873 * @param Delay: Delay in ms 00874 */ 00875 void IOE_Delay(uint32_t Delay) 00876 { 00877 HAL_Delay(Delay); 00878 } 00879 00880 /********************************* LINK LCD ***********************************/ 00881 00882 /** 00883 * @brief Initializes LCD low level. 00884 */ 00885 void LCD_IO_Init(void) 00886 { 00887 if(Is_LCD_IO_Initialized == 0) 00888 { 00889 Is_LCD_IO_Initialized = 1; 00890 FSMC_BANK3_Init(); 00891 } 00892 } 00893 00894 /** 00895 * @brief Writes data on LCD data register. 00896 * @param Data: Data to be written 00897 */ 00898 void LCD_IO_WriteData(uint16_t Data) 00899 { 00900 /* Write 16-bit Reg */ 00901 FSMC_BANK3_WriteData(Data); 00902 } 00903 00904 /** 00905 * @brief Write register value. 00906 * @param pData Pointer on the register value 00907 * @param Size Size of byte to transmit to the register 00908 */ 00909 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00910 { 00911 uint32_t counter; 00912 uint16_t *ptr = (uint16_t *) pData; 00913 00914 for (counter = 0; counter < Size; counter+=2) 00915 { 00916 /* Write 16-bit Reg */ 00917 FSMC_BANK3_WriteData(*ptr); 00918 ptr++; 00919 } 00920 } 00921 00922 /** 00923 * @brief Writes register on LCD register. 00924 * @param Reg: Register to be written 00925 */ 00926 void LCD_IO_WriteReg(uint8_t Reg) 00927 { 00928 /* Write 16-bit Index, then Write Reg */ 00929 FSMC_BANK3_WriteReg(Reg); 00930 } 00931 00932 /** 00933 * @brief Reads data from LCD data register. 00934 * @param Reg: Register to be read 00935 * @retval Read data. 00936 */ 00937 uint16_t LCD_IO_ReadData(uint16_t Reg) 00938 { 00939 FSMC_BANK3_WriteReg(Reg); 00940 00941 /* Read 16-bit Reg */ 00942 return FSMC_BANK3_ReadData(); 00943 } 00944 00945 /********************************* LINK AUDIO *********************************/ 00946 /** 00947 * @brief Initializes Audio low level. 00948 */ 00949 void AUDIO_IO_Init(void) 00950 { 00951 I2Cx_Init(); 00952 } 00953 00954 /** 00955 * @brief DeInitializes Audio low level. 00956 * @note This function is intentionally kept empty, user should define it. 00957 */ 00958 void AUDIO_IO_DeInit(void) 00959 { 00960 00961 } 00962 00963 /** 00964 * @brief Writes a single data. 00965 * @param Addr: I2C address 00966 * @param Reg: Reg address 00967 * @param Value: Data to be written 00968 */ 00969 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00970 { 00971 I2Cx_Write(Addr, Reg, Value); 00972 } 00973 00974 /** 00975 * @brief Reads a single data. 00976 * @param Addr: I2C address 00977 * @param Reg: Reg address 00978 * @retval Data to be read 00979 */ 00980 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg) 00981 { 00982 return I2Cx_Read(Addr, Reg); 00983 } 00984 00985 /***************************** LINK CAMERA ************************************/ 00986 00987 /** 00988 * @brief Initializes Camera low level. 00989 */ 00990 void CAMERA_IO_Init(void) 00991 { 00992 I2Cx_Init(); 00993 } 00994 00995 /** 00996 * @brief Camera writes single data. 00997 * @param Addr: I2C address 00998 * @param Reg: Reg address 00999 * @param Value: Data to be written 01000 */ 01001 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01002 { 01003 I2Cx_Write(Addr, Reg, Value); 01004 } 01005 01006 /** 01007 * @brief Camera reads single data. 01008 * @param Addr: I2C address 01009 * @param Reg: Reg address 01010 * @retval Read data 01011 */ 01012 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg) 01013 { 01014 return I2Cx_Read(Addr, Reg); 01015 } 01016 01017 /** 01018 * @brief Camera delay. 01019 * @param Delay: Delay in ms 01020 */ 01021 void CAMERA_Delay(uint32_t Delay) 01022 { 01023 HAL_Delay(Delay); 01024 } 01025 01026 /******************************** LINK I2C EEPROM *****************************/ 01027 01028 /** 01029 * @brief Initializes peripherals used by the I2C EEPROM driver. 01030 */ 01031 void EEPROM_IO_Init(void) 01032 { 01033 I2Cx_Init(); 01034 } 01035 01036 /** 01037 * @brief Write data to I2C EEPROM driver in using DMA channel 01038 * @param DevAddress: Target device address 01039 * @param MemAddress: Internal memory address 01040 * @param pBuffer: Pointer to data buffer 01041 * @param BufferSize: Amount of data to be sent 01042 * @retval HAL status 01043 */ 01044 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01045 { 01046 return (I2Cx_WriteMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01047 } 01048 01049 /** 01050 * @brief Reads data from I2C EEPROM driver in using DMA channel. 01051 * @param DevAddress: Target device address 01052 * @param MemAddress: Internal memory address 01053 * @param pBuffer: Pointer to data buffer 01054 * @param BufferSize: Amount of data to be read 01055 * @retval HAL status 01056 */ 01057 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01058 { 01059 return (I2Cx_ReadMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01060 } 01061 01062 /** 01063 * @brief Checks if target device is ready for communication. 01064 * @note This function is used with Memory devices 01065 * @param DevAddress: Target device address 01066 * @param Trials: Number of trials 01067 * @retval HAL status 01068 */ 01069 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01070 { 01071 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01072 } 01073 01074 /** 01075 * @} 01076 */ 01077 01078 /** 01079 * @} 01080 */ 01081 01082 /** 01083 * @} 01084 */ 01085 01086 /** 01087 * @} 01088 */ 01089 01090 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 14:22:29 for STM324xG_EVAL BSP User Manual by
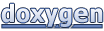