STM32469I_EVAL BSP User Manual
|
stm32469i_eval_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_eval_ts.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 12-January-2016 00007 * @brief This file provides a set of functions needed to manage the Touch 00008 * Screen on STM32469I-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the touch screen module of the STM32469I-EVAL 00044 evaluation board on the K.O.D Optica Technology 480x800 TFT-LCD mounted on 00045 MB1166 daughter board. The touch screen driver IC inside the K.O.D module KM-040TMP-02 00046 is a FT6206 by Focal Tech. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the TS module using the BSP_TS_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 communication layer configuration to start the TS use. The LCD size properties 00054 (x and y) are passed as parameters. 00055 o If TS interrupt mode is desired, you must configure the TS interrupt mode 00056 by calling the function BSP_TS_ITConfig(). The TS interrupt mode is generated 00057 as an external interrupt whenever a touch is detected. 00058 The interrupt mode internally uses the IO functionalities driver driven by 00059 the IO expander, to configure the IT line. 00060 00061 + Touch screen use 00062 o The touch screen state is captured whenever the function BSP_TS_GetState() is 00063 used. This function returns information about the last LCD touch occurred 00064 in the TS_StateTypeDef structure. 00065 o If TS interrupt mode is used, the function BSP_TS_ITGetStatus() is needed to get 00066 the interrupt status. To clear the IT pending bits, you should call the 00067 function BSP_TS_ITClear(). 00068 o The IT is handled using the corresponding external interrupt IRQ handler, 00069 the user IT callback treatment is implemented on the same external interrupt 00070 callback. 00071 00072 ------------------------------------------------------------------------------*/ 00073 00074 /* Includes ------------------------------------------------------------------*/ 00075 #include "stm32469i_eval.h" 00076 #include "stm32469i_eval_ts.h" 00077 00078 /** @addtogroup BSP 00079 * @{ 00080 */ 00081 00082 /** @addtogroup STM32469I_EVAL 00083 * @{ 00084 */ 00085 00086 /** @defgroup STM32469I-EVAL_TS STM32469I EVAL TS 00087 * @{ 00088 */ 00089 00090 /** @defgroup STM32469I-EVAL_TS_Private_Types_Definitions STM32469I EVAL TS Private Types Definitions 00091 * @{ 00092 */ 00093 /** 00094 * @} 00095 */ 00096 00097 /** @defgroup STM32469I-EVAL_TS_Private_Defines STM32469I EVAL TS Private Types Defines 00098 * @{ 00099 */ 00100 /** 00101 * @} 00102 */ 00103 00104 /** @defgroup STM32469I-EVAL_TS_Private_Macros STM32469I EVAL TS Private Macros 00105 * @{ 00106 */ 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM32469I-EVAL_TS_Imported_Variables STM32469I EVAL TS Imported Variables 00112 * @{ 00113 */ 00114 /** 00115 * @} 00116 */ 00117 00118 /** @defgroup STM32469I-EVAL_TS_Private_Variables STM32469I EVAL TS Private Variables 00119 * @{ 00120 */ 00121 static TS_DrvTypeDef *ts_driver; 00122 static uint8_t ts_orientation; 00123 static uint8_t I2C_Address = 0; 00124 00125 /* Table for touchscreen event information display on LCD : table indexed on enum @ref TS_TouchEventTypeDef information */ 00126 char * ts_event_string_tab[TOUCH_EVENT_NB_MAX] = { "None", 00127 "Press down", 00128 "Lift up", 00129 "Contact" 00130 }; 00131 00132 /* Table for touchscreen gesture Id information display on LCD : table indexed on enum @ref TS_GestureIdTypeDef information */ 00133 char * ts_gesture_id_string_tab[GEST_ID_NB_MAX] = { "None", 00134 "Move Up", 00135 "Move Right", 00136 "Move Down", 00137 "Move Left", 00138 "Zoom In", 00139 "Zoom Out" 00140 }; 00141 00142 /** 00143 * @} 00144 */ 00145 00146 /** @defgroup STM32469I-EVAL_TS_Private_Function_Prototypes STM32469I EVAL TS Private Function Prototypes 00147 * @{ 00148 */ 00149 00150 /** 00151 * @} 00152 */ 00153 00154 /** @defgroup STM32469I-EVAL_TS_Public_Functions STM32469I EVAL TS Public Functions 00155 * @{ 00156 */ 00157 00158 /** 00159 * @brief Initializes and configures the touch screen functionalities and 00160 * configures all necessary hardware resources (GPIOs, I2C, clocks..). 00161 * @param ts_SizeX : Maximum X size of the TS area on LCD 00162 * @param ts_SizeY : Maximum Y size of the TS area on LCD 00163 * @retval TS_OK if all initializations are OK. Other value if error. 00164 */ 00165 uint8_t BSP_TS_Init(uint16_t ts_SizeX, uint16_t ts_SizeY) 00166 { 00167 uint8_t ts_status = TS_OK; 00168 00169 /* Note : I2C_Address is un-initialized here, but is not used at all in init function */ 00170 /* but the prototype of Init() is like that in template and should be respected */ 00171 00172 /* Initialize the communication channel to sensor (I2C) if necessary */ 00173 /* that is initialization is done only once after a power up */ 00174 ft6x06_ts_drv.Init(I2C_Address); 00175 00176 /* Scan FT6x06 TouchScreen IC controller ID register by I2C Read */ 00177 /* Verify this is a FT6x06, otherwise this is an error case */ 00178 if(ft6x06_ts_drv.ReadID(TS_I2C_ADDRESS) == FT6206_ID_VALUE) 00179 { 00180 /* Found FT6206 : Initialize the TS driver structure */ 00181 ts_driver = &ft6x06_ts_drv; 00182 00183 I2C_Address = TS_I2C_ADDRESS; 00184 00185 /* Get LCD chosen orientation */ 00186 if(ts_SizeX < ts_SizeY) 00187 { 00188 ts_orientation = TS_SWAP_NONE; 00189 } 00190 else 00191 { 00192 ts_orientation = TS_SWAP_XY | TS_SWAP_Y; 00193 } 00194 00195 if(ts_status == TS_OK) 00196 { 00197 /* Software reset the TouchScreen */ 00198 ts_driver->Reset(I2C_Address); 00199 00200 /* Calibrate, Configure and Start the TouchScreen driver */ 00201 ts_driver->Start(I2C_Address); 00202 00203 } /* of if(ts_status == TS_OK) */ 00204 } 00205 else 00206 { 00207 ts_status = TS_DEVICE_NOT_FOUND; 00208 } 00209 00210 return (ts_status); 00211 } 00212 00213 /** 00214 * @brief Configures and enables the touch screen interrupts. 00215 * @retval TS_OK if all initializations are OK. Other value if error. 00216 */ 00217 uint8_t BSP_TS_ITConfig(void) 00218 { 00219 uint8_t ts_status = TS_ERROR; 00220 uint8_t io_status = IO_ERROR; 00221 00222 /* Initialize the IO */ 00223 io_status = BSP_IO_Init(); 00224 if(io_status != IO_OK) 00225 { 00226 return (ts_status); 00227 } 00228 00229 /* Configure TS IT line IO : is active low on FT6206 (see data sheet) */ 00230 /* Configure TS_INT_PIN (MFX_IO_14) low level to generate MFX_IRQ_OUT in EXTI on MCU */ 00231 /* This will call HAL_GPIO_EXTI_Callback() that is setting variable 'mfx_exti_received' to 1b1' */ 00232 io_status = BSP_IO_ConfigPin(TS_INT_PIN, IO_MODE_IT_LOW_LEVEL_PU); 00233 if(io_status != IO_OK) 00234 { 00235 return (ts_status); 00236 } 00237 00238 /* Enable the TS in interrupt mode */ 00239 /* In that case the INT output of FT6206 when new touch is available */ 00240 /* is active low and directed on MFX IO14 */ 00241 ts_driver->EnableIT(I2C_Address); 00242 00243 /* If arrived here : set good status on exit */ 00244 ts_status = TS_OK; 00245 00246 return (ts_status); 00247 } 00248 00249 /** 00250 * @brief Gets the touch screen interrupt status. 00251 * @retval TS_IRQ_PENDING if touchscreen IRQ is pending, TS_NO_IRQ_PENDING when no IRQ TS is pending. 00252 */ 00253 uint8_t BSP_TS_ITGetStatus(void) 00254 { 00255 uint8_t itStatus = TS_NO_IRQ_PENDING; /* By default no IRQ TS pending */ 00256 uint32_t mfx_irq_status = 0; /* No MFX IRQ by default */ 00257 00258 /* Check status of MFX_IO14 in particular which is the Touch Screen INT pin active low */ 00259 mfx_irq_status = BSP_IO_ITGetStatus(TS_INT_PIN); 00260 if(mfx_irq_status != 0) /* Note : returned mfx_irq_status = 0x4000 == (1<<TS_INT_PIN) == (1<<14) */ 00261 { 00262 /* This is Touch Screen INT case : so this is a new touch available that produced the IRQ EXTI */ 00263 itStatus = TS_IRQ_PENDING; 00264 } 00265 00266 /* Return the TS IT status */ 00267 return (itStatus); 00268 } 00269 00270 /** 00271 * @brief Returns status and positions of the touch screen. 00272 * @param TS_State: Pointer to touch screen current state structure 00273 * @retval TS_OK if all initializations are OK. Other value if error. 00274 */ 00275 uint8_t BSP_TS_GetState(TS_StateTypeDef *TS_State) 00276 { 00277 static uint32_t _x[TS_MAX_NB_TOUCH] = {0, 0}; 00278 static uint32_t _y[TS_MAX_NB_TOUCH] = {0, 0}; 00279 uint8_t ts_status = TS_OK; 00280 uint16_t tmp; 00281 uint16_t Raw_x[TS_MAX_NB_TOUCH]; 00282 uint16_t Raw_y[TS_MAX_NB_TOUCH]; 00283 uint16_t xDiff; 00284 uint16_t yDiff; 00285 uint32_t index; 00286 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00287 uint32_t weight = 0; 00288 uint32_t area = 0; 00289 uint32_t event = 0; 00290 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00291 00292 /* Check and update the number of touches active detected */ 00293 TS_State->touchDetected = ts_driver->DetectTouch(I2C_Address); 00294 if(TS_State->touchDetected) 00295 { 00296 for(index=0; index < TS_State->touchDetected; index++) 00297 { 00298 /* Get each touch coordinates */ 00299 ts_driver->GetXY(I2C_Address, &(Raw_x[index]), &(Raw_y[index])); 00300 00301 if(ts_orientation & TS_SWAP_XY) 00302 { 00303 tmp = Raw_x[index]; 00304 Raw_x[index] = Raw_y[index]; 00305 Raw_y[index] = tmp; 00306 } 00307 00308 if(ts_orientation & TS_SWAP_X) 00309 { 00310 Raw_x[index] = FT_6206_MAX_WIDTH - 1 - Raw_x[index]; 00311 } 00312 00313 if(ts_orientation & TS_SWAP_Y) 00314 { 00315 Raw_y[index] = FT_6206_MAX_HEIGHT - 1 - Raw_y[index]; 00316 } 00317 00318 xDiff = Raw_x[index] > _x[index]? (Raw_x[index] - _x[index]): (_x[index] - Raw_x[index]); 00319 yDiff = Raw_y[index] > _y[index]? (Raw_y[index] - _y[index]): (_y[index] - Raw_y[index]); 00320 00321 if ((xDiff + yDiff) > 5) 00322 { 00323 _x[index] = Raw_x[index]; 00324 _y[index] = Raw_y[index]; 00325 } 00326 00327 00328 TS_State->touchX[index] = _x[index]; 00329 TS_State->touchY[index] = _y[index]; 00330 00331 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00332 00333 /* Get touch info related to the current touch */ 00334 ft6x06_TS_GetTouchInfo(I2C_Address, index, &weight, &area, &event); 00335 00336 /* Update TS_State structure */ 00337 TS_State->touchWeight[index] = weight; 00338 TS_State->touchArea[index] = area; 00339 00340 /* Remap touch event */ 00341 switch(event) 00342 { 00343 case FT6206_TOUCH_EVT_FLAG_PRESS_DOWN : 00344 TS_State->touchEventId[index] = TOUCH_EVENT_PRESS_DOWN; 00345 break; 00346 case FT6206_TOUCH_EVT_FLAG_LIFT_UP : 00347 TS_State->touchEventId[index] = TOUCH_EVENT_LIFT_UP; 00348 break; 00349 case FT6206_TOUCH_EVT_FLAG_CONTACT : 00350 TS_State->touchEventId[index] = TOUCH_EVENT_CONTACT; 00351 break; 00352 case FT6206_TOUCH_EVT_FLAG_NO_EVENT : 00353 TS_State->touchEventId[index] = TOUCH_EVENT_NO_EVT; 00354 break; 00355 default : 00356 ts_status = TS_ERROR; 00357 break; 00358 } /* of switch(event) */ 00359 00360 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00361 00362 } /* of for(index=0; index < TS_State->touchDetected; index++) */ 00363 00364 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00365 /* Get gesture Id */ 00366 ts_status = BSP_TS_Get_GestureId(TS_State); 00367 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00368 00369 } /* end of if(TS_State->touchDetected != 0) */ 00370 00371 return (ts_status); 00372 } 00373 00374 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00375 /** 00376 * @brief Update gesture Id following a touch detected. 00377 * @param TS_State: Pointer to touch screen current state structure 00378 * @retval TS_OK if all initializations are OK. Other value if error. 00379 */ 00380 uint8_t BSP_TS_Get_GestureId(TS_StateTypeDef *TS_State) 00381 { 00382 uint32_t gestureId = 0; 00383 uint8_t ts_status = TS_OK; 00384 00385 /* Get gesture Id */ 00386 ft6x06_TS_GetGestureID(I2C_Address, &gestureId); 00387 00388 /* Remap gesture Id to a TS_GestureIdTypeDef value */ 00389 switch(gestureId) 00390 { 00391 case FT6206_GEST_ID_NO_GESTURE : 00392 TS_State->gestureId = GEST_ID_NO_GESTURE; 00393 break; 00394 case FT6206_GEST_ID_MOVE_UP : 00395 TS_State->gestureId = GEST_ID_MOVE_UP; 00396 break; 00397 case FT6206_GEST_ID_MOVE_RIGHT : 00398 TS_State->gestureId = GEST_ID_MOVE_RIGHT; 00399 break; 00400 case FT6206_GEST_ID_MOVE_DOWN : 00401 TS_State->gestureId = GEST_ID_MOVE_DOWN; 00402 break; 00403 case FT6206_GEST_ID_MOVE_LEFT : 00404 TS_State->gestureId = GEST_ID_MOVE_LEFT; 00405 break; 00406 case FT6206_GEST_ID_ZOOM_IN : 00407 TS_State->gestureId = GEST_ID_ZOOM_IN; 00408 break; 00409 case FT6206_GEST_ID_ZOOM_OUT : 00410 TS_State->gestureId = GEST_ID_ZOOM_OUT; 00411 break; 00412 default : 00413 ts_status = TS_ERROR; 00414 break; 00415 } /* of switch(gestureId) */ 00416 00417 return(ts_status); 00418 } 00419 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00420 00421 00422 /** 00423 * @brief Clears all touch screen interrupts. 00424 */ 00425 void BSP_TS_ITClear(void) 00426 { 00427 /* Clear TS_INT_PIN IRQ in MFX */ 00428 BSP_IO_ITClearPin(TS_INT_PIN); 00429 } 00430 00431 /** 00432 * @} 00433 */ 00434 00435 /** @defgroup STM32469I-EVAL_TS_Private_Functions STM32469I EVAL TS Private Functions 00436 * @{ 00437 */ 00438 00439 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00440 /** 00441 * @brief Function used to reset all touch data before a new acquisition 00442 * of touch information. 00443 * @param TS_State: Pointer to touch screen current state structure 00444 * @retval TS_OK if OK, TE_ERROR if problem found. 00445 */ 00446 uint8_t BSP_TS_ResetTouchData(TS_StateTypeDef *TS_State) 00447 { 00448 uint8_t ts_status = TS_ERROR; 00449 uint32_t index; 00450 00451 if (TS_State != (TS_StateTypeDef *)NULL) 00452 { 00453 TS_State->gestureId = GEST_ID_NO_GESTURE; 00454 TS_State->touchDetected = 0; 00455 00456 for(index = 0; index < TS_MAX_NB_TOUCH; index++) 00457 { 00458 TS_State->touchX[index] = 0; 00459 TS_State->touchY[index] = 0; 00460 TS_State->touchArea[index] = 0; 00461 TS_State->touchEventId[index] = TOUCH_EVENT_NO_EVT; 00462 TS_State->touchWeight[index] = 0; 00463 } 00464 00465 ts_status = TS_OK; 00466 00467 } /* of if (TS_State != (TS_StateTypeDef *)NULL) */ 00468 00469 return (ts_status); 00470 } 00471 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00472 /** 00473 * @} 00474 */ 00475 00476 /** 00477 * @} 00478 */ 00479 00480 /** 00481 * @} 00482 */ 00483 00484 /** 00485 * @} 00486 */ 00487 00488 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 12 2016 17:51:25 for STM32469I_EVAL BSP User Manual by
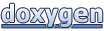