STM32469I_EVAL BSP User Manual
|
stm32469i_eval_sram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_eval_sram.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 12-January-2016 00007 * @brief This file includes the SRAM driver for the IS61WV102416BLL-10M memory 00008 * device mounted on STM32469I-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the IS61WV102416BLL-10M SRAM external memory mounted 00044 on STM32469I-EVAL evaluation board. 00045 - This driver does not need a specific component driver for the SRAM device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the SRAM external memory using the BSP_SRAM_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 FMC controller configuration to interface with the external SRAM memory. 00054 00055 + SRAM read/write operations 00056 o SRAM external memory can be accessed with read/write operations once it is 00057 initialized. 00058 Read/write operation can be performed with AHB access using the functions 00059 BSP_SRAM_ReadData()/BSP_SRAM_WriteData(), or by DMA transfer using the functions 00060 BSP_SRAM_ReadData_DMA()/BSP_SRAM_WriteData_DMA(). 00061 o The AHB access is performed with 16-bit width transaction, the DMA transfer 00062 configuration is fixed at single (no burst) halfword transfer 00063 (see the BSP_SRAM_MspInit() __weak function). This function BSP_SRAM_MspInit() 00064 can be surcharged by application code above BSP. 00065 o User can implement his own functions for read/write access with his desired 00066 configurations. 00067 o If interrupt mode is used for DMA transfer, the function BSP_SRAM_DMA_IRQHandler() 00068 is called in IRQ handler file, to serve the generated interrupt once the DMA 00069 transfer is complete. 00070 00071 ------------------------------------------------------------------------------*/ 00072 00073 /* Includes ------------------------------------------------------------------*/ 00074 #include "stm32469i_eval_sram.h" 00075 00076 /** @addtogroup BSP 00077 * @{ 00078 */ 00079 00080 /** @addtogroup STM32469I_EVAL 00081 * @{ 00082 */ 00083 00084 /** @defgroup STM32469I-EVAL_SRAM STM32469I EVAL SRAM 00085 * @{ 00086 */ 00087 00088 /** @defgroup STM32469I-EVAL_SRAM_Private_Types_Definitions STM32469I EVAL SRAM Private Types Definitions 00089 * @{ 00090 */ 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM32469I-EVAL_SRAM_Private_Defines STM32469I EVAL Private Defines 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM32469I-EVAL_SRAM_Private_Macros STM32469I EVAL Private Macros 00103 * @{ 00104 */ 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM32469I-EVAL_SRAM_Private_Variables STM32469I EVAL Private Variables 00110 * @{ 00111 */ 00112 static SRAM_HandleTypeDef sramHandle; 00113 static FMC_NORSRAM_TimingTypeDef Timing; 00114 /** 00115 * @} 00116 */ 00117 00118 /** @defgroup STM32469I-EVAL_SRAM_Private_Function_Prototypes STM32469I EVAL Private Function Prototypes 00119 * @{ 00120 */ 00121 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM32469I-EVAL_SRAM_Private_Functions STM32469I EVAL Private Functions 00127 * @{ 00128 */ 00129 00130 /** 00131 * @} 00132 */ 00133 00134 /** @defgroup STM32469I-EVAL_SRAM_Exported_Functions STM32469I EVAL Exported Functions 00135 * @{ 00136 */ 00137 00138 /** 00139 * @brief Initializes the SRAM device. 00140 * @retval SRAM status 00141 */ 00142 uint8_t BSP_SRAM_Init(void) 00143 { 00144 sramHandle.Instance = FMC_NORSRAM_DEVICE; 00145 sramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00146 00147 /* SRAM device configuration */ 00148 Timing.AddressSetupTime = 2; 00149 Timing.AddressHoldTime = 1; 00150 Timing.DataSetupTime = 2; 00151 Timing.BusTurnAroundDuration = 1; 00152 Timing.CLKDivision = 2; 00153 Timing.DataLatency = 2; 00154 Timing.AccessMode = FMC_ACCESS_MODE_A; 00155 00156 sramHandle.Init.NSBank = FMC_NORSRAM_BANK2; 00157 sramHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00158 sramHandle.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00159 sramHandle.Init.MemoryDataWidth = SRAM_MEMORY_WIDTH; 00160 sramHandle.Init.BurstAccessMode = SRAM_BURSTACCESS; 00161 sramHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00162 sramHandle.Init.WrapMode = FMC_WRAP_MODE_DISABLE; 00163 sramHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00164 sramHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00165 sramHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00166 sramHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00167 sramHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00168 sramHandle.Init.WriteBurst = SRAM_WRITEBURST; 00169 sramHandle.Init.ContinuousClock = CONTINUOUSCLOCK_FEATURE; 00170 00171 /* SRAM controller initialization */ 00172 /* __weak function can be surcharged by the application code */ 00173 BSP_SRAM_MspInit(&sramHandle, (void*)NULL); 00174 if(HAL_SRAM_Init(&sramHandle, &Timing, &Timing) != HAL_OK) 00175 { 00176 return SRAM_ERROR; 00177 } 00178 else 00179 { 00180 return SRAM_OK; 00181 } 00182 } 00183 00184 /** 00185 * @brief DeInitializes the SRAM device. 00186 * @retval SRAM status : SRAM_OK or SRAM_ERROR. 00187 */ 00188 uint8_t BSP_SRAM_DeInit(void) 00189 { 00190 static uint8_t sramstatus = SRAM_ERROR; 00191 00192 /* SRAM device configuration */ 00193 sramHandle.Instance = FMC_NORSRAM_DEVICE; 00194 00195 if(HAL_SRAM_DeInit(&sramHandle) == HAL_OK) 00196 { 00197 sramstatus = SRAM_OK; 00198 00199 /* SRAM controller De-initialization */ 00200 BSP_SRAM_MspDeInit(&sramHandle, (void *)NULL); 00201 } 00202 00203 return sramstatus; 00204 } 00205 00206 /** 00207 * @brief Reads an amount of data from the SRAM device in polling mode. 00208 * @param uwStartAddress: Read start address 00209 * @param pData: Pointer to data to be read 00210 * @param uwDataSize: Size of read data from the memory 00211 * @retval SRAM status : SRAM_OK or SRAM_ERROR. 00212 */ 00213 uint8_t BSP_SRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00214 { 00215 if(HAL_SRAM_Read_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00216 { 00217 return SRAM_ERROR; 00218 } 00219 else 00220 { 00221 return SRAM_OK; 00222 } 00223 } 00224 00225 /** 00226 * @brief Reads an amount of data from the SRAM device in DMA mode. 00227 * @param uwStartAddress: Read start address 00228 * @param pData: Pointer to data to be read 00229 * @param uwDataSize: Size of read data from the memory 00230 * @retval SRAM status : SRAM_OK or SRAM_ERROR. 00231 */ 00232 uint8_t BSP_SRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00233 { 00234 if(HAL_SRAM_Read_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00235 { 00236 return SRAM_ERROR; 00237 } 00238 else 00239 { 00240 return SRAM_OK; 00241 } 00242 } 00243 00244 /** 00245 * @brief Writes an amount of data from the SRAM device in polling mode. 00246 * @param uwStartAddress: Write start address 00247 * @param pData: Pointer to data to be written 00248 * @param uwDataSize: Size of written data from the memory 00249 * @retval SRAM status : SRAM_OK or SRAM_ERROR. 00250 */ 00251 uint8_t BSP_SRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00252 { 00253 if(HAL_SRAM_Write_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00254 { 00255 return SRAM_ERROR; 00256 } 00257 else 00258 { 00259 return SRAM_OK; 00260 } 00261 } 00262 00263 /** 00264 * @brief Writes an amount of data from the SRAM device in DMA mode. 00265 * @param uwStartAddress: Write start address 00266 * @param pData: Pointer to data to be written 00267 * @param uwDataSize: Size of written data from the memory 00268 * @retval SRAM status : SRAM_OK or SRAM_ERROR. 00269 */ 00270 uint8_t BSP_SRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00271 { 00272 if(HAL_SRAM_Write_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00273 { 00274 return SRAM_ERROR; 00275 } 00276 else 00277 { 00278 return SRAM_OK; 00279 } 00280 } 00281 00282 /** 00283 * @brief Handles SRAM DMA transfer interrupt request. 00284 */ 00285 void BSP_SRAM_DMA_IRQHandler(void) 00286 { 00287 HAL_DMA_IRQHandler(sramHandle.hdma); 00288 } 00289 00290 /** 00291 * @brief Initializes SRAM MSP. 00292 * @note This function can be surcharged by application code. 00293 * @param hsram : pointer on SRAM handle 00294 * @param Params : pointer on additional configuration parameters, can be NULL. 00295 */ 00296 __weak void BSP_SRAM_MspInit(SRAM_HandleTypeDef *hsram, void *Params) 00297 { 00298 static DMA_HandleTypeDef dma_handle; 00299 GPIO_InitTypeDef gpio_init_structure; 00300 00301 if(hsram != (SRAM_HandleTypeDef *)NULL) 00302 { 00303 /* Enable FMC clock */ 00304 __HAL_RCC_FMC_CLK_ENABLE(); 00305 00306 /* Enable chosen DMAx clock */ 00307 __SRAM_DMAx_CLK_ENABLE(); 00308 00309 /* Enable GPIOs clock */ 00310 __HAL_RCC_GPIOD_CLK_ENABLE(); 00311 __HAL_RCC_GPIOE_CLK_ENABLE(); 00312 __HAL_RCC_GPIOF_CLK_ENABLE(); 00313 __HAL_RCC_GPIOG_CLK_ENABLE(); 00314 00315 /* Common GPIO configuration */ 00316 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00317 gpio_init_structure.Pull = GPIO_PULLUP; 00318 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00319 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00320 00321 /* GPIOD configuration */ 00322 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_8 |\ 00323 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 |\ 00324 GPIO_PIN_14 | GPIO_PIN_15; 00325 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00326 00327 /* GPIOE configuration */ 00328 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3| GPIO_PIN_4 | GPIO_PIN_7 |\ 00329 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00330 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00331 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00332 00333 /* GPIOF configuration */ 00334 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00335 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00336 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00337 00338 /* GPIOG configuration */ 00339 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00340 GPIO_PIN_5 | GPIO_PIN_9; 00341 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00342 00343 /* Configure common DMA parameters */ 00344 dma_handle.Init.Channel = SRAM_DMAx_CHANNEL; 00345 dma_handle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00346 dma_handle.Init.PeriphInc = DMA_PINC_ENABLE; 00347 dma_handle.Init.MemInc = DMA_MINC_ENABLE; 00348 dma_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00349 dma_handle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00350 dma_handle.Init.Mode = DMA_NORMAL; 00351 dma_handle.Init.Priority = DMA_PRIORITY_HIGH; 00352 dma_handle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00353 dma_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00354 dma_handle.Init.MemBurst = DMA_MBURST_SINGLE; 00355 dma_handle.Init.PeriphBurst = DMA_PBURST_SINGLE; 00356 00357 dma_handle.Instance = SRAM_DMAx_STREAM; 00358 00359 /* Associate the DMA handle */ 00360 __HAL_LINKDMA(hsram, hdma, dma_handle); 00361 00362 /* Deinitialize the Stream for new transfer */ 00363 HAL_DMA_DeInit(&dma_handle); 00364 00365 /* Configure the DMA Stream */ 00366 HAL_DMA_Init(&dma_handle); 00367 00368 /* NVIC configuration for DMA transfer complete interrupt */ 00369 HAL_NVIC_SetPriority(SRAM_DMAx_IRQn, 5, 0); 00370 HAL_NVIC_EnableIRQ(SRAM_DMAx_IRQn); 00371 00372 } /* of if(hsram != (SRAM_HandleTypeDef *)NULL) */ 00373 } 00374 00375 /** 00376 * @brief DeInitializes SRAM MSP. 00377 * @note This function can be surcharged by application code. 00378 * @param hsram : pointer on SRAM handle 00379 * @param Params: pointer on additional configuration parameters, can be NULL. 00380 */ 00381 __weak void BSP_SRAM_MspDeInit(SRAM_HandleTypeDef *hsram, void *Params) 00382 { 00383 static DMA_HandleTypeDef dma_handle; 00384 00385 if(hsram != (SRAM_HandleTypeDef *)NULL) 00386 { 00387 /* Disable NVIC configuration for DMA interrupt */ 00388 HAL_NVIC_DisableIRQ(SRAM_DMAx_IRQn); 00389 00390 /* Deinitialize the stream for new transfer */ 00391 dma_handle.Instance = SRAM_DMAx_STREAM; 00392 HAL_DMA_DeInit(&dma_handle); 00393 00394 /* DeInit GPIO pins can be done in the application 00395 (by surcharging this __weak function) */ 00396 00397 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the application 00398 by surcharging this __weak function */ 00399 00400 } /* of if(hsram != (SRAM_HandleTypeDef *)NULL) */ 00401 } 00402 00403 /** 00404 * @} 00405 */ 00406 00407 /** 00408 * @} 00409 */ 00410 00411 /** 00412 * @} 00413 */ 00414 00415 /** 00416 * @} 00417 */ 00418 00419 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 12 2016 17:51:25 for STM32469I_EVAL BSP User Manual by
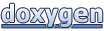