STM32469I_EVAL BSP User Manual
|
stm32469i_eval_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_eval_sd.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 12-January-2016 00007 * @brief This file includes the uSD card driver mounted on STM32469I-EVAL 00008 * evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the micro SD external card mounted on STM32469I-EVAL 00044 evaluation board. 00045 - This driver does not need a specific component driver for the micro SD device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the micro SD card using the BSP_SD_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 SDIO interface configuration to interface with the external micro SD. It 00054 also includes the micro SD initialization sequence. 00055 o To check the SD card presence you can use the function BSP_SD_IsDetected() which 00056 returns the detection status 00057 o If SD presence detection interrupt mode is desired, you must configure the 00058 SD detection interrupt mode by calling the function BSP_SD_ITConfig(). The interrupt 00059 is generated as an external interrupt whenever the micro SD card is 00060 plugged/unplugged in/from the evaluation board. The SD detection interrupt 00061 is handeled by calling the function BSP_SD_DetectIT() which is called in the IRQ 00062 handler file, the user callback is implemented in the function BSP_SD_DetectCallback(). 00063 o The function BSP_SD_GetCardInfo() is used to get the micro SD card information 00064 which is stored in the structure "HAL_SD_CardInfoTypedef". 00065 00066 + Micro SD card operations 00067 o The micro SD card can be accessed with read/write block(s) operations once 00068 it is reay for access. The access cand be performed whether using the polling 00069 mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), or by DMA 00070 transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA() 00071 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00072 is complete, the SD interrupt is handeled using the function BSP_SD_IRQHandler(), 00073 the DMA Tx/Rx transfer complete are handeled using the functions 00074 BSP_SD_DMA_Tx_IRQHandler()/BSP_SD_DMA_Rx_IRQHandler(). The corresponding user callbacks 00075 are implemented by the user at application level. 00076 o The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying 00077 the number of blocks to erase. 00078 o The SD runtime status is returned when calling the function BSP_SD_GetStatus(). 00079 00080 ------------------------------------------------------------------------------*/ 00081 00082 /* Includes ------------------------------------------------------------------*/ 00083 #include "stm32469i_eval_sd.h" 00084 00085 /** @addtogroup BSP 00086 * @{ 00087 */ 00088 00089 /** @addtogroup STM32469I_EVAL 00090 * @{ 00091 */ 00092 00093 /** @defgroup STM32469I-EVAL_SD STM32469I EVAL SD 00094 * @{ 00095 */ 00096 00097 00098 /** @defgroup STM32469I-EVAL_SD_Private_TypesDefinitions STM32469I EVAL SD Private TypesDef 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32469I-EVAL_SD_Private_Defines STM32469I EVAL SD Private Defines 00106 * @{ 00107 */ 00108 /** 00109 * @} 00110 */ 00111 00112 /** @defgroup STM32469I-EVAL_SD_Private_Macros STM32469I EVAL SD Private Macro 00113 * @{ 00114 */ 00115 /** 00116 * @} 00117 */ 00118 00119 /** @defgroup STM32469I-EVAL_SD_Private_Variables STM32469I EVAL SD Private Variables 00120 * @{ 00121 */ 00122 static SD_HandleTypeDef uSdHandle; 00123 static SD_CardInfo uSdCardInfo; 00124 static uint8_t UseExtiModeDetection = 0; 00125 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup STM32469I-EVAL_SD_Private_FunctionPrototypes STM32469I EVAL SD Private Prototypes 00131 * @{ 00132 */ 00133 /** 00134 * @} 00135 */ 00136 00137 /** @defgroup STM32469I-EVAL_SD_Private_Functions STM32469I EVAL SD Private Functions 00138 * @{ 00139 */ 00140 00141 /** 00142 * @brief Initializes the SD card device. 00143 * @retval SD status 00144 */ 00145 uint8_t BSP_SD_Init(void) 00146 { 00147 uint8_t sd_state = MSD_OK; 00148 00149 /* PLLSAI is dedicated to LCD periph. Do not use it to get 48MHz*/ 00150 00151 /* uSD device interface configuration */ 00152 uSdHandle.Instance = SDIO; 00153 00154 uSdHandle.Init.ClockEdge = SDIO_CLOCK_EDGE_RISING; 00155 uSdHandle.Init.ClockBypass = SDIO_CLOCK_BYPASS_DISABLE; 00156 uSdHandle.Init.ClockPowerSave = SDIO_CLOCK_POWER_SAVE_DISABLE; 00157 uSdHandle.Init.BusWide = SDIO_BUS_WIDE_1B; 00158 uSdHandle.Init.HardwareFlowControl = SDIO_HARDWARE_FLOW_CONTROL_ENABLE; 00159 uSdHandle.Init.ClockDiv = SDIO_TRANSFER_CLK_DIV; 00160 00161 /* Configure IO functionalities for SD detect pin */ 00162 BSP_IO_Init(); 00163 00164 /* Check if the SD card is plugged in the slot */ 00165 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_INPUT_PU); 00166 if(BSP_SD_IsDetected() != SD_PRESENT) 00167 { 00168 return MSD_ERROR_SD_NOT_PRESENT; 00169 } 00170 00171 /* Msp SD initialization */ 00172 BSP_SD_MspInit(&uSdHandle, NULL); 00173 00174 /* HAL SD initialization */ 00175 if(HAL_SD_Init(&uSdHandle, &uSdCardInfo) != SD_OK) 00176 { 00177 sd_state = MSD_ERROR; 00178 } 00179 00180 /* Configure SD Bus width */ 00181 if(sd_state == MSD_OK) 00182 { 00183 /* Enable wide operation */ 00184 if(HAL_SD_WideBusOperation_Config(&uSdHandle, SDIO_BUS_WIDE_4B) != SD_OK) 00185 { 00186 sd_state = MSD_ERROR; 00187 } 00188 else 00189 { 00190 sd_state = MSD_OK; 00191 } 00192 } 00193 return sd_state; 00194 } 00195 00196 /** 00197 * @brief DeInitializes the SD card device. 00198 * @retval SD status 00199 */ 00200 uint8_t BSP_SD_DeInit(void) 00201 { 00202 uint8_t sd_state = MSD_OK; 00203 00204 uSdHandle.Instance = SDIO; 00205 00206 /* Set back Mfx pin to INPUT mode in case it was in exti */ 00207 UseExtiModeDetection = 0; 00208 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_INPUT_PU); 00209 00210 /* HAL SD deinitialization */ 00211 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00212 { 00213 sd_state = MSD_ERROR; 00214 } 00215 00216 /* Msp SD deinitialization */ 00217 uSdHandle.Instance = SDIO; 00218 BSP_SD_MspDeInit(&uSdHandle, NULL); 00219 00220 return sd_state; 00221 } 00222 00223 /** 00224 * @brief Configures Interrupt mode for SD detection pin. 00225 * @retval Returns 0 00226 */ 00227 uint8_t BSP_SD_ITConfig(void) 00228 { 00229 /* Configure Interrupt mode for SD detection pin */ 00230 /* Note: disabling exti mode can be done calling SD_DeInit() */ 00231 UseExtiModeDetection = 1; 00232 BSP_SD_IsDetected(); 00233 00234 return 0; 00235 } 00236 00237 /** 00238 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00239 * @retval Returns if SD is detected or not 00240 */ 00241 uint8_t BSP_SD_IsDetected(void) 00242 { 00243 __IO uint8_t status = SD_PRESENT; 00244 00245 /* Check SD card detect pin */ 00246 if((BSP_IO_ReadPin(SD_DETECT_PIN)&SD_DETECT_PIN) != SD_DETECT_PIN) 00247 { 00248 if (UseExtiModeDetection) 00249 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_IT_RISING_EDGE_PU); 00250 00251 } 00252 else 00253 { 00254 status = SD_NOT_PRESENT; 00255 if (UseExtiModeDetection) 00256 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_IT_FALLING_EDGE_PU); 00257 } 00258 00259 return status; 00260 } 00261 00262 00263 00264 /** 00265 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00266 * @param pData: Pointer to the buffer that will contain the data to transmit 00267 * @param ReadAddr: Address from where data is to be read 00268 * @param BlockSize: SD card data block size, that should be 512 00269 * @param NumOfBlocks: Number of SD blocks to read 00270 * @retval SD status 00271 */ 00272 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00273 { 00274 if(HAL_SD_ReadBlocks(&uSdHandle, pData, ReadAddr, BlockSize, NumOfBlocks) != SD_OK) 00275 { 00276 return MSD_ERROR; 00277 } 00278 else 00279 { 00280 return MSD_OK; 00281 } 00282 } 00283 00284 /** 00285 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00286 * @param pData: Pointer to the buffer that will contain the data to transmit 00287 * @param WriteAddr: Address from where data is to be written 00288 * @param BlockSize: SD card data block size, that should be 512 00289 * @param NumOfBlocks: Number of SD blocks to write 00290 * @retval SD status 00291 */ 00292 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00293 { 00294 if(HAL_SD_WriteBlocks(&uSdHandle, pData, WriteAddr, BlockSize, NumOfBlocks) != SD_OK) 00295 { 00296 return MSD_ERROR; 00297 } 00298 else 00299 { 00300 return MSD_OK; 00301 } 00302 } 00303 00304 /** 00305 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00306 * @param pData: Pointer to the buffer that will contain the data to transmit 00307 * @param ReadAddr: Address from where data is to be read 00308 * @param BlockSize: SD card data block size, that should be 512 00309 * @param NumOfBlocks: Number of SD blocks to read 00310 * @retval SD status 00311 */ 00312 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00313 { 00314 uint8_t sd_state = MSD_OK; 00315 00316 /* Read block(s) in DMA transfer mode */ 00317 if(HAL_SD_ReadBlocks_DMA(&uSdHandle, pData, ReadAddr, BlockSize, NumOfBlocks) != SD_OK) 00318 { 00319 sd_state = MSD_ERROR; 00320 } 00321 00322 /* Wait until transfer is complete */ 00323 if(sd_state == MSD_OK) 00324 { 00325 if(HAL_SD_CheckReadOperation(&uSdHandle, (uint32_t)SD_DATATIMEOUT) != SD_OK) 00326 { 00327 sd_state = MSD_ERROR; 00328 } 00329 else 00330 { 00331 sd_state = MSD_OK; 00332 } 00333 } 00334 00335 return sd_state; 00336 } 00337 00338 /** 00339 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00340 * @param pData: Pointer to the buffer that will contain the data to transmit 00341 * @param WriteAddr: Address from where data is to be written 00342 * @param BlockSize: SD card data block size, that should be 512 00343 * @param NumOfBlocks: Number of SD blocks to write 00344 * @retval SD status 00345 */ 00346 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00347 { 00348 uint8_t sd_state = MSD_OK; 00349 00350 /* Write block(s) in DMA transfer mode */ 00351 if(HAL_SD_WriteBlocks_DMA(&uSdHandle, pData, WriteAddr, BlockSize, NumOfBlocks) != SD_OK) 00352 { 00353 sd_state = MSD_ERROR; 00354 } 00355 00356 /* Wait until transfer is complete */ 00357 if(sd_state == MSD_OK) 00358 { 00359 if(HAL_SD_CheckWriteOperation(&uSdHandle, (uint32_t)SD_DATATIMEOUT) != SD_OK) 00360 { 00361 sd_state = MSD_ERROR; 00362 } 00363 else 00364 { 00365 sd_state = MSD_OK; 00366 } 00367 } 00368 00369 return sd_state; 00370 } 00371 00372 /** 00373 * @brief Erases the specified memory area of the given SD card. 00374 * @param StartAddr: Start byte address 00375 * @param EndAddr: End byte address 00376 * @retval SD status 00377 */ 00378 uint8_t BSP_SD_Erase(uint64_t StartAddr, uint64_t EndAddr) 00379 { 00380 if(HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) != SD_OK) 00381 { 00382 return MSD_ERROR; 00383 } 00384 else 00385 { 00386 return MSD_OK; 00387 } 00388 } 00389 00390 /** 00391 * @brief Initializes the SD MSP. 00392 * @param hsd: SD handle 00393 * @param Params : pointer on additional configuration parameters, can be NULL. 00394 */ 00395 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00396 { 00397 static DMA_HandleTypeDef dma_rx_handle; 00398 static DMA_HandleTypeDef dma_tx_handle; 00399 GPIO_InitTypeDef gpio_init_structure; 00400 00401 /* SD pins are in conflict with Camera pins therefore Camera is power down */ 00402 /* __weak function can be modified by the application */ 00403 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00404 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00405 /* De-assert the camera STANDBY pin (active high) */ 00406 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00407 /* Assert the camera RSTI pin (active low) */ 00408 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00409 HAL_Delay(100); 00410 00411 /* Enable SDIO clock */ 00412 __HAL_RCC_SDIO_CLK_ENABLE(); 00413 00414 /* Enable DMA2 clocks */ 00415 __DMAx_TxRx_CLK_ENABLE(); 00416 00417 /* Enable GPIOs clock */ 00418 __HAL_RCC_GPIOC_CLK_ENABLE(); 00419 __HAL_RCC_GPIOD_CLK_ENABLE(); 00420 00421 /* Common GPIO configuration */ 00422 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00423 gpio_init_structure.Pull = GPIO_PULLUP; 00424 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00425 gpio_init_structure.Alternate = GPIO_AF12_SDIO; 00426 00427 /* GPIOC configuration */ 00428 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12; 00429 00430 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00431 00432 /* GPIOD configuration */ 00433 gpio_init_structure.Pin = GPIO_PIN_2; 00434 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00435 00436 /* NVIC configuration for SDIO interrupts */ 00437 HAL_NVIC_SetPriority(SDIO_IRQn, 5, 0); 00438 HAL_NVIC_EnableIRQ(SDIO_IRQn); 00439 00440 /* Configure DMA Rx parameters */ 00441 dma_rx_handle.Init.Channel = SD_DMAx_Rx_CHANNEL; 00442 dma_rx_handle.Init.Direction = DMA_PERIPH_TO_MEMORY; 00443 dma_rx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00444 dma_rx_handle.Init.MemInc = DMA_MINC_ENABLE; 00445 dma_rx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00446 dma_rx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00447 dma_rx_handle.Init.Mode = DMA_PFCTRL; 00448 dma_rx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00449 dma_rx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00450 dma_rx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00451 dma_rx_handle.Init.MemBurst = DMA_MBURST_INC4; 00452 dma_rx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00453 00454 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00455 00456 /* Associate the DMA handle */ 00457 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle); 00458 00459 /* Deinitialize the stream for new transfer */ 00460 HAL_DMA_DeInit(&dma_rx_handle); 00461 00462 /* Configure the DMA stream */ 00463 HAL_DMA_Init(&dma_rx_handle); 00464 00465 /* Configure DMA Tx parameters */ 00466 dma_tx_handle.Init.Channel = SD_DMAx_Tx_CHANNEL; 00467 dma_tx_handle.Init.Direction = DMA_MEMORY_TO_PERIPH; 00468 dma_tx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00469 dma_tx_handle.Init.MemInc = DMA_MINC_ENABLE; 00470 dma_tx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00471 dma_tx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00472 dma_tx_handle.Init.Mode = DMA_PFCTRL; 00473 dma_tx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00474 dma_tx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00475 dma_tx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00476 dma_tx_handle.Init.MemBurst = DMA_MBURST_INC4; 00477 dma_tx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00478 00479 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00480 00481 /* Associate the DMA handle */ 00482 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle); 00483 00484 /* Deinitialize the stream for new transfer */ 00485 HAL_DMA_DeInit(&dma_tx_handle); 00486 00487 /* Configure the DMA stream */ 00488 HAL_DMA_Init(&dma_tx_handle); 00489 00490 /* NVIC configuration for DMA transfer complete interrupt */ 00491 HAL_NVIC_SetPriority(SD_DMAx_Rx_IRQn, 6, 0); 00492 HAL_NVIC_EnableIRQ(SD_DMAx_Rx_IRQn); 00493 00494 /* NVIC configuration for DMA transfer complete interrupt */ 00495 HAL_NVIC_SetPriority(SD_DMAx_Tx_IRQn, 6, 0); 00496 HAL_NVIC_EnableIRQ(SD_DMAx_Tx_IRQn); 00497 } 00498 00499 /** 00500 * @brief DeInitializes the SD MSP. 00501 * @param hsd: SD handle 00502 * @param Params : pointer on additional configuration parameters, can be NULL. 00503 */ 00504 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00505 { 00506 static DMA_HandleTypeDef dma_rx_handle; 00507 static DMA_HandleTypeDef dma_tx_handle; 00508 00509 /* Disable NVIC for DMA transfer complete interrupts */ 00510 HAL_NVIC_DisableIRQ(SD_DMAx_Rx_IRQn); 00511 HAL_NVIC_DisableIRQ(SD_DMAx_Tx_IRQn); 00512 00513 /* Deinitialize the stream for new transfer */ 00514 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00515 HAL_DMA_DeInit(&dma_rx_handle); 00516 00517 /* Deinitialize the stream for new transfer */ 00518 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00519 HAL_DMA_DeInit(&dma_tx_handle); 00520 00521 /* Disable NVIC for SDIO interrupts */ 00522 HAL_NVIC_DisableIRQ(SDIO_IRQn); 00523 00524 /* DeInit GPIO pins can be done in the application 00525 (by surcharging this __weak function) */ 00526 00527 /* Disable SDIO clock */ 00528 __HAL_RCC_SDIO_CLK_DISABLE(); 00529 00530 /* GPOI pins clock and DMA cloks can be shut down in the applic 00531 by surcgarging this __weak function */ 00532 } 00533 00534 /** 00535 * @brief Handles SD card interrupt request. 00536 */ 00537 void BSP_SD_IRQHandler(void) 00538 { 00539 HAL_SD_IRQHandler(&uSdHandle); 00540 } 00541 00542 /** 00543 * @brief Handles SD DMA Tx transfer interrupt request. 00544 */ 00545 void BSP_SD_DMA_Tx_IRQHandler(void) 00546 { 00547 HAL_DMA_IRQHandler(uSdHandle.hdmatx); 00548 } 00549 00550 /** 00551 * @brief Handles SD DMA Rx transfer interrupt request. 00552 */ 00553 void BSP_SD_DMA_Rx_IRQHandler(void) 00554 { 00555 HAL_DMA_IRQHandler(uSdHandle.hdmarx); 00556 } 00557 00558 /** 00559 * @brief Gets the current SD card data status. 00560 * @retval Data transfer state. 00561 * This value can be one of the following values: 00562 * @arg SD_TRANSFER_OK: No data transfer is acting 00563 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00564 * @arg SD_TRANSFER_ERROR: Data transfer error 00565 */ 00566 HAL_SD_TransferStateTypedef BSP_SD_GetStatus(void) 00567 { 00568 return(HAL_SD_GetStatus(&uSdHandle)); 00569 } 00570 00571 /** 00572 * @brief Get SD information about specific SD card. 00573 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00574 */ 00575 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypedef *CardInfo) 00576 { 00577 /* Get SD card Information */ 00578 HAL_SD_Get_CardInfo(&uSdHandle, CardInfo); 00579 } 00580 00581 /** 00582 * @} 00583 */ 00584 00585 /** 00586 * @} 00587 */ 00588 00589 /** 00590 * @} 00591 */ 00592 00593 /** 00594 * @} 00595 */ 00596 00597 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 12 2016 17:51:25 for STM32469I_EVAL BSP User Manual by
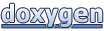