STM32469I_EVAL BSP User Manual
|
stm32469i_eval_nor.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_eval_nor.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 12-January-2016 00007 * @brief This file includes a standard driver for the M29W256GL70ZA6E NOR flash memory 00008 * device mounted on STM32469I-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the M29W128GL NOR flash external memory mounted 00044 on STM32469I-EVAL evaluation board. 00045 - This driver does not need a specific component driver for the NOR device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the NOR external memory using the BSP_NOR_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 FMC controller configuration to interface with the external NOR memory. 00054 00055 + NOR flash operations 00056 o NOR external memory can be accessed with read/write operations once it is 00057 initialized. 00058 Read/write operation can be performed with AHB access using the functions 00059 BSP_NOR_ReadData()/BSP_NOR_WriteData(). The BSP_NOR_WriteData() performs write operation 00060 of an amount of data by unit (halfword). You can also perform a program data 00061 operation of an amount of data using the function BSP_NOR_ProgramData(). 00062 o The function BSP_NOR_Read_ID() returns the chip IDs stored in the structure 00063 "NOR_IDTypeDef". (see the NOR IDs in the memory data sheet) 00064 o Perform erase block operation using the function BSP_NOR_Erase_Block() and by 00065 specifying the block address. You can perform an erase operation of the whole 00066 chip by calling the function BSP_NOR_Erase_Chip(). 00067 o After other operations, the function BSP_NOR_ReturnToReadMode() allows the NOR 00068 flash to return to read mode to perform read operations on it. 00069 00070 ------------------------------------------------------------------------------*/ 00071 00072 /* Includes ------------------------------------------------------------------*/ 00073 #include "stm32469i_eval_nor.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM32469I_EVAL 00080 * @{ 00081 */ 00082 00083 /** @defgroup STM32469I-EVAL_NOR STM32469I EVAL NOR 00084 * @{ 00085 */ 00086 00087 /* Private typedef -----------------------------------------------------------*/ 00088 00089 /** @defgroup STM32469I-EVAL_NOR_Private_Types_Definitions STM32469I EVAL NOR Private Types Definitions 00090 * @{ 00091 */ 00092 00093 /** 00094 * @} 00095 */ 00096 00097 /* Private define ------------------------------------------------------------*/ 00098 00099 /** @defgroup STM32469I-EVAL_NOR_Private_Defines STM32469I EVAL NOR Private Defines 00100 * @{ 00101 */ 00102 00103 /** 00104 * @} 00105 */ 00106 /* Private macro -------------------------------------------------------------*/ 00107 00108 /** @defgroup STM32469I-EVAL_NOR_Private_Macros STM32469I EVAL NOR Private Macros 00109 * @{ 00110 */ 00111 /** 00112 * @} 00113 */ 00114 00115 /* Private variables ---------------------------------------------------------*/ 00116 00117 /** @defgroup STM32469I-EVAL_NOR_Private_Variables STM32469I EVAL NOR Private Variables 00118 * @{ 00119 */ 00120 NOR_HandleTypeDef NorHandle; 00121 static FMC_NORSRAM_TimingTypeDef Timing; 00122 00123 /** 00124 * @} 00125 */ 00126 00127 /* Private function prototypes -----------------------------------------------*/ 00128 00129 /** @defgroup STM32469I-EVAL_NOR_Private_Function_Prototypes STM32469I EVAL NOR Private Function Prototypes 00130 * @{ 00131 */ 00132 00133 00134 00135 /** 00136 * @} 00137 */ 00138 00139 /** 00140 * @brief Initializes the NOR device. 00141 * @retval NOR memory status 00142 */ 00143 uint8_t BSP_NOR_Init(void) 00144 { 00145 NorHandle.Instance = FMC_NORSRAM_DEVICE; 00146 NorHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00147 00148 /* NOR device configuration */ 00149 Timing.AddressSetupTime = 8; 00150 Timing.AddressHoldTime = 3; 00151 Timing.DataSetupTime = 9; 00152 Timing.BusTurnAroundDuration = 0; 00153 Timing.CLKDivision = 2; 00154 Timing.DataLatency = 2; 00155 Timing.AccessMode = FMC_ACCESS_MODE_A; 00156 00157 NorHandle.Init.NSBank = FMC_NORSRAM_BANK1; 00158 NorHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00159 NorHandle.Init.MemoryType = FMC_MEMORY_TYPE_NOR; 00160 NorHandle.Init.MemoryDataWidth = NOR_MEMORY_WIDTH; 00161 NorHandle.Init.BurstAccessMode = NOR_BURSTACCESS; 00162 NorHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00163 NorHandle.Init.WrapMode = FMC_WRAP_MODE_DISABLE; 00164 NorHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00165 NorHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00166 NorHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_ENABLE; 00167 NorHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00168 NorHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_ENABLE; 00169 NorHandle.Init.WriteBurst = NOR_WRITEBURST; 00170 NorHandle.Init.ContinuousClock = CONTINUOUSCLOCK_FEATURE; 00171 00172 /* NOR controller initialization */ 00173 BSP_NOR_MspInit(&NorHandle, NULL); 00174 00175 if(HAL_NOR_Init(&NorHandle, &Timing, &Timing) != HAL_OK) 00176 { 00177 return NOR_STATUS_ERROR; 00178 } 00179 else 00180 { 00181 return NOR_STATUS_OK; 00182 } 00183 } 00184 00185 /** 00186 * @brief DeInitializes the NOR device. 00187 * @retval NOR memory status 00188 */ 00189 uint8_t BSP_NOR_DeInit(void) 00190 { 00191 /* NOR controller initialization */ 00192 BSP_NOR_MspDeInit(&NorHandle, NULL); 00193 00194 if(HAL_NOR_DeInit(&NorHandle) != HAL_OK) 00195 { 00196 return NOR_STATUS_ERROR; 00197 } 00198 else 00199 { 00200 return NOR_STATUS_OK; 00201 } 00202 } 00203 00204 /** 00205 * @brief Reads an amount of data from the NOR device. 00206 * @param uwStartAddress: Read start address 00207 * @param pData: Pointer to data to be read 00208 * @param uwDataSize: Size of data to read 00209 * @retval NOR memory status 00210 */ 00211 uint8_t BSP_NOR_ReadData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00212 { 00213 if(HAL_NOR_ReadBuffer(&NorHandle, NOR_DEVICE_ADDR + uwStartAddress, pData, uwDataSize) != HAL_OK) 00214 { 00215 return NOR_STATUS_ERROR; 00216 } 00217 else 00218 { 00219 return NOR_STATUS_OK; 00220 } 00221 } 00222 00223 /** 00224 * @brief Returns the NOR memory to read mode. 00225 */ 00226 void BSP_NOR_ReturnToReadMode(void) 00227 { 00228 HAL_NOR_ReturnToReadMode(&NorHandle); 00229 } 00230 00231 /** 00232 * @brief Writes an amount of data to the NOR device. 00233 * @param uwStartAddress: Write start address 00234 * @param pData: Pointer to data to be written 00235 * @param uwDataSize: Size of data to write 00236 * @retval NOR memory status 00237 */ 00238 uint8_t BSP_NOR_WriteData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00239 { 00240 uint32_t index = uwDataSize; 00241 00242 while(index > 0) 00243 { 00244 /* Write data to NOR */ 00245 HAL_NOR_Program(&NorHandle, (uint32_t *)(NOR_DEVICE_ADDR + uwStartAddress), pData); 00246 00247 /* Read NOR device status */ 00248 if(HAL_NOR_GetStatus(&NorHandle, NOR_DEVICE_ADDR, PROGRAM_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00249 { 00250 return NOR_STATUS_ERROR; 00251 } 00252 00253 /* Update the counters */ 00254 index--; 00255 uwStartAddress += 2; 00256 pData++; 00257 } 00258 00259 return NOR_STATUS_OK; 00260 } 00261 00262 /** 00263 * @brief Programs an amount of data to the NOR device. 00264 * @param uwStartAddress: Write start address 00265 * @param pData: Pointer to data to be written 00266 * @param uwDataSize: Size of data to write 00267 * @retval NOR memory status 00268 */ 00269 uint8_t BSP_NOR_ProgramData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00270 { 00271 /* Send NOR program buffer operation */ 00272 HAL_NOR_ProgramBuffer(&NorHandle, uwStartAddress, pData, uwDataSize); 00273 00274 /* Return the NOR memory status */ 00275 if(HAL_NOR_GetStatus(&NorHandle, NOR_DEVICE_ADDR, PROGRAM_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00276 { 00277 return NOR_STATUS_ERROR; 00278 } 00279 else 00280 { 00281 return NOR_STATUS_OK; 00282 } 00283 } 00284 00285 /** 00286 * @brief Erases the specified block of the NOR device. 00287 * @param BlockAddress: Block address to erase 00288 * @retval NOR memory status 00289 */ 00290 uint8_t BSP_NOR_Erase_Block(uint32_t BlockAddress) 00291 { 00292 /* Send NOR erase block operation */ 00293 HAL_NOR_Erase_Block(&NorHandle, BlockAddress, NOR_DEVICE_ADDR); 00294 00295 /* Return the NOR memory status */ 00296 if(HAL_NOR_GetStatus(&NorHandle, NOR_DEVICE_ADDR, BLOCKERASE_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00297 { 00298 return NOR_STATUS_ERROR; 00299 } 00300 else 00301 { 00302 return NOR_STATUS_OK; 00303 } 00304 } 00305 00306 /** 00307 * @brief Erases the entire NOR chip. 00308 * @retval NOR memory status 00309 */ 00310 uint8_t BSP_NOR_Erase_Chip(void) 00311 { 00312 /* Send NOR Erase chip operation */ 00313 HAL_NOR_Erase_Chip(&NorHandle, NOR_DEVICE_ADDR); 00314 00315 /* Return the NOR memory status */ 00316 if(HAL_NOR_GetStatus(&NorHandle, NOR_DEVICE_ADDR, CHIPERASE_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00317 { 00318 return NOR_STATUS_ERROR; 00319 } 00320 else 00321 { 00322 return NOR_STATUS_OK; 00323 } 00324 } 00325 00326 /** 00327 * @brief Reads NOR flash IDs. 00328 * @param pNOR_ID : Pointer to NOR ID structure 00329 * @retval NOR memory status 00330 */ 00331 uint8_t BSP_NOR_Read_ID(NOR_IDTypeDef *pNOR_ID) 00332 { 00333 if(HAL_NOR_Read_ID(&NorHandle, pNOR_ID) != HAL_OK) 00334 { 00335 return NOR_STATUS_ERROR; 00336 } 00337 else 00338 { 00339 return NOR_STATUS_OK; 00340 } 00341 } 00342 00343 /** 00344 * @brief Initializes the NOR MSP. 00345 * @param hnor: pointer to nor structure 00346 * @param Params : pointer on additional configuration parameters, can be NULL. 00347 */ 00348 __weak void BSP_NOR_MspInit(NOR_HandleTypeDef *hnor, void *Params) 00349 { 00350 GPIO_InitTypeDef gpio_init_structure; 00351 00352 /* Enable FMC clock */ 00353 __HAL_RCC_FMC_CLK_ENABLE(); 00354 00355 /* Enable GPIOs clock */ 00356 __HAL_RCC_GPIOD_CLK_ENABLE(); 00357 __HAL_RCC_GPIOE_CLK_ENABLE(); 00358 __HAL_RCC_GPIOF_CLK_ENABLE(); 00359 __HAL_RCC_GPIOG_CLK_ENABLE(); 00360 00361 /* Common GPIO configuration */ 00362 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00363 gpio_init_structure.Pull = GPIO_PULLUP; 00364 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00365 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00366 00367 /* GPIOD configuration */ 00368 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 |\ 00369 GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00370 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00371 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00372 00373 /* GPIOE configuration */ 00374 gpio_init_structure.Pin = GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 |\ 00375 GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00376 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00377 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00378 00379 /* GPIOF configuration */ 00380 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00381 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00382 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00383 00384 /* GPIOG configuration */ 00385 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00386 GPIO_PIN_5; 00387 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00388 } 00389 00390 /** 00391 * @brief DeInitializes the NOR MSP. 00392 * @param hnor pointer to NOR structure 00393 * @param Params : pointer on additional configuration parameters, can be NULL. 00394 * @retval status 00395 */ 00396 __weak uint8_t BSP_NOR_MspDeInit(NOR_HandleTypeDef *hnor, void *Params) 00397 { 00398 /* FMC won't be disabled because common to other functionalities */ 00399 00400 /* Disable only NOR specific GPIOs */ 00401 HAL_GPIO_DeInit(GPIOD, (GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7)); 00402 00403 return HAL_OK; 00404 00405 } 00406 00407 /** 00408 * @brief NOR BSP Wait for Ready/Busy signal. 00409 * @param hnor: Pointer to NOR handle 00410 * @param Timeout: Timeout duration 00411 */ 00412 void HAL_NOR_MspWait(NOR_HandleTypeDef *hnor, uint32_t Timeout) 00413 { 00414 uint32_t timeout = Timeout; 00415 00416 /* Polling on Ready/Busy signal */ 00417 while((HAL_GPIO_ReadPin(NOR_READY_BUSY_GPIO, NOR_READY_BUSY_PIN) != NOR_BUSY_STATE) && (timeout > 0)) 00418 { 00419 timeout--; 00420 } 00421 00422 timeout = Timeout; 00423 00424 /* Polling on Ready/Busy signal */ 00425 while((HAL_GPIO_ReadPin(NOR_READY_BUSY_GPIO, NOR_READY_BUSY_PIN) != NOR_READY_STATE) && (timeout > 0)) 00426 { 00427 timeout--; 00428 } 00429 } 00430 00431 /** 00432 * @} 00433 */ 00434 00435 /** 00436 * @} 00437 */ 00438 00439 /** 00440 * @} 00441 */ 00442 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 12 2016 17:51:25 for STM32469I_EVAL BSP User Manual by
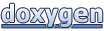