STM32469I_EVAL BSP User Manual
|
stm32469i_eval_camera.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_eval_camera.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 12-January-2016 00007 * @brief This file includes the driver for Camera modules mounted on 00008 * STM32469I-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info: ------------------------------------------------------------------ 00040 User NOTES 00041 1. How to use this driver: 00042 -------------------------- 00043 - This driver is used to drive the camera. 00044 - The S5K5CAG component driver MUST be included with this driver. 00045 00046 2. Driver description: 00047 --------------------- 00048 + Initialization steps: 00049 o Initialize the camera using the BSP_CAMERA_Init() function. 00050 o Start the camera capture/snapshot using the CAMERA_Start() function. 00051 o Suspend, resume or stop the camera capture using the following functions: 00052 - BSP_CAMERA_Suspend() 00053 - BSP_CAMERA_Resume() 00054 - BSP_CAMERA_Stop() 00055 00056 + Options 00057 o Increase or decrease on the fly the brightness and/or contrast 00058 using the following function: 00059 - BSP_CAMERA_ContrastBrightnessConfig 00060 o Add a special effect on the fly using the following functions: 00061 - BSP_CAMERA_BlackWhiteConfig() 00062 - BSP_CAMERA_ColorEffectConfig() 00063 00064 ------------------------------------------------------------------------------*/ 00065 00066 /* Includes ------------------------------------------------------------------*/ 00067 #include "stm32469i_eval_camera.h" 00068 00069 /** @addtogroup BSP 00070 * @{ 00071 */ 00072 00073 /** @addtogroup STM32469I_EVAL 00074 * @{ 00075 */ 00076 00077 /** @defgroup STM32469I-EVAL_CAMERA STM32469I EVAL CAMERA 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM32469I-EVAL_CAMERA_Private_TypesDefinitions STM32469I EVAL CAMERA Private TypesDefinitions 00082 * @{ 00083 */ 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM32469I-EVAL_CAMERA_Private_Defines STM32469I EVAL CAMERA Private Defines 00089 * @{ 00090 */ 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM32469I-EVAL_CAMERA_Private_Macros STM32469I EVAL CAMERA Private Macros 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM32469I-EVAL_CAMERA_Imported_Variables STM32469I EVAL CAMERA Imported Variables 00103 * @{ 00104 */ 00105 /** 00106 * @brief DMA2D handle variable 00107 */ 00108 extern DMA2D_HandleTypeDef hdma2d_eval; 00109 /** 00110 * @} 00111 */ 00112 00113 /** @defgroup STM32469I-EVAL_CAMERA_Private_Variables STM32469I EVAL CAMERA Private Variables 00114 * @{ 00115 */ 00116 static DCMI_HandleTypeDef hDcmiEval; 00117 CAMERA_DrvTypeDef *CameraDrv; 00118 00119 /* Camera current resolution naming (QQVGA, VGA, ...) */ 00120 uint32_t CameraCurrentResolution; 00121 00122 /* Camera image rotation on LCD Displayed frame buffer */ 00123 uint32_t CameraRotation = CAMERA_ROTATION_INVALID; 00124 00125 static uint32_t CameraHwAddress; 00126 00127 /** 00128 * @} 00129 */ 00130 00131 /** @defgroup STM32469I-EVAL_CAMERA_Private_FunctionPrototypes STM32469I EVAL CAMERA Private FunctionPrototypes 00132 * @{ 00133 */ 00134 static uint32_t GetSize(uint32_t Resolution); 00135 /** 00136 * @} 00137 */ 00138 00139 /** @defgroup STM32469I-EVAL_CAMERA_Public_Functions STM32469I EVAL CAMERA Public Functions 00140 * @{ 00141 */ 00142 00143 /** 00144 * @brief Set Camera image rotation on LCD Displayed frame buffer. 00145 * @param rotation : uint32_t rotation of camera image in preview buffer sent to LCD 00146 * need to be of type Camera_ImageRotationTypeDef 00147 * @retval Camera status 00148 */ 00149 uint8_t BSP_CAMERA_SetRotation(uint32_t rotation) 00150 { 00151 uint8_t status = CAMERA_ERROR; 00152 00153 if(rotation < CAMERA_ROTATION_INVALID) 00154 { 00155 /* Set Camera image rotation on LCD Displayed frame buffer */ 00156 CameraRotation = rotation; 00157 status = CAMERA_OK; 00158 } 00159 00160 return status; 00161 } 00162 00163 /** 00164 * @brief Get Camera image rotation on LCD Displayed frame buffer. 00165 * @retval rotation : uint32_t value of type Camera_ImageRotationTypeDef 00166 */ 00167 uint32_t BSP_CAMERA_GetRotation(void) 00168 { 00169 return(CameraRotation); 00170 } 00171 00172 /** 00173 * @brief Initializes the camera. 00174 * @param Resolution : camera sensor requested resolution (x, y) : standard resolution 00175 * naming QQVGA, QVGA, VGA ... 00176 * @retval Camera status 00177 */ 00178 uint8_t BSP_CAMERA_Init(uint32_t Resolution) 00179 { 00180 DCMI_HandleTypeDef *phdcmi; 00181 uint8_t status = CAMERA_ERROR; 00182 00183 /* Get the DCMI handle structure */ 00184 phdcmi = &hDcmiEval; 00185 00186 /*** Configures the DCMI to interface with the camera module ***/ 00187 /* DCMI configuration */ 00188 phdcmi->Init.CaptureRate = DCMI_CR_ALL_FRAME; 00189 phdcmi->Init.HSPolarity = DCMI_HSPOLARITY_HIGH; 00190 phdcmi->Init.SynchroMode = DCMI_SYNCHRO_HARDWARE; 00191 phdcmi->Init.VSPolarity = DCMI_VSPOLARITY_HIGH; 00192 phdcmi->Init.ExtendedDataMode = DCMI_EXTEND_DATA_8B; 00193 phdcmi->Init.PCKPolarity = DCMI_PCKPOLARITY_RISING; 00194 00195 phdcmi->Instance = DCMI; 00196 00197 /* Configure IO functionalities for CAMERA detect pin */ 00198 BSP_IO_Init(); 00199 /* Apply Camera Module hardware reset */ 00200 BSP_CAMERA_HwReset(); 00201 00202 /* Check if the CAMERA Module is plugged on board */ 00203 if(BSP_IO_ReadPin(CAM_PLUG_PIN) == BSP_IO_PIN_SET) 00204 { 00205 status = CAMERA_NOT_DETECTED; 00206 return status; /* Exit with error */ 00207 } 00208 00209 /* Read ID of Camera module via I2C */ 00210 if (s5k5cag_ReadID(CAMERA_I2C_ADDRESS) == S5K5CAG_ID) 00211 { 00212 /* Initialize the camera driver structure */ 00213 CameraDrv = &s5k5cag_drv; 00214 CameraHwAddress = CAMERA_I2C_ADDRESS; 00215 00216 /* DCMI Initialization */ 00217 BSP_CAMERA_MspInit(&hDcmiEval, NULL); 00218 HAL_DCMI_Init(phdcmi); 00219 00220 /* Camera Module Initialization via I2C to the wanted 'Resolution' */ 00221 CameraDrv->Init(CameraHwAddress, Resolution); 00222 00223 CameraCurrentResolution = Resolution; 00224 00225 /* Return CAMERA_OK status */ 00226 status = CAMERA_OK; 00227 } 00228 else 00229 { 00230 /* Return CAMERA_NOT_SUPPORTED status */ 00231 status = CAMERA_NOT_SUPPORTED; 00232 } 00233 00234 return status; 00235 } 00236 00237 00238 /** 00239 * @brief DeInitializes the camera. 00240 * @retval Camera status 00241 */ 00242 uint8_t BSP_CAMERA_DeInit(void) 00243 { 00244 hDcmiEval.Instance = DCMI; 00245 00246 HAL_DCMI_DeInit(&hDcmiEval); 00247 BSP_CAMERA_MspDeInit(&hDcmiEval, NULL); 00248 return CAMERA_OK; 00249 } 00250 00251 /** 00252 * @brief Starts the camera capture in continuous mode. 00253 * @param buff: pointer to the camera output buffer 00254 */ 00255 void BSP_CAMERA_ContinuousStart(uint8_t *buff) 00256 { 00257 /* Start the camera capture */ 00258 HAL_DCMI_Start_DMA(&hDcmiEval, DCMI_MODE_CONTINUOUS, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00259 } 00260 00261 /** 00262 * @brief Starts the camera capture in snapshot mode. 00263 * @param buff: pointer to the camera output buffer 00264 */ 00265 void BSP_CAMERA_SnapshotStart(uint8_t *buff) 00266 { 00267 /* Start the camera capture */ 00268 HAL_DCMI_Start_DMA(&hDcmiEval, DCMI_MODE_SNAPSHOT, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00269 } 00270 00271 /** 00272 * @brief Suspend the CAMERA capture 00273 */ 00274 void BSP_CAMERA_Suspend(void) 00275 { 00276 /* Disable the DMA */ 00277 __HAL_DMA_DISABLE(hDcmiEval.DMA_Handle); 00278 /* Disable the DCMI */ 00279 __HAL_DCMI_DISABLE(&hDcmiEval); 00280 00281 } 00282 00283 /** 00284 * @brief Resume the CAMERA capture 00285 */ 00286 void BSP_CAMERA_Resume(void) 00287 { 00288 /* Enable the DCMI */ 00289 __HAL_DCMI_ENABLE(&hDcmiEval); 00290 /* Enable the DMA */ 00291 __HAL_DMA_ENABLE(hDcmiEval.DMA_Handle); 00292 } 00293 00294 /** 00295 * @brief Stop the CAMERA capture 00296 * @retval Camera status 00297 */ 00298 uint8_t BSP_CAMERA_Stop(void) 00299 { 00300 uint8_t status = CAMERA_ERROR; 00301 00302 if(HAL_DCMI_Stop(&hDcmiEval) == HAL_OK) 00303 { 00304 status = CAMERA_OK; 00305 } 00306 00307 /* Set Camera in Power Down */ 00308 BSP_CAMERA_PwrDown(); 00309 00310 return status; 00311 } 00312 00313 /** 00314 * @brief CANERA hardware reset 00315 */ 00316 void BSP_CAMERA_HwReset(void) 00317 { 00318 /* Camera sensor RESET sequence */ 00319 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00320 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00321 00322 /* Assert the camera STANDBY pin (active high) */ 00323 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_SET); 00324 00325 /* Assert the camera RSTI pin (active low) */ 00326 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00327 00328 HAL_Delay(100); /* RST and XSDN signals asserted during 100ms */ 00329 00330 /* De-assert the camera STANDBY pin (active high) */ 00331 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00332 00333 HAL_Delay(3); /* RST de-asserted and XSDN asserted during 3ms */ 00334 00335 /* De-assert the camera RSTI pin (active low) */ 00336 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_SET); 00337 00338 HAL_Delay(6); /* RST de-asserted during 3ms */ 00339 } 00340 00341 /** 00342 * @brief CAMERA power down 00343 */ 00344 void BSP_CAMERA_PwrDown(void) 00345 { 00346 /* Camera power down sequence */ 00347 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00348 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00349 00350 /* De-assert the camera STANDBY pin (active high) */ 00351 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00352 00353 /* Assert the camera RSTI pin (active low) */ 00354 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00355 } 00356 00357 /** 00358 * @brief Configures the camera contrast and brightness. 00359 * @param contrast_level: Contrast level 00360 * This parameter can be one of the following values: 00361 * @arg CAMERA_CONTRAST_LEVEL4: for contrast +2 00362 * @arg CAMERA_CONTRAST_LEVEL3: for contrast +1 00363 * @arg CAMERA_CONTRAST_LEVEL2: for contrast 0 00364 * @arg CAMERA_CONTRAST_LEVEL1: for contrast -1 00365 * @arg CAMERA_CONTRAST_LEVEL0: for contrast -2 00366 * @param brightness_level: Contrast level 00367 * This parameter can be one of the following values: 00368 * @arg CAMERA_BRIGHTNESS_LEVEL4: for brightness +2 00369 * @arg CAMERA_BRIGHTNESS_LEVEL3: for brightness +1 00370 * @arg CAMERA_BRIGHTNESS_LEVEL2: for brightness 0 00371 * @arg CAMERA_BRIGHTNESS_LEVEL1: for brightness -1 00372 * @arg CAMERA_BRIGHTNESS_LEVEL0: for brightness -2 00373 */ 00374 void BSP_CAMERA_ContrastBrightnessConfig(uint32_t contrast_level, uint32_t brightness_level) 00375 { 00376 if(CameraDrv->Config != NULL) 00377 { 00378 CameraDrv->Config(CameraHwAddress, CAMERA_CONTRAST_BRIGHTNESS, contrast_level, brightness_level); 00379 } 00380 } 00381 00382 /** 00383 * @brief Configures the camera white balance. 00384 * @param Mode: black_white mode 00385 * This parameter can be one of the following values: 00386 * @arg CAMERA_BLACK_WHITE_BW 00387 * @arg CAMERA_BLACK_WHITE_NEGATIVE 00388 * @arg CAMERA_BLACK_WHITE_BW_NEGATIVE 00389 * @arg CAMERA_BLACK_WHITE_NORMAL 00390 */ 00391 void BSP_CAMERA_BlackWhiteConfig(uint32_t Mode) 00392 { 00393 if(CameraDrv->Config != NULL) 00394 { 00395 CameraDrv->Config(CameraHwAddress, CAMERA_BLACK_WHITE, Mode, 0); 00396 } 00397 } 00398 00399 /** 00400 * @brief Configures the camera color effect. 00401 * @param Effect: Color effect 00402 * This parameter can be one of the following values: 00403 * @arg CAMERA_COLOR_EFFECT_NONE 00404 * @arg CAMERA_COLOR_EFFECT_BLUE 00405 * @arg CAMERA_COLOR_EFFECT_GREEN 00406 * @arg CAMERA_COLOR_EFFECT_RED 00407 * @arg CAMERA_COLOR_EFFECT_ANTIQUE 00408 */ 00409 void BSP_CAMERA_ColorEffectConfig(uint32_t Effect) 00410 { 00411 if(CameraDrv->Config != NULL) 00412 { 00413 CameraDrv->Config(CameraHwAddress, CAMERA_COLOR_EFFECT, Effect, 0); 00414 } 00415 } 00416 00417 /** 00418 * @brief Handles DCMI interrupt request. 00419 */ 00420 void BSP_CAMERA_IRQHandler(void) 00421 { 00422 HAL_DCMI_IRQHandler(&hDcmiEval); 00423 } 00424 00425 /** 00426 * @brief Handles DMA interrupt request. 00427 */ 00428 void BSP_CAMERA_DMA_IRQHandler(void) 00429 { 00430 HAL_DMA_IRQHandler(hDcmiEval.DMA_Handle); 00431 } 00432 00433 /** 00434 * @brief Get the capture size in pixels unit. 00435 * @param Resolution: the current resolution. 00436 * @retval capture size in pixels unit. 00437 */ 00438 static uint32_t GetSize(uint32_t Resolution) 00439 { 00440 uint32_t size = 0; 00441 00442 /* Get capture size */ 00443 switch (Resolution) 00444 { 00445 case CAMERA_R160x120: 00446 { 00447 size = 0x2580; 00448 } 00449 break; 00450 case CAMERA_R320x240: 00451 { 00452 size = 0x9600; 00453 } 00454 break; 00455 case CAMERA_R480x272: 00456 { 00457 size = 0xFF00; 00458 } 00459 break; 00460 case CAMERA_R640x480: 00461 { 00462 size = 0x25800; 00463 } 00464 break; 00465 default: 00466 { 00467 break; 00468 } 00469 } 00470 00471 return size; 00472 } 00473 00474 /** 00475 * @brief Initializes the DCMI MSP. 00476 * @param hdcmi: HDMI handle 00477 * @param Params : pointer on additional configuration parameters, can be NULL. 00478 */ 00479 __weak void BSP_CAMERA_MspInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00480 { 00481 static DMA_HandleTypeDef hdma_eval; 00482 GPIO_InitTypeDef gpio_init_structure; 00483 00484 /*** Enable peripherals and GPIO clocks ***/ 00485 /* Enable DCMI clock */ 00486 __HAL_RCC_DCMI_CLK_ENABLE(); 00487 00488 /* Enable DMA2 clock */ 00489 __HAL_RCC_DMA2_CLK_ENABLE(); 00490 00491 /* Enable GPIO clocks */ 00492 __HAL_RCC_GPIOA_CLK_ENABLE(); 00493 __HAL_RCC_GPIOB_CLK_ENABLE(); 00494 __HAL_RCC_GPIOC_CLK_ENABLE(); 00495 __HAL_RCC_GPIOD_CLK_ENABLE(); 00496 __HAL_RCC_GPIOE_CLK_ENABLE(); 00497 00498 /*** Configure the GPIO ***/ 00499 /* Configure DCMI GPIO as alternate function */ 00500 gpio_init_structure.Pin = GPIO_PIN_4 | GPIO_PIN_6; 00501 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00502 gpio_init_structure.Pull = GPIO_PULLUP; 00503 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00504 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00505 HAL_GPIO_Init(GPIOA, &gpio_init_structure); 00506 00507 gpio_init_structure.Pin = GPIO_PIN_7; 00508 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00509 gpio_init_structure.Pull = GPIO_PULLUP; 00510 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00511 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00512 HAL_GPIO_Init(GPIOB, &gpio_init_structure); 00513 00514 gpio_init_structure.Pin = GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_8 |\ 00515 GPIO_PIN_9 | GPIO_PIN_11; 00516 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00517 gpio_init_structure.Pull = GPIO_PULLUP; 00518 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00519 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00520 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00521 00522 gpio_init_structure.Pin = GPIO_PIN_3 | GPIO_PIN_6; 00523 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00524 gpio_init_structure.Pull = GPIO_PULLUP; 00525 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00526 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00527 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00528 00529 gpio_init_structure.Pin = GPIO_PIN_5 | GPIO_PIN_6; 00530 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00531 gpio_init_structure.Pull = GPIO_PULLUP; 00532 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00533 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00534 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00535 00536 /*** Configure the DMA ***/ 00537 /* Set the parameters to be configured */ 00538 hdma_eval.Init.Channel = DMA_CHANNEL_1; 00539 hdma_eval.Init.Direction = DMA_PERIPH_TO_MEMORY; 00540 hdma_eval.Init.PeriphInc = DMA_PINC_DISABLE; 00541 hdma_eval.Init.MemInc = DMA_MINC_ENABLE; 00542 hdma_eval.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00543 hdma_eval.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00544 hdma_eval.Init.Mode = DMA_CIRCULAR; 00545 hdma_eval.Init.Priority = DMA_PRIORITY_HIGH; 00546 hdma_eval.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00547 hdma_eval.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00548 hdma_eval.Init.MemBurst = DMA_MBURST_SINGLE; 00549 hdma_eval.Init.PeriphBurst = DMA_PBURST_SINGLE; 00550 00551 hdma_eval.Instance = DMA2_Stream1; 00552 00553 /* Associate the initialized DMA handle to the DCMI handle */ 00554 __HAL_LINKDMA(hdcmi, DMA_Handle, hdma_eval); 00555 00556 /*** Configure the NVIC for DCMI and DMA ***/ 00557 /* NVIC configuration for DCMI transfer complete interrupt */ 00558 HAL_NVIC_SetPriority(DCMI_IRQn, 5, 0); 00559 HAL_NVIC_EnableIRQ(DCMI_IRQn); 00560 00561 /* NVIC configuration for DMA2D transfer complete interrupt */ 00562 HAL_NVIC_SetPriority(DMA2_Stream1_IRQn, 5, 0); 00563 HAL_NVIC_EnableIRQ(DMA2_Stream1_IRQn); 00564 00565 /* Configure the DMA stream */ 00566 HAL_DMA_Init(hdcmi->DMA_Handle); 00567 } 00568 00569 /** 00570 * @brief DeInitializes the DCMI MSP. 00571 * @param hdcmi: HDMI handle 00572 * @param Params : pointer on additional configuration parameters, can be NULL. 00573 */ 00574 __weak void BSP_CAMERA_MspDeInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00575 { 00576 /* Disable NVIC for DCMI transfer complete interrupt */ 00577 HAL_NVIC_DisableIRQ(DCMI_IRQn); 00578 00579 /* Disable NVIC for DMA2 transfer complete interrupt */ 00580 HAL_NVIC_DisableIRQ(DMA2_Stream1_IRQn); 00581 00582 /* Configure the DMA stream */ 00583 HAL_DMA_DeInit(hdcmi->DMA_Handle); 00584 00585 /* Disable DCMI clock */ 00586 __HAL_RCC_DCMI_CLK_DISABLE(); 00587 00588 /* GPIO pins clock and DMA clock can be shut down in the application 00589 by surcharging this __weak function */ 00590 } 00591 00592 /** 00593 * @brief Line event callback 00594 * @param hdcmi: pointer to the DCMI handle 00595 */ 00596 void HAL_DCMI_LineEventCallback(DCMI_HandleTypeDef *hdcmi) 00597 { 00598 BSP_CAMERA_LineEventCallback(); 00599 } 00600 00601 /** 00602 * @brief Line Event callback. 00603 */ 00604 __weak void BSP_CAMERA_LineEventCallback(void) 00605 { 00606 /* NOTE : This function Should not be modified, when the callback is needed, 00607 the HAL_DCMI_LineEventCallback could be implemented in the user file 00608 */ 00609 } 00610 00611 /** 00612 * @brief VSYNC event callback 00613 * @param hdcmi: pointer to the DCMI handle 00614 */ 00615 void HAL_DCMI_VsyncEventCallback(DCMI_HandleTypeDef *hdcmi) 00616 { 00617 BSP_CAMERA_VsyncEventCallback(); 00618 } 00619 00620 /** 00621 * @brief VSYNC Event callback. 00622 */ 00623 __weak void BSP_CAMERA_VsyncEventCallback(void) 00624 { 00625 /* NOTE : This function Should not be modified, when the callback is needed, 00626 the HAL_DCMI_VsyncEventCallback could be implemented in the user file 00627 */ 00628 } 00629 00630 /** 00631 * @brief Frame event callback 00632 * @param hdcmi: pointer to the DCMI handle 00633 */ 00634 void HAL_DCMI_FrameEventCallback(DCMI_HandleTypeDef *hdcmi) 00635 { 00636 BSP_CAMERA_FrameEventCallback(); 00637 } 00638 00639 /** 00640 * @brief Frame Event callback. 00641 */ 00642 __weak void BSP_CAMERA_FrameEventCallback(void) 00643 { 00644 /* NOTE : This function Should not be modified, when the callback is needed, 00645 the HAL_DCMI_FrameEventCallback could be implemented in the user file 00646 */ 00647 } 00648 00649 /** 00650 * @brief Error callback 00651 * @param hdcmi: pointer to the DCMI handle 00652 */ 00653 void HAL_DCMI_ErrorCallback(DCMI_HandleTypeDef *hdcmi) 00654 { 00655 BSP_CAMERA_ErrorCallback(); 00656 } 00657 00658 /** 00659 * @brief Error callback. 00660 */ 00661 __weak void BSP_CAMERA_ErrorCallback(void) 00662 { 00663 /* NOTE : This function Should not be modified, when the callback is needed, 00664 the HAL_DCMI_ErrorCallback could be implemented in the user file 00665 */ 00666 } 00667 00668 /** 00669 * @} 00670 */ 00671 00672 /** 00673 * @} 00674 */ 00675 00676 /** 00677 * @} 00678 */ 00679 00680 /** 00681 * @} 00682 */ 00683 00684 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 12 2016 17:51:25 for STM32469I_EVAL BSP User Manual by
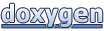