STM32412G-Discovery BSP User Manual
|
stm32412g_discovery_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32412g_discovery_lcd.h 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 27-January-2017 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32412g_discovery_lcd.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32412G_DISCOVERY_LCD_H 00041 #define __STM32412G_DISCOVERY_LCD_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32412g_discovery.h" 00049 #include "../Components/ls016b8uy/ls016b8uy.h" 00050 #include "../Components/st7789h2/st7789h2.h" 00051 #include "../../../Utilities/Fonts/fonts.h" 00052 00053 /** @addtogroup BSP 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM32412G_DISCOVERY 00058 * @{ 00059 */ 00060 00061 /** @addtogroup STM32412G_DISCOVERY_LCD 00062 * @{ 00063 */ 00064 00065 /** @defgroup STM32412G_DISCOVERY_LCD_Exported_Types STM32412G DISCOVERY LCD Exported Types 00066 * @{ 00067 */ 00068 typedef struct 00069 { 00070 uint32_t TextColor; 00071 uint32_t BackColor; 00072 sFONT *pFont; 00073 }LCD_DrawPropTypeDef; 00074 00075 typedef struct 00076 { 00077 int16_t X; 00078 int16_t Y; 00079 }Point, * pPoint; 00080 00081 /** 00082 * @brief Line mode structures definition 00083 */ 00084 typedef enum 00085 { 00086 CENTER_MODE = 0x01, /* Center mode */ 00087 RIGHT_MODE = 0x02, /* Right mode */ 00088 LEFT_MODE = 0x03 /* Left mode */ 00089 }Line_ModeTypdef; 00090 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM32412G_DISCOVERY_LCD_Exported_Constants STM32412G DISCOVERY LCD Exported Constants 00096 * @{ 00097 */ 00098 /** 00099 * @brief LCD status structure definition 00100 */ 00101 #define LCD_OK ((uint8_t)0x00) 00102 #define LCD_ERROR ((uint8_t)0x01) 00103 #define LCD_TIMEOUT ((uint8_t)0x02) 00104 00105 /** 00106 * @brief LCD orientation 00107 */ 00108 #define LCD_ORIENTATION_PORTRAIT ((uint8_t)0x00) /*!< Portrait orientation choice of LCD screen */ 00109 #define LCD_ORIENTATION_LANDSCAPE ((uint8_t)0x01) /*!< Landscape orientation choice of LCD screen */ 00110 #define LCD_ORIENTATION_LANDSCAPE_ROT180 ((uint8_t)0x02) /*!< Landscape rotated 180� orientation choice of LCD screen */ 00111 00112 /** 00113 * @brief LCD color 00114 */ 00115 #define LCD_COLOR_BLUE ((uint16_t)0x001F) 00116 #define LCD_COLOR_GREEN ((uint16_t)0x07E0) 00117 #define LCD_COLOR_RED ((uint16_t)0xF800) 00118 #define LCD_COLOR_CYAN ((uint16_t)0x07FF) 00119 #define LCD_COLOR_MAGENTA ((uint16_t)0xF81F) 00120 #define LCD_COLOR_YELLOW ((uint16_t)0xFFE0) 00121 #define LCD_COLOR_LIGHTBLUE ((uint16_t)0x841F) 00122 #define LCD_COLOR_LIGHTGREEN ((uint16_t)0x87F0) 00123 #define LCD_COLOR_LIGHTRED ((uint16_t)0xFC10) 00124 #define LCD_COLOR_LIGHTMAGENTA ((uint16_t)0xFC1F) 00125 #define LCD_COLOR_LIGHTYELLOW ((uint16_t)0xFFF0) 00126 #define LCD_COLOR_DARKBLUE ((uint16_t)0x0010) 00127 #define LCD_COLOR_DARKGREEN ((uint16_t)0x0400) 00128 #define LCD_COLOR_DARKRED ((uint16_t)0x8000) 00129 #define LCD_COLOR_DARKCYAN ((uint16_t)0x0410) 00130 #define LCD_COLOR_DARKMAGENTA ((uint16_t)0x8010) 00131 #define LCD_COLOR_DARKYELLOW ((uint16_t)0x8400) 00132 #define LCD_COLOR_WHITE ((uint16_t)0xFFFF) 00133 #define LCD_COLOR_LIGHTGRAY ((uint16_t)0xD69A) 00134 #define LCD_COLOR_GRAY ((uint16_t)0x8410) 00135 #define LCD_COLOR_DARKGRAY ((uint16_t)0x4208) 00136 #define LCD_COLOR_BLACK ((uint16_t)0x0000) 00137 #define LCD_COLOR_BROWN ((uint16_t)0xA145) 00138 #define LCD_COLOR_ORANGE ((uint16_t)0xFD20) 00139 00140 /** 00141 * @brief LCD default font 00142 */ 00143 #define LCD_DEFAULT_FONT Font12 00144 00145 /** 00146 * @brief LCD special pins 00147 */ 00148 /* LCD reset pin */ 00149 #define LCD_RESET_PIN GPIO_PIN_11 00150 #define LCD_RESET_GPIO_PORT GPIOD 00151 #define LCD_RESET_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00152 #define LCD_RESET_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00153 00154 /* LCD tearing effect pin */ 00155 #define LCD_TE_PIN GPIO_PIN_3 00156 #define LCD_TE_GPIO_PORT GPIOG 00157 #define LCD_TE_GPIO_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00158 #define LCD_TE_GPIO_CLK_DISABLE() __HAL_RCC_GPIOG_CLK_DISABLE() 00159 00160 /* Backlight control pin */ 00161 #define LCD_BL_CTRL_PIN GPIO_PIN_5 00162 #define LCD_BL_CTRL_GPIO_PORT GPIOF 00163 #define LCD_BL_CTRL_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00164 #define LCD_BL_CTRL_GPIO_CLK_DISABLE() __HAL_RCC_GPIOF_CLK_DISABLE() 00165 00166 /** 00167 * @} 00168 */ 00169 00170 /** @defgroup STM32412G_DISCOVERY_LCD_Exported_Functions STM32412G DISCOVERY LCD Exported Functions 00171 * @{ 00172 */ 00173 uint8_t BSP_LCD_Init(void); 00174 uint8_t BSP_LCD_InitEx(uint32_t orientation); 00175 uint8_t BSP_LCD_DeInit(void); 00176 uint32_t BSP_LCD_GetXSize(void); 00177 uint32_t BSP_LCD_GetYSize(void); 00178 00179 uint16_t BSP_LCD_GetTextColor(void); 00180 uint16_t BSP_LCD_GetBackColor(void); 00181 void BSP_LCD_SetTextColor(__IO uint16_t Color); 00182 void BSP_LCD_SetBackColor(__IO uint16_t Color); 00183 void BSP_LCD_SetFont(sFONT *fonts); 00184 sFONT *BSP_LCD_GetFont(void); 00185 00186 void BSP_LCD_Clear(uint16_t Color); 00187 void BSP_LCD_ClearStringLine(uint16_t Line); 00188 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00189 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Line_ModeTypdef Mode); 00190 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00191 00192 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos); 00193 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGB_Code); 00194 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00195 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00196 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); 00197 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00198 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00199 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount); 00200 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00201 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp); 00202 void BSP_LCD_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pbmp); 00203 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00204 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00205 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount); 00206 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00207 00208 void BSP_LCD_DisplayOff(void); 00209 void BSP_LCD_DisplayOn(void); 00210 00211 /* These functions can be modified in case the current settings 00212 need to be changed for specific application needs */ 00213 __weak void BSP_LCD_MspInit(void); 00214 __weak void BSP_LCD_MspDeInit(void); 00215 00216 /** 00217 * @} 00218 */ 00219 00220 /** 00221 * @} 00222 */ 00223 00224 /** 00225 * @} 00226 */ 00227 00228 /** 00229 * @} 00230 */ 00231 00232 #ifdef __cplusplus 00233 } 00234 #endif 00235 00236 #endif /* __STM32412G_DISCOVERY_LCD_H */ 00237 00238 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 24 2017 11:28:05 for STM32412G-Discovery BSP User Manual by
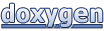