STM32412G-Discovery BSP User Manual
|
Functions | |
uint32_t | BSP_GetVersion (void) |
This method returns the STM32412G DISCOVERY BSP Driver revision. | |
void | BSP_LED_Init (Led_TypeDef Led) |
Configures LEDs. | |
void | BSP_LED_DeInit (Led_TypeDef Led) |
DeInit LEDs. | |
void | BSP_LED_On (Led_TypeDef Led) |
Turns selected LED On. | |
void | BSP_LED_Off (Led_TypeDef Led) |
Turns selected LED Off. | |
void | BSP_LED_Toggle (Led_TypeDef Led) |
Toggles the selected LED. | |
void | BSP_PB_Init (Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) |
Configures button GPIO and EXTI Line. | |
void | BSP_PB_DeInit (Button_TypeDef Button) |
Push Button DeInit. | |
uint32_t | BSP_PB_GetState (Button_TypeDef Button) |
Returns the selected button state. | |
uint8_t | BSP_JOY_Init (JOYMode_TypeDef Joy_Mode) |
Configures all joystick's buttons in GPIO or EXTI modes. | |
void | BSP_JOY_DeInit (void) |
Unconfigures all GPIOs used as joystick's buttons. | |
JOYState_TypeDef | BSP_JOY_GetState (void) |
Returns the current joystick status. | |
void | BSP_COM_Init (COM_TypeDef COM, UART_HandleTypeDef *huart) |
Configures COM port. | |
void | BSP_COM_DeInit (COM_TypeDef COM, UART_HandleTypeDef *huart) |
DeInit COM port. | |
static void | I2Cx_DeInit (I2C_HandleTypeDef *i2c_handler) |
Deinitializes I2C interface. | |
static void | I2Cx_MspInit (I2C_HandleTypeDef *i2c_handler) |
Initializes I2C MSP. | |
static void | I2Cx_Init (I2C_HandleTypeDef *i2c_handler) |
Initializes I2C HAL. | |
static HAL_StatusTypeDef | I2Cx_ReadMultiple (I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) |
Reads multiple data. | |
static HAL_StatusTypeDef | I2Cx_WriteMultiple (I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) |
Writes a value in a register of the device through BUS in using DMA mode. | |
static HAL_StatusTypeDef | I2Cx_IsDeviceReady (I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
static void | I2Cx_Error (I2C_HandleTypeDef *i2c_handler, uint8_t Addr) |
Manages error callback by re-initializing I2C. | |
static void | FMC_BANK1_WriteData (uint16_t Data) |
Writes register value. | |
static void | FMC_BANK1_WriteReg (uint8_t Reg) |
Writes register address. | |
static uint16_t | FMC_BANK1_ReadData (void) |
Reads register value. | |
void | LCD_IO_WriteData (uint16_t RegValue) |
Writes data on LCD data register. | |
void | LCD_IO_WriteMultipleData (uint16_t *pData, uint32_t Size) |
Writes several data on LCD data register. | |
void | LCD_IO_WriteReg (uint8_t Reg) |
Writes register on LCD register. | |
uint16_t | LCD_IO_ReadData (void) |
Reads data from LCD data register. | |
void | LCD_IO_Delay (uint32_t Delay) |
LCD delay. | |
void | AUDIO_IO_Write (uint8_t Addr, uint16_t Reg, uint16_t Value) |
Writes a single data. | |
uint16_t | AUDIO_IO_Read (uint8_t Addr, uint16_t Reg) |
Reads a single data. | |
void | AUDIO_IO_Delay (uint32_t Delay) |
AUDIO Codec delay. | |
HAL_StatusTypeDef | EEPROM_IO_WriteData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Write data to I2C EEPROM driver in using DMA channel. | |
HAL_StatusTypeDef | EEPROM_IO_ReadData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Read data from I2C EEPROM driver in using DMA channel. | |
HAL_StatusTypeDef | EEPROM_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
void | TS_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
Writes a single data. | |
uint8_t | TS_IO_Read (uint8_t Addr, uint8_t Reg) |
Reads a single data. | |
uint16_t | TS_IO_ReadMultiple (uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) |
Reads multiple data with I2C communication channel from TouchScreen. | |
void | TS_IO_WriteMultiple (uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) |
Writes multiple data with I2C communication channel from MCU to TouchScreen. | |
void | TS_IO_Delay (uint32_t Delay) |
Delay function used in TouchScreen low level driver. |
Function Documentation
void AUDIO_IO_Delay | ( | uint32_t | Delay | ) |
AUDIO Codec delay.
- Parameters:
-
Delay,: Delay in ms
Definition at line 1026 of file stm32412g_discovery.c.
uint16_t AUDIO_IO_Read | ( | uint8_t | Addr, |
uint16_t | Reg | ||
) |
Reads a single data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address
- Return values:
-
Data to be read
Definition at line 1007 of file stm32412g_discovery.c.
References hI2cAudioHandler, and I2Cx_ReadMultiple().
void AUDIO_IO_Write | ( | uint8_t | Addr, |
uint16_t | Reg, | ||
uint16_t | Value | ||
) |
Writes a single data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address Value,: Data to be written
Definition at line 990 of file stm32412g_discovery.c.
References hI2cAudioHandler, and I2Cx_WriteMultiple().
void BSP_COM_DeInit | ( | COM_TypeDef | COM, |
UART_HandleTypeDef * | huart | ||
) |
DeInit COM port.
- Parameters:
-
COM,: COM port to be configured. This parameter can be one of the following values: COM1 COM2 huart,: Pointer to a UART_HandleTypeDef structure that contains the configuration information for the specified USART peripheral.
Definition at line 521 of file stm32412g_discovery.c.
References COM_USART, and DISCOVERY_COMx_CLK_DISABLE.
void BSP_COM_Init | ( | COM_TypeDef | COM, |
UART_HandleTypeDef * | huart | ||
) |
Configures COM port.
- Parameters:
-
COM,: COM port to be configured. This parameter can be one of the following values: COM1 COM2 huart,: Pointer to a UART_HandleTypeDef structure that contains the configuration information for the specified USART peripheral.
Definition at line 482 of file stm32412g_discovery.c.
References COM_RX_AF, COM_RX_PIN, COM_RX_PORT, COM_TX_AF, COM_TX_PIN, COM_TX_PORT, COM_USART, DISCOVERY_COMx_CLK_ENABLE, DISCOVERY_COMx_RX_GPIO_CLK_ENABLE, and DISCOVERY_COMx_TX_GPIO_CLK_ENABLE.
uint32_t BSP_GetVersion | ( | void | ) |
This method returns the STM32412G DISCOVERY BSP Driver revision.
- Return values:
-
version,: 0xXYZR (8bits for each decimal, R for RC)
Definition at line 205 of file stm32412g_discovery.c.
References __STM32412G_DISCOVERY_BSP_VERSION.
void BSP_JOY_DeInit | ( | void | ) |
Unconfigures all GPIOs used as joystick's buttons.
Definition at line 431 of file stm32412g_discovery.c.
References JOY_PIN, JOY_PORT, JOY_SEL, JOYn, and JOYx_GPIO_CLK_ENABLE.
JOYState_TypeDef BSP_JOY_GetState | ( | void | ) |
uint8_t BSP_JOY_Init | ( | JOYMode_TypeDef | Joy_Mode | ) |
Configures all joystick's buttons in GPIO or EXTI modes.
- Parameters:
-
Joy_Mode,: Joystick mode. This parameter can be one of the following values: JOY_MODE_GPIO: Joystick pins will be used as simple IOs JOY_MODE_EXTI: Joystick pins will be connected to EXTI line with interrupt generation capability
- Return values:
-
HAL_OK,: if all initializations are OK. Other value if error.
Definition at line 392 of file stm32412g_discovery.c.
References JOY_IRQn, JOY_MODE_EXTI, JOY_MODE_GPIO, JOY_PIN, JOY_PORT, JOY_SEL, JOYn, and JOYx_GPIO_CLK_ENABLE.
void BSP_LED_DeInit | ( | Led_TypeDef | Led | ) |
DeInit LEDs.
- Parameters:
-
Led,: LED to be configured. This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
- Note:
- Led DeInit does not disable the GPIO clock nor disable the Mfx
Definition at line 249 of file stm32412g_discovery.c.
References GPIO_PIN, and LEDx_GPIO_PORT.
void BSP_LED_Init | ( | Led_TypeDef | Led | ) |
Configures LEDs.
- Parameters:
-
Led,: LED to be configured. This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
Definition at line 219 of file stm32412g_discovery.c.
References GPIO_PIN, LEDx_GPIO_CLK_ENABLE, and LEDx_GPIO_PORT.
void BSP_LED_Off | ( | Led_TypeDef | Led | ) |
Turns selected LED Off.
- Parameters:
-
Led,: LED to be set off This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
Definition at line 283 of file stm32412g_discovery.c.
References GPIO_PIN, and LEDx_GPIO_PORT.
void BSP_LED_On | ( | Led_TypeDef | Led | ) |
Turns selected LED On.
- Parameters:
-
Led,: LED to be set on This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
Definition at line 269 of file stm32412g_discovery.c.
References GPIO_PIN, and LEDx_GPIO_PORT.
void BSP_LED_Toggle | ( | Led_TypeDef | Led | ) |
Toggles the selected LED.
- Parameters:
-
Led,: LED to be toggled This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
Definition at line 297 of file stm32412g_discovery.c.
References GPIO_PIN, and LEDx_GPIO_PORT.
void BSP_PB_DeInit | ( | Button_TypeDef | Button | ) |
Push Button DeInit.
- Parameters:
-
Button,: Button to be configured This parameter can be one of the following values: - BUTTON_WAKEUP: Wakeup Push Button
- Note:
- On STM32412G-DISCOVERY board, the Wakeup button is mapped on the same push button which is the joystick selection button.
- PB DeInit does not disable the GPIO clock
Definition at line 358 of file stm32412g_discovery.c.
References BUTTON_IRQn, BUTTON_PIN, and BUTTON_PORT.
uint32_t BSP_PB_GetState | ( | Button_TypeDef | Button | ) |
Returns the selected button state.
- Parameters:
-
Button,: Button to be checked This parameter can be one of the following values: - BUTTON_WAKEUP: Wakeup Push Button
- Note:
- On STM32412G-DISCOVERY board, the Wakeup button is mapped on the same push button which is the joystick selection button.
- Return values:
-
The Button GPIO pin value (GPIO_PIN_RESET = button pressed)
Definition at line 377 of file stm32412g_discovery.c.
References BUTTON_PIN, and BUTTON_PORT.
void BSP_PB_Init | ( | Button_TypeDef | Button, |
ButtonMode_TypeDef | ButtonMode | ||
) |
Configures button GPIO and EXTI Line.
- Parameters:
-
Button,: Button to be configured This parameter can be one of the following values: - BUTTON_WAKEUP: Wakeup Push Button
ButtonMode,: Button mode This parameter can be one of the following values: - BUTTON_MODE_GPIO: Button will be used as simple IO
- BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt generation capability
- Note:
- On STM32412G-DISCOVERY board, the Wakeup button is mapped on the same push button which is the joystick selection button.
Definition at line 315 of file stm32412g_discovery.c.
References BUTTON_IRQn, BUTTON_MODE_EXTI, BUTTON_MODE_GPIO, BUTTON_PIN, BUTTON_PORT, and WAKEUP_BUTTON_GPIO_CLK_ENABLE.
HAL_StatusTypeDef EEPROM_IO_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
DevAddress,: Target device address Trials,: Number of trials
- Return values:
-
HAL status
Definition at line 1074 of file stm32412g_discovery.c.
References hI2cExtHandler, and I2Cx_IsDeviceReady().
Referenced by BSP_EEPROM_Init(), and BSP_EEPROM_WaitEepromStandbyState().
HAL_StatusTypeDef EEPROM_IO_ReadData | ( | uint16_t | DevAddress, |
uint16_t | MemAddress, | ||
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Read data from I2C EEPROM driver in using DMA channel.
- Parameters:
-
DevAddress,: Target device address MemAddress,: Internal memory address pBuffer,: Pointer to data buffer BufferSize,: Amount of data to be read
- Return values:
-
HAL status
Definition at line 1062 of file stm32412g_discovery.c.
References hI2cExtHandler, and I2Cx_ReadMultiple().
Referenced by BSP_EEPROM_ReadBuffer().
HAL_StatusTypeDef EEPROM_IO_WriteData | ( | uint16_t | DevAddress, |
uint16_t | MemAddress, | ||
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Write data to I2C EEPROM driver in using DMA channel.
- Parameters:
-
DevAddress,: Target device address MemAddress,: Internal memory address pBuffer,: Pointer to data buffer BufferSize,: Amount of data to be sent
- Return values:
-
HAL status
Definition at line 1049 of file stm32412g_discovery.c.
References hI2cExtHandler, and I2Cx_WriteMultiple().
Referenced by BSP_EEPROM_WritePage().
static uint16_t FMC_BANK1_ReadData | ( | void | ) | [static] |
Reads register value.
- Return values:
-
Read value
Definition at line 894 of file stm32412g_discovery.c.
References FMC_BANK1.
Referenced by LCD_IO_ReadData().
static void FMC_BANK1_WriteData | ( | uint16_t | Data | ) | [static] |
Writes register value.
- Parameters:
-
Data,: Data to be written
Definition at line 872 of file stm32412g_discovery.c.
References FMC_BANK1.
Referenced by LCD_IO_WriteData(), and LCD_IO_WriteMultipleData().
static void FMC_BANK1_WriteReg | ( | uint8_t | Reg | ) | [static] |
Writes register address.
- Parameters:
-
Reg,: Register to be written
Definition at line 883 of file stm32412g_discovery.c.
References FMC_BANK1.
Referenced by LCD_IO_WriteReg().
static void I2Cx_DeInit | ( | I2C_HandleTypeDef * | i2c_handler | ) | [static] |
Deinitializes I2C interface.
- Parameters:
-
i2c_handler : I2C handler
Definition at line 755 of file stm32412g_discovery.c.
References DISCOVERY_AUDIO_I2Cx, DISCOVERY_EXT_I2Cx, and hI2cAudioHandler.
Referenced by AUDIO_IO_DeInit().
static void I2Cx_Error | ( | I2C_HandleTypeDef * | i2c_handler, |
uint8_t | Addr | ||
) | [static] |
Manages error callback by re-initializing I2C.
- Parameters:
-
i2c_handler : I2C handler Addr,: I2C Address
Definition at line 742 of file stm32412g_discovery.c.
References I2Cx_Init().
Referenced by I2Cx_ReadMultiple(), and I2Cx_WriteMultiple().
static void I2Cx_Init | ( | I2C_HandleTypeDef * | i2c_handler | ) | [static] |
Initializes I2C HAL.
- Parameters:
-
i2c_handler : I2C handler
Definition at line 635 of file stm32412g_discovery.c.
References DISCOVERY_AUDIO_I2Cx, DISCOVERY_EXT_I2Cx, hI2cAudioHandler, and I2Cx_MspInit().
Referenced by AUDIO_IO_Init(), EEPROM_IO_Init(), I2Cx_Error(), and TS_IO_Init().
static HAL_StatusTypeDef I2Cx_IsDeviceReady | ( | I2C_HandleTypeDef * | i2c_handler, |
uint16_t | DevAddress, | ||
uint32_t | Trials | ||
) | [static] |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
i2c_handler : I2C handler DevAddress,: Target device address Trials,: Number of trials
- Return values:
-
HAL status
Definition at line 732 of file stm32412g_discovery.c.
Referenced by EEPROM_IO_IsDeviceReady().
static void I2Cx_MspInit | ( | I2C_HandleTypeDef * | i2c_handler | ) | [static] |
Initializes I2C MSP.
- Parameters:
-
i2c_handler : I2C handler
Definition at line 547 of file stm32412g_discovery.c.
References DISCOVERY_AUDIO_I2Cx_CLK_ENABLE, DISCOVERY_AUDIO_I2Cx_ER_IRQn, DISCOVERY_AUDIO_I2Cx_EV_IRQn, DISCOVERY_AUDIO_I2Cx_FORCE_RESET, DISCOVERY_AUDIO_I2Cx_RELEASE_RESET, DISCOVERY_AUDIO_I2Cx_SCL_PIN, DISCOVERY_AUDIO_I2Cx_SCL_SDA_AF, DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_CLK_ENABLE, DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, DISCOVERY_AUDIO_I2Cx_SDA_PIN, DISCOVERY_EXT_I2Cx_CLK_ENABLE, DISCOVERY_EXT_I2Cx_ER_IRQn, DISCOVERY_EXT_I2Cx_EV_IRQn, DISCOVERY_EXT_I2Cx_FORCE_RESET, DISCOVERY_EXT_I2Cx_RELEASE_RESET, DISCOVERY_EXT_I2Cx_SCL_AF, DISCOVERY_EXT_I2Cx_SCL_PIN, DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_CLK_ENABLE, DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, DISCOVERY_EXT_I2Cx_SDA_AF, DISCOVERY_EXT_I2Cx_SDA_PIN, and hI2cAudioHandler.
Referenced by I2Cx_Init().
static HAL_StatusTypeDef I2Cx_ReadMultiple | ( | I2C_HandleTypeDef * | i2c_handler, |
uint8_t | Addr, | ||
uint16_t | Reg, | ||
uint16_t | MemAddress, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) | [static] |
Reads multiple data.
- Parameters:
-
i2c_handler : I2C handler Addr,: I2C address Reg,: Reg address MemAddress,: Memory address Buffer,: Pointer to data buffer Length,: Length of the data
- Return values:
-
HAL status
Definition at line 674 of file stm32412g_discovery.c.
References I2Cx_Error().
Referenced by AUDIO_IO_Read(), EEPROM_IO_ReadData(), TS_IO_Read(), and TS_IO_ReadMultiple().
static HAL_StatusTypeDef I2Cx_WriteMultiple | ( | I2C_HandleTypeDef * | i2c_handler, |
uint8_t | Addr, | ||
uint16_t | Reg, | ||
uint16_t | MemAddress, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) | [static] |
Writes a value in a register of the device through BUS in using DMA mode.
- Parameters:
-
i2c_handler : I2C handler Addr,: Device address on BUS Bus. Reg,: The target register address to write MemAddress,: Memory address Buffer,: The target register value to be written Length,: buffer size to be written
- Return values:
-
HAL status
Definition at line 704 of file stm32412g_discovery.c.
References I2Cx_Error().
Referenced by AUDIO_IO_Write(), EEPROM_IO_WriteData(), TS_IO_Write(), and TS_IO_WriteMultiple().
void LCD_IO_Delay | ( | uint32_t | Delay | ) |
uint16_t LCD_IO_ReadData | ( | void | ) |
Reads data from LCD data register.
- Return values:
-
Read data.
Definition at line 952 of file stm32412g_discovery.c.
References FMC_BANK1_ReadData().
void LCD_IO_WriteData | ( | uint16_t | RegValue | ) |
Writes data on LCD data register.
- Parameters:
-
RegValue,: Data to be written
Definition at line 917 of file stm32412g_discovery.c.
References FMC_BANK1_WriteData().
void LCD_IO_WriteMultipleData | ( | uint16_t * | pData, |
uint32_t | Size | ||
) |
Writes several data on LCD data register.
- Parameters:
-
pData,: pointer on data to be written Size,: data amount in 16bits short unit
Definition at line 928 of file stm32412g_discovery.c.
References FMC_BANK1_WriteData().
void LCD_IO_WriteReg | ( | uint8_t | Reg | ) |
Writes register on LCD register.
- Parameters:
-
Reg,: Register to be written
Definition at line 942 of file stm32412g_discovery.c.
References FMC_BANK1_WriteReg().
void TS_IO_Delay | ( | uint32_t | Delay | ) |
Delay function used in TouchScreen low level driver.
- Parameters:
-
Delay,: Delay in ms
Definition at line 1145 of file stm32412g_discovery.c.
uint8_t TS_IO_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) |
Reads a single data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address
- Return values:
-
Data to be read
Definition at line 1105 of file stm32412g_discovery.c.
References hI2cAudioHandler, and I2Cx_ReadMultiple().
uint16_t TS_IO_ReadMultiple | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) |
Reads multiple data with I2C communication channel from TouchScreen.
- Parameters:
-
Addr,: I2C address Reg,: Register address Buffer,: Pointer to data buffer Length,: Length of the data
- Return values:
-
HAL status
Definition at line 1123 of file stm32412g_discovery.c.
References hI2cAudioHandler, and I2Cx_ReadMultiple().
void TS_IO_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
Writes a single data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address Value,: Data to be written
Definition at line 1094 of file stm32412g_discovery.c.
References hI2cAudioHandler, and I2Cx_WriteMultiple().
void TS_IO_WriteMultiple | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) |
Writes multiple data with I2C communication channel from MCU to TouchScreen.
- Parameters:
-
Addr,: I2C address Reg,: Register address Buffer,: Pointer to data buffer Length,: Length of the data
Definition at line 1136 of file stm32412g_discovery.c.
References hI2cAudioHandler, and I2Cx_WriteMultiple().
Generated on Tue Jan 24 2017 11:28:05 for STM32412G-Discovery BSP User Manual by
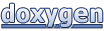