STM32412G-Discovery BSP User Manual
|
stm32412g_discovery_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32412g_discovery_lcd.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 27-January-2017 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM32412G-DISCOVERY board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive indirectly an LCD TFT. 00044 - This driver supports the LS016B8UY LCD. 00045 - The LS016B8UY component driver MUST be included with this driver. 00046 00047 2. Driver description: 00048 --------------------- 00049 + Initialization steps: 00050 o Initialize the LCD using the BSP_LCD_Init() function. 00051 00052 + Display on LCD 00053 o Clear the hole LCD using BSP_LCD_Clear() function or only one specified string 00054 line using the BSP_LCD_ClearStringLine() function. 00055 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00056 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00057 o Display a string line on the specified position (x,y in pixel) and align mode 00058 using the BSP_LCD_DisplayStringAtLine() function. 00059 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00060 on LCD using the available set of functions. 00061 00062 ------------------------------------------------------------------------------*/ 00063 00064 /* Includes ------------------------------------------------------------------*/ 00065 #include "stm32412g_discovery_lcd.h" 00066 #include "../../../Utilities/Fonts/fonts.h" 00067 #include "../../../Utilities/Fonts/font24.c" 00068 #include "../../../Utilities/Fonts/font20.c" 00069 #include "../../../Utilities/Fonts/font16.c" 00070 #include "../../../Utilities/Fonts/font12.c" 00071 #include "../../../Utilities/Fonts/font8.c" 00072 00073 /** @addtogroup BSP 00074 * @{ 00075 */ 00076 00077 /** @addtogroup STM32412G_DISCOVERY 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM32412G_DISCOVERY_LCD STM32412G-DISCOVERY LCD 00082 * @{ 00083 */ 00084 00085 /** @defgroup STM32412G_DISCOVERY_LCD_Private_Macros STM32412G Discovery Lcd Private Macros 00086 * @{ 00087 */ 00088 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00089 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00090 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM32412G_DISCOVERY_LCD_Private_Variables STM32412G Discovery Lcd Private Variables 00096 * @{ 00097 */ 00098 LCD_DrawPropTypeDef DrawProp; 00099 static LCD_DrvTypeDef *LcdDrv; 00100 /** 00101 * @} 00102 */ 00103 00104 /** @defgroup STM32412G_DISCOVERY_LCD_Private_FunctionPrototypes STM32412G Discovery Lcd Private FunctionPrototypes 00105 * @{ 00106 */ 00107 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00108 static void SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00109 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32412G_DISCOVERY_LCD_Private_Functions STM32412G Discovery Lcd Private Functions 00115 * @{ 00116 */ 00117 /** 00118 * @brief Initializes the LCD. 00119 * @retval LCD state 00120 */ 00121 uint8_t BSP_LCD_Init(void) 00122 { 00123 return (BSP_LCD_InitEx(LCD_ORIENTATION_LANDSCAPE)); 00124 } 00125 /** 00126 * @brief Initializes the LCD with a given orientation. 00127 * @param orientation: LCD_ORIENTATION_PORTRAIT or LCD_ORIENTATION_LANDSCAPE 00128 * @retval LCD state 00129 */ 00130 uint8_t BSP_LCD_InitEx(uint32_t orientation) 00131 { 00132 uint8_t ret = LCD_ERROR; 00133 00134 /* Default value for draw propriety */ 00135 DrawProp.BackColor = 0xFFFF; 00136 DrawProp.pFont = &Font24; 00137 DrawProp.TextColor = 0x0000; 00138 00139 /* Initialize LCD special pins GPIOs */ 00140 BSP_LCD_MspInit(); 00141 00142 /* Backlight control signal assertion */ 00143 HAL_GPIO_WritePin(LCD_BL_CTRL_GPIO_PORT, LCD_BL_CTRL_PIN, GPIO_PIN_SET); 00144 00145 /* Apply hardware reset according to procedure indicated in FRD154BP2901 documentation */ 00146 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_RESET); 00147 HAL_Delay(5); /* Reset signal asserted during 5ms */ 00148 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_SET); 00149 HAL_Delay(10); /* Reset signal released during 10ms */ 00150 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_RESET); 00151 HAL_Delay(20); /* Reset signal asserted during 20ms */ 00152 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_SET); 00153 HAL_Delay(10); /* Reset signal released during 10ms */ 00154 00155 if(ST7789H2_drv.ReadID() == ST7789H2_ID) 00156 { 00157 LcdDrv = &ST7789H2_drv; 00158 00159 /* LCD Init */ 00160 LcdDrv->Init(); 00161 00162 if(orientation == LCD_ORIENTATION_PORTRAIT) 00163 { 00164 ST7789H2_SetOrientation(ST7789H2_ORIENTATION_PORTRAIT); 00165 } 00166 else if(orientation == LCD_ORIENTATION_LANDSCAPE_ROT180) 00167 { 00168 ST7789H2_SetOrientation(ST7789H2_ORIENTATION_LANDSCAPE_ROT180); 00169 } 00170 else 00171 { 00172 /* Default landscape orientation is selected */ 00173 } 00174 00175 /* Initialize the font */ 00176 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00177 00178 ret = LCD_OK; 00179 } 00180 else if(ls016b8uy_drv.ReadID() == LS016B8UY_ID) 00181 { 00182 LcdDrv = &ls016b8uy_drv; 00183 00184 /* LCD Init */ 00185 LcdDrv->Init(); 00186 00187 /* Initialize the font */ 00188 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00189 00190 ret = LCD_OK; 00191 } 00192 00193 return ret; 00194 } 00195 00196 /** 00197 * @brief DeInitializes the LCD. 00198 * @retval LCD state 00199 */ 00200 uint8_t BSP_LCD_DeInit(void) 00201 { 00202 /* Actually LcdDrv does not provide a DeInit function */ 00203 return LCD_OK; 00204 } 00205 00206 /** 00207 * @brief Gets the LCD X size. 00208 * @retval Used LCD X size 00209 */ 00210 uint32_t BSP_LCD_GetXSize(void) 00211 { 00212 return(LcdDrv->GetLcdPixelWidth()); 00213 } 00214 00215 /** 00216 * @brief Gets the LCD Y size. 00217 * @retval Used LCD Y size 00218 */ 00219 uint32_t BSP_LCD_GetYSize(void) 00220 { 00221 return(LcdDrv->GetLcdPixelHeight()); 00222 } 00223 00224 /** 00225 * @brief Gets the LCD text color. 00226 * @retval Used text color. 00227 */ 00228 uint16_t BSP_LCD_GetTextColor(void) 00229 { 00230 return DrawProp.TextColor; 00231 } 00232 00233 /** 00234 * @brief Gets the LCD background color. 00235 * @retval Used background color 00236 */ 00237 uint16_t BSP_LCD_GetBackColor(void) 00238 { 00239 return DrawProp.BackColor; 00240 } 00241 00242 /** 00243 * @brief Sets the LCD text color. 00244 * @param Color: Text color code RGB(5-6-5) 00245 */ 00246 void BSP_LCD_SetTextColor(uint16_t Color) 00247 { 00248 DrawProp.TextColor = Color; 00249 } 00250 00251 /** 00252 * @brief Sets the LCD background color. 00253 * @param Color: Background color code RGB(5-6-5) 00254 */ 00255 void BSP_LCD_SetBackColor(uint16_t Color) 00256 { 00257 DrawProp.BackColor = Color; 00258 } 00259 00260 /** 00261 * @brief Sets the LCD text font. 00262 * @param fonts: Font to be used 00263 */ 00264 void BSP_LCD_SetFont(sFONT *fonts) 00265 { 00266 DrawProp.pFont = fonts; 00267 } 00268 00269 /** 00270 * @brief Gets the LCD text font. 00271 * @retval Used font 00272 */ 00273 sFONT *BSP_LCD_GetFont(void) 00274 { 00275 return DrawProp.pFont; 00276 } 00277 00278 /** 00279 * @brief Clears the hole LCD. 00280 * @param Color: Color of the background 00281 */ 00282 void BSP_LCD_Clear(uint16_t Color) 00283 { 00284 uint32_t counter = 0; 00285 uint32_t y_size = 0; 00286 uint32_t color_backup = DrawProp.TextColor; 00287 00288 DrawProp.TextColor = Color; 00289 y_size = BSP_LCD_GetYSize(); 00290 00291 for(counter = 0; counter < y_size; counter++) 00292 { 00293 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00294 } 00295 DrawProp.TextColor = color_backup; 00296 BSP_LCD_SetTextColor(DrawProp.TextColor); 00297 } 00298 00299 /** 00300 * @brief Clears the selected line. 00301 * @param Line: Line to be cleared 00302 * This parameter can be one of the following values: 00303 * @arg 0..9: if the Current fonts is Font16x24 00304 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00305 * @arg 0..29: if the Current fonts is Font8x8 00306 */ 00307 void BSP_LCD_ClearStringLine(uint16_t Line) 00308 { 00309 uint32_t color_backup = DrawProp.TextColor; 00310 00311 DrawProp.TextColor = DrawProp.BackColor;; 00312 00313 /* Draw a rectangle with background color */ 00314 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00315 00316 DrawProp.TextColor = color_backup; 00317 BSP_LCD_SetTextColor(DrawProp.TextColor); 00318 } 00319 00320 /** 00321 * @brief Displays one character. 00322 * @param Xpos: Start column address 00323 * @param Ypos: Line where to display the character shape. 00324 * @param Ascii: Character ascii code 00325 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00326 */ 00327 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00328 { 00329 DrawChar(Xpos, Ypos, &DrawProp.pFont->table[(Ascii-' ') *\ 00330 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00331 } 00332 00333 /** 00334 * @brief Displays characters on the LCD. 00335 * @param Xpos: X position (in pixel) 00336 * @param Ypos: Y position (in pixel) 00337 * @param Text: Pointer to string to display on LCD 00338 * @param Mode: Display mode 00339 * This parameter can be one of the following values: 00340 * @arg CENTER_MODE 00341 * @arg RIGHT_MODE 00342 * @arg LEFT_MODE 00343 */ 00344 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Line_ModeTypdef Mode) 00345 { 00346 uint16_t refcolumn = 1, i = 0; 00347 uint32_t size = 0, xsize = 0; 00348 uint8_t *ptr = Text; 00349 00350 /* Get the text size */ 00351 while (*ptr++) size ++ ; 00352 00353 /* Characters number per line */ 00354 xsize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00355 00356 switch (Mode) 00357 { 00358 case CENTER_MODE: 00359 { 00360 refcolumn = Xpos + ((xsize - size)* DrawProp.pFont->Width) / 2; 00361 break; 00362 } 00363 case LEFT_MODE: 00364 { 00365 refcolumn = Xpos; 00366 break; 00367 } 00368 case RIGHT_MODE: 00369 { 00370 refcolumn = - Xpos + ((xsize - size)*DrawProp.pFont->Width); 00371 break; 00372 } 00373 default: 00374 { 00375 refcolumn = Xpos; 00376 break; 00377 } 00378 } 00379 00380 /* Check that the Start column is located in the screen */ 00381 if ((refcolumn < 1) || (refcolumn >= 0x8000)) 00382 { 00383 refcolumn = 1; 00384 } 00385 00386 /* Send the string character by character on lCD */ 00387 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00388 { 00389 /* Display one character on LCD */ 00390 BSP_LCD_DisplayChar(refcolumn, Ypos, *Text); 00391 /* Decrement the column position by 16 */ 00392 refcolumn += DrawProp.pFont->Width; 00393 /* Point on the next character */ 00394 Text++; 00395 i++; 00396 } 00397 } 00398 00399 /** 00400 * @brief Displays a character on the LCD. 00401 * @param Line: Line where to display the character shape 00402 * This parameter can be one of the following values: 00403 * @arg 0..9: if the Current fonts is Font16x24 00404 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00405 * @arg 0..29: if the Current fonts is Font8x8 00406 * @param ptr: Pointer to string to display on LCD 00407 */ 00408 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00409 { 00410 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00411 } 00412 00413 /** 00414 * @brief Reads an LCD pixel. 00415 * @param Xpos: X position 00416 * @param Ypos: Y position 00417 * @retval RGB pixel color 00418 */ 00419 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00420 { 00421 uint16_t ret = 0; 00422 00423 if(LcdDrv->ReadPixel != NULL) 00424 { 00425 ret = LcdDrv->ReadPixel(Xpos, Ypos); 00426 } 00427 00428 return ret; 00429 } 00430 00431 /** 00432 * @brief Draws a pixel on LCD. 00433 * @param Xpos: X position 00434 * @param Ypos: Y position 00435 * @param RGB_Code: Pixel color in RGB mode (5-6-5) 00436 */ 00437 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGB_Code) 00438 { 00439 if(LcdDrv->WritePixel != NULL) 00440 { 00441 LcdDrv->WritePixel(Xpos, Ypos, RGB_Code); 00442 } 00443 } 00444 00445 /** 00446 * @brief Draws an horizontal line. 00447 * @param Xpos: X position 00448 * @param Ypos: Y position 00449 * @param Length: Line length 00450 */ 00451 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00452 { 00453 uint32_t index = 0; 00454 00455 if(LcdDrv->DrawHLine != NULL) 00456 { 00457 LcdDrv->DrawHLine(DrawProp.TextColor, Xpos, Ypos, Length); 00458 } 00459 else 00460 { 00461 for(index = 0; index < Length; index++) 00462 { 00463 BSP_LCD_DrawPixel((Xpos + index), Ypos, DrawProp.TextColor); 00464 } 00465 } 00466 } 00467 00468 /** 00469 * @brief Draws a vertical line. 00470 * @param Xpos: X position 00471 * @param Ypos: Y position 00472 * @param Length: Line length 00473 */ 00474 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00475 { 00476 uint32_t index = 0; 00477 00478 if(LcdDrv->DrawVLine != NULL) 00479 { 00480 LcdDrv->DrawVLine(DrawProp.TextColor, Xpos, Ypos, Length); 00481 } 00482 else 00483 { 00484 for(index = 0; index < Length; index++) 00485 { 00486 BSP_LCD_DrawPixel(Xpos, Ypos + index, DrawProp.TextColor); 00487 } 00488 } 00489 } 00490 00491 /** 00492 * @brief Draws an uni-line (between two points). 00493 * @param x1: Point 1 X position 00494 * @param y1: Point 1 Y position 00495 * @param x2: Point 2 X position 00496 * @param y2: Point 2 Y position 00497 */ 00498 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00499 { 00500 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00501 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00502 curpixel = 0; 00503 00504 deltax = ABS(x2 - x1); /* The difference between the x's */ 00505 deltay = ABS(y2 - y1); /* The difference between the y's */ 00506 x = x1; /* Start x off at the first pixel */ 00507 y = y1; /* Start y off at the first pixel */ 00508 00509 if (x2 >= x1) /* The x-values are increasing */ 00510 { 00511 xinc1 = 1; 00512 xinc2 = 1; 00513 } 00514 else /* The x-values are decreasing */ 00515 { 00516 xinc1 = -1; 00517 xinc2 = -1; 00518 } 00519 00520 if (y2 >= y1) /* The y-values are increasing */ 00521 { 00522 yinc1 = 1; 00523 yinc2 = 1; 00524 } 00525 else /* The y-values are decreasing */ 00526 { 00527 yinc1 = -1; 00528 yinc2 = -1; 00529 } 00530 00531 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00532 { 00533 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00534 yinc2 = 0; /* Don't change the y for every iteration */ 00535 den = deltax; 00536 num = deltax / 2; 00537 numadd = deltay; 00538 numpixels = deltax; /* There are more x-values than y-values */ 00539 } 00540 else /* There is at least one y-value for every x-value */ 00541 { 00542 xinc2 = 0; /* Don't change the x for every iteration */ 00543 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00544 den = deltay; 00545 num = deltay / 2; 00546 numadd = deltax; 00547 numpixels = deltay; /* There are more y-values than x-values */ 00548 } 00549 00550 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00551 { 00552 BSP_LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00553 num += numadd; /* Increase the numerator by the top of the fraction */ 00554 if (num >= den) /* Check if numerator >= denominator */ 00555 { 00556 num -= den; /* Calculate the new numerator value */ 00557 x += xinc1; /* Change the x as appropriate */ 00558 y += yinc1; /* Change the y as appropriate */ 00559 } 00560 x += xinc2; /* Change the x as appropriate */ 00561 y += yinc2; /* Change the y as appropriate */ 00562 } 00563 } 00564 00565 /** 00566 * @brief Draws a rectangle. 00567 * @param Xpos: X position 00568 * @param Ypos: Y position 00569 * @param Width: Rectangle width 00570 * @param Height: Rectangle height 00571 */ 00572 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00573 { 00574 /* Draw horizontal lines */ 00575 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00576 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00577 00578 /* Draw vertical lines */ 00579 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00580 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00581 } 00582 00583 /** 00584 * @brief Draws a circle. 00585 * @param Xpos: X position 00586 * @param Ypos: Y position 00587 * @param Radius: Circle radius 00588 */ 00589 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00590 { 00591 int32_t decision; /* Decision Variable */ 00592 uint32_t current_x; /* Current X Value */ 00593 uint32_t current_y; /* Current Y Value */ 00594 00595 decision = 3 - (Radius << 1); 00596 current_x = 0; 00597 current_y = Radius; 00598 00599 while (current_x <= current_y) 00600 { 00601 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos - current_y), DrawProp.TextColor); 00602 00603 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos - current_y), DrawProp.TextColor); 00604 00605 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos - current_x), DrawProp.TextColor); 00606 00607 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos - current_x), DrawProp.TextColor); 00608 00609 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos + current_y), DrawProp.TextColor); 00610 00611 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos + current_y), DrawProp.TextColor); 00612 00613 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos + current_x), DrawProp.TextColor); 00614 00615 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos + current_x), DrawProp.TextColor); 00616 00617 /* Initialize the font */ 00618 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00619 00620 if (decision < 0) 00621 { 00622 decision += (current_x << 2) + 6; 00623 } 00624 else 00625 { 00626 decision += ((current_x - current_y) << 2) + 10; 00627 current_y--; 00628 } 00629 current_x++; 00630 } 00631 } 00632 00633 /** 00634 * @brief Draws an poly-line (between many points). 00635 * @param Points: Pointer to the points array 00636 * @param PointCount: Number of points 00637 */ 00638 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00639 { 00640 int16_t x = 0, y = 0; 00641 00642 if(PointCount < 2) 00643 { 00644 return; 00645 } 00646 00647 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00648 00649 while(--PointCount) 00650 { 00651 x = Points->X; 00652 y = Points->Y; 00653 Points++; 00654 BSP_LCD_DrawLine(x, y, Points->X, Points->Y); 00655 } 00656 } 00657 00658 /** 00659 * @brief Draws an ellipse on LCD. 00660 * @param Xpos: X position 00661 * @param Ypos: Y position 00662 * @param XRadius: Ellipse X radius 00663 * @param YRadius: Ellipse Y radius 00664 */ 00665 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00666 { 00667 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00668 float k = 0, rad1 = 0, rad2 = 0; 00669 00670 rad1 = XRadius; 00671 rad2 = YRadius; 00672 00673 k = (float)(rad2/rad1); 00674 00675 do { 00676 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp.TextColor); 00677 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp.TextColor); 00678 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp.TextColor); 00679 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp.TextColor); 00680 00681 e2 = err; 00682 if (e2 <= x) { 00683 err += ++x*2+1; 00684 if (-y == x && e2 <= y) e2 = 0; 00685 } 00686 if (e2 > y) err += ++y*2+1; 00687 } 00688 while (y <= 0); 00689 } 00690 00691 /** 00692 * @brief Draws a bitmap picture (16 bpp). 00693 * @param Xpos: Bmp X position in the LCD 00694 * @param Ypos: Bmp Y position in the LCD 00695 * @param pbmp: Pointer to Bmp picture address. 00696 */ 00697 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp) 00698 { 00699 uint32_t height = 0; 00700 uint32_t width = 0; 00701 00702 00703 /* Read bitmap width */ 00704 width = *(uint16_t *) (pbmp + 18); 00705 width |= (*(uint16_t *) (pbmp + 20)) << 16; 00706 00707 /* Read bitmap height */ 00708 height = *(uint16_t *) (pbmp + 22); 00709 height |= (*(uint16_t *) (pbmp + 24)) << 16; 00710 00711 SetDisplayWindow(Xpos, Ypos, width, height); 00712 00713 if(LcdDrv->DrawBitmap != NULL) 00714 { 00715 LcdDrv->DrawBitmap(Xpos, Ypos, pbmp); 00716 } 00717 SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00718 } 00719 00720 /** 00721 * @brief Draws RGB Image (16 bpp). 00722 * @param Xpos: X position in the LCD 00723 * @param Ypos: Y position in the LCD 00724 * @param Xsize: X size in the LCD 00725 * @param Ysize: Y size in the LCD 00726 * @param pdata: Pointer to the RGB Image address. 00727 * @retval None 00728 */ 00729 void BSP_LCD_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pdata) 00730 { 00731 SetDisplayWindow(Xpos, Ypos, Xsize, Ysize); 00732 00733 if(LcdDrv->DrawRGBImage != NULL) 00734 { 00735 LcdDrv->DrawRGBImage(Xpos, Ypos, Xsize, Ysize, pdata); 00736 } 00737 SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00738 } 00739 00740 /** 00741 * @brief Draws a full rectangle. 00742 * @param Xpos: X position 00743 * @param Ypos: Y position 00744 * @param Width: Rectangle width 00745 * @param Height: Rectangle height 00746 */ 00747 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00748 { 00749 BSP_LCD_SetTextColor(DrawProp.TextColor); 00750 do 00751 { 00752 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00753 } 00754 while(Height--); 00755 } 00756 00757 /** 00758 * @brief Draws a full circle. 00759 * @param Xpos: X position 00760 * @param Ypos: Y position 00761 * @param Radius: Circle radius 00762 */ 00763 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00764 { 00765 int32_t decision; /* Decision Variable */ 00766 uint32_t current_x; /* Current X Value */ 00767 uint32_t current_y; /* Current Y Value */ 00768 00769 decision = 3 - (Radius << 1); 00770 00771 current_x = 0; 00772 current_y = Radius; 00773 00774 BSP_LCD_SetTextColor(DrawProp.TextColor); 00775 00776 while (current_x <= current_y) 00777 { 00778 if(current_y > 0) 00779 { 00780 BSP_LCD_DrawHLine(Xpos - current_y, Ypos + current_x, 2*current_y); 00781 BSP_LCD_DrawHLine(Xpos - current_y, Ypos - current_x, 2*current_y); 00782 } 00783 00784 if(current_x > 0) 00785 { 00786 BSP_LCD_DrawHLine(Xpos - current_x, Ypos - current_y, 2*current_x); 00787 BSP_LCD_DrawHLine(Xpos - current_x, Ypos + current_y, 2*current_x); 00788 } 00789 if (decision < 0) 00790 { 00791 decision += (current_x << 2) + 6; 00792 } 00793 else 00794 { 00795 decision += ((current_x - current_y) << 2) + 10; 00796 current_y--; 00797 } 00798 current_x++; 00799 } 00800 00801 BSP_LCD_SetTextColor(DrawProp.TextColor); 00802 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00803 } 00804 00805 /** 00806 * @brief Draws a full poly-line (between many points). 00807 * @param Points: Pointer to the points array 00808 * @param PointCount: Number of points 00809 */ 00810 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 00811 { 00812 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 00813 uint16_t IMAGE_LEFT = 0, IMAGE_RIGHT = 0, IMAGE_TOP = 0, IMAGE_BOTTOM = 0; 00814 00815 IMAGE_LEFT = IMAGE_RIGHT = Points->X; 00816 IMAGE_TOP= IMAGE_BOTTOM = Points->Y; 00817 00818 for(counter = 1; counter < PointCount; counter++) 00819 { 00820 pixelX = POLY_X(counter); 00821 if(pixelX < IMAGE_LEFT) 00822 { 00823 IMAGE_LEFT = pixelX; 00824 } 00825 if(pixelX > IMAGE_RIGHT) 00826 { 00827 IMAGE_RIGHT = pixelX; 00828 } 00829 00830 pixelY = POLY_Y(counter); 00831 if(pixelY < IMAGE_TOP) 00832 { 00833 IMAGE_TOP = pixelY; 00834 } 00835 if(pixelY > IMAGE_BOTTOM) 00836 { 00837 IMAGE_BOTTOM = pixelY; 00838 } 00839 } 00840 00841 if(PointCount < 2) 00842 { 00843 return; 00844 } 00845 00846 X_center = (IMAGE_LEFT + IMAGE_RIGHT)/2; 00847 Y_center = (IMAGE_BOTTOM + IMAGE_TOP)/2; 00848 00849 X_first = Points->X; 00850 Y_first = Points->Y; 00851 00852 while(--PointCount) 00853 { 00854 X = Points->X; 00855 Y = Points->Y; 00856 Points++; 00857 X2 = Points->X; 00858 Y2 = Points->Y; 00859 00860 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 00861 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 00862 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 00863 } 00864 00865 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 00866 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 00867 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 00868 } 00869 00870 /** 00871 * @brief Draws a full ellipse. 00872 * @param Xpos: X position 00873 * @param Ypos: Y position 00874 * @param XRadius: Ellipse X radius 00875 * @param YRadius: Ellipse Y radius 00876 */ 00877 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00878 { 00879 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00880 float k = 0, rad1 = 0, rad2 = 0; 00881 00882 rad1 = XRadius; 00883 rad2 = YRadius; 00884 00885 k = (float)(rad2/rad1); 00886 00887 do 00888 { 00889 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos+y), (2*(uint16_t)(x/k) + 1)); 00890 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos-y), (2*(uint16_t)(x/k) + 1)); 00891 00892 e2 = err; 00893 if (e2 <= x) 00894 { 00895 err += ++x*2+1; 00896 if (-y == x && e2 <= y) e2 = 0; 00897 } 00898 if (e2 > y) err += ++y*2+1; 00899 } 00900 while (y <= 0); 00901 } 00902 00903 /** 00904 * @brief Enables the display. 00905 */ 00906 void BSP_LCD_DisplayOn(void) 00907 { 00908 LcdDrv->DisplayOn(); 00909 } 00910 00911 /** 00912 * @brief Disables the display. 00913 */ 00914 void BSP_LCD_DisplayOff(void) 00915 { 00916 LcdDrv->DisplayOff(); 00917 } 00918 00919 00920 /** 00921 * @brief Initializes the LCD GPIO special pins MSP. 00922 */ 00923 __weak void BSP_LCD_MspInit(void) 00924 { 00925 GPIO_InitTypeDef gpio_init_structure; 00926 00927 /* Enable GPIOs clock */ 00928 LCD_RESET_GPIO_CLK_ENABLE(); 00929 LCD_TE_GPIO_CLK_ENABLE(); 00930 LCD_BL_CTRL_GPIO_CLK_ENABLE(); 00931 00932 /* LCD_RESET GPIO configuration */ 00933 gpio_init_structure.Pin = LCD_RESET_PIN; /* LCD_RESET pin has to be manually controlled */ 00934 gpio_init_structure.Pull = GPIO_NOPULL; 00935 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00936 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00937 HAL_GPIO_Init(LCD_RESET_GPIO_PORT, &gpio_init_structure); 00938 00939 /* LCD_TE GPIO configuration */ 00940 gpio_init_structure.Pin = LCD_TE_PIN; /* LCD_TE pin has to be manually managed */ 00941 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00942 HAL_GPIO_Init(LCD_TE_GPIO_PORT, &gpio_init_structure); 00943 00944 /* LCD_BL_CTRL GPIO configuration */ 00945 gpio_init_structure.Pin = LCD_BL_CTRL_PIN; /* LCD_BL_CTRL pin has to be manually controlled */ 00946 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00947 HAL_GPIO_Init(LCD_BL_CTRL_GPIO_PORT, &gpio_init_structure); 00948 } 00949 00950 /** 00951 * @brief DeInitializes LCD GPIO special pins MSP. 00952 */ 00953 __weak void BSP_LCD_MspDeInit(void) 00954 { 00955 GPIO_InitTypeDef gpio_init_structure; 00956 00957 /* LCD_RESET GPIO deactivation */ 00958 gpio_init_structure.Pin = LCD_RESET_PIN; 00959 HAL_GPIO_DeInit(LCD_RESET_GPIO_PORT, gpio_init_structure.Pin); 00960 00961 /* LCD_TE GPIO deactivation */ 00962 gpio_init_structure.Pin = LCD_TE_PIN; 00963 HAL_GPIO_DeInit(LCD_TE_GPIO_PORT, gpio_init_structure.Pin); 00964 00965 /* LCD_BL_CTRL GPIO deactivation */ 00966 gpio_init_structure.Pin = LCD_BL_CTRL_PIN; 00967 HAL_GPIO_DeInit(LCD_BL_CTRL_GPIO_PORT, gpio_init_structure.Pin); 00968 00969 /* GPIO pins clock can be shut down in the application 00970 by surcharging this __weak function */ 00971 } 00972 00973 /****************************************************************************** 00974 Static Functions 00975 *******************************************************************************/ 00976 00977 /** 00978 * @brief Draws a character on LCD. 00979 * @param Xpos: Line where to display the character shape 00980 * @param Ypos: Start column address 00981 * @param c: Pointer to the character data 00982 */ 00983 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 00984 { 00985 uint32_t i = 0, j = 0; 00986 uint16_t height, width; 00987 uint8_t offset; 00988 uint8_t *pchar; 00989 uint32_t line; 00990 00991 height = DrawProp.pFont->Height; 00992 width = DrawProp.pFont->Width; 00993 00994 offset = 8 *((width + 7)/8) - width ; 00995 00996 for(i = 0; i < height; i++) 00997 { 00998 pchar = ((uint8_t *)c + (width + 7)/8 * i); 00999 01000 switch(((width + 7)/8)) 01001 { 01002 case 1: 01003 line = pchar[0]; 01004 break; 01005 01006 case 2: 01007 line = (pchar[0]<< 8) | pchar[1]; 01008 break; 01009 01010 case 3: 01011 default: 01012 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01013 break; 01014 } 01015 01016 for (j = 0; j < width; j++) 01017 { 01018 if(line & (1 << (width- j + offset- 1))) 01019 { 01020 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp.TextColor); 01021 } 01022 else 01023 { 01024 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp.BackColor); 01025 } 01026 } 01027 Ypos++; 01028 } 01029 } 01030 01031 /** 01032 * @brief Sets display window. 01033 * @param Xpos: LCD X position 01034 * @param Ypos: LCD Y position 01035 * @param Width: LCD window width 01036 * @param Height: LCD window height 01037 */ 01038 static void SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01039 { 01040 if(LcdDrv->SetDisplayWindow != NULL) 01041 { 01042 LcdDrv->SetDisplayWindow(Xpos, Ypos, Width, Height); 01043 } 01044 } 01045 01046 /** 01047 * @brief Fills a triangle (between 3 points). 01048 * @param x1: Point 1 X position 01049 * @param y1: Point 1 Y position 01050 * @param x2: Point 2 X position 01051 * @param y2: Point 2 Y position 01052 * @param x3: Point 3 X position 01053 * @param y3: Point 3 Y position 01054 */ 01055 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01056 { 01057 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01058 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 01059 curpixel = 0; 01060 01061 deltax = ABS(x2 - x1); /* The difference between the x's */ 01062 deltay = ABS(y2 - y1); /* The difference between the y's */ 01063 x = x1; /* Start x off at the first pixel */ 01064 y = y1; /* Start y off at the first pixel */ 01065 01066 if (x2 >= x1) /* The x-values are increasing */ 01067 { 01068 xinc1 = 1; 01069 xinc2 = 1; 01070 } 01071 else /* The x-values are decreasing */ 01072 { 01073 xinc1 = -1; 01074 xinc2 = -1; 01075 } 01076 01077 if (y2 >= y1) /* The y-values are increasing */ 01078 { 01079 yinc1 = 1; 01080 yinc2 = 1; 01081 } 01082 else /* The y-values are decreasing */ 01083 { 01084 yinc1 = -1; 01085 yinc2 = -1; 01086 } 01087 01088 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01089 { 01090 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01091 yinc2 = 0; /* Don't change the y for every iteration */ 01092 den = deltax; 01093 num = deltax / 2; 01094 numadd = deltay; 01095 numpixels = deltax; /* There are more x-values than y-values */ 01096 } 01097 else /* There is at least one y-value for every x-value */ 01098 { 01099 xinc2 = 0; /* Don't change the x for every iteration */ 01100 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01101 den = deltay; 01102 num = deltay / 2; 01103 numadd = deltax; 01104 numpixels = deltay; /* There are more y-values than x-values */ 01105 } 01106 01107 for (curpixel = 0; curpixel <= numpixels; curpixel++) 01108 { 01109 BSP_LCD_DrawLine(x, y, x3, y3); 01110 01111 num += numadd; /* Increase the numerator by the top of the fraction */ 01112 if (num >= den) /* Check if numerator >= denominator */ 01113 { 01114 num -= den; /* Calculate the new numerator value */ 01115 x += xinc1; /* Change the x as appropriate */ 01116 y += yinc1; /* Change the y as appropriate */ 01117 } 01118 x += xinc2; /* Change the x as appropriate */ 01119 y += yinc2; /* Change the y as appropriate */ 01120 } 01121 } 01122 01123 /** 01124 * @} 01125 */ 01126 01127 /** 01128 * @} 01129 */ 01130 01131 /** 01132 * @} 01133 */ 01134 01135 /** 01136 * @} 01137 */ 01138 01139 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 24 2017 11:28:05 for STM32412G-Discovery BSP User Manual by
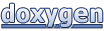