STM32412G-Discovery BSP User Manual
|
stm32412g_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32412g_discovery.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 27-January-2017 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM32412G-DISCOVERY board 00009 * (MB1209) from STMicroelectronics. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "stm32412g_discovery.h" 00042 00043 00044 /** @addtogroup BSP 00045 * @{ 00046 */ 00047 00048 /** @addtogroup STM32412G_DISCOVERY 00049 * @{ 00050 */ 00051 00052 /** @defgroup STM32412G_DISCOVERY_LOW_LEVEL STM32412G-DISCOVERY LOW LEVEL 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32412G_DISCOVERY_LOW_LEVEL_Private_TypesDefinitions STM32412G Discovery Low Level Private Typedef 00057 * @{ 00058 */ 00059 typedef struct 00060 { 00061 __IO uint16_t REG; 00062 __IO uint16_t RAM; 00063 }LCD_CONTROLLER_TypeDef; 00064 /** 00065 * @} 00066 */ 00067 00068 /** @defgroup STM32412G_DISCOVERY_LOW_LEVEL_Private_Defines STM32412G Discovery Low Level Private Def 00069 * @{ 00070 */ 00071 /** 00072 * @brief STM32412G DISCOVERY BSP Driver version number V2.0.0 00073 */ 00074 #define __STM32412G_DISCOVERY_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00075 #define __STM32412G_DISCOVERY_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00076 #define __STM32412G_DISCOVERY_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00077 #define __STM32412G_DISCOVERY_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00078 #define __STM32412G_DISCOVERY_BSP_VERSION ((__STM32412G_DISCOVERY_BSP_VERSION_MAIN << 24)\ 00079 |(__STM32412G_DISCOVERY_BSP_VERSION_SUB1 << 16)\ 00080 |(__STM32412G_DISCOVERY_BSP_VERSION_SUB2 << 8 )\ 00081 |(__STM32412G_DISCOVERY_BSP_VERSION_RC)) 00082 00083 /* We use BANK1 as we use FMC_NE1 signal */ 00084 #define FMC_BANK1_BASE ((uint32_t)(0x60000000 | 0x00000000)) 00085 #define FMC_BANK3_BASE ((uint32_t)(0x60000000 | 0x08000000)) 00086 #define FMC_BANK1 ((LCD_CONTROLLER_TypeDef *) FMC_BANK1_BASE) 00087 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup STM32412G_DISCOVERY_LOW_LEVEL_Private_Variables STM32412G Discovery Low Level Variables 00093 * @{ 00094 */ 00095 00096 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00097 LED2_PIN, 00098 LED3_PIN, 00099 LED4_PIN}; 00100 00101 00102 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT}; 00103 00104 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN}; 00105 00106 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn}; 00107 00108 GPIO_TypeDef* JOY_PORT[JOYn] = {SEL_JOY_GPIO_PORT, 00109 DOWN_JOY_GPIO_PORT, 00110 LEFT_JOY_GPIO_PORT, 00111 RIGHT_JOY_GPIO_PORT, 00112 UP_JOY_GPIO_PORT}; 00113 00114 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00115 DOWN_JOY_PIN, 00116 LEFT_JOY_PIN, 00117 RIGHT_JOY_PIN, 00118 UP_JOY_PIN}; 00119 00120 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00121 DOWN_JOY_EXTI_IRQn, 00122 LEFT_JOY_EXTI_IRQn, 00123 RIGHT_JOY_EXTI_IRQn, 00124 UP_JOY_EXTI_IRQn}; 00125 00126 USART_TypeDef* COM_USART[COMn] = {DISCOVERY_COM1}; 00127 00128 GPIO_TypeDef* COM_TX_PORT[COMn] = {DISCOVERY_COM1_TX_GPIO_PORT}; 00129 00130 GPIO_TypeDef* COM_RX_PORT[COMn] = {DISCOVERY_COM1_RX_GPIO_PORT}; 00131 00132 const uint16_t COM_TX_PIN[COMn] = {DISCOVERY_COM1_TX_PIN}; 00133 00134 const uint16_t COM_RX_PIN[COMn] = {DISCOVERY_COM1_RX_PIN}; 00135 00136 const uint16_t COM_TX_AF[COMn] = {DISCOVERY_COM1_TX_AF}; 00137 00138 const uint16_t COM_RX_AF[COMn] = {DISCOVERY_COM1_RX_AF}; 00139 00140 static I2C_HandleTypeDef hI2cAudioHandler; 00141 static I2C_HandleTypeDef hI2cExtHandler; 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /** @defgroup STM32412G_DISCOVERY_LOW_LEVEL_Private_FunctionPrototypes STM32412G Discovery Low Level Private Prototypes 00148 * @{ 00149 */ 00150 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler); 00151 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler); 00152 00153 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00154 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00155 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials); 00156 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr); 00157 00158 static void FMC_BANK1_WriteData(uint16_t Data); 00159 static void FMC_BANK1_WriteReg(uint8_t Reg); 00160 static uint16_t FMC_BANK1_ReadData(void); 00161 static void FMC_BANK1_Init(void); 00162 static void FMC_BANK1_MspInit(void); 00163 00164 /* LCD IO functions */ 00165 void LCD_IO_Init(void); 00166 void LCD_IO_WriteData(uint16_t RegValue); 00167 void LCD_IO_WriteReg(uint8_t Reg); 00168 void LCD_IO_WriteMultipleData(uint16_t *pData, uint32_t Size); 00169 uint16_t LCD_IO_ReadData(void); 00170 void LCD_IO_Delay(uint32_t Delay); 00171 00172 /* AUDIO IO functions */ 00173 void AUDIO_IO_Init(void); 00174 void AUDIO_IO_DeInit(void); 00175 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00176 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00177 void AUDIO_IO_Delay(uint32_t Delay); 00178 00179 /* I2C EEPROM IO function */ 00180 void EEPROM_IO_Init(void); 00181 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00182 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00183 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00184 00185 /* TouchScreen (TS) IO functions */ 00186 void TS_IO_Init(void); 00187 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00188 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00189 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00190 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00191 void TS_IO_Delay(uint32_t Delay); 00192 00193 /** 00194 * @} 00195 */ 00196 00197 /** @defgroup STM32412G_DISCOVERY_LOW_LEVEL_Private_Functions STM32412G Discovery Low Level Private Functions 00198 * @{ 00199 */ 00200 00201 /** 00202 * @brief This method returns the STM32412G DISCOVERY BSP Driver revision 00203 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00204 */ 00205 uint32_t BSP_GetVersion(void) 00206 { 00207 return __STM32412G_DISCOVERY_BSP_VERSION; 00208 } 00209 00210 /** 00211 * @brief Configures LEDs. 00212 * @param Led: LED to be configured. 00213 * This parameter can be one of the following values: 00214 * @arg LED1 00215 * @arg LED2 00216 * @arg LED3 00217 * @arg LED4 00218 */ 00219 void BSP_LED_Init(Led_TypeDef Led) 00220 { 00221 GPIO_InitTypeDef gpio_init_structure; 00222 00223 /* Enable the GPIO_LED clock */ 00224 LEDx_GPIO_CLK_ENABLE(); 00225 00226 /* Configure the GPIO_LED pin */ 00227 gpio_init_structure.Pin = GPIO_PIN[Led]; 00228 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00229 gpio_init_structure.Pull = GPIO_PULLUP; 00230 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00231 00232 HAL_GPIO_Init(LEDx_GPIO_PORT, &gpio_init_structure); 00233 00234 /* By default, turn off LED */ 00235 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_SET); 00236 } 00237 00238 00239 /** 00240 * @brief DeInit LEDs. 00241 * @param Led: LED to be configured. 00242 * This parameter can be one of the following values: 00243 * @arg LED1 00244 * @arg LED2 00245 * @arg LED3 00246 * @arg LED4 00247 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00248 */ 00249 void BSP_LED_DeInit(Led_TypeDef Led) 00250 { 00251 GPIO_InitTypeDef gpio_init_structure; 00252 00253 /* Turn off LED */ 00254 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_RESET); 00255 /* DeInit the GPIO_LED pin */ 00256 gpio_init_structure.Pin = GPIO_PIN[Led]; 00257 HAL_GPIO_DeInit(LEDx_GPIO_PORT, gpio_init_structure.Pin); 00258 } 00259 00260 /** 00261 * @brief Turns selected LED On. 00262 * @param Led: LED to be set on 00263 * This parameter can be one of the following values: 00264 * @arg LED1 00265 * @arg LED2 00266 * @arg LED3 00267 * @arg LED4 00268 */ 00269 void BSP_LED_On(Led_TypeDef Led) 00270 { 00271 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_RESET); 00272 } 00273 00274 /** 00275 * @brief Turns selected LED Off. 00276 * @param Led: LED to be set off 00277 * This parameter can be one of the following values: 00278 * @arg LED1 00279 * @arg LED2 00280 * @arg LED3 00281 * @arg LED4 00282 */ 00283 void BSP_LED_Off(Led_TypeDef Led) 00284 { 00285 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_SET); 00286 } 00287 00288 /** 00289 * @brief Toggles the selected LED. 00290 * @param Led: LED to be toggled 00291 * This parameter can be one of the following values: 00292 * @arg LED1 00293 * @arg LED2 00294 * @arg LED3 00295 * @arg LED4 00296 */ 00297 void BSP_LED_Toggle(Led_TypeDef Led) 00298 { 00299 HAL_GPIO_TogglePin(LEDx_GPIO_PORT, GPIO_PIN[Led]); 00300 } 00301 00302 /** 00303 * @brief Configures button GPIO and EXTI Line. 00304 * @param Button: Button to be configured 00305 * This parameter can be one of the following values: 00306 * @arg BUTTON_WAKEUP: Wakeup Push Button 00307 * @param ButtonMode: Button mode 00308 * This parameter can be one of the following values: 00309 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00310 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00311 * with interrupt generation capability 00312 * @note On STM32412G-DISCOVERY board, the Wakeup button is mapped on 00313 * the same push button which is the joystick selection button. 00314 */ 00315 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00316 { 00317 GPIO_InitTypeDef gpio_init_structure; 00318 00319 /* Enable the BUTTON clock */ 00320 WAKEUP_BUTTON_GPIO_CLK_ENABLE(); 00321 00322 if(ButtonMode == BUTTON_MODE_GPIO) 00323 { 00324 /* Configure Button pin as input */ 00325 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00326 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00327 gpio_init_structure.Pull = GPIO_PULLDOWN; 00328 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00329 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00330 } 00331 00332 if(ButtonMode == BUTTON_MODE_EXTI) 00333 { 00334 /* Configure Button pin as input with External interrupt */ 00335 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00336 gpio_init_structure.Pull = GPIO_PULLDOWN; 00337 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00338 00339 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00340 00341 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00342 00343 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00344 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00345 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00346 } 00347 } 00348 00349 /** 00350 * @brief Push Button DeInit. 00351 * @param Button: Button to be configured 00352 * This parameter can be one of the following values: 00353 * @arg BUTTON_WAKEUP: Wakeup Push Button 00354 * @note On STM32412G-DISCOVERY board, the Wakeup button is mapped on 00355 * the same push button which is the joystick selection button. 00356 * @note PB DeInit does not disable the GPIO clock 00357 */ 00358 void BSP_PB_DeInit(Button_TypeDef Button) 00359 { 00360 GPIO_InitTypeDef gpio_init_structure; 00361 00362 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00363 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00364 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00365 } 00366 00367 00368 /** 00369 * @brief Returns the selected button state. 00370 * @param Button: Button to be checked 00371 * This parameter can be one of the following values: 00372 * @arg BUTTON_WAKEUP: Wakeup Push Button 00373 * @note On STM32412G-DISCOVERY board, the Wakeup button is mapped on 00374 * the same push button which is the joystick selection button. 00375 * @retval The Button GPIO pin value (GPIO_PIN_RESET = button pressed) 00376 */ 00377 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00378 { 00379 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00380 } 00381 00382 00383 /** 00384 * @brief Configures all joystick's buttons in GPIO or EXTI modes. 00385 * @param Joy_Mode: Joystick mode. 00386 * This parameter can be one of the following values: 00387 * JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00388 * JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00389 * with interrupt generation capability 00390 * @retval HAL_OK: if all initializations are OK. Other value if error. 00391 */ 00392 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00393 { 00394 JOYState_TypeDef joykey; 00395 GPIO_InitTypeDef GPIO_InitStruct; 00396 00397 /* Initialized the Joystick. */ 00398 for(joykey = JOY_SEL; joykey < (JOY_SEL + JOYn) ; joykey++) 00399 { 00400 /* Enable the JOY clock */ 00401 JOYx_GPIO_CLK_ENABLE(joykey); 00402 00403 GPIO_InitStruct.Pin = JOY_PIN[joykey]; 00404 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00405 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00406 00407 if (Joy_Mode == JOY_MODE_GPIO) 00408 { 00409 /* Configure Joy pin as input */ 00410 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00411 HAL_GPIO_Init(JOY_PORT[joykey], &GPIO_InitStruct); 00412 } 00413 else if (Joy_Mode == JOY_MODE_EXTI) 00414 { 00415 /* Configure Joy pin as input with External interrupt */ 00416 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00417 HAL_GPIO_Init(JOY_PORT[joykey], &GPIO_InitStruct); 00418 00419 /* Enable and set Joy EXTI Interrupt to the lowest priority */ 00420 HAL_NVIC_SetPriority((IRQn_Type)(JOY_IRQn[joykey]), 0x0F, 0x00); 00421 HAL_NVIC_EnableIRQ((IRQn_Type)(JOY_IRQn[joykey])); 00422 } 00423 } 00424 00425 return HAL_OK; 00426 } 00427 00428 /** 00429 * @brief Unconfigures all GPIOs used as joystick's buttons. 00430 */ 00431 void BSP_JOY_DeInit(void) 00432 { 00433 JOYState_TypeDef joykey; 00434 00435 /* Initialized the Joystick. */ 00436 for(joykey = JOY_SEL; joykey < (JOY_SEL + JOYn) ; joykey++) 00437 { 00438 /* Enable the JOY clock */ 00439 JOYx_GPIO_CLK_ENABLE(joykey); 00440 00441 HAL_GPIO_DeInit(JOY_PORT[joykey], JOY_PIN[joykey]); 00442 } 00443 } 00444 00445 /** 00446 * @brief Returns the current joystick status. 00447 * @retval Code of the joystick key pressed 00448 * This code can be one of the following values: 00449 * @arg JOY_NONE 00450 * @arg JOY_SEL 00451 * @arg JOY_DOWN 00452 * @arg JOY_LEFT 00453 * @arg JOY_RIGHT 00454 * @arg JOY_UP 00455 */ 00456 JOYState_TypeDef BSP_JOY_GetState(void) 00457 { 00458 JOYState_TypeDef joykey; 00459 00460 for (joykey = JOY_SEL; joykey < (JOY_SEL + JOYn) ; joykey++) 00461 { 00462 if (HAL_GPIO_ReadPin(JOY_PORT[joykey], JOY_PIN[joykey]) != GPIO_PIN_RESET) 00463 { 00464 /* Return Code Joystick key pressed */ 00465 return joykey; 00466 } 00467 } 00468 00469 /* No Joystick key pressed */ 00470 return JOY_NONE; 00471 } 00472 00473 /** 00474 * @brief Configures COM port. 00475 * @param COM: COM port to be configured. 00476 * This parameter can be one of the following values: 00477 * COM1 00478 * COM2 00479 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00480 * configuration information for the specified USART peripheral. 00481 */ 00482 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00483 { 00484 GPIO_InitTypeDef gpio_init_structure; 00485 00486 /* Enable GPIO clock */ 00487 DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(COM); 00488 DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(COM); 00489 00490 /* Enable USART clock */ 00491 DISCOVERY_COMx_CLK_ENABLE(COM); 00492 00493 /* Configure USART Tx as alternate function */ 00494 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00495 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00496 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00497 gpio_init_structure.Pull = GPIO_PULLUP; 00498 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00499 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00500 00501 /* Configure USART Rx as alternate function */ 00502 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00503 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00504 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00505 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00506 00507 /* USART configuration */ 00508 huart->Instance = COM_USART[COM]; 00509 HAL_UART_Init(huart); 00510 } 00511 00512 /** 00513 * @brief DeInit COM port. 00514 * @param COM: COM port to be configured. 00515 * This parameter can be one of the following values: 00516 * COM1 00517 * COM2 00518 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00519 * configuration information for the specified USART peripheral. 00520 */ 00521 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00522 { 00523 /* USART configuration */ 00524 huart->Instance = COM_USART[COM]; 00525 HAL_UART_DeInit(huart); 00526 00527 /* Enable USART clock */ 00528 DISCOVERY_COMx_CLK_DISABLE(COM); 00529 00530 /* DeInit GPIO pins can be done in the application 00531 (by surcharging this __weak function) */ 00532 00533 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the application 00534 by surcharging this __weak function */ 00535 } 00536 00537 00538 /******************************************************************************* 00539 BUS OPERATIONS 00540 *******************************************************************************/ 00541 00542 /******************************* I2C Routines *********************************/ 00543 /** 00544 * @brief Initializes I2C MSP. 00545 * @param i2c_handler : I2C handler 00546 */ 00547 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler) 00548 { 00549 GPIO_InitTypeDef gpio_init_structure; 00550 00551 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00552 { 00553 /* AUDIO I2C MSP init */ 00554 00555 /*** Configure the GPIOs ***/ 00556 /* Enable GPIO clock */ 00557 DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00558 00559 /* Configure I2C Tx as alternate function */ 00560 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SCL_PIN; 00561 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00562 gpio_init_structure.Pull = GPIO_NOPULL; 00563 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00564 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SCL_SDA_AF; 00565 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00566 00567 /* Configure I2C Rx as alternate function */ 00568 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SDA_PIN; 00569 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00570 00571 /*** Configure the I2C peripheral ***/ 00572 /* Enable I2C clock */ 00573 DISCOVERY_AUDIO_I2Cx_CLK_ENABLE(); 00574 00575 /* Force the I2C peripheral clock reset */ 00576 DISCOVERY_AUDIO_I2Cx_FORCE_RESET(); 00577 00578 /* Release the I2C peripheral clock reset */ 00579 DISCOVERY_AUDIO_I2Cx_RELEASE_RESET(); 00580 00581 /* Enable and set I2Cx Interrupt to a lower priority */ 00582 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_EV_IRQn, 0x0F, 0x00); 00583 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_EV_IRQn); 00584 00585 /* Enable and set I2Cx Interrupt to a lower priority */ 00586 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_ER_IRQn, 0x0F, 0x00); 00587 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_ER_IRQn); 00588 } 00589 else 00590 { 00591 /* External and Arduino connector I2C MSP init */ 00592 00593 /*** Configure the GPIOs ***/ 00594 /* Enable GPIO clock */ 00595 DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00596 00597 /* Configure I2C Tx as alternate function */ 00598 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SCL_PIN; 00599 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00600 gpio_init_structure.Pull = GPIO_NOPULL; 00601 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00602 gpio_init_structure.Alternate = DISCOVERY_EXT_I2Cx_SCL_AF; 00603 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00604 00605 /* Configure I2C Rx as alternate function */ 00606 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SDA_PIN; 00607 gpio_init_structure.Alternate = DISCOVERY_EXT_I2Cx_SDA_AF; 00608 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00609 00610 /*** Configure the I2C peripheral ***/ 00611 /* Enable I2C clock */ 00612 DISCOVERY_EXT_I2Cx_CLK_ENABLE(); 00613 00614 /* Force the I2C peripheral clock reset */ 00615 DISCOVERY_EXT_I2Cx_FORCE_RESET(); 00616 00617 /* Release the I2C peripheral clock reset */ 00618 DISCOVERY_EXT_I2Cx_RELEASE_RESET(); 00619 00620 /* Enable and set I2Cx Interrupt to a lower priority */ 00621 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_EV_IRQn, 0x0F, 0x00); 00622 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_EV_IRQn); 00623 00624 /* Enable and set I2Cx Interrupt to a lower priority */ 00625 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_ER_IRQn, 0x0F, 0x00); 00626 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_ER_IRQn); 00627 } 00628 } 00629 00630 00631 /** 00632 * @brief Initializes I2C HAL. 00633 * @param i2c_handler : I2C handler 00634 */ 00635 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler) 00636 { 00637 if(HAL_I2C_GetState(i2c_handler) == HAL_I2C_STATE_RESET) 00638 { 00639 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00640 { 00641 /* Audio and LCD I2C configuration */ 00642 i2c_handler->Instance = DISCOVERY_AUDIO_I2Cx; 00643 } 00644 else 00645 { 00646 /* External, EEPROM and Arduino connector I2C configuration */ 00647 i2c_handler->Instance = DISCOVERY_EXT_I2Cx; 00648 } 00649 i2c_handler->Init.ClockSpeed = DISCOVERY_I2C_SPEED; 00650 i2c_handler->Init.DutyCycle = I2C_DUTYCYCLE_2; 00651 i2c_handler->Init.OwnAddress1 = 0; 00652 i2c_handler->Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00653 i2c_handler->Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00654 i2c_handler->Init.OwnAddress2 = 0; 00655 i2c_handler->Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00656 i2c_handler->Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00657 00658 /* Init the I2C */ 00659 I2Cx_MspInit(i2c_handler); 00660 HAL_I2C_Init(i2c_handler); 00661 } 00662 } 00663 00664 /** 00665 * @brief Reads multiple data. 00666 * @param i2c_handler : I2C handler 00667 * @param Addr: I2C address 00668 * @param Reg: Reg address 00669 * @param MemAddress: Memory address 00670 * @param Buffer: Pointer to data buffer 00671 * @param Length: Length of the data 00672 * @retval HAL status 00673 */ 00674 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, 00675 uint8_t Addr, 00676 uint16_t Reg, 00677 uint16_t MemAddress, 00678 uint8_t *Buffer, 00679 uint16_t Length) 00680 { 00681 HAL_StatusTypeDef status = HAL_OK; 00682 00683 status = HAL_I2C_Mem_Read(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00684 00685 /* Check the communication status */ 00686 if(status != HAL_OK) 00687 { 00688 /* I2C error occurred */ 00689 I2Cx_Error(i2c_handler, Addr); 00690 } 00691 return status; 00692 } 00693 00694 /** 00695 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00696 * @param i2c_handler : I2C handler 00697 * @param Addr: Device address on BUS Bus. 00698 * @param Reg: The target register address to write 00699 * @param MemAddress: Memory address 00700 * @param Buffer: The target register value to be written 00701 * @param Length: buffer size to be written 00702 * @retval HAL status 00703 */ 00704 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, 00705 uint8_t Addr, 00706 uint16_t Reg, 00707 uint16_t MemAddress, 00708 uint8_t *Buffer, 00709 uint16_t Length) 00710 { 00711 HAL_StatusTypeDef status = HAL_OK; 00712 00713 status = HAL_I2C_Mem_Write(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00714 00715 /* Check the communication status */ 00716 if(status != HAL_OK) 00717 { 00718 /* Re-Initialize the I2C Bus */ 00719 I2Cx_Error(i2c_handler, Addr); 00720 } 00721 return status; 00722 } 00723 00724 /** 00725 * @brief Checks if target device is ready for communication. 00726 * @note This function is used with Memory devices 00727 * @param i2c_handler : I2C handler 00728 * @param DevAddress: Target device address 00729 * @param Trials: Number of trials 00730 * @retval HAL status 00731 */ 00732 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials) 00733 { 00734 return (HAL_I2C_IsDeviceReady(i2c_handler, DevAddress, Trials, 1000)); 00735 } 00736 00737 /** 00738 * @brief Manages error callback by re-initializing I2C. 00739 * @param i2c_handler : I2C handler 00740 * @param Addr: I2C Address 00741 */ 00742 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr) 00743 { 00744 /* De-initialize the I2C communication bus */ 00745 HAL_I2C_DeInit(i2c_handler); 00746 00747 /* Re-Initialize the I2C communication bus */ 00748 I2Cx_Init(i2c_handler); 00749 } 00750 00751 /** 00752 * @brief Deinitializes I2C interface 00753 * @param i2c_handler : I2C handler 00754 */ 00755 static void I2Cx_DeInit(I2C_HandleTypeDef *i2c_handler) 00756 { 00757 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00758 { 00759 /* Audio and LCD I2C configuration */ 00760 i2c_handler->Instance = DISCOVERY_AUDIO_I2Cx; 00761 } 00762 else 00763 { 00764 /* External, EEPROM and Arduino connector I2C configuration */ 00765 i2c_handler->Instance = DISCOVERY_EXT_I2Cx; 00766 } 00767 00768 /* Disable I2C block */ 00769 __HAL_I2C_DISABLE(i2c_handler); 00770 00771 /* DeInit the I2S */ 00772 HAL_I2C_DeInit(i2c_handler); 00773 } 00774 00775 /*************************** FMC Routines ************************************/ 00776 /** 00777 * @brief Initializes FMC_BANK1 MSP. 00778 */ 00779 static void FMC_BANK1_MspInit(void) 00780 { 00781 GPIO_InitTypeDef gpio_init_structure; 00782 00783 /* Enable FSMC clock */ 00784 __HAL_RCC_FSMC_CLK_ENABLE(); 00785 00786 /* Enable GPIOs clock */ 00787 __HAL_RCC_GPIOD_CLK_ENABLE(); 00788 __HAL_RCC_GPIOE_CLK_ENABLE(); 00789 __HAL_RCC_GPIOF_CLK_ENABLE(); 00790 00791 /* Common GPIO configuration */ 00792 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00793 gpio_init_structure.Pull = GPIO_PULLUP; 00794 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00795 gpio_init_structure.Alternate = GPIO_AF12_FSMC; 00796 00797 /* GPIOD configuration */ /* GPIO_PIN_7 is FMC_NE1 */ 00798 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_8 |\ 00799 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_14 | GPIO_PIN_15 | GPIO_PIN_7; 00800 00801 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00802 00803 /* GPIOE configuration */ 00804 gpio_init_structure.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00805 GPIO_PIN_12 |GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00806 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00807 00808 /* GPIOF configuration */ 00809 gpio_init_structure.Pin = GPIO_PIN_0 ; 00810 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00811 } 00812 00813 00814 /** 00815 * @brief Initializes LCD IO. 00816 */ 00817 static void FMC_BANK1_Init(void) 00818 { 00819 SRAM_HandleTypeDef hsram; 00820 FMC_NORSRAM_TimingTypeDef sram_timing; 00821 FMC_NORSRAM_TimingTypeDef sram_timing_write; 00822 00823 /*** Configure the SRAM Bank 1 ***/ 00824 /* Configure IPs */ 00825 hsram.Instance = FSMC_NORSRAM_DEVICE; 00826 hsram.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00827 00828 /* Timing for READING */ 00829 sram_timing.AddressSetupTime = 9; 00830 sram_timing.AddressHoldTime = 1; 00831 sram_timing.DataSetupTime = 36; 00832 sram_timing.BusTurnAroundDuration = 1; 00833 sram_timing.CLKDivision = 2; 00834 sram_timing.DataLatency = 2; 00835 sram_timing.AccessMode = FSMC_ACCESS_MODE_A; 00836 00837 /* Timing for WRITTING*/ 00838 sram_timing_write.AddressSetupTime = 1; 00839 sram_timing_write.AddressHoldTime = 1; 00840 sram_timing_write.DataSetupTime = 7; 00841 sram_timing_write.BusTurnAroundDuration = 0; 00842 sram_timing_write.CLKDivision = 2; 00843 sram_timing_write.DataLatency = 2; 00844 sram_timing_write.AccessMode = FSMC_ACCESS_MODE_A; 00845 00846 hsram.Init.NSBank = FSMC_NORSRAM_BANK1; 00847 hsram.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00848 hsram.Init.MemoryType = FSMC_MEMORY_TYPE_SRAM; 00849 hsram.Init.MemoryDataWidth = FSMC_NORSRAM_MEM_BUS_WIDTH_16; 00850 hsram.Init.BurstAccessMode = FSMC_BURST_ACCESS_MODE_DISABLE; 00851 hsram.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00852 hsram.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00853 hsram.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00854 hsram.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00855 hsram.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00856 hsram.Init.ExtendedMode = FSMC_EXTENDED_MODE_ENABLE; 00857 hsram.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00858 hsram.Init.WriteBurst = FSMC_WRITE_BURST_DISABLE; 00859 hsram.Init.WriteFifo = FSMC_WRITE_FIFO_DISABLE; 00860 hsram.Init.PageSize = FSMC_PAGE_SIZE_NONE; 00861 hsram.Init.ContinuousClock = FSMC_CONTINUOUS_CLOCK_SYNC_ONLY; 00862 00863 /* Initialize the SRAM controller */ 00864 FMC_BANK1_MspInit(); 00865 HAL_SRAM_Init(&hsram, &sram_timing, &sram_timing_write); 00866 } 00867 00868 /** 00869 * @brief Writes register value. 00870 * @param Data: Data to be written 00871 */ 00872 static void FMC_BANK1_WriteData(uint16_t Data) 00873 { 00874 /* Write 16-bit Reg */ 00875 FMC_BANK1->RAM = Data; 00876 __DSB(); 00877 } 00878 00879 /** 00880 * @brief Writes register address. 00881 * @param Reg: Register to be written 00882 */ 00883 static void FMC_BANK1_WriteReg(uint8_t Reg) 00884 { 00885 /* Write 16-bit Index, then write register */ 00886 FMC_BANK1->REG = Reg; 00887 __DSB(); 00888 } 00889 00890 /** 00891 * @brief Reads register value. 00892 * @retval Read value 00893 */ 00894 static uint16_t FMC_BANK1_ReadData(void) 00895 { 00896 return FMC_BANK1->RAM; 00897 } 00898 00899 /******************************************************************************* 00900 LINK OPERATIONS 00901 *******************************************************************************/ 00902 00903 /********************************* LINK LCD ***********************************/ 00904 00905 /** 00906 * @brief Initializes LCD low level. 00907 */ 00908 void LCD_IO_Init(void) 00909 { 00910 FMC_BANK1_Init(); 00911 } 00912 00913 /** 00914 * @brief Writes data on LCD data register. 00915 * @param RegValue: Data to be written 00916 */ 00917 void LCD_IO_WriteData(uint16_t RegValue) 00918 { 00919 /* Write 16-bit Reg */ 00920 FMC_BANK1_WriteData(RegValue); 00921 } 00922 00923 /** 00924 * @brief Writes several data on LCD data register. 00925 * @param pData: pointer on data to be written 00926 * @param Size: data amount in 16bits short unit 00927 */ 00928 void LCD_IO_WriteMultipleData(uint16_t *pData, uint32_t Size) 00929 { 00930 uint32_t i; 00931 00932 for (i = 0; i < Size; i++) 00933 { 00934 FMC_BANK1_WriteData(pData[i]); 00935 } 00936 } 00937 00938 /** 00939 * @brief Writes register on LCD register. 00940 * @param Reg: Register to be written 00941 */ 00942 void LCD_IO_WriteReg(uint8_t Reg) 00943 { 00944 /* Write 16-bit Index, then Write Reg */ 00945 FMC_BANK1_WriteReg(Reg); 00946 } 00947 00948 /** 00949 * @brief Reads data from LCD data register. 00950 * @retval Read data. 00951 */ 00952 uint16_t LCD_IO_ReadData(void) 00953 { 00954 return FMC_BANK1_ReadData(); 00955 } 00956 00957 /** 00958 * @brief LCD delay 00959 * @param Delay: Delay in ms 00960 */ 00961 void LCD_IO_Delay(uint32_t Delay) 00962 { 00963 HAL_Delay(Delay); 00964 } 00965 00966 /********************************* LINK AUDIO *********************************/ 00967 00968 /** 00969 * @brief Initializes Audio low level. 00970 */ 00971 void AUDIO_IO_Init(void) 00972 { 00973 I2Cx_Init(&hI2cAudioHandler); 00974 } 00975 00976 /** 00977 * @brief Deinitializes Audio low level. 00978 */ 00979 void AUDIO_IO_DeInit(void) 00980 { 00981 I2Cx_DeInit(&hI2cAudioHandler); 00982 } 00983 00984 /** 00985 * @brief Writes a single data. 00986 * @param Addr: I2C address 00987 * @param Reg: Reg address 00988 * @param Value: Data to be written 00989 */ 00990 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 00991 { 00992 uint16_t tmp = Value; 00993 00994 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 00995 00996 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 00997 00998 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 00999 } 01000 01001 /** 01002 * @brief Reads a single data. 01003 * @param Addr: I2C address 01004 * @param Reg: Reg address 01005 * @retval Data to be read 01006 */ 01007 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 01008 { 01009 uint16_t read_value = 0, tmp = 0; 01010 01011 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01012 01013 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01014 01015 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01016 01017 read_value = tmp; 01018 01019 return read_value; 01020 } 01021 01022 /** 01023 * @brief AUDIO Codec delay 01024 * @param Delay: Delay in ms 01025 */ 01026 void AUDIO_IO_Delay(uint32_t Delay) 01027 { 01028 HAL_Delay(Delay); 01029 } 01030 01031 /******************************** LINK I2C EEPROM *****************************/ 01032 01033 /** 01034 * @brief Initializes peripherals used by the I2C EEPROM driver. 01035 */ 01036 void EEPROM_IO_Init(void) 01037 { 01038 I2Cx_Init(&hI2cExtHandler); 01039 } 01040 01041 /** 01042 * @brief Write data to I2C EEPROM driver in using DMA channel. 01043 * @param DevAddress: Target device address 01044 * @param MemAddress: Internal memory address 01045 * @param pBuffer: Pointer to data buffer 01046 * @param BufferSize: Amount of data to be sent 01047 * @retval HAL status 01048 */ 01049 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01050 { 01051 return (I2Cx_WriteMultiple(&hI2cExtHandler, DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01052 } 01053 01054 /** 01055 * @brief Read data from I2C EEPROM driver in using DMA channel. 01056 * @param DevAddress: Target device address 01057 * @param MemAddress: Internal memory address 01058 * @param pBuffer: Pointer to data buffer 01059 * @param BufferSize: Amount of data to be read 01060 * @retval HAL status 01061 */ 01062 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01063 { 01064 return (I2Cx_ReadMultiple(&hI2cExtHandler, DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01065 } 01066 01067 /** 01068 * @brief Checks if target device is ready for communication. 01069 * @note This function is used with Memory devices 01070 * @param DevAddress: Target device address 01071 * @param Trials: Number of trials 01072 * @retval HAL status 01073 */ 01074 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01075 { 01076 return (I2Cx_IsDeviceReady(&hI2cExtHandler, DevAddress, Trials)); 01077 } 01078 01079 /************************** LINK TS (TouchScreen) *****************************/ 01080 /** 01081 * @brief Initializes Touchscreen low level. 01082 */ 01083 void TS_IO_Init(void) 01084 { 01085 I2Cx_Init(&hI2cAudioHandler); 01086 } 01087 01088 /** 01089 * @brief Writes a single data. 01090 * @param Addr: I2C address 01091 * @param Reg: Reg address 01092 * @param Value: Data to be written 01093 */ 01094 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01095 { 01096 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 01097 } 01098 01099 /** 01100 * @brief Reads a single data. 01101 * @param Addr: I2C address 01102 * @param Reg: Reg address 01103 * @retval Data to be read 01104 */ 01105 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 01106 { 01107 uint8_t read_value = 0; 01108 01109 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 01110 01111 return read_value; 01112 } 01113 01114 /** 01115 * @brief Reads multiple data with I2C communication 01116 * channel from TouchScreen. 01117 * @param Addr: I2C address 01118 * @param Reg: Register address 01119 * @param Buffer: Pointer to data buffer 01120 * @param Length: Length of the data 01121 * @retval HAL status 01122 */ 01123 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01124 { 01125 return I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01126 } 01127 01128 /** 01129 * @brief Writes multiple data with I2C communication 01130 * channel from MCU to TouchScreen. 01131 * @param Addr: I2C address 01132 * @param Reg: Register address 01133 * @param Buffer: Pointer to data buffer 01134 * @param Length: Length of the data 01135 */ 01136 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01137 { 01138 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01139 } 01140 01141 /** 01142 * @brief Delay function used in TouchScreen low level driver. 01143 * @param Delay: Delay in ms 01144 */ 01145 void TS_IO_Delay(uint32_t Delay) 01146 { 01147 HAL_Delay(Delay); 01148 } 01149 01150 /** 01151 * @} 01152 */ 01153 01154 /** 01155 * @} 01156 */ 01157 01158 /** 01159 * @} 01160 */ 01161 01162 /** 01163 * @} 01164 */ 01165 01166 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 24 2017 11:28:05 for STM32412G-Discovery BSP User Manual by
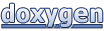