STM32373C_EVAL BSP User Manual
|
stm32373c_eval_audio.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32373c_eval_audio.h 00004 * @author MCD Application Team 00005 * @brief This file contains all the functions prototypes for the 00006 * stm32373c_eval_audio.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32373C_EVAL_AUDIO_H 00039 #define __STM32373C_EVAL_AUDIO_H 00040 00041 /* Includes ------------------------------------------------------------------*/ 00042 /* Include AUDIO component driver */ 00043 #include "../Components/cs43l22/cs43l22.h" 00044 #include "stm32373c_eval.h" 00045 00046 /** @addtogroup BSP 00047 * @{ 00048 */ 00049 00050 /** @addtogroup STM32373C_EVAL 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32373C_EVAL_AUDIO 00055 * @{ 00056 */ 00057 00058 /** @defgroup STM32373C_EVAL_AUDIO_Exported_Types Exported Types 00059 * @{ 00060 */ 00061 typedef enum 00062 { 00063 AUDIO_OK = 0x00, 00064 AUDIO_ERROR = 0x01, 00065 AUDIO_TIMEOUT = 0x02 00066 00067 }AUDIO_StatusTypeDef; 00068 00069 /** 00070 * @} 00071 */ 00072 00073 /** @defgroup STM32373C_EVAL_AUDIO_Exported_Constants Exported Constants 00074 * @{ 00075 */ 00076 00077 /* Audio Codec hardware I2C address */ 00078 #define AUDIO_I2C_ADDRESS 0x94 00079 00080 /*---------------------------------------------------------------------------- 00081 AUDIO OUT CONFIGURATION 00082 ----------------------------------------------------------------------------*/ 00083 00084 /* I2S peripheral configuration defines */ 00085 #define I2Sx SPI1 00086 #define I2Sx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00087 #define I2Sx_CLK_DISABLE() __HAL_RCC_SPI1_CLK_DISABLE() 00088 #define I2Sx_FORCE_RESET() __HAL_RCC_SPI1_FORCE_RESET() 00089 #define I2Sx_RELEASE_RESET() __HAL_RCC_SPI1_RELEASE_RESET() 00090 00091 #define I2Sx_WS_PIN GPIO_PIN_6 00092 #define I2Sx_SCK_PIN GPIO_PIN_7 00093 #define I2Sx_MCK_PIN GPIO_PIN_8 00094 #define I2Sx_SD_PIN GPIO_PIN_9 00095 00096 #define I2Sx_GPIO_PORT GPIOC 00097 #define I2Sx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00098 #define I2Sx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00099 #define I2Sx_AF GPIO_AF5_SPI1 00100 00101 /* I2S DMA Stream definitions */ 00102 #define I2Sx_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00103 #define I2Sx_DMAx_CLK_DISABLE() __HAL_RCC_DMA1_CLK_DISABLE() 00104 #define I2Sx_DMAx_CHANNEL DMA1_Channel3 00105 #define I2Sx_DMAx_IRQ DMA1_Channel3_IRQn 00106 #define I2Sx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00107 #define I2Sx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00108 #define DMA_MAX_SZE 0xFFFF 00109 00110 /* Select the interrupt preemption priority and subpriority for the DMA interrupt */ 00111 #define AUDIO_OUT_IRQ_PREPRIO 0x0E /* Select the preemption priority level(0 is the highest) */ 00112 #define AUDIO_OUT_IRQ_SUBPRIO 0 /* Select the sub-priority level (0 is the highest) */ 00113 /*----------------------------------------------------------------------------*/ 00114 00115 /*------------------------------------------------------------------------------ 00116 OPTIONAL Configuration defines parameters 00117 ------------------------------------------------------------------------------*/ 00118 #define AUDIODATA_SIZE 2 /* 16-bits audio data size */ 00119 00120 #define DMA_MAX(_X_) (((_X_) <= DMA_MAX_SZE)? (_X_):DMA_MAX_SZE) 00121 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM32373C_EVAL_AUDIO_Exported_Variables Exported Variables 00127 * @{ 00128 */ 00129 00130 /** 00131 * @} 00132 */ 00133 00134 /** @defgroup STM32373C_EVAL_AUDIO_Exported_Macros Exported Macros 00135 * @{ 00136 */ 00137 /** 00138 * @} 00139 */ 00140 00141 /* Exported functions --------------------------------------------------------*/ 00142 /** @defgroup STM32373C_EVAL_AUDIO_Exported_Functions Exported Functions 00143 * @{ 00144 */ 00145 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00146 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size); 00147 uint8_t BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00148 uint8_t BSP_AUDIO_OUT_Pause(void); 00149 uint8_t BSP_AUDIO_OUT_Resume(void); 00150 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option); 00151 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume); 00152 uint8_t BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq); 00153 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Command); 00154 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output); 00155 00156 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00157 /* This function is called when the requested data has been completely transferred.*/ 00158 void BSP_AUDIO_OUT_TransferComplete_CallBack(void); 00159 00160 /* This function is called when half of the requested buffer has been transferred. */ 00161 void BSP_AUDIO_OUT_HalfTransfer_CallBack(void); 00162 00163 /* This function is called when an Interrupt due to transfer error on or peripheral 00164 error occurs. */ 00165 void BSP_AUDIO_OUT_Error_CallBack(void); 00166 /** 00167 * @} 00168 */ 00169 00170 /** 00171 * @} 00172 */ 00173 00174 /** 00175 * @} 00176 */ 00177 00178 /** 00179 * @} 00180 */ 00181 00182 #ifdef __cplusplus 00183 } 00184 #endif 00185 00186 #endif /* __STM32373C_EVAL_AUDIO_H */ 00187 00188 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 11:20:44 for STM32373C_EVAL BSP User Manual by
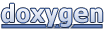