STM32373C_EVAL BSP User Manual
|
stm32373c_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32373c_eval.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage LEDs, 00006 * push-button and COM port available on STM32373C-EVAL evaluation 00007 * board (MB988) RevB from STMicroelectronics. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Includes ------------------------------------------------------------------*/ 00039 #include "stm32373c_eval.h" 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup STM32373C_EVAL 00046 * @brief This file provides firmware functions to manage Leds, push-buttons, 00047 * COM ports, SD card and temperature sensor available on 00048 * STM32373C-EVAL evaluation board from STMicroelectronics. 00049 * @{ 00050 */ 00051 00052 /** @addtogroup STM32373C_EVAL_Common 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM32373C_EVAL_Private_Constants 00057 * @{ 00058 */ 00059 /* LINK LCD */ 00060 #define START_BYTE 0x70 00061 #define SET_INDEX 0x00 00062 #define READ_STATUS 0x01 00063 #define LCD_WRITE_REG 0x02 00064 #define LCD_READ_REG 0x03 00065 00066 /* LINK SD Card */ 00067 #define SD_DUMMY_BYTE 0xFF 00068 #define SD_NO_RESPONSE_EXPECTED 0x80 00069 00070 /** 00071 * @brief STM32373C EVAL BSP Driver version number V2.2.1 00072 */ 00073 #define __STM32373C_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00074 #define __STM32373C_EVAL_BSP_VERSION_SUB1 (0x02) /*!< [23:16] sub1 version */ 00075 #define __STM32373C_EVAL_BSP_VERSION_SUB2 (0x01) /*!< [15:8] sub2 version */ 00076 #define __STM32373C_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00077 #define __STM32373C_EVAL_BSP_VERSION ((__STM32373C_EVAL_BSP_VERSION_MAIN << 24)\ 00078 |(__STM32373C_EVAL_BSP_VERSION_SUB1 << 16)\ 00079 |(__STM32373C_EVAL_BSP_VERSION_SUB2 << 8 )\ 00080 |(__STM32373C_EVAL_BSP_VERSION_RC)) 00081 /** 00082 * @} 00083 */ 00084 00085 /** @addtogroup STM32373C_EVAL_Private_Variables 00086 * @{ 00087 */ 00088 /** 00089 * @brief LED variables 00090 */ 00091 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00092 LED2_GPIO_PORT, 00093 LED3_GPIO_PORT, 00094 LED4_GPIO_PORT}; 00095 00096 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00097 LED2_PIN, 00098 LED3_PIN, 00099 LED4_PIN}; 00100 00101 /** 00102 * @brief BUTTON variables 00103 */ 00104 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {TAMPER_BUTTON_GPIO_PORT, 00105 KEY_BUTTON_GPIO_PORT, 00106 SEL_JOY_GPIO_PORT, 00107 LEFT_JOY_GPIO_PORT, 00108 RIGHT_JOY_GPIO_PORT, 00109 DOWN_JOY_GPIO_PORT, 00110 UP_JOY_GPIO_PORT}; 00111 00112 const uint16_t BUTTON_PIN[BUTTONn] = {TAMPER_BUTTON_PIN, 00113 KEY_BUTTON_PIN, 00114 SEL_JOY_PIN, 00115 LEFT_JOY_PIN, 00116 RIGHT_JOY_PIN, 00117 DOWN_JOY_PIN, 00118 UP_JOY_PIN}; 00119 00120 const uint16_t BUTTON_IRQn[BUTTONn] = {TAMPER_BUTTON_EXTI_IRQn, 00121 KEY_BUTTON_EXTI_IRQn, 00122 SEL_JOY_EXTI_IRQn, 00123 LEFT_JOY_EXTI_IRQn, 00124 RIGHT_JOY_EXTI_IRQn, 00125 DOWN_JOY_EXTI_IRQn, 00126 UP_JOY_EXTI_IRQn}; 00127 00128 /** 00129 * @brief JOYSTICK variables 00130 */ 00131 GPIO_TypeDef* JOY_PORT[JOYn] = {SEL_JOY_GPIO_PORT, 00132 LEFT_JOY_GPIO_PORT, 00133 RIGHT_JOY_GPIO_PORT, 00134 DOWN_JOY_GPIO_PORT, 00135 UP_JOY_GPIO_PORT}; 00136 00137 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00138 LEFT_JOY_PIN, 00139 RIGHT_JOY_PIN, 00140 DOWN_JOY_PIN, 00141 UP_JOY_PIN}; 00142 00143 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00144 LEFT_JOY_EXTI_IRQn, 00145 RIGHT_JOY_EXTI_IRQn, 00146 DOWN_JOY_EXTI_IRQn, 00147 UP_JOY_EXTI_IRQn}; 00148 00149 /** 00150 * @brief COM variables 00151 */ 00152 #if defined(HAL_UART_MODULE_ENABLED) 00153 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00154 00155 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00156 00157 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00158 00159 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00160 00161 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00162 00163 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00164 00165 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00166 #endif /* HAL_UART_MODULE_ENABLED) */ 00167 00168 /** 00169 * @brief BUS variables 00170 */ 00171 #if defined(HAL_I2C_MODULE_ENABLED) 00172 uint32_t I2c1Timeout = EVAL_I2C1_TIMEOUT_MAX; /*<! Value of Timeout when I2C1 communication fails */ 00173 uint32_t I2c2Timeout = EVAL_I2C2_TIMEOUT_MAX; /*<! Value of Timeout when I2C2 communication fails */ 00174 I2C_HandleTypeDef heval_I2c1; 00175 I2C_HandleTypeDef heval_I2c2; 00176 #endif /* HAL_I2C_MODULE_ENABLED */ 00177 00178 #if defined(HAL_SPI_MODULE_ENABLED) 00179 uint32_t SpixTimeout = EVAL_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00180 static SPI_HandleTypeDef heval_Spi; 00181 #endif /* HAL_SPI_MODULE_ENABLED */ 00182 00183 /** 00184 * @} 00185 */ 00186 00187 /** @defgroup STM32373C_EVAL_BUS Bus Operation functions 00188 * @{ 00189 */ 00190 #if defined(HAL_I2C_MODULE_ENABLED) 00191 /* I2Cx bus function */ 00192 static void I2C1_Init(void); 00193 static HAL_StatusTypeDef I2C1_TransmitData(uint8_t *pBuffer, uint16_t Length); 00194 static void I2C1_Error(void); 00195 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c); 00196 00197 static void I2C2_Init(void); 00198 static void I2C2_WriteData(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t Value); 00199 static HAL_StatusTypeDef I2C2_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00200 static uint8_t I2C2_ReadData(uint16_t Addr, uint8_t Reg, uint16_t RegSize); 00201 static HAL_StatusTypeDef I2C2_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00202 static HAL_StatusTypeDef I2C2_ReceiveData(uint16_t Addr, uint8_t * pBuffer, uint16_t Length); 00203 static HAL_StatusTypeDef I2C2_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00204 static void I2C2_Error(void); 00205 static void I2C2_MspInit(I2C_HandleTypeDef *hi2c); 00206 00207 /* Link functions for EEPROM peripheral */ 00208 void EEPROM_IO_Init(void); 00209 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00210 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00211 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00212 00213 /* Link functions for Temperature Sensor peripheral */ 00214 void TSENSOR_IO_Init(void); 00215 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length); 00216 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length); 00217 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00218 00219 /* Link functions for Audio Codec peripheral */ 00220 void AUDIO_IO_Init(void); 00221 void AUDIO_IO_DeInit(void); 00222 void AUDIO_IO_Write(uint16_t DevAddress, uint8_t Reg, uint8_t Value); 00223 uint8_t AUDIO_IO_Read(uint16_t DevAddress, uint8_t Reg); 00224 00225 /* Link functions for CEC peripheral */ 00226 void HDMI_CEC_IO_Init(void); 00227 HAL_StatusTypeDef HDMI_CEC_IO_WriteData(uint8_t * pBuffer, uint16_t BufferSize); 00228 HAL_StatusTypeDef HDMI_CEC_IO_ReadData(uint16_t DevAddress, uint8_t * pBuffer, uint16_t BufferSize); 00229 #endif /* HAL_I2C_MODULE_ENABLED */ 00230 00231 #if defined(HAL_SPI_MODULE_ENABLED) 00232 /* SPIx bus function */ 00233 static void SPIx_Init(void); 00234 static void SPIx_Write(uint8_t Value); 00235 static uint32_t SPIx_Read(void); 00236 static void SPIx_Error (void); 00237 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00238 00239 /* Link functions for LCD peripheral over SPI */ 00240 void LCD_IO_Init(void); 00241 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00242 void LCD_IO_WriteReg(uint8_t Reg); 00243 uint16_t LCD_IO_ReadData(uint16_t Reg); 00244 void LCD_Delay(uint32_t delay); 00245 00246 /* Link functions for SD Card peripheral over SPI */ 00247 void SD_IO_Init(void); 00248 HAL_StatusTypeDef SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response); 00249 HAL_StatusTypeDef SD_IO_WaitResponse(uint8_t Response); 00250 void SD_IO_WriteDummy(void); 00251 void SD_IO_WriteByte(uint8_t Data); 00252 uint8_t SD_IO_ReadByte(void); 00253 #endif /* HAL_SPI_MODULE_ENABLED */ 00254 00255 /** 00256 * @} 00257 */ 00258 00259 /** @addtogroup STM32373C_EVAL_Exported_Functions 00260 * @{ 00261 */ 00262 00263 /** 00264 * @brief This method returns the STM32373C EVAL BSP Driver revision 00265 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00266 */ 00267 uint32_t BSP_GetVersion(void) 00268 { 00269 return __STM32373C_EVAL_BSP_VERSION; 00270 } 00271 00272 /** 00273 * @brief Configures LED GPIO. 00274 * @param Led Specifies the Led to be configured. 00275 * This parameter can be one of following parameters: 00276 * @arg LED1 00277 * @arg LED2 00278 * @arg LED3 00279 * @arg LED4 00280 * @retval None 00281 */ 00282 void BSP_LED_Init(Led_TypeDef Led) 00283 { 00284 GPIO_InitTypeDef GPIO_InitStruct; 00285 00286 /* Enable the GPIO_LED clock */ 00287 LEDx_GPIO_CLK_ENABLE(Led); 00288 00289 /* Configure the GPIO_LED pin */ 00290 GPIO_InitStruct.Pin = LED_PIN[Led]; 00291 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00292 GPIO_InitStruct.Pull = GPIO_NOPULL; 00293 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00294 00295 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00296 00297 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00298 } 00299 00300 /** 00301 * @brief Turns selected LED On. 00302 * @param Led Specifies the Led to be set on. 00303 * This parameter can be one of following parameters: 00304 * @arg LED1 00305 * @arg LED2 00306 * @arg LED3 00307 * @arg LED4 00308 * @retval None 00309 */ 00310 void BSP_LED_On(Led_TypeDef Led) 00311 { 00312 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00313 } 00314 00315 /** 00316 * @brief Turns selected LED Off. 00317 * @param Led Specifies the Led to be set off. 00318 * This parameter can be one of following parameters: 00319 * @arg LED1 00320 * @arg LED2 00321 * @arg LED3 00322 * @arg LED4 00323 * @retval None 00324 */ 00325 void BSP_LED_Off(Led_TypeDef Led) 00326 { 00327 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00328 } 00329 00330 /** 00331 * @brief Toggles the selected LED. 00332 * @param Led Specifies the Led to be toggled. 00333 * This parameter can be one of following parameters: 00334 * @arg LED1 00335 * @arg LED2 00336 * @arg LED3 00337 * @arg LED4 00338 * @retval None 00339 */ 00340 void BSP_LED_Toggle(Led_TypeDef Led) 00341 { 00342 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00343 } 00344 00345 /** 00346 * @brief Configures push button GPIO and EXTI Line. 00347 * @param Button Button to be configured 00348 * This parameter can be one of the following values: 00349 * @arg BUTTON_TAMPER: Tamper Push Button 00350 * @arg BUTTON_KEY : Key Push Button 00351 * @param Mode Button mode 00352 * This parameter can be one of the following values: 00353 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00354 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00355 * with interrupt generation capability 00356 * @retval None 00357 */ 00358 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode) 00359 { 00360 GPIO_InitTypeDef GPIO_InitStruct; 00361 00362 /* Enable the corresponding Push Button clock */ 00363 BUTTONx_GPIO_CLK_ENABLE(Button); 00364 00365 /* Configure Button pin as input */ 00366 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00367 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00368 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00369 00370 if (Mode == BUTTON_MODE_GPIO) 00371 { 00372 /* Configure Button pin as input */ 00373 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00374 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00375 } 00376 00377 if (Mode == BUTTON_MODE_EXTI) 00378 { 00379 /* Configure Button pin as input with External interrupt */ 00380 if(Button != BUTTON_KEY) 00381 { 00382 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00383 } 00384 else 00385 { 00386 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00387 } 00388 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00389 00390 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00391 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00392 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00393 } 00394 } 00395 00396 /** 00397 * @brief Returns the selected button state. 00398 * @param Button Button to be checked. 00399 * This parameter can be one of the following values: 00400 * @arg BUTTON_TAMPER: Tamper Push Button 00401 * @arg BUTTON_KEY: Key Push Button 00402 * @retval The Button GPIO pin value 00403 */ 00404 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00405 { 00406 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00407 } 00408 00409 /** 00410 * @brief Configures all button of the joystick in GPIO or EXTI modes. 00411 * @param Joy_Mode Joystick mode. 00412 * This parameter can be one of the following values: 00413 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00414 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00415 * with interrupt generation capability 00416 * @retval HAL_OK: if all initializations are OK. Other value if error. 00417 */ 00418 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00419 { 00420 JOYState_TypeDef joykey; 00421 GPIO_InitTypeDef GPIO_InitStruct; 00422 00423 /* Initialized the Joystick. */ 00424 for(joykey = JOY_SEL; joykey < (JOY_SEL+JOYn) ; joykey++) 00425 { 00426 /* Enable the JOY clock */ 00427 JOYx_GPIO_CLK_ENABLE(joykey); 00428 00429 GPIO_InitStruct.Pin = JOY_PIN[joykey]; 00430 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00431 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00432 00433 if (Joy_Mode == JOY_MODE_GPIO) 00434 { 00435 /* Configure Joy pin as input */ 00436 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00437 HAL_GPIO_Init(JOY_PORT[joykey], &GPIO_InitStruct); 00438 } 00439 00440 if (Joy_Mode == JOY_MODE_EXTI) 00441 { 00442 /* Configure Joy pin as input with External interrupt */ 00443 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00444 HAL_GPIO_Init(JOY_PORT[joykey], &GPIO_InitStruct); 00445 00446 /* Enable and set Joy EXTI Interrupt to the lowest priority */ 00447 HAL_NVIC_SetPriority((IRQn_Type)(JOY_IRQn[joykey]), 0x0F, 0x00); 00448 HAL_NVIC_EnableIRQ((IRQn_Type)(JOY_IRQn[joykey])); 00449 } 00450 } 00451 00452 return HAL_OK; 00453 } 00454 00455 /** 00456 * @brief Returns the current joystick status. 00457 * @retval Code of the joystick key pressed 00458 * This code can be one of the following values: 00459 * @arg JOY_NONE 00460 * @arg JOY_SEL 00461 * @arg JOY_DOWN 00462 * @arg JOY_LEFT 00463 * @arg JOY_RIGHT 00464 * @arg JOY_UP 00465 * @arg JOY_NONE 00466 */ 00467 JOYState_TypeDef BSP_JOY_GetState(void) 00468 { 00469 JOYState_TypeDef joykey; 00470 00471 for(joykey = JOY_SEL; joykey < (JOY_SEL+JOYn) ; joykey++) 00472 { 00473 if(HAL_GPIO_ReadPin(JOY_PORT[joykey], JOY_PIN[joykey]) == GPIO_PIN_SET) 00474 { 00475 /* Return Code Joystick key pressed */ 00476 return joykey; 00477 } 00478 } 00479 00480 /* No Joystick key pressed */ 00481 return JOY_NONE; 00482 } 00483 00484 #if defined(HAL_UART_MODULE_ENABLED) 00485 /** 00486 * @brief Configures COM port. 00487 * @param COM Specifies the COM port to be configured. 00488 * This parameter can be one of following parameters: 00489 * @arg COM1 00490 * @param huart pointer to a UART_HandleTypeDef structure that 00491 * contains the configuration information for the specified UART peripheral. 00492 * @retval None 00493 */ 00494 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00495 { 00496 GPIO_InitTypeDef GPIO_InitStruct; 00497 00498 /* Enable GPIO clock */ 00499 COMx_TX_GPIO_CLK_ENABLE(COM); 00500 COMx_RX_GPIO_CLK_ENABLE(COM); 00501 00502 /* Enable USART clock */ 00503 COMx_CLK_ENABLE(COM); 00504 00505 /* Configure USART Tx as alternate function push-pull */ 00506 GPIO_InitStruct.Pin = COM_TX_PIN[COM]; 00507 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00508 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00509 GPIO_InitStruct.Pull = GPIO_PULLUP; 00510 GPIO_InitStruct.Alternate = COM_TX_AF[COM]; 00511 HAL_GPIO_Init(COM_TX_PORT[COM], &GPIO_InitStruct); 00512 00513 /* Configure USART Rx as alternate function push-pull */ 00514 GPIO_InitStruct.Pin = COM_RX_PIN[COM]; 00515 GPIO_InitStruct.Alternate = COM_RX_AF[COM]; 00516 HAL_GPIO_Init(COM_RX_PORT[COM], &GPIO_InitStruct); 00517 00518 /* USART configuration */ 00519 huart->Instance = COM_USART[COM]; 00520 HAL_UART_Init(huart); 00521 } 00522 #endif /* HAL_UART_MODULE_ENABLED) */ 00523 /** 00524 * @} 00525 */ 00526 00527 /** @addtogroup STM32373C_EVAL_BUS 00528 * @{ 00529 */ 00530 /******************************************************************************* 00531 BUS OPERATIONS 00532 *******************************************************************************/ 00533 #if defined(HAL_I2C_MODULE_ENABLED) 00534 /******************************* I2C Routines**********************************/ 00535 /** 00536 * @brief Initializes I2C1 MSP 00537 * @param hi2c I2C handle 00538 * @retval None 00539 */ 00540 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c) 00541 { 00542 GPIO_InitTypeDef GPIO_InitStruct; 00543 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00544 00545 if (hi2c->Instance == EVAL_I2C1) 00546 { 00547 /*##-1- Set source clock to SYSCLK for I2C1 ################################################*/ 00548 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00549 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00550 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00551 00552 /*##-2- Configure the GPIOs ################################################*/ 00553 00554 /* Enable GPIO clock */ 00555 EVAL_I2C1_GPIO_CLK_ENABLE(); 00556 00557 /* Configure I2C SCL & SDA as alternate function */ 00558 GPIO_InitStruct.Pin = (EVAL_I2C1_SCL_PIN | EVAL_I2C1_SDA_PIN); 00559 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00560 GPIO_InitStruct.Pull = GPIO_NOPULL; 00561 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00562 GPIO_InitStruct.Alternate = EVAL_I2C1_AF; 00563 HAL_GPIO_Init(EVAL_I2C1_GPIO_PORT, &GPIO_InitStruct); 00564 00565 /*##-3- Configure the Eval I2C peripheral #######################################*/ 00566 /* Enable I2C clock */ 00567 EVAL_I2C1_CLK_ENABLE(); 00568 00569 /* Force the I2C peripheral clock reset */ 00570 EVAL_I2C1_FORCE_RESET(); 00571 00572 /* Release the I2C peripheral clock reset */ 00573 EVAL_I2C1_RELEASE_RESET(); 00574 } 00575 } 00576 00577 /** 00578 * @brief I2C Bus initialization 00579 * @retval None 00580 */ 00581 static void I2C1_Init(void) 00582 { 00583 if(HAL_I2C_GetState(&heval_I2c1) == HAL_I2C_STATE_RESET) 00584 { 00585 heval_I2c1.Instance = EVAL_I2C1; 00586 heval_I2c1.Init.Timing = I2C1_TIMING; 00587 heval_I2c1.Init.OwnAddress1 = HDMI_CEC_I2C_ADDRESS; 00588 heval_I2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00589 heval_I2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00590 heval_I2c1.Init.OwnAddress2 = 0; 00591 heval_I2c1.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00592 heval_I2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00593 heval_I2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00594 00595 /* Init the I2C */ 00596 I2C1_MspInit(&heval_I2c1); 00597 HAL_I2C_Init(&heval_I2c1); 00598 } 00599 } 00600 00601 /** 00602 * @brief Write buffer through I2C. 00603 * @param pBuffer The address of the data to be written 00604 * @param Length buffer size to be written 00605 * @retval None 00606 */ 00607 static HAL_StatusTypeDef I2C1_TransmitData(uint8_t *pBuffer, uint16_t Length) 00608 { 00609 HAL_StatusTypeDef status = HAL_OK; 00610 00611 status = HAL_I2C_Slave_Transmit(&heval_I2c1, pBuffer, Length, I2c1Timeout); 00612 00613 /* Check the communication status */ 00614 if(status != HAL_OK) 00615 { 00616 /* Execute user timeout callback */ 00617 I2C1_Error(); 00618 return HAL_ERROR; 00619 } 00620 return HAL_OK; 00621 } 00622 00623 /** 00624 * @brief Manages error callback by re-initializing I2C. 00625 * @retval None 00626 */ 00627 static void I2C1_Error(void) 00628 { 00629 /* De-initialize the I2C communication BUS */ 00630 HAL_I2C_DeInit(&heval_I2c1); 00631 00632 /* Re-Initiaize the I2C communication BUS */ 00633 I2C1_Init(); 00634 } 00635 00636 /** 00637 * @brief Initializes I2C2 MSP. 00638 * @param hi2c I2C handle 00639 * @retval None 00640 */ 00641 static void I2C2_MspInit(I2C_HandleTypeDef *hi2c) 00642 { 00643 GPIO_InitTypeDef GPIO_InitStruct; 00644 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00645 00646 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C2; 00647 RCC_PeriphCLKInitStruct.I2c2ClockSelection = RCC_I2C2CLKSOURCE_SYSCLK; 00648 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00649 00650 /* Enable GPIO clock */ 00651 EVAL_I2C2_GPIO_CLK_ENABLE(); 00652 00653 /* Configure I2C SCL, SDA and SMBUS as alternate function */ 00654 GPIO_InitStruct.Pin = (EVAL_I2C2_SCL_PIN | EVAL_I2C2_SDA_PIN | EVAL_I2C2_SMBUS_PIN); 00655 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00656 GPIO_InitStruct.Pull = GPIO_NOPULL; 00657 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00658 GPIO_InitStruct.Alternate = EVAL_I2C2_AF; 00659 HAL_GPIO_Init(EVAL_I2C2_GPIO_PORT, &GPIO_InitStruct); 00660 00661 /* Enable I2C clock */ 00662 EVAL_I2C2_CLK_ENABLE(); 00663 00664 /* Force the I2C peripheral clock reset */ 00665 EVAL_I2C2_FORCE_RESET(); 00666 00667 /* Release the I2C peripheral clock reset */ 00668 EVAL_I2C2_RELEASE_RESET(); 00669 } 00670 00671 /** 00672 * @brief I2C Bus initialization 00673 * @retval None 00674 */ 00675 static void I2C2_Init(void) 00676 { 00677 if(HAL_I2C_GetState(&heval_I2c2) == HAL_I2C_STATE_RESET) 00678 { 00679 heval_I2c2.Instance = EVAL_I2C2; 00680 heval_I2c2.Init.Timing = I2C2_TIMING; 00681 heval_I2c2.Init.OwnAddress1 = 0; 00682 heval_I2c2.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00683 heval_I2c2.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00684 heval_I2c2.Init.OwnAddress2 = 0; 00685 heval_I2c2.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00686 heval_I2c2.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00687 heval_I2c2.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00688 00689 /* Init the I2C */ 00690 I2C2_MspInit(&heval_I2c2); 00691 HAL_I2C_Init(&heval_I2c2); 00692 } 00693 } 00694 00695 /** 00696 * @brief Write a value in a register of the device through I2C. 00697 * @param Addr Device address on I2C Bus. 00698 * @param Reg The target register address to write 00699 * @param RegSize The target register size (can be 8BIT or 16BIT) 00700 * @param Value The target register value to be written 00701 * @retval None 00702 */ 00703 static void I2C2_WriteData(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t Value) 00704 { 00705 HAL_StatusTypeDef status = HAL_OK; 00706 00707 status = HAL_I2C_Mem_Write(&heval_I2c2, Addr, (uint16_t)Reg, RegSize, &Value, 1, I2c2Timeout); 00708 00709 /* Check the communication status */ 00710 if(status != HAL_OK) 00711 { 00712 /* Execute user timeout callback */ 00713 I2C2_Error(); 00714 } 00715 } 00716 00717 /** 00718 * @brief Write buffer through I2C. 00719 * @param Addr Device address on I2C Bus. 00720 * @param Reg The target register address to write 00721 * @param RegSize The target register size (can be 8BIT or 16BIT) 00722 * @param pBuffer The address of the data to be written 00723 * @param Length buffer size to be written 00724 * @retval HAL Status 00725 */ 00726 static HAL_StatusTypeDef I2C2_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00727 { 00728 HAL_StatusTypeDef status = HAL_OK; 00729 00730 status = HAL_I2C_Mem_Write(&heval_I2c2, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2c2Timeout); 00731 00732 /* Check the communication status */ 00733 if(status != HAL_OK) 00734 { 00735 /* Execute user timeout callback */ 00736 I2C2_Error(); 00737 } 00738 return status; 00739 } 00740 00741 /** 00742 * @brief Read a register of the device through I2C. 00743 * @param Addr Device address on I2C Bus. 00744 * @param pBuffer The address to store the read data 00745 * @param Length buffer size to be read 00746 * @retval None 00747 */ 00748 static HAL_StatusTypeDef I2C2_ReceiveData(uint16_t Addr, uint8_t * pBuffer, uint16_t Length) 00749 { 00750 HAL_StatusTypeDef status = HAL_OK; 00751 00752 status = HAL_I2C_Master_Receive(&heval_I2c2, Addr, pBuffer, Length, I2c2Timeout); 00753 00754 /* Check the communication status */ 00755 if(status != HAL_OK) 00756 { 00757 /* Execute user timeout callback */ 00758 I2C2_Error(); 00759 } 00760 return status; 00761 } 00762 00763 /** 00764 * @brief Read a register of the device through I2C 00765 * @param Addr Device address on I2C. 00766 * @param Reg The target register address to read 00767 * @param RegSize The target register size (can be 8BIT or 16BIT) 00768 * @retval Read register value 00769 */ 00770 static uint8_t I2C2_ReadData(uint16_t Addr, uint8_t Reg, uint16_t RegSize) 00771 { 00772 HAL_StatusTypeDef status = HAL_OK; 00773 uint8_t value = 0; 00774 00775 status = HAL_I2C_Mem_Read(&heval_I2c2, Addr, Reg, RegSize, &value, 1, I2c2Timeout); 00776 00777 /* Check the communication status */ 00778 if(status != HAL_OK) 00779 { 00780 /* Execute user timeout callback */ 00781 I2C2_Error(); 00782 } 00783 return value; 00784 } 00785 00786 /** 00787 * @brief Reads buffer through I2C. 00788 * @param Addr Device address on I2C 00789 * @param Reg The target address to read 00790 * @param RegSize The target register size (can be 8BIT or 16BIT) 00791 * @param pBuffer The address to store the read data 00792 * @param Length buffer size to be read 00793 * @retval None 00794 */ 00795 static HAL_StatusTypeDef I2C2_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00796 { 00797 HAL_StatusTypeDef status = HAL_OK; 00798 00799 status = HAL_I2C_Mem_Read(&heval_I2c2, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2c2Timeout); 00800 00801 /* Check the communication status */ 00802 if(status != HAL_OK) 00803 { 00804 /* Execute user timeout callback */ 00805 I2C2_Error(); 00806 } 00807 return status; 00808 } 00809 00810 /** 00811 * @brief Checks if target device is ready for communication. 00812 * @note This function is used with Memory devices 00813 * @param DevAddress Target device address 00814 * @param Trials Number of trials 00815 * @retval HAL status 00816 */ 00817 static HAL_StatusTypeDef I2C2_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00818 { 00819 return (HAL_I2C_IsDeviceReady(&heval_I2c2, DevAddress, Trials, I2c2Timeout)); 00820 } 00821 00822 /** 00823 * @brief Manages error callback by re-initializing I2C. 00824 * @retval None 00825 */ 00826 static void I2C2_Error(void) 00827 { 00828 /* De-initialize the I2C communication BUS */ 00829 HAL_I2C_DeInit(&heval_I2c2); 00830 00831 /* Re-Initiaize the I2C communication BUS */ 00832 I2C2_Init(); 00833 } 00834 00835 #endif /*HAL_I2C_MODULE_ENABLED*/ 00836 00837 #if defined(HAL_SPI_MODULE_ENABLED) 00838 /******************************* SPI Routines *********************************/ 00839 /** 00840 * @brief Initializes SPI MSP. 00841 * @param hspi SPI handle 00842 * @retval None 00843 */ 00844 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00845 { 00846 GPIO_InitTypeDef GPIO_InitStruct; 00847 00848 /* Enable SPI clock */ 00849 EVAL_SPIx_CLK_ENABLE(); 00850 00851 /* enable SPI gpio clock */ 00852 EVAL_SPIx_GPIO_CLK_ENABLE(); 00853 00854 /* configure SPI SCK, MOSI and MISO */ 00855 GPIO_InitStruct.Pin = (EVAL_SPIx_SCK_PIN | EVAL_SPIx_MOSI_PIN | EVAL_SPIx_MISO_PIN); 00856 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00857 GPIO_InitStruct.Pull = GPIO_NOPULL; 00858 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00859 GPIO_InitStruct.Alternate = EVAL_SPIx_AF; 00860 HAL_GPIO_Init(EVAL_SPIx_GPIO_PORT, &GPIO_InitStruct); 00861 00862 /* Force the SPI peripheral clock reset */ 00863 EVAL_SPIx_FORCE_RESET(); 00864 00865 /* Release the SPI peripheral clock reset */ 00866 EVAL_SPIx_RELEASE_RESET(); 00867 } 00868 00869 /** 00870 * @brief Initializes SPI HAL. 00871 * @retval None 00872 */ 00873 static void SPIx_Init(void) 00874 { 00875 if(HAL_SPI_GetState(&heval_Spi) == HAL_SPI_STATE_RESET) 00876 { 00877 /* SPI Config */ 00878 heval_Spi.Instance = EVAL_SPIx; 00879 /* SPI baudrate is set to 18 MHz (PCLK2/SPI_BaudRatePrescaler = 72/4 = 18 MHz) */ 00880 heval_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_4; 00881 heval_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00882 heval_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00883 heval_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00884 heval_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00885 heval_Spi.Init.CRCPolynomial = 7; 00886 heval_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00887 heval_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00888 heval_Spi.Init.NSS = SPI_NSS_SOFT; 00889 heval_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00890 heval_Spi.Init.Mode = SPI_MODE_MASTER; 00891 00892 SPIx_MspInit(&heval_Spi); 00893 HAL_SPI_Init(&heval_Spi); 00894 } 00895 } 00896 00897 /** 00898 * @brief SPI Read 4 bytes from device 00899 * @retval Value read on the SPI 00900 */ 00901 static uint32_t SPIx_Read(void) 00902 { 00903 HAL_StatusTypeDef status = HAL_OK; 00904 uint32_t readvalue = 0x0; 00905 uint32_t writevalue = 0xFFFFFFFF; 00906 00907 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &writevalue, (uint8_t*) &readvalue, 1, SpixTimeout); 00908 00909 /* Check the communication status */ 00910 if(status != HAL_OK) 00911 { 00912 /* Execute user timeout callback */ 00913 SPIx_Error(); 00914 } 00915 00916 return readvalue; 00917 } 00918 00919 /** 00920 * @brief SPI Write a byte to device 00921 * @param Value value to be written 00922 * @retval None 00923 */ 00924 static void SPIx_Write(uint8_t Value) 00925 { 00926 HAL_StatusTypeDef status = HAL_OK; 00927 00928 status = HAL_SPI_Transmit(&heval_Spi, (uint8_t*) &Value, 1, SpixTimeout); 00929 00930 /* Check the communication status */ 00931 if(status != HAL_OK) 00932 { 00933 /* Execute user timeout callback */ 00934 SPIx_Error(); 00935 } 00936 } 00937 00938 /** 00939 * @brief SPI error treatment function 00940 * @retval None 00941 */ 00942 static void SPIx_Error (void) 00943 { 00944 /* De-initialize the SPI communication BUS */ 00945 HAL_SPI_DeInit(&heval_Spi); 00946 00947 /* Re- Initiaize the SPI communication BUS */ 00948 SPIx_Init(); 00949 } 00950 00951 #endif /*HAL_SPI_MODULE_ENABLED*/ 00952 00953 /****************************************************************************** 00954 LINK OPERATIONS 00955 *******************************************************************************/ 00956 /** 00957 * @} 00958 */ 00959 00960 /** @defgroup STM32373C_EVAL_LINK_OPERATIONS Link Operation functions 00961 * @{ 00962 */ 00963 00964 #if defined(HAL_SPI_MODULE_ENABLED) 00965 /********************************* LINK LCD ***********************************/ 00966 00967 /** 00968 * @brief Configures the LCD_SPI interface. 00969 * @retval None 00970 */ 00971 void LCD_IO_Init(void) 00972 { 00973 GPIO_InitTypeDef GPIO_InitStruct; 00974 00975 /* Configure the LCD Control pins ------------------------------------------*/ 00976 LCD_NCS_GPIO_CLK_ENABLE(); 00977 00978 /* Configure NCS in Output Push-Pull mode */ 00979 GPIO_InitStruct.Pin = LCD_NCS_PIN; 00980 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00981 GPIO_InitStruct.Pull = GPIO_NOPULL; 00982 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00983 HAL_GPIO_Init(LCD_NCS_GPIO_PORT, &GPIO_InitStruct); 00984 00985 /* Set or Reset the control line */ 00986 LCD_CS_LOW(); 00987 LCD_CS_HIGH(); 00988 00989 SPIx_Init(); 00990 } 00991 00992 /** 00993 * @brief Write register value. 00994 * @param pData Pointer on the register value 00995 * @param Size Size of byte to transmit to the register 00996 * @retval None 00997 */ 00998 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00999 { 01000 uint32_t counter = 0; 01001 01002 /* Reset LCD control line(/CS) and Send data */ 01003 LCD_CS_LOW(); 01004 01005 /* Send Start Byte */ 01006 SPIx_Write(START_BYTE | LCD_WRITE_REG); 01007 01008 for (counter = Size; counter != 0; counter--) 01009 { 01010 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01011 { 01012 } 01013 /* Need to invert bytes for LCD*/ 01014 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *(pData+1); 01015 01016 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01017 { 01018 } 01019 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *pData; 01020 counter--; 01021 pData += 2; 01022 } 01023 01024 /* Wait until the bus is ready before releasing Chip select */ 01025 while(((heval_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 01026 { 01027 } 01028 01029 /* Reset LCD control line(/CS) and Send data */ 01030 LCD_CS_HIGH(); 01031 } 01032 01033 /** 01034 * @brief register address. 01035 * @param Reg 01036 * @retval None 01037 */ 01038 void LCD_IO_WriteReg(uint8_t Reg) 01039 { 01040 /* Reset LCD control line(/CS) and Send command */ 01041 LCD_CS_LOW(); 01042 01043 /* Send Start Byte */ 01044 SPIx_Write(START_BYTE | SET_INDEX); 01045 01046 /* Write 16-bit Reg Index (High Byte is 0) */ 01047 SPIx_Write(0x00); 01048 SPIx_Write(Reg); 01049 01050 /* Deselect : Chip Select high */ 01051 LCD_CS_HIGH(); 01052 } 01053 01054 /** 01055 * @brief Read register value. 01056 * @retval None 01057 */ 01058 uint16_t LCD_IO_ReadData(uint16_t Reg) 01059 { 01060 uint32_t readvalue = 0; 01061 01062 /* Send Reg value to Read */ 01063 LCD_IO_WriteReg(Reg); 01064 01065 /* Reset LCD control line(/CS) and Send command */ 01066 LCD_CS_LOW(); 01067 01068 /* Send Start Byte */ 01069 SPIx_Write(START_BYTE | LCD_READ_REG); 01070 01071 /* Read Upper Byte */ 01072 SPIx_Write(0xFF); 01073 readvalue = SPIx_Read(); 01074 readvalue = readvalue << 8; 01075 readvalue |= SPIx_Read(); 01076 01077 HAL_Delay(10); 01078 01079 /* Deselect : Chip Select high */ 01080 LCD_CS_HIGH(); 01081 return readvalue; 01082 } 01083 01084 /** 01085 * @brief Wait for loop in ms. 01086 * @param Delay in ms. 01087 * @retval None 01088 */ 01089 void LCD_Delay (uint32_t Delay) 01090 { 01091 HAL_Delay(Delay); 01092 } 01093 01094 /******************************** LINK SD Card ********************************/ 01095 01096 /** 01097 * @brief Initializes the SD Card and put it into StandBy State (Ready for 01098 * data transfer). 01099 * @retval None 01100 */ 01101 void SD_IO_Init(void) 01102 { 01103 GPIO_InitTypeDef GPIO_InitStruct; 01104 uint8_t counter; 01105 01106 /* SD_CS_GPIO and SD_DETECT_GPIO Periph clock enable */ 01107 SD_CS_GPIO_CLK_ENABLE(); 01108 SD_DETECT_GPIO_CLK_ENABLE(); 01109 01110 /* Configure SD_CS_PIN pin: SD Card CS pin */ 01111 GPIO_InitStruct.Pin = SD_CS_PIN; 01112 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01113 GPIO_InitStruct.Pull = GPIO_PULLUP; 01114 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 01115 HAL_GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStruct); 01116 01117 /* Configure SD_DETECT_PIN pin: SD Card detect pin */ 01118 GPIO_InitStruct.Pin = SD_DETECT_PIN; 01119 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING_FALLING; 01120 GPIO_InitStruct.Pull = GPIO_PULLUP; 01121 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &GPIO_InitStruct); 01122 01123 /* Enable and set SD EXTI Interrupt to the lowest priority */ 01124 HAL_NVIC_SetPriority(SD_DETECT_EXTI_IRQn, 0x0F, 0); 01125 HAL_NVIC_EnableIRQ(SD_DETECT_EXTI_IRQn); 01126 01127 /*------------Put SD in SPI mode--------------*/ 01128 /* SD SPI Config */ 01129 SPIx_Init(); 01130 01131 /* SD chip select high */ 01132 SD_CS_HIGH(); 01133 01134 /* Send dummy byte 0xFF, 10 times with CS high */ 01135 /* Rise CS and MOSI for 80 clocks cycles */ 01136 for (counter = 0; counter <= 9; counter++) 01137 { 01138 /* Send dummy byte 0xFF */ 01139 SD_IO_WriteByte(SD_DUMMY_BYTE); 01140 } 01141 } 01142 01143 /** 01144 * @brief Write a byte on the SD. 01145 * @param Data byte to send. 01146 * @retval None 01147 */ 01148 void SD_IO_WriteByte(uint8_t Data) 01149 { 01150 /* Send the byte */ 01151 SPIx_Write(Data); 01152 } 01153 01154 /** 01155 * @brief Read a byte from the SD. 01156 * @retval The received byte. 01157 */ 01158 uint8_t SD_IO_ReadByte(void) 01159 { 01160 uint8_t data = 0; 01161 01162 /* Get the received data */ 01163 data = SPIx_Read(); 01164 01165 /* Return the shifted data */ 01166 return data; 01167 } 01168 01169 /** 01170 * @brief Send 5 bytes command to the SD card and get response 01171 * @param Cmd The user expected command to send to SD card. 01172 * @param Arg The command argument. 01173 * @param Crc The CRC. 01174 * @param Response Expected response from the SD card 01175 * @retval HAL_StatusTypeDef HAL Status 01176 */ 01177 HAL_StatusTypeDef SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) 01178 { 01179 uint32_t counter = 0x00; 01180 uint8_t frame[6]; 01181 01182 /* Prepare Frame to send */ 01183 frame[0] = (Cmd | 0x40); /* Construct byte 1 */ 01184 frame[1] = (uint8_t)(Arg >> 24); /* Construct byte 2 */ 01185 frame[2] = (uint8_t)(Arg >> 16); /* Construct byte 3 */ 01186 frame[3] = (uint8_t)(Arg >> 8); /* Construct byte 4 */ 01187 frame[4] = (uint8_t)(Arg); /* Construct byte 5 */ 01188 frame[5] = (Crc); /* Construct CRC: byte 6 */ 01189 01190 /* SD chip select low */ 01191 SD_CS_LOW(); 01192 01193 /* Send Frame */ 01194 for (counter = 0; counter < 6; counter++) 01195 { 01196 SD_IO_WriteByte(frame[counter]); /* Send the Cmd bytes */ 01197 } 01198 01199 if(Response != SD_NO_RESPONSE_EXPECTED) 01200 { 01201 return SD_IO_WaitResponse(Response); 01202 } 01203 01204 return HAL_OK; 01205 } 01206 01207 /** 01208 * @brief Wait response from the SD card 01209 * @param Response Expected response from the SD card 01210 * @retval HAL_StatusTypeDef HAL Status 01211 */ 01212 HAL_StatusTypeDef SD_IO_WaitResponse(uint8_t Response) 01213 { 01214 uint32_t timeout = 0xFFF; 01215 01216 /* Check if response is got or a timeout is happen */ 01217 while ((SD_IO_ReadByte() != Response) && timeout) 01218 { 01219 timeout--; 01220 } 01221 01222 if (timeout == 0) 01223 { 01224 /* After time out */ 01225 return HAL_TIMEOUT; 01226 } 01227 else 01228 { 01229 /* Right response got */ 01230 return HAL_OK; 01231 } 01232 } 01233 01234 /** 01235 * @brief Send dummy byte with CS High 01236 * @retval None 01237 */ 01238 void SD_IO_WriteDummy(void) 01239 { 01240 /* SD chip select high */ 01241 SD_CS_HIGH(); 01242 01243 /* Send Dummy byte 0xFF */ 01244 SD_IO_WriteByte(SD_DUMMY_BYTE); 01245 } 01246 01247 #endif /* HAL_SPI_MODULE_ENABLED */ 01248 01249 #if defined(HAL_I2C_MODULE_ENABLED) 01250 /********************************* LINK I2C EEPROM *****************************/ 01251 /** 01252 * @brief Initializes peripherals used by the I2C EEPROM driver. 01253 * @retval None 01254 */ 01255 void EEPROM_IO_Init(void) 01256 { 01257 I2C2_Init(); 01258 } 01259 01260 /** 01261 * @brief Write data to I2C EEPROM driver 01262 * @param DevAddress Target device address 01263 * @param MemAddress Internal memory address 01264 * @param pBuffer Pointer to data buffer 01265 * @param BufferSize Amount of data to be sent 01266 * @retval HAL status 01267 */ 01268 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01269 { 01270 return (I2C2_WriteBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01271 } 01272 01273 /** 01274 * @brief Read data from I2C EEPROM driver 01275 * @param DevAddress Target device address 01276 * @param MemAddress Internal memory address 01277 * @param pBuffer Pointer to data buffer 01278 * @param BufferSize Amount of data to be read 01279 * @retval HAL status 01280 */ 01281 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01282 { 01283 return (I2C2_ReadBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01284 } 01285 01286 /** 01287 * @brief Checks if target device is ready for communication. 01288 * @note This function is used with Memory devices 01289 * @param DevAddress Target device address 01290 * @param Trials Number of trials 01291 * @retval HAL status 01292 */ 01293 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01294 { 01295 return (I2C2_IsDeviceReady(DevAddress, Trials)); 01296 } 01297 01298 /********************************* LINK I2C TEMPERATURE SENSOR *****************************/ 01299 /** 01300 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 01301 * @retval None 01302 */ 01303 void TSENSOR_IO_Init(void) 01304 { 01305 I2C2_Init(); 01306 } 01307 01308 /** 01309 * @brief Writes one byte to the TSENSOR. 01310 * @param DevAddress Target device address 01311 * @param pBuffer Pointer to data buffer 01312 * @param WriteAddr TSENSOR's internal address to write to. 01313 * @param Length Number of data to write 01314 * @retval None 01315 */ 01316 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length) 01317 { 01318 I2C2_WriteBuffer(DevAddress, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01319 } 01320 01321 /** 01322 * @brief Reads one byte from the TSENSOR. 01323 * @param DevAddress Target device address 01324 * @param pBuffer pointer to the buffer that receives the data read from the TSENSOR. 01325 * @param ReadAddr TSENSOR's internal address to read from. 01326 * @param Length Number of data to read 01327 * @retval None 01328 */ 01329 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length) 01330 { 01331 I2C2_ReadBuffer(DevAddress, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01332 } 01333 01334 /** 01335 * @brief Checks if Temperature Sensor is ready for communication. 01336 * @param DevAddress Target device address 01337 * @param Trials Number of trials 01338 * @retval HAL status 01339 */ 01340 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01341 { 01342 return (I2C2_IsDeviceReady(DevAddress, Trials)); 01343 } 01344 01345 01346 /********************************* LINK AUDIO CODEC ***********************************/ 01347 /** 01348 * @brief Initializes peripherals used by the Audio Codec driver. 01349 * @retval None 01350 */ 01351 void AUDIO_IO_Init(void) 01352 { 01353 GPIO_InitTypeDef GPIO_InitStruct; 01354 01355 /* Enable Reset GPIO Clock */ 01356 AUDIO_RESET_GPIO_CLK_ENABLE(); 01357 01358 /* Audio reset pin configuration -------------------------------------------------*/ 01359 GPIO_InitStruct.Pin = AUDIO_RESET_PIN; 01360 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01361 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 01362 GPIO_InitStruct.Pull = GPIO_NOPULL; 01363 HAL_GPIO_Init(AUDIO_RESET_GPIO_PORT, &GPIO_InitStruct); 01364 01365 I2C2_Init(); 01366 01367 /* Power Down the codec */ 01368 CODEC_AUDIO_POWER_OFF(); 01369 01370 /* wait for a delay to insure registers erasing */ 01371 HAL_Delay(5); 01372 01373 /* Power on the codec */ 01374 CODEC_AUDIO_POWER_ON(); 01375 01376 /* wait for a delay to insure registers erasing */ 01377 HAL_Delay(5); 01378 } 01379 01380 /** 01381 * @brief DeInitializes Audio low level. 01382 * @note This function is intentionally kept empty, user should define it. 01383 */ 01384 void AUDIO_IO_DeInit(void) 01385 { 01386 01387 } 01388 01389 /** 01390 * @brief Writes a single data on the Audio Codec. 01391 * @param DevAddress Target device address 01392 * @param Reg Target Register address 01393 * @param Value Data to be written 01394 * @retval None 01395 */ 01396 void AUDIO_IO_Write(uint16_t DevAddress, uint8_t Reg, uint8_t Value) 01397 { 01398 I2C2_WriteData(DevAddress, Reg, I2C_MEMADD_SIZE_8BIT, Value); 01399 } 01400 01401 /** 01402 * @brief Reads a single data from the Audio Codec. 01403 * @param DevAddress Target device address 01404 * @param Reg Target Register address 01405 * @retval Data to be read 01406 */ 01407 uint8_t AUDIO_IO_Read(uint16_t DevAddress, uint8_t Reg) 01408 { 01409 uint8_t value; 01410 01411 value = I2C2_ReadData(DevAddress, Reg, I2C_MEMADD_SIZE_8BIT); 01412 01413 return value; 01414 } 01415 01416 /****************************** LINK HDMI CEC *********************************/ 01417 /** 01418 * @brief Initializes CEC low level. 01419 * @retval None 01420 */ 01421 void HDMI_CEC_IO_Init (void) 01422 { 01423 GPIO_InitTypeDef GPIO_InitStruct; 01424 01425 /* Enable CEC clock */ 01426 __HAL_RCC_CEC_CLK_ENABLE(); 01427 01428 /* Enable CEC LINE GPIO clock */ 01429 HDMI_CEC_LINE_CLK_ENABLE(); 01430 01431 /* Configure CEC LINE GPIO as alternate function open drain */ 01432 GPIO_InitStruct.Pin = HDMI_CEC_LINE_PIN; 01433 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 01434 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 01435 GPIO_InitStruct.Pull = GPIO_NOPULL; 01436 GPIO_InitStruct.Alternate = HDMI_CEC_LINE_AF; 01437 HAL_GPIO_Init(HDMI_CEC_LINE_GPIO_PORT, &GPIO_InitStruct); 01438 01439 /* CEC IRQ Channel configuration */ 01440 HAL_NVIC_SetPriority((IRQn_Type)HDMI_CEC_IRQn, 0xF, 0x0); 01441 HAL_NVIC_EnableIRQ((IRQn_Type)HDMI_CEC_IRQn); 01442 01443 /* Enable CEC HPD SINK GPIO clock */ 01444 HDMI_CEC_HPD_SINK_CLK_ENABLE(); 01445 01446 /* Configure CEC HPD SINK GPIO as output push pull */ 01447 GPIO_InitStruct.Pin = HDMI_CEC_HPD_SINK_PIN; 01448 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01449 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 01450 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 01451 HAL_GPIO_Init(HDMI_CEC_HPD_SINK_GPIO_PORT, &GPIO_InitStruct); 01452 01453 I2C1_Init(); 01454 01455 /* Enable CEC HPD SOURCE GPIO clock */ 01456 HDMI_CEC_HPD_SOURCE_CLK_ENABLE(); 01457 01458 /* Configure CEC HPD SOURCE GPIO as output push pull */ 01459 GPIO_InitStruct.Pin = HDMI_CEC_HPD_SOURCE_PIN; 01460 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 01461 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 01462 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 01463 HAL_GPIO_Init(HDMI_CEC_HPD_SOURCE_GPIO_PORT, &GPIO_InitStruct); 01464 01465 I2C2_Init(); 01466 } 01467 01468 /** 01469 * @brief Write data to I2C HDMI CEC driver 01470 * @param pBuffer Pointer to data buffer 01471 * @param BufferSize Amount of data to be sent 01472 * @retval HAL status 01473 */ 01474 HAL_StatusTypeDef HDMI_CEC_IO_WriteData(uint8_t * pBuffer, uint16_t BufferSize) 01475 { 01476 return (I2C1_TransmitData(pBuffer, BufferSize)); 01477 } 01478 01479 /** 01480 * @brief Read data to I2C HDMI CEC driver 01481 * @param DevAddress Target device address 01482 * @param pBuffer Pointer to data buffer 01483 * @param BufferSize Amount of data to be sent 01484 * @retval HAL status 01485 */ 01486 HAL_StatusTypeDef HDMI_CEC_IO_ReadData(uint16_t DevAddress, uint8_t * pBuffer, uint16_t BufferSize) 01487 { 01488 return (I2C2_ReceiveData(DevAddress, pBuffer, BufferSize)); 01489 } 01490 01491 #endif /* HAL_I2C_MODULE_ENABLED */ 01492 01493 /** 01494 * @} 01495 */ 01496 01497 /** 01498 * @} 01499 */ 01500 01501 /** 01502 * @} 01503 */ 01504 01505 /** 01506 * @} 01507 */ 01508 01509 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 11:20:43 for STM32373C_EVAL BSP User Manual by
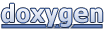