STM3210E_EVAL BSP User Manual
|
stm3210e_eval_nor.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_nor.c 00004 * @author MCD Application Team 00005 * @version V6.0.1 00006 * @date 18-December-2015 00007 * @brief This file includes a standard driver for the M29W128GL70ZA6E NOR flash memory 00008 * device mounted on STM3210E-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the M29W128GL NOR flash external memory mounted 00044 on STM3210E-EVAL evaluation board. 00045 - This driver does not need a specific component driver for the NOR device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the NOR external memory using the BSP_NOR_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 FSMC controller configuration to interface with the external NOR memory. 00054 00055 + NOR flash operations 00056 o NOR external memory can be accessed with read/write operations once it is 00057 initialized. 00058 Read/write operation can be performed with AHB access using the functions 00059 BSP_NOR_ReadData()/BSP_NOR_WriteData(). The BSP_NOR_WriteData() performs write operation 00060 of an amount of data by unit (halfword). You can also perform a program data 00061 operation of an amount of data using the function BSP_NOR_ProgramData(). 00062 o The function BSP_NOR_Read_ID() returns the chip IDs stored in the structure 00063 "NOR_IDTypeDef". (see the NOR IDs in the memory data sheet) 00064 o Perform erase block operation using the function BSP_NOR_Erase_Block() and by 00065 specifying the block address. You can perform an erase operation of the whole 00066 chip by calling the function BSP_NOR_Erase_Chip(). 00067 o After other operations, the function BSP_NOR_ReturnToReadMode() allows the NOR 00068 flash to return to read mode to perform read operations on it. 00069 00070 ------------------------------------------------------------------------------*/ 00071 00072 /* Includes ------------------------------------------------------------------*/ 00073 #include "stm3210e_eval_nor.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM3210E_EVAL 00080 * @{ 00081 */ 00082 00083 /** @defgroup STM3210E_EVAL_NOR STM3210E_EVAL NOR 00084 * @{ 00085 */ 00086 00087 /* Private typedef -----------------------------------------------------------*/ 00088 00089 /** @defgroup STM3210E_EVAL_NOR_Private_Types_Definitions Private_Types_Definitions 00090 * @{ 00091 */ 00092 00093 /** 00094 * @} 00095 */ 00096 00097 /* Private define ------------------------------------------------------------*/ 00098 00099 /** @defgroup STM3210E_EVAL_NOR_Private_Defines Private_Defines 00100 * @{ 00101 */ 00102 00103 /** 00104 * @} 00105 */ 00106 00107 /* Private macro -------------------------------------------------------------*/ 00108 00109 /** @defgroup STM3210E_EVAL_NOR_Private_Macros Private_Macros 00110 * @{ 00111 */ 00112 00113 /** 00114 * @} 00115 */ 00116 00117 /* Private variables ---------------------------------------------------------*/ 00118 00119 /** @defgroup STM3210E_EVAL_NOR_Private_Variables Private_Variables 00120 * @{ 00121 */ 00122 static NOR_HandleTypeDef norHandle; 00123 static FSMC_NORSRAM_TimingTypeDef Timing; 00124 00125 /** 00126 * @} 00127 */ 00128 00129 /* Private function prototypes -----------------------------------------------*/ 00130 00131 /** @defgroup STM3210E_EVAL_NOR_Private_Function_Prototypes Private_Function_Prototypes 00132 * @{ 00133 */ 00134 00135 static void NOR_MspInit(void); 00136 00137 /** 00138 * @} 00139 */ 00140 00141 /* Private functions ---------------------------------------------------------*/ 00142 00143 /** @defgroup STM3210E_EVAL_NOR_Exported_Functions Exported_Functions 00144 * @{ 00145 */ 00146 00147 /** 00148 * @brief Initializes the NOR device. 00149 * @retval NOR memory status 00150 */ 00151 uint8_t BSP_NOR_Init(void) 00152 { 00153 norHandle.Instance = FSMC_NORSRAM_DEVICE; 00154 norHandle.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00155 00156 /* NOR device configuration */ 00157 Timing.AddressSetupTime = 2; 00158 Timing.AddressHoldTime = 1; 00159 Timing.DataSetupTime = 5; 00160 Timing.BusTurnAroundDuration = 0; 00161 Timing.CLKDivision = 2; 00162 Timing.DataLatency = 2; 00163 Timing.AccessMode = FSMC_ACCESS_MODE_B; 00164 00165 norHandle.Init.NSBank = FSMC_NORSRAM_BANK2; 00166 norHandle.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00167 norHandle.Init.MemoryType = FSMC_MEMORY_TYPE_NOR; 00168 norHandle.Init.MemoryDataWidth = NOR_MEMORY_WIDTH; 00169 norHandle.Init.BurstAccessMode = NOR_BURSTACCESS; 00170 norHandle.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00171 norHandle.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00172 norHandle.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00173 norHandle.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00174 norHandle.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00175 norHandle.Init.ExtendedMode = FSMC_EXTENDED_MODE_DISABLE; 00176 norHandle.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00177 norHandle.Init.WriteBurst = NOR_WRITEBURST; 00178 00179 /* NOR controller initialization */ 00180 NOR_MspInit(); 00181 00182 if(HAL_NOR_Init(&norHandle, &Timing, &Timing) != HAL_OK) 00183 { 00184 return NOR_STATUS_ERROR; 00185 } 00186 else 00187 { 00188 return NOR_STATUS_OK; 00189 } 00190 } 00191 00192 /** 00193 * @brief Reads an amount of data from the NOR device. 00194 * @param uwStartAddress: Read start address 00195 * @param pData: Pointer to data to be read 00196 * @param uwDataSize: Size of data to read 00197 * @retval NOR memory status 00198 */ 00199 uint8_t BSP_NOR_ReadData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00200 { 00201 if(HAL_NOR_ReadBuffer(&norHandle, NOR_DEVICE_ADDR + uwStartAddress, pData, uwDataSize) != HAL_OK) 00202 { 00203 return NOR_STATUS_ERROR; 00204 } 00205 else 00206 { 00207 return NOR_STATUS_OK; 00208 } 00209 } 00210 00211 /** 00212 * @brief Returns the NOR memory to read mode. 00213 * @retval None 00214 */ 00215 void BSP_NOR_ReturnToReadMode(void) 00216 { 00217 HAL_NOR_ReturnToReadMode(&norHandle); 00218 } 00219 00220 /** 00221 * @brief Writes an amount of data to the NOR device. 00222 * @param uwStartAddress: Write start address 00223 * @param pData: Pointer to data to be written 00224 * @param uwDataSize: Size of data to write 00225 * @retval NOR memory status 00226 */ 00227 uint8_t BSP_NOR_WriteData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00228 { 00229 uint32_t index = uwDataSize; 00230 00231 while(index > 0) 00232 { 00233 /* Write data to NOR */ 00234 HAL_NOR_Program(&norHandle, (uint32_t *)(NOR_DEVICE_ADDR + uwStartAddress), pData); 00235 00236 /* Read NOR device status */ 00237 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, PROGRAM_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00238 { 00239 return NOR_STATUS_ERROR; 00240 } 00241 00242 /* Update the counters */ 00243 index--; 00244 uwStartAddress += 2; 00245 pData++; 00246 } 00247 00248 return NOR_STATUS_OK; 00249 } 00250 00251 /** 00252 * @brief Programs an amount of data to the NOR device. 00253 * @param uwStartAddress: Write start address 00254 * @param pData: Pointer to data to be written 00255 * @param uwDataSize: Size of data to write 00256 * @retval NOR memory status 00257 */ 00258 uint8_t BSP_NOR_ProgramData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00259 { 00260 /* Send NOR program buffer operation */ 00261 HAL_NOR_ProgramBuffer(&norHandle, uwStartAddress, pData, uwDataSize); 00262 00263 /* Return the NOR memory status */ 00264 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, PROGRAM_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00265 { 00266 return NOR_STATUS_ERROR; 00267 } 00268 else 00269 { 00270 return NOR_STATUS_OK; 00271 } 00272 } 00273 00274 /** 00275 * @brief Erases the specified block of the NOR device. 00276 * @param BlockAddress: Block address to erase 00277 * @retval NOR memory status 00278 */ 00279 uint8_t BSP_NOR_Erase_Block(uint32_t BlockAddress) 00280 { 00281 /* Send NOR erase block operation */ 00282 HAL_NOR_Erase_Block(&norHandle, BlockAddress, NOR_DEVICE_ADDR); 00283 00284 /* Return the NOR memory status */ 00285 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, BLOCKERASE_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00286 { 00287 return NOR_STATUS_ERROR; 00288 } 00289 else 00290 { 00291 return NOR_STATUS_OK; 00292 } 00293 } 00294 00295 /** 00296 * @brief Erases the entire NOR chip. 00297 * @retval NOR memory status 00298 */ 00299 uint8_t BSP_NOR_Erase_Chip(void) 00300 { 00301 /* Send NOR Erase chip operation */ 00302 HAL_NOR_Erase_Chip(&norHandle, NOR_DEVICE_ADDR); 00303 00304 /* Return the NOR memory status */ 00305 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, CHIPERASE_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00306 { 00307 return NOR_STATUS_ERROR; 00308 } 00309 else 00310 { 00311 return NOR_STATUS_OK; 00312 } 00313 } 00314 00315 /** 00316 * @brief Reads NOR flash IDs. 00317 * @param pNOR_ID : Pointer to NOR ID structure 00318 * @retval NOR memory status 00319 */ 00320 uint8_t BSP_NOR_Read_ID(NOR_IDTypeDef *pNOR_ID) 00321 { 00322 if(HAL_NOR_Read_ID(&norHandle, pNOR_ID) != HAL_OK) 00323 { 00324 return NOR_STATUS_ERROR; 00325 } 00326 else 00327 { 00328 return NOR_STATUS_OK; 00329 } 00330 } 00331 00332 /** 00333 * @brief Initializes the NOR MSP. 00334 * @retval None 00335 */ 00336 static void NOR_MspInit(void) 00337 { 00338 GPIO_InitTypeDef gpioinitstruct = {0}; 00339 00340 /* Enable FSMC clock */ 00341 __HAL_RCC_FSMC_CLK_ENABLE(); 00342 00343 /* Enable GPIOs clock */ 00344 __HAL_RCC_GPIOD_CLK_ENABLE(); 00345 __HAL_RCC_GPIOE_CLK_ENABLE(); 00346 __HAL_RCC_GPIOF_CLK_ENABLE(); 00347 __HAL_RCC_GPIOG_CLK_ENABLE(); 00348 00349 /* Common GPIO configuration */ 00350 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00351 gpioinitstruct.Pull = GPIO_PULLUP; 00352 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00353 00354 /*-- GPIO Configuration ------------------------------------------------------*/ 00355 /*!< NOR Data lines configuration */ 00356 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8 | GPIO_PIN_9 | 00357 GPIO_PIN_10 | GPIO_PIN_14 | GPIO_PIN_15; 00358 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00359 00360 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00361 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | 00362 GPIO_PIN_14 | GPIO_PIN_15; 00363 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00364 00365 /*!< NOR Address lines configuration */ 00366 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00367 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | 00368 GPIO_PIN_14 | GPIO_PIN_15; 00369 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00370 00371 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | 00372 GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5; 00373 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00374 00375 gpioinitstruct.Pin = GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13; 00376 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00377 00378 gpioinitstruct.Pin = GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6; 00379 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00380 00381 /*!< NOE and NWE configuration */ 00382 gpioinitstruct.Pin = GPIO_PIN_4 | GPIO_PIN_5; 00383 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00384 00385 /*!< NE2 configuration */ 00386 gpioinitstruct.Pin = GPIO_PIN_9 | GPIO_PIN_12; 00387 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00388 00389 /*!< Configure PD6 for NOR memory Ready/Busy signal */ 00390 gpioinitstruct.Pin = GPIO_PIN_6; 00391 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00392 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00393 00394 } 00395 00396 /** 00397 * @brief NOR BSP Wait for Ready/Busy signal. 00398 * @param hnor: Pointer to NOR handle 00399 * @param Timeout: Timeout duration 00400 * @retval None 00401 */ 00402 void HAL_NOR_MspWait(NOR_HandleTypeDef *hnor, uint32_t Timeout) 00403 { 00404 uint32_t timeout = Timeout; 00405 00406 /* Polling on Ready/Busy signal */ 00407 while((HAL_GPIO_ReadPin(NOR_READY_BUSY_GPIO, NOR_READY_BUSY_PIN) != NOR_BUSY_STATE) && (timeout > 0)) 00408 { 00409 timeout--; 00410 } 00411 00412 timeout = Timeout; 00413 00414 /* Polling on Ready/Busy signal */ 00415 while((HAL_GPIO_ReadPin(NOR_READY_BUSY_GPIO, NOR_READY_BUSY_PIN) != NOR_READY_STATE) && (timeout > 0)) 00416 { 00417 timeout--; 00418 } 00419 } 00420 00421 /** 00422 * @} 00423 */ 00424 00425 /** 00426 * @} 00427 */ 00428 00429 /** 00430 * @} 00431 */ 00432 00433 /** 00434 * @} 00435 */ 00436 00437 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 10 2015 17:39:43 for STM3210E_EVAL BSP User Manual by
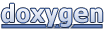