STM3210E_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_SD_Init (void) |
Initializes the SD card device. | |
uint8_t | BSP_SD_ITConfig (void) |
Configures Interrupt mode for SD detection pin. | |
void | BSP_SD_DetectIT (void) |
SD detect IT treatment. | |
void | BSP_SD_DetectCallback (void) |
SD detect IT detection callback. | |
uint8_t | BSP_SD_ReadBlocks (uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) |
Reads block(s) from a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocks (uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) |
Writes block(s) to a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_ReadBlocks_DMA (uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) |
Reads block(s) from a specified address in an SD card, in DMA mode. | |
uint8_t | BSP_SD_WriteBlocks_DMA (uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) |
Writes block(s) to a specified address in an SD card, in DMA mode. | |
uint8_t | BSP_SD_Erase (uint64_t StartAddr, uint64_t EndAddr) |
Erases the specified memory area of the given SD card. | |
void | BSP_SD_IRQHandler (void) |
Handles SD card interrupt request. | |
void | BSP_SD_DMA_Tx_IRQHandler (void) |
Handles SD DMA Tx transfer interrupt request. | |
void | BSP_SD_DMA_Rx_IRQHandler (void) |
Handles SD DMA Rx transfer interrupt request. | |
HAL_SD_TransferStateTypedef | BSP_SD_GetStatus (void) |
Gets the current SD card data status. | |
void | BSP_SD_GetCardInfo (HAL_SD_CardInfoTypedef *CardInfo) |
Get SD information about specific SD card. | |
uint8_t | BSP_SD_IsDetected (void) |
Detects if SD card is correctly plugged in the memory slot or not. |
Function Documentation
void BSP_SD_DetectCallback | ( | void | ) |
SD detect IT detection callback.
- Return values:
-
None
Definition at line 219 of file stm3210e_eval_sd.c.
Referenced by BSP_SD_DetectIT().
void BSP_SD_DetectIT | ( | void | ) |
SD detect IT treatment.
- Return values:
-
None
Definition at line 208 of file stm3210e_eval_sd.c.
References BSP_SD_DetectCallback().
void BSP_SD_DMA_Rx_IRQHandler | ( | void | ) |
Handles SD DMA Rx transfer interrupt request.
- Return values:
-
None
Definition at line 373 of file stm3210e_eval_sd.c.
References uSdHandle.
void BSP_SD_DMA_Tx_IRQHandler | ( | void | ) |
Handles SD DMA Tx transfer interrupt request.
- Return values:
-
None
Definition at line 364 of file stm3210e_eval_sd.c.
References uSdHandle.
uint8_t BSP_SD_Erase | ( | uint64_t | StartAddr, |
uint64_t | EndAddr | ||
) |
Erases the specified memory area of the given SD card.
- Parameters:
-
StartAddr,: Start byte address EndAddr,: End byte address
- Return values:
-
SD status
Definition at line 339 of file stm3210e_eval_sd.c.
void BSP_SD_GetCardInfo | ( | HAL_SD_CardInfoTypedef * | CardInfo | ) |
Get SD information about specific SD card.
- Parameters:
-
CardInfo,: Pointer to HAL_SD_CardInfoTypedef structure
- Return values:
-
None
Definition at line 396 of file stm3210e_eval_sd.c.
References uSdHandle.
HAL_SD_TransferStateTypedef BSP_SD_GetStatus | ( | void | ) |
Gets the current SD card data status.
- Return values:
-
Data transfer state. This value can be one of the following values: - SD_TRANSFER_OK: No data transfer is acting
- SD_TRANSFER_BUSY: Data transfer is acting
- SD_TRANSFER_ERROR: Data transfer error
Definition at line 386 of file stm3210e_eval_sd.c.
References uSdHandle.
uint8_t BSP_SD_Init | ( | void | ) |
Initializes the SD card device.
- Return values:
-
SD status.
Definition at line 122 of file stm3210e_eval_sd.c.
References BSP_SD_IsDetected(), MSD_ERROR, MSD_OK, SD_MspInit(), SD_PRESENT, uSdCardInfo, and uSdHandle.
void BSP_SD_IRQHandler | ( | void | ) |
Handles SD card interrupt request.
- Return values:
-
None
Definition at line 355 of file stm3210e_eval_sd.c.
References uSdHandle.
uint8_t BSP_SD_IsDetected | ( | void | ) |
Detects if SD card is correctly plugged in the memory slot or not.
- Return values:
-
Returns if SD is detected or not
Definition at line 192 of file stm3210e_eval_sd.c.
References SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_NOT_PRESENT, and SD_PRESENT.
Referenced by BSP_SD_Init().
uint8_t BSP_SD_ITConfig | ( | void | ) |
Configures Interrupt mode for SD detection pin.
- Return values:
-
Returns 0
Definition at line 170 of file stm3210e_eval_sd.c.
References SD_DETECT_GPIO_PORT, SD_DETECT_IRQn, and SD_DETECT_PIN.
uint8_t BSP_SD_ReadBlocks | ( | uint32_t * | pData, |
uint64_t | ReadAddr, | ||
uint32_t | BlockSize, | ||
uint32_t | NumOfBlocks | ||
) |
Reads block(s) from a specified address in an SD card, in polling mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit ReadAddr,: Address from where data is to be read BlockSize,: SD card data block size, that should be 512 NumOfBlocks,: Number of SD blocks to read
- Return values:
-
SD status
Definition at line 235 of file stm3210e_eval_sd.c.
uint8_t BSP_SD_ReadBlocks_DMA | ( | uint32_t * | pData, |
uint64_t | ReadAddr, | ||
uint32_t | BlockSize, | ||
uint32_t | NumOfBlocks | ||
) |
Reads block(s) from a specified address in an SD card, in DMA mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit ReadAddr,: Address from where data is to be read BlockSize,: SD card data block size, that should be 512 NumOfBlocks,: Number of SD blocks to read
- Return values:
-
SD status
Definition at line 275 of file stm3210e_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_DATATIMEOUT, SD_DMAConfigRx(), and uSdHandle.
uint8_t BSP_SD_WriteBlocks | ( | uint32_t * | pData, |
uint64_t | WriteAddr, | ||
uint32_t | BlockSize, | ||
uint32_t | NumOfBlocks | ||
) |
Writes block(s) to a specified address in an SD card, in polling mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit WriteAddr,: Address from where data is to be written BlockSize,: SD card data block size, that should be 512 NumOfBlocks,: Number of SD blocks to write
- Return values:
-
SD status
Definition at line 255 of file stm3210e_eval_sd.c.
uint8_t BSP_SD_WriteBlocks_DMA | ( | uint32_t * | pData, |
uint64_t | WriteAddr, | ||
uint32_t | BlockSize, | ||
uint32_t | NumOfBlocks | ||
) |
Writes block(s) to a specified address in an SD card, in DMA mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit WriteAddr,: Address from where data is to be written BlockSize,: SD card data block size, that should be 512 NumOfBlocks,: Number of SD blocks to write
- Return values:
-
SD status
Definition at line 308 of file stm3210e_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_DATATIMEOUT, SD_DMAConfigTx(), and uSdHandle.
Generated on Thu Dec 10 2015 17:39:43 for STM3210E_EVAL BSP User Manual by
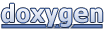