STM3210E_EVAL BSP User Manual
|
stm3210e_eval_audio.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_audio.c 00004 * @author MCD Application Team 00005 * @version V6.0.1 00006 * @date 18-December-2015 00007 * @brief This file provides the Audio driver for the STM3210E-Eval 00008 * board. 00009 @verbatim 00010 ============================================================================== 00011 ##### How to use this driver ##### 00012 ============================================================================== 00013 [..] 00014 (#) This driver supports STM32F103xE devices on STM3210E-Eval Kit: 00015 (++) to play an audio file (all functions names start by BSP_AUDIO_OUT_xxx) 00016 00017 [..] 00018 (#) PLAY A FILE: 00019 (++) Call the function BSP_AUDIO_OUT_Init( 00020 OutputDevice: physical output mode (OUTPUT_DEVICE_SPEAKER, 00021 OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_BOTH) 00022 Volume: initial volume to be set (0 is min (mute), 100 is max (100%) 00023 AudioFreq: Audio frequency in Hz (8000, 16000, 22500, 32000 ...) 00024 this parameter is relative to the audio file/stream type. 00025 ) 00026 This function configures all the hardware required for the audio application 00027 (codec, I2C, I2S, GPIOs, DMA and interrupt if needed). This function returns 0 00028 if configuration is OK. 00029 If the returned value is different from 0 or the function is stuck then the 00030 communication with the codec (try to un-plug the power or reset device in this case). 00031 (+++) OUTPUT_DEVICE_SPEAKER: only speaker will be set as output for the 00032 audio stream. 00033 (+++) OUTPUT_DEVICE_HEADPHONE: only headphones will be set as output for 00034 the audio stream. 00035 (+++) OUTPUT_DEVICE_BOTH: both Speaker and Headphone are used as outputs 00036 for the audio stream at the same time. 00037 (++) Call the function BSP_AUDIO_OUT_Play( 00038 pBuffer: pointer to the audio data file address 00039 Size: size of the buffer to be sent in Bytes 00040 ) 00041 to start playing (for the first time) from the audio file/stream. 00042 (++) Call the function BSP_AUDIO_OUT_Pause() to pause playing 00043 (++) Call the function BSP_AUDIO_OUT_Resume() to resume playing. 00044 Note. After calling BSP_AUDIO_OUT_Pause() function for pause, 00045 only BSP_AUDIO_OUT_Resume() should be called for resume 00046 (it is not allowed to call BSP_AUDIO_OUT_Play() in this case). 00047 Note. This function should be called only when the audio file is played 00048 or paused (not stopped). 00049 (++) For each mode, you may need to implement the relative callback functions 00050 into your code. 00051 The Callback functions are named BSP_AUDIO_OUT_XXXCallBack() and only 00052 their prototypes are declared in the stm3210e_eval_audio.h file. 00053 (refer to the example for more details on the callbacks implementations) 00054 (++) To Stop playing, to modify the volume level, the frequency or to mute, 00055 use the functions BSP_AUDIO_OUT_Stop(), BSP_AUDIO_OUT_SetVolume(), 00056 AUDIO_OUT_SetFrequency() BSP_AUDIO_OUT_SetOutputMode and BSP_AUDIO_OUT_SetMute(). 00057 (++) The driver API and the callback functions are at the end of the 00058 stm3210e_eval_audio.h file. 00059 00060 (++) This driver provide the High Audio Layer: consists of the function API 00061 exported in the stm3210e_eval_audio.h file (BSP_AUDIO_OUT_Init(), 00062 BSP_AUDIO_OUT_Play() ...) 00063 (++) This driver provide also the Media Access Layer (MAL): which consists 00064 of functions allowing to access the media containing/providing the 00065 audio file/stream. These functions are also included as local functions into 00066 the stm3210e_eval_audio.c file (I2SOUT_Init()...) 00067 00068 [..] 00069 ##### Known Limitations ##### 00070 ============================================================================== 00071 (#) When using the Speaker, if the audio file quality is not high enough, the 00072 speaker output may produce high and uncomfortable noise level. To avoid 00073 this issue, to use speaker output properly, try to increase audio file 00074 sampling rate (typically higher than 48KHz). 00075 This operation will lead to larger file size. 00076 00077 (#) Communication with the audio codec (through I2C) may be corrupted if it 00078 is interrupted by some user interrupt routines (in this case, interrupts 00079 could be disabled just before the start of communication then re-enabled 00080 when it is over). Note that this communication is only done at the 00081 configuration phase (BSP_AUDIO_OUT_Init() or BSP_AUDIO_OUT_Stop()) 00082 and when Volume control modification is performed (BSP_AUDIO_OUT_SetVolume() 00083 or BSP_AUDIO_OUT_SetMute()or BSP_AUDIO_OUT_SetOutputMode()). 00084 When the audio data is played, no communication is required with the audio codec. 00085 00086 (#) Parsing of audio file is not implemented (in order to determine audio file 00087 properties: Mono/Stereo, Data size, File size, Audio Frequency, Audio Data 00088 header size ...). The configuration is fixed for the given audio file. 00089 00090 (#) Mono audio streaming is not supported (in order to play mono audio streams, 00091 each data should be sent twice on the I2S or should be duplicated on the 00092 source buffer. Or convert the stream in stereo before playing). 00093 00094 (#) Supports only 16-bit audio data size. 00095 00096 @endverbatim 00097 ****************************************************************************** 00098 * @attention 00099 * 00100 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00101 * 00102 * Redistribution and use in source and binary forms, with or without modification, 00103 * are permitted provided that the following conditions are met: 00104 * 1. Redistributions of source code must retain the above copyright notice, 00105 * this list of conditions and the following disclaimer. 00106 * 2. Redistributions in binary form must reproduce the above copyright notice, 00107 * this list of conditions and the following disclaimer in the documentation 00108 * and/or other materials provided with the distribution. 00109 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00110 * may be used to endorse or promote products derived from this software 00111 * without specific prior written permission. 00112 * 00113 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00114 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00115 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00116 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00117 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00118 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00119 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00120 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00121 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00122 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00123 * 00124 ****************************************************************************** 00125 */ 00126 00127 /* Includes ------------------------------------------------------------------*/ 00128 #include "stm32f1xx_hal.h" /* Include HAL driver definitions to have access to I2S literals in audio driver */ 00129 #include "stm3210e_eval_audio.h" 00130 00131 /* Private variables ---------------------------------------------------------*/ 00132 00133 /** @addtogroup BSP 00134 * @{ 00135 */ 00136 00137 /** @addtogroup STM3210E_EVAL 00138 * @{ 00139 */ 00140 00141 /** @addtogroup STM3210E_EVAL_AUDIO 00142 * @brief This file includes the low layer audio driver available on STM3210E-Eval 00143 * eval board. 00144 * @{ 00145 */ 00146 00147 /** @defgroup STM3210E_EVAL_AUDIO_Private_Types AUDIO_Private_Types 00148 * @{ 00149 */ 00150 /** 00151 * @} 00152 */ 00153 00154 /** @defgroup STM3210E_EVAL_AUDIO_Private_Defines AUDIO_Private_Defines 00155 * @{ 00156 */ 00157 00158 /** 00159 * @} 00160 */ 00161 00162 /** @defgroup STM3210E_EVAL_AUDIO_Private_Macros AUDIO Private_Macros 00163 * @{ 00164 */ 00165 /** 00166 * @} 00167 */ 00168 00169 /** @defgroup STM3210E_EVAL_AUDIO_Private_Variables AUDIO_Private_Variables 00170 * @{ 00171 */ 00172 #define AUDIO_SAMPLE_SENDDUMMYDATA_SIZE 8 00173 00174 const uint16_t audio_sample_SendDummyData[] = 00175 {0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000}; 00176 00177 static __IO uint32_t SendDummyData = DISABLE; /* Variable to switch between sending dummy data or audio data on bus I2S */ 00178 00179 00180 /*### PLAY ###*/ 00181 static AUDIO_DrvTypeDef *pAudioDrv; 00182 I2S_HandleTypeDef hAudioOutI2s; 00183 00184 /** 00185 * @} 00186 */ 00187 00188 /** @defgroup STM3210E_EVAL_AUDIO_Private_Function_Prototypes AUDIO_Private_Function_Prototypes 00189 * @{ 00190 */ 00191 static void I2SOUT_MspInit(void); 00192 static void I2SOUT_Init(uint32_t AudioFreq); 00193 static uint8_t I2SOUT_SendDummyData_Start(uint16_t* pBuffer, uint32_t Size); 00194 static uint8_t I2SOUT_SendDummyData_Stop(void); 00195 00196 /** 00197 * @} 00198 */ 00199 00200 /** @defgroup STM3210E_EVAL_AUDIO_OUT_Exported_Functions AUDIO_OUT_Exported_Functions 00201 * @{ 00202 */ 00203 00204 /** 00205 * @brief Configure the audio peripherals. 00206 * @param OutputDevice: OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, 00207 * OUTPUT_DEVICE_BOTH. 00208 * @param Volume: Initial volume level (from 0 (Mute) to 100 (Max)) 00209 * @param AudioFreq: Audio frequency used to play the audio stream. 00210 * @retval 0 if correct communication, else wrong communication 00211 */ 00212 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) 00213 { 00214 uint8_t ret = AUDIO_ERROR; 00215 00216 /* I2S data transfer preparation: */ 00217 /* Prepare the Media to be used for the audio transfer from memory to I2S */ 00218 /* peripheral. */ 00219 /* Note: This function must be called before audio codec init because */ 00220 /* I2S initialisation parameter "MCLKOutput" determines whether the */ 00221 /* audio Codec is driven by I2S clock (I2S_MCLKOUTPUT_ENABLE) */ 00222 /* or by its own internal PLL (I2S_MCLKOUTPUT_DISABLE). */ 00223 /* Configure the I2S peripheral */ 00224 00225 /* Switch to send dummy data on bus I2S (switch used in I2SOUT_Init() */ 00226 SendDummyData = ENABLE; 00227 00228 I2SOUT_Init(AudioFreq); 00229 00230 /* Assign value to codec driver parameter I2S_MCLKOutput in function of */ 00231 /* I2S initialisation parameter "MCLKOutput". */ 00232 if (hAudioOutI2s.Init.MCLKOutput == I2S_MCLKOUTPUT_DISABLE) 00233 { 00234 ak4343_MCLKOutput(0); 00235 } 00236 else 00237 { 00238 ak4343_MCLKOutput(1); 00239 } 00240 00241 /* Initialize the audio driver structure */ 00242 pAudioDrv = &ak4343_drv; 00243 00244 /* Generate I2S master clock MCK */ 00245 /* (Start the audio player to send dummy data on I2S bus) */ 00246 if(I2SOUT_SendDummyData_Start((uint16_t *)audio_sample_SendDummyData, AUDIO_SAMPLE_SENDDUMMYDATA_SIZE) == 0) 00247 { 00248 if (pAudioDrv->Init(AUDIO_I2C_ADDRESS, OutputDevice, Volume, AudioFreq) == 0) 00249 { 00250 ret = AUDIO_OK; 00251 } 00252 else 00253 { 00254 ret = AUDIO_ERROR; 00255 } 00256 00257 I2SOUT_SendDummyData_Stop(); 00258 00259 /* Switch to send audio data on bus I2S */ 00260 SendDummyData = DISABLE; 00261 00262 I2SOUT_Init(AudioFreq); 00263 } 00264 else 00265 { 00266 ret = AUDIO_ERROR; 00267 } 00268 00269 00270 return ret; 00271 } 00272 00273 /** 00274 * @brief Starts playing audio stream from a data buffer for a determined size. 00275 * @param pBuffer: Pointer to the buffer 00276 * @param Size: Number of audio data BYTES. 00277 * @retval AUDIO_OK if correct communication, else wrong communication 00278 */ 00279 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size) 00280 { 00281 /* Call the audio Codec Play function */ 00282 if(pAudioDrv->Play(AUDIO_I2C_ADDRESS, pBuffer, Size) != 0) 00283 { 00284 return AUDIO_ERROR; 00285 } 00286 else 00287 { 00288 /* Update the Media layer and enable it for play */ 00289 HAL_I2S_Transmit_DMA(&hAudioOutI2s, pBuffer, DMA_MAX(Size)); 00290 00291 return AUDIO_OK; 00292 } 00293 } 00294 00295 /** 00296 * @brief Sends n-Bytes on the I2S interface. 00297 * @param pData: pointer on data address 00298 * @param Size: number of data to be written 00299 * @retval None 00300 */ 00301 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size) 00302 { 00303 HAL_I2S_Transmit_DMA(&hAudioOutI2s, pData, Size); 00304 } 00305 00306 /** 00307 * @brief This function Pauses the audio file stream. In case 00308 * of using DMA, the DMA Pause feature is used. 00309 * @note When calling BSP_AUDIO_OUT_Pause() function for pause, only 00310 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00311 * function for resume could lead to unexpected behavior). 00312 * @retval AUDIO_OK if correct communication, else wrong communication 00313 */ 00314 uint8_t BSP_AUDIO_OUT_Pause(void) 00315 { 00316 /* Call the Audio Codec Pause/Resume function */ 00317 if(pAudioDrv->Pause(AUDIO_I2C_ADDRESS) != 0) 00318 { 00319 return AUDIO_ERROR; 00320 } 00321 else 00322 { 00323 /* Call the Media layer pause function */ 00324 HAL_I2S_DMAPause(&hAudioOutI2s); 00325 00326 /* Return AUDIO_OK if all operations are OK */ 00327 return AUDIO_OK; 00328 } 00329 } 00330 00331 /** 00332 * @brief This function Resumes the audio file stream. 00333 * @note When calling BSP_AUDIO_OUT_Pause() function for pause, only 00334 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00335 * function for resume could lead to unexpected behavior). 00336 * @retval AUDIO_OK if correct communication, else wrong communication 00337 */ 00338 uint8_t BSP_AUDIO_OUT_Resume(void) 00339 { 00340 /* Call the Audio Codec Pause/Resume function */ 00341 if(pAudioDrv->Resume(AUDIO_I2C_ADDRESS) != 0) 00342 { 00343 return AUDIO_ERROR; 00344 } 00345 else 00346 { 00347 /* Call the Media layer resume function */ 00348 HAL_I2S_DMAResume(&hAudioOutI2s); 00349 /* Return AUDIO_OK if all operations are OK */ 00350 return AUDIO_OK; 00351 } 00352 } 00353 00354 /** 00355 * @brief Stops audio playing and Power down the Audio Codec. 00356 * @param Option: could be one of the following parameters 00357 * - CODEC_PDWN_HW: completely shut down the codec (physically). 00358 * Then need to reconfigure the Codec after power on. 00359 * @retval AUDIO_OK if correct communication, else wrong communication 00360 */ 00361 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option) 00362 { 00363 /* Call DMA Stop to disable DMA stream before stopping codec */ 00364 HAL_I2S_DMAStop(&hAudioOutI2s); 00365 00366 /* Call Audio Codec Stop function */ 00367 if(pAudioDrv->Stop(AUDIO_I2C_ADDRESS, Option) != 0) 00368 { 00369 return AUDIO_ERROR; 00370 } 00371 else 00372 { 00373 if(Option == CODEC_PDWN_HW) 00374 { 00375 /* Wait at least 100us */ 00376 HAL_Delay(1); 00377 00378 /* Power Down the codec */ 00379 HAL_GPIO_WritePin(AUDIO_RESET_GPIO, AUDIO_RESET_PIN, GPIO_PIN_RESET); 00380 00381 } 00382 /* Return AUDIO_OK when all operations are correctly done */ 00383 return AUDIO_OK; 00384 } 00385 } 00386 00387 /** 00388 * @brief Controls the current audio volume level. 00389 * @param Volume: Volume level to be set in percentage from 0% to 100% (0 for 00390 * Mute and 100 for Max volume level). 00391 * @retval AUDIO_OK if correct communication, else wrong communication 00392 */ 00393 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume) 00394 { 00395 /* Call the codec volume control function with converted volume value */ 00396 if(pAudioDrv->SetVolume(AUDIO_I2C_ADDRESS, Volume) != 0) 00397 { 00398 return AUDIO_ERROR; 00399 } 00400 else 00401 { 00402 /* Return AUDIO_OK when all operations are correctly done */ 00403 return AUDIO_OK; 00404 } 00405 } 00406 00407 /** 00408 * @brief Enables or disables the MUTE mode by software 00409 * @param Cmd: could be AUDIO_MUTE_ON to mute sound or AUDIO_MUTE_OFF to 00410 * unmute the codec and restore previous volume level. 00411 * @retval AUDIO_OK if correct communication, else wrong communication 00412 */ 00413 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd) 00414 { 00415 /* Call the Codec Mute function */ 00416 if(pAudioDrv->SetMute(AUDIO_I2C_ADDRESS, Cmd) != 0) 00417 { 00418 return AUDIO_ERROR; 00419 } 00420 else 00421 { 00422 /* Return AUDIO_OK when all operations are correctly done */ 00423 return AUDIO_OK; 00424 } 00425 } 00426 00427 /** 00428 * @brief Switch dynamically (while audio file is played) the output target 00429 * (speaker or headphone). 00430 * @param Output: specifies the audio output target: OUTPUT_DEVICE_SPEAKER, 00431 * OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_BOTH 00432 * @retval AUDIO_OK if correct communication, else wrong communication 00433 */ 00434 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output) 00435 { 00436 /* Call the Codec output Device function */ 00437 if(pAudioDrv->SetOutputMode(AUDIO_I2C_ADDRESS, Output) != 0) 00438 { 00439 return AUDIO_ERROR; 00440 } 00441 else 00442 { 00443 /* Return AUDIO_OK when all operations are correctly done */ 00444 return AUDIO_OK; 00445 } 00446 } 00447 00448 /** 00449 * @brief Update the audio frequency. 00450 * @param AudioFreq: Audio frequency used to play the audio stream. 00451 * @retval None 00452 * @note This API should be called after the BSP_AUDIO_OUT_Init() to adjust the 00453 * audio frequency. 00454 */ 00455 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq) 00456 { 00457 /* Update the I2S audio frequency configuration */ 00458 I2SOUT_Init(AudioFreq); 00459 } 00460 00461 /** 00462 * @brief Tx Transfer completed callbacks 00463 * @param hi2s: I2S handle 00464 * @retval None 00465 */ 00466 void HAL_I2S_TxCpltCallback(I2S_HandleTypeDef *hi2s) 00467 { 00468 if(hi2s->Instance == I2SOUT) 00469 { 00470 /* Configuration change depending on sending dummy data or audio data on bus I2S */ 00471 if (SendDummyData != ENABLE) 00472 { 00473 /* Call the user function which will manage directly transfer complete */ 00474 BSP_AUDIO_OUT_TransferComplete_CallBack(); 00475 } 00476 else 00477 { 00478 /* No action */ 00479 } 00480 } 00481 00482 } 00483 00484 /** 00485 * @brief Tx Transfer Half completed callbacks 00486 * @param hi2s: I2S handle 00487 * @retval None 00488 */ 00489 void HAL_I2S_TxHalfCpltCallback(I2S_HandleTypeDef *hi2s) 00490 { 00491 if(hi2s->Instance == I2SOUT) 00492 { 00493 /* Manage the remaining file size and new address offset: This function 00494 should be coded by user (its prototype is already declared in stm3210e_eval_audio.h) */ 00495 BSP_AUDIO_OUT_HalfTransfer_CallBack(); 00496 } 00497 } 00498 00499 /** 00500 * @brief I2S error callbacks 00501 * @param hi2s: I2S handle 00502 * @retval None 00503 */ 00504 void HAL_I2S_ErrorCallback(I2S_HandleTypeDef *hi2s) 00505 { 00506 /* Manage the error generated on DMA FIFO: This function 00507 should be coded by user (its prototype is already declared in stm3210e_eval_audio.h) */ 00508 if(hi2s->Instance == I2SOUT) 00509 { 00510 BSP_AUDIO_OUT_Error_CallBack(); 00511 } 00512 } 00513 00514 /** 00515 * @brief Manages the DMA full Transfer complete event. 00516 * @retval None 00517 */ 00518 __weak void BSP_AUDIO_OUT_TransferComplete_CallBack(void) 00519 { 00520 } 00521 00522 /** 00523 * @brief Manages the DMA Half Transfer complete event. 00524 * @retval None 00525 */ 00526 __weak void BSP_AUDIO_OUT_HalfTransfer_CallBack(void) 00527 { 00528 } 00529 00530 /** 00531 * @brief Manages the DMA FIFO error event. 00532 * @retval None 00533 */ 00534 __weak void BSP_AUDIO_OUT_Error_CallBack(void) 00535 { 00536 } 00537 00538 /****************************************************************************** 00539 Static Function 00540 *******************************************************************************/ 00541 00542 /** 00543 * @brief AUDIO OUT I2S MSP Init 00544 * @retval None 00545 */ 00546 static void I2SOUT_MspInit(void) 00547 { 00548 static DMA_HandleTypeDef hdma_i2stx; 00549 GPIO_InitTypeDef gpioinitstruct = {0}; 00550 I2S_HandleTypeDef *hi2s = &hAudioOutI2s; 00551 00552 /* Enable I2SOUT clock */ 00553 I2SOUT_CLK_ENABLE(); 00554 00555 /*** Configure the GPIOs ***/ 00556 /* Enable I2S GPIO clocks */ 00557 I2SOUT_SCK_SD_CLK_ENABLE(); 00558 I2SOUT_WS_CLK_ENABLE(); 00559 00560 /* I2SOUT pins configuration: WS, SCK and SD pins -----------------------------*/ 00561 gpioinitstruct.Pin = I2SOUT_SCK_PIN | I2SOUT_SD_PIN; 00562 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00563 gpioinitstruct.Pull = GPIO_NOPULL; 00564 gpioinitstruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 00565 HAL_GPIO_Init(I2SOUT_SCK_SD_GPIO_PORT, &gpioinitstruct); 00566 00567 gpioinitstruct.Pin = I2SOUT_WS_PIN ; 00568 HAL_GPIO_Init(I2SOUT_WS_GPIO_PORT, &gpioinitstruct); 00569 00570 /* I2SOUT pins configuration: MCK pin */ 00571 I2SOUT_MCK_CLK_ENABLE(); 00572 gpioinitstruct.Pin = I2SOUT_MCK_PIN; 00573 HAL_GPIO_Init(I2SOUT_MCK_GPIO_PORT, &gpioinitstruct); 00574 00575 /* Enable the I2S DMA clock */ 00576 I2SOUT_DMAx_CLK_ENABLE(); 00577 00578 if(hi2s->Instance == I2SOUT) 00579 { 00580 /* Configure the hdma_i2stx handle parameters */ 00581 hdma_i2stx.Init.Direction = DMA_MEMORY_TO_PERIPH; 00582 hdma_i2stx.Init.PeriphInc = DMA_PINC_DISABLE; 00583 hdma_i2stx.Init.MemInc = DMA_MINC_ENABLE; 00584 hdma_i2stx.Init.PeriphDataAlignment = I2SOUT_DMAx_PERIPH_DATA_SIZE; 00585 hdma_i2stx.Init.MemDataAlignment = I2SOUT_DMAx_MEM_DATA_SIZE; 00586 /* Configuration change depending on sending dummy data or audio data on bus I2S */ 00587 if (SendDummyData != ENABLE) 00588 { 00589 hdma_i2stx.Init.Mode = DMA_NORMAL; 00590 } 00591 else 00592 { 00593 hdma_i2stx.Init.Mode = DMA_CIRCULAR; 00594 } 00595 hdma_i2stx.Init.Priority = DMA_PRIORITY_HIGH; 00596 00597 hdma_i2stx.Instance = I2SOUT_DMAx_CHANNEL; 00598 00599 /* Associate the DMA handle */ 00600 __HAL_LINKDMA(hi2s, hdmatx, hdma_i2stx); 00601 00602 /* Deinitialize the Channel for new transfer */ 00603 HAL_DMA_DeInit(&hdma_i2stx); 00604 00605 /* Configure the DMA Channel */ 00606 HAL_DMA_Init(&hdma_i2stx); 00607 } 00608 00609 /* I2S DMA IRQ Channel configuration */ 00610 HAL_NVIC_SetPriority(I2SOUT_DMAx_IRQ, AUDIO_OUT_IRQ_PREPRIO, 0); 00611 HAL_NVIC_EnableIRQ(I2SOUT_DMAx_IRQ); 00612 } 00613 00614 /** 00615 * @brief Initializes the Audio Codec audio interface (I2S) 00616 * @param AudioFreq: Audio frequency to be configured for the I2S peripheral. 00617 */ 00618 static void I2SOUT_Init(uint32_t AudioFreq) 00619 { 00620 /* Initialize the hAudioOutI2s Instance parameter */ 00621 hAudioOutI2s.Instance = I2SOUT; 00622 00623 /* Disable I2S block */ 00624 __HAL_I2S_DISABLE(&hAudioOutI2s); 00625 00626 /* Perform MSP initialization at first function call */ 00627 if(HAL_I2S_GetState(&hAudioOutI2s) == HAL_I2S_STATE_RESET) 00628 { 00629 I2SOUT_MspInit(); 00630 } 00631 00632 /* I2SOUT peripheral configuration */ 00633 hAudioOutI2s.Init.AudioFreq = AudioFreq; 00634 hAudioOutI2s.Init.CPOL = I2S_CPOL_LOW; 00635 hAudioOutI2s.Init.DataFormat = I2S_DATAFORMAT_16B; 00636 hAudioOutI2s.Init.MCLKOutput = I2S_MCLKOUTPUT_ENABLE; 00637 hAudioOutI2s.Init.Mode = I2S_MODE_MASTER_TX; 00638 hAudioOutI2s.Init.Standard = I2S_STANDARD; 00639 00640 /* Initialize the I2S peripheral with the structure above */ 00641 HAL_I2S_Init(&hAudioOutI2s); 00642 00643 } 00644 00645 /** 00646 * @brief Starts sending dummy data as audio stream: function used to 00647 generate I2S master clock MCK for audio codec device. 00648 * @param pBuffer: Pointer to the buffer 00649 * @param Size: Number of audio data BYTES. 00650 * @retval AUDIO_OK if correct communication, else wrong communication 00651 */ 00652 static uint8_t I2SOUT_SendDummyData_Start(uint16_t* pBuffer, uint32_t Size) 00653 { 00654 /* Update the Media layer and enable it for play */ 00655 HAL_I2S_Transmit_DMA(&hAudioOutI2s, pBuffer, DMA_MAX(Size)); 00656 00657 return AUDIO_OK; 00658 00659 } 00660 00661 /** 00662 * @brief Stops sending dummy data as audio stream: function used to stop to 00663 generate I2S master clock MCK for audio codec device. 00664 * @retval AUDIO_OK if correct communication, else wrong communication 00665 */ 00666 static uint8_t I2SOUT_SendDummyData_Stop(void) 00667 { 00668 /* Call DMA Stop to disable DMA stream before stopping codec */ 00669 HAL_I2S_DMAStop(&hAudioOutI2s); 00670 00671 return AUDIO_OK; 00672 00673 } 00674 00675 00676 /** 00677 * @} 00678 */ 00679 00680 /** 00681 * @} 00682 */ 00683 00684 /** 00685 * @} 00686 */ 00687 00688 /** 00689 * @} 00690 */ 00691 00692 /** 00693 * @} 00694 */ 00695 00696 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 10 2015 17:39:43 for STM3210E_EVAL BSP User Manual by
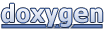